Create AI-powered tutorials effortlessly: Learn, teach, and share knowledge with our intuitive platform. (Get started for free)
Implementing Time-Limited Docker Container Execution with Python SDK A Practical Guide
Implementing Time-Limited Docker Container Execution with Python SDK A Practical Guide - Introduction to Docker SDK for Python and Time-Limited Execution
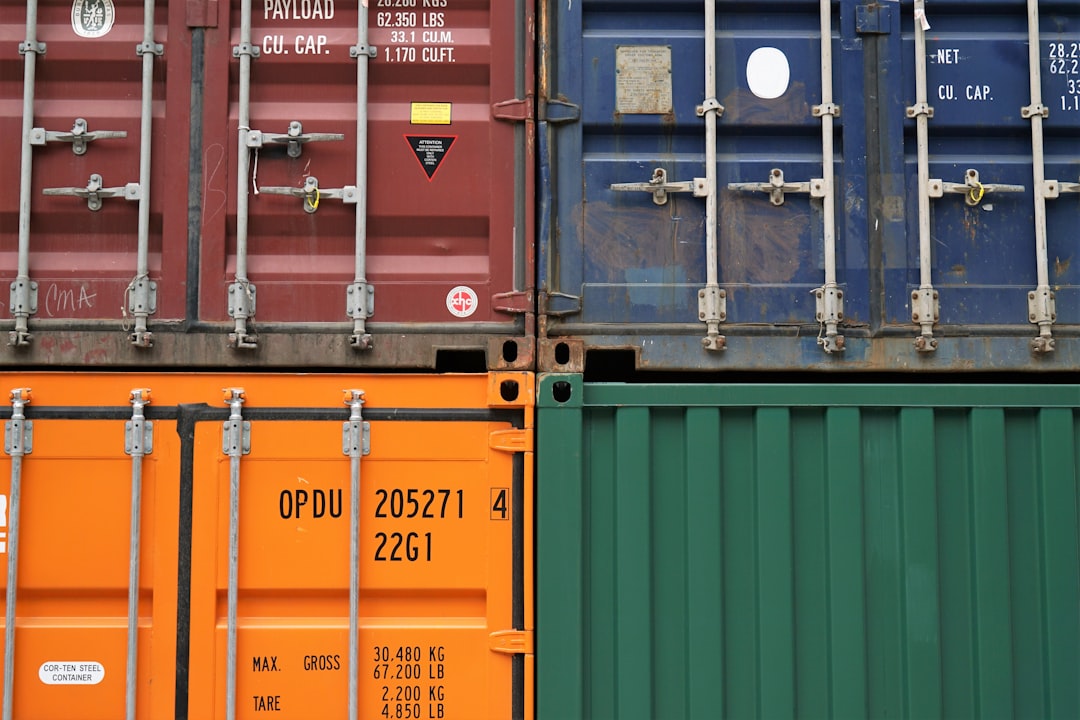
The Docker SDK for Python gives you a way to control Docker from your Python code. This is a powerful tool because you can run Docker commands, create and manage containers, and even work with Docker's Swarm feature (for managing groups of containers). This flexibility is important for automating tasks or building applications that work with Docker.
We're going to look at how to use the SDK to set time limits on how long your Docker containers can run. This is important because it helps prevent containers from running forever and using up too much of your resources. By understanding the features of the SDK and how to set these limits, you can ensure that your containers run efficiently and don't become a drain on your system.
The Docker SDK for Python offers a powerful way to interact with the Docker engine from Python code. This opens up a lot of possibilities, including running, managing, and controlling containers in a very programmatic way. It's like having a remote control for Docker, all managed by Python.
One particularly interesting aspect is time-limited execution. This essentially lets you set an alarm for your containers - a deadline. You can think of it as a way to prevent runaway processes from hogging your system. It's like setting a timer, which is not a feature baked into Docker itself, but rather something you build using the SDK and Python's own tools.
The SDK lets you define a timeout for your container, which is pretty convenient for things like batch processing. However, handling timeouts and container states can be tricky. You might want to consider custom signal handling to make sure your program can gracefully shut down or even retry if things go awry.
Overall, time-limited execution with the Docker SDK can be a valuable tool for managing containerized applications and testing their resilience in the face of unexpected interruptions. It's a bit like having a safety net for your code, ensuring it doesn't run amok.
Implementing Time-Limited Docker Container Execution with Python SDK A Practical Guide - Setting Up the Development Environment for Docker and Python
Setting up a development environment for Docker and Python is essential for building and managing containerized applications effectively. You begin by creating a Dockerfile that defines the base image, typically something like `FROM python:3.9`. This Dockerfile also contains instructions for building the Docker image for your Python application. This process helps to isolate your application's dependencies and environment from the host system. Running Docker containers for development is then made simple with commands like `docker run -d -p 8000:8000 `, which starts your container and maps ports for local access. This kind of setup is crucial for working with Python and Docker, allowing you to streamline your development process and manage your project's dependencies smoothly. For multi-container applications, using tools like Docker Compose and IDE extensions can greatly simplify setup and management. These tools create a seamless development experience, enabling you to build and test your projects more effectively.
Setting up a development environment for Docker and Python is a critical first step when working with Dockerized Python applications. A Dockerfile serves as a blueprint for creating a Docker image, which effectively packages your application with all its dependencies. Typically, you'd start with a base image like `python:3.9` in the Dockerfile, indicating the desired Python version. Once built, running a Docker container can be achieved with commands like `docker run -d -p 8000:8000 `, which maps ports for local access.
Docker's key strength lies in its ability to isolate environments, ensuring that projects don't conflict with each other due to incompatible dependencies. Developers can work directly with source files on their local machines, with changes reflected in the running container. Setting up virtual environments within Docker containers provides an extra layer of isolation, separating project dependencies from the host operating system.
There are some caveats, though. While Docker offers significant advantages, it's crucial to be aware of its limitations. Docker SDK for Python, while comprehensive, doesn't expose all Docker functionalities. For more intricate networking configurations, you might have to fall back on the Docker CLI. It's also crucial to remember that Docker introduces some overhead, albeit minimal compared to virtual machines. It's a trade-off, with containers being generally faster to start and execute, but with a slight performance penalty.
Further, Docker encourages version control for image tags in Dockerfiles. Using a "latest" tag can be risky as dependencies might change unexpectedly with image updates. It's essential to be mindful of versioning and to carefully select image tags to prevent compatibility issues.
Docker Compose emerges as a powerful tool when handling multi-container applications, enabling the management of interconnected services through a YAML configuration file. Testing within Docker is crucial, as Docker images can mimic production environments, mitigating the "works on my machine" issue. Using Docker effectively can significantly improve testing workflows and contribute to a more reliable and consistent development process.
Moreover, Python scripts can be leveraged throughout the Docker lifecycle for various tasks like image building or container management. This automation not only reduces human error but also can significantly contribute to seamless integration with CI/CD workflows, which is important for modern development processes.
Implementing Time-Limited Docker Container Execution with Python SDK A Practical Guide - Creating a Basic Docker Container with Python SDK
Creating a basic Docker container using the Python SDK involves writing a Dockerfile. This Dockerfile is like a recipe that tells Docker how to build your Python application into a container. You start by picking a base image, for example, `python:3.8`, which provides the basic environment needed to run your Python code. Then, you add commands to the Dockerfile like `COPY` to transfer your application files into the container and `RUN` to install any necessary dependencies. The Python SDK, in turn, provides tools for building, running, and managing these containers. It offers control over networking and even service handling, giving you a more programmatic way to interact with Docker.
However, even though the Python SDK streamlines working with Docker, it's important to realize it doesn't replace the Docker command-line interface (CLI) entirely. For more complex tasks, you may still need to use the Docker CLI. Despite these limitations, understanding how to build basic containers with the Python SDK is a valuable skill. It lets you deploy Python applications in isolated environments more easily and effectively.
The Docker SDK for Python provides a way to interact with Docker directly from your Python code. This offers a lot of flexibility and control, allowing you to perform operations on containers, images, networks, and even Docker Swarm services, all within a Python environment.
One of the interesting things you can do with the SDK is set time limits on how long a Docker container can run. This can be helpful if you're worried about containers running indefinitely and consuming too many resources. This ability to set timeouts lets you effectively manage containers by preventing them from becoming a drain on your system.
The SDK itself is fairly powerful, but building time-limited execution into your applications does require a bit of work. You can't just use Python's `time.sleep()` function to achieve this. You'll need to implement your own monitoring mechanism and possibly include custom signal handling to gracefully shut down a container if it reaches its execution time limit.
Beyond time limits, the SDK allows you to manage the lifecycle of Docker containers in various ways. For instance, you can modify configuration settings like environment variables or networking aspects during container runtime. This provides a way to tweak a running container's behavior without needing to rebuild the container image.
Furthermore, the SDK allows you to set resource limits on your containers. This is helpful if you're working with containers that are using a lot of resources like memory or CPU. By setting limits, you can make sure a single container doesn't take over all of your system's resources.
Docker's layered filesystem and its efficient caching mechanisms mean that Docker images are built and run quickly and efficiently. This is an advantage for developers since you can spin up and tear down containers as needed for rapid prototyping.
Overall, the Docker SDK for Python offers a lot of potential for managing your containerized applications. While the SDK provides access to a large set of features, you should be mindful that it doesn't map directly to every Docker command. If you find yourself needing more fine-grained control over your container's network setup, you might need to work with the Docker CLI. It's also worth keeping in mind that, while Docker offers a significant performance advantage over virtual machines, it does introduce some performance overhead.
Implementing Time-Limited Docker Container Execution with Python SDK A Practical Guide - Implementing Resource Limits and Constraints
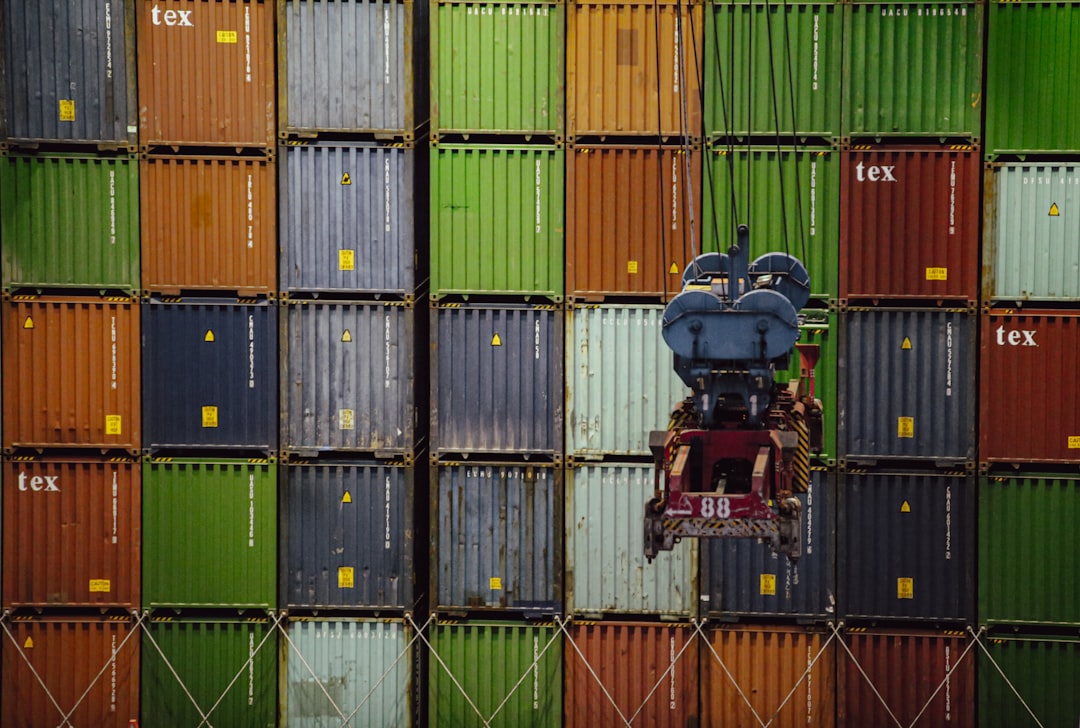
Implementing resource limits and constraints in Docker containers is vital for efficient resource utilization, particularly when running multiple containers. Docker provides mechanisms like Control Groups (cgroups), allowing you to set limits on resources such as memory, CPU, and even I/O operations. These limits prevent individual containers from consuming too much of the host system's resources, ensuring stability and preventing issues like Out-of-Memory errors. Without these constraints, a container could potentially consume vast amounts of resources, leading to system instability.
Docker Compose, a helpful tool for managing multiple Docker services, provides a convenient way to define resource constraints for different services within your application. This makes it easier to ensure that each service operates within its allocated resource boundaries.
Effectively implementing resource limits and constraints is crucial for managing a healthy and stable containerized environment. It's like setting up a fair system where each container gets a reasonable share of resources, preventing any one container from dominating and impacting the performance of other applications.
Controlling how much of your computer's resources a Docker container can use is essential for keeping things stable. Docker uses a kernel feature called "Control Groups" (cgroups) to manage this. It's like having a system of traffic lights for your container's access to things like CPU, memory, and disk space. You can use the Docker SDK for Python to fine-tune how much of each resource a container gets. For example, you can set a specific portion of CPU for a container, making sure it doesn't hog everything.
This kind of control is helpful for monitoring and understanding how containers perform in real-time. Tools like Prometheus and Grafana can show you exactly what your containers are doing, so you can adjust their resource limits if needed.
However, there are some things to watch out for. Limiting resources too much can slow down your containers. You need to find the right balance between control and performance. Docker Compose helps manage this by letting you set limits for multiple containers in a single configuration file.
By default, Docker containers have no resource limits, which is not ideal for production environments where you might have many containers running at once. The way Docker handles resources can also differ depending on whether you're running on AWS, Azure, or a local setup, so it's important to understand each platform's approach. And, just like with anything else, what works in development might not work perfectly in a production setting. It's vital to test and adjust your resource limits based on how your app behaves in a real-world scenario.
There can be a bit of overhead when you're setting hard limits. This can affect how quickly your containers start up and how smoothly they run, so make sure you're not going overboard with restrictions. It's also worth remembering that some apps might not be able to handle resource limits very well, potentially leading to crashes or lost data. In this case, robust monitoring and error handling become even more crucial.
Implementing Time-Limited Docker Container Execution with Python SDK A Practical Guide - Designing a Timer Mechanism for Container Termination
Designing a timer mechanism for container termination is critical for managing Docker workloads effectively, especially when you want to limit how long containers can run. This is important because Docker containers, by default, can run forever, potentially leading to resource exhaustion. A timer mechanism automatically stops containers after a set amount of time, ensuring they don't hog resources indefinitely.
You can create this timer using the Docker SDK for Python. It involves handling signals to gracefully shut down containers and setting up checks to monitor execution times. This custom timeout system allows developers to fine-tune how long containers run, making them more predictable and resilient. It's like having a safety net that prevents containers from running out of control.
Creating a system to gracefully terminate containers based on time is a valuable addition to your Docker management strategy. It helps to allocate resources efficiently and promotes stability by preventing rogue processes from disrupting other applications.
Designing a timer mechanism for container termination isn't as straightforward as it might seem. While we can use Python's timer functions, the precision they offer might not be enough. We need to consider scenarios where sub-second precision is essential, especially with time-critical applications.
Handling signals within containers is different from regular signal handling in applications. Since containers are isolated, managing the communication between the host and the container becomes tricky. This requires custom signal handlers that ensure smooth communication during termination.
A major challenge arises from the potential loss of unsaved state or data in memory when a container is terminated abruptly. We need robust data persistence mechanisms to avoid losing critical information.
Understanding Docker exit codes is crucial for debugging. For instance, an exit code of 137 tells us the container was killed because it exceeded memory limits, offering valuable insights into resource management issues.
In multi-container applications, implementing a timer for one container could impact its dependencies. Careful design is necessary to prevent instability across services.
Cgroups and timers might appear redundant, but they work together. Timers limit execution time, while cgroups control real-time resource allocation. This is crucial for ensuring a container doesn't hog resources even if it completes quickly.
Timer-based termination can lead to downtime. Therefore, a well-designed system requires robust fallback mechanisms for maintaining service continuity. Load balancing or traffic rerouting can be implemented for seamless operation.
Adding timers and signal management might add CPU overhead, especially with multiple monitoring threads or processes. It's crucial to measure this overhead to prevent performance degradation.
Time-limited container execution can significantly benefit CI/CD pipelines by preventing resource hogging during testing phases. This allows for efficient build resource usage and faster feedback loops.
Stateful containers, like databases, pose unique challenges. We need strategies for safely throttling or terminating these containers to prevent data loss and ensure state recovery across executions.
Implementing Time-Limited Docker Container Execution with Python SDK A Practical Guide - Best Practices and Security Considerations for Time-Limited Containers
Implementing time-limited Docker containers can be beneficial for managing workloads and resources, but security should be a top priority. You should treat container image security as paramount, as vulnerabilities or malicious elements in untrusted images can pose significant risks to your applications. Implementing Docker Content Trust and minimizing the connectivity between containers will bolster your defenses. Furthermore, limiting the privileges containers operate with is a fundamental best practice for mitigating potential security risks. Regular vulnerability scans and timely updates for your base images are crucial for ensuring a secure container environment. Consistent monitoring of container runtime behavior will help identify potential threats. A well-structured approach to security ensures that time-limited container deployments are secure and resilient without compromising safety.
Let's delve into the intricacies of making sure our Docker containers don't go wild with resource usage. While the Docker SDK for Python helps us manage containers, it's critical to remember that not all applications are created equal. Some are resource hogs, and others might need more careful handling to avoid unexpected crashes.
One key point is that monitoring resource consumption is not just about setting hard limits. It's about getting insights into how our containers are performing in real-time. It's like keeping an eye on a racing car's dashboard - we want to see if the engine is running smoothly or if it's overheating. If we see a container consistently maxing out its CPU or memory, it might be a sign of a bug or an inefficient design.
Cgroups are a powerful tool for resource management, and they go beyond just CPU and memory. They can also be used to control I/O, which is essential for applications that rely heavily on disk access, like databases. Imagine a container that needs to constantly read and write data; setting I/O limits ensures that other processes on the system don't suffer due to its high disk activity.
Gracefully terminating containers is all about using Unix signals correctly. We can't just yank the plug; we need to give the container a chance to clean up its act before it exits. This might involve saving any in-memory data or performing a final flush to a database. Properly implemented signal handling ensures that a container exits gracefully without causing issues for other applications.
Exit codes are not just numbers; they are clues to why a container terminated. An exit code of 137 tells us that the container died because it ran out of memory, giving us a valuable piece of information to debug issues or optimize our resource limits.
In multi-container applications, where we might have a bunch of interconnected containers working together, we need to think about how terminating one container might affect the others. If a database container abruptly exits, applications dependent on it might fail. We need to design our applications to handle these events gracefully, ensuring that data consistency and service availability are maintained.
Adding timers and signal handlers can add a bit of overhead, which could impact the responsiveness of our system, especially if we have a lot of containers running. It's important to monitor system performance and make sure that these features don't cause any noticeable slowdown.
Stateful containers like databases need special care. When a database container shuts down unexpectedly, we risk losing data. We need to make sure that data is properly backed up or that our databases can recover their state from a recent checkpoint.
CI/CD pipelines are a fantastic example of where time-limited containers shine. They can help prevent long-running build jobs from bogging down our development servers, ensuring that feedback loops remain fast and efficient.
By being mindful of resource limits, we can optimize performance. We can allocate more resources to applications that demand it, ensuring that they run smoothly without hogging resources from other containers. The way containers behave can also depend on where they are running. A container running on a cloud platform like AWS or Azure might behave differently than one running locally on our own machine.
Overall, the quest for efficient container management is an ongoing journey. By learning how to optimize resource usage, implement robust termination mechanisms, and handle stateful containers effectively, we can ensure that our Docker applications run smoothly and consistently, without becoming a drain on our resources.
Create AI-powered tutorials effortlessly: Learn, teach, and share knowledge with our intuitive platform. (Get started for free)
More Posts from aitutorialmaker.com: