Python List For Loop A Step-by-Step Guide to Enumerate Function Implementation
Python List For Loop A Step-by-Step Guide to Enumerate Function Implementation - Understanding the Basic Syntax of Python Enumerate Function
The Python `enumerate` function offers a concise way to loop through iterables while keeping track of the item's position within the sequence. Its core syntax is `enumerate(iterable, start=0)`, where 'iterable' represents the data structure you're working with (like a list or tuple) and 'start' optionally sets the initial index value (it defaults to zero). During each iteration of a loop using `enumerate`, you gain access to two elements: the current index and the associated value from the iterable. This streamlined approach avoids the need for manually managing index counters, resulting in cleaner and more readable code compared to some other languages. You can use `enumerate` with a variety of iterable types, which adds to its usefulness in various situations. Ultimately, gaining mastery over this function can improve your Python coding efficiency and contribute to writing more robust, readable programs.
The `enumerate()` function within Python automates the process of indexing items during iteration, making code cleaner and more efficient than manually managing indices. You can even modify the starting index for specific situations, such as when you need to produce outputs that align with user expectations, for instance, by starting the index at 1 instead of the usual 0.
Interestingly, `enumerate()` generates an iterator called an 'enumerate' object, which is less often discussed. This feature is beneficial because it avoids creating a whole new list in memory when iterating over data. This is a potential memory saver when dealing with large datasets.
The output of `enumerate()` is a series of tuples, each consisting of an index and its corresponding item. This dual structure is well-suited to unpacking in a loop, leading to more streamlined and readable code.
However, despite its usefulness, a lot of newer Python programmers don't leverage the power of `enumerate()` enough. They often rely on traditional loops that include a range function and manual index management. These can result in more complex and harder-to-maintain code in the long run.
`enumerate()` shines when you're dealing with tasks involving data manipulation, for example, when working with CSV files or processing data records. Tracking the record or row number is invaluable for debugging and reporting issues.
In situations where many loop iterations involve keeping track of an index, using `enumerate()` can improve the execution speed of the program because it's optimized internally in Python for index-related operations compared to manual index management.
`enumerate()` integrates nicely with list comprehensions, making your code more succinct, especially when your logic requires both the item and its index. This lets you keep the logic straightforward and easy to understand.
`enumerate()` is extremely flexible and can be used with any object that is iterable such as lists, tuples and even strings, making it a versatile tool within Python's range of capabilities, not limited just to lists.
Gaining a good grasp of the `enumerate()` function helps with moving to other more advanced concepts in Python such as generators and comprehensions. Mastering this function is part of a solid Python programming foundation for anyone looking to become a capable programmer. It really is an important skill to possess.
Python List For Loop A Step-by-Step Guide to Enumerate Function Implementation - Python Enumerate Loop Mechanics and Memory Usage
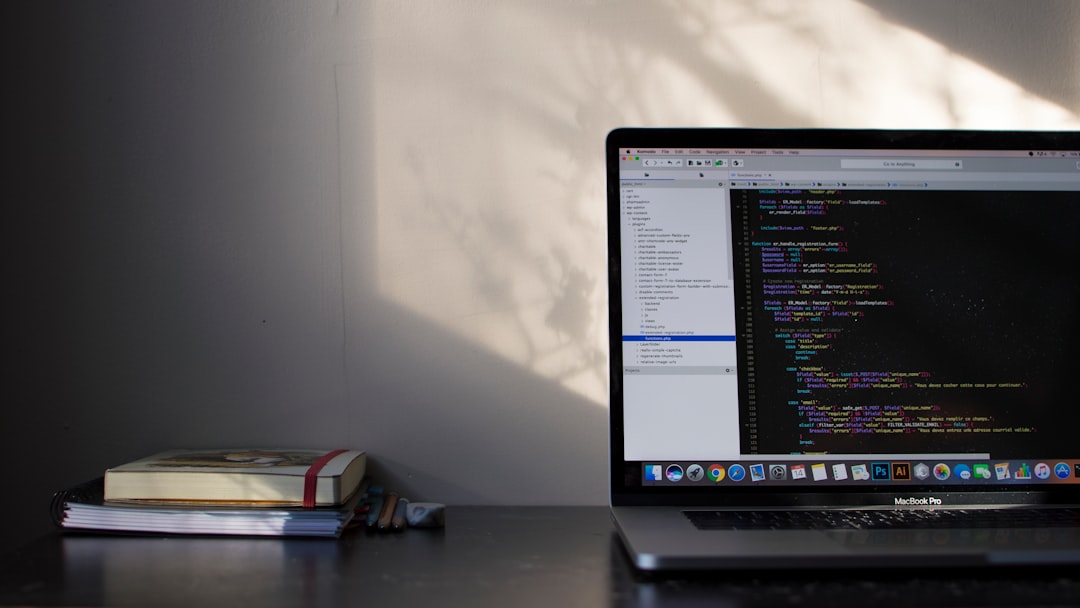
When diving into the mechanics of Python's `enumerate` function and how it impacts memory usage, it's helpful to realize that while it simplifies code by automatically managing index tracking within loops, it does come at a slight cost: increased memory consumption. Each time `enumerate` is used, it creates an iterator that bundles together the current loop index and the corresponding item from the iterable. This pairing, while useful, naturally needs more memory compared to a basic loop that doesn't generate this extra data structure. Despite this small increase in memory use, `enumerate`'s advantages – like easier-to-read code and flexibility across many data types – often outweigh the minor memory overhead. This is particularly true in complex applications handling large datasets where clean code and maintainability become even more vital. It's noteworthy that, despite `enumerate`'s usefulness, many new Python learners don't always use it to its full potential, sometimes falling back to more cumbersome methods. This underscores that recognizing and fully understanding the power of `enumerate` is a key part of enhancing your Python skills. By mastering `enumerate`, you not only improve your looping techniques but also develop good coding practices for effectively working with iterables in the language.
Python's `enumerate` function is a handy tool for iterating through any iterable, like lists or tuples, while keeping track of the position of each item. It essentially provides both the index and the value at each step in the loop. While it's not a radical departure from traditional loops, it eliminates the need for manual index tracking, which results in cleaner code that's often easier to understand.
`enumerate`'s efficiency stems from its use of iterators, which can save a considerable amount of memory, especially when dealing with larger datasets. Rather than creating a full copy of the data with indices, it cleverly generates the indices as needed during the iteration. This can be important in situations where memory constraints are a factor. Interestingly, its output is a series of tuples, which often make the code look a bit tidier when you unpack them within your loop.
One interesting detail is that `enumerate` allows you to customize the starting point for the index using the `start` parameter, which defaults to zero. For instance, you can start with 1 if your output needs to be in a more human-friendly format, for example, when displaying numbered lists to a user.
Beyond its memory efficiency, `enumerate` is often faster than traditional loop approaches when managing indices, because Python has optimized it for this particular task. So, in scenarios involving many iterations, this can become noticeable. It plays well with other iterables too, like tuples or even strings, highlighting its flexibility.
One of the overlooked advantages of `enumerate` is its role in preventing those pesky "off-by-one" errors that frequently occur when manually managing indices. These little mistakes can be a pain to track down. It also makes list comprehensions easier to read and more compact, which improves code readability and clarity.
While not necessarily a complex feature, understanding `enumerate` is considered essential for writing clean, concise, and efficient Python code. It's something of a Pythonic idiom, or a best practice, for handling indexed loops. It's a useful tool for those who want to write better-structured code, especially in the context of data science or manipulating large datasets. It's also a stepping stone to more advanced Python techniques like generators or comprehensions. In conclusion, learning `enumerate` is a valuable part of any Python developer's skillset.
Python List For Loop A Step-by-Step Guide to Enumerate Function Implementation - Iterating Through Lists with Custom Starting Index
The `enumerate` function in Python provides a convenient way to loop through lists while simultaneously tracking the index of each element. While it typically starts counting from 0, a unique aspect is its ability to begin the index at a different value. This customization is achieved by supplying a second argument to the `enumerate` function, such as `enumerate(my_list, start=1)`. This feature can be extremely handy when your output needs to be presented in a specific way, like when creating a numbered list that starts from 1 for better readability in user interfaces. However, it's worth noting that many Python newcomers continue to lean on more traditional loops and manual index management, which can sometimes lead to less maintainable and potentially inefficient code. Embracing and using the `enumerate` function to its full potential is vital for developing higher-quality code and avoiding typical coding mistakes like "off-by-one" errors that can crop up with manual indexing.
1. The ability to customize the starting index of the `enumerate` function using the `start` parameter is quite useful, as it lets you adapt the indexing behavior to match specific needs. This is handy when you need to align program output with user expectations, or when dealing with other data structures where the indexing starts at a number other than 0.
2. While less often discussed, `enumerate` can demonstrably enhance the performance of your loops. Python's internals are optimized for `enumerate`, which means that the overhead of managing indices is reduced, especially when dealing with a large number of iterations. This can lead to noticeable improvements in runtime, especially for programs that heavily involve iterating through data.
3. While `enumerate`'s convenience is often highlighted, it's worth considering that its efficiency also comes with memory benefits. Since `enumerate` works by creating an iterator, it avoids constructing a full copy of the list, thus minimizing the amount of memory used. This is important for programs working with extensive datasets or applications constrained by memory limitations.
4. `enumerate` returns a sequence of tuples, where each tuple contains both the index and the corresponding item from the list. This dual nature of the output is very practical for code readability and simplifying manipulations within the loop. It provides a way to access both index and value in a very compact way within the loop.
5. One underappreciated aspect of using `enumerate` is its ability to help prevent the frustrating "off-by-one" errors, which often occur when manually tracking indices. It's these kinds of small, hard to find errors that can be a big headache during debugging. By automating the index handling, `enumerate` drastically reduces the likelihood of these types of mistakes.
6. It's worth noting that `enumerate` isn't limited to just lists. It works with any iterable, including tuples, strings, or even custom objects you might create. This makes it a remarkably versatile tool across a wide range of programming tasks where you might need to iterate through some kind of sequence.
7. Programs using `enumerate` tend to be easier to understand compared to loops that manually manage index counters. This makes them easier to maintain, especially when other developers may be working on the same code base. Cleaner code is often the result of using a tool such as `enumerate`.
8. The combination of `enumerate` and list comprehensions can lead to concise and very readable Python code. Being able to access both index and value in a comprehension makes for extremely efficient code when the operation needs both pieces of information. It’s a testament to how well `enumerate` integrates with other powerful Python constructs.
9. `enumerate` is particularly useful for tasks that involve manipulating or parsing data, like when working with CSV files or datasets with structured information. It helps to establish a direct link between a data point and its location in a dataset, making it a powerful tool for debugging and performing validations during data analysis.
10. Developing fluency with `enumerate` can be seen as a crucial step towards mastering more advanced topics in Python. It helps establish a solid understanding of how iterators work and forms a building block for understanding generators, which are a powerful technique for creating customized iterables. You could consider `enumerate` an essential skill to have in your programming tool belt.
Python List For Loop A Step-by-Step Guide to Enumerate Function Implementation - Adding Counter Variables to Python List Iterations
When working with Python lists and loops, incorporating counter variables can significantly improve the clarity and efficiency of your code. The `enumerate` function provides a built-in way to accomplish this by pairing each element in an iterable with its corresponding index during loop iterations. This eliminates the necessity of manually managing counters, reducing the chances of common errors like off-by-one issues and making your code easier to maintain. Further, `enumerate` lets you specify the starting index for your counter, which is very useful for situations where you need to align your output with specific conventions or user expectations. While many Python beginners might stick with more traditional looping techniques that explicitly handle indices, the `enumerate` function presents a cleaner and more effective approach. It's often overlooked, but embracing its capabilities can make your code more efficient and readable. As you continue your journey as a Python programmer, learning and integrating the `enumerate` function into your practices builds a strong foundation for exploring more advanced Python techniques and helps foster a good coding style.
1. The `enumerate()` function neatly handles index management by performing index calculations internally, resulting in cleaner code. This design reduces the mental overhead for developers who would otherwise need to keep track of a separate index variable.
2. When using `enumerate()` with a custom starting index, the behavior adapts based on the `start` parameter, enabling developers to align output with various contexts. This is handy for user interfaces or reports that might require more natural numbering, improving the user experience without needing extra code.
3. A less-discussed benefit of `enumerate()` is its production of an iterator, allowing for what's called "lazy evaluation". This implies that indices are created on-demand, avoiding any memory bloat from creating a new list for just the indices. This is especially beneficial when working with large datasets.
4. Employing `enumerate()` can surprisingly lead to faster code execution. Python’s internal optimizations for these built-in functions often outperform manually written loops, particularly when iterating through lists repeatedly. This can positively impact the overall application's performance.
5. The output of `enumerate()` is a series of tuples, pairing each item in a list with its index. This well-defined structure enhances code readability and simplifies situations where both the index and the item are needed. It makes accessing both pieces of information easier than manually retrieving them, reducing errors.
6. Automating index tracking with `enumerate()` helps prevent typical programming snags like "off-by-one" errors. This reduction in human error leads to more robust code, especially valuable in critical applications where accuracy is paramount.
7. `enumerate()` isn't just for lists; it can work with any iterable, like strings or tuples. This wide compatibility makes it a valuable tool for developers, covering tasks from basic loops to more complicated parsing.
8. Combining `enumerate()` with list comprehensions not only boosts efficiency but also keeps the code clear. Elegant one-line statements retain both the index and the value, making for concise Python that's still understandable. This combination is a key ingredient in creating easy-to-read Python code.
9. In data analysis, `enumerate()` plays a vital part by linking data points with their locations, making it simpler to spot errors or anomalies. This capability can streamline debugging, especially within larger datasets.
10. Developing mastery over `enumerate()` acts as a stepping stone towards more advanced Python features like generators and specialized iterables. This expands a developer's grasp of Python's abilities and helps them write code that's more effective and maintainable over time.
Python List For Loop A Step-by-Step Guide to Enumerate Function Implementation - Using Enumerate with Different Python Data Structures
The `enumerate` function proves incredibly useful when working with different Python data structures. It seamlessly integrates with lists, tuples, and even strings, making it a versatile tool for looping through data while simultaneously tracking the index of each element. This dual access to both value and position within the sequence makes code more efficient and readable, especially when compared to manually managing indices. A key feature is the ability to specify a starting index for the counter, giving you the power to tailor your output to user expectations or data formatting needs. Even though `enumerate` is a valuable function for better coding practices, some programmers, particularly beginners, tend to rely on more conventional loop methods which can lead to less readable and harder-to-maintain code. By recognizing and effectively using the `enumerate` function, programmers can write clearer, more streamlined code while cultivating strong programming habits, making their codebase more robust and easier for others to understand.
The `enumerate()` function in Python offers a streamlined approach to looping through data structures by automatically keeping track of each item's position. It's a clever way to bypass the need for intricate manual index tracking, resulting in cleaner and more readable code.
One intriguing aspect is that `enumerate()` produces an iterator object rather than a whole new list. This means it cleverly generates indices as you loop through the data, leading to a more efficient use of memory, which is especially helpful when dealing with massive datasets.
Despite its built-in status, many Python users, especially those just starting, tend to favor more traditional loop methods. This suggests a possible gap in learning more efficient coding styles, as the traditional approach can often lead to less performant code.
One major benefit of `enumerate()` is that it significantly lessens the risk of 'off-by-one' errors. These errors are notorious for cropping up in loop control and can lead to hard-to-find issues when dealing with data. `enumerate()`'s automated index management eliminates a common source of potential bugs.
Although it seems straightforward, the `start` parameter within `enumerate()` lets you define a custom starting index for your loops. This feature has great value when working with interfaces that require more user-friendly numbering (like lists that start at 1 instead of 0). This ability makes it adaptable for many kinds of situations.
The beauty of `enumerate()` is its flexibility. It isn't limited to lists – it works with various iterables including tuples and even strings. This versatility makes it a useful tool for a wide range of coding scenarios that require iterating through sequential data.
The output of `enumerate()` is a series of tuples, neatly combining the index and the item at each step in the loop. This pairing streamlines your code by avoiding the need to manage a separate index variable, which makes the code more clear.
Integrating `enumerate()` within list comprehensions creates remarkably clean and efficient code. It gives you the power to easily access both the index and the item within the concise syntax of a comprehension. This allows for concise code that's still quite understandable.
`enumerate()` makes debugging more straightforward. Since it explicitly connects each data point to its position within the sequence, you can quickly spot errors or inconsistencies in data handling during your debugging process.
Finally, understanding `enumerate()` is fundamental to grasping more complex Python concepts such as generators and comprehensions. It's a core piece of building a strong foundation for writing better, more reliable, and maintainable code, especially in complex programming projects.
Python List For Loop A Step-by-Step Guide to Enumerate Function Implementation - Error Handling and Common Pitfalls in Python Enumerate
While Python's `enumerate` function simplifies looping through sequences by providing both index and value, it's crucial to understand its intricacies to avoid common pitfalls. One key issue is that `enumerate` returns an iterator of tuples, each containing an index and the corresponding item. Not recognizing this structure can lead to errors when trying to access the index and value within the loop. Additionally, the default starting index is zero, but many situations call for a different start index (for example, if you want to present output starting at 1). Not realizing you can customize this can result in errors or undesirable output. Also, relying too much on manual index tracking with range loops rather than using `enumerate` can introduce "off-by-one" errors, making debugging trickier and the code less readable. To fully leverage the benefits of `enumerate` and write cleaner, more efficient Python code, it's vital to grasp its core features and integrate it strategically into your coding style.
1. While `enumerate` simplifies index management and reduces error chances, modifying the iterable during iteration can still cause issues. Adding or removing items can lead to index mismatches or skipped elements, creating subtle and difficult-to-find bugs.
2. Using `enumerate` with something that isn't iterable will result in a `TypeError`. Understanding which data structures work with `enumerate` is key to avoiding this type of error.
3. Although `enumerate` improves memory usage through iterators, performance problems can still arise with extensive datasets if the initial data isn't optimized. The memory requirements of the original data can offset the advantages of `enumerate` in these cases.
4. Improper use of the `start` parameter when using `enumerate` can lead to confusing outputs. Incorrectly setting the starting index can result in outputs that don't meet the program's expectations, causing alignment problems in user interfaces and other program outputs.
5. Using `enumerate` within nested loops seems simple enough, but if the inner loops also modify elements, it can get tricky. Understanding which index corresponds to which value at each level can become complex.
6. One potential pitfall is that beginners might not realize they are defeating the purpose of `enumerate` if they manually adjust the index while it's being used. Doing this removes the advantage of automatic index handling, making code less readable and more error-prone.
7. There are times when using `enumerate` might not be ideal. For example, while debugging, you might need to use breakpoints to investigate the state of the loop. Manually managing indices sometimes provides a finer level of control for exploring more complex logic.
8. When creating your own iterable objects (like classes that can be looped through), integrating `enumerate` might produce unexpected behavior if your custom iterable doesn't follow Python's standard iteration protocols. This could lead to runtime errors.
9. Despite being a main advantage of `enumerate`, off-by-one errors can still happen if you're not careful. They often occur when directly manipulating the indexed values in your code, especially if you forget that the index starts at zero by default.
10. Using multiple `enumerate` iterators on the same iterable in a complicated function can lead to confusion about which iterator is being changed at any given moment. Keeping track of multiple indices can easily get messy and lead to incorrect logic.
More Posts from aitutorialmaker.com: