Create AI-powered tutorials effortlessly: Learn, teach, and share knowledge with our intuitive platform. (Get started for free)
Mastering the Fundamentals A Beginner's Guide to Low-Level Programming with Assembly and Machine Code
Mastering the Fundamentals A Beginner's Guide to Low-Level Programming with Assembly and Machine Code - Understanding the Basics of Computer Architecture
Grasping the fundamentals of computer architecture is a vital step for anyone venturing into low-level programming, especially when working with assembly and machine code. Computer architecture fundamentally concerns the building blocks of a computer system, including the core component, the CPU. The CPU, in turn, consists of the ALU, which performs computations, and the control unit, which orchestrates the execution of instructions. This interplay directly impacts how higher-level programming languages are converted into low-level machine code, ultimately influencing how efficiently programs run and utilize system resources. Furthermore, comprehending how data is represented within the computer and how it interacts with hardware like registers and the memory stack is crucial for optimizing code and addressing security vulnerabilities. In an increasingly complex software landscape, a thorough understanding of these fundamental concepts equips programmers to create effective software solutions and build efficient embedded systems. Given the continuous evolution of computing, mastering these underlying mechanisms lays the groundwork for a robust and future-proof understanding of software development.
Computer architecture's essence lies in the characteristics visible to software, like how memory addresses are used, the instructions a processor understands, and the size of data pieces. These aspects directly impact how programs behave.
At the heart of it all is the CPU, composed of the ALU (Arithmetic Logic Unit), responsible for calculations, and the control unit, which dictates the order of program execution. These two components work in tandem to make your programs function.
A critical aspect for programmers is understanding how code written in high-level languages gets transformed into machine-readable code. This journey involves compiling to assembly language, and eventually to a sequence of binary instructions that the computer's CPU can process directly. This transformation is essential to link the human-readable instructions of a high-level language to the machine's low-level operations.
For creating resource-conscious embedded systems, a good grasp of computer architecture's basics is crucial. Efficient code and smart data management become important considerations when designing systems with strict limitations on size, power, and performance.
Assembly language offers a closer view into the core operations of a machine compared to higher-level languages. It acts like a bridge, allowing programmers to scrutinize what's happening under the hood of software, gaining a more nuanced comprehension of how things work at the fundamental level.
Security concerns in coding often require knowledge of potential weak points that can be exploited at the lower levels. By understanding how these vulnerabilities manifest, programmers can develop more resilient code, even when using languages that abstract away much of the lower-level complexity.
When working with assembly language effectively, the foundations of data representation and low-level programming are indispensable. This is particularly true when targeting specific architectures like the x86 64-bit system which has become increasingly prevalent in computers.
Computer architecture courses often integrate practical exercises, assessments, and even AI-driven tools. This blended learning approach aims to help solidify understanding and provide opportunities to apply newly gained knowledge to realistic scenarios.
Hardware components like stacks and registers are fundamental pieces in how computers work, forming a core model of operation. They are essential components to grasp if you're considering starting your journey into assembly language programming.
There are numerous resources, from condensed crash courses to step-by-step guides, designed to gently introduce beginners to the foundational ideas of computer architecture and low-level programming. These resources can greatly aid your learning and help bridge the gap between abstract concepts and practical understanding.
Mastering the Fundamentals A Beginner's Guide to Low-Level Programming with Assembly and Machine Code - Introduction to Machine Code Binary Instructions
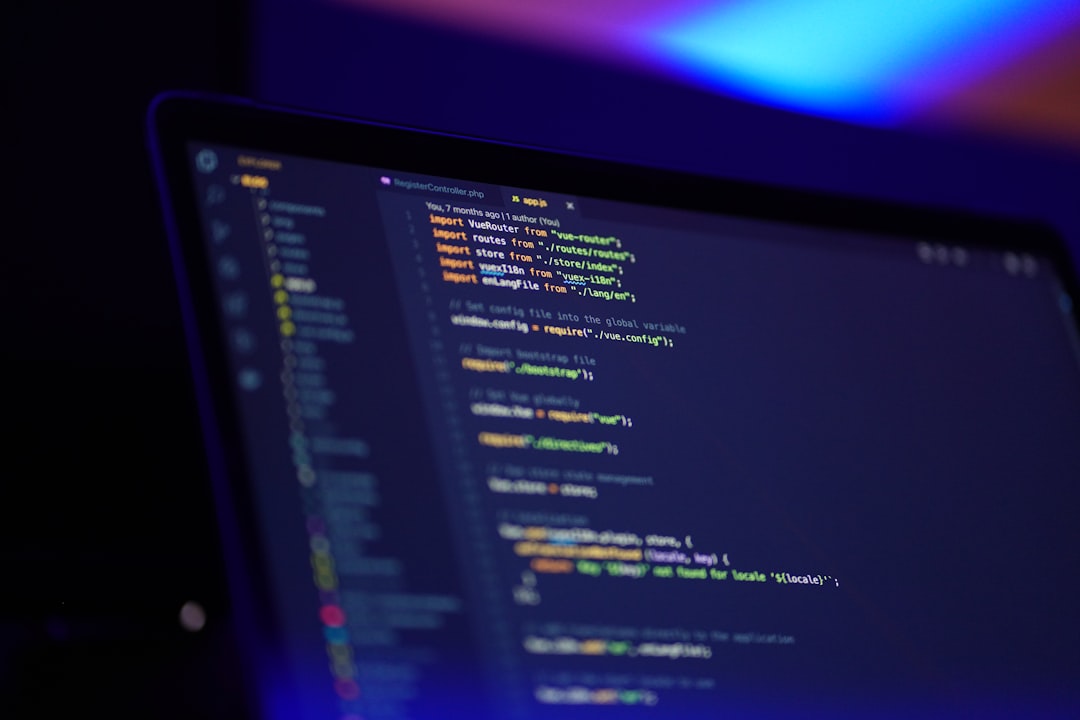
At the core of a computer's operations lies machine code, also known as machine language or native code. It's the most basic form of programming language, built from binary or hexadecimal instructions that the CPU can directly understand and execute. This is in contrast to high-level languages, which offer a level of abstraction and can often be used across various systems. Machine code and its closely related counterpart, assembly language, offer a way to directly interface with the hardware of a specific computer architecture. This direct interaction gives you the ability to influence performance and manipulate features that higher-level languages might not easily allow. It's crucial for anyone wanting to refine performance, improve security, or utilize hardware features unavailable in more abstract languages. This becomes especially critical in specialized architectures like x86, where mastering the associated machine code can strongly impact your software's speed and effectiveness. When you immerse yourself in the world of machine code, you gain a deeper understanding of how computers actually function, enabling you to craft software that's more powerful and efficient.
Machine code, the most basic form of programming language, is essentially a series of 0s and 1s that the CPU directly understands. This binary representation starkly contrasts with human-readable code, emphasizing the chasm between our intentions and how a computer executes them. Each CPU possesses a unique set of instructions, termed an Instruction Set Architecture (ISA), defining the operations it can perform. This architecture-specific nature means code designed for one processor might not function on another, a crucial consideration for developers seeking cross-platform compatibility.
The efficiency of these binary instructions often hinges on the size of their opcodes, representing the instruction's operation. Shorter opcodes generally translate to more compact code, a significant advantage for embedded systems and performance-critical scenarios. Furthermore, machine code leverages a variety of addressing modes, impacting how the operand, or data being processed, is interpreted. Immediate, direct, indirect, and indexed addressing are examples, each influencing how the CPU interacts with memory and data.
Within assembly language, we find directives like `.data` and `.text` which don't become machine code themselves but serve as organizational tools. These directives guide the assembler, enhancing the readability and maintainability of the code, which is a welcome aid when navigating complex low-level programming tasks. Certain instruction sets favor fixed-length binary instructions, simplifying the decoding process, while others opt for variable-length instructions to provide greater flexibility. The choice between these two approaches significantly impacts the performance and intricate design of the CPU.
Another intriguing aspect is endianness, describing the order in which bytes are stored in memory. In little-endian systems, the least significant byte comes first, leading to interesting outcomes when data needs to be exchanged between systems using contrasting endianness. It's also worth noting that while less frequently discussed, specialized debugging tools can help engineers analyze machine code. Disassemblers, for example, translate machine code back into assembly language, allowing for examination and troubleshooting without hindering program execution.
The stack, a pivotal memory region, plays a critical role in managing function calls, storing local variables, and tracking return addresses. However, the stack's actions, executed through machine-level instructions, can also introduce specific vulnerabilities like buffer overflows if not carefully managed. Interestingly, the human element surprisingly affects the efficiency of machine code. Factors like the way a programmer structures their code and employs comments can have an impact on how well optimizers can exploit patterns to improve the way instructions are processed. Essentially, well-structured assembly code can potentially lead to better performance.
Mastering the Fundamentals A Beginner's Guide to Low-Level Programming with Assembly and Machine Code - Assembly Language Syntax and Structure
Assembly language acts as a bridge, allowing programmers to communicate with a computer's processor in a way that's more understandable than raw machine code. It achieves this by using a structured syntax consisting of instructions called opcodes and associated operands. These operands can point to memory locations or specify data types, detailing the actions the CPU should perform. However, it's crucial to understand that each assembly language is designed for a specific processor architecture. This architecture-specific nature, while beneficial for efficiency, limits the portability of code written in assembly between different systems.
Despite the challenges of learning assembly due to its complexity and low-level focus, it provides programmers with a deep understanding of how computer systems operate. This understanding, in turn, enhances their abilities to debug code and optimize programs. It also helps in creating efficient software, particularly crucial for writing intricate, high-performance applications. Consequently, understanding assembly language syntax and structure is paramount for anyone looking to advance their skills in low-level programming, offering a pathway to writing more sophisticated software. It requires a certain level of dedication but offers profound insights into the fundamentals of how computer systems function.
Assembly language, being a low-level language, provides a human-readable representation of machine code tailored to a specific computer architecture. It acts as a bridge, allowing programmers to directly translate their instructions into the machine's native language, thereby facilitating a closer interaction between human intent and machine execution. The benefit of this direct link is the ability to generate faster and more efficient code compared to higher-level programming languages, making it valuable for applications where performance is critical.
Beyond speed gains, assembly language also offers a unique lens into the inner workings of computer systems. By understanding the language, programmers gain a profound appreciation of the hardware's nuances, which translates into improved debugging and optimization techniques. This understanding is particularly important when dealing with low-level issues or when targeting specific hardware features.
However, the syntax of assembly is inextricably linked to the hardware architecture. It's built around opcodes, which represent the core operations, followed by references to memory addresses and data types that these operations work with. Consequently, this tight connection makes assembly code not portable across different systems, as the set of instructions varies across different CPU families and generations.
Despite its architecture-specific nature, assembly language remains indispensable for developing system-level programs. In domains like operating systems, device drivers, and embedded systems where the need for direct hardware control is paramount, assembly shines. Also, when examining security-related code, understanding assembly language provides valuable insights into potential vulnerabilities that might otherwise be concealed by higher-level abstractions.
Interestingly, despite the initial impression of assembly being utterly complex and daunting, once you become familiar with the concepts within one specific architecture, adapting to others, such as ARM or RISC-V, is easier than expected. This is because they share underlying conceptual patterns. The transition involves mainly learning the specific instructions and syntax variations that are unique to each ISA.
To truly grasp assembly, hands-on practice is vital. This often involves working with a specific processor architecture like Intel's x86 family which has become ubiquitous in consumer computing. This practical experience not only reinforces the theoretical concepts but also allows one to experiment with the language's power and explore its limitations.
Ultimately, mastering assembly language is crucial for those aiming to deepen their understanding of computer systems, especially for those involved in the future of computing and those focused on lower-level programming tasks. Given that many systems and devices are designed with specific hardware in mind, it provides the means to interact with it in a more direct manner than higher-level languages would allow. Furthermore, given the continuing advancement and shift in the architectures themselves, this knowledge offers a stronger footing for navigating the changing landscape. While the realm of high-level languages provides a smoother and simpler pathway into software development, assembly offers a path to delve into the core functionality of computing, bringing about a deeper understanding that can aid in the creation of more efficient and secure systems.
Mastering the Fundamentals A Beginner's Guide to Low-Level Programming with Assembly and Machine Code - Memory Management in Low-Level Programming
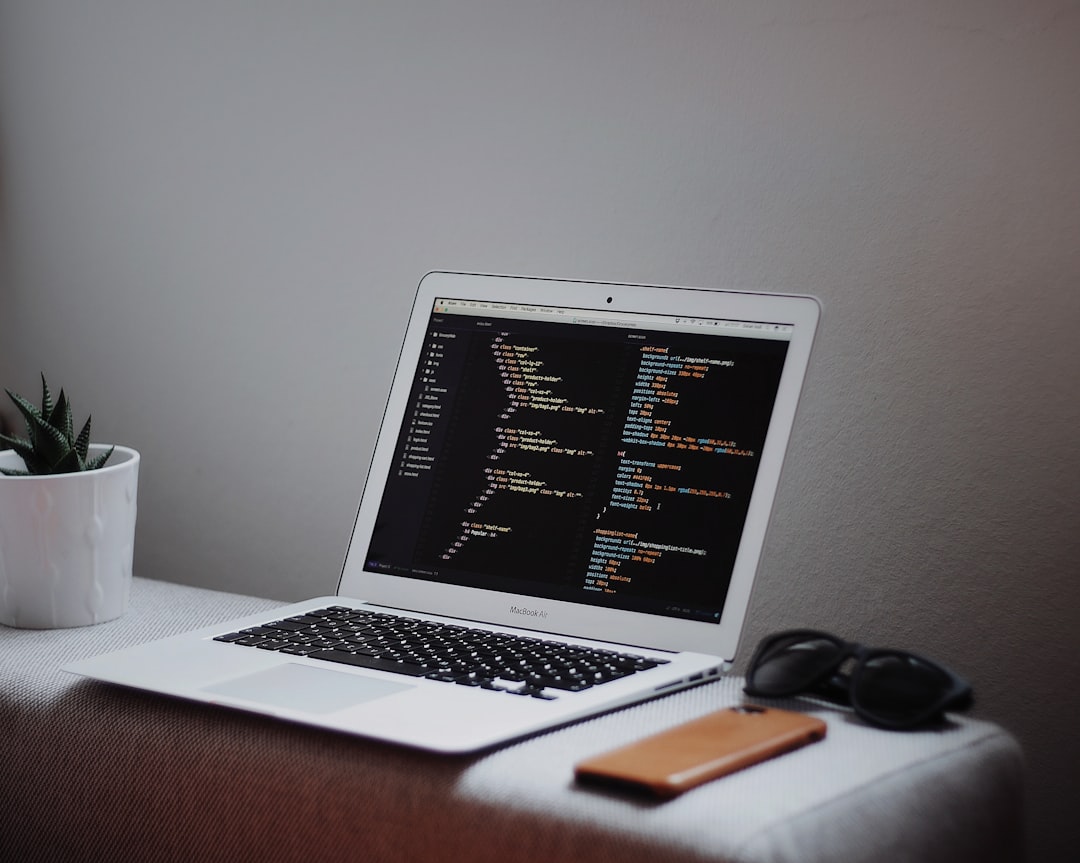
When working at the low level, memory management becomes a crucial factor influencing a program's efficiency and overall performance. This involves controlling how memory is allocated and released for various program components. Low-level languages, like C or C++, often use pointers to directly work with memory locations, offering precise control over where data is stored. However, this fine-grained control comes with a price. Programmers must make careful choices between gaining maximum performance and ensuring their code doesn't inadvertently cause problems like buffer overflows, where data spills outside its allocated space. Learning how memory works in this context isn't just about understanding specific language features; it gives you a much clearer idea of how more abstract high-level languages hide these details under the hood. This leads to a more comprehensive understanding of the bigger picture of system architecture and resource utilization. In essence, understanding memory management at this level strengthens your programming skills and enables you to create software that is not only fast but also robust and less likely to crash, especially in settings where system resources are limited, like embedded systems often found in devices and machines.
Memory management in low-level programming, especially when working with Assembly and Machine Code, is about directly controlling how the computer's memory is used. Think of it like being the conductor of an orchestra, ensuring that each section of the memory (like the string, woodwind, and brass sections) has the space and resources it needs without causing conflicts. This is a stark contrast to higher-level languages like Python or JavaScript, which often abstract away the complexities of managing memory.
Low-level languages, like C, provide a more direct line to the hardware, which offers the potential for creating finely-tuned, high-performance applications. However, this direct control also means that the programmer is responsible for ensuring that memory is properly allocated and freed, making memory errors more of a possibility if not handled carefully. Pointers, variables holding memory addresses, become your primary tool in this process. You can directly manipulate memory through them, but this power can also be a source of potential problems if not used correctly.
Learning how memory and the CPU work together is a fundamental skill that helps in writing more efficient programs and solving tricky problems across programming languages. You can get a more refined understanding of the underlying logic in more advanced scenarios, like debugging more difficult software malfunctions. Low-level programming concepts have a wider influence than just low-level coding itself. For instance, the design of garbage collection in higher-level languages evolved from these more basic notions of how to deal with memory management.
Memory management in low-level contexts can be a precarious balancing act. Performance often comes at a cost. The more control you have over the memory, the more likely it is that you'll create a more optimized application. On the flip side, this can easily lead to more memory-related bugs and errors if not handled correctly. These mistakes can have significant repercussions as errors or unexpected behavior ripple throughout the program.
One key reason to learn low-level concepts is to create new computer systems and devices. By gaining an intimate knowledge of how memory is handled, you can learn to design better operating systems, write better compilers, and develop the software that runs these future systems. This knowledge helps in avoiding some issues that even higher-level languages can be vulnerable to.
In the context of security, understanding low-level memory management is key. Many security vulnerabilities in higher-level languages have their roots in the problems that can arise in lower-level contexts. If you know the potential pitfalls of pointer arithmetic or stack operations, it's possible to code in higher-level languages in ways that mitigate these problems.
Assembly language, the human-readable version of machine code, has evolved substantially over the years. Each CPU has a specific instruction set, so you must consider that when designing code that you wish to run across different architectures. Furthermore, there is often a very intricate connection between the instructions and the machine's underlying workings.
The best way to learn these concepts is through doing. Beginners can often find the most value from working on smaller projects like writing an emulator. These sorts of endeavors can help you bridge the gap between theory and practice. While sometimes seemingly abstract, these core concepts ultimately provide a deeper understanding of how a computer works and are valuable for anyone seeking to create truly optimized software or work on the future of how computers are designed.
Mastering the Fundamentals A Beginner's Guide to Low-Level Programming with Assembly and Machine Code - Implementing Basic Algorithms in Assembly
Understanding how to implement basic algorithms in assembly is vital for programmers who want to fine-tune their code's performance and have a deeper understanding of how software interacts with the hardware. While assembly offers a much more fine-grained level of control compared to high-level languages, it requires a strong understanding of the machine's architecture and the specific syntax of the language. It's not easy, but it's quite rewarding. Translating simple algorithms like sorting or searching into assembly code provides a clear picture of how each operation connects to CPU instructions and memory management. When you master these fundamental implementations, not only will your code become more efficient, but you'll also develop the necessary knowledge to address more challenging programming tasks. This enhanced understanding leads to a wider range of problem-solving capabilities across different programming environments. As with other aspects of low-level programming, hands-on experience is vital. Experimenting with these algorithms helps reinforce concepts and prepare you for real-world use cases in system or embedded systems programming.
Implementing basic algorithms in assembly language offers a unique perspective on programming, primarily because of the direct control it affords over hardware. You can manipulate CPU registers and memory directly, leading to optimized performance, which is especially crucial in scenarios with limited resources like embedded systems. However, this intimate control also reveals the complexity of assembly instructions. Each instruction can translate into several machine operations, making optimization a nuanced task. Understanding this intricate relationship between instruction and execution is key to achieving the desired performance gains.
Debugging assembly code can be a formidable challenge, mainly due to its low-level nature. Unlike higher-level languages with more helpful error messages, debugging in assembly often involves cryptic bugs that are directly tied to CPU behavior, requiring a comprehensive understanding of the system's architecture. This is further complicated by the impact of endianness, which can cause unexpected behavior when implementing algorithms across different systems. What might function flawlessly in a little-endian system could yield incorrect results in a big-endian environment, underscoring the need for detailed knowledge of the underlying hardware.
Assembly language shines when it comes to performance-critical applications, as it often allows tasks to be executed faster than in higher-level languages. For instance, real-time systems, like those controlling aerospace operations, can see dramatic improvements from custom-tailored assembly code, minimizing delays and maximizing throughput. However, this direct link to hardware also introduces unique security concerns. Without a solid grasp of memory management at the low level, developers can inadvertently create weaknesses in security-sensitive applications through vulnerabilities like buffer overflows or stack smashing.
There's a tendency to think of assembly as a unified language, but the reality is more complex. Each CPU architecture has its own specific assembly language, meaning code written for one system isn't directly compatible with another. For example, code written in x86 assembly needs significant changes to run on an ARM system. This architecture-specific nature forces developers to tailor their implementations carefully. Furthermore, optimization can be tied to specific hardware features. Some architectures offer SIMD, where a single instruction can be executed on multiple data points simultaneously, making a huge difference in demanding computing tasks. This highlights the importance of being aware of these features when crafting assembly-based algorithms.
Learning assembly isn't without its challenges. The initial learning curve can be steep, but a surprising pattern emerges. Once the basic concepts are understood for one architecture, adapting to others becomes easier because they share fundamental operational principles. This prior knowledge can help speed up the process of learning new assembly languages. This is particularly relevant for real-time applications where strict timing constraints must be met. Systems like embedded controllers are a prime example, where assembly code provides a pathway to efficiently meet those stringent deadlines, a feat that can be challenging in higher-level languages. The combination of direct hardware access, performance benefits, and real-time capabilities makes assembly a compelling choice for applications demanding both efficiency and precision.
While high-level languages provide a more accessible path into software development, a thorough understanding of assembly language and its nuances can yield a much deeper appreciation for how computers actually work. This translates into improved optimization, better debugging capabilities, and a deeper understanding of the connections between hardware and software, ultimately leading to more secure and efficient code. In the ever-changing landscape of computer architectures, having a firm grasp of the fundamentals provided by assembly language can prove to be an invaluable tool for programmers navigating the complexities of modern computing.
Mastering the Fundamentals A Beginner's Guide to Low-Level Programming with Assembly and Machine Code - Debugging and Optimization Techniques for Assembly Code
Debugging and optimizing assembly code is essential for programmers who want to leverage the full potential of low-level programming. To effectively debug assembly, one needs to fully grasp how the CPU functions, allowing for the use of tools like GDB to set breakpoints and analyze the contents of memory. Optimizing assembly necessitates a deep understanding of the targeted architecture's instructions sets – such as ARM or x86 – and how these instructions interact with the hardware itself. This leads to writing code that is faster and uses resources more efficiently. While assembly programming can produce highly efficient programs, it also presents challenges such as issues stemming from the specific architecture being used, and obscure bugs that are not readily apparent in higher-level programming. Ultimately, becoming adept at these techniques enhances both software performance and a programmer's understanding of the complex link between software and hardware.
Debugging and optimizing assembly code presents a unique set of challenges and opportunities. The inherent ambiguity of low-level languages often necessitates a deep understanding of the CPU's inner workings to decipher errors. Unlike higher-level languages that often provide helpful error messages, pinpointing issues in assembly can be tricky, demanding a firm grasp of instruction execution and CPU behavior. It's a surprising fact that sometimes, having fewer assembly instructions can lead to faster program execution. This is because carefully crafted assembly can potentially leverage hardware features that higher-level language translations might miss, creating a path to enhanced performance.
Optimization within assembly is often tied to specific hardware characteristics. For example, processors that have SIMD instructions allow for the parallel processing of multiple data points within a single instruction, potentially greatly accelerating demanding computations. Understanding these features is vital when developing optimized code for certain applications. How data is stored within memory, also called endianness, can unexpectedly alter the behavior of algorithms implemented in assembly. A simple sorting routine might produce different results on a little-endian vs big-endian machine without proper adaptation. This is a stark reminder that the target architecture is always a core aspect when working with assembly code.
The fine-grained control that assembly provides over memory can be a double-edged sword. While this fine-grained control can boost performance, it can lead to security weaknesses like buffer overflows if memory management isn't handled diligently. Understanding this potential pitfall is essential when creating code for security-sensitive systems. It's worth noting that many of the modern features that higher-level languages provide, like garbage collection, have roots in the more fundamental low-level techniques of assembly memory management. This demonstrates a broader point; learning low-level languages can sometimes offer a fresh perspective on the design decisions made in other programming domains.
Assembly language isn't solely focused on sequential operations; it can leverage techniques like multi-threading or SIMD to introduce parallel processing. This duality offers a level of flexibility in tailoring programs for specific needs. This is particularly important when coding for real-time systems where strict timing deadlines are critical. In fields like aerospace or telecommunications, assembly can significantly enhance the ability to meet these demanding deadlines. A fascinating fact is that well-structured assembly code can potentially improve the efficiency of optimization processes. A structured approach enables optimizers to better exploit patterns within the code, leading to more efficient translated machine code.
While assembly can seem complex, the initial learning experience has a surprising element. Once you understand the core concepts of one CPU architecture, adjusting to a different one often becomes easier. This is because foundational elements of computer architecture remain consistent across different assembly languages. So while the initial challenges of learning are clear, the effort yields transferable knowledge across various ISAs. This means that in the constantly evolving landscape of computer architectures, having a foundation in assembly can provide a more flexible toolkit for tackling new challenges that emerge in hardware and software. It's clear that while higher-level languages offer a streamlined path into software development, the fundamentals of assembly provide a deeper appreciation of the interconnectedness of hardware and software, ultimately leading to more robust and performant code.
Create AI-powered tutorials effortlessly: Learn, teach, and share knowledge with our intuitive platform. (Get started for free)
More Posts from aitutorialmaker.com: