Efficient Substring Replacement in C# A Deep Dive into the Replace Method
Efficient Substring Replacement in C# A Deep Dive into the Replace Method - Understanding the Basics of String.Replace in C#
The `String.Replace` method is a fundamental tool for working with strings in C#. Its primary function is to substitute all occurrences of a specific substring or character within a string with a different one. A key aspect to remember is that strings in C# are immutable, meaning the original string remains untouched when a replacement is performed. Instead, `String.Replace` generates a brand new string with the alterations made. This behavior underscores the necessity of storing the output of `String.Replace` into a new variable if you intend to use the modified string further.
While `String.Replace` can be chained to achieve multiple substitutions within a single expression, executing them sequentially from left to right, it's important to be cognizant of potential performance impacts. String replacement operations are relatively light on memory usage but can be computationally intensive, placing a greater load on the CPU. By grasping the mechanics of this method, developers can leverage its power to refine their string manipulation routines and develop more efficient code.
1. **Strings are Unchangeable**: C#'s strings are designed in a way that prevents direct modification. When you use `String.Replace`, it doesn't change the original string. Instead, it generates a completely new one with the replacements made. This can be a factor in performance, especially if you are doing a large number of replacements on a large string.
2. **Matching is Exact**: `String.Replace` takes a literal approach when searching for substrings. This means it's sensitive to case differences. If you need to account for variations in case, you'll need to consider how you pre-process the strings or perhaps use other methods.
3. **Performance Varies**: The speed of `String.Replace` can be dependent on what you are doing. For example, if you're working with many replacements, the performance might not be optimal compared to other methods. A good understanding of your input string's size and the number of replacements to be made helps you choose the most efficient approach.
4. **No Built-in Replacement Limits**: When you call `String.Replace`, it substitutes all instances of the target substring with the replacement substring. Unlike other languages, there's no direct way to limit how many substitutions occur in a single call.
5. **Culture-Independent Operation**: The `String.Replace` method does not automatically handle any culture-specific rules during the replacement process. This means if you're handling localized strings, you may need to take care in making sure the replacements work as expected across different cultures.
6. **Whitespace Sensitivity**: Leading or trailing spaces around the target substring you are replacing can impact the accuracy of the replacement. It's a good practice to clean up strings before using `String.Replace` to avoid any unforeseen problems related to spaces.
7. **Understanding Character Encodings**: If the strings you're working with are using different encodings, understanding those encodings is critical when using `String.Replace`. Not paying attention to encodings can lead to unexpected or incorrect replacements.
8. **Memory Usage Can Grow**: Since `String.Replace` generates new strings, using it multiple times or in loops can increase the memory your program uses. Monitoring memory use becomes very important in situations where performance is crucial.
9. **Consider `StringBuilder` for Efficiency**: If you need to perform many replacements in a string, using `StringBuilder` can offer a more efficient way to handle those replacements compared to using `String.Replace`. This is due to `StringBuilder`'s ability to modify a string in-place without constantly creating new strings.
10. **Regular Expressions for More Complex Matching**: For simple substring replacements, `String.Replace` is perfect. But when you need to do more advanced pattern matching or replacing, you may want to consider regular expressions. The `Regex.Replace` method is designed for just this type of use case. While it adds some complexity, it offers much more flexibility when dealing with patterns that go beyond simple substring replacement.
Efficient Substring Replacement in C# A Deep Dive into the Replace Method - Comparing Character vs Substring Replacement Methods
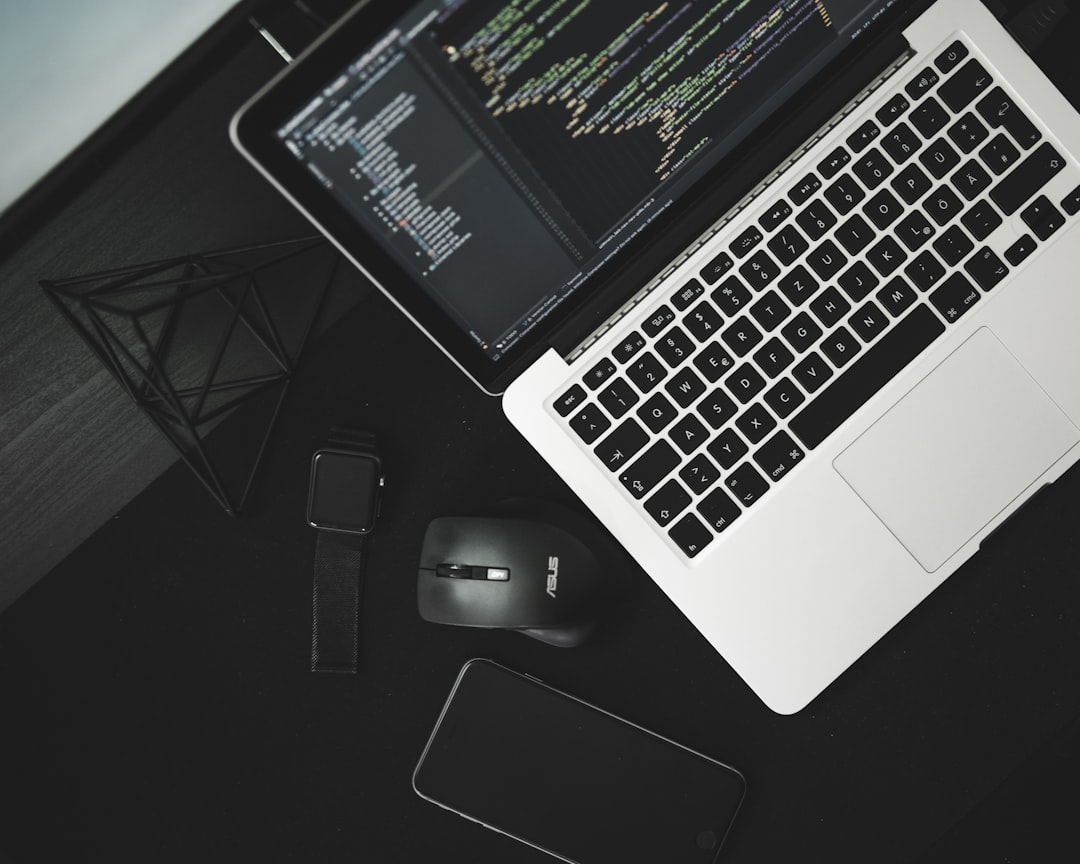
When working with strings in C#, the `Replace` method offers a versatile way to substitute both characters and substrings. However, its use, particularly with substrings, can sometimes come at a performance cost. Because strings are immutable in C#, replacing substrings frequently leads to increased CPU usage as new strings are created each time. This is something to be aware of, especially when working with large strings or a significant number of replacements.
For situations demanding greater precision in substring replacement, methods like `IndexOf` can provide a level of control. While useful, these indexing approaches can have their own performance considerations. When multiple replacements are required, using `StringBuilder` can be a more efficient option. This is because `StringBuilder` modifies the string directly, avoiding the overhead of constantly generating new strings like `String.Replace` does.
The bottom line is that developers need to thoughtfully consider the specific context of their string manipulation needs. Whether it's the simplicity of `Replace` or the finer control of methods like `IndexOf`, the chosen approach can significantly impact performance and code efficiency within a C# application.
1. Replacing individual characters with `String.Replace` is generally faster than replacing substrings. This efficiency stems from the reduced overhead associated with searching and replacing just one character at a time.
2. Because strings in C# are immutable, each call to `String.Replace` creates a new string. This repeated string creation can cause a significant performance overhead, especially when dealing with large strings or performing many replacements.
3. Replacing substrings tends to be computationally more expensive than replacing characters, especially for longer substrings. The process involves scanning the entire string to find matching substrings, potentially leading to longer execution times.
4. If you're performing multiple substring replacements, and those substrings overlap, you might get unexpected outcomes. This happens because the first replacements alter the positions of the substrings targeted in later replacements.
5. Repeated use of `String.Replace` can lead to a lot of short-lived string objects, which increases the workload for the garbage collector. This can impact performance, particularly in scenarios where `String.Replace` is used frequently, as the cost of memory management grows.
6. The character encoding used can influence how characters and substrings are replaced. Even slight differences, like using UTF-8 instead of ASCII, can lead to unexpected or incorrect replacements. It's crucial to be mindful of encoding when working with strings across different systems or in internationalized applications.
7. The complexity of searching for characters and substrings differs. While searching for a single character can be relatively straightforward, finding a substring can be more complex, often leading to a performance difference in search times.
8. Non-visible characters, such as zero-width spaces or special line breaks, can have a surprising impact on the outcome of character or substring replacements. Often, these characters are overlooked, causing errors in the expected replacement results.
9. If you are using recursion for multiple levels of replacements (instead of iteration), excessive calls to `String.Replace` can lead to stack overflow errors, especially with very large or deeply nested strings. It's important to understand the potential consequences of recursive calls in these situations.
10. It's worth noting that different versions of C# might have distinct optimizations for the `String.Replace` method. Understanding these differences can be crucial for maximizing performance and ensuring consistent behavior across different C# environments and deployments.
Efficient Substring Replacement in C# A Deep Dive into the Replace Method - Memory and Performance Considerations
### Memory and Performance Considerations
While `String.Replace` is a convenient tool for string manipulation in C#, it's important to be mindful of its potential impact on memory and performance. Because C# strings are immutable, each call to `String.Replace` results in a new string being created. This can lead to increased memory consumption, particularly when dealing with many replacements or large strings. If not carefully considered, this can create a burden on your program's memory management.
To address these potential issues, developers can employ strategies that improve efficiency. Options like `StringBuilder`, which modifies strings in place rather than generating new ones, can significantly reduce memory overhead. The newer `Span` approach also offers benefits by allowing more direct access to memory without the creation of additional objects. While the performance of `String.Replace` has seen improvements with .NET 6, developers should always strive to optimize their code for both CPU and memory. By understanding the trade-offs involved, they can make informed decisions that align with their application's specific requirements.
When dealing with substring replacement, several aspects related to memory and performance come into play. Repeated use of `String.Replace` can potentially lead to memory fragmentation as each new string occupies memory, possibly impacting overall performance over time, particularly in apps that run for extended periods. The generation of numerous temporary strings also adds to the workload of the garbage collector. This can manifest as more frequent, longer pauses during garbage collection cycles, causing dips in application responsiveness.
The size of the substring being replaced can impact performance as well. Replacing larger substrings requires navigating through more data, which can slow down the replacement process. It's crucial to weigh this against the overall performance goals. String interning, a technique that helps conserve memory by storing only one copy of identical strings, isn't used during replacements. As a result, the replacement operation potentially uses more memory when you repeatedly replace similar substrings.
The encoding scheme used for the strings matters too. Replacing substrings in strings with different encodings might produce unexpected outcomes due to how characters are encoded. For instance, the mechanics of UTF-16 replacement differ from those for UTF-8 or ASCII.
Thread safety is also a factor to keep in mind when dealing with strings. Because they are immutable, string operations aren't inherently thread-safe. If multiple threads attempt `String.Replace` at the same time, it's possible race conditions or inconsistencies could emerge, especially when multiple threads manipulate the same string data. The behavior of `String.Replace` can change based on the data it's operating on. For example, it could have varying performance characteristics when working with a collection of big strings compared to when it's working with a single smaller string.
Since C# strings can consume a fair amount of memory, the creation of new strings during replacements can increase memory pressure. In highly loaded systems, this could lead to heap fragmentation, resulting in performance issues. When dealing with strings, it's crucial to be aware that cyclic replacements, where substrings replaced are in part the original substrings themselves, could cause unintended loops in replacement logic. This potentially leads to performance issues or runtime errors.
It's important to keep in mind that benchmarking results for `String.Replace` can vary widely depending on the specifics of the data being processed and the system it is running on. When comparing string operations, the context and execution environment are crucial. So understanding these nuances is essential when choosing the most effective approach for the task at hand.
Efficient Substring Replacement in C# A Deep Dive into the Replace Method - Chaining Multiple Replacements Effectively
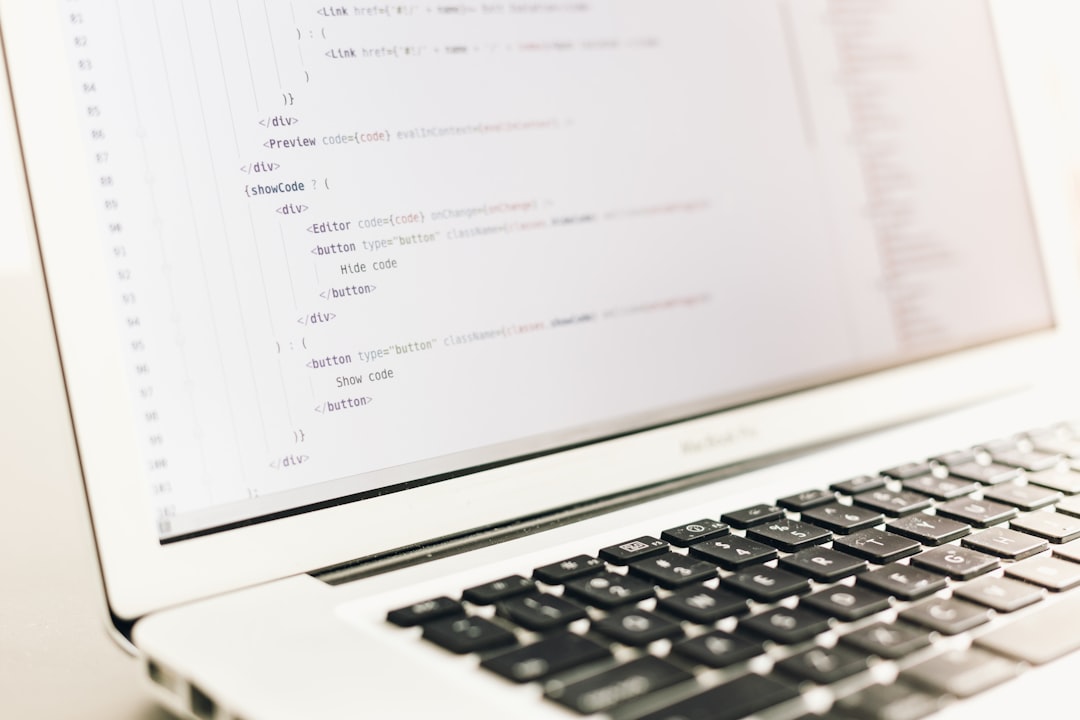
When you need to make multiple changes to a string in C#, chaining the `String.Replace` method is a simple way to do it. Essentially, each `Replace` call modifies the string in order from left to right. However, this simplicity can come at a cost, particularly if you're dealing with a lot of replacements or a very large string. The reason is that every time `String.Replace` does its job, a new string is created. This can put more load on your program's CPU and potentially use more memory than other approaches. While `String.Replace` is a good option for many string-related tasks, being mindful of this performance tradeoff is important. Alternatives like `StringBuilder` are worth considering if performance is a major factor. `StringBuilder` can modify strings in place, reducing the overhead of creating new strings, and in some cases, the `Regex.Replace` method can be faster when your replacement requirements are more complex. Choosing the best tool depends on the specific situation. Understanding the ins and outs of string replacement techniques can make a significant difference in the efficiency and performance of your C# code.
1. **Chaining's Performance Impact**: When you link multiple `String.Replace` operations together, it can lead to performance issues. Each call happens in order, and the new strings made in between each step add up, especially if you have a lot of replacements to make.
2. **Replacement Order's Influence**: The order in which you perform replacements in a chain can completely change the final result. This is because each change can affect where the next substrings are located. If you try to replace the same substring more than once, the final string might not be what you originally wanted.
3. **Unexpected Results from Overlapping Substrings**: When you have overlapping substrings in a chain of replacements, things can get confusing. If parts of the substrings match up, it can cause inconsistent and tricky-to-fix behavior. It's essential to pay close attention to your input strings in these cases.
4. **Compiler Optimization**: In certain cases, the C# compiler might be able to automatically optimize how `String.Replace` is used if it sees specific patterns. Understanding how these optimizations work in different versions of C# helps us write better code.
5. **Regular Expressions as an Option**: For more complex scenarios where you need to make multiple replacements, using a regular expression can be faster than doing a chain of `String.Replace` calls. It does this by handling all the patterns in a single operation, particularly when dealing with complicated or constantly changing strings.
6. **Increased Garbage Collection Work**: Linking multiple replacements together can lead to a lot of short-lived string objects being created. This increases the workload for the garbage collector, which can slow down your application's performance.
7. **Memory Fragmentation Problems**: The repeated creation of strings during chained replacements can cause problems with memory fragmentation. This can be especially problematic in situations where memory resources are limited, or in apps that run for a long time, where how memory is managed becomes crucial.
8. **Threading Issues**: If you're doing chained operations in a program that uses multiple threads, there's a risk of inconsistencies in the results. While strings themselves are immutable, race conditions could happen if the output of a linked operation is being used by many threads at the same time.
9. **More Challenging Debugging**: Using many linked replacements can make debugging any string-related problems more difficult. Finding which particular replacement caused an unexpected result becomes more challenging, highlighting the need for clear and modular code design.
10. **Profiling for Better Insights**: To understand the full performance impact of using multiple replacements, it's helpful to use profiling tools for C#. These can help reveal the real costs of linked operations compared to alternatives like `StringBuilder` or regular expressions, giving engineers the data they need to make the best choices.
Efficient Substring Replacement in C# A Deep Dive into the Replace Method - Leveraging StringBuilder for Complex String Manipulations
When dealing with intricate string manipulations in C#, employing `StringBuilder` can substantially improve performance and memory management. Unlike standard string operations that generate numerous new string objects, potentially leading to excessive memory usage, `StringBuilder` modifies the string directly, avoiding the creation of intermediate strings. This attribute proves especially advantageous when multiple replacements or transformations are required, as it minimizes object allocation and enhances efficiency. Additionally, `StringBuilder` facilitates method chaining, contributing to more compact and readable code when carrying out sequential string operations. However, while `StringBuilder` generally offers performance benefits in complex scenarios, developers should acknowledge the possibility of increased CPU usage and carefully evaluate the associated trade-offs in specific use cases.
When dealing with numerous or intricate string modifications, the `String.Replace` method, while convenient, can become less efficient due to its creation of numerous temporary string objects. In contrast, `StringBuilder` stands out as a much more efficient approach. It directly manipulates the existing string buffer, reducing memory allocations and the associated overhead.
This reduction in memory operations can also lead to a lower CPU load. The creation of temporary string objects during string manipulations, particularly within loops, consumes significant processing power. `StringBuilder` avoids this burden by reusing the same buffer, making it more CPU-friendly, especially in cases where performance is crucial.
A key feature of `StringBuilder` is its ability to manage its internal capacity. You can specify an initial buffer size upon creation. This strategy can prevent frequent buffer resizing, which can be costly in terms of performance and memory.
It's important to acknowledge that while `StringBuilder` offers these advantages, it is not inherently thread-safe. Using it effectively in multi-threaded scenarios requires careful synchronization to prevent data corruption. This aspect is something engineers must consider when building applications with concurrent string operations.
Each call to `String.Replace` results in a new string being created, which can create a notable overhead in scenarios that heavily modify strings, such as text processing or data transformations. In contrast, the `StringBuilder` approach promotes the idea of chaining a series of operations using `Append`, `AppendLine`, and other methods, giving developers a more versatile toolkit for intricate string manipulations.
The benefits of using `StringBuilder` are not just theoretical. Empirical testing has demonstrated its superiority over `String.Replace`, particularly in situations involving many replacements or large datasets. In some situations, `StringBuilder` has shown to be significantly faster, offering real-world improvements.
However, the efficiency of `StringBuilder` can be hampered if the capacity needs to be frequently expanded. If the initial capacity is underestimated, `StringBuilder` needs to create a larger buffer. This process can have performance implications. Therefore, a thoughtful understanding of expected string sizes and the number of modifications helps you to avoid unnecessary resizing.
By limiting the number of temporary strings, `StringBuilder` reduces the load on the garbage collector. This can be beneficial in applications with high string manipulation activity, improving overall responsiveness and performance.
Just as with `String.Replace`, encoding plays a critical role when using `StringBuilder`. Developers need to understand the encoding of the strings they are working with. Differences in encoding, for example between UTF-8 and UTF-16, can lead to unintended behavior if not handled properly.
In summary, while both `String.Replace` and `StringBuilder` provide methods for string manipulation in C#, `StringBuilder` stands out as a more performant choice in complex or frequent string manipulation situations. By carefully understanding the tradeoffs and using `StringBuilder` appropriately, developers can write efficient and robust applications that gracefully manage strings and memory usage.
Efficient Substring Replacement in C# A Deep Dive into the Replace Method - Optimizing Replacements with Dictionary-Based Approaches
When dealing with multiple substring replacements in C#, a dictionary-based approach can offer a significant performance boost. This method leverages the speed of dictionaries to map each substring you want to replace to its corresponding replacement value. This eliminates the need for repeated, potentially costly, linear searches through the string. Instead of repeatedly searching and replacing substrings one by one, you can process all replacements in a single pass through the string, using the dictionary to look up each replacement efficiently. This reduces the amount of work the CPU has to do and can lead to lower memory use, especially when handling large numbers of replacements.
As developers aim for optimized string manipulation, understanding how dictionary lookups can streamline the replacement process is important. This approach can make your code not only faster and more efficient but also potentially easier to understand, as it allows for more flexible string handling. By applying these strategies, developers can create more robust and responsive applications, particularly in situations with complex string transformations or large input data.
1. **Dictionary Lookups for Speed**: Employing dictionaries for substring replacements can significantly boost performance because looking up a value in a dictionary usually takes a fixed amount of time, regardless of the dictionary's size. This is especially handy when you need to make many different replacements since it quickly narrows down where to search.
2. **Processing in One Go**: When you need to swap out many substrings, using a dictionary to map old substrings to new ones lets you do it in a single pass through the input string. This is a big difference from using `String.Replace` multiple times, where each replacement happens one after the other, potentially making your code slower and less efficient.
3. **Managing Overlap Concerns**: Defining replacement patterns in a dictionary lets you dictate the order of replacements, which can help prevent issues when substrings overlap. This helps avoid the confusing situations where one replacement changes the location of a substring that you're trying to replace later.
4. **Adaptable Replacement Rules**: A dictionary-based approach offers the ability to change replacement criteria while the program is running. This flexibility can be very helpful when implementing complex logic for substitutions without needing to write overly complex code.
5. **Memory Efficiency**: While dictionaries themselves use a bit of memory, the benefits often outweigh the cost. Since you're creating fewer intermediate strings during replacements, this method can actually save memory, particularly when doing a lot of string manipulation.
6. **Streamlining with Tuples**: Using tuples to store both the search and replacement strings inside the dictionary can simplify how you set up the dictionary. This makes the code easier to read and also helps you write faster and cleaner code, especially when you need to make changes to your replacement configurations.
7. **Thread Safety is Key**: When using dictionaries for replacements, it's important to think about what happens when multiple threads try to access the dictionary at the same time. Dictionary operations aren't inherently safe for threads, so if your application uses multiple threads, you'll need to use synchronization techniques to prevent errors.
8. **Benchmarking for Optimal Choice**: It's vital to test the performance of dictionary-based replacements versus more traditional methods. By measuring performance differences, you can get a better idea of when dictionaries offer the biggest benefits and when they might not be the best choice.
9. **Reduced Garbage Collection Burden**: Because you're creating fewer temporary strings with dictionary-based replacements, the garbage collector in your application has less work to do. This can make your application run smoother, particularly in programs that run for a long time, where memory fragmentation can become a performance issue.
10. **Handling Large Data Well**: As the amount of data you're working with increases, the advantages of dictionary-based replacements become more pronounced. Their ability to manage large and diverse lists of substitutions without a significant decrease in performance makes them a very appealing option compared to older string manipulation techniques.
More Posts from aitutorialmaker.com: