Understanding Python SQLite Connections vs
Cursors A Deep Dive into Database Interaction Efficiency
Understanding Python SQLite Connections vs
Cursors A Deep Dive into Database Interaction Efficiency - Python SQLite Connections The Foundation of Database Interaction
The core of interacting with SQLite databases within Python lies in establishing a connection. The `sqlite3` module's `connect` function creates a Connection object, which becomes the gateway to your database. Through this connection, you can then issue SQL instructions using a cursor. It's important to recognize that SQLite employs locking mechanisms to maintain data integrity, particularly when multiple connections attempt simultaneous updates. This can lead to delays if other connections are waiting to access the database. The `timeout` parameter influences how long a connection will wait for a lock to be released, impacting performance in situations with heavy database traffic. Understanding the distinct roles of connections (for managing the database link) and cursors (for executing commands and fetching results) is essential for developers looking to build robust and efficient applications that utilize SQLite. Furthermore, it's noteworthy that SQLite is automatically bundled with Python, readily available for use without extra installations, and stores all data within a single file. This streamlined approach avoids the complexities of managing a dedicated database server, while still offering solid data handling capabilities. SQLite connections act as the bridge between your Python programs and the database, enabling efficient data retrieval and storage. However, the need for careful management of connections and understanding their interplay with cursors for optimizing efficiency, remains critical.
To interact with an SQLite database from Python, we first need to establish a connection using the `sqlite3.connect()` function. This function returns a Connection object, which acts as a handle to the database file. If multiple connections try to modify the database at the same time, SQLite will lock it, pausing any other access until the current transaction is finalized. This locking mechanism can create delays if other connections are waiting.
The `timeout` parameter within the `connect` function dictates how long a connection will wait for a lock release before giving up and throwing an error. By default, it waits for 5 seconds. After establishing a connection, we can start issuing SQL commands through a Cursor object. A cursor acts as an intermediary, allowing us to execute all the standard database operations (CRUD: Create, Read, Update, Delete).
Crucially, when performing database modifications, we must explicitly commit the changes to ensure they are permanently saved. Also, it is a good practice to close the connection when finished to release resources used by the database. Interestingly, if the database file doesn't already exist, SQLite will automatically create it when the `connect` function is called.
The beauty of SQLite is that it comes bundled with Python, so you don't need to install anything extra to use it. Furthermore, because it stores all its data within a single file, you don't need a separate server to manage it, leading to simpler deployments. Python’s interaction with SQLite is effective for managing data, making it a good option across program executions.
The distinction between SQLite's connections and cursors is vital for efficient development. The Connection object controls access to the database while the Cursor object is the tool we use for executing specific SQL commands and retrieving results from those commands. Understanding these two aspects of SQLite is key to effective database interactions within Python.
Understanding Python SQLite Connections vs
Cursors A Deep Dive into Database Interaction Efficiency - Cursors as Intermediaries Between Connections and SQL Commands
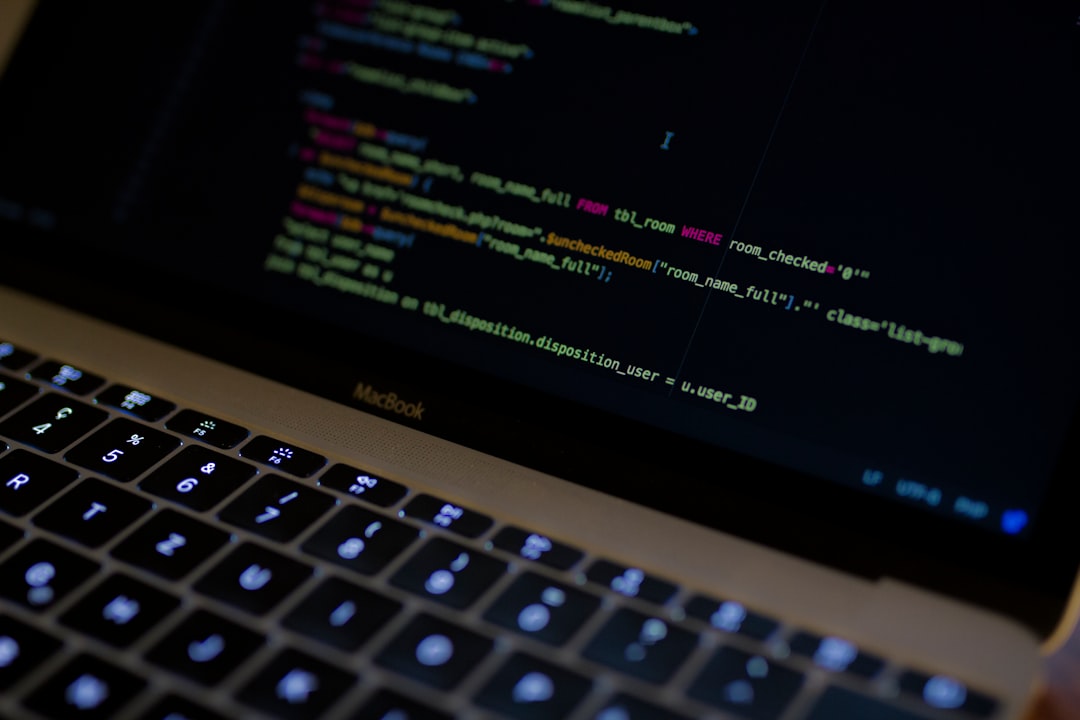
Within the context of Python's interaction with SQLite, cursors act as a crucial bridge between the established database connection and the execution of SQL commands. This intermediary role allows for a more streamlined and efficient approach to database interactions. Cursors aren't just limited to retrieving data; they also provide the means to pinpoint and modify specific rows within a table, a capability supported by SQL standards. This fine-grained control over data manipulation enhances the overall effectiveness of database operations.
By separating the responsibilities of managing the connection to the database (handled by the connection object) from the task of executing SQL commands and handling the results (the cursor's domain), we gain a clearer separation of concerns within our code. This structured approach leads to cleaner and more maintainable applications. This separation allows for more precise control over result retrieval and better optimization of query processing. Effectively leveraging cursors within the SQLite connection framework is paramount for developers seeking to construct powerful and efficient applications relying on SQLite's capabilities. Understanding the synergy between these two key components is pivotal for building high-quality database applications in Python.
Cursors in SQLite act as intermediaries, handling the execution of SQL commands within the context of a database connection. They're relatively lightweight, efficiently managing the state of query execution and result retrieval without imposing a large overhead. While a single connection can oversee multiple cursors, each cursor is restricted to working with just one SQL command at a time, ensuring clear and organized command execution.
This intermediary role gives cursors the ability to retrieve data in different ways, whether it's row by row, multiple rows at once, or in batches, allowing developers to fine-tune data retrieval based on their application's specific needs. Using the `execute()` method with parameterized queries not only safeguards against SQL injection vulnerabilities but also makes running the same query repeatedly more efficient.
Moreover, cursors manage their internal state and provide options for customizing how they function. For example, they can define row factories, which control how retrieved rows are presented, adding a degree of flexibility for data handling. All actions performed through a cursor occur within the context of a database transaction controlled by its associated connection. This means that changes made through a cursor aren't written to the database permanently until a commit is explicitly triggered.
However, even with SQLite's lightweight design, performance issues can arise if cursors are managed poorly. Hanging onto cursors for longer than needed can consume excessive resources and potentially lead to locking problems. SQLite's concurrency model relies on database-level locking, meaning that if one cursor is executing a transaction, other cursors might have to wait, impacting the application's responsiveness. Unlike some other database systems, SQLite doesn't require manual closing of cursors in most cases. Instead, the cursor is automatically cleaned up when the associated connection is closed.
Debugging SQL commands that are run through cursors can present a challenge. But by carefully monitoring logs and employing error handling techniques like try-except blocks, programmers can effectively track down any issues that occur during execution. This combination of features makes cursors a crucial component for efficient SQLite interactions in Python. Understanding how to utilize and manage them is a key step in building robust and performant database applications.
Understanding Python SQLite Connections vs
Cursors A Deep Dive into Database Interaction Efficiency - Establishing and Managing Database Connections in Python
Within Python, establishing and maintaining a connection to an SQLite database is fundamental for interacting with the data stored within. The `sqlite3` module provides the `connect` function, which establishes the connection and returns a Connection object. This object acts as the conduit to your database, allowing you to send SQL instructions through a cursor. Efficiently managing database connections is paramount to achieving good performance and scalability in Python applications, as they are a limited resource. Creating and closing connections repeatedly can be resource intensive. Employing methods such as connection pooling can alleviate this burden by reusing existing connections, lessening overhead and improving response times. Importantly, since SQLite uses locking mechanisms to safeguard data integrity, understanding these mechanisms is vital for crafting applications that perform smoothly when multiple connections might need access to the database at the same time. This careful management is crucial for ensuring that database interaction is smooth and efficient in any application.
1. **Ephemeral Connections**: Unlike some database systems where connections are kept open for extended periods, SQLite connections tend to be short-lived. It's generally considered a good practice to establish a connection only when you need it for a transaction, then close it right after. This helps keep resource usage down.
2. **Auto-Commit Behavior**: SQLite operates in auto-commit mode by default. This means if you don't explicitly commit your database commands, any changes will be discarded when the connection is closed. You need to be cautious about this when working with transactions to ensure you don't accidentally lose data.
3. **Memory Footprint**: Each SQLite connection takes up some memory. If you're dealing with large datasets or have a lot of concurrent connections—which goes against SQLite's usual purpose—being mindful of how you manage connections can prevent memory issues from becoming a problem.
4. **Thread-Safety Considerations**: When establishing a connection, the `check_same_thread` parameter in `sqlite3.connect()` lets you control whether or not the connection can be used across multiple threads. The default setting prevents this, potentially causing complications if not addressed carefully.
5. **Database-Level Locking**: SQLite locks the entire database during read and write operations. This means if a long write operation is ongoing, read operations could get blocked. While this ensures data integrity, it can negatively impact performance in applications with many users accessing the database concurrently.
6. **Connection Pooling Absence**: Unlike some advanced database systems that offer built-in connection pooling, SQLite doesn't provide this capability. So, if your application requires high availability or scalability, managing connections yourself can become a chore.
7. **Batch Inserts for Speed**: When adding multiple rows to a SQLite database, wrapping those inserts in a single transaction can deliver significant performance improvements. Committing everything at once minimizes the overhead of individual commit operations.
8. **Resource Contention Issues**: SQLite can encounter issues when many connections try to read or write simultaneously. Understanding how the `timeout` parameter can be adjusted and incorporating basic retry mechanisms can help to address these conflicts.
9. **Flexible but Potentially Inconsistent Typing**: SQLite has dynamic typing, meaning it doesn't enforce strict data types for columns. While flexible, this lack of type enforcement can lead to data inconsistencies if not properly managed.
10. **Error Handling Complexity**: SQLite returns a variety of error codes, making it essential for developers to put in place robust error handling. Being able to understand and interpret these error codes makes debugging easier and ensures that applications behave reliably when things go wrong within the database.
Understanding Python SQLite Connections vs
Cursors A Deep Dive into Database Interaction Efficiency - CRUD Operations Through Cursor Objects
Within Python's SQLite environment, CRUD operations are primarily handled through cursor objects. These objects act as intermediaries between your Python code and the database, enabling you to execute SQL statements for creating, reading, updating, and deleting data. This approach not only provides a structured way to interact with the database but also promotes cleaner and more maintainable code.
It's crucial to remember that any changes made through a cursor are not permanently stored until you commit the transaction. This emphasizes the need for careful management of the cursor's lifecycle to prevent resource issues. Cursors provide flexibility when retrieving data using methods like `fetchall()` and `fetchone()`. Their capability to manage various data retrieval scenarios makes them invaluable tools for any SQLite application. Essentially, cursors streamline the way developers interact with SQLite databases, offering an efficient method for data management within Python applications. However, be aware that improper management can lead to complications like resource hogging and locking issues.
1. **Cursor's Transient Nature:** In contrast to database connections, which require careful management due to their resource-intensive nature, cursors are designed to have a short lifespan. Their creation and access are relatively lightweight operations, making them well-suited for executing SQL commands efficiently without the overhead of maintaining a persistent object. This makes them very convenient for short-lived actions.
2. **Automatic Resource Cleanup:** Cursors manage their memory allocations internally and are automatically disposed of when the associated database connection closes. This feature simplifies resource management in applications, helping prevent memory leaks and ensuring that resources are released promptly. It simplifies our life a lot.
3. **Preventing SQL Injection:** Using cursors, developers can employ parameterized queries as a defense mechanism against SQL injection attacks. Parameterized queries provide a secure method to interact with the database as the values are treated as data, not as part of the query itself, thereby preventing malicious input. This is a significant improvement over the alternative of including user-provided data directly within SQL queries. Also, this method provides SQLite with the opportunity to optimize the execution plan of a query, improving performance for frequently used queries.
4. **Batching for Performance**: Cursors can execute multiple SQL statements in a single batch, a strategy that can lead to substantial performance gains. This capability lets us bundle many database interactions into a single operation instead of sending multiple requests, which reduces the overhead associated with sending and processing commands one at a time. Though it might sound a minor improvement, for busy databases, every bit counts.
5. **Tailoring Result Presentation:** SQLite cursors provide a unique level of adaptability by allowing us to specify how query results are presented through row factories. With row factories, developers can control the way that rows are represented as Python objects, adding a layer of customization to how data is processed in the application. It gives us more control than we might need in simple database applications.
6. **Centralized Error Handling:** Cursors contain error handling logic specifically for the execution of SQL statements, simplifying the overall handling of errors in applications. This local approach to error handling provides better organization and makes error management easier in complex applications, particularly when dealing with numerous different database interactions.
7. **Reusing Cursors for Efficiency:** As long as their use does not interfere with ongoing database operations, cursors can be reused to execute multiple SQL commands. This allows us to avoid re-creating cursors repeatedly, saving time and resources, particularly useful when our applications interact with the database in various ways during a single execution or a series of related operations. This can streamline the code and simplify application logic.
8. **Single-Command Focus**: Each cursor operates on a single SQL statement at a time. This focused approach keeps things simpler, reducing potential for ambiguity or confusion. Having only one SQL command active at a time in a cursor promotes clearer execution logic, allowing us to keep better track of what each part of the code is doing in relation to the database.
9. **Flexible Result Retrieval**: The results of queries executed through cursors can be retrieved in a variety of ways, such as row-by-row or in larger batches. This gives us flexibility to tailor data retrieval to the application’s specific needs. While this might appear to be only a feature that benefits specific performance issues, it gives us a level of control over how the program accesses the data, making it more adaptive to various application requirements and constraints.
10. **Concurrency Challenges:** While SQLite supports running multiple cursors concurrently through a single database connection, improper management of these cursors can lead to performance issues and contention. This emphasizes the importance of handling cursors carefully. It underscores the importance of proper lifecycle management of cursors to prevent performance degradations in multi-user or high-concurrency scenarios. SQLite's inherent concurrency model doesn't offer the same level of sophistication as some other database systems, so developers must carefully consider these aspects when designing their applications.
Understanding Python SQLite Connections vs
Cursors A Deep Dive into Database Interaction Efficiency - Best Practices for Cursor Lifecycle Management
Within the context of Python's SQLite interactions, properly managing the lifecycle of cursors is essential for achieving efficient and robust database operations. Utilizing context managers is a recommended approach, as they streamline the process of opening and closing cursors automatically. This automated handling helps to prevent situations where cursors remain open unnecessarily, potentially causing resource leaks or database locks.
When tackling more intricate operations, it's crucial to exercise caution when employing explicit cursors. While offering greater control, relying solely on implicit cursors for all situations might restrict the flexibility and efficiency needed for more complex queries. It's important to close cursors immediately after you're finished with them, and ensure that any database changes are explicitly committed to guarantee data integrity and resource release.
Adhering to these best practices for cursor lifecycle management doesn't just boost performance, but also enhances the overall quality and maintainability of your code when building applications that interact with SQLite databases. While SQLite is often associated with simplicity, neglecting proper cursor management can introduce complications that hinder performance and introduce unnecessary complexity into your application's logic.
When working with SQLite in Python, cursors act as intermediaries, managing the execution of SQL statements within a database connection. They're surprisingly handy, offering ways to make your code efficient and secure. However, understanding their lifecycle is crucial to prevent potential issues.
One thing that's quite useful is the automatic deallocation that occurs when you close the related connection. This feature automatically cleans up cursors, reducing the chance of memory issues and making resource management easier. This automatic handling makes things less complicated for everyday operations.
Cursors are also adept at optimizing query performance through the use of batch executions. Running several SQL statements at once significantly reduces the overhead associated with repeatedly establishing connections and executing separate commands. This ability is particularly valuable when you need to process a lot of data at once.
Another beneficial feature is the use of parameterized queries. Not only are these queries a security boon, as they prevent SQL injection attacks by treating user inputs as data, but they also allow SQLite to create more efficient execution plans. This, in turn, can lead to improvements in the performance of often-used queries.
Cursors allow you to dictate the output format of result sets through row factories. This flexibility is great for customizing how data is represented within your application. This added flexibility can be helpful in tailoring the application to certain needs or presentation requirements.
However, despite their usefulness, there are a few things to keep in mind. Each cursor can only execute one command at a time. While this might seem like a minor detail, it can cause problems if you're not careful about managing multiple commands. You wouldn't want to accidently initiate conflicting operations simultaneously, it could end up leading to unexpected behavior.
In situations with many users or multiple operations happening at once, improperly managed cursors can lead to resource contention issues. This is especially true since SQLite is fundamentally a single-threaded database. This means that several transactions occurring at the same time may cause unexpected delays or errors unless handled with care.
Cursors also have their own error handling capabilities, which makes identifying and troubleshooting issues easier. This feature keeps the handling of errors organized, which is beneficial in large or complex applications.
Additionally, cursors are intended for short-term use. This means you should create a cursor when needed and not keep it hanging around. This helps avoid resource waste and promotes a more efficient usage pattern.
Another noteworthy fact is that SQLite, while allowing multiple cursors through a connection, still faces potential concurrency problems. This limitation highlights the need for careful consideration when managing concurrent transactions to ensure application responsiveness.
Finally, any actions performed through a cursor are temporary until you explicitly commit them. This is a good thing, since it allows us to rollback changes. But, if not properly managed, it can result in errors where data modifications are inadvertently lost.
By keeping these unique attributes of cursors in mind and incorporating best practices, developers can fully leverage their capabilities to create more performant and stable applications that interact with SQLite databases. It requires careful planning, especially in larger and more complex database applications.
Understanding Python SQLite Connections vs
Cursors A Deep Dive into Database Interaction Efficiency - Connection Commits and Transaction Handling in SQLite
SQLite's connection commits and transaction handling are vital for ensuring data accuracy and efficient database interactions. Transactions bundle a set of database operations, treating them as a single unit of work. This means either all the operations within a transaction are completed successfully and saved to the database (committed), or if any part of the transaction fails, all changes are reversed (rolled back). To finalize changes made within a transaction, you must explicitly use `connection.commit()`. When utilizing the `with` statement to manage a database connection, SQLite automatically handles transaction rollbacks if errors occur, safeguarding data consistency. Properly managing transactions is key to building stable and reliable applications, especially when dealing with multiple database operations. Understanding the intricacies of transaction handling can help developers avoid issues such as resource conflicts and database locking, leading to more responsive and efficient applications.
1. **Connection's Fleeting Nature**: SQLite connections are typically short-lived, best suited for quick, transaction-focused interactions. Keeping connections open longer than needed can lead to unnecessary resource usage and potential lock contention. This ephemeral nature promotes efficient database resource management.
2. **Implicit Transaction Behavior**: SQLite's default transaction behavior is a bit unusual. If you don't explicitly start a transaction, each SQL statement gets its own mini-transaction, potentially causing a lot of overhead due to frequent commits. Understanding this subtle aspect is vital for optimized database performance.
3. **Tailoring Result Handling**: We can change how the results of database queries are presented in Python by using "row factories". This lets developers shape how data is retrieved, potentially optimizing the retrieval process for various application needs. This level of fine-tuning can be helpful in different situations.
4. **Thread-Safety Management**: When establishing a connection, we have the option to control if it can be used by multiple threads at once using the `check_same_thread` parameter. This feature manages concurrency and potentially simplifies error handling in multithreaded Python applications. It's a valuable safety net to consider when designing.
5. **Concurrency Limitations**: SQLite has a rather simple concurrency model—it locks the entire database when doing any write operation. This means that even reads can be blocked, which, while ensuring data integrity, can lead to performance problems when many users are accessing the database. It's an area to be aware of when building high-traffic applications.
6. **Prepared Statements**: Parameterized queries are a great tool that not only protect against SQL injection attacks but also help SQLite optimize queries. It can reuse query execution plans for similar queries, enhancing performance over time. This method is valuable, especially when dealing with frequent or repetitive database operations.
7. **One Command at a Time**: Cursors within SQLite only handle a single SQL command at any given moment. This simplifies things, but we need to be careful when dealing with multiple database operations. This design can introduce some complexity when working with complex multi-step operations involving concurrent SQL statements.
8. **Automatic Cursor Cleanup**: When the associated connection is closed, SQLite automatically releases any cursors, a useful feature not always present in database interactions. This automatic disposal of cursors minimizes the risk of resource leaks and removes a potential source of errors. It helps keep the code cleaner and less prone to issues.
9. **Batch Operations Benefit**: We can significantly speed things up by using transactions that encompass multiple SQL commands. This helps minimize overhead caused by repeated commits. This approach is especially useful when dealing with large batches of inserts or updates. It's a notable technique that impacts database interaction speed.
10. **Detailed Error Handling**: SQLite has a rich set of error codes, aiding in pinpointing database problems. Having a structured error-handling approach around these codes can make debugging simpler and enhance the overall stability of applications. It provides valuable insight for diagnosing and resolving database issues in a comprehensive manner.
More Posts from aitutorialmaker.com: