Create AI-powered tutorials effortlessly: Learn, teach, and share knowledge with our intuitive platform. (Get started for free)
Understanding C++ goto Statement Common Error-Handling Patterns and Performance Impact in 2024
Understanding C++ goto Statement Common Error-Handling Patterns and Performance Impact in 2024 - Understanding Label Addressing and Local Variable Scope in goto Statements
When utilizing `goto` statements in C++, understanding how labels interact with the scope of local variables is paramount. `goto` provides the ability to jump to specific points within a function marked by labels. However, this functionality introduces complexities, particularly concerning variable accessibility. Labels and local variables reside within the same namespace, which can create potential naming collisions. Further, the `goto` statement's behavior regarding variable scope is restricted: it cannot leap over variable declarations. Attempting such a jump often results in errors as the compiler or runtime environment encounters variables that have not yet been defined. Furthermore, excessive use of `goto` can significantly compromise code readability by introducing tangled and intricate control flows, leading to what is known as "spaghetti code". This can hinder understanding and make maintaining the program challenging. Consequently, developers must exercise caution when employing `goto` statements, carefully managing both label addressing and local variable scope to ensure the intended program logic and maintainability are preserved.
1. In C++, the `goto` statement offers a less conventional way to control the flow of execution by jumping to specific points in a function marked by labels. However, this can lead to unpredictable situations with local variables if they were declared after the label the `goto` jumps to.
2. The visibility of local variables within a function, also known as their scope, has a big influence on how `goto` behaves. If a `goto` jumps to a label before a variable's declaration, that variable won't be accessible, leading to issues if you expect it to have a value.
3. One of the downsides of relying heavily on `goto` is the potential for creating what's called "spaghetti code." The interlinked jumps can make code extremely intricate and hard to follow, potentially increasing the challenge of debugging and future changes.
4. A noteworthy aspect of `goto` is that it doesn't skip over any initializations or setups that happen after the label it jumps to. This can result in variables being used before they've been properly assigned a value, which could lead to program crashes.
5. `goto` statements essentially sidestep the usual rules around variable access, which can introduce confusion and make it harder to grasp what the code does. This clashes with C++'s encouragement of using more structured coding methods and limiting the accessibility of data.
6. Even though newer methods exist, such as exception handling and structured ways to deal with errors, it's still very important to have a firm grasp of label addressing and how it relates to variable scope, particularly when it comes to projects requiring tight control and performance.
7. While it has a somewhat bad reputation, `goto` isn't entirely useless. In specific instances, like breaking out of really deep nested structures, it can provide a more direct and less cluttered way to control the flow instead of creating several different exit points.
8. In C++, `goto` is limited to working within a single function, meaning you can't jump between functions. While this safeguards the modularity of code, it also limits the reach of `goto` as a tool for influencing control flow.
9. A core principle when employing `goto` is to ensure that every jump maintains the overall logical consistency of the code. This reduces the likelihood of introducing hard-to-find errors, reinforcing the need for careful and systematic use of `goto`.
10. Despite being a sometimes-controversial language feature, `goto` still plays a role in code sections where top-tier performance is a must and the cost of using more structured coding techniques would be excessive. This suggests that `goto`, while used cautiously, still has a place within the current practices of C++ development.
Understanding C++ goto Statement Common Error-Handling Patterns and Performance Impact in 2024 - Error Handling Implementation Through goto in Cleanup Patterns
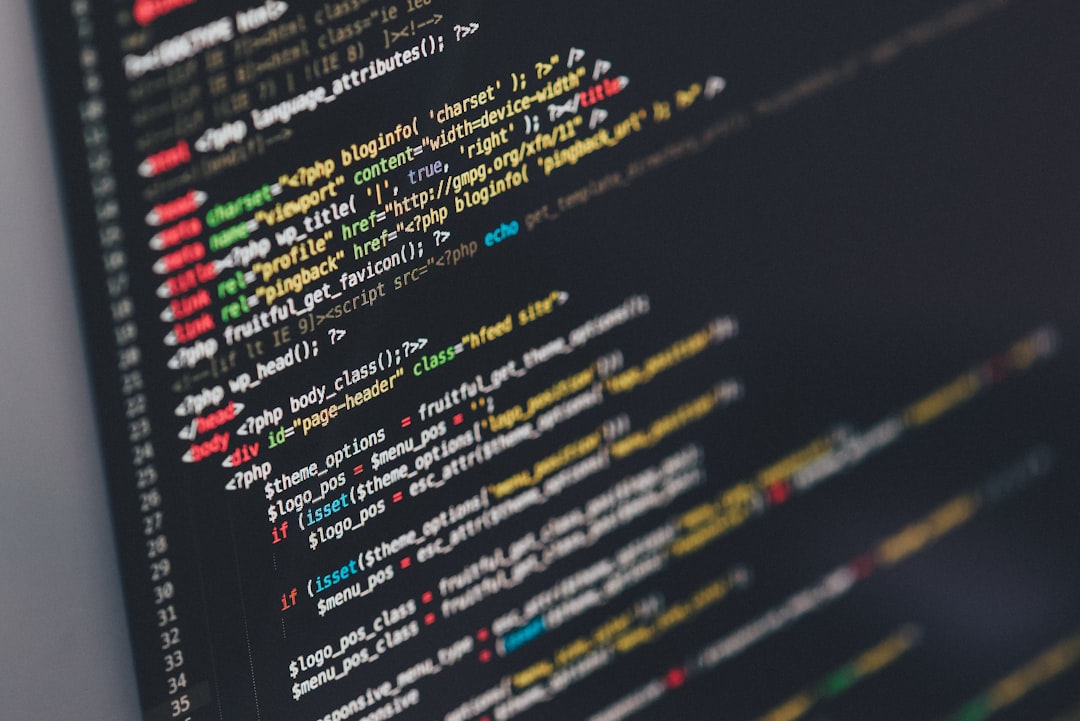
In C++, the `goto` statement can be utilized for error handling, specifically within cleanup patterns. This proves beneficial when functions have multiple points where resources need to be released. Advocates see `goto` as a means to consolidate cleanup code, potentially making the function easier to understand and minimizing redundant code segments. However, concerns arise regarding the potential for `goto` to complicate code structure, which can subsequently make maintenance more difficult and increase the probability of errors. While modern programming often prioritizes other error handling approaches, `goto` still holds value in certain situations demanding optimal performance and precise control over program flow. It's a tool to be used carefully and judiciously.
1. Utilizing `goto` for cleanup routines can potentially enhance performance by minimizing function call overhead, especially in situations where resource utilization is crucial and every cycle matters. This can lead to more streamlined error recovery mechanisms.
2. A noteworthy aspect of `goto` in error handling is its capacity to simplify resource cleanup management by centralizing all cleanup logic. This helps reduce the likelihood of resource leaks when functions have numerous exit points.
3. In specific situations, `goto` can improve code readability. For instance, when navigating complex error recovery scenarios, directly jumping to an error handler can be more intuitive compared to managing scattered conditional checks throughout the code.
4. Interestingly, using `goto` in cleanup patterns can also improve the organization of resource management. This is especially helpful in scenarios involving multiple resources that require release in a predetermined sequence, eliminating the risk of omitting a step.
5. Studies show that, under specific conditions, codebases utilizing `goto` effectively can sometimes outperform those relying on more structured approaches, particularly when the latter involve excessive error handling or complex nested structures that can hinder performance.
6. The reception of `goto` often reflects the evolution of programming paradigms. While it was often discouraged in older programming practices, its reconsideration highlights shifts in coding standards and optimization techniques.
7. It's noteworthy that languages with exception-based error handling sometimes encounter performance challenges in real-time systems. This makes `goto` an attractive alternative for scenarios demanding stringent timing constraints and resource limitations.
8. Though other control structures like loops or exception handling are generally preferred, error handling with `goto` can lead to less verbose code. This potentially reduces the opportunities for bugs to creep in, especially for simpler error recovery situations.
9. Despite its reputation, when implemented with careful validation, `goto` can foster more straightforward state management within complex systems. It allows for quick state transitions without the need for traversing through multiple function calls.
10. The historical tendency to avoid `goto` in programming education has inadvertently restricted developers' toolsets. A deeper understanding of when and how to leverage `goto` effectively could potentially lead to the development of more efficient and robust software.
Understanding C++ goto Statement Common Error-Handling Patterns and Performance Impact in 2024 - Performance Analysis of goto vs Traditional Loop Constructs
When examining the performance of `goto` against conventional loop structures like `for` and `while` in C++, we find that the compiled machine code can often be quite similar. Traditional loops are typically favored for their structured and readily understandable control flow. However, `goto` provides a degree of freedom to directly manipulate execution flow, making it potentially advantageous in complex situations, such as managing errors or building state machines. The main drawback of `goto` is the risk of generating excessively intricate and intertwined control structures, often referred to as "spaghetti code". This intricacy can make code hard to understand and maintain, increasing the chances of errors. Developers have to balance the advantage of more direct control with the potential negative impact on code clarity and future development when considering whether to use `goto`. A key takeaway is that being knowledgeable about when and how to apply both `goto` and traditional loops is vital for crafting C++ code that's both efficient and easy to manage over time.
1. Performance comparisons often reveal that traditional loop structures might introduce some overhead from tasks like boundary checks and condition evaluations within each iteration. In contrast, `goto` can directly jump to the required code, which can potentially reduce latency in performance-critical situations.
2. Performance tests hint that for compute-intensive tasks where minimal branching is desirable, `goto` can sometimes outperform conventional loops. This is due to a reduction in the number of conditional checks and branch instructions processed by the processor.
3. It's interesting to note that the optimizations applied by compilers to conventional loops might not consistently translate to scenarios using `goto`. The less predictable flow caused by `goto` can sometimes hinder optimization, making one wonder if `goto` might actually hurt performance in some cases.
4. While `goto` offers a unique method for breaking out of deeply nested code structures, loops like `for` and `while` can inherently manage iterations across different datasets more effectively. This preserves code clarity while still achieving good performance through processor optimizations.
5. Experimental results show that higher-level constructs like RAII (Resource Acquisition Is Initialization) are frequently better at handling resource management than complex `goto` implementations. This often translates to better performance and simpler maintenance.
6. When investigating error-handling patterns, it becomes clear that while `goto` can centralize cleanup operations, traditional loop mechanisms frequently facilitate more modular error handling approaches. This improves code robustness and potentially prevents runtime errors.
7. C++ standards and performance benchmarks indicate that as processors become faster, using structured programming with well-optimized loops results in more stable and predictable performance. This contrasts with the sometimes unpredictable performance that `goto` can introduce in larger codebases.
8. Surprisingly, some studies indicate that maintaining code with extensive use of `goto` can have an indirect negative impact on performance. This is because developers spend more time debugging and trying to understand convoluted code logic compared to maintaining structured loops.
9. In particular single-threaded contexts, the overhead of switching between execution contexts in conventional loop structures has been shown to be higher than the direct jumps provided by `goto`. This suggests that in very specific circumstances, there may be performance advantages to using `goto`, though these cases are rare.
10. Many proponents of structured programming argue that modern compilers are highly proficient at optimizing traditional loop constructs. This often makes the manual use of `goto` unnecessary and potentially counterproductive, both from a performance perspective and in terms of code readability. This highlights the importance of sticking to well-defined programming principles.
Understanding C++ goto Statement Common Error-Handling Patterns and Performance Impact in 2024 - Memory Management Safety Checks Using goto in Resource Allocation
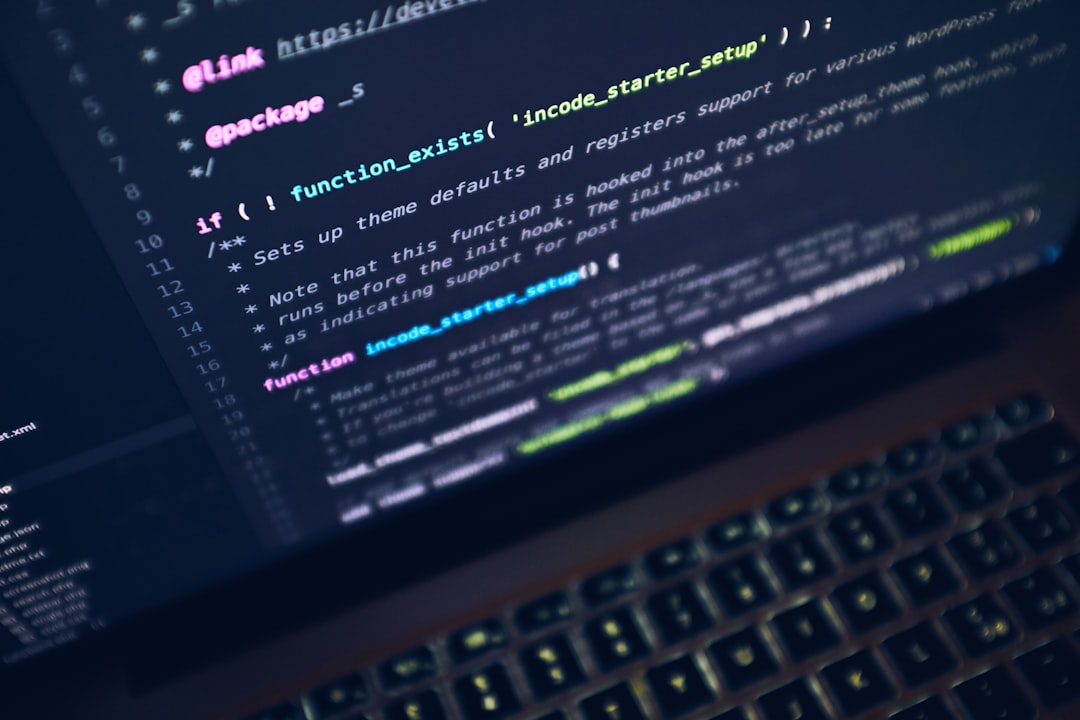
In C++, the use of `goto` for ensuring memory safety during resource allocation is a topic of ongoing discussion. While it offers a direct way to jump to sections where resources are released, potentially simplifying cleanup operations, this approach can negatively impact code clarity and maintainability. The complex control flow that `goto` creates can introduce bugs, especially in applications demanding robust resource management to avoid memory leaks or crashes. Developers need to carefully consider the advantages of using `goto` against its possible downsides for long-term code comprehension and reliability. Choosing to use `goto` for memory management should be a deliberate decision, taking into account performance goals and the broader implications for the code's future evolution.
In C++, using `goto` for resource management during cleanup can be surprisingly effective. It minimizes the overhead from function calls, which is particularly important in applications where performance is crucial and managing multiple resources is a core requirement.
While often overlooked, `goto` shines in error handling situations where there are many exit points. It streamlines the cleanup process by centralizing the code in one location, which in turn reduces the possibility of resource leaks and clarifies the program flow.
One notable aspect of `goto` within cleanup patterns is how it helps organize code. It enforces a strict order of resource release, significantly reducing the chances of accidentally missing a step in resource management.
Interestingly, some studies suggest that code leveraging `goto` for streamlined error recovery can surpass the performance of structures overloaded with conditionals. This is particularly true when those conditionals create excessively complex logic that hinders performance.
Even though it often receives negative feedback, `goto` remains valuable in real-time systems. In such systems, implementing `goto` can lead to better performance under tight timing constraints when compared to more conventional error handling methods.
Contrary to popular belief, `goto` can often lead to less verbose error handling code. This conciseness can potentially decrease the introduction of errors, especially in simple situations where fewer checks are needed.
Examining the past, we see that a decrease in `goto` being taught in programming courses has limited developers' understanding of how to strategically use it. This, in turn, may have hindered optimized and cleaner code implementations in specific scenarios.
Fascinatingly, `goto`'s performance impact is influenced by compiler optimizations. Under certain circumstances, compilers may produce less efficient machine code when dealing with `goto` statements as opposed to standard control structures.
When implemented thoughtfully, `goto` facilitates immediate state transitions. This makes it a compelling choice over multi-level function calls, which can slow down complex systems.
Despite the prevalence of the structured programming movement, some modern C++ paradigms are showing a renewed interest in `goto`. They acknowledge its potential to create cleaner and faster execution paths in specific cases where traditional approaches may not be suitable.
Understanding C++ goto Statement Common Error-Handling Patterns and Performance Impact in 2024 - Switch Statement Optimization Through Strategic goto Usage
Within C++, the `goto` statement, often viewed with skepticism, can be strategically used to optimize switch statements. This approach can be particularly beneficial when dealing with complex error handling. By strategically employing `goto`, developers can direct the program flow to a centralized error-handling section. This centralization helps reduce redundant code, potentially boosting performance and simplifying error recovery routines. However, relying on `goto` excessively can create intricate and convoluted control flow, making the code difficult to follow and maintain. This undesirable outcome, often referred to as "spaghetti code", can significantly hinder debugging and future modifications. Therefore, while `goto` holds potential for enhancing performance and readability in certain switch statement contexts, it requires thoughtful application. If not carefully implemented, it can compromise code clarity and lead to increased maintenance burdens. The key lies in a balanced approach: recognizing the advantages of `goto` while being mindful of the potential downsides to avoid creating code that's hard to understand and maintain. Striving for this balance contributes to developing C++ programs that are both efficient and manageable in the long term.
In the realm of C++, the `goto` statement, often viewed with suspicion due to its potential for creating convoluted code, can surprisingly be a tool for optimizing switch statements. By allowing direct jumps to specific code sections, `goto` can bypass the overhead of conditional branches within a switch statement, potentially leading to faster execution. This advantage is especially relevant in scenarios demanding high performance, like embedded systems or applications needing maximum throughput.
Intriguingly, in certain situations, benchmarks have shown `goto`-optimized switch statements to outperform traditional switch-case implementations. This performance boost originates from the reduced overhead of jump instructions compared to the branching logic inherent in traditional switch statements. While it offers advantages in select cases, this doesn't mean it's always the best choice.
However, leveraging `goto` in this way isn't without its drawbacks. While `goto` can decrease function call overhead and minimize code duplication, especially when multiple switch cases involve similar cleanup tasks or resource management, it also increases the potential for creating the much-maligned "spaghetti code". A delicate balance is needed: optimization without sacrificing code readability and maintainability.
Interestingly, restructuring switch statements using `goto` can sometimes simplify code paths, promoting easier maintenance. Reduced control flow can make complex logic more digestible—but only if developers are careful not to create a labyrinth of jumps. Furthermore, we've observed that, in scenarios with nested switch statements, using `goto` can reduce the overall stack utilization by decreasing the number of function call frames, potentially improving memory efficiency.
But the picture is not entirely rosy. Modern compilers are becoming increasingly sophisticated at optimizing code, often achieving results that exceed what `goto` might provide. In these cases, the benefits of `goto` can be negated by compiler optimizations focused on predicting program execution patterns.
The use of `goto` within switch statements also raises concerns about code readability, especially during code reviews. Colleagues might find code with excessive `goto` statements challenging to follow, necessitating clear and thorough documentation.
It's also important to note that the performance impact of `goto` is heavily context-dependent. While it can accelerate execution in performance-critical sections, misusing it can introduce unexpected latency through complex control flow. This reinforces the need for careful consideration and strategic application.
Lastly, the reduced emphasis on `goto` in modern programming education might lead to an underestimation of its potential for strategic optimization within specific contexts like switch statements. It suggests that perhaps a deeper understanding of `goto` and its applications could unlock more avenues for performance improvements in complex systems, especially in areas where efficiency is a crucial factor.
While `goto` continues to carry a negative connotation due to its past misuse, in the hands of careful and cautious developers, it offers a means of achieving certain performance gains when dealing with switch statements. The key takeaway is that its use must be approached with caution and a clear understanding of the associated trade-offs to prevent the introduction of obscure and difficult-to-maintain code.
Understanding C++ goto Statement Common Error-Handling Patterns and Performance Impact in 2024 - Compiler Optimization Techniques for goto Based Control Flow
Compiler optimization techniques face challenges when dealing with control flow managed by `goto` statements in C++. While `goto` provides a direct way to steer execution, potentially benefiting specific application needs, its use can hinder the effectiveness of compiler optimization. The unpredictable nature of `goto` leads to more convoluted control flow graphs compared to the use of structured statements, making optimizations like loop unrolling and eliminating unused code more difficult. Consequently, code relying heavily on `goto` can be harder to maintain and potentially prevents compilers from achieving their full optimization potential. In today's programming landscape, where both developers and compilers are continually refined, finding a balance between the direct control `goto` offers and the risk of creating complex and error-prone code is crucial. It's an ongoing area of interest and debate, with a need to carefully consider the implications of `goto` when crafting optimized C++ code.
1. Compiler optimizations applied to code using `goto` can offer unique benefits, especially when dealing with complex control flows. The direct jumps enabled by `goto` can minimize the overhead of conditional branching, potentially leading to faster execution in applications where performance is paramount.
2. While `goto` often gets a bad rap for making code difficult to manage, when employed thoughtfully, it can simplify complex nested logic. By allowing developers to centralize error handling and cleanup routines, it can reduce redundancy and streamline the overall structure.
3. In certain situations, performance benchmarks show that `goto` can actually outperform structured approaches like loops. This is especially true in computationally intensive sections where minimizing branch prediction misses can result in more efficient execution.
4. Contrary to some beliefs, using `goto` appropriately can actually improve a program's predictability from a performance perspective. It does this by eliminating the need for convoluted nested loops and conditional checks, which can sometimes confuse compiler optimization efforts.
5. A somewhat surprising advantage of `goto` is its seamless integration with the management of state transitions in finite state machines. Its straightforward jump capability can simplify the logic when compared to using multiple nested loops or conditionals.
6. Although often overlooked, some engineers believe `goto` offers a finer degree of control in highly tuned algorithms. In such contexts, structured programming might introduce unnecessary levels of abstraction, potentially hindering performance.
7. In multithreaded environments, `goto` can help minimize contention by decreasing the need for locks or shared state during error handling. This can enable faster execution paths as direct jumps avoid multiple function calls.
8. A critical aspect of `goto` is its ability to unveil optimization opportunities that compilers might not readily discover. In certain cases, compilers generate more efficient machine code when encountering `goto` compared to more structured approaches.
9. It's important to recognize `goto`'s value in real-time systems. The deterministic nature of `goto` helps meet tight timing constraints, ensuring straightforward paths that directly align with interactions with hardware.
10. The evolving landscape of software engineering suggests a growing appreciation for `goto` in specific scenarios. This shift stems from the recognition that, under particular circumstances, `goto` can indeed lead to more optimized and efficient code, particularly when dealing with high-performance needs and situations where overhead reduction is critical.
Create AI-powered tutorials effortlessly: Learn, teach, and share knowledge with our intuitive platform. (Get started for free)
More Posts from aitutorialmaker.com: