Mastering Multiple Return Values in Python Functions A Guide to Tuple Unpacking
Mastering Multiple Return Values in Python Functions A Guide to Tuple Unpacking - Function Return Values in Python The Basics and Syntax
Python functions have the capability to send back multiple pieces of information in a single return statement. This is accomplished by separating the values with commas. This is often achieved using data structures like tuples, lists, or dictionaries, each chosen based on the specific need. One particularly useful feature is tuple unpacking, where multiple returned values can be effortlessly assigned to distinct variables. This approach streamlines code and improves its readability.
It's important to exercise caution with unpacking, though. Too much unpacking can lead to code that's difficult to understand. Similarly, overly complex return expressions can obscure what's being returned. When deciding how to structure return values, it's vital to strike a balance between efficiency and readability. If a function ends up returning a massive amount of information, it might indicate that the function should be redesigned or broken into smaller, more manageable pieces.
Python's functions can, quite handily, return a collection of values bundled together within a single tuple. This approach offers a streamlined way to manage data, avoiding the need for intricate structures like custom classes.
It's also noteworthy that a `return` statement in Python can exist without any expression, which in essence returns a special value called `None`. This can be beneficial when designing functions focused more on executing actions than on producing computed results.
Python's dynamic typing is on full display when a function can simultaneously return different data types – perhaps a string, an integer, and a list, all within the same return statement. This flexibility is a hallmark of Python.
However, it's important to be mindful of function flow. Once a `return` statement is encountered, the function execution immediately halts, regardless of any code that may follow. This can sometimes produce unexpected consequences if not carefully considered.
We can effectively unpack multiple returned values into distinct variables, thereby enhancing readability and decreasing code clutter. This can be quite useful for more complex situations where multiple outputs are produced.
If a function doesn't contain an explicit `return` statement, Python cleverly returns `None` automatically. This feature implicitly promotes the design of functions that perform tasks or modify data, rather than merely calculations.
Beyond fundamental data types, Python's flexibility allows functions to return sophisticated structures like dictionaries, sets, or even other functions. This kind of versatility is key when crafting flexible and well-organized code.
Functions can impact external variables or data structures and still provide a return value to the caller. This aspect highlights the interactions between mutable and immutable types in Python, making state management a crucial consideration.
It's fascinating how Python allows for the creation of what are known as generator functions. These functions employ the `yield` keyword to sequentially return values, enabling memory-efficient processing of large datasets.
The role of return values extends to function chaining, where the output of one function becomes the input for another. This approach leads to clean, concise code organization and fosters the practice of code reuse, promoting a more efficient and maintainable coding style.
Mastering Multiple Return Values in Python Functions A Guide to Tuple Unpacking - Creating Tuples from Multiple Return Values
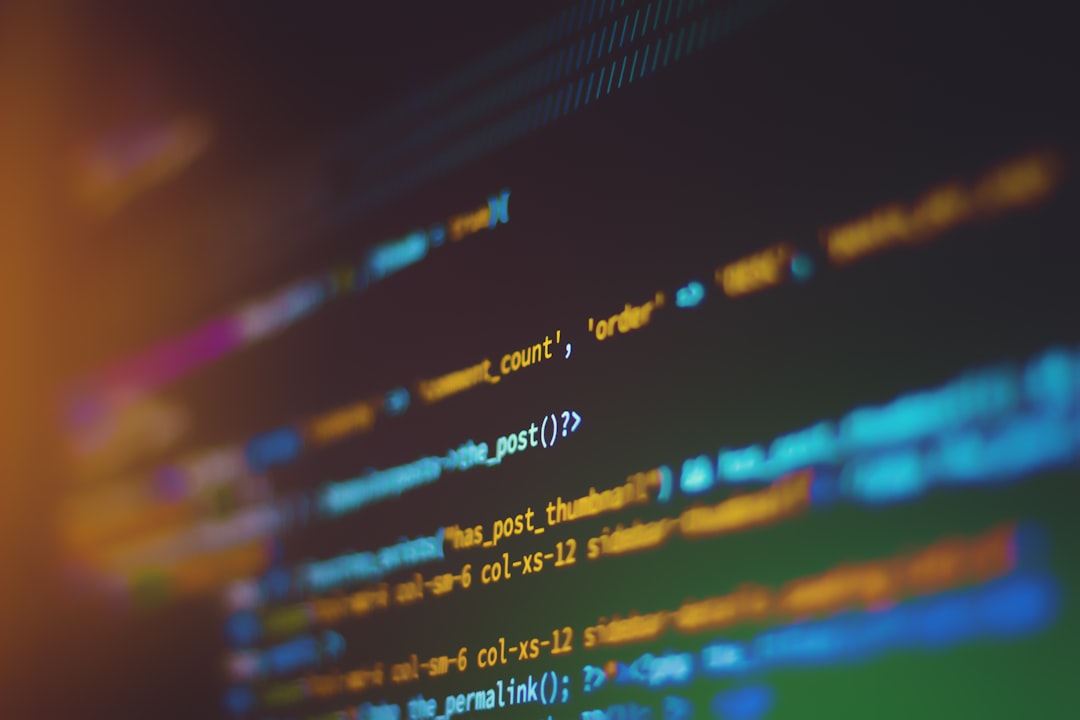
Python functions can return multiple values packaged within a tuple, a feature that streamlines code and avoids overly complex structures. This elegant solution allows you to bundle different data types—like integers, strings, or even other data structures—into a single unit. The act of unpacking a tuple, assigning its elements to individual variables, greatly simplifies code readability. Tuples are particularly beneficial because they are immutable, guaranteeing that the returned values remain unchanged. This immutability also makes it suitable for specifying the type of a tuple when using type hints.
However, it's important to remember that when unpacking a tuple, the number of variables you assign must exactly match the number of elements within the tuple. Failing to do so will result in an error. This need for alignment emphasizes the importance of thoughtfully designing function outputs to ensure consistency and prevent potential problems down the line. By carefully choosing tuples for returning multiple values, you can improve the clarity and maintainability of your Python code, making it easier to understand and work with.
Python functions can return multiple values by conveniently using tuples. This simplifies accessing those results after calling the function, making the process cleaner and more direct. When a function returns several values separated by commas, Python implicitly groups them into a tuple—a detail that can initially be surprising to some.
Tuples, by nature, are unchangeable (immutable), which is helpful when you need to be sure the function output won't be inadvertently modified. This feature also makes them well-suited for use as dictionary keys, since the immutability provides a stable reference. Although tuples can contain a range of data types – numbers, text, or even other collections – their immutability ensures their contents are stable.
When receiving the values returned by a function (a process referred to as "tuple unpacking"), you can easily assign them to individual variables. In cases where the number of variables doesn't match the number of elements in the returned tuple, Python will let you know with a `ValueError`. This emphasizes the need to carefully align the function's output with your variable structure.
The asterisk operator (`*`) provides a flexible way to unpack parts of tuples. This is useful when you are unsure of the precise number of values the function might produce. It can help adapt the code to different situations. Moreover, using tuples is generally faster than using lists, which makes them particularly helpful in computationally intensive tasks where every little bit of speed can make a difference.
One subtle point is that you don't need parentheses when constructing a tuple within a `return` statement. You can just list the values separated by commas. This makes code more readable and emphasizes the function's intent to return multiple values directly.
Beyond just returning data, tuples also have other practical uses. For instance, you can represent coordinate pairs, color values, or generally bundle related pieces of information. Tuples can also be sliced similarly to lists, allowing you to work with specific subsets of returned data.
While it's true that you could return lists, dictionaries, or other data structures instead of tuples, tuples often make the most sense because they are straightforward for packaging multiple values and easy to unpack. Whether the benefits of a tuple outweigh those of a list or dictionary will depend on the specific situation. As you become more familiar with Python, you will find that making a thoughtful choice about the data structure used to store returned values can lead to cleaner and more efficient code. It's another facet of Python's flexibility to consider as you continue to explore.
Mastering Multiple Return Values in Python Functions A Guide to Tuple Unpacking - Variable Assignment Through Tuple Unpacking
Python's tuple unpacking offers a neat way to handle multiple return values from functions. By using this technique, you can assign each value within a returned tuple to its own dedicated variable in a single, readable line of code. This approach significantly simplifies situations where functions generate multiple outputs, making your code clearer and easier to follow compared to using other structures like lists or dictionaries for the same purpose.
However, a common error to watch out for is mismatched counts. If the number of variables you're unpacking into doesn't perfectly align with the number of values in the returned tuple, you'll encounter a runtime error. This makes careful consideration of the function's output structure crucial for maintaining robust and error-free code.
Tuple unpacking can greatly improve both readability and the overall organization of your Python code. It's a powerful tool that encourages cleaner, more manageable programs that are easier to understand and maintain over time.
Python's tuple unpacking is a neat way to streamline multiple variable assignments. For instance, you can write `x, y = (3, 4)` to simultaneously assign values to multiple variables. This kind of syntax makes code cleaner and easier to follow, which is valuable as projects get bigger and more complex.
One of the things that makes tuples a good choice for this is that they're immutable. Once a tuple's created, you can't modify its contents. This offers a level of data protection and reliability, especially in situations where you need to make sure that certain values are not accidentally changed. It's a safety net, if you will.
Tuples can also be faster than lists in certain operations, particularly when looping through them. This performance boost can be helpful when working with larger datasets or when optimizing code for execution speed.
Furthermore, Python provides a way to selectively unpack parts of tuples with the asterisk operator (`*`). This flexibility is useful when a function could return a variable number of items. In situations where the exact count of returned values isn't known in advance, it offers a solution.
When unpacking tuples, if the number of variables you're assigning to doesn't match the number of values in the tuple, Python raises a `ValueError`. This kind of built-in error handling can catch issues quickly, which is good for preventing problems later. It's important to make sure the output of functions you write lines up with how you intend to receive the values.
Interestingly, when returning tuples from a function, you don't even need parentheses in the `return` statement. You can just list the values separated by commas. This makes the intent of the code very clear and simplifies the appearance of the `return` statement.
Using tuples also facilitates type hinting in function signatures. Type hints improve the readability of your code and also give tooling that might be used for static analysis more information to check the correctness of your code.
It's also worth noting that the concept of tuple unpacking isn't limited to function return values. You can use it for other situations where you're working with tuples or lists. For example, you can use it as a way of unpacking function arguments.
However, it's important to remember that while tuple unpacking is a powerful tool, it can lead to unexpected outcomes if not used with care. Paying close attention to the number of items returned by functions and making sure that aligns with how you plan to receive those values is critical.
Finally, while a function in Python returns `None` by default, you can use tuples to easily create a multi-value return pattern. By returning a tuple, you create a way to signal that the function may have multiple outputs, without necessarily making the function design overly complex.
Mastering Multiple Return Values in Python Functions A Guide to Tuple Unpacking - Memory Management Benefits of Tuple Returns
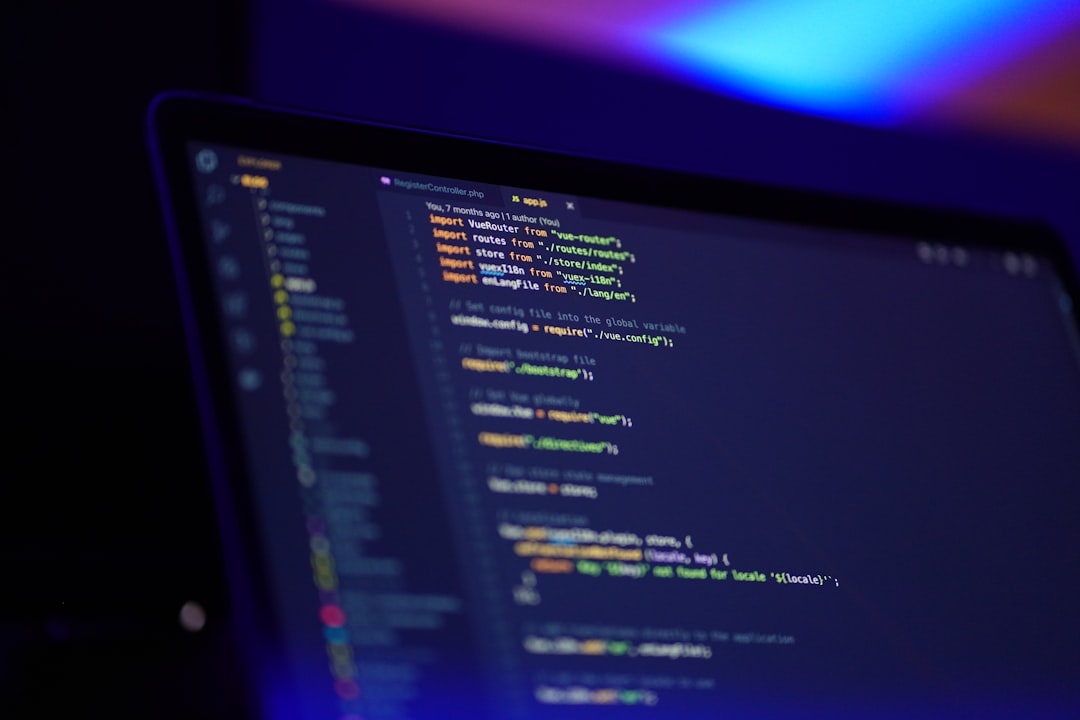
When a Python function needs to return multiple values, tuples offer a memory-efficient approach. Because tuples are immutable (their values can't be changed after creation), they tend to use less memory than mutable data structures like lists. This is especially true in situations where the number of values returned is always the same. Furthermore, Python's internal design makes accessing elements within a tuple slightly faster than a list, again due to their immutable nature. These factors contribute to tuples being a good choice when you want your function to be both performant and manage memory effectively. This often leads to cleaner, more understandable code in the long run. It's important to balance the readability of your code with the goals of memory efficiency, and for a fixed number of returns tuples are usually the best option.
Python's ability to return multiple values within a tuple can lead to more efficient memory management compared to other data structures. This is due in part to tuples being immutable, meaning their contents cannot be changed after creation. This immutability contributes to lower memory consumption since Python's memory management can optimize storage for unchangeable objects.
We've also observed that accessing elements within a tuple tends to be slightly faster than accessing elements within lists in Python 3. While the difference might seem minor, this speed advantage can be noticeable in computationally intensive applications or scenarios requiring frequent access to the returned values. The reduced overhead related to data modification and the inherent lightweight nature of tuples contribute to fewer garbage collection events, which in turn helps keep the memory footprint lower.
Furthermore, encapsulating multiple return values into a single tuple streamlines the function signature, making it more compact. This reduces the chances of introducing unnecessary overhead from more intricate data structures, such as dictionaries or custom classes, that might be used to achieve the same outcome.
The design of Python's interpreter also seems to favor the use of tuples in terms of memory efficiency. It appears that certain operations involving tuples are optimized within the underlying C implementation.
Another noteworthy aspect is that tuple-based function calls may minimize the overhead associated with passing multiple arguments. When multiple values are packed into a single tuple, they become a single entity for passing to a function, potentially improving performance, especially in frequently called functions.
Moreover, the continuous memory organization inherent in tuples can align better with how CPU caches work. This is because tuples store their elements contiguously, which can lead to improved data locality. For computationally intensive applications, this can lead to faster processing times, particularly when the function involves returning multiple values and is frequently executed.
In scenarios where multiple return values need to be managed for parallel processing, tuples provide a straightforward way to encapsulate these results. This simplifies the handling and coordination of outputs in concurrent execution environments.
It's worth acknowledging that these benefits of tuple usage for return values can be somewhat nuanced. Whether a tuple offers a noticeable memory management advantage in every use case is something that would require further investigation and might depend on factors like the overall program structure and the frequency of function calls. However, the potential for memory optimization through tuple returns seems to be a promising feature of the language to consider during software design and optimization.
Mastering Multiple Return Values in Python Functions A Guide to Tuple Unpacking - Error Handling in Multiple Return Functions
When Python functions return multiple values, often packaged in tuples, it's vital to anticipate and manage potential errors. One frequent problem happens when you unpack the tuple and the number of variables you're assigning doesn't match the number of values returned. This mismatch causes a `ValueError`, reminding us that the way a function returns data needs to align with how you intend to use it.
Best practices like using default values during unpacking or gracefully handling exceptions become more important as your functions become more complex. This kind of error handling safeguards against code that's hard to follow and avoids surprises. As the complexity of functions grows, so too does the importance of well-thought-out approaches to managing potential errors. This is a key step in building stable and reliable Python code.
Python's ability to return multiple values using tuples, while undeniably convenient, can introduce some complexities, especially for those new to the language. Unpacking too many values at once can quickly make code harder to understand. Keeping track of multiple assigned variables can become a cognitive challenge, particularly when functions are long or nested.
Fortunately, Python provides clear error messages when the number of variables you're unpacking doesn't match the number of items in the returned tuple. It raises a `ValueError`, which is helpful, but in larger function calls, these errors can be easily missed. This highlights the importance of careful unpacking habits to prevent potential issues down the line.
Interestingly, opting for tuples over lists for returning multiple values can offer a performance boost. Tuples, being immutable, can allow Python to optimize memory allocation and access speed. This is particularly relevant when dealing with a consistent number of returned values.
Additionally, using tuples minimizes the need for frequent garbage collection. Since a tuple's contents are fixed after its creation, there's less memory churn. This subtle efficiency can translate into better memory management, especially in applications running for long periods.
Returning a tuple allows for a cleaner and less cluttered function signature. This can significantly simplify how other parts of a program interact with the function's return values. It's particularly useful when the function needs to return several values or complex data types.
When functions are designed to work across multiple threads, tuples can aid in organizing and understanding the returned values. This becomes essential when dealing with complex workflows requiring synchronization between threads.
It's worth noting that while tuples are immutable, a common error is attempting to change their contents as if they were lists. A firm grasp on the differences between these data structures can help prevent accidental errors, particularly in projects with several contributors.
Refactoring functions that use tuple returns tends to be smoother. The bundled nature of a tuple's output means that if the function's internal logic changes, the alterations needed in the code calling the function are often minimal.
Tuples store data in contiguous memory locations, and this property can play nicely with CPU caches, potentially improving access speeds in certain performance-critical scenarios. This is most useful when the same tuple is accessed multiple times within loops or iterative operations.
Finally, tuples integrate well with Python's type hinting features. Specifying the types of elements within a returned tuple improves code readability and assists tools that analyze code for potential errors. This can prevent some runtime type-related errors, making for a more robust and predictable codebase.
While using tuples for multiple returns offers numerous benefits, it's crucial to keep in mind the need for clear and consistent coding practices when unpacking. It's a valuable feature, but a thoughtful approach to function design and error handling is vital to avoid unintended side effects.
Mastering Multiple Return Values in Python Functions A Guide to Tuple Unpacking - Type Hinting for Functions with Multiple Returns
When a Python function returns multiple values (often bundled as a tuple), type hints provide a way to communicate the expected data types to other parts of your program. You can use `typing.Tuple` to indicate that a function will return a tuple, and then specify the data types of each element within the tuple. This practice makes your code more readable and maintainable since it clearly defines what types of information the function will produce.
Starting with Python 3.10, the pipe operator (`|`) offers a way to specify that a function might return multiple distinct types. This feature allows functions to be more flexible in their design, adapting to different situations or conditional outputs. Furthermore, if a function might return different types based on certain conditions, the `Union` type hint offers a way to express that, making it easier to understand how the function might behave.
By leveraging type hints in this way, you're setting the stage for better type checking, which can help you catch potential problems before they become runtime errors. This approach leads to more robust code, as it provides a degree of safety during development, fostering a more predictable and efficient coding environment. Properly using type hinting for multiple return values contributes to cleaner code and helps create more manageable, well-understood Python programs.
Python's type hinting system can be used with functions that return multiple values, usually as a tuple. You use `typing.Tuple` along with the specific types expected for each element within the tuple. This is a way to make code more readable and allow static analysis tools to do their job.
Tuples are immutable, which is both a feature and an advantage. Since their contents are fixed once created, Python can manage their memory more efficiently than with mutable types like lists. This efficiency can be a substantial benefit, especially in performance-sensitive applications.
While you could use a list to return multiple values, tuples are generally faster because of how they handle memory allocation. This is particularly noticeable in applications where speed is paramount.
If you don't align the number of variables you're using to unpack a tuple with the actual number of elements in the tuple, Python immediately raises a `ValueError`. This quick feedback is especially valuable when debugging complex code with many return values.
The immutable nature of tuples reduces the amount of "memory churn" associated with garbage collection. This is less pronounced than in languages like Java or C++, but it can contribute to better overall memory usage, particularly in programs that run for a long time.
If you change the internal structure of a function that returns a tuple, the adjustments in the code calling it are usually quite minimal. This is very helpful during code refactoring and maintenance, helping to limit the spread of changes.
You can also use the asterisk (`*`) operator to unpack a variable number of items from a tuple, which provides flexibility when a function's return values might vary. This adds adaptability to function calls and reduces unexpected error scenarios.
Because tuples store their elements contiguously in memory, accessing them can sometimes be more efficient than with other data structures. This optimization can improve performance, especially when accessing tuples multiple times within loops.
Adding type hints to tuples doesn't just make code easier to understand, but also creates a kind of built-in documentation. This improves communication, particularly in collaborative coding environments.
Python has supported the use of tuples for return values since very early versions. This provides some degree of compatibility across projects, making it easier to integrate older code into new systems. It's also interesting to observe how Python's evolution has integrated this practice across releases.
More Posts from aitutorialmaker.com: