Create AI-powered tutorials effortlessly: Learn, teach, and share knowledge with our intuitive platform. (Get started for free)
7 Practical Strategies for Mastering Object-Oriented Programming in 2024
7 Practical Strategies for Mastering Object-Oriented Programming in 2024 - Embrace Design Patterns for Efficient Problem-Solving
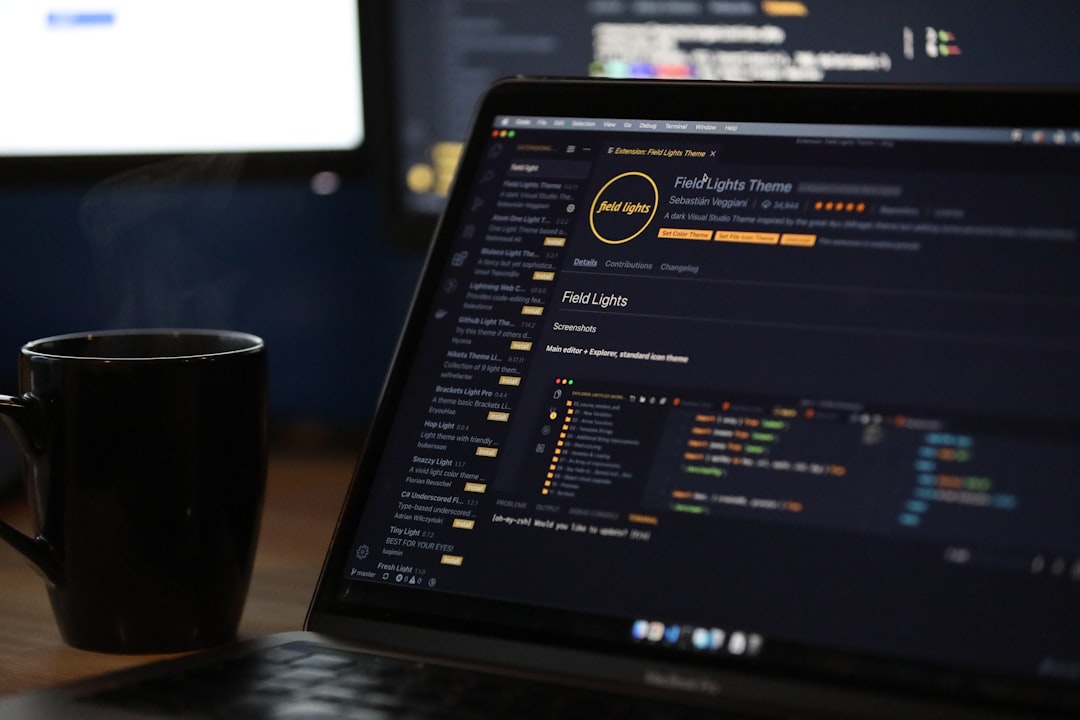
Design patterns are a set of proven solutions for recurring challenges in software design. They act like blueprints that guide you in building specific parts of your application, saving you time and effort. For instance, the Singleton Pattern ensures that you have only one instance of a class throughout your program, which is useful when dealing with resources like a cache or a thread pool. These patterns can be seen as a kind of shared language among programmers, enabling a more consistent approach to problem-solving. This shared understanding makes it easier for developers to collaborate, understand each other's code, and maintain projects over time.
Design patterns are essentially blueprints for common solutions to recurring problems in software design. Think of them as the "Gang of Four" (GoF)'s take on tried-and-true coding strategies, a collection of over 20 patterns, each tackling a different design challenge. The GoF's approach promotes effective communication among developers and reduces misinterpretations by providing a standardized vocabulary for discussing design solutions.
One example is the Singleton pattern, which ensures a class has only one instance, granting a single access point for that instance. This can be crucial for resource management but can complicate testing due to its hidden dependencies.
While the use of design patterns can drastically cut development time – studies suggest up to a 15% reduction – it's essential to remember that not every pattern is universally applicable. Overusing patterns in simple scenarios might lead to unnecessary complexity.
The Observer pattern, for instance, offers a push-based model where changes in one object ("subject") automatically notify all dependent objects ("observers"). This dynamic and flexible communication system excels in GUI applications but may not be necessary for simpler scenarios.
Another notable pattern is the Strategy pattern, which encapsulates a method allowing its swapping at runtime. This allows developers to modify the behavior of their code without altering the core code itself, adhering to the Open/Closed Principle.
While the Factory pattern primarily involves object creation, it also encapsulates the logic for deciding which objects to create, promoting loose coupling and high cohesion within applications.
The Adapter pattern bridges the gap between incompatible interfaces, seamlessly integrating existing code without major modifications. This pattern shines when extending the capabilities of legacy systems.
However, research suggests that misusing design patterns can lead to over-engineering. Implementing complex solutions for simple problems can hinder maintainability and readability of code.
Patterns like Command, for instance, encapsulate requests as objects, facilitating undo functionality without altering application infrastructure. This proves advantageous in user-centric software. It's crucial to strike a balance: employing patterns for their intended purpose, ensuring they simplify rather than complicate the codebase.
7 Practical Strategies for Mastering Object-Oriented Programming in 2024 - Implement Dependency Injection to Enhance Modularity
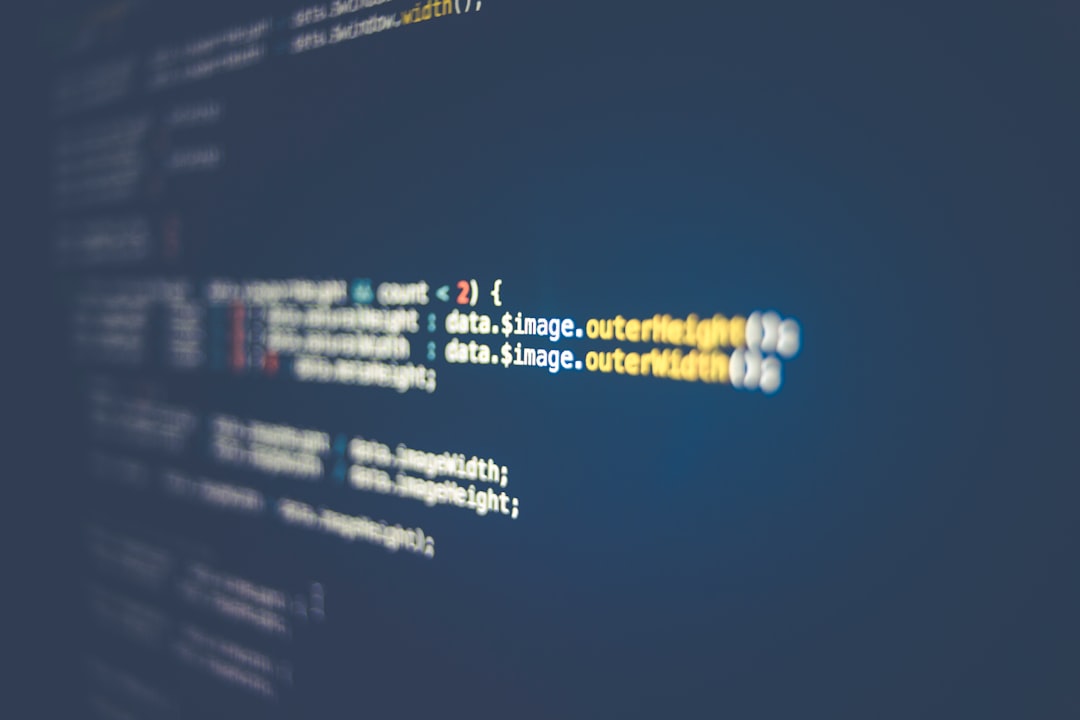
Dependency Injection (DI) is a cornerstone of modularity in object-oriented programming. It decouples classes by shifting dependencies outside of them, leading to more adaptable and maintainable code. This allows for easy swapping of implementations, like different HTTP clients, without touching the core logic. It makes testing simpler and improves code organization. However, managing the complexity introduced by DI, particularly dependency lifecycles, is essential to avoid potential pitfalls in maintainability. For developers aiming to master advanced OOP and build scalable applications, understanding and implementing DI is a crucial step.
Dependency Injection (DI) is a design pattern that has been gaining momentum in the software development world, especially within the realm of Object-Oriented Programming (OOP). Its core principle involves separating a class from its dependencies, making them externally injectable. This decoupling, while offering significant benefits, also poses certain challenges.
One of the key advantages of DI is its contribution to the separation of concerns, a foundational principle in software design. By decoupling a class from its dependencies, developers can isolate and modify components without impacting the entire application. This isolation makes code significantly easier to understand and maintain.
Another noteworthy benefit of DI lies in its impact on testing. By injecting mock objects, developers gain the ability to create controlled and predictable test environments, streamlining the testing process. Research suggests that DI can reduce testing time by 30-50%. However, this effectiveness hinges on the proper implementation of DI.
However, like any powerful tool, DI comes with potential drawbacks. One such concern is performance overhead. The indirection introduced by dependency resolution can lead to slower application startup times and increased memory usage, especially if not managed carefully. This can be particularly pronounced in the case of DI frameworks, which may further exacerbate the situation.
DI also embodies the concept of Inversion of Control (IoC). This inversion refers to the control flow within the application. Traditionally, a class would create its own dependencies, but in DI, these dependencies are injected from outside. This approach fosters a more flexible and adaptable architecture.
Applications built with DI often demonstrate improved scalability. This scalability stems from the modular structure that DI promotes, enabling developers to expand functionalities without disrupting existing code. This is particularly advantageous in fast-paced development environments where rapid iterations are essential.
Despite its advantages, DI also introduces complexities, particularly in configuration. Developers need to carefully define bindings and scopes for dependencies, which can sometimes lead to confusion and monitoring challenges. This potential for complexity requires a diligent approach to managing these aspects.
While DI is a powerful tool, excessive reliance on DI frameworks can introduce a degree of coupling between your application and the framework itself. This coupling can complicate future transitions or refactoring efforts, making it crucial to understand the implications of framework choices.
One of the often overlooked benefits of DI is its positive impact on code readability. The explicit definition of dependencies improves the overall clarity of the code, making it easier to understand the flow of data and dependencies within the application. This improved readability fosters better team collaboration and smoother onboarding processes for new developers.
While DI offers numerous benefits, it is important to acknowledge the potential trade-off between performance and flexibility. In scenarios where performance is paramount, directly managing dependencies might be more beneficial. Applications that prioritize flexibility can reap the rewards of DI.
DI seamlessly complements several design patterns like Factory and Strategy, further strengthening modularity. This synergy results in robust, adaptable software systems.
In conclusion, DI offers a compelling blend of benefits and challenges. It promotes modularity, facilitates testing, enhances scalability, and improves code readability. However, developers need to be mindful of the potential performance implications and the added complexity associated with configuring and managing dependencies. As with any tool, understanding its strengths and limitations is key to using DI effectively and leveraging its advantages while minimizing its potential drawbacks.
7 Practical Strategies for Mastering Object-Oriented Programming in 2024 - Master Advanced JavaScript Techniques for OOP
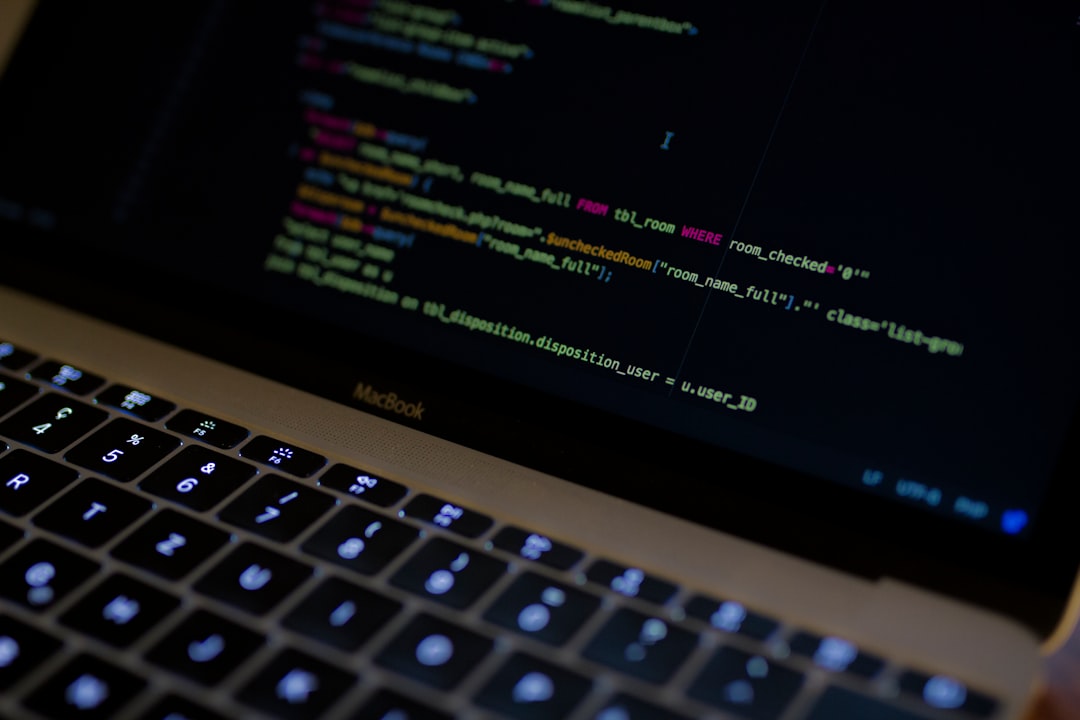
Mastering advanced JavaScript techniques for OOP is essential for building well-structured, maintainable, and scalable applications. While ES6 has made it easier to define classes, it's crucial to go beyond the basics and delve into the nuanced world of prototypal inheritance. Understanding how JavaScript objects inherit properties and methods from their prototypes is vital for achieving efficient object-oriented programming. This includes comprehending the impact of the `this` keyword, a concept that can be tricky for beginners. In the context of JavaScript's flexible and dynamic nature, mastering closures and their role in managing scope and data encapsulation is also paramount.
As JavaScript evolves, the demand for asynchronous programming increases. This is where understanding promises and the async/await syntax becomes critical. These techniques are essential for handling asynchronous operations, such as network requests, in a way that doesn't block the execution of the main thread, ensuring a smoother user experience.
Modern software development often involves data management, and good programming practices involve using getters and setters for object properties. These techniques provide a controlled mechanism for accessing and manipulating data within objects, leading to more robust and predictable applications. By embracing these advanced JavaScript techniques, developers can craft efficient, scalable, and maintainable code that meets the challenges of modern software development.
Mastering advanced JavaScript techniques for object-oriented programming (OOP) involves delving into the language's unique features, which sometimes differ from traditional OOP languages. The way JavaScript handles inheritance through prototypes, rather than the traditional class-based approach, creates flexibility and dynamism but requires understanding.
Function constructors in JavaScript serve as a blueprint for creating objects. When combined with the `new` keyword, they leverage the power of prototypes to enable inheritance. This allows for the creation of complex object models, supporting encapsulation and abstract data types, all within the framework of JavaScript's dynamic nature.
The concept of "first-class functions" in JavaScript opens a world of possibilities. By treating functions like objects, developers can pass them as arguments, return them from other functions, and assign them to variables. This enhances code modularity and reusability, allowing for more flexible implementation of OOP principles.
JavaScript also embraces closures, which are essential for achieving data privacy. By creating private variables within functions, developers can control access to data and hide implementation details. This adheres to the encapsulation principle, which is central to robust object-oriented designs.
Another advanced feature of JavaScript is mixins. These allow sharing properties and behaviors between multiple objects without requiring a strict parent-child relationship. Mixins provide an alternative to traditional inheritance, enabling code reuse without the constraints of class-based structures.
The metaprogramming capabilities in JavaScript are worth exploring. Features like proxies and the Reflect APIs allow developers to create layers of behavior around objects. These mechanisms are particularly useful for implementing complex patterns like the Proxy pattern. This allows for intercepting and modifying operations on objects, creating a dynamic environment for advanced OOP techniques.
JavaScript's approach to asynchronous programming offers opportunities for integrating OOP principles within asynchronous code. Features like Promises and async/await are particularly valuable for managing state and flow in asynchronous environments. They provide a way to gracefully handle asynchronous code without sacrificing object-oriented principles.
Furthermore, JavaScript's inherent event-driven nature complements OOP principles. This enables the creation of loosely-coupled systems that communicate through events. The Observer design pattern aligns seamlessly with this paradigm, enhancing modularity and scalability.
JavaScript also allows for dynamic object modification. Developers can modify object structures on-the-fly using methods like `Object.assign()` and dynamic property assignment. This flexibility introduces opportunities and challenges for those utilizing OOP paradigms, requiring thoughtful application and careful consideration of potential side effects.
Although JavaScript's roots lie in prototype-based inheritance, the introduction of the `class` syntax in ECMAScript 2015 provided developers with a more familiar syntax from traditional OOP languages. While seemingly similar to classes in other OOP languages, it still fundamentally maps back to prototypal inheritance. The `class` syntax essentially provides an alternative way to achieve the same outcomes while remaining consistent with the underlying model. This syntax serves as a powerful tool for developers, offering a familiar structure while honoring the unique nature of JavaScript.
The journey to mastery in JavaScript OOP requires an understanding of the language's unique approach to fundamental OOP principles. It's important to explore these features, understand how they diverge from traditional OOP languages, and leverage the benefits they offer.
7 Practical Strategies for Mastering Object-Oriented Programming in 2024 - Practice Inheritance and Polymorphism Across Languages
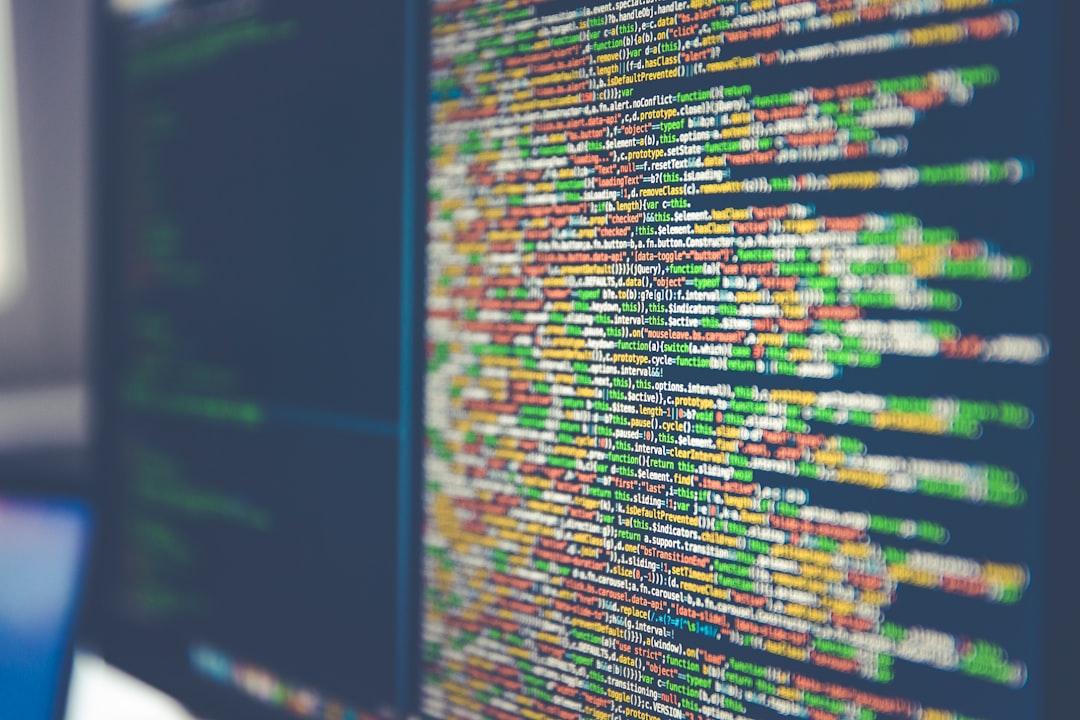
In object-oriented programming (OOP), two essential concepts are inheritance and polymorphism. Inheritance lets a new class inherit features from existing ones, promoting code reuse and creating a hierarchical structure. Polymorphism allows methods to behave differently based on the object calling them, adding flexibility. However, every programming language has its own approach to implementing these concepts, so practicing them across languages is crucial for a deeper understanding. By practicing in various languages, you discover both the core principles and the language-specific ways they're implemented. This practice is crucial for understanding how inheritance and polymorphism work fundamentally. It also prepares you to handle coding challenges that involve multiple programming languages.
Inheritance and polymorphism are cornerstones of Object-Oriented Programming (OOP), but how they are implemented across different languages can be surprisingly diverse. Languages like Java employ class-based inheritance, whereas JavaScript takes a more flexible approach with prototypal inheritance. This highlights how OOP concepts can be adapted to suit distinct programming paradigms.
Let's delve into some curious differences. Languages like Java and C# restrict inheritance to a single parent class, potentially leading to the "diamond problem" if multiple classes define the same method. Languages like C++ tackle this with multiple inheritance, but it comes with its own complexities. Polymorphism itself also manifests in varied forms. Compile-time polymorphism (method overloading) and run-time polymorphism (method overriding) offer distinct capabilities, with languages like C++ supporting both, while Java leans more towards run-time polymorphism.
The interplay between static and dynamic typing adds another layer of intrigue. Statically typed languages like Java define variable types at compile time, whereas dynamically typed languages like Python do so at runtime. This fundamental difference influences how polymorphic behavior is realized, often giving dynamic languages a more flexible edge.
In languages like Python, the Method Resolution Order (MRO) becomes critical, dictating how methods are resolved in complex inheritance hierarchies. Python employs the C3 linearization algorithm, ensuring a predictable and consistent method resolution process, contrasting with other languages' simpler models.
Abstract classes and interfaces are also interpreted differently. Java distinguishes between these, promoting a clear separation of implementation and behavior. Languages with structural typing like Go prioritize interface satisfaction over explicit declarations, offering a more flexible approach.
Functional languages like Scala embrace first-class functions, which are treated as objects, enabling a unique form of polymorphism that goes beyond inheritance. Metaclasses in languages like Python allow developers to alter class creation dynamically, enabling advanced OOP patterns that can modify inheritance and polymorphism on the fly, a level of dynamism often missing in statically typed languages.
Rust, with its emphasis on trait-based polymorphism, moves away from classical inheritance models, allowing developers to define shared behaviors without needing explicit parent-child relationships. This shift from inheritance to composition offers a new perspective.
The choice between inheritance and composition has a direct impact on performance. While inheritance promotes reuse, deep inheritance hierarchies can slow down method resolution times, prompting developers to consider composition as a more efficient alternative when necessary.
The practice of inheritance and polymorphism across various languages reveals a rich tapestry of techniques and philosophies, revealing the dynamic and adaptable nature of OOP itself.
7 Practical Strategies for Mastering Object-Oriented Programming in 2024 - Develop Hands-On Projects to Reinforce Core Concepts
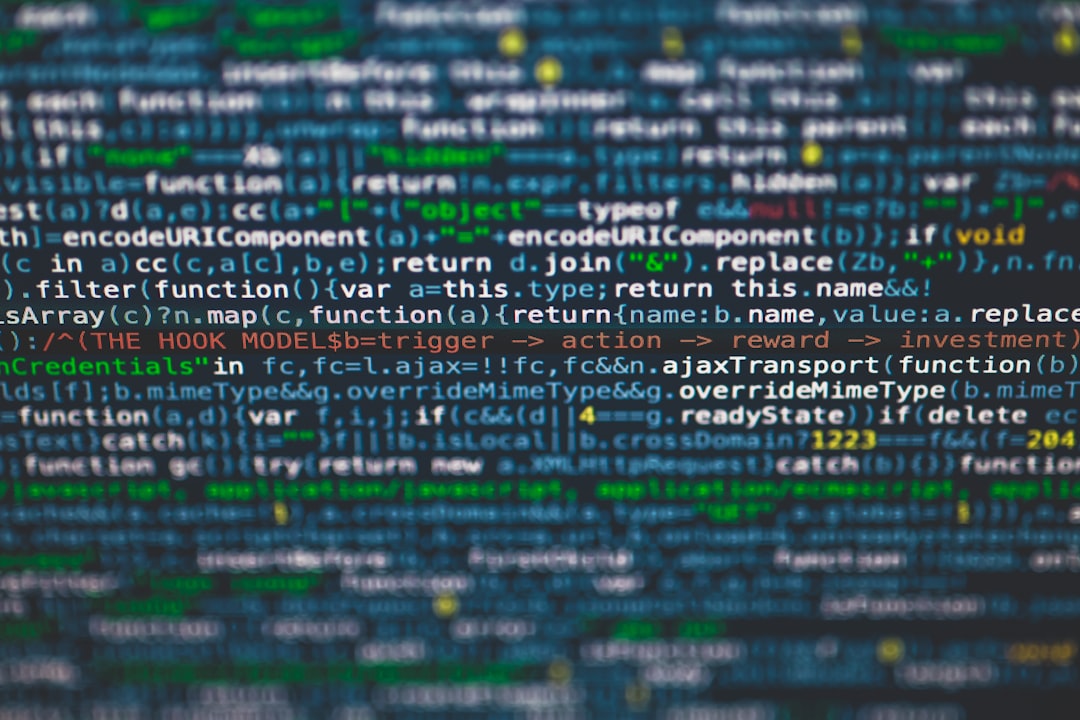
Building hands-on projects is a vital way to get a good grasp of object-oriented programming (OOP) in 2024. Trying out practical exercises that solve real-world issues can really boost your understanding of core OOP concepts like classes, inheritance, encapsulation, and polymorphism. It's a good idea to start with small projects, where you can build your skills before moving on to bigger, more challenging applications. Besides helping you remember what you learn, these projects also help you develop solid programming skills as you learn by doing and figuring things out. Working together on projects, reviewing each other's code, and talking about what you've learned can also make a big difference in your learning process, as it helps you see OOP from different angles.
Developing hands-on projects is a powerful way to reinforce core OOP concepts. It's like taking the theory you've learned and putting it into action, building real things instead of just memorizing definitions.
There are a bunch of reasons why this approach works so well. Firstly, practical projects help you retain information much better. When you're actively building something, you have to constantly call upon the principles you've learned. It's like your brain is making a bunch of connections to make sure you understand the concepts you're using.
This process also helps you learn from your mistakes. When you're coding, you're bound to make mistakes, but those mistakes are like opportunities to learn. It’s like figuring out the puzzle in a way you didn’t think about before, leading to a deeper understanding.
Furthermore, projects make theory come to life. You can start to see how abstract concepts like inheritance and polymorphism actually work in the real world. It’s like watching a theory movie become a real-life experience, making it much easier to understand.
One of the most valuable parts of doing projects is that you get to work with others. This is important for learning how to communicate your ideas clearly, and how to work effectively as part of a team. You can learn from how other people approach problems and share your own insights, expanding your perspective on how things work.
Projects also provide an immediate feedback loop. You get instant results and can quickly see if you're on the right track. This means you learn and adapt much faster, making your journey to becoming an OOP master more efficient.
Lastly, doing projects can help you stay motivated. It’s like working on a fun puzzle you get to keep building on, and it can really encourage you to keep learning and improving. This feeling of ownership is what can really make a difference in your progress.
Beyond OOP knowledge, practical projects can build up other useful skills, like problem-solving and critical thinking. These are valuable skills that you can use in any tech field. It's like a hidden bonus to your OOP journey, making you more well-rounded as a developer.
If you want to become a true OOP master, hands-on experience is absolutely essential. It's like mastering a musical instrument; the more you play, the better you'll get. So, start building some projects, and watch your OOP skills soar!
7 Practical Strategies for Mastering Object-Oriented Programming in 2024 - Explore Modern OOP Features in Popular Frameworks
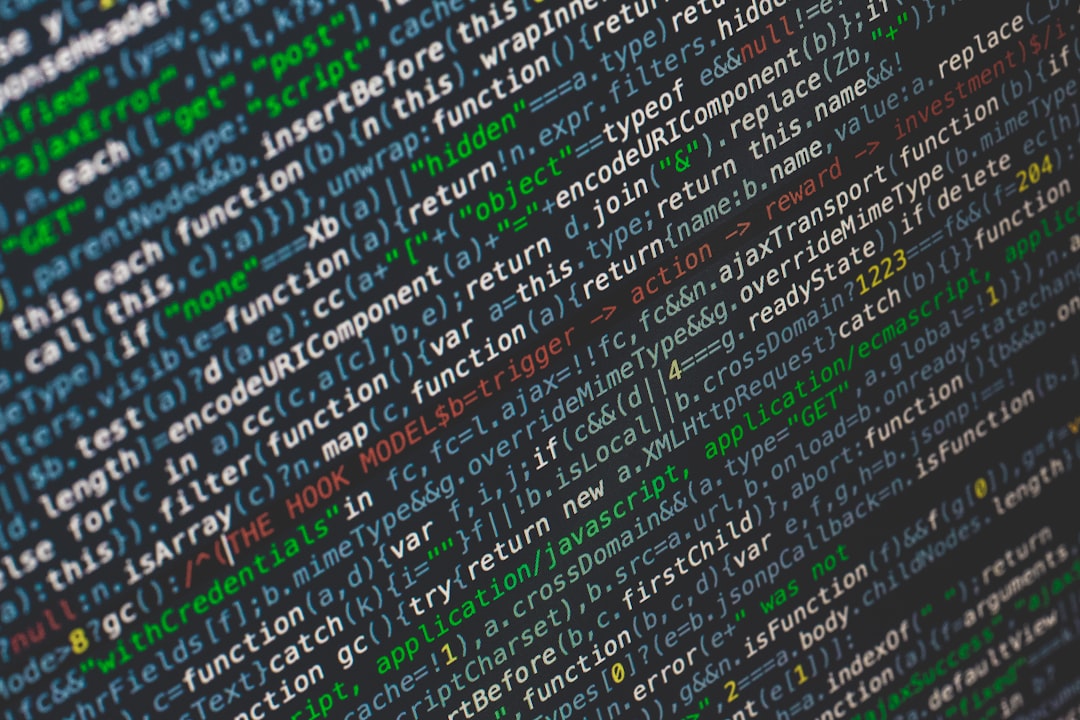
Exploring modern OOP features within popular frameworks involves recognizing that many languages and frameworks have incorporated advanced OOP principles to solve new challenges. For example, TypeScript, an addition to the JavaScript world, offers enhanced type-checking and structural typing for a more refined OOP experience. Similarly, frameworks like Spring in Java utilize Dependency Injection to encourage modular coding, improving testability and reducing rigid connections between components. While these improvements are valuable, they introduce their own set of complexities. Developers must be mindful of performance implications and the risk of over-engineering. Ultimately, mastering these modern features enables programmers to create applications that are more efficient, maintainable, and scalable across diverse development environments.
The modern world of Object-Oriented Programming (OOP) is far from static. Frameworks constantly push the boundaries of traditional OOP, leading to surprising twists and turns in how we design and build software. It's a bit like discovering hidden treasures in the attic of OOP – these unexpected features often offer solutions we didn't even know we needed.
Take, for instance, dynamic class creation. Imagine building a program where classes are created on the fly, based on user input or changing data. This isn't just theoretical. Frameworks in Ruby and JavaScript make this a reality, granting unprecedented flexibility. It's a bit like a programming magician pulling new classes out of a hat, adapting to unpredictable demands with ease.
But the fun doesn't stop there. Mixins, a feature favored by frameworks like Ruby on Rails, let classes share methods and properties without rigid hierarchies. It's like a casual party where classes drop by and pick up useful skills without needing to be formally related. It's a more relaxed approach, making code reuse simpler and less restrictive.
Method chaining is another unexpected twist. In frameworks like Angular and Vue, multiple method calls can be strung together in a single statement. This enhances readability, creating cleaner code that almost flows like a conversation rather than a rigid command list.
The role of interfaces is also evolving. Frameworks like TypeScript and PHP use them as contracts, ensuring classes adhere to specific functionalities, but without dictating the exact implementation. It's like outlining the duties of a job without specifying the exact steps to fulfill those duties. This promotes modularity and facilitates testing with more certainty.
Asynchronous object behavior, often found in modern frameworks, allows objects to work in the background without slowing down the main program. This is like having assistants handling tasks while you continue focusing on the primary project. This approach is critical for responsive web applications, handling numerous simultaneous requests with grace.
The Factory Pattern has gotten an upgrade. Frameworks like Angular and Spring don't just create objects, they manage their entire lifecycle. It's like having a concierge managing object creation, dependency resolution, and configuration, ensuring everything is in place.
JavaScript, the ever-evolving language, takes a different approach to inheritance with its prototype chain. This dynamic method allows objects to inherit from other objects directly, leading to highly flexible and adaptable systems. It's like a family tree where branches can connect to any other branch, defying conventional structures.
Encapsulation itself is being revisited. Frameworks now allow developers to introduce private fields and protected methods in JavaScript, enhancing privacy control within objects. This refined approach brings fresh air to a traditional OOP principle.
Static factory methods have become the new cool kids on the block. These methods are often used instead of constructors for creating objects, offering cleaner syntax and more abstract control over instance creation. This pattern often allows for flexible object creation, providing a more nuanced approach to building instances.
The rise of immutability, particularly in frameworks favoring functional programming, is another interesting trend. Libraries like Redux in JavaScript use immutable data structures to enhance performance and predictability, offering cleaner state management in complex applications.
These evolving features within OOP are a reminder that the world of software development is constantly adapting. Frameworks push boundaries, and as curious researchers and engineers, we must keep exploring, embracing, and applying these new advancements to build the next generation of software.
Create AI-powered tutorials effortlessly: Learn, teach, and share knowledge with our intuitive platform. (Get started for free)
More Posts from aitutorialmaker.com: