Create AI-powered tutorials effortlessly: Learn, teach, and share knowledge with our intuitive platform. (Get started for free)
Mastering JavaScript Closures A Deep Dive into Function Scope and Data Privacy
Mastering JavaScript Closures A Deep Dive into Function Scope and Data Privacy - Understanding the Basics of JavaScript Closures
JavaScript closures are a fundamental concept for understanding how functions interact with variables from different scopes. At their core, closures are functions that remember and retain access to their parent function's environment, even after the parent function has finished running. This "remembering" capability is due to the closure's access to the parent function's lexical environment, which holds all the variables in scope when the closure was created.
This special access allows closures to encapsulate data, essentially creating a protective barrier around variables. This is incredibly useful for organizing your code and keeping sensitive information hidden from other parts of your program. For example, you can create 'private' variables that are accessible only through the closure, furthering the control over data access and preventing accidental modification.
Closures also play a key role in how data can persist beyond the lifespan of a function. Because they retain access to their parent's environment, closures can hold onto variables even when the parent function has completed execution, facilitating data persistence and enabling you to craft advanced patterns like function factories.
Essentially, understanding closures provides a deeper understanding of how JavaScript manages data and function execution. This insight is invaluable for writing more sophisticated and maintainable code, leveraging the power of function scope and controlled access to variables.
1. JavaScript closures allow functions to remember where they were born, so to speak, granting them continued access to variables within their parent function's environment even after the parent has finished running. It's this neat trick that powers up patterns like keeping data safe and building function factories.
2. It's a common belief that closures can lead to memory problems, but that's not entirely true. They're actually quite handy for handling operations that take time to finish, but if you're not careful and keep unnecessary references hanging around, you can indeed end up with a memory hog.
3. Creating functions in JavaScript can be a bit expensive, and closures add a bit more overhead since they need to hold onto references from their parent functions. So, if you're really focused on performance, understanding this trade-off can help you make smart decisions about where and when to use closures.
4. Closures aren't exclusive to JavaScript; they're a concept found in many programming languages like Python and Ruby. However, the way JavaScript lets us treat functions as first-class citizens really amplifies their potential, particularly in web development where they are great for events and asynchronous code.
5. How closures work can sometimes surprise you, particularly when dealing with loops. When closures grab loop variables, they might actually end up with the final value of the variable once the loop finishes. This behavior can deviate from what you'd intuitively expect about how variables should behave within a loop.
6. ES6 brought in the "let" and "const" keywords, which improved the closure game by giving us block-scoped variables. This change has given developers more control when using closures, leading to fewer of those weird bugs that often popped up with closures in older JavaScript code.
7. Memoization, a clever optimization trick that relies on closures, can seriously boost performance. By caching the results of computationally expensive functions, closures enable functions to spit out already calculated results – showing off how closures can be practical in real-world scenarios.
8. Closures help us create private variables, something that isn't naturally part of JavaScript's object-oriented design. By returning an inner function that handles a variable defined in the outer function, we can effectively build structures where data is hidden away from outside interference.
9. Understanding and using closures are crucial for managing asynchronous programming in JavaScript, particularly when dealing with callbacks and promises. Closures help keep the right context and state in order, preventing common errors that can pop up when things happen out of sync.
10. Troubleshooting closure-related issues can be tricky, mainly because the relationships between scopes and variable environments aren't always easy to see. This added complexity can create some tough-to-fix bugs if developers don't pay attention to variable lifetimes and closures throughout the development process.
Mastering JavaScript Closures A Deep Dive into Function Scope and Data Privacy - The Relationship Between Function Scope and Closures
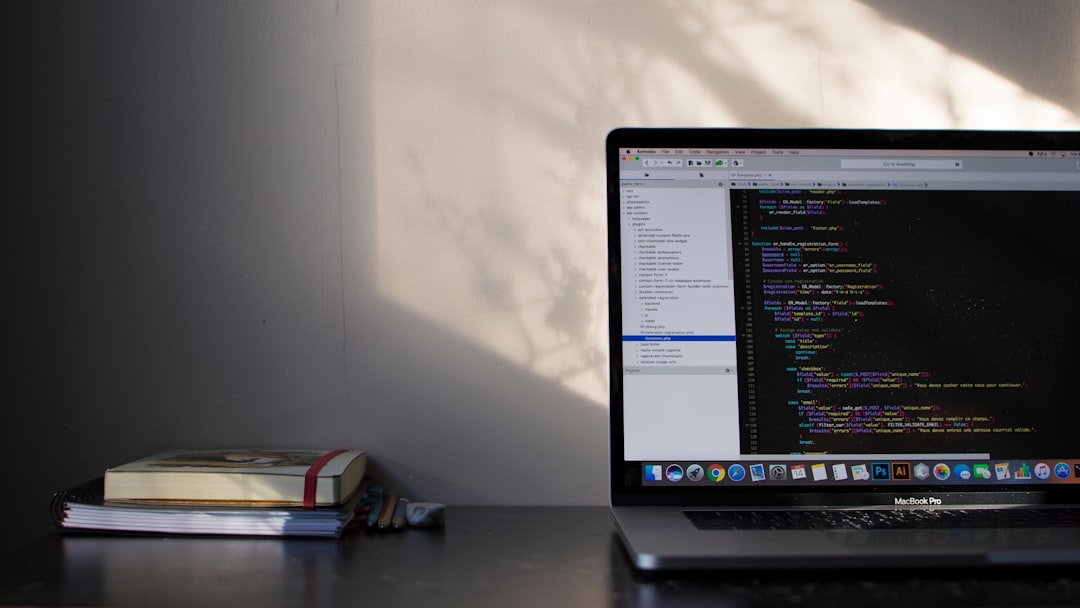
The core of closures in JavaScript is deeply intertwined with function scope, specifically how functions can access and preserve variables from their surrounding environments. A closure's ability to retain access to its parent function's scope, even after that parent function has finished, is a defining characteristic. This means a closure essentially "remembers" the variables it had access to during its creation. This ability to retain and control access to variables is a key element of what makes closures so useful. Not only can closures keep data private by creating a sort of 'hidden' layer around variables but they also enable a range of coding patterns such as function factories and event handlers.
The power of closures really becomes apparent when you deal with code that doesn't run linearly. In asynchronous scenarios, closures are essential for callback functions to be able to hold onto variables that were in scope when the callbacks were set up. However, this potent feature also has a hidden side – closures can sometimes behave in unexpected ways, especially when interacting with loop variables. This underscores the need for a deep understanding of how function scope works and interacts with closures to avoid errors and ensure that your code functions as intended. While the features and patterns closures unlock are beneficial to programming in JavaScript, developers should also be cognizant of the potential drawbacks, particularly regarding performance and potential pitfalls if not implemented mindfully.
1. When a JavaScript closure comes into being, it doesn't just grab variables from its parent function's scope – it grabs the whole lexical environment. This means any changes made to those variables, even after the parent function is done, are essentially 'remembered' by the closure until it's no longer needed.
2. Functions can accidentally create closures that live for a long time, which can be a problem with memory. If those closures keep holding onto large objects or data that's no longer used, it can cause the program to use more memory than it should.
3. The idea of a 'closure' can be confusing, especially for those new to JavaScript. It's not just about the variable's values when the closure is first made, but also how inner functions can alter those variables. This can make it harder to figure out why something's not behaving as expected.
4. Closures play a big part in building JavaScript modules in a modern way. They help group data and only let specific parts of the code access the functionality. This creates a cleaner structure and improves how the code is organized.
5. Closures really boost how functional programming is done in JavaScript. Functions can return other functions, which is helpful for techniques like currying and partial application. This often leads to more reusable and clear code.
6. In some cases, using closures too much in loops or other parts of the code that involve heavy computations can make things run slower. This is because they keep a 'snapshot' of the surrounding scope, which can add overhead.
7. The way closures work can lead to unexpected bugs in code where things happen asynchronously. For example, if a closure references a variable from a loop, and that closure runs long after the loop is finished, the variable's value might not be what the developer initially intended.
8. It's interesting that closures don't just capture the values of variables; they can maintain a state across multiple times the returned function is used. This can lead to some unique behaviors, such as 'accumulator' patterns often used in functional programming.
9. Closures are vital when dealing with events in JavaScript. They make sure that event callbacks always have access to the correct variables, keeping the context of what's happening even if the surrounding environment changes.
10. When working with others on a project, it's important to be mindful of how closures are used. If shared data within closures can be changed, it can cause unpredictable outcomes if multiple developers alter the same functions or variables without understanding how everything interacts.
Mastering JavaScript Closures A Deep Dive into Function Scope and Data Privacy - Implementing Data Privacy Through Closure Techniques
JavaScript's closure mechanism provides a powerful way to protect data by creating a sort of private space within your code. Closures, you'll recall, allow functions to hang onto variables from their parent function's environment even after the parent has finished. This means you can essentially hide variables and methods inside a closure, preventing them from being accessed or modified from outside. This is a key aspect of data encapsulation, promoting a more controlled and organized approach to how your data is handled.
This ability to create private data isn't just a neat trick; it forms the foundation for more complex coding patterns. Structures like function factories and modules can rely on closures to keep internal data private and ensure that only specific parts of your code can interact with them. This kind of control not only leads to cleaner code but also makes it easier to reuse and manage components of your program.
However, it's crucial to keep in mind that closures, while powerful, can also bring some potential problems. Their ability to hold onto data can lead to memory issues if not managed correctly. Also, the way closures interact with asynchronous code and loops can sometimes lead to behaviors that aren't immediately obvious. A thorough understanding of how closures behave and the potential trade-offs is vital for writing efficient, reliable, and easy-to-understand JavaScript programs.
1. Closures aren't just about hiding single variables; they can also shield entire data structures, letting us build complex private states that stay consistent across different functions. This prevents outside access without proper authorization, which is crucial for maintaining data integrity.
2. Using closures for privacy is a great way to build modules. Functions within a module can freely interact with the hidden data, but the outside world is kept at bay. This isolation improves encapsulation and helps avoid accidental clashes between variable names.
3. The real magic of closures shines in scenarios where code execution isn't always sequential, like asynchronous operations. When dealing with callbacks, closures let functions hold onto their environment, keeping the right context and data available even when things get interrupted.
4. It's interesting how closures can lead to some unexpected issues. When an inner function modifies variables in its outer function, it can get confusing to track the values of those variables, potentially causing some tricky bugs that are tough to spot.
5. Closures and loops can be a bit quirky. When creating closures inside loops, they sometimes end up grabbing the final value of the loop variable instead of its value at the specific iteration where the closure was made. This behavior can be counterintuitive, leading to surprising outcomes.
6. Modern JavaScript, with its `async/await` features, pairs extremely well with closures for asynchronous code. The combination results in cleaner, easier-to-read code, especially when handling errors. It's a testament to the enduring relevance of closures in the ever-evolving JavaScript landscape.
7. While closures are great at protecting data, they can make debugging a bit harder. When you have multiple nested functions and layers of closures, it can become difficult to follow where the data is flowing. This increased complexity can make troubleshooting more challenging.
8. Something to keep in mind about closures is the performance hit they can have. Even though they're really useful, developers need to be mindful of the extra resources they consume, especially when you have a lot of function calls or a large number of closures. In those scenarios, the performance might suffer as more memory gets used.
9. Closures play a pivotal role in functional programming styles within JavaScript. Features like currying and partial application, which increase code reusability, become much easier to implement with closures. This shift helps bring JavaScript closer to functional programming principles.
10. It's fascinating how closures can be used to create basic state machines in JavaScript. We can create functions that internally manage state and transitions, but the specific details of the state management remain hidden from the functions that use them. This hidden mechanism lets us interact with state without revealing the underlying complexities.
Mastering JavaScript Closures A Deep Dive into Function Scope and Data Privacy - Common Use Cases for Closures in Modern JavaScript
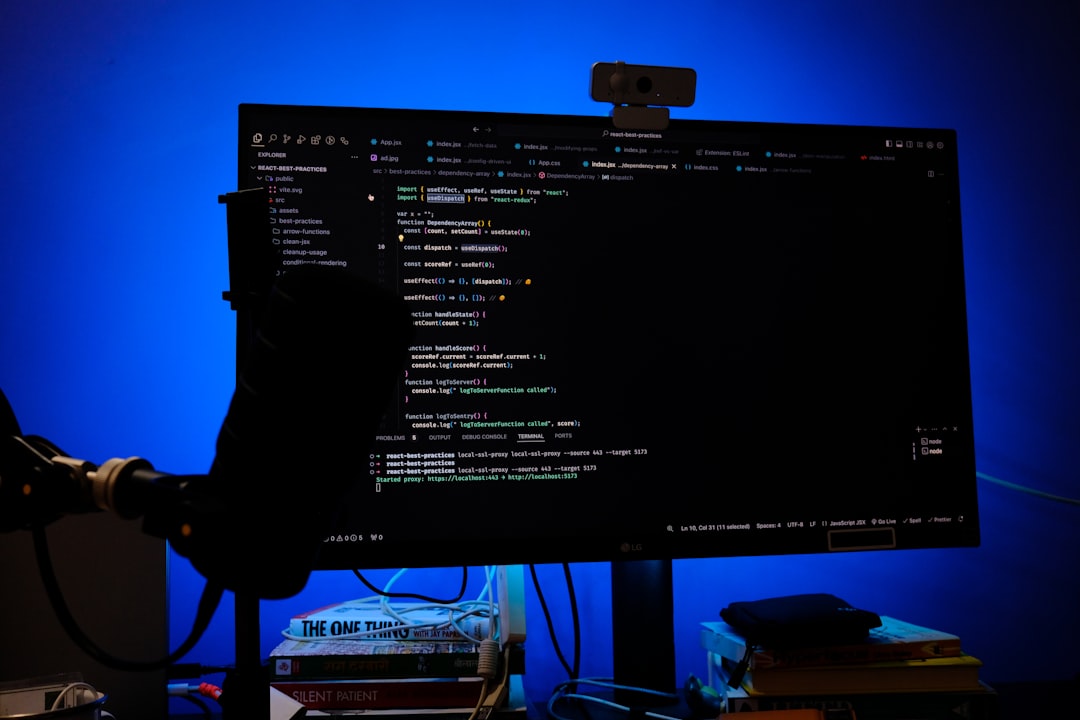
Closures in modern JavaScript prove quite useful in a variety of situations, contributing to both code structure and how things function. One key area is the creation of private variables. By restricting access to data within a function, closures enhance data privacy and maintain its integrity. Another critical application is their role in managing data throughout a series of function calls, which is especially important for asynchronous operations where maintaining context is paramount. However, there are some tricky aspects of closures. For example, problems can occur when using closures in loops or if they aren't handled carefully regarding memory usage, leading to unexpected outcomes or performance drops. If used thoughtfully and with understanding, closures offer efficient solutions for organizing complex JavaScript applications, but require care and knowledge to avoid unintended consequences.
1. Closures allow us to create functions that "remember" some of their arguments, letting us add more later. This "partial application" idea shows how closures make functions more flexible and reusable, which is quite helpful in JavaScript applications.
2. In frameworks where things react to changes, closures can simplify how we keep track of the state of parts of the application. Since closures can hold this state without making it accessible to everything globally, it keeps things tidier and easier to maintain.
3. When multiple things happen at the same time, closures help keep event handlers organized. By capturing the state of an event, closures ensure that each event works independently even if they overlap or their lifecycles are intertwined.
4. The way closures are structured, with nested functions and hidden scopes, can make debugging a challenge. It's like having multiple layers of states to follow. This is why developers need a solid understanding of how variable lifetimes and scopes work within closures.
5. If we need to limit how often a function is called, for instance, when someone is typing or resizing a window, closures are useful for "debouncing" events. By controlling the speed at which a function is called, we can prevent excessive calls and improve performance.
6. With "factory functions", we can use closures to produce functions that have unique characteristics. They act like little function factories! We can dynamically create specific behaviors without making everything available or creating global variables that might clash.
7. When we want to keep certain settings secret while offering a clean way for others to use them, closures can act as a buffer. We can hide the internal settings but allow public functions to control them, creating a nice level of abstraction.
8. There can be unusual results when closures and asynchronous code mix. A closure might hold onto a variable's reference instead of its current value at the time the closure was made. This can lead to outcomes that seem to go against what a developer expected.
9. In newer JavaScript frameworks, closures play a role in keeping track of component lifecycles. They make it possible to preserve component state during re-renders without relying on variables shared by everyone. This boosts the modularity of code.
10. By using closures, developers can tuck away intricate logic within functions. Think of custom iterators – they can deliver values over time while keeping their implementation hidden from the person using them. This approach generally results in cleaner and safer interfaces.
Mastering JavaScript Closures A Deep Dive into Function Scope and Data Privacy - Advanced Closure Patterns for Experienced Developers
"Advanced Closure Patterns for Experienced Developers" delves deeper into the practical applications of closures, moving beyond foundational concepts. Experienced developers can employ closures to create more sophisticated and elegant solutions. This includes crafting intricate patterns like function factories to generate custom functions, using memoization to optimize performance by caching results, and designing systems that hold onto and manage state. These advanced techniques exemplify how closures can expertly encapsulate logic and data, leading to more organized and manageable code. However, this added power comes with a need for caution. Developers need to be aware of potential challenges like unexpected behavior within asynchronous functions or potential performance bottlenecks from excessive closure use. A solid grasp of these more complex patterns empowers JavaScript developers to leverage the full capability of closures while proactively mitigating potential issues.
1. Sophisticated closure patterns let developers build functions that only run when needed, a strategy called lazy loading. This can give apps a performance boost by avoiding unnecessary calculations and making them more responsive.
2. Closures can be used to build a technique called event delegation, where a single event listener handles events for several elements within it. This can lower memory use by reducing the number of event listeners and make applications run faster.
3. The Module Pattern, which keeps code organized and clean, can be built using closures as its foundation. This pattern uses closures to create a hidden space for variables and methods, creating clear boundaries and interfaces.
4. With a clever use of closures, you can implement "currying," where a function is transformed into a series of nested functions. This not only makes functions more reusable but also enables partial application of their arguments.
5. However, while closures are great for creating private scopes, it can get tricky when you need to debug. Tracking errors through many nested functions can be difficult, making it challenging to pinpoint the source of unexpected behaviors.
6. Advanced closure techniques provide a way to create functions that remember past calculations, which is called memoization. This strategy can save a lot of processing time by storing the results of expensive function calls and reusing them. It's very useful for apps that require a lot of processing.
7. Closures can be used to encapsulate state in paradigms like React hooks, where keeping state information through updates is essential. This allows for keeping component logic predictable while encapsulating its state management, making it easier to understand and manage components.
8. A simple observer pattern can be built on the foundation of closures. This enables objects to maintain their private state and signal others when it changes. This way, developers can manage dependencies between parts of an app and improve communication between them.
9. The asynchronous nature of JavaScript execution can sometimes lead to unexpected closure behavior. When closures are created within loops, if they're not handled carefully, they can end up capturing the loop variable by reference, causing all closures to ultimately get the same final value rather than the value at each iteration.
10. Closures have an important role in functional programming techniques. They allow you to build combinators, functions that can take functions as inputs or create functions as outputs. This modular approach simplifies the development of complex functionalities by breaking them down into smaller, reusable pieces.
Create AI-powered tutorials effortlessly: Learn, teach, and share knowledge with our intuitive platform. (Get started for free)
More Posts from aitutorialmaker.com: