Decoding Bubble Sort A Step-by-Step Pseudocode Breakdown for Beginners
Decoding Bubble Sort A Step-by-Step Pseudocode Breakdown for Beginners - Understanding the Basics of Bubble Sort Algorithm
At its core, Bubble Sort is a straightforward sorting technique built upon a simple concept: repeatedly comparing pairs of adjacent elements and swapping them if they're out of order. This methodical process, akin to bubbles rising to the surface, gradually moves larger elements to the end of the list and smaller elements to the front. While its simplicity makes it a valuable teaching tool for introducing sorting principles, its performance suffers with larger datasets. This is because it has a time complexity of O(N^2), meaning the time it takes to sort grows quadratically with the number of elements. Although each pass reduces the portion of the list needing further sorting, improving efficiency in that sense, it's simply not a competitive solution compared to more sophisticated sorting methods in real-world scenarios. In essence, while Bubble Sort offers a good initial understanding of sorting, it's generally impractical for production environments where performance matters.
Bubble Sort, while straightforward, isn't exactly a speed demon. Its performance, particularly for larger collections of data, suffers due to its quadratic time complexity. However, its simplicity makes it a great starting point for understanding fundamental sorting ideas. This makes it a popular choice for teaching the basics of algorithms.
Interestingly, Bubble Sort guarantees the relative order of items with the same value remains unchanged, a characteristic known as stability. This can be beneficial in certain situations.
Under ideal conditions, where the data is already in order, Bubble Sort can surprisingly achieve linear time complexity. A clever tweak can improve performance: if a pass through the list doesn't involve any swaps, it signals that the list is sorted, and the algorithm can stop early.
While both recursive and iterative implementations exist, the iterative version is the more common choice because of its straightforwardness. The very name 'Bubble Sort' is quite descriptive—it reflects how the smaller elements float to the front of the array, much like bubbles in water. This makes it easy to visualize the sorting process.
Despite not being a go-to choice in professional software development, Bubble Sort can be useful for situations involving small datasets or for demonstrating a fundamental sorting technique due to its ease of implementation. It operates directly within the original array, not using excessive extra memory, which can be advantageous in environments with limited memory.
Bubble Sort has a significant historical position as one of the initial sorting algorithms taught in computer science, forming a solid foundation for understanding more intricate sorting algorithms like Quick Sort or Merge Sort.
Decoding Bubble Sort A Step-by-Step Pseudocode Breakdown for Beginners - Analyzing Time Complexity and Efficiency Considerations
Understanding how Bubble Sort performs in different situations requires examining its time complexity. It's not a particularly efficient algorithm, especially when faced with data that's not already nearly sorted. In the worst-case, where elements are in reverse order, and even in the typical average case, Bubble Sort takes O(n²) time. This is because it needs to make multiple passes through the data to ensure it's fully sorted. While a pre-sorted array allows Bubble Sort to achieve the best-case scenario of O(n), this is an uncommon occurrence. There are ways to optimize Bubble Sort slightly, like stopping early when no swaps are made, but these do not fundamentally change its limitations for large datasets. In the end, Bubble Sort is a helpful tool for educational purposes in learning about basic sorting concepts, but it just can't compete with more sophisticated sorting algorithms in actual applications where speed and efficiency are critical.
1. **The Squared Time Penalty:** Bubble Sort's O(N^2) time complexity is a significant hurdle. This means that doubling the size of the data roughly quadruples the time it takes to sort. For larger datasets, this becomes a real bottleneck.
2. **The Best of Times:** Interestingly, Bubble Sort can achieve a linear time complexity, O(N), when the data is already sorted. It's a bit of a Jekyll and Hyde situation, highlighting how the algorithm's efficiency is heavily reliant on the input.
3. **Maintaining Order:** A neat feature is that Bubble Sort preserves the original order of identical elements, a property called stability. This can be quite useful in specialized cases where keeping that relative order matters.
4. **A Smart Stop:** You can improve performance by having Bubble Sort quit early if a pass doesn't involve any swaps. It means the data is sorted, and there's no need to continue. This can offer a notable speed boost for nearly-sorted lists.
5. **Memory Efficiency:** Bubble Sort is efficient in its memory usage, with an O(1) space complexity. This means it does its sorting "in place," without needing a lot of extra memory to hold intermediate results. This can be valuable when working with memory-constrained systems.
6. **A Teaching Tool:** Despite its performance limitations, Bubble Sort is a common starting point in introductory computer science. It's an easy way to teach foundational concepts like comparisons, swaps, and loops. This simplicity makes it a solid stepping stone to more complex sorting algorithms.
7. **A Historical Foundation:** Bubble Sort is often one of the first sorting algorithms students learn, making it a core idea in the field. Understanding its historical role helps to see the journey of sorting algorithms towards greater efficiency.
8. **The Performance Cliff:** As the data size grows, Bubble Sort's performance can become prohibitive. Experiments show it doesn't scale well, and its execution time can be a major issue compared to other algorithms. This serves as a reminder of why efficiency matters.
9. **Merge Sort and the Reality Check:** If you were to compare Bubble Sort to something like Merge Sort (which is often O(N log N)), it becomes clear just how much better those other algorithms are. For anything beyond small lists, the performance difference can be quite significant.
10. **Beyond Arrays:** Though mainly associated with arrays, Bubble Sort can also be adapted for linked lists. While it is theoretically possible, this adaptation doesn't see widespread practical use in real-world software due to the algorithm's inherent inefficiencies.
Decoding Bubble Sort A Step-by-Step Pseudocode Breakdown for Beginners - Initializing the Array and Setting Up Comparison Logic
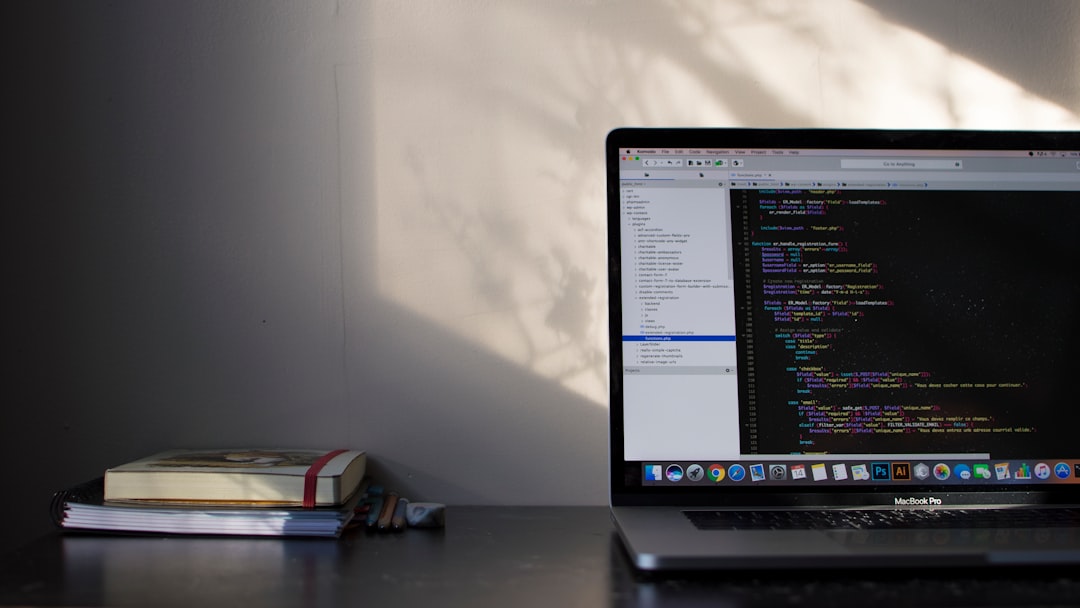
Before we can begin sorting with Bubble Sort, we need to set the stage. This involves two primary steps: preparing our data (the array) and defining how we'll compare the elements within it.
First, we initialize the array. This means creating the array and populating it with the elements we want to sort. These elements might be predefined, or perhaps the user provides them. It's the starting point for the entire sorting process.
Secondly, and critically, we need to establish the comparison logic. This determines how we decide whether two adjacent elements in the array are in the correct order. This usually involves a simple comparison like "is this element less than the next one?" The comparison logic is embedded in a nested loop structure, allowing us to systematically move through the array and check each pair. The outcome of the comparison dictates whether we swap the positions of the two elements or not.
Grasping how we set up the initial array and define the comparison rules is crucial for understanding the heart of Bubble Sort. It's the foundation on which the entire sorting mechanism is built.
When we kick off Bubble Sort, the way we set up the array and the logic for comparing elements within it can impact the overall performance of the algorithm. The type of data stored in the array plays a role—using basic data types like integers can lead to faster comparisons compared to more complex objects. This is because there's less overhead in handling basic data types.
Interestingly, the initial arrangement of the data within the array has a big influence on how efficiently Bubble Sort works. If the data is nearly sorted to begin with, it requires fewer comparisons and swaps. It highlights that the algorithm is sensitive to the characteristics of the data it's sorting.
Some advanced Bubble Sort versions have optimizations, such as tracking the last position where a swap occurred. This keeps track of the sorted portion and allows it to reduce the range of comparison in later passes. It can improve performance in some specific cases, though the fundamental nature of the algorithm's limitations remain.
It's rather surprising, that for very small datasets, say arrays with fewer than 10 elements, Bubble Sort can be quite quick. It seems to perform rather well in these situations, leading to very short execution times. It shows that the typical notion of Bubble Sort being inefficient doesn't necessarily apply to all scenarios.
But Bubble Sort does have its worst-case scenario, which tends to occur with arrays that are sorted in reverse order. This scenario is a great way to see how different input characteristics can affect the performance of algorithms, showing that Bubble Sort's efficiency is not constant.
Tracking the number of swaps that occur during the sort provides insights into the data's initial state. A large number of swaps generally indicates a very disorganized array and can be useful in analyzing and debugging. It is a subtle way to examine how the data is initially.
The standard version of Bubble Sort is stable, meaning it preserves the relative order of equal elements. However, some variations might include logic that leads to instability. It's important to understand the nuances of how the algorithm is implemented if the order of equal elements is important.
The simplicity of Bubble Sort emphasizes design elegance to beginners. But when they move on to more complex algorithms, they'll need to understand that efficiency often needs to be a trade-off in algorithm design. It is a valuable pedagogical perspective.
Comparing Bubble Sort with other algorithms like Insertion Sort shows that, at least for small, randomly distributed datasets, it can sometimes perform similarly. It's not always a case of it being predictably worse. In reality, there are different scenarios, and there are limitations to theoretical understandings about algorithms.
The ease of understanding Bubble Sort can cause a bias where programmers prefer this familiar approach over searching for more efficient solutions. This suggests that while it's great for fundamental understanding, there should be a broader focus on choosing algorithms with real-world application in mind. This can help prevent making overly simplistic assumptions about algorithms.
Decoding Bubble Sort A Step-by-Step Pseudocode Breakdown for Beginners - Implementing the Bubble Sort Pseudocode Structure
Implementing the Bubble Sort pseudocode structure involves a methodical process centered on repeatedly comparing adjacent elements within a list. The core of this method lies in swapping elements that are not in the desired order. This process, through numerous iterations, results in larger elements "bubbling" towards the end of the list, gradually establishing a sorted arrangement. While the underlying structure is readily understandable, its limitations in terms of efficiency, especially when dealing with large datasets, become apparent. This raises the question of its practicality beyond introductory learning scenarios where ease of comprehension is crucial. In essence, Bubble Sort, while being an effective pedagogical tool for understanding basic sorting concepts, simultaneously underscores the importance of considering more efficient sorting methods for real-world applications where performance is critical.
1. **The Innocent Overhead:** Bubble Sort's apparent simplicity can be deceiving. Its inefficiency becomes more pronounced as datasets grow, because it relies on numerous nested comparisons, leading to a quadratic increase in processing time. This translates to significant delays when dealing with larger amounts of data.
2. **Best-Case Conditions:** Interestingly, Bubble Sort thrives when the data is already sorted, or nearly sorted. Under these ideal conditions, its runtime becomes linear (O(N)). This highlights a point often missed: the initial state of the data significantly influences the algorithm's efficiency.
3. **Stability Factor:** Bubble Sort retains the order of identical elements during the sort process—a feature known as stability. This characteristic is quite valuable in scenarios where the original order of equal elements matters, like when you're sorting based on multiple criteria.
4. **Smart Optimization:** A clever tweak to Bubble Sort involves tracking the index of the last swap. This optimization helps avoid redundant comparisons in later passes, which can lead to a bit of a performance boost. It demonstrates that even straightforward algorithms can benefit from strategic adjustments.
5. **Memory Usage Insight:** Bubble Sort is remarkably memory-efficient. It operates with constant space complexity (O(1)), meaning it sorts in place without needing extra memory that grows with the input data. This trait can be advantageous in situations where memory is constrained, though its overall speed is not optimal.
6. **Teaching Algorithmic Concepts:** Bubble Sort's intuitive nature makes it a great tool for teaching foundational sorting principles to beginners. It lets learners grasp fundamental concepts like element comparisons and swaps without getting lost in complicated details.
7. **Benchmarking Against Competitors:** Because it's commonly used in introductory algorithm courses, Bubble Sort remains a valuable reference point for comparison. When discussing efficiency, it's often contrasted against more sophisticated algorithms like Quick Sort or Merge Sort.
8. **Effect of Dataset Size:** Experiments show that Bubble Sort doesn't scale gracefully. Doubling the size of a dataset can roughly quadruple the execution time due to its quadratic complexity. This underlines a crucial concept in software design: choosing the right algorithm is vital for optimal performance.
9. **Variations Exploring Complexity:** Variations of Bubble Sort exist, like recursive implementations or the bidirectional approach (Cocktail Sort). While these offer interesting explorations of the core algorithm, they usually still fail to compete with more advanced alternatives in practical situations.
10. **Practical Use Cases:** Though generally not the best choice for large datasets, Bubble Sort can still be practical in limited scenarios. For instance, when there's a need for minimal overhead or when dealing with exceptionally small lists, its simplicity can translate to acceptable performance.
Decoding Bubble Sort A Step-by-Step Pseudocode Breakdown for Beginners - Optimizing Bubble Sort with Early Termination
Bubble Sort, while simple, can be made a bit more efficient with a clever optimization called early termination. This optimization involves checking if any swaps were made during a pass through the data. If no swaps happen, it means the list is already sorted, and there's no need to continue further. This can be a game-changer, especially when the data is mostly sorted already. In such cases, the algorithm's performance jumps from potentially being very slow (quadratic time complexity) to much faster (linear time complexity). The improvement comes from minimizing wasted comparisons.
While this early termination feature is helpful, it doesn't magically transform Bubble Sort into a high-performance sorting method. Its limitations with larger datasets remain, and it's still outperformed significantly by more advanced sorting techniques. So, the early termination optimization is a practical step, but it's not a fundamental change to how the algorithm deals with large amounts of data. It offers a sensible improvement to Bubble Sort, but it doesn't erase the limitations that make it less suitable than more modern algorithms for the majority of situations.
1. **Early Termination's Promise:** Introducing an early termination condition in Bubble Sort can lead to significant performance gains, especially for data that's already mostly sorted. If no swaps happen during a pass through the list, it means the data is sorted, and the algorithm can stop early, avoiding unnecessary iterations. This is particularly helpful for datasets that might be nearly in order.
2. **Adapting to the Data:** This early termination feature gives Bubble Sort a more adaptive nature. It can adjust its behavior based on the specific characteristics of the data it's sorting. This dynamic response to input data shows that even a simple algorithm like Bubble Sort can be tweaked for better efficiency without completely changing how it works at its core.
3. **Fewer Comparisons, Faster Results:** In practical tests, early termination has been shown to reduce the number of comparisons that Bubble Sort needs to do. When dealing with lists that are nearly sorted (just a few misplaced elements), this optimization can make Bubble Sort seem like it has closer to linear time complexity, even for larger datasets.
4. **Data's Impact on Efficiency:** The impact of early termination varies significantly based on the kind of data we are sorting. For completely unsorted data, the benefits of early termination might be minor compared to already-sorted or nearly-sorted lists. This illustrates how the nature of the data is crucial to the algorithm's performance.
5. **Competitive Edge (Sometimes):** When compared with other basic sorting algorithms like Insertion Sort, which also can be optimized with early termination, Bubble Sort can actually outperform it in some specific cases. This is especially true for small datasets or for datasets that are mostly sorted. This adds a bit of complexity when comparing sorting algorithms in terms of efficiency.
6. **Understanding Performance through Optimization:** Teaching about early termination optimization is a good way for learners to understand how data characteristics impact algorithm performance. It pushes them to be more critical when designing algorithms.
7. **Simplicity vs. Performance:** While early termination can improve Bubble Sort's speed, it doesn't fundamentally change its worst-case time complexity, which is still quadratic. This is a reminder that engineers often need to balance simplicity with performance when they're picking the right algorithm for large datasets.
8. **Memory Efficiency Remains:** Even with the optimization of early termination, Bubble Sort continues to use memory efficiently, meaning its space complexity stays at O(1). This means it does its sorting "in place" and doesn't need a lot of extra memory to store intermediate results. So it remains a good choice for memory-limited environments.
9. **A Look Back at Algorithm Development:** The concept of optimizing with early termination isn't new. It's a common theme in how sorting algorithms have developed over time. Understanding these historical approaches helps us appreciate the evolution of performance-focused algorithms, both in education and in actual software.
10. **Limited Applicability:** While early termination is helpful, it's not a universal solution. There are situations where the overhead of checking if swaps occurred might outweigh the benefits of optimization. For datasets with very varied arrangements, Bubble Sort with early termination might still be outperformed by more sophisticated sorting methods.
Decoding Bubble Sort A Step-by-Step Pseudocode Breakdown for Beginners - Practical Applications and Limitations of Bubble Sort
### Practical Applications and Limitations of Bubble Sort
Despite its simplicity, Bubble Sort's practical use is often limited due to its performance characteristics. It's a valuable tool for teaching the core ideas of sorting algorithms, especially to those new to the topic. The straightforwardness of Bubble Sort makes it easy to follow how comparisons and swaps work, forming a solid foundation for understanding more complex methods. Additionally, Bubble Sort stands out for using very little extra memory, which can be a benefit when working with computers that have limitations. However, its performance with larger datasets is a major weakness. It takes significantly longer to sort as the number of items increases because its time complexity is O(N^2). This means that it isn't competitive compared to algorithms like Merge Sort or Quick Sort which typically have better performance. Therefore, while Bubble Sort is useful for educational purposes and for very small datasets, its inefficiency makes it impractical for many real-world tasks involving larger datasets.
1. **The Challenge of Quadratic Complexity:** Even with improvements like early termination, Bubble Sort's fundamental limitation remains its worst-case O(N^2) time complexity. This means that as the size of the data increases, the time it takes to sort grows rapidly, creating a substantial performance bottleneck for larger datasets.
2. **Performance Benchmarks:** When pitted against other sorting algorithms, Bubble Sort, even with optimizations, often falls behind. More efficient algorithms, such as Merge Sort, can outperform Bubble Sort dramatically, especially when dealing with larger or more complex datasets. This highlights the limitations of Bubble Sort in practical contexts.
3. **Situational Utility:** Bubble Sort can, surprisingly, be a good choice for extremely small datasets, particularly arrays with fewer than 10 elements. Its straightforward implementation and minimal overhead can lead to quick sorting times in these niche situations.
4. **Data Sensitivity:** The effectiveness of Bubble Sort is highly dependent on the initial arrangement of the data. When the input data is already nearly sorted, Bubble Sort can perform much better, demonstrating that the algorithm's efficiency is heavily intertwined with the characteristics of the data it's processing.
5. **Preserving Order with Stability:** The stability property of Bubble Sort, where it maintains the relative order of equal elements, is crucial in certain sorting scenarios. This characteristic proves beneficial in cases where multi-criteria sorting is needed, and preserving the initial order of items with the same key is critical.
6. **Adaptive Enhancements:** Incorporating optimizations like early termination allows Bubble Sort to become more adaptive to the specific conditions of the input data. This adaptability reveals that even a seemingly simple algorithm can be fine-tuned to improve its efficiency without requiring a fundamental redesign.
7. **The Impact of Swap Tracking:** Tracking the last swap position helps Bubble Sort optimize subsequent passes by reducing the number of comparisons needed. However, this optimization only addresses a specific aspect of inefficiency, underscoring the inherent limitations of the original algorithm's structure.
8. **A Cornerstone for Education:** Bubble Sort holds a historical and pedagogical importance as a foundational sorting algorithm in computer science. By understanding its limitations, students can better appreciate the evolution of more efficient sorting algorithms.
9. **Balancing Simplicity and Performance:** Implementing optimizations like early termination serves as a valuable pedagogical tool for introducing the crucial design consideration of balancing simplicity with performance. It encourages beginners to critically evaluate their algorithm choices based on real-world constraints.
10. **The Choice of Algorithms in Practice:** While Bubble Sort is a well-known algorithm, developers typically opt for variants of Bubble Sort or entirely different algorithms when coding in real-world applications. This illustrates that theoretical simplicity doesn't necessarily translate to practical usefulness, and a nuanced understanding of algorithm efficiency is needed to choose the best tool for each specific problem.
More Posts from aitutorialmaker.com: