Create AI-powered tutorials effortlessly: Learn, teach, and share knowledge with our intuitive platform. (Get started for free)
7 Intriguing Python Challenges to Boost Beginner Skills in 2024
7 Intriguing Python Challenges to Boost Beginner Skills in 2024 - Print Message Challenge Builds Console Output Skills
Starting your Python journey with the "Print Message Challenge" is a smart move. This seemingly simple task of using the `print` function to display text or other data on the console is foundational. It introduces you to the core concept of Python's output capabilities and helps you grasp how different data types – like strings and numbers – are presented. While basic, this challenge establishes a crucial link to later, more intricate projects, especially when dealing with manipulating data in libraries like Pandas. It's a powerful reminder that consistent practice through coding exercises is crucial for building a solid foundation in any programming language. Conquering this initial hurdle boosts your confidence and encourages a deeper understanding of Python's capabilities.
The "Print Message" challenge, a cornerstone of introductory Python exercises, serves as a springboard for developing fundamental console output skills. It's through this simple task that learners become acquainted with the `print()` function, a vital tool for displaying information to the user. This function, capable of handling a range of data types, from simple strings to more complex structures, is a crucial building block for any Python programmer.
While seemingly straightforward, the "Print Message" challenge also subtly introduces aspects of control flow. Programmers are encouraged to manipulate when and how the message is printed, potentially utilizing loops or conditional statements. This practice can lead to a deeper understanding of how a program's logic influences output.
However, the challenge also underscores the importance of debugging. Unexpected outputs prompt learners to carefully examine their code, enhancing their problem-solving and attention to detail. While many readily available debugging tools can speed up this process, beginners might not immediately leverage them, providing a learning opportunity on efficient troubleshooting.
Beyond its core purpose, the challenge contributes to a more holistic understanding of Python. It highlights the interrelationship between data types, particularly string manipulation, and paves the way for understanding how type-related issues might surface in more intricate scenarios. Integrating this exercise with other code segments or larger projects emphasizes the relevance of print statements in contexts like logging or user interface interactions.
It's worth noting that, while print statements might appear insignificant, their use can impact performance, especially in large-scale applications. This aspect serves as an early reminder for responsible coding habits. It's also a chance to advocate for the importance of well-commented code, particularly when explaining print statement functions within the context of a larger program.
Furthermore, the Print Message Challenge serves as an excellent starting point for exploring collaborative learning. In team settings, comparing different solutions unveils varied approaches and programming styles, enriching the overall learning experience. This collaborative aspect enhances a team's understanding of best practices and code optimization.
Finally, the challenge naturally prompts learners to investigate more advanced string formatting options such as f-strings or the `format` method. This gradual progression encourages a desire to enhance their coding abilities and expand their skillset within Python.
7 Intriguing Python Challenges to Boost Beginner Skills in 2024 - Radians to Degrees Converter Sharpens Math Programming
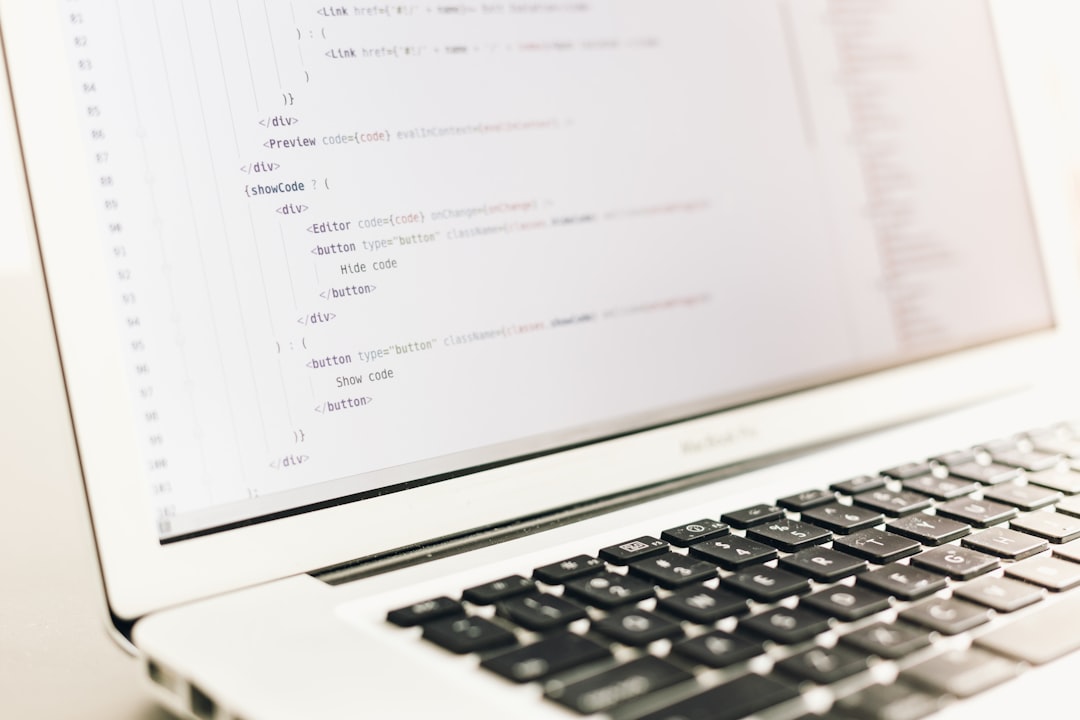
The "Radians to Degrees Converter" challenge is a valuable exercise for beginners to sharpen their math programming skills within Python. It highlights the importance of understanding angle conversions and how they relate to various applications, particularly those using trigonometric functions. The `math` module's `math.degrees()` function makes this conversion process remarkably easy, illustrating the fundamental relationship between radians and degrees. Furthermore, this exercise can be expanded upon by using libraries like NumPy for conversions involving arrays, introducing concepts of efficient data handling.
While the core of the challenge lies in the straightforward conversion, it subtly underscores the need for mathematical understanding within a programming context. Trigonometric functions, for example, heavily rely on radians, so appreciating this relationship is essential for accurately interpreting mathematical outcomes within code. By tackling this type of challenge, beginners get a hands-on introduction to how mathematical concepts directly translate into practical code. This not only reinforces fundamental math knowledge but also equips them with a stronger foundation for approaching more advanced Python programming topics in the future. It's a reminder that mastering core concepts like this forms a strong base for tackling increasingly complex problems.
1. **Radians and Degrees: A Fundamental Difference**: While both radians and degrees measure angles, radians are intrinsically linked to the circle's radius, making them a more natural choice for mathematical computations in code. It might surprise some to discover that one radian is roughly 57.2958 degrees.
2. **Circle's Radius and Radians**: The concept of radians is rooted in the circle's radius. One radian is the angle formed at the circle's center when the arc length equals the radius. This underscores a vital connection between geometry and trigonometry that often goes unnoticed in initial programming.
3. **Python's Trigonometric Function Preference**: Many programming languages, including Python, default to radians for trigonometric functions like sine and cosine. Overlooking this can introduce significant errors in calculations, especially when developing graphical programs or simulations.
4. **Calculus and Radians**: The significance of radians extends to calculus, where derivatives of trigonometric functions like sine and cosine are elegantly defined using radians. This often gets overlooked by beginning programmers.
5. **Cyclic Nature of Angles**: Grasping the cyclic nature of angles, whether measured in radians or degrees, is quite valuable. For example, an angle of 2π radians (or 360 degrees) signifies a complete rotation, directly impacting animations and movement in coding projects.
6. **Building a Radians to Degrees Converter**: A simple "Radians to Degrees Converter" can be both a practical coding exercise and a valuable learning experience. This deceptively simple project allows programmers to refine arithmetic operations and function building, emphasizing the need for accurate calculations.
7. **Accuracy Concerns in Conversion**: When switching between radians and degrees, precision is vital. The floating-point math used in programming can lead to rounding errors, particularly relevant in applications involving physics simulations or graphical transformations.
8. **Triangle Geometry and Angles**: The conversion between radians and degrees is intimately related to triangle geometry. In a right triangle, the angles and their radian equivalents are fundamental for understanding the relationships within the triangle, which can be essential for geometry-focused programs.
9. **Historical Roots of Radians**: The utilization of radians has historical roots, emerging from mathematicians' efforts to simplify circle and angle calculations. Understanding this historical perspective can add a deeper dimension to a beginning programmer's education.
10. **Programming Libraries and Radians**: Many Python libraries, such as NumPy and SciPy, rely on radians for mathematical operations. This reinforces the importance of understanding unit conversions when using external libraries, as misinterpretations can lead to faulty results or inefficient code.
List Sorting Check Strengthens Data Structure Knowledge
Developing a grasp of how to sort lists in Python is vital for anyone wanting to better understand data structures. Python offers two primary ways to sort: `sort()` directly alters the list it's called upon, while `sorted()` generates a fresh, sorted list without touching the original. This difference is particularly important when dealing with diverse data types and structures, from simple lists to intricate arrangements like nested lists or dictionaries. Using the 'key' argument, learners can customize sorting rules, which is critical for tackling real-world data challenges. Learning these sorting methods not only strengthens a person's coding skills but also prepares them for more advanced algorithm design and managing data across different types of programs.
Exploring list sorting in Python can surprisingly lead to a deeper grasp of core data structure principles. For instance, Python offers two key methods for sorting: `sort()`, which directly modifies the list it's called upon, and `sorted()`, which creates a new, sorted list while leaving the original untouched. Understanding these differences is crucial.
Delving further, the `sort()` method accepts a 'key' parameter—often a lambda function—to customize the sorting logic. This allows us to sort based on specific components of sublists or even more intricate data structures, offering a flexible approach to organizing data. Moreover, `sorted()` can handle diverse data types like tuples, sets, strings, and dictionaries by converting them into a sorted list, indicating the breadth of its applicability.
Speaking of strings, when you sort strings with `sorted()`, it effectively treats them as sequences of characters and returns a sorted list of those characters, demonstrating the underlying character-by-character approach.
Beyond fundamental list operations, a common scenario involves sorting lists of lists. Typically, this involves specifying a certain index from within the sublists to determine the sorting order. This is a good example of how sorting directly relates to how we handle more intricate data organization. This highlights the need to understand how to manipulate the structure of a list during the sorting process.
It's important to note that in Python, lists are mutable, meaning their order can be easily adjusted by modifying elements directly. Data structures like Pandas Series, for instance, can use the `sort_values()` method, which is similar to how we sort DataFrames, broadening the scope of sorting techniques beyond basic lists.
Thinking more strategically, the decorator pattern can be used to modify list values temporarily during the sorting process, then reverting back to the original values once the sorting is done. This kind of approach highlights Python's flexibility for implementing efficient sorting strategies.
Further, Python lists have this neat feature of holding various data types in a single list. This could involve a mix of integers, strings, and even other lists within a single list, providing an incredible degree of flexibility in how we manage information.
All of this points towards the pivotal role of sorting in Python programming. The ability to sort elements effectively is a critical skill for any Python beginner, laying the groundwork for essential data manipulation and efficient algorithm development. However, it is important to analyze the specific situation in order to decide the most appropriate sorting method for a given task. This knowledge helps us develop a deeper and more informed approach to solving problems.
It's intriguing how this seemingly simple idea of sorting a list leads to such a rich understanding of how data structures and algorithms function. As we navigate more sophisticated Python programming in the future, having a strong foundation in list sorting proves to be extremely valuable.
7 Intriguing Python Challenges to Boost Beginner Skills in 2024 - Binary to Decimal Conversion Deepens Number System Understanding
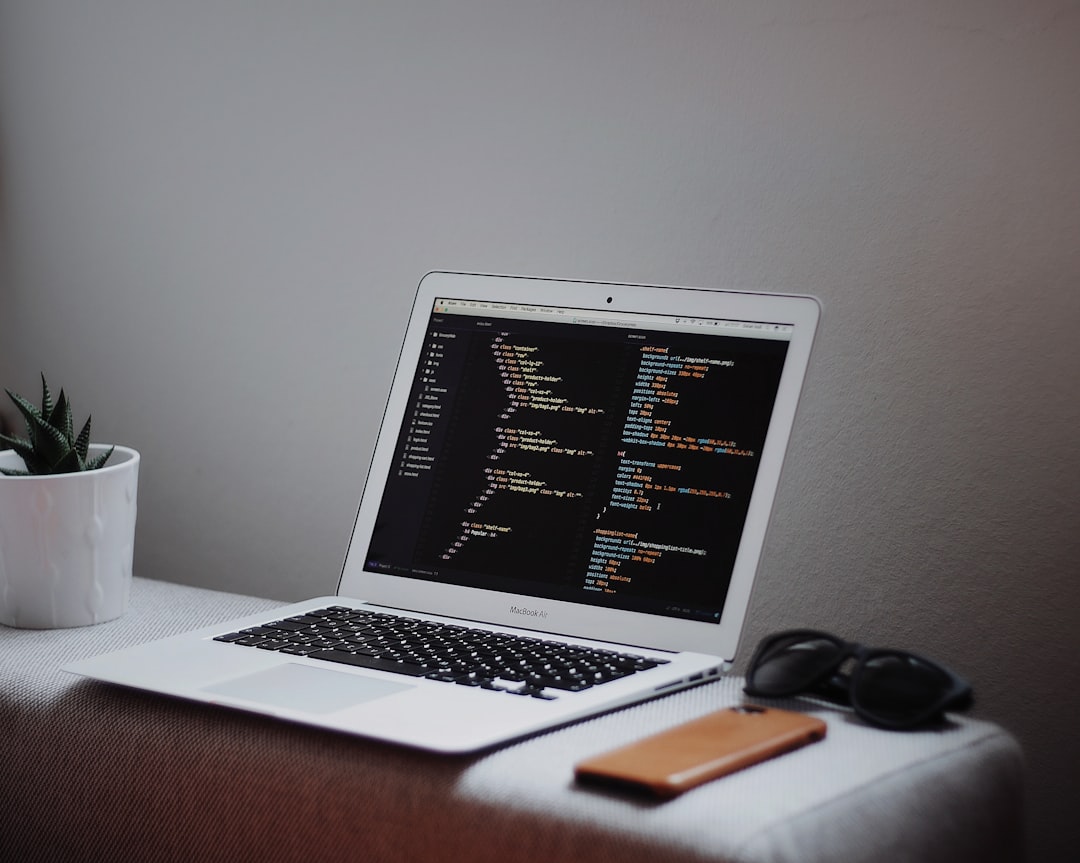
Comprehending how binary numbers translate into decimal form is key to understanding diverse number systems within the context of programming. The binary system, relying exclusively on 0 and 1, lies at the heart of computer science and digital technology. As a result, beginners must develop a strong grasp of this fundamental system. While Python makes binary to decimal conversion relatively simple using tools like the `int()` function, there are other paths to explore, such as iterative approaches with loops. This not only strengthens problem-solving abilities but also fosters a broader understanding of how various number systems are connected. Tackling challenges that involve this conversion process improves coding practice and helps build a deeper awareness of the logic and architecture that underpins languages like Python. Ultimately, this knowledge strengthens the base for future endeavors in computer science.
Delving into binary-to-decimal conversion provides a deeper appreciation for how number systems function, a core concept in computer science and programming. The binary system, utilizing just two digits (0 and 1), starkly contrasts with the familiar decimal system (base-10). This difference highlights how numbers can be represented in fundamentally different ways.
Python offers several approaches for performing this conversion. The `int()` function, coupled with a base parameter of 2, efficiently converts a binary string to its decimal equivalent. Alternatively, one can craft custom functions, perhaps leveraging iterative loops or employing weighted bit positions to understand the conversion process more granularly. The `bin()` function offers a complementary perspective by converting decimal numbers into binary, illustrating the conversion process in reverse.
Beyond the practical methods, exploring the underlying principles of binary representation is illuminating. Each digit, or "bit," holds exponential significance. For example, in an 8-bit number, the leftmost bit represents 2⁷ (128 in decimal), while the rightmost bit represents 2⁰ (1 in decimal). This exponential growth is crucial for understanding how relatively small binary numbers can translate into large decimal values.
Furthermore, the binary system's inherent connection to computer architecture is fundamental. Transistors in processors, the building blocks of computers, exist in two states: on or off, which are represented by 1s and 0s. This physical constraint is why binary forms the bedrock of how computers process and store information.
It's also worth noting that the concept of binary numbers is not a modern invention. Although it wasn't fully developed until 1703, when Gottfried Wilhelm Leibniz formally introduced it, other civilizations have shown hints of it in their systems of counting and calculations throughout history.
Exploring these aspects—from the mathematical underpinnings to the practical Python methods and the deeper connection to the field's history—helps solidify the significance of binary and decimal conversion. It reinforces the idea that a thorough understanding of number systems is essential for anyone striving to understand how computers function at a deeper level. While seemingly simple at first, this conversion problem highlights a core facet of computing and provides valuable insights into various fields of study within computer science. It serves as a bridge between fundamental mathematical concepts and the practical application of algorithms that are central to modern computing.
7 Intriguing Python Challenges to Boost Beginner Skills in 2024 - Loves Me Not Game Improves String Manipulation Abilities
The "Loves Me Not" game presents a fun way for beginners to build up their string manipulation skills in Python. The game involves assessing a particular string pattern and making decisions based on how that string is structured. This involves using techniques like string slicing and possibly string justification. It demonstrates how string operations are not just theoretical, but very helpful in creating code that is both readable and effective. For budding programmers, gaining a firm grasp of string manipulation is a crucial first step. It lays the foundation for tackling more advanced coding projects as they progress in software development. This type of exercise can help beginners build confidence and competence in their Python programming journey.
One of the Python challenges geared towards beginners, particularly for improving string manipulation skills, is the "Loves Me Not" game. This game likely revolves around evaluating specific string patterns and might involve decision-making based on string operations. It's part of a set of beginner-friendly challenges that also includes sorting list checks, binary-to-decimal conversions, and generating the Tribonacci sequence.
Python's extensive string manipulation capabilities are crucial for writing readable and effective code. You can learn techniques like left-justifying strings, as illustrated by using the `ljust` method. A wide variety of practice resources exist, encompassing 15 dedicated string exercises and 18 broader problems designed to solidify beginners' skills in string manipulation.
Understanding string slicing is essential for extracting substrings using colon notation. It's a fundamental skill for tackling various software development challenges. Python's built-in string methods streamline text data handling and contribute to more maintainable projects.
There's a consistent theme of advocating for continuous learning in the Python community. Various resources recommend daily practice of Python tricks, including string-related exercises, to progressively improve coding skills. While it is sometimes suggested as a tool to motivate learning, it's unclear whether the notion of daily practice has any evidence base for success. It's easy to understand why developers in the Python community would recommend frequent practice, however, as they are well aware that string manipulation is a common component across a broad range of applications. Nonetheless, I remain curious to see if this is ultimately proven effective.
7 Intriguing Python Challenges to Boost Beginner Skills in 2024 - Tribonacci Sequence Generator Introduces Basic Recursion
The Tribonacci Sequence Generator provides a good way for beginner Python programmers to learn about recursion. This sequence works by adding up the three previous numbers to get the next number, starting with 0, 0, and 1. Writing a recursive function to generate this sequence helps new coders understand how function calls work and why base cases are necessary for preventing infinite loops. While this is a good learning opportunity, it's important to remember that a basic recursive solution can be slow due to repetitive calculations. Learning how to improve the efficiency of a recursive solution by using techniques like memoization not only sharpens programming skills, but also adds a practical dimension to this particular coding challenge.
The Tribonacci sequence, a fascinating cousin of the more familiar Fibonacci sequence, introduces the concept of recursion in a beginner-friendly way. Each number in the Tribonacci sequence is the sum of the three preceding numbers, typically starting with 0, 0, and 1. This simple rule, expressed mathematically as \( T(n) = T(n-1) + T(n-2) + T(n-3) \), highlights how recursion can capture intricate patterns within programming.
However, the inherent recursive nature of the sequence can lead to a bit of a trap. For larger values of \( n \), the depth of recursion needed to generate the \( n \)th term can become quite substantial, which may lead to a stack overflow error. This practical problem underscores the importance of understanding recursion limits and proper error handling in code.
Interestingly, the straightforward recursive implementation can be quite inefficient due to repeated calculations of the same values. A neat technique called memoization, which involves caching previously computed values, can significantly enhance the performance of the function. This offers an early introduction to optimizing algorithms, a skill crucial as programmers progress in their journey.
Beyond these technical aspects, the Tribonacci sequence surfaces in some unexpected places, such as within computer graphics and population growth modeling. This illustrates how abstract mathematical sequences can find practical applications in the real world. We can also visualize the Tribonacci sequence using a binary tree, where each node has three branches. This kind of representation is useful for understanding the branching pattern inherent in recursive functions and how they connect to data structures.
Comparing the Tribonacci and Fibonacci sequences reveals how the growth of the numbers in each sequence unfolds. Interestingly, the Tribonacci sequence grows even faster than Fibonacci numbers, creating an opportunity for researchers to learn more about different kinds of recurrence relationships. The computational complexity of a naive recursive Tribonacci generator is exponential, denoted as \( O(3^n) \). This rapid growth highlights the impact of algorithm design on efficiency and helps illustrate the need for understanding this aspect as coders move onto more advanced problems.
The Tribonacci numbers also hold a fascinating relationship with the roots of a specific polynomial equation, namely \( x^3 - x^2 - x - 1 = 0 \). This illustrates the surprising connection between seemingly simple programming tasks and the underlying mathematical structures that give them form. We can also convert a recursive Tribonacci function into a dynamic programming solution, which demonstrates how the optimization techniques of storing values in an array (for example) can impact memory and runtime.
Overall, the Tribonacci sequence provides a fun and informative starting point for exploring recursion. It allows new programmers to experiment with different recursive implementations, perhaps even creating visual representations of the sequence. It is a reminder of the interconnectedness between mathematical patterns, computational processes, and algorithm optimization, all of which are critical to mastering the fundamentals of programming.
7 Intriguing Python Challenges to Boost Beginner Skills in 2024 - Credit Card Number Masker Enhances Data Privacy Skills
The "Credit Card Number Masker" challenge provides a practical avenue for beginners to hone their data privacy skills within Python. This involves techniques like masking sensitive data by replacing parts of a credit card number with asterisks, highlighting the crucial role of safeguarding personally identifiable information. The challenge might incorporate libraries like Faker to generate synthetic data, allowing learners to experiment with data masking in a safe environment. Concepts like k-anonymity, explored through the use of Pandas, introduce how data can be modified while preserving a level of user privacy. Furthermore, newer Python versions, through their use of f-strings, streamline the implementation of these masking techniques. This demonstrates how the coding landscape is adapting to modern data security and privacy demands. By engaging in this challenge, new programmers not only strengthen their coding abilities but also begin to develop a strong awareness of the ethical dimensions that come with working with sensitive data.
Credit card number masking, a technique that involves hiding parts of a credit card number, is a fascinating way to enhance data privacy. This method, often implemented by replacing certain digits with asterisks, serves as a guard against potential data breaches and unauthorized access, particularly crucial in the context of financial information.
Python provides several means to achieve this, offering a great way to learn about data masking and security. Some methods involve replacing portions of the credit card number with asterisks or utilizing string operations to only retain a subset of the digits, such as the last four. Libraries like Faker can also be valuable in generating fake data and replacing sensitive information with placeholder data during testing.
Additionally, a privacy model called K-anonymity can be applied using Python and libraries like Pandas to ensure that individuals within a dataset are not easily identifiable. This method attempts to ensure a degree of anonymity for each individual, though it's important to note that this is not a failsafe method for preventing identification.
Recent Python versions like Python 3.6 or later introduced f-strings, which makes working with string formatting, including the masking of credit card numbers, a lot simpler. It demonstrates how Python is constantly evolving and improving to meet the needs of its developers and users.
Masking is not the only technique to consider. Data suppression, data generalization, and the creation of synthetic data are also examples of techniques for protecting sensitive information, showing the breadth and depth of tools available to secure data.
When developing a credit card masker, decisions need to be made regarding the quantity of digits to expose or mask. This process might require calculating the difference between the total number of digits in a credit card and the number of digits to mask. Understanding the tradeoff between transparency and security is key in this process.
The protection of Personally Identifiable Information (PII), like credit card information, is paramount. Without proper protections, there is a risk that unauthorized parties could access and use PII for malicious purposes.
The need for data privacy in handling sensitive information like credit card numbers is vital. It's a responsibility for developers to consider how to design and implement systems that protect users from potential security breaches. There are online educational resources like courses available on DataCamp that explore anonymization strategies and masking in Python, including other practices and techniques, providing accessible pathways for beginners to learn about this important topic.
While the implementation can be simple, I think that the decision of how much to mask is more challenging than it might first appear. The user experience is a core consideration as users might not readily trust applications that present their data in a masked format. There can be an uncomfortable tension between trying to secure data while remaining user-friendly. I remain curious as to whether there are potential unintended consequences associated with the use of masking techniques.
Create AI-powered tutorials effortlessly: Learn, teach, and share knowledge with our intuitive platform. (Get started for free)
More Posts from aitutorialmaker.com: