Create AI-powered tutorials effortlessly: Learn, teach, and share knowledge with our intuitive platform. (Get started for free)
A Complete Guide to Reading Binary Files in C++ Using ifstreamread()
A Complete Guide to Reading Binary Files in C++ Using ifstreamread() - Opening Binary Files With ifstream And Setting The Binary Mode Flag
When working with binary files in C++ using `ifstream`, it's vital to explicitly specify the binary mode. This is achieved by using the `ios::binary` flag during the file opening process with the `open()` method. This step is essential as it prevents any automatic transformations that are often applied to text files, such as newline character conversions. These transformations can corrupt the raw binary data. While `ifstream` can handle both binary and text data, neglecting `ios::binary` when dealing with binary files can lead to unpredictable data corruption and incorrect interpretations.
Moreover, remember that once an `ifstream` object has been successfully linked to a file, attempting to open it again with a subsequent call to `open()` will fail. This means proper management of file objects and their state is crucial to avoid unintended behavior. Using the binary mode flag and following appropriate file handling practices helps in improving the clarity of your code and makes it more maintainable, especially when working collaboratively.
When working with `ifstream` in C++, it's important to remember that the default mode is text mode. This means that the stream might apply transformations to the data, such as interpreting newline characters differently or treating specific sequences as whitespace. This can be problematic if you're trying to read binary data, which should be treated as raw bytes without any interpretation.
Using the binary mode flag, `std::ios::binary`, during the file opening process with `open()` is crucial for ensuring that the data is read exactly as it's stored. This is essential for maintaining data integrity, especially when dealing with files containing null characters or specific byte patterns that could be misunderstood in text mode.
Binary files often contain complex data structures, requiring a clear understanding of the file's format to correctly parse the data. The arrangement of data within the file can vary substantially depending on how the file was created, so understanding the design of your target files is a critical part of processing binary data successfully.
Binary mode is essential for handling files produced by applications that operate at the byte level. This includes a wide array of files, such as images, executables, or files designed for specific applications. Ignoring this detail can result in incorrect interpretations of the file data.
How files handle end-of-line characters can be operating system dependent, adding another level of complexity to binary file reading. For example, Windows traditionally uses CRLF for end-of-line sequences, while Unix-based systems use LF. Understanding this can be relevant when transferring files between systems or when working with files created across different platforms.
Beyond the more obvious challenges of binary reading, there are less apparent subtleties to consider. Byte order (endianness) for integers and floating-point values in a binary file can be very different across computer systems. It's critical to understand and take care of these aspects when dealing with cross-platform or heterogeneous environments.
The C++ standard is clear that using formatted input operations on binary files can lead to undefined behavior. This underscores the importance of using the correct stream mode when interacting with binary data. Using the incorrect mode is one of the most frequent ways of introducing silent data corruption and unexpected application behavior.
Proper stream management is vital when dealing with binary files. Failure to close streams correctly can result in resources, like file handles, remaining open and can cause leaks, eventually leading to system instability. The `close` method is a simple safeguard against these potential errors.
Unlike text files, binary files are inherently not human-readable. This presents some unique challenges in debugging. If something goes wrong while processing a binary file, analyzing the raw contents doesn't offer immediate clues about the source of errors. This emphasizes the need for very meticulous and thorough documentation of the binary file format itself.
All in all, understanding the subtleties of binary files and how C++ handles them through `ifstream` is vital for any engineer who wants to develop robust and reliable C++ applications that deal with binary data.
A Complete Guide to Reading Binary Files in C++ Using ifstreamread() - Managing File Stream Buffer Size For Binary File Operations
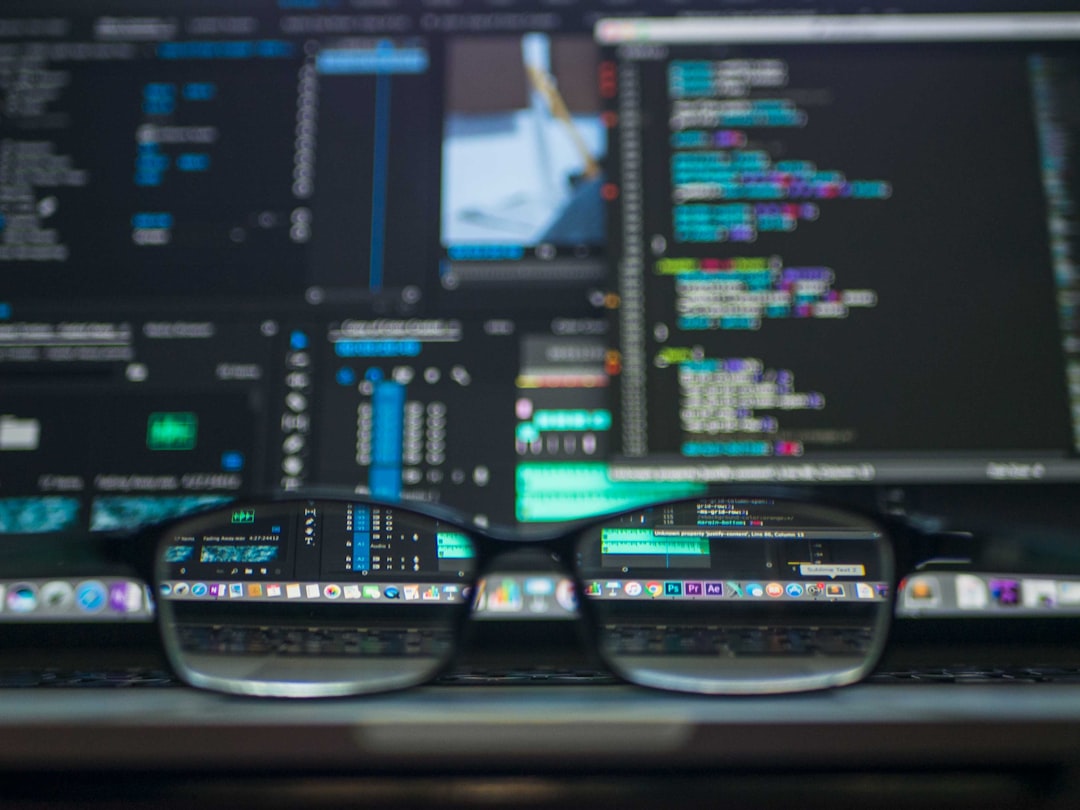
When dealing with binary file operations in C++, efficiently managing the buffer size used by the file stream is crucial, particularly for large files. While many systems employ a default buffer size of 4096 bytes, adjusting this can optimize performance. For typical binary file operations, it's often best to keep buffer sizes within a reasonable range – from 1 kilobyte to 32 kilobytes. However, some specific operations, like copying files asynchronously, might see improvements with larger buffers, perhaps up to 2 megabytes. The goal is to reduce the overhead of frequently calling the operating system's read/write functions. Choosing an appropriate buffer size requires considering how it interacts with the file's internal structure and format to ensure correct data retrieval while maximizing speed. It's a delicate balance between minimizing the frequency of calls to lower-level system routines and potentially causing memory management complexities with excessively large buffers.
When dealing with binary files, the size of the buffer used by the file stream significantly impacts performance. Larger buffers generally translate to fewer read/write operations, leading to better efficiency, especially for large binary files where I/O is the limiting factor. Finding the perfect buffer size, however, requires balancing various factors.
Experimenting with different sizes—like 4KB, 16KB, or even 64KB—can help identify the sweet spot, as performance can vary greatly based on the system and storage characteristics. However, it's crucial to remember that file systems have their own limitations and requirements regarding buffer sizes. Certain file systems might have specific alignment rules that can add overhead if not properly addressed.
Moreover, mismanaging buffer sizes can cause problems related to memory usage. Buffers that are too large can waste memory, whereas those too small can result in excessive memory fragmentation and frequent memory allocation calls. In contrast to line buffering used in text files, binary operations benefit from block buffering where larger chunks are processed in a single go, minimizing overhead.
When working with multiple threads, buffer sizes and thread synchronization become critical. Improperly sized buffers can cause contention among threads, impacting throughput and creating delays. In addition to efficient processing, managing errors and interruptions becomes crucial when handling binary data. A well-managed buffer can help ensure data integrity by only committing data to the file after successful operations, thus reducing the risk of data corruption due to unexpected events.
The significance of buffer alignment comes into play when working with multi-byte integers and floating-point numbers. Incorrect buffer sizes can lead to misalignment, resulting in performance penalties or, in certain cases, application crashes due to architecture-specific hardware constraints. Operating system caches also interact with file buffers. A well-chosen buffer size can minimize the frequency of disk access calls, leading to faster reads and writes and boosting overall application performance.
Ultimately, while some buffer sizes are commonly used, determining the most effective size for a given application often requires profiling and testing. Specific system factors, such as CPU architecture and the speed of the storage device, play a crucial role in finding the best settings for optimal performance. It's an iterative process of investigation and analysis to find what works best in each situation.
A Complete Guide to Reading Binary Files in C++ Using ifstreamread() - Reading Raw Bytes Into Memory Using ifstreamread Function
The `ifstream::read` function in C++ provides a way to directly read raw bytes from a binary file into memory. This is done by creating an `ifstream` object and using its `read` method, where you specify the memory buffer and the number of bytes you want to read. This method offers a straightforward way to access the binary file's raw data without any interpretation. Efficiently managing the size of the buffer you use is important, as reading larger chunks of data at a time can improve performance by reducing the number of times your program needs to interact with the file system. This approach can also decrease the impact of I/O operations on the application. When reading raw bytes from a file, it's important that your reading approach aligns with the file's underlying structure to prevent unexpected memory issues or corrupted data. Thorough error handling is essential, and it is vital to understand the structure and format of the binary data being read to ensure accurate processing. Following these guidelines can help create C++ programs that reliably and accurately process binary data.
1. When dealing with raw bytes, the system's architecture and data types can introduce alignment constraints. Failing to adhere to these constraints when reading can lead to performance issues or crashes, especially when working with data types like `double` or `long long`. This is particularly crucial on systems where memory alignment impacts operation efficiency.
2. The order in which bytes represent multi-byte data types (endianness) can differ between systems. If this isn't considered when reading binary data, it can cause significant issues in data interpretation, especially in applications designed to work across different systems. Understanding and handling endianness is a vital aspect of binary file reading.
3. Keeping an eye on the `ifstream`'s status is vital. Unforeseen issues, like hitting the end of the file or encountering an error, can result in silent failures, where the program runs but produces inaccurate results. Consistently checking the stream's state and implementing robust error handling is essential.
4. Utilizing functions like `seekg()` can enhance performance by enabling us to move the file pointer to specific locations in the file. This is beneficial in situations where certain offsets within the binary file correspond to specific structures, allowing us to optimize the reading process.
5. If we're not mindful of the buffer size, there's a risk of buffer overflows, a critical issue that can lead to crashes or security weaknesses. Always make sure that the amount of data being read doesn't surpass the allocated buffer capacity. This is a vital precaution for ensuring the stability and security of applications dealing with binary files.
6. Each binary file format may have its unique specifications on how data should be read. Failing to understand the format of the target file when reading raw bytes can lead to misinterpretations or data loss. It's crucial to carefully document and understand the file format before processing.
7. Reading binary data tends to be quicker compared to text data. This is because binary files are compact and do not involve parsing or interpreting character sequences. This advantage makes byte-level operations very attractive for performance-sensitive applications.
8. In contrast to text files, binary files can contain null bytes (`'\0'`). We need to be careful when handling these null bytes, as their presence can cause premature string termination if treated like a character in a text stream, potentially leading to data loss.
9. Remember that reads from an `ifstream` into a buffer are stateful. If earlier reads have been handled incorrectly (like encountering errors), future read operations might fail. This implies we should consistently check if read operations are successful to avoid issues stemming from prior read errors.
10. While static buffers are commonly used, employing dynamically allocated buffers can adapt to file sizes or content. This offers more flexibility in memory management, especially when handling large datasets or files containing variable-length records. Dynamic buffers are beneficial when dealing with data that may vary significantly in size.
A Complete Guide to Reading Binary Files in C++ Using ifstreamread() - Structuring Data Types To Match Binary File Layout
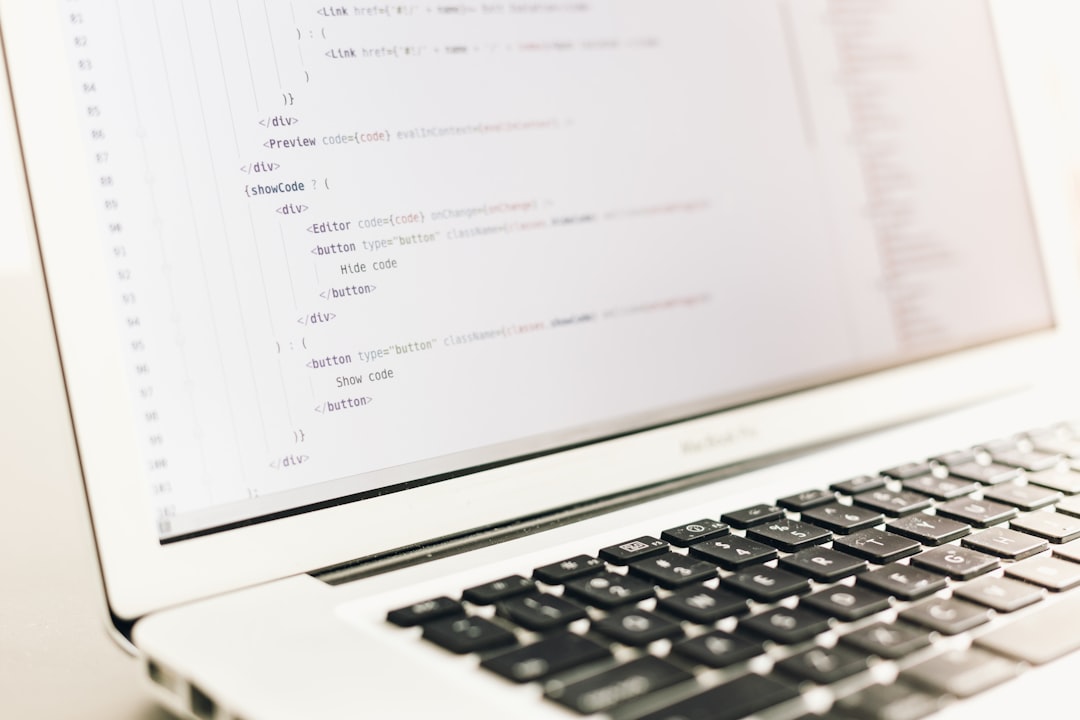
Effectively working with binary files in C++ often involves carefully aligning your data structures with the file's layout. Binary files provide a way to store and retrieve intricate data structures, like structs and arrays, efficiently. You can read or write these structures as a single unit using functions such as `fread` and `fwrite`. However, this method depends on a thorough understanding of the data's organization within the file, including the order in which bytes are arranged (endianness) and how data is aligned in memory. This alignment can differ between various computer systems.
To ensure that the data is read and written correctly, the way you define a struct in memory should accurately reflect the layout in the binary file. Failing to take into account these aspects can introduce data corruption and hamper your file operations. Therefore, carefully planning your data structures, and diligently documenting the format of the binary file you're working with, are crucial for developing robust and reliable C++ code.
When we work with binary files, the way data is structured within them becomes extremely important. It's not just about the types of data and their sizes, but also about things like padding and alignment within the computer's memory. Compilers often add extra bytes (padding) to ensure data is aligned properly with the CPU, which can cause issues if we don't account for them when reading the data.
Each computing system (like a Windows machine or a Linux server) has its own conventions for how data types are arranged in memory. This can affect how we structure the data in binary files. For instance, an x86 processor might align data on byte boundaries, while an ARM processor might need a more complex arrangement.
When dealing with binary files, we need to pay really close attention to every detail. Even small differences between how we expect the data to be organized and how it's actually stored in the file can lead to errors that are tough to find. This becomes especially tricky when we have structures where the size or order of the data changes during the compilation process.
There can be differences in how data is represented between C++ and a binary file, leading to challenges, particularly when it comes to localization. For example, two integer numbers might be stored in different formats (like little-endian and big-endian). If a file created on one type of system is read on another, the values we get might not be what we expect.
The `sizeof` operator in C++ can provide a different value than the actual number of bytes written in a binary file because of the potential for padding and alignment. It's really important to carefully figure out how the compiler organizes the data in our code. Miscalculations can lead to problems reading the file or, in worse cases, corrupting the data.
We might imagine that named structures in a binary file mirror their definitions in our C++ code, but that's not always the case. Without a thorough understanding of how the data is organized, we can easily misinterpret how the members are arranged, especially with complex or compressed structures that don't follow the layout we might expect.
If a binary file is created by a different program or in a different language, the underlying data structures in the file might not line up with what our C++ code expects. This mismatch frequently leads to difficult-to-debug errors and can cause data loss if we don't fully grasp the file format.
The C++ standard library doesn't enforce a specific binary file format, which can create problems across different systems or compiler versions. This means we need to meticulously document how our binary files are structured and collaborate well with other developers who might be working with the same files to avoid problems.
When we store complex structures in binary files, we often rely on systematic patterns. For example, we ensure that each data type contributes a predictable amount of bytes to the file's layout. Without these patterns, figuring out the correct order to read each part of the structure can become a challenge and prone to errors.
Finally, tools that let us visually explore and examine the contents of a binary file can be a tremendous help in understanding how it's organized. These tools reveal the hidden arrangement of bytes and can help us debug issues that might be difficult to uncover by just looking at our code.
A Complete Guide to Reading Binary Files in C++ Using ifstreamread() - Handling Error States And End Of File Detection
When reading binary files using `ifstream` in C++, it's crucial to manage potential error states and detect the end of the file (EOF). The EOF condition signifies that no more data is available to read, acting as a crucial signal to stop further read attempts. Properly handling this is vital to ensure your program behaves as expected.
C++ provides methods like `good()`, `bad()`, `fail()`, and `eof()` to assess the current state of the `ifstream` object. These are important for building robust error checks into your code. If you don't diligently check the stream's status after each read operation, you risk silently encountering corrupted data or unexpected program terminations.
Ignoring error states can have serious consequences, and it's better to have your application gracefully handle errors rather than crash or behave unexpectedly. Consistently monitoring the `ifstream` object and implementing appropriate error handling logic can help you write more stable and predictable code that safely interacts with binary files. Alongside error management, good file management, like closing files when finished, also contributes to a more resilient and reliable application.
When working with binary files in C++, effectively managing error states and detecting the end of a file are essential to ensure your applications behave as expected and don't crash due to unforeseen issues. Errors can manifest in various ways, and ignoring them could lead to corrupted data being processed without any warning. Constantly checking the `ifstream` object's status can reveal if unexpected issues occurred.
It's crucial to distinguish between reaching the end of a file and encountering an actual error during the read process. The `eof()` method provides a clear indicator of when the end of a file has been reached. However, if something went wrong during a read operation, such as corrupted or misaligned data, it's more likely that `fail()` will trigger. Properly discerning these cases is vital for understanding the root cause of the problem.
C++ provides handy stream states like `goodbit`, `badbit`, `eofbit`, and `failbit`. These states are like flags signaling various conditions encountered during file operations. By managing and interpreting these states properly, engineers can build programs that respond gracefully to errors, improving overall stability and resilience.
The read buffer's size can also influence error detection. When employing a small buffer, the read operation is called more frequently. This can lead to a greater likelihood of running into problems like corrupted data that a larger, appropriately sized buffer might have handled better by loading a bigger chunk of data at once.
Binary files frequently have restrictions on how data types are organized and arranged in memory. These rules for alignment can differ across systems. If the data structure defined in your code does not perfectly match the file layout, read errors are likely to occur. This mismatch can lead to data corruption or severe application failures if not managed adequately.
Compiler differences across various platforms and architectures can lead to the same binary file behaving differently during file reading operations. For instance, certain compiler settings or underlying instruction sets can impact how binary data is represented in memory. Recognizing and planning for these variances is essential to ensuring consistent behavior and handling errors across different environments, making error handling more complex.
The `std::ios::binary` flag is not only crucial for preserving the raw data in a file. It also impacts the way errors are detected and handled. Setting the `std::ios::binary` flag disables any unintended character translations that might inadvertently mask or interfere with the detection of errors during data retrieval.
Using text-oriented input functions when reading binary data can cause unintentional character interpretations during input. This can lead to immediate errors due to unexpected or corrupted data. Consequently, it is generally safer to avoid text-oriented input entirely in the context of binary operations and stick to reading raw byte sequences for error handling.
Troubleshooting issues with binary files can be significantly more challenging than with text files due to the inherent non-human-readable nature of the data. When errors occur, simply viewing the contents of a binary file doesn't offer immediate clues. Engineers commonly use various logging techniques and tools to record and store details about the stream state and the errors encountered, aiding in debugging and understanding the source of issues.
Relying solely on `eof()` as an indicator that the read operation is successful can be problematic. There are situations where `eof()` returns `true`, yet the read operation failed due to corrupt data. It's always advisable to immediately check the stream's state right after a read operation to avoid being misled and prevent potential issues.
In conclusion, understanding how to manage error states and detect the end of file is essential for effectively working with binary data in C++. By implementing thorough error handling routines, recognizing different error conditions, and managing stream states, we can improve the reliability and resilience of our C++ applications dealing with binary files. Continuous monitoring of the stream state and understanding potential pitfalls during EOF detection are vital aspects of producing robust and reliable applications.
A Complete Guide to Reading Binary Files in C++ Using ifstreamread() - Memory Safety Practices When Reading Binary Files
When working with binary files in C++, ensuring memory safety is crucial to prevent data corruption and application errors. This involves setting the file stream to binary mode using `ios::binary` to avoid unwanted data transformations and ensure that the raw data is read as it's stored. Robust error handling is essential to catch issues like insufficient data or failures during stream operations, safeguarding against unexpected program behavior. Moreover, you must manage buffers effectively. Using buffers that are too small can lead to inefficient reading, and misaligned buffers can cause crashes or performance degradation. Furthermore, meticulously align data structures in your C++ code with the binary file's layout, preventing misinterpretations and data inconsistencies across platforms. Combining these memory safety practices results in more reliable and robust C++ applications that handle binary files accurately.
When working with binary files in C++, several aspects need careful consideration to avoid unexpected issues. Failing to set the `std::ios::binary` flag can lead to unintended text interpretations of binary data, potentially introducing system-specific changes that corrupt the original data. This can result in subtle errors that are difficult to track down.
Another challenge is dealing with endianness, the way integers and floating-point numbers are stored in memory. Different systems might use big-endian or little-endian representation, leading to severe data corruption if not addressed during cross-platform file reading.
Memory alignment, the way data is arranged in memory, is also crucial. Certain data types might have alignment restrictions based on the system's architecture, and ignoring these requirements can lead to performance issues, access violations, or crashes, especially when working with larger data types like structs with `double` or `long long`.
It's essential to be aware of the `ifstream` object's state after each read operation. Ignoring error signals like those from `fail()` or `eof()` without proper checks can lead to processing data that hasn't been read correctly, potentially causing subtle data inaccuracies.
Dynamically allocated buffers are often more efficient for reading binary data. This flexibility lets applications adapt to file structures with variable-sized data records, leading to more efficient memory usage and potentially improving performance.
Binary file sizes can vary tremendously, making buffer size selection critical. Large files may benefit from larger buffers to optimize read operations, but using buffers that are too large can lead to wasted memory. It's often helpful to benchmark different buffer sizes to find the best option for each application.
If corrupted data isn't handled correctly, it can lead to situations where the application appears to function but produces incorrect results without any clear indication of the problem. It's beneficial to regularly validate the integrity of the data through checks of the `ifstream` object.
Binary files are not human-readable in their raw form. Understanding the format and structure is essential to identify any issues. Having a standard for documenting binary file formats becomes vital for effective collaboration between developers.
Compiler behavior can vary when dealing with binary data structures. The same code might work flawlessly on one compiler but fail or misinterpret data on another. This variability necessitates establishing consistent data handling practices across all environments.
Finally, debugging binary file issues can be difficult due to the non-human-readable nature of the data. Robust error logging during file operations can provide valuable insights when errors occur. By capturing details about stream states and errors, engineers can often quickly pinpoint the source of an issue.
Create AI-powered tutorials effortlessly: Learn, teach, and share knowledge with our intuitive platform. (Get started for free)
More Posts from aitutorialmaker.com: