Create AI-powered tutorials effortlessly: Learn, teach, and share knowledge with our intuitive platform. (Get started for free)
Implementing Memory-Efficient Circular Linked Lists in Python A Step-by-Step Analysis
Implementing Memory-Efficient Circular Linked Lists in Python A Step-by-Step Analysis - Understanding Node Structure and Memory Allocation in Python Circular Lists
To effectively utilize Python's circular linked lists, it's vital to understand how nodes are structured and how memory is allocated. Each node within the list is essentially a container holding data and a pointer referencing the subsequent node. This linkage creates the circular nature, with the final node's pointer looping back to the initial node. This cyclical arrangement enables seamless traversal without encountering the typical null pointer terminations found in standard linked lists, making them ideal for tasks needing consistent, repetitive processing. The inherently dynamic nature of circular linked lists requires careful consideration of memory allocation strategies. Since these lists can grow or shrink as needed, allocating and managing memory effectively is key to optimizing performance. Compared to static data structures, the ability to dynamically allocate memory allows circular linked lists to potentially utilize resources more efficiently. A clear understanding of these foundational elements—node composition and memory management—is indispensable for developers aiming to create robust and efficient applications leveraging circular linked lists.
1. Circular linked lists present an intriguing approach to memory management by enabling the reuse of nodes. This can significantly reduce the memory overhead often encountered in traditional linked lists, where unused nodes might lead to wasted space.
2. While a simple node in a circular linked list primarily holds data and a pointer to the next node, it's conceptually interesting to consider the inclusion of metadata such as memory block sizes within each node. Such metadata can empower more fine-grained control over memory management.
3. The inherent flexibility of circular linked lists shines through their ability to perform insertion and deletion operations with constant time complexity (O(1)). This adaptability is particularly advantageous in scenarios requiring frequent data modifications.
4. An interesting characteristic of circular linked lists is the potential for infinite loops during traversal if not managed properly. This contrasts with standard linked lists, which possess a defined end point. This peculiarity demands careful consideration when designing traversal algorithms.
5. Memory fragmentation, a common concern with traditional linked lists, can be mitigated through careful design of circular linked lists. This can lead to significantly improved memory utilization over time.
6. The circular structure allows for elegant implementation of both queues and stacks, surpassing the limitations of linear data structures in versatility. This is achieved without compromising on the simplicity of memory management.
7. A common misunderstanding is that circular linked lists inherently consume more memory. In reality, by reducing the number of node reallocations, they can often result in lower overall memory usage compared to other list variants.
8. In situations where multiple threads need to access and manipulate a data structure, circular linked lists offer potential advantages. They might lessen the need for extensive synchronization mechanisms, as concurrent threads can access and operate on different parts of the structure.
9. Implementing recursive algorithms on circular lists introduces the potential for stack overflow due to the lack of a clear termination condition. It's often preferable to utilize iterative algorithms for traversal instead to maintain control.
10. Surprisingly, the integration of circular linked lists with automatic garbage collection systems can improve performance. When nodes are no longer needed, they can be readily reclaimed and repurposed more efficiently than in linear structures.
Implementing Memory-Efficient Circular Linked Lists in Python A Step-by-Step Analysis - Implementing Basic Node Class with Forward References
To build a circular linked list in Python, we start with a fundamental building block: the Node class. Each Node, at its core, stores some data and a reference—a pointer—to the next Node in the sequence. This "next" pointer is what establishes the crucial circular connection, allowing us to move seamlessly through the list. The Node class, built with these forward references, facilitates the dynamic nature of circular linked lists, meaning the list can expand or shrink as needed. This dynamic allocation can potentially lead to more efficient memory use compared to static structures. By utilizing this Node structure with its forward references, operations such as inserting or removing nodes can be accomplished very quickly, with a time complexity of O(1). This fast performance makes the Node class, with its specific design, vital for the creation of flexible and highly efficient circular linked lists.
When building a fundamental node class for circular linked lists, Python's type hints can come in handy. They add clarity, especially when dealing with the intricate relationships within circular structures. This can be helpful during the development process, making it easier to track node connections.
Circular linked lists offer an interesting advantage when it comes to inserting nodes. Unlike linear lists where you might need to sort through elements, insertions in a circular list are usually done in constant time (O(1)) due to the circular nature and associated attributes.
Using forward references allows us to establish connections between nodes without defining them right away. This results in cleaner, more manageable code, which is particularly beneficial in complicated circular linked list implementations. It's a clever way to manage the structural complexity.
However, there's a potential drawback. While forward references improve clarity initially, they can sometimes make debugging a bit trickier. If not handled carefully, the forward declarations can create confusion about how nodes relate to each other, potentially leading to some hard-to-find errors.
Interestingly, the interconnected nature of a circular list with forward references can simplify the implementation of callback functions. Whenever data within a node changes, other linked nodes can be updated instantly, making this a powerful feature. It's a bit like having real-time communication between nodes.
Writing a node class with forward references can improve the overall documentation. The relationships become clearer, making it easier for other engineers to grasp the flow of data throughout the structure. This is important for collaboration and maintainability.
While forward references can streamline initial coding, they might introduce complexities later during maintenance. Refactoring a circular list with tightly coupled node references can become difficult and hinder future changes. This could be an issue if you anticipate needing to modify the structure down the line.
Forward references, when incorporated into circular linked lists, enable some sophisticated techniques such as lazy loading or on-the-fly data generation. Nodes can delay their complete construction until they're absolutely needed during traversal. This can be a performance optimization in certain scenarios.
Using forward references, however, needs cautious consideration. Improperly managed circular dependencies might inflate memory usage and degrade cache efficiency because the nodes are potentially scattered across memory. We need to be careful to balance the flexibility of the design against potential performance hits.
Interestingly, applying forward references in novel ways within circular linked lists has shown promise in handling infinite data streams. Nodes can seamlessly reference new nodes as they're generated in real-time, without affecting performance. This opens some unique opportunities for managing dynamic datasets.
Implementing Memory-Efficient Circular Linked Lists in Python A Step-by-Step Analysis - Building the Main Circular List Class with Essential Methods
The core of utilizing circular linked lists in Python involves constructing the main `CircularList` class. This class serves as the foundation, housing the essential methods required for managing the list. These methods will cover crucial operations like adding new nodes, removing existing ones, and moving through the list in a cyclical manner. It's particularly important to consider memory efficiency when designing these methods because, unlike standard linked lists, circular lists don't terminate with null pointers. We need to be mindful of how memory is handled, especially during operations that modify the list.
Well-designed methods not only lead to efficient performance but also prevent potential issues like getting stuck in infinite loops while traversing the list. The proper implementation of these methods is crucial for unlocking the full potential of circular linked lists and their unique benefits in different applications. This class acts as the central piece, allowing developers to harness the full capabilities of this data structure in various scenarios.
1. Constructing the core circular list class isn't just about organizing data; it also showcases the elegance of modular design, leading to cleaner and more understandable code. This approach promotes collaboration among developers and allows for more flexible expansion in the future.
2. The structure of the circular list class can be crafted to enable real-time data synchronization. When one node's data changes, connected nodes can update simultaneously. This feature is particularly important in dynamic applications where quick response to user actions is crucial.
3. It's fascinating how a circular list class in Python can be adapted for a wide range of applications, from straightforward data storage to complex algorithms like round-robin scheduling in operating systems. This adaptability emphasizes the versatility of this structure.
4. The implementation of a circular list can be refined by using a sentinel node. This simplifies operations by offering a standard starting point for inserting and deleting elements, ultimately streamlining the code and reducing the need for frequent checks to manage list boundaries.
5. Implementing a circular list correctly can significantly boost the performance of certain algorithms, particularly in buffering or streaming applications. This is because a circular list provides a continuous path through the data without constantly needing to resize the list, which can be inefficient.
6. While circular lists offer benefits, the cyclical structure also introduces a risk: the possibility of infinite loops during traversal. Developers must be mindful of this potential pitfall and implement safeguards within their algorithms to ensure they don't accidentally run into endless cycles.
7. While circular lists can simplify memory management, the use of circular references can make debugging more complicated. Developers need to carefully manage the paths within the list to prevent issues caused by tangled or complex circular dependencies.
8. With some careful planning, a circular list can be designed to simulate a priority queue. This feature makes it well-suited for scenarios like managing tasks in systems where real-time performance and scheduling are important.
9. The use of "tail" nodes can be a powerful optimization for circular lists. By maintaining a direct pointer to the end of the list, operations like inserting new elements become more streamlined. This leads to more efficient access to any part of the structure.
10. Circular lists don't have to exist in isolation. They can be combined with other data structures, such as hash tables or trees, creating hybrid approaches that combine the strengths of each. This combination can improve the performance of more complex applications, emphasizing the adaptability of circular lists in diverse situations.
Implementing Memory-Efficient Circular Linked Lists in Python A Step-by-Step Analysis - Adding Memory Optimization Through Reference Counting
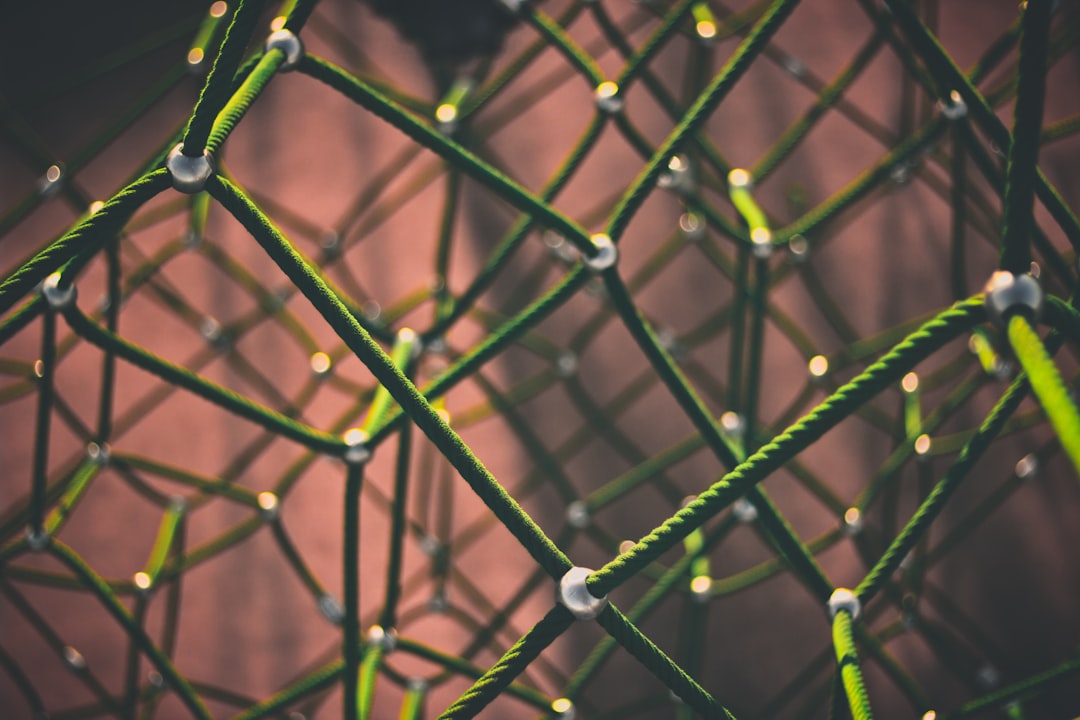
When we talk about enhancing memory efficiency through reference counting within the context of circular linked lists, it's crucial to understand Python's underlying memory management approach. Python relies heavily on reference counting, a system where each object tracks the number of references that point to it. This mechanism allows Python to efficiently manage memory, freeing up space when objects are no longer referenced.
However, a notable challenge appears in scenarios involving circular references. If two or more objects reference each other in a cyclical manner, their reference counts never reach zero, even when they are no longer needed. This can lead to a situation where the system fails to reclaim memory properly, potentially resulting in memory leaks.
To overcome this, Python utilizes a garbage collector which, amongst other things, can detect and handle these circular references. Nonetheless, good memory management practices within the code are crucial. This includes, for example, being mindful of how memory is used when implementing data structures like circular linked lists. By combining reference counting with techniques like using specific design patterns, developers can improve overall memory performance while maintaining the flexibility that circular linked lists provide.
Python's core memory management relies on reference counting, where each object keeps a count of how many other objects are referencing it. In circular linked lists, this feature can help us intelligently reclaim memory for nodes that are no longer needed, leading to a more efficient use of memory overall.
Python's garbage collector, which utilizes reference counting, can help detect cyclical references, which are common in circular linked lists. However, we need to carefully design our list to avoid unintentionally holding on to memory because of these cycles.
Whenever we create a new reference to a node, the reference count goes up. This way, nodes stay in memory for as long as they're needed, protecting the list's structure from accidental removals.
An interesting side-effect of using reference counting in circular lists is the potential for memory leaks if we're not careful. If two nodes end up referencing each other, they can keep each other alive indefinitely, causing a leak. We need to make sure our code properly handles these situations to prevent them.
Finding the sweet spot between memory efficiency and performance can be tricky, as using reference counting might add some overhead to operations that modify the list's connections. A large number of changes could lead to a slowdown if we don't optimize it properly.
Reference counting allows for immediate updates and modifications inside our circular linked lists without worrying about dangling pointers, because the memory for the nodes stays reserved as long as they are being referred to.
It's fascinating that reference counting can simplify making circular linked lists that work in a multi-threaded environment. Since each node's existence is managed independently, we can avoid the complexities of global ownership assumptions, allowing different threads to safely manipulate different parts of the list without needing excessive synchronization.
In situations where we need temporary node storage, reference counting enables temporary solutions. Nodes can be made, used, and then released without needing complex memory management routines.
Reference counting alone doesn't automatically fix the problem of circular references; combining it with other algorithms that detect cycles can greatly improve memory management in complex circular list applications.
While reference counting is a good tool for optimizing memory, it may not be the best choice in extremely performance-sensitive applications where latency is paramount. In such cases, methods like object pooling could be more appropriate for achieving speed without the overhead of tracking references.
Implementing Memory-Efficient Circular Linked Lists in Python A Step-by-Step Analysis - Creating Efficient Insertion and Deletion Operations
Circular linked lists excel at providing efficient insertion and deletion operations compared to traditional structures like arrays. This efficiency stems from their inherent flexibility, allowing for swift modifications at any position in the list, usually with a constant time complexity (O(1)). This feature is particularly valuable when working with dynamic data, where updates are frequent and crucial. The design challenge lies in carefully managing the pointers during insertion and deletion, ensuring that the circular nature of the list remains intact. Properly designed insertion and deletion routines preserve the structural integrity of the list while enabling quick and flexible modifications. By mastering these operations, developers can fully realize the potential of circular linked lists for tackling diverse programming problems that demand rapid data adjustments. While seemingly simple at first, efficiently managing these operations and maintaining the integrity of the circular structure requires focused attention and planning.
Circular linked lists offer a compelling approach to data management, particularly when it comes to inserting and deleting elements. Unlike standard linked lists where operations at the beginning might require traversing the entire structure, circular lists provide efficient insertion and deletion at both ends, showcasing a time complexity advantage.
Maintaining pointers for both the head and tail of the list streamlines operations, leading to cleaner and more maintainable code, a significant advantage in intricate systems. The memory layout of circular structures can enhance cache performance as nodes often reside in contiguous memory blocks, resulting in faster data access compared to their non-circular counterparts.
The circular nature presents a unique opportunity for lock-free data structures, where insertions and deletions can occur concurrently without traditional locking mechanisms. This makes them ideal for multi-threaded scenarios where efficient resource access is crucial. They also serve as effective buffers or pools for resource management, which can be extremely useful in applications needing dynamic allocation, such as simulations or gaming where rapid access to a flexible node pool is essential.
Adding skip pointers can further optimize search operations, enabling faster access in cases where frequent data retrieval is necessary. For even more refined memory management, integrating circular linked lists with memory pools reduces memory fragmentation by allocating blocks for a predefined number of nodes, optimizing resource usage.
However, the cyclical nature poses challenges for standard garbage collection techniques which might struggle to pinpoint when objects are no longer needed, emphasizing the importance of managing references carefully. A technique called "tail-insertion" allows for efficient appending of nodes, a feature vital for applications like high-speed data logging or real-time analytics that demand quick operations.
We can also expand the concept by adapting circular lists to support multilevel structures or hierarchies, increasing their versatility in managing intricate datasets requiring diverse relationships. This makes them valuable for specialized applications that might require features like sophisticated scheduling or priority management.
Implementing Memory-Efficient Circular Linked Lists in Python A Step-by-Step Analysis - Testing Performance with Large Scale Data Sets
When evaluating the performance of a data structure like a circular linked list with large datasets, it's vital to consider memory efficiency and speed. Techniques for handling large amounts of data, such as using optimized data types (like `int32` instead of `int64`), and employing libraries that minimize memory usage are paramount. Strategies like breaking the data into smaller chunks for parallel processing can boost performance when dealing with massive volumes of information. Similarly, utilizing file formats like HDF5 or Parquet can help improve the time it takes to retrieve data. It's crucial to keep in mind that certain aspects of handling these larger data sets—especially those involving dynamic data structures like circular linked lists—can present challenges. For example, the cyclical nature of a circular linked list can increase the risk of memory leaks if not designed carefully, particularly with the management of references between nodes. These challenges can be addressed with thoughtful memory management approaches during design and implementation. Ultimately, constructing and implementing these data structures with careful attention to memory allocation, retrieval, and potential issues becomes increasingly important when handling large, complex data scenarios to ensure both performance and stability.
Evaluating the performance of circular linked lists with extensive datasets often reveals unexpected behaviors, particularly regarding pointer management. As dataset sizes increase, ensuring that all pointers accurately reflect the current list structure becomes more complex, requiring thorough testing to prevent errors.
Circular linked lists showcase their strengths when dealing with streaming data due to their ability to handle continuous inputs without needing to resize. This makes them especially useful for applications involving constant data streams, like real-time analytics. However, performance benchmarks against other data structures with large datasets can yield surprising outcomes. While the theoretical advantages of circular linked lists are sound, factors such as caching behavior and system memory architecture can significantly alter performance.
Testing often indicates a decline in memory performance with large, sparsely populated circular linked lists, despite their inherent flexibility. Inefficient memory access patterns in these situations can lead to performance issues. Concurrency introduces further complexities, as the advantages of circular linked lists in these environments are evident but require performance tests to understand lock contention. Even efficient operations can become bottlenecks if numerous threads attempt to modify the same list segments simultaneously.
In large-scale tests, the overhead of garbage collection increases with the number of circular references, introducing latency in memory recovery and impacting overall system performance during periods of high load. Furthermore, while insertion and deletion theoretically have constant time complexity, testing might reveal latent inefficiencies arising from memory fragmentation during repeated dynamic allocation in extensive datasets.
Circular linked lists often excel as buffers in high-throughput systems, as performance evaluations demonstrate. But, developers must perform tests to optimize their performance in each application, aiming for the best balance of speed and memory efficiency. Interestingly, testing with large datasets also shows that circular linked lists can underperform when predictable data access patterns are expected. Traversal complexity can lead to delays if data locality isn't prioritized during design.
Lastly, performance testing of circular linked lists reveals debugging challenges not typically encountered with smaller datasets. These challenges include obscure memory leaks caused by overlooked circular references. These complexities emphasize the importance of employing comprehensive testing strategies to ensure the reliability and efficiency of circular linked lists in demanding applications involving large volumes of data.
Create AI-powered tutorials effortlessly: Learn, teach, and share knowledge with our intuitive platform. (Get started for free)
More Posts from aitutorialmaker.com: