Create AI-powered tutorials effortlessly: Learn, teach, and share knowledge with our intuitive platform. (Get started for free)
7 Essential JavaScript Array Methods Every Beginner Should Master in 2024
7 Essential JavaScript Array Methods Every Beginner Should Master in 2024 - Map Method Transforming Temperature Data from Celsius to Fahrenheit
The `map` method in JavaScript offers a streamlined approach to converting Celsius temperatures to Fahrenheit. It enables us to generate a new array where each Celsius value from the original array is transformed into its Fahrenheit equivalent using the formula \( F = \frac{9}{5}C + 32 \). Let's say we have a list of Celsius temperatures like `[0, 10, 20, 30, 40]`; applying the `map` method with a function that applies this formula provides a clean and concise way to get the corresponding Fahrenheit values. Compared to a traditional `for` loop approach, `map` improves the readability and clarity of the code while aligning with a functional style of programming that's increasingly favored. Understanding and utilizing the `map` method, along with other key array methods, is essential for any aspiring JavaScript developer seeking to build robust and maintainable applications. While `for` loops have their place, in this and many other scenarios, `map` is the modern, preferred approach.
The formula to convert Celsius to Fahrenheit, \( F = C \times \frac{9}{5} + 32 \), reveals a non-linear relationship between these scales. It's somewhat unexpected considering how seemingly simple the Celsius scale appears.
While Celsius uses water's properties, setting 0° for freezing and 100° for boiling, Fahrenheit arbitrarily defines freezing at 32° and boiling at 212°. This different starting point can be a constant source of confusion when converting.
The `map` method allows us to efficiently transform large datasets of temperature readings into a different scale. It neatly demonstrates how functional programming can simplify repetitive operations and make code more understandable.
Practical scenarios often highlight the potential for misinterpretations due to differing scales. 35°C (95°F) can feel hot, while 0°C (32°F) might be experienced as cold. It reminds us that temperature perception relies on context.
Especially in scientific contexts, and globally, Celsius is usually preferred as the standard. However, Fahrenheit remains commonplace in the United States in everyday life. Thus, being fluent in both systems becomes important, particularly when working across cultures or fields.
Interestingly, at approximately -40 degrees, both Celsius and Fahrenheit converge, representing a single point where conversion is unnecessary.
Furthermore, using `map` isn't just about clarity, it can drastically speed up the conversion process, particularly when working with large amounts of data. This performance boost makes `map` more efficient than older methods like for loops.
The origin of temperature scales is quite fascinating. Fahrenheit, for instance, created his scale by using a brine solution as a reference, adding yet another layer of historical intrigue to the origins of this scale.
Crucially, the different sizes of the degrees in each scale greatly affect conversions. A change of 1°C is equal to 1.8°F, requiring careful calculations, especially when high precision is needed.
JavaScript and similar languages have array methods like `map`, `filter`, and `reduce` as core features. Mastering them is vital for any JavaScript programmer because these functions are so helpful in managing various kinds of data manipulations. Not just temperature conversion but numerous other scenarios.
7 Essential JavaScript Array Methods Every Beginner Should Master in 2024 - Filter Method Finding All Even Numbers in a Shopping Cart Total
The `filter` method in JavaScript empowers you to pick out specific elements from an array based on a set of conditions. Imagine you have a shopping cart total represented as an array of numbers. You might want to find all the even numbers within that total, perhaps for a promotional offer or analytical purpose. The `filter` method provides a straightforward way to achieve this. You'd give it a custom function (a callback) that checks if each number in the array is divisible by 2. The `filter` method then creates a new array containing only the even numbers that satisfy this condition. A key advantage of `filter` is that it doesn't alter the original array. This ensures that the original data remains untouched while simultaneously letting you efficiently manage and analyze specific parts of it. In 2024, mastering the `filter` method is a significant step towards becoming a more proficient JavaScript developer. Its ability to dissect and refine arrays opens up various possibilities for sophisticated data management within your applications.
### Filter Method Finding All Even Numbers in a Shopping Cart Total
The `filter` method in JavaScript is a powerful tool for creating a new array containing only elements that pass a specific test. This test is defined by a callback function that's applied to each element in the original array. The core idea is that `filter` returns a new array containing only the elements that satisfy the condition expressed in the callback. It doesn't modify the initial array; it's a non-destructive operation, making it ideal for keeping your data organized and predictable.
To pinpoint even numbers within an array, we can use `filter` with a callback function that checks if a number is evenly divisible by 2 (i.e., `number % 2 === 0`). This approach efficiently isolates the even numbers from a dataset.
The `filter` method's syntax is quite straightforward: `const filteredArray = array.filter(callbackFunction(element, index, array), thisArg)`. The `callbackFunction` takes three arguments: the current element, its index within the array, and the array itself. You can leverage this flexibility for more advanced filtering needs.
While simple, best practice emphasizes clarity in the callback functions used in `filter`. For instance, when selecting only even numbers, using a callback like `number => number % 2 === 0` communicates the intent of the code more effectively compared to overly terse, obscure alternatives.
The `filter` method offers a way to isolate specific subsets from a larger dataset. This makes it useful in situations where you need to isolate certain values for processing or display. Imagine a scenario where you're processing a shopping cart with various item prices, each represented as a number. The `filter` method could be used to select only items with even-numbered prices, perhaps for a promotional offer or to separate items into categories based on pricing patterns.
Let's look at an example:
```javascript
const numbers = [5, 2, 1, 0, 1, 3, 4, 7];
const evenNumbers = numbers.filter(number => number % 2 === 0);
console.log(evenNumbers); // Outputs: [2, 0, 4]
```
As part of the JavaScript array prototype, `filter` often works alongside other array methods like `map` and `reduce` in more complex data manipulations. This capability significantly increases its utility. It's worth noting that `filter` doesn't execute the callback on empty array elements, preventing irrelevant values from influencing the result.
It's also worth mentioning that `filter` is a performance-optimized approach compared to using traditional `for` loops. It's particularly valuable when processing large arrays, where performance becomes critical.
Beyond the obvious application of finding even numbers, understanding `filter` helps to approach more complex filtering scenarios in other parts of your code.
Browser compatibility is rarely a concern nowadays, as all mainstream browsers fully support `filter`. However, always ensure compatibility testing as part of your development process to catch any unusual behavior on less common or outdated browsers.
Ultimately, the `filter` method is a powerful and versatile tool in a JavaScript developer's arsenal, offering a clean and efficient way to isolate data subsets based on specific criteria. It's an essential method to master for any aspiring JavaScript developer, particularly when dealing with larger and more intricate data structures.
7 Essential JavaScript Array Methods Every Beginner Should Master in 2024 - Reduce Method Calculating Monthly Expenses from Daily Transactions
The `reduce` method in JavaScript is a valuable tool for accumulating data, especially when dealing with financial information like monthly expenses derived from daily transactions. Its core function is to condense an array into a single value. It achieves this by iterating through the array and applying a custom function (a callback) to each element, along with a so-called accumulator. This accumulator keeps track of the running total or, more generally, a value built up over the course of iterating through the array. The callback typically takes the current accumulator and the current array element and uses them to compute a new accumulator value. This continues until all elements in the array have been processed, at which point the final accumulated value becomes the result of the `reduce` method call. Unlike the `forEach` method, which is only used for side effects, `reduce` can produce a single final value, making it ideal for calculating totals or generating other accumulated results. For instance, imagine an array that stores daily transaction amounts. By using `reduce` and providing it a function that sums the accumulator and the current transaction, you can efficiently calculate your total monthly expenses. As a foundational method for data aggregation and manipulation, `reduce` is especially relevant for beginners seeking proficiency in JavaScript, particularly when working with financial applications or tasks involving data transformation. While traditional looping approaches can achieve similar results, they tend to be more verbose and less efficient. `reduce`, on the other hand, offers a cleaner and more concise way to perform these kinds of operations.
### Reduce Method: Calculating Monthly Expenses from Daily Transactions
The `reduce` method, introduced in ECMAScript 5, offers a compact way to transform an array into a single value. It's like distilling a bunch of daily expenses into one monthly total. We do this by providing a custom function to `reduce`, which, in turn, processes each item in the array. This custom function receives four arguments: an accumulator (which stores the running total), the current transaction being processed, its position in the array, and the entire array itself. It's like having a small, built-in state machine for each transformation.
While it excels at simple tasks like summing up numbers, `reduce`'s utility extends far beyond mere aggregation. For example, we can use it to categorize expenses, creating a more insightful picture of our spending patterns. It can even help us build complex data structures, allowing us to organize data into reports from flat lists.
It's important to note that `reduce` is a higher-order function, a term that initially might feel obscure. Simply put, it accepts another function as an argument. This ability to manipulate functions directly is a core tenet of functional programming, and the way that `reduce` uses a callback to maintain state throughout the iterations highlights its functional flavor.
Additionally, `reduce` offers the flexibility of setting an initial value, which can be important, especially if the array might be empty. If we don't, the first item in the array becomes the starting point for the reduction, which might not always be appropriate. Setting the starting point carefully can prevent surprises.
Like `map` and `filter`, `reduce` is optimized for performance, outperforming traditional `for` loops when the dataset is large. This makes it a valuable tool when we need to keep computational cost in mind. We can also combine it with other array methods to streamline complex data transformations. For example, we could filter out transactions from a specific vendor, then `reduce` the remaining values to find the total spent at that vendor over a month.
Keeping with the principles of functional programming, `reduce` is designed to avoid modifying the original array. It works on a copy, so the source data remains untouched. This is especially important when we're dealing with financial data, where accurate records are paramount.
Beyond the fundamental features, the callback function in `reduce` can access the index of each item and the full array itself. This can enable us to write complex conditional logic within the aggregation process, which might not be as straightforward with other iteration methods. Furthermore, `reduce` can produce a wide range of outputs. It isn't restricted to returning just numbers; it can create arrays, objects, or even more complex data structures based on the logic inside the callback function.
Finally, like most powerful tools, using `reduce` effectively requires awareness of its limitations. It can yield incorrect or unexpected results if we aren't careful about the initial value. Handling those cases properly is an important part of using `reduce` in production-quality code. Especially in applications where data reliability, like financial tools, is a critical aspect, these error-handling aspects should never be overlooked.
7 Essential JavaScript Array Methods Every Beginner Should Master in 2024 - Push Method Adding New User Profiles to a Social Media Feed
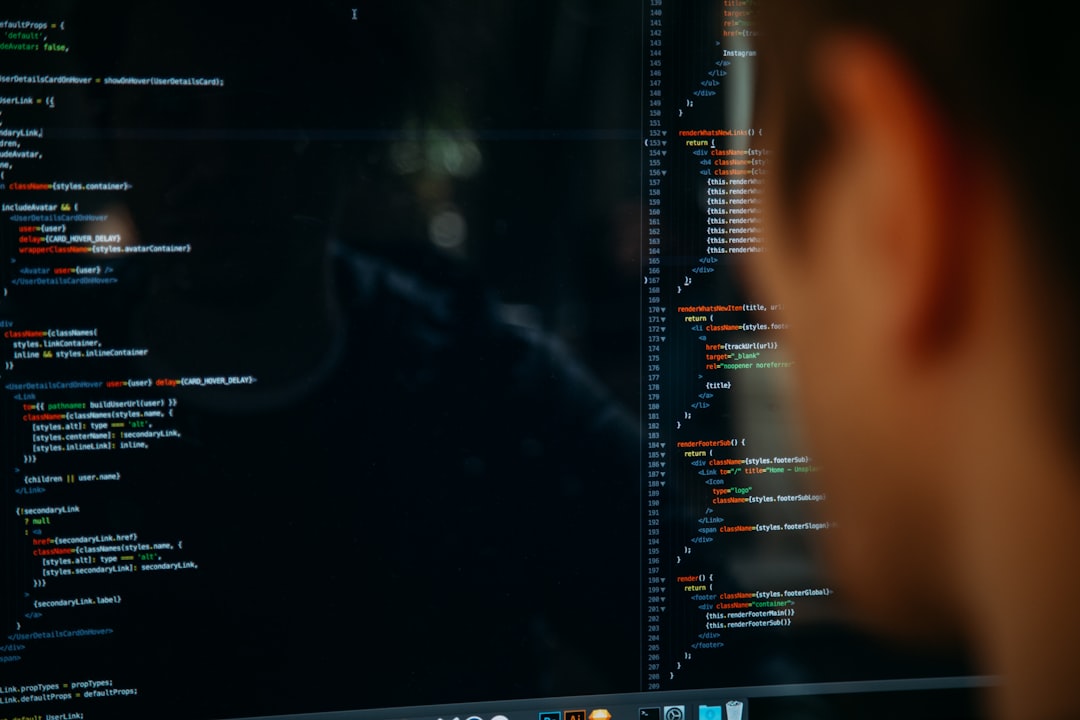
### Push Method: Adding New User Profiles to a Social Media Feed
The `push` method in JavaScript is a fundamental tool for building dynamic social media feeds. It allows you to effortlessly add one or more new user profiles to the end of an array, which could be the underlying structure of your social media feed. Using `arr.push(element1, element2, ...)` is a straightforward way to expand the array representing your feed as new users join. This creates a live, up-to-date feed reflecting the latest additions.
However, it's crucial to acknowledge that while `push` modifies the original array in place, it can sometimes create complications if you're aiming for a more functional style of code. The issue is that `push` doesn't return the updated array itself but instead returns the new length of the modified array. This difference in the returned value can lead to confusion when chaining method calls. Nonetheless, for beginners, understanding and using `push` is a critical step in mastering dynamic array manipulation. It’s a valuable method for handling changing lists and is frequently used in scenarios where you need to adapt to user actions quickly, making it vital for a beginner to understand for developing modern web applications. While seemingly simple, it's worth understanding the potential gotchas of how `push` modifies the original array and returns a length rather than a reference to the updated array.
The `push` method in JavaScript is a straightforward way to add one or more elements to the end of an array, returning the new length of the array. It's like extending a social media feed by adding new user profiles. This method modifies the original array, making it longer based on the number of elements added.
The syntax is simple: `arr.push(element1, element2, ..., elementN)`. We can add several user profiles with a single `push` call. However, chaining multiple `push` calls within a functional style of programming isn't very intuitive because it returns the new length of the array instead of a modified array itself.
It's a popular way to dynamically update a social media feed. As users sign up or are recommended, new profiles can be appended to the feed's underlying array using `push`. This simplifies feed management in web applications.
Other related methods include `pop` (for removing the last element), `unshift` (for adding to the beginning), and `shift` (for removing from the beginning). Understanding these methods is essential for beginners working with JavaScript arrays.
While generally useful, `push` can create challenges, especially when working with JSON's `stringify` method for sets. In these situations, the array might need to be converted to handle these structures properly.
However, in many scenarios, it helps keep code concise and readable, like when managing user interactions on social media or similar dynamic lists. It's generally considered a core part of JavaScript array operations, and beginners will find it helpful in many application contexts.
When working with `push` in a social media context, we can observe some interesting properties. It's optimized for adding elements efficiently, reducing the need for complex indexing routines. Developers can avoid many manual indexing mistakes when working with `push`. However, it's important to consider the implications for multi-user environments. When different parts of an app try to `push` simultaneously, race conditions can become a challenge.
Furthermore, since `push` modifies the original array directly, it can create side effects if not carefully managed. The good news is that JavaScript optimizes arrays for growth, meaning it might not always cause significant slowdowns when growing. Even so, it's vital to be mindful of potential memory leaks if the arrays continue growing without any elements being removed, leading to memory consumption problems.
The `push` method also blends well with other array methods, supporting more advanced functional-style programming. This flexibility improves the overall quality of your code, making it easier to read and maintain. In the realm of real-time applications, `push` can work in conjunction with events for updates to user profiles without needing a full refresh.
It's also worth noting that using `push` excessively in frontend frameworks can have implications for user interface responsiveness. Rapid changes to arrays might lead to a slowdown in user interface updates. This illustrates how choosing the right tools, even simple ones like `push`, is important for designing a responsive and engaging user experience.
In summary, while `push` is generally a helpful and often-used method, it requires careful consideration in certain situations, especially in large or complex applications. As you grow your understanding of Javascript, keep an eye out for such nuances in common methods.
7 Essential JavaScript Array Methods Every Beginner Should Master in 2024 - Pop Method Removing the Last Item from a Todo List
### Pop Method: Removing the Last Item from a Todo List
The `pop` method in JavaScript is a straightforward way to manage dynamic lists, like a to-do list, by removing the very last item. It directly modifies the original array, making it shorter and changing its contents. This makes it a suitable choice for managing data structures that follow the "last in, first out" (LIFO) principle, which is common in stack-like structures. It's easy to use: just `array.pop()`. The method also returns the item it removed, which can be useful in some situations. However, if the array is empty when you call `pop`, it will simply return `undefined`. This can cause issues in your code if you aren't prepared for this scenario. For anyone wanting to develop adaptable and responsive applications, understanding `pop` is crucial, especially when working with dynamically changing data.
### Pop Method: Removing the Last Item from a Todo List
The `pop` method in JavaScript is a straightforward way to remove the last item from an array and get that item back. This makes it efficient for handling scenarios like managing a todo list where you need to both remove the last task and potentially access that task's data.
When using `pop`, it's vital to be aware that it changes the array's length. This is important when working with loops or conditions based on the array's size. If you don't take this into account, it could lead to errors and unexpected outcomes.
Interestingly, `pop` works very well with stack data structures, where the last thing in is the first thing out (LIFO). This principle can be valuable in modeling behaviors like an undo button in a program.
While `pop` generally offers good performance, constantly resizing an array using it can affect how fast it runs. If the array grows a lot before elements are removed, the JavaScript engine might have to rearrange memory, which can create overhead.
One thing to keep in mind is that if you call `pop` on an empty array, it will return `undefined`. If you don't check for this situation and try to use the result, you might encounter subtle errors, potentially leading to operations based on undefined values.
From a functional programming perspective, `pop` stands out because it changes the original array. This differs from the idea of immutability that's common in functional programming. This can pose challenges when aiming for a more functional coding style, particularly when handling application state in frameworks like React.
You can use `pop` effectively in conditional logic. For example, a developer can check the current status of a todo list and decide whether to remove the last item based on certain conditions. This versatility makes `pop` useful for managing various states.
When working with asynchronous code, such as handling user actions in a web app, you might encounter timing issues when using `pop`. If multiple operations attempt to change the same array at the same time, you need to ensure the `pop` operation doesn't clash with other parts of your code.
`Pop` can also be beneficial in real-time scenarios. For instance, when handling dynamic todo lists, combining `pop` with event listeners allows for quick and responsive user interfaces. Updates are reflected instantly without having to redraw the entire component.
Finally, it's possible to combine `pop` with other array methods like `map` or `filter`. This can enhance the way you handle complex todo list interactions. For example, you could filter out certain tasks and then remove the last remaining task. Using methods together this way can make code easier to read and maintain.
7 Essential JavaScript Array Methods Every Beginner Should Master in 2024 - Splice Method Managing Playlist Songs by Adding and Removing Tracks
The `splice` method in JavaScript is a versatile tool for managing dynamic data, particularly useful for handling playlists where you need to add, remove, or replace songs. It directly alters the original array, making changes in place. You need to provide at least two arguments: the starting position (index) within the array and how many elements to remove from that point. Conveniently, you can also add new elements at that location by simply adding them after those first two arguments. This makes it quite flexible for managing playlists. While it changes the initial array, it does provide a return value: the array of elements that were removed. This is helpful for knowing exactly what's been taken out and could be used for things like undo functions. For new JavaScript developers, understanding `splice` is a core skill for any application that involves interacting with collections of data, especially where users might be directly changing the data, such as a playlist app or a to-do list application. Understanding how `splice` both changes the original array and gives you information about the changes is important.
### Splice Method: Managing Playlist Songs by Adding and Removing Tracks
The `splice` method in JavaScript is a powerful tool for manipulating arrays, offering a way to add, remove, or replace elements directly within the array itself. This makes it ideal for managing things like music playlists where you need to dynamically modify the order or contents of a list of songs. While seemingly simple, it's important to understand some of the subtleties of `splice` to use it effectively, particularly for beginners.
For instance, it's interesting to note that `splice` actually returns an array of the elements that were removed during the operation. This can be useful for keeping track of what's been taken out, allowing developers to potentially provide visual feedback to the user, or potentially to create undo functionality. One could imagine a music player application where deleted songs are held in a temporary list until a user explicitly chooses to permanently remove them, leveraging this `splice` output.
Furthermore, `splice` allows you to modify multiple elements at once, making it useful for batch operations. For instance, imagine needing to update the order of several songs within a playlist; `splice` can efficiently rearrange the sequence through a single operation, potentially optimizing performance in comparison to performing multiple individual replacements. While this is an attractive capability, it's important to bear in mind that, as with any method that directly alters an array, the original array is modified. This can create potential challenges if you're not careful, especially in scenarios where you need to preserve the original data. Sometimes using temporary arrays to hold the intermediate states of the playlist before it's altered is a good approach when working with `splice` to preserve the original for later use.
Another aspect of `splice` that initially can be confusing for beginners is the use of negative indices. You can use negative numbers to refer to elements from the end of the array, making it relatively straightforward to, for example, remove the last song in the playlist with `splice(-1,1)`. It's a convenient feature that shortcuts some of the calculations required in more traditional array operations.
While generally useful, `splice` is not without potential pitfalls. For instance, depending on the size of the array, repeated use of `splice` can potentially impact performance. The nature of array operations involves re-indexing when adding or removing elements, and this can incur a higher time complexity than O(1) operations. Thus, in situations where performance is critical, developers should be mindful of how frequently they use `splice`, especially with large playlists or other extensive datasets.
Furthermore, in line with general principles of functional programming, the fact that `splice` is a mutating operation—it directly changes the array—can cause issues in some programming environments or when maintaining state across different parts of an application. Reactive frameworks, like React, for example, often promote the idea of immutability to ensure that application state updates can be managed and tracked in a predictable and efficient way. This preference often discourages the use of mutating methods like `splice` unless developers specifically need it for a given purpose.
On the other hand, `splice` can be useful for building features such as "undo" functionality. When removing elements from the playlist, instead of discarding them completely, they could be kept in a temporary array. Using this mechanism, `splice` could be used to restore those elements to the main playlist at a later point. It offers a way to implement more sophisticated error recovery in application features that manipulate arrays.
Moreover, understanding the limits of `splice` is critical for avoiding potential errors. For example, if you attempt to remove more songs than are present in the playlist, JavaScript will handle the operation without error. However, if the code assumes the presence of a certain number of elements after `splice`, there can be unexpected behavior if the number of elements is smaller than expected.
Similarly, it's essential to exercise caution when utilizing `splice` within loops that iterate through the same array. Modifying the array's size while looping through it can result in unexpected behavior—skipped indexes or even infinite loops—if the code is not explicitly handling the dynamic changes to the length. This sort of situation emphasizes that a good grasp of the method's implications is needed, especially in complex applications where managing the integrity of data is important.
In conclusion, the `splice` method offers a powerful and flexible approach to managing playlists, allowing for efficient addition, removal, and modification of songs within an array. However, understanding its characteristics, like its mutating nature, its return value, and its potential performance implications, is essential for effective and error-free implementation. For beginners, practicing `splice` and understanding these aspects are essential in preparing for the nuances of JavaScript development, particularly in scenarios that require careful management of complex dynamic data.
7 Essential JavaScript Array Methods Every Beginner Should Master in 2024 - ForEach Method Sending Email Notifications to Inactive Users
The `forEach` method in JavaScript is a handy way to go through each item in an array and do something with it. This makes it a good fit for things like sending emails to users who haven't been active for a while. Because `forEach` doesn't create a new array, it's really useful when you just want to do something—like send an email—without needing to change the data. This means you can easily trigger actions directly on your user objects, making your code simpler and easier to read. `forEach` helps you avoid creating unnecessary variables to store data when your goal is simply to take action on existing data, making it a valuable tool for those just learning JavaScript. For beginners, understanding `forEach` and how it handles actions without changing the structure of your arrays is a valuable skill that can make your code more efficient. Being able to easily loop through things and perform actions on them is a core concept in programming, and `forEach` is a great way to achieve this.
Let's delve into the `forEach` method, specifically how it can be used for sending email notifications to inactive users. One of the things that caught my attention is that unlike `map` or `reduce`, `forEach` doesn't give you a new array back; it only runs a provided function on each array item. This means you need to be very careful because if you think it's going to return a value or a new structure, it probably won't, and it can lead to some surprising results.
Something else interesting about `forEach` is how it handles things in order. It goes through each array item one after the other, synchronously. If your function involves waiting for something to finish (like sending an email, which typically takes some time), the next item won't be processed until the first is done. In other words, if you're sending out lots of emails, it might slow things down because it does each one sequentially rather than sending them out in parallel.
Additionally, because we often use `forEach` for actions that change things outside the array, like sending emails or updating a database, it's easy for the purpose of the code to become unclear. I find that when code relies on side effects like that, it's a lot harder to test and make sure it does what it's supposed to do.
When working with a lot of data, `forEach` might not always be the best choice when it comes to performance. While it's generally simple to use, the processing overhead of each item might start to matter when dealing with a massive number of users who need notification. A traditional `for` loop might be more efficient in those kinds of scenarios.
Also, because `forEach` isn't designed to have a `try...catch` inside the callback function, handling errors can get messy. It means that if you want to deal with errors in a specific way, you have to put all your error-handling logic directly inside your loop, and that can make your code less readable, particularly when you want to quickly stop the loop upon encountering a critical issue.
There's also the question of context within the function that's passed to `forEach`. If you're not careful about how `this` is used inside that function, you can run into trouble, particularly if your function needs to reference something from the surrounding environment of the code.
Interestingly, `forEach` skips over empty elements in sparse arrays. So, if you're using this approach to target inactive users, and there are holes in your array, those users won't get their email, which is something you'd have to address through additional steps.
When you're trying to design logic that needs to skip over certain users based on conditions, `forEach` may not be the best choice. It doesn't let you use things like `break` or `continue` to control the flow. It simply runs the function for each item, without giving you the power to halt or skip to the next iteration easily.
Despite some of the challenges, you can still align `forEach` with more functional patterns. It can be paired with things like `filter` to select the right users before the loop starts, which can clean up the code and make it more focused on the specific operations needed for inactive users.
Although it's not ideally suited for data manipulation, I find `forEach` is incredibly valuable for monitoring and logging activities related to users. You could use it to record specific actions, such as when a user logs in or how long they've been idle, without the need to re-construct a data structure. It's quite convenient for tracking behavior over time and then triggering email notifications based on those logs without altering the original data set.
In summary, while it's a straightforward and useful method for simple actions like sending notifications, `forEach` has some hidden complexities and drawbacks. Understanding when it's appropriate and when other tools might be better choices is an important part of mastering JavaScript. In 2024, as we build increasingly complex web apps and need to handle large and potentially evolving data sets, keeping in mind these nuances will be crucial for building reliable and high-performing applications.
Create AI-powered tutorials effortlessly: Learn, teach, and share knowledge with our intuitive platform. (Get started for free)
More Posts from aitutorialmaker.com: