Create AI-powered tutorials effortlessly: Learn, teach, and share knowledge with our intuitive platform. (Get started for free)
7 Essential PyGame Functions for Creating Your First 2D Game Prototype in 2024
7 Essential PyGame Functions for Creating Your First 2D Game Prototype in 2024 - Initialize Game Window and Clock with pygame.init() and pygame.display.set_mode()
To get your Pygame game off the ground, you'll need to prepare the game window and a clock for managing time. First, `pygame.init()` is essential for initializing all the Pygame modules your game relies on. Next, `pygame.display.set_mode()` establishes the game window, defining its size. This function returns a Surface object, acting as your canvas for drawing game elements. Furthermore, `pygame.time.Clock()` creates a clock object that plays a crucial role in controlling your game's framerate and ensuring smooth animations. By initializing these elements at the outset, you set the stage for a visually appealing and responsive gaming experience.
To get started with Pygame, you need to initialize all the imported modules using `pygame.init()`. This function returns a tuple indicating the success of initialization, which can be handy for debugging and identifying issues early on. It's a good practice to examine the return values if you're curious about the inner workings.
Next, the `pygame.display.set_mode()` function creates the window for your game. It's more than just a basic window; it allows you to choose from various modes, such as fullscreen or resizable windows, by supplying corresponding flags like `pygame.FULLSCREEN` or `pygame.RESIZABLE`. This level of control is crucial for adapting your game to different environments.
Beyond the window, Pygame has a built-in `Clock` object. This object is incredibly important for controlling the game's frame rate, which impacts performance and ensures consistent gameplay across diverse hardware. Maintaining a stable frame rate helps to create a smooth and enjoyable experience.
A crucial aspect to consider early on is the Pygame coordinate system. The top-left corner of the game window is the origin (0,0). The x-axis extends horizontally to the right, and the y-axis goes vertically downward. Understanding this system is vital for accurately positioning objects within your game world.
Furthermore, it's important to handle events effectively after setting up the game window. Events, such as user input, need to be handled in a consistent loop, or the game window can freeze and become unresponsive. It's like the window needing a constant stream of feedback; otherwise, it's unable to respond to actions and can quickly become useless. This is why game design often emphasizes consistent event management.
Maintaining a smooth refresh rate is vital to prevent flickering and to ensure a visually pleasant gaming experience. Pygame's functions like `flip()` and `update()` are the key tools for managing the content on the display surface. A quick visual refresh is often desirable and keeps the player's visual experience from becoming jarring or flickering.
The window that `pygame.display.set_mode()` creates is a "surface." This means it can serve as a canvas for your game's visual components, so you're not limited to just a basic game container. It functions as a core space onto which you can draw using Pygame's various rendering tools and functions, effectively creating the visual experience of your game.
Although not typical, Pygame lets you create multiple windows through `pygame.display.set_mode()` using different display surfaces. This could be useful in scenarios involving more elaborate user interfaces or specific tool development, although these are not that common.
Finally, the window itself has customizable aspects, like the title and icon, which you can manage with `pygame.display.set_caption()` and `pygame.display.set_icon()`. These aspects contribute to the game's identity and presentation. How a player perceives a game starts even before playing, so you should not underestimate the value of these components, as they can influence first impressions.
7 Essential PyGame Functions for Creating Your First 2D Game Prototype in 2024 - Create Game Objects Using pygame.sprite.Sprite for Basic Collisions
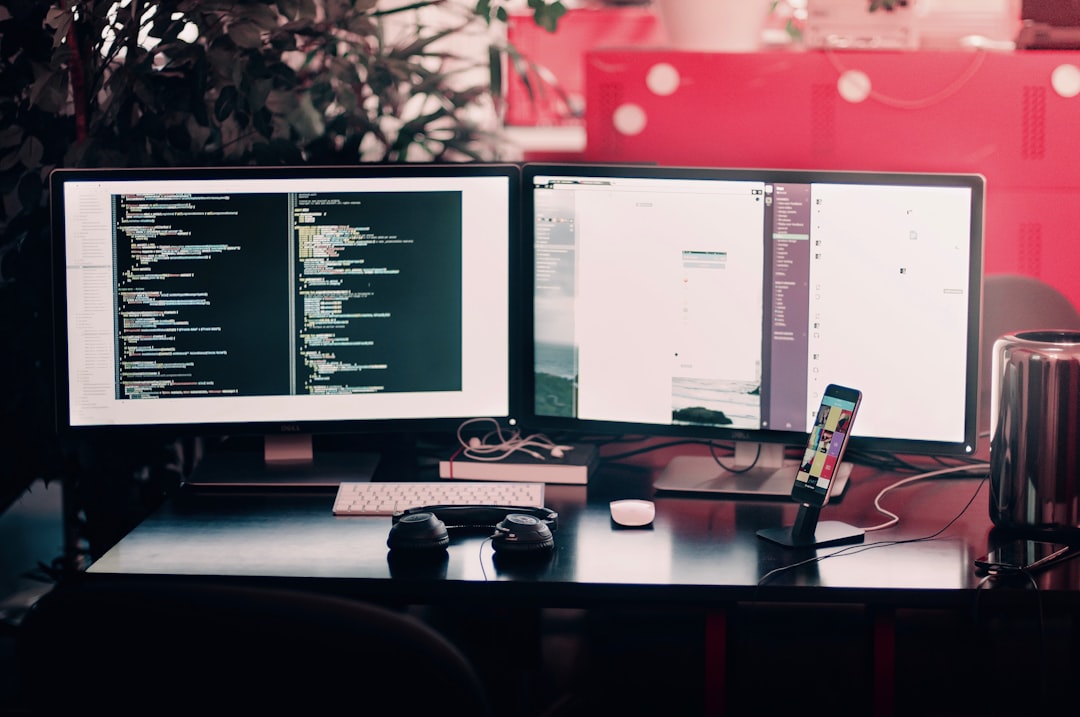
When crafting 2D games in Pygame, using the `pygame.sprite.Sprite` class is a key technique for establishing basic collision detection among game elements. Essentially, you structure your game's objects, like characters, projectiles, or obstacles, as sprites. This approach lets you take advantage of Pygame's integrated collision features, which can improve the management and efficiency of interactions between these objects.
The `pygame.Rect` class offers a simple way to determine if two objects overlap, which forms the basis of collision detection. Simultaneously, using the Sprite class helps structure how the game entities appear and behave, which in turn enhances the overall performance of your game. Pygame also includes a Group class that facilitates managing a collection of sprites. You can apply operations such as drawing or updating all the sprites in a group at once, resulting in code that is cleaner and more effective.
Understanding how sprites and the collision detection features work is crucial for developing fun and interactive game mechanics. These foundational mechanics, in turn, include factors like player movement, actions a player takes, and how various objects in the game interact. By properly leveraging these Pygame functions, you can craft a more dynamic and engaging 2D game prototype.
In Pygame, collision detection, a core aspect of game development, is often achieved using the `pygame.sprite.Sprite` class, which forms the foundation of object-based collision management. While the `pygame.Rect` class offers basic collision capabilities through its `colliderect` method, it's the `Sprite` class that offers a more structured and organized approach. The `Sprite` class, in essence, serves as a blueprint for creating the various entities within the game, allowing for streamlined management of objects and efficient collision detection.
Beyond basic collision checks, Pygame provides `pygame.sprite.Group`, a collection of sprites that facilitates batch operations. This simplifies tasks such as drawing multiple sprites simultaneously or performing collective collision detection across groups. It's a welcome efficiency boost when dealing with a multitude of game objects.
However, simply using sprites for collision detection does not tell us how it works or when to use these features. For basic collision checks, each sprite is paired with a `rect` attribute, a `pygame.Rect` object representing the sprite's bounding box. This enables simple collision detection between rectangular regions. While efficient and simple to implement, this method limits the complexity of the collision shapes involved. This approach appears sufficient for simple cases and scenarios involving primarily rectangular game elements, like boxes and bricks.
Pygame also leverages an optimization known as "dirty rectangles". Essentially, only portions of the screen altered during a frame are refreshed. This approach helps reduce the load on the system and allows for smoother gameplay, especially with many objects on-screen. This optimization highlights the design choices that impact performance in the context of game development.
Moreover, the built-in collision detection methods provide more than just overlaps. Functions like `collide_rect()` and `collide_circle()` enable response mechanisms to collision events. This allows for more interactive gameplay where objects don't merely bump into each other, but react based on the type of collision or game logic involved.
Moving beyond this, Pygame offers a means to layer sprite groups using `pygame.sprite.LayeredUpdates`. This functionality allows for a clear visual order, where some sprites are automatically rendered in front of or behind others. This feature helps in managing the visual hierarchy without having to manually implement complex rendering procedures.
Further simplifying sprite management is the internal reference counting system. Each sprite keeps track of how many other groups or objects are referencing it. When a sprite is no longer referenced, Pygame automatically removes it, effectively eliminating the need for manual cleanup and helping to prevent memory leaks. This feature speaks to the internal workings of the library and promotes efficient resource management.
Sprites can also encapsulate their event handling logic. Developers can override methods like `update()` to add specific behavior to a sprite class. This means that intricate interactions can be baked directly into the sprite definition, leading to cleaner and more modular code.
Yet, some scenarios may require more complex collision detection than simple rectangle checks. `pygame.mask` allows for more intricate collision detection using pixel-based collision checks. This feature is particularly useful for game elements with unusual or non-rectangular shapes and enables greater flexibility in determining how interactions occur between game objects. This kind of approach could be used to enable more sophisticated gameplay interactions.
Finally, Pygame's sprite framework is well-suited to animation. Sprites can readily manage animations using lists, with helper functions like `pygame.time.get_ticks()` managing the timing. This makes it relatively straightforward to implement animation for actions like walking or jumping without needing external libraries.
7 Essential PyGame Functions for Creating Your First 2D Game Prototype in 2024 - Handle Keyboard and Mouse Events with pygame.event.get()
To make your Pygame game responsive to player actions, you need a way to handle keyboard and mouse inputs. `pygame.event.get()` is your primary tool for this. It retrieves a collection of events that have occurred since the last time it was called, essentially acting as a queue of user actions. This allows your game to react to player inputs in real time, whether it's a key press or a mouse click.
Beyond just capturing events, you can use `pygame.key.get_pressed()` to check if specific keys are currently being held down. This is especially useful for implementing continuous actions like player movement. For instance, if the left arrow key is held, your character might move continuously to the left.
By combining `pygame.event.get()` and `pygame.key.get_pressed()`, you can create a variety of complex interactions. It's through this process that your game becomes interactive and enjoyable, allowing players to steer the game and impact its environment. You'll need to put this within a continuous loop (often called the game loop or event loop) to keep checking for events and updating the game state. This loop is fundamental to game development and is how you ensure the game continuously reacts to player actions and other events. Without this constant event processing, your game would be static and unresponsive. The event loop, combined with the tools for handling user inputs, is fundamental to making a dynamic game.
Pygame's event handling system, centered around `pygame.event.get()`, provides a mechanism for handling keyboard and mouse input in a flexible and efficient manner, offering a few notable insights for curious game developers.
Firstly, the event system manages input through a queue, similar to a line at a store. This queue is organized using a First In First Out (FIFO) structure, meaning events are processed in the order they're received. This can be handy for situations where the order of player inputs is crucial to how the game progresses, or in games with complex interactions. This structure also allows you to selectively process only the events relevant to your game at any given time.
Furthermore, Pygame differentiates inputs into numerous types, such as key presses (`KEYDOWN`, `KEYUP`) and mouse button interactions (`MOUSEBUTTONDOWN`, `MOUSEBUTTONUP`). This granularity allows you to program your game to react differently based on the exact type of input, allowing for a much richer player interaction experience. It's more akin to a precise orchestra conductor than a drummer pounding out a basic beat.
Beyond the basic events provided, Pygame lets you design your own, custom events. This can be quite useful for incorporating events that are specific to your game. For example, you could implement a "game over" or "level complete" event that is triggered when certain conditions are met.
One unexpected aspect of `pygame.event.get()` is that it doesn't block the game loop. This is significant because it means that while the game is waiting for user input, it can still be carrying out other processes like updating game objects, drawing visuals, or even checking for other conditions. This results in a more smooth, responsive experience.
Interestingly, you can even filter the events that come from `pygame.event.get()` using tools like list comprehensions. You can pick and choose which events are handled, essentially letting you ignore events that are not important. This can make the game run faster and make your code easier to read. It's like sifting through a pile of information to focus only on the gold nuggets, reducing waste and improving focus.
In addition to events, Pygame provides real-time access to the current mouse position using `pygame.mouse.get_pos()`, independent of event queue. This means that a game can respond to mouse movement continuously without waiting for a mouse click or button release. You could, for instance, use it to show a crosshair that follows the mouse across the game screen. It also could help create a kind of predictive movement or "aim assist" by using the mouse location.
There is also a time stamp in every event, allowing you to determine when a given event occurred. Using these timestamps can be helpful for coordinating game actions or implementing time-sensitive game mechanics, offering more robust gameplay interaction. It's similar to how a musical score will indicate when to start and stop different instruments or melodies.
The event system can even be used to manage the states of the game. This can be especially helpful when there are multiple states in the game or there are multiple modes. It's like having a specific set of instructions for what the game should do in a specific phase. Pressing a key or mouse click could trigger a state change.
However, it's worth considering that constant polling of the event queue can cause performance issues, especially in games with a large number of objects or complex interactions. As with any tool, using `pygame.event.get()` improperly can produce undesirable effects. The developer has to balance responsiveness and efficiency. It's like a chef who manages a multitude of burners to ensure everything cooks to perfection.
Perhaps surprisingly, Pygame's event handling system is designed to work across multiple operating systems, meaning you can often write code once and have it run on Windows, macOS, and Linux, greatly improving your time and development effort. It’s a boon for developers seeking to reach a wider audience without modifying the core gameplay logic for each platform. This cross-platform capability illustrates the potential power of abstracting away the low-level aspects of operating systems so that you can focus on the actual game elements.
In conclusion, Pygame’s event handling system allows a developer to create engaging game interactions through both keyboard and mouse events using the `pygame.event.get()` function. While simple at first glance, a deeper dive reveals the capability for highly customized event responses, efficient filtering, and integration with state management. Understanding these functionalities can elevate your game development capabilities and enrich your game designs.
7 Essential PyGame Functions for Creating Your First 2D Game Prototype in 2024 - Draw Shapes and Images Through pygame.draw and surface.blit()
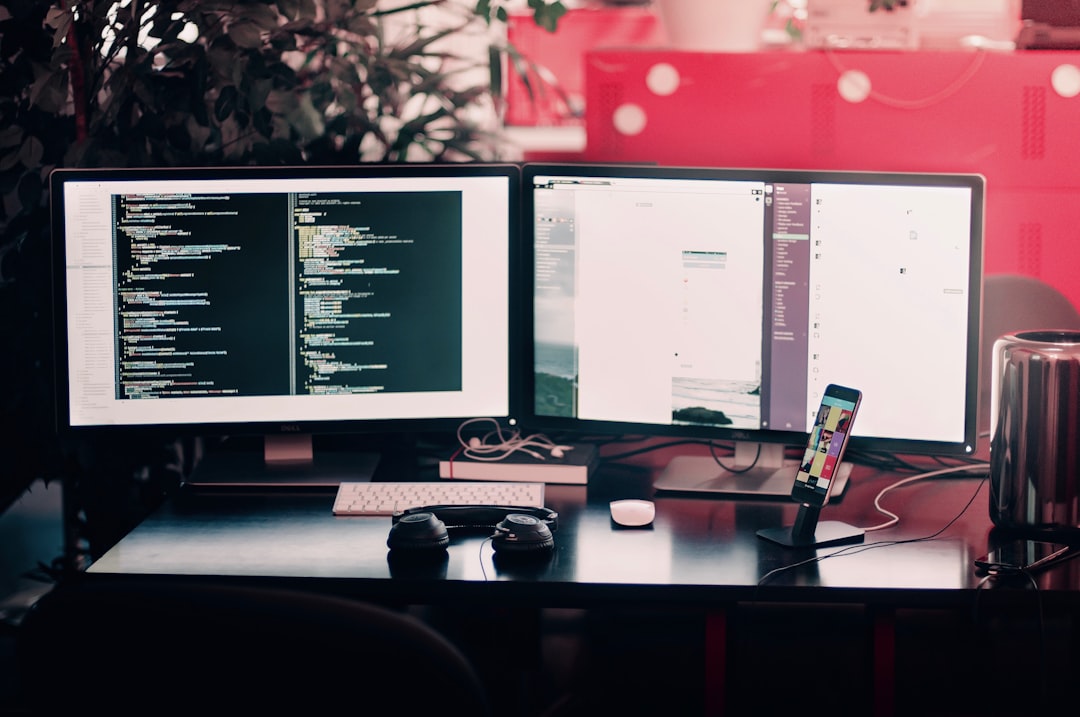
Creating compelling visuals in your Pygame game involves drawing shapes and images. The `pygame.draw` module provides tools for directly creating fundamental geometric shapes, like circles and rectangles, on a Surface. This is useful for defining the underlying structure of a game's environment or creating the visual elements of user interface elements. For displaying more complex images, `Surface.blit()` is used to draw an image onto the game window. This creates a richer and more engaging visual experience. You can load images into Pygame through `pygame.image.load()`, and you'll likely want to follow up by converting the Surface using `convert()` for potential performance improvements. It's important to be mindful about how and when you're using these functions as inefficient or poorly designed drawing practices can lead to a sluggish game experience, especially when handling more complex visuals. You have to be mindful of these operations to prevent performance bottlenecks as your game becomes more complex.
Pygame's `pygame.draw` module and the `surface.blit()` method are fundamental for rendering shapes and images within your game. `surface.blit()` leverages a highly optimized approach to pixel manipulation, making it well-suited for the fast-paced nature of games. Interestingly, Pygame supports palette-based drawing, which can offer a significant performance boost, especially on older or less powerful hardware. Clever use of color palettes can even be incorporated into the game's visual style.
Furthermore, the `surface.blit()` method offers per-pixel alpha blending, a feature allowing you to achieve sophisticated transparency effects like fog or shadows, adding depth and visual appeal to your 2D game worlds. This capability can lead to more visually engaging environments and detailed visuals in your projects.
The `pygame.draw` module facilitates on-the-fly shape creation. This dynamism can be harnessed for creating visual cues or dynamic feedback for player interactions. It's a powerful way to enhance user interaction and gameplay. Also, `pygame.Rect` objects can be used to limit the area where shapes are drawn, which can help you control the exact placement of shapes within the game scene. This precision can be valuable for maintaining a consistent and carefully designed look and feel.
Beyond standard shapes, you can draw custom polygons and lines using the `pygame.draw.polygon()` and `pygame.draw.lines()` functions, allowing for creative freedom in designing visual elements within the game. It lets you move beyond just standard geometrical shapes.
`pygame.display.flip()` takes advantage of modern hardware acceleration, which improves performance when you're updating the entire game screen. This is especially relevant when you are displaying numerous graphics on the screen at once. It's a key optimization technique.
The act of drawing shapes can also be used as a quick approach to collision detection and debugging. You can easily visualize the areas your game considers as "collidable" through a drawn representation, enhancing the feedback during development. Pygame's optimization strategy for "dirty rectangles" further streamlines performance by only redrawing the portions of the screen that have changed. This approach minimizes overhead and can dramatically impact visual refresh rates and efficiency.
An interesting aspect is Pygame's support for offscreen rendering. You can create and manipulate surfaces that are not displayed immediately and later bring them to the game display as needed. This approach allows you to pre-render complex graphics or animations, leading to smoother game flow and reduced latency.
By thoughtfully incorporating these elements of `pygame.draw` and `surface.blit()`, you can enhance the performance and visual quality of your 2D Pygame games, demonstrating sophisticated development choices in your projects. The combination of these tools leads to a richer game development experience, opening up more interesting design possibilities.
7 Essential PyGame Functions for Creating Your First 2D Game Prototype in 2024 - Add Background Music and Sound Effects via pygame.mixer
Adding background music and sound effects to your Pygame game can significantly enhance the overall experience. The `pygame.mixer` module provides the tools to integrate audio seamlessly. You'll start by initializing Pygame's audio system and loading your audio files – background music and sound effects are handled separately. Background music is typically looped continuously to create a consistent soundscape, while sound effects are triggered as needed, like explosions or jumps.
While Pygame can handle different audio formats, the `.wav` format is often preferred for sound effects due to its reliability, and `.mp3` is typically suitable for background music although occasional playback problems are possible. You have some control over audio, including volume adjustments and managing channels for playing multiple sounds at once. This multi-channel approach allows background music to continue while simultaneously playing sound effects. Understanding how to effectively utilize `pygame.mixer` can really elevate your game, creating a more immersive experience for the player. If you neglect this element, you could easily create a very underwhelming game, especially considering how important sound is in a game.
To incorporate background music and sound effects into a Pygame game, you'll rely on the `pygame.mixer` module. It's a versatile tool that supports various audio formats, including WAV, MP3, and OGG. This flexibility allows for balancing sound quality and file size based on a game's needs. However, be cautious, as MP3 playback can occasionally be unstable. The WAV format tends to be the more reliable choice for sound effects.
One of the benefits of `pygame.mixer` is its ability to manage multiple audio channels. This allows the game to play background music and sound effects concurrently without one interfering with the other. Adjusting the volume of individual channels gives you granular control over the audio environment and allows emphasis on certain sound cues within the game.
Loading sound files can sometimes be a performance bottleneck, especially with larger files. It's wise to consider techniques like preloading frequently used sounds and keeping sound effects short to minimize this issue. Otherwise, a game can have noticeable delays when sound files are loaded during gameplay.
The volume of both music and effects can be tweaked dynamically through `pygame.mixer`. This allows the game to adjust the background music volume when a crucial sound effect or dialogue sequence occurs. For example, a game could lower music volume to emphasize a character's voice during important moments. This can enhance immersion within the game environment.
The `pygame.mixer.music` module specializes in continuous background audio playback. It supports looping and can transition smoothly between tracks. It's an excellent tool for creating the audio environment and can add depth and immersion to a game.
`pygame.mixer` provides basic audio mixing, letting you combine multiple sound sources into a unified output. This lets you craft complex and nuanced soundscapes, layering audio components together without needing extra software. It's a simple but efficient approach.
One thing to note is that the `pygame.mixer` itself doesn't inherently support advanced audio effects such as echo or reverb. You'll need to be creative and combine different sounds or adjust playback speed and volume to get this effect. It demonstrates the creativity needed to work around library limitations and highlights the importance of audio design skills.
Integrating `pygame.mixer` into an event-based game loop allows sound effects to be triggered by specific game events like character actions (e.g., triggering a jump sound). This keeps the sounds in line with the game, and it gives meaningful visual and auditory cues to the player, further enriching gameplay.
Pygame's audio functions tend to behave consistently across diverse operating systems, like Windows, macOS, and Linux. This is invaluable as it lets you focus on creating the game experience rather than worrying about sound code functioning differently across each platform.
However, it is crucial to be mindful of audio latency, which can occur particularly when working with complex or extensive audio files. These concerns can affect player input and the overall feel of the game if they become noticeable. Striking the right balance between sound quality and timely audio feedback is vital for an engaging gameplay experience.
Ultimately, `pygame.mixer` provides a powerful and readily accessible set of tools for integrating dynamic audio into your game. However, using it effectively involves a consideration of formats, file sizes, potential latencies, and how it can augment the gameplay. A well-crafted approach using the tools provided can considerably enhance a Pygame project.
7 Essential PyGame Functions for Creating Your First 2D Game Prototype in 2024 - Control Game Speed Using pygame.time.Clock().tick()
Maintaining a consistent and smooth gameplay experience often relies on managing the speed at which your game runs. Pygame's `pygame.time.Clock()` class offers a crucial mechanism for achieving this. You can use the `tick()` method to set a desired frame rate—for instance, 60 frames per second. This ensures that your game renders and updates consistently across different hardware, making the gameplay experience more predictable and less susceptible to variations in system performance.
Further, you can modify the frame rate, the number of times the game updates per second, by changing the value passed to `clock.tick()`. This enables fine-grained control over game speed, which can be useful when you want to adjust other game elements, like character movement speed or how quickly animations play. Without a consistent frame rate management system, you risk game elements acting erratically and leading to an uneven or jarring experience. Understanding how to utilize `pygame.time.Clock().tick()` effectively is therefore vital for any game developer seeking to create responsive and enjoyable 2D game prototypes. It's a foundational aspect for improving a user's experience.
The `pygame.time.Clock()` class, specifically its `tick()` method, is a powerful tool for managing the speed and frame rate of a Pygame game. It's not simply about setting a maximum frame rate; it also provides a mechanism for precise time management, which is crucial for creating a polished gaming experience.
For instance, `tick()` returns the number of milliseconds that have passed since the last call. This information is valuable for understanding how well a game is performing and where potential bottlenecks might exist. In addition, using `tick()` helps to synchronize the game loop with real time, which is vital for genres like rhythm games or racing games where precise timing is a key part of gameplay.
Moreover, the frame rate isn't a fixed constraint. You can adjust the tick rate dynamically based on system performance. If your game starts lagging in more demanding scenes, reducing the tick value through `tick()` can help to maintain a smoother gameplay experience. It's like having a governor on the game's speed to ensure it stays within operational parameters.
However, there are nuances to how frame rates interact with other parts of the game. For example, many game engines use a fixed time step for physics calculations. This means the frame rate impacts how collisions and movement are handled in the game. If the frame rate fluctuates erratically, this could lead to unpredictable game physics and possibly a disjointed experience.
The frame rate can be customized to suit a specific game design. Through experimentation, developers can manipulate the feel of a game, tweaking how responsive or fluid the gameplay feels.
Beyond visual smoothness, using `tick()` leads to other benefits. It reduces CPU usage during idle periods in the game loop, helping preserve battery life on mobile devices or lower heat output on systems with limited cooling capabilities.
The event handling mechanism in Pygame interacts with the timing provided by `tick()`. This interaction results in more predictable and consistent input processing. The player inputs, in turn, are processed at more consistent intervals, leading to greater control and responsiveness for the player.
While `tick()` provides a significant boost, setting a frame rate too high (beyond what the display can handle) can create unnecessary processing overhead. If the tick rate doesn't align with graphics updates, the gain in performance decreases, highlighting the importance of understanding your target hardware.
Maintaining a stable frame rate also minimizes visual issues like screen tearing, improving the overall visual quality of the game. It's similar to maintaining a constant speed with a car—without jolts and jerks in the process.
Lastly, implementing `tick()` contributes to a well-structured game loop, fostering a more organized and easily maintainable design. This approach becomes critical as a game becomes more complex and has a larger number of development contributors.
Through understanding and utilizing `pygame.time.Clock().tick()`, game developers can gain significant control over their games' speed and responsiveness. This leads to enhanced gameplay, improved performance, and cleaner game loop designs. Mastering this function provides you with the tools to optimize your game, making it both a fun and efficient game for players to interact with.
7 Essential PyGame Functions for Creating Your First 2D Game Prototype in 2024 - Detect Object Collisions Through pygame.sprite.collide_rect()
When building 2D games, detecting when objects collide is a core component. Pygame's `pygame.sprite.collide_rect()` function is a helpful tool for this. It specifically lets you see if two sprites—game objects like players, enemies, or projectiles—are hitting each other by checking their rectangular boundaries. Basically, it determines if the rectangular areas that surround each sprite overlap.
This collision detection is based on the `colliderect` method within the `pygame.Rect` class. This simplifies managing how objects react when they bump into each other. To make collision detection more efficient when you have a lot of sprites, you can group sprites together using `pygame.sprite.Group`. This lets you check for collisions across all the sprites in the group at once, optimizing your game's performance.
However, keep in mind that `pygame.sprite.collide_rect()` primarily deals with rectangular collision areas. For more intricate object shapes, like a spaceship or a character with unusual body parts, you might need more advanced collision techniques. These limitations shouldn't deter you, as understanding `pygame.sprite.collide_rect()` provides a solid foundation for game mechanics that require object interactions.
Within the realm of Pygame, detecting collisions between game objects is a fundamental aspect of creating engaging interactions. The `pygame.sprite.collide_rect()` function offers a relatively straightforward approach by employing axis-aligned bounding boxes, essentially rectangles, to determine if objects overlap. While simple, this method proves efficient for a wide range of 2D games, especially when dealing with many objects simultaneously.
The efficiency of `collide_rect()` is particularly noticeable when using `pygame.sprite.Group`. By grouping sprites together, you can check for collisions across a collection of sprites in a batch operation, making it well-suited for managing larger numbers of entities in a game. This bulk processing reduces the workload of constantly checking individual objects, leading to improved performance.
Pygame's clever design means each sprite has a `rect` attribute, a `pygame.Rect` object. This decision makes a lot of sense since the same bounding rectangle is used for both drawing the sprite and detecting collisions. It simplifies game logic and streamlines the process.
However, a key consideration when using `collide_rect()` is the difference between static and dynamic collisions. It's efficient for situations where objects are not moving frequently, such as the walls or stationary obstacles in a platformer. But when dealing with constantly moving objects or more intricate interactions, using `pygame.mask` for pixel-perfect collision detection can be more appropriate, though it can also add complexity. This highlights a classic trade-off: simple and quick versus accurate but potentially resource-intensive.
Another point worth emphasizing is that `collide_rect()` merely detects collisions; it doesn't dictate what happens after a collision occurs. You, the developer, need to write separate code to determine how your game reacts to an overlap. This can involve changing the position of objects, triggering sound effects, or adjusting the game state. While it offers flexibility, it can also increase the complexity of your game logic if you have intricate interactions.
For game elements with more complicated shapes, Pygame's `pygame.mask` module provides a way to perform pixel-perfect collisions. This approach can be ideal for accurate interaction detection, especially if objects have irregular shapes or non-rectangular boundaries. While very precise, be aware that the increased accuracy can come at a performance cost.
It's also important to note that `collide_rect()` doesn't inherently deal with scenarios where multiple collisions happen at once. If multiple objects overlap, you'll need to process the collision data in a way that manages how each collision affects the game. This can introduce some coding complexities if the number of object interactions gets large.
Layered updates, enabled by `pygame.sprite.LayeredUpdates`, complement the use of `collide_rect()`. Layered updates allow you to control the rendering order of your sprites, which can impact the way collisions look visually and how you perceive them within the game. It's another example of how Pygame components combine to manage visual feedback and collision detection.
Collision boundaries are not always fixed within a sprite's `rect`. You might need to modify the bounding box (the `rect` attribute) to better fit the shape of a character's hitbox. For example, if you have a player with a broadsword or a large weapon, you might adjust the `rect` so that the collision region aligns with the character's attack area more accurately.
Another noteworthy aspect is that you can change a sprite's `rect` dynamically during gameplay. This opens up possibilities for game elements to adjust their collision boundaries based on the game's conditions or actions. For example, a character might shrink or grow, and you could update their `rect` accordingly. While useful, managing this adaptability requires vigilance to avoid unexpected behavior.
In summary, `pygame.sprite.collide_rect()` provides a good starting point for basic collision detection in your Pygame games, offering both speed and convenience, especially when managing groups of sprites. However, you have to consider the trade-offs between speed and accuracy for more complex scenarios, and you'll need to build your own collision response logic. Understanding the strengths and limitations of `collide_rect()` can help developers choose the right approach for collision detection within their 2D game prototypes.
Create AI-powered tutorials effortlessly: Learn, teach, and share knowledge with our intuitive platform. (Get started for free)
More Posts from aitutorialmaker.com: