Create AI-powered tutorials effortlessly: Learn, teach, and share knowledge with our intuitive platform. (Get started for free)
7 Overlooked JavaScript Concepts Taught in Free Online Courses for 2024
7 Overlooked JavaScript Concepts Taught in Free Online Courses for 2024 - Closures and Their Practical Applications in Modern JavaScript
Closures are a fundamental concept in JavaScript that allows functions to retain access to variables from their surrounding scope, even after those functions have finished executing. This capability has profound implications for how we write and structure code. Closures enable us to achieve data encapsulation, creating private variables that are protected from external interference. This is incredibly valuable when we need to maintain a specific state within a particular function or object.
Furthermore, closures play a pivotal role in functional programming paradigms within JavaScript. Currying, a technique that breaks down complex functions into simpler, more manageable components, relies on closures. This enhances code reusability and flexibility. Closures also underpin techniques like memoization, which can significantly improve performance by storing the results of expensive function calls and reusing them when the same input is encountered again.
However, it’s important to be aware of potential pitfalls. Since closures retain references to variables in their enclosing scope, they can lead to unintended memory retention if not handled properly, potentially causing performance problems. A solid understanding of scope and how closures operate is crucial to prevent bugs and optimize code.
In today's JavaScript development environment, mastering closures is no longer a luxury but a necessity. They underpin many common coding patterns, allowing for efficient and organized code. Ignoring this fundamental concept can hinder a developer's ability to write truly robust and efficient applications. Understanding and utilizing closures effectively is a key aspect of writing modern JavaScript that scales and performs.
Closures in JavaScript allow inner functions to remember and access variables from their outer function's scope, even after the outer function has finished executing. This is frequently used to create encapsulated, private variables, a cornerstone of data hiding. However, this feature can also cause surprises, especially within loops. If not handled cautiously, closures in loops might end up referencing the final loop iteration's value for all iterations due to how they capture variables by reference.
Closures have become a core concept for modern JavaScript frameworks like React and Angular, enabling clean and intuitive state management. By encapsulating stateful operations within functions, frameworks can manage state in a more controlled and predictable fashion. They are not simply a neat trick; they're the foundation of how JavaScript manages events. Event handlers often need to preserve their own scope, allowing for interactions without resorting to global variables.
While beneficial for avoiding global state clutter, closures can also lead to memory leaks if not properly managed. The persistence of outer function references can cause memory issues if not handled with care. They can be potent tools for implementing function currying, a functional programming technique that lets developers craft flexible and reusable functions by pre-setting arguments. This greatly improves code expressiveness and readability.
Nesting closures (closures inside closures) can result in convoluted code that's hard to debug. To keep large codebases understandable, having a solid grasp of JavaScript's scope chains is crucial. Closures also enable the creation of higher-order functions, which take or return functions as arguments. This supports various functional programming patterns and practices in JavaScript.
Closures play a critical part in managing state in asynchronous programming—within callback functions or Promises, for example. They are essential for keeping track of data over time within asynchronous actions without resorting to the global scope. The concept of closures originated in languages like Scheme and Lisp, highlighting the enduring nature of lexical scoping and how it has been incorporated into more modern languages like JavaScript to manage state and functionality in a wide range of applications, from small scripts to large web apps.
7 Overlooked JavaScript Concepts Taught in Free Online Courses for 2024 - Asynchronous Programming with Promises and Async/Await
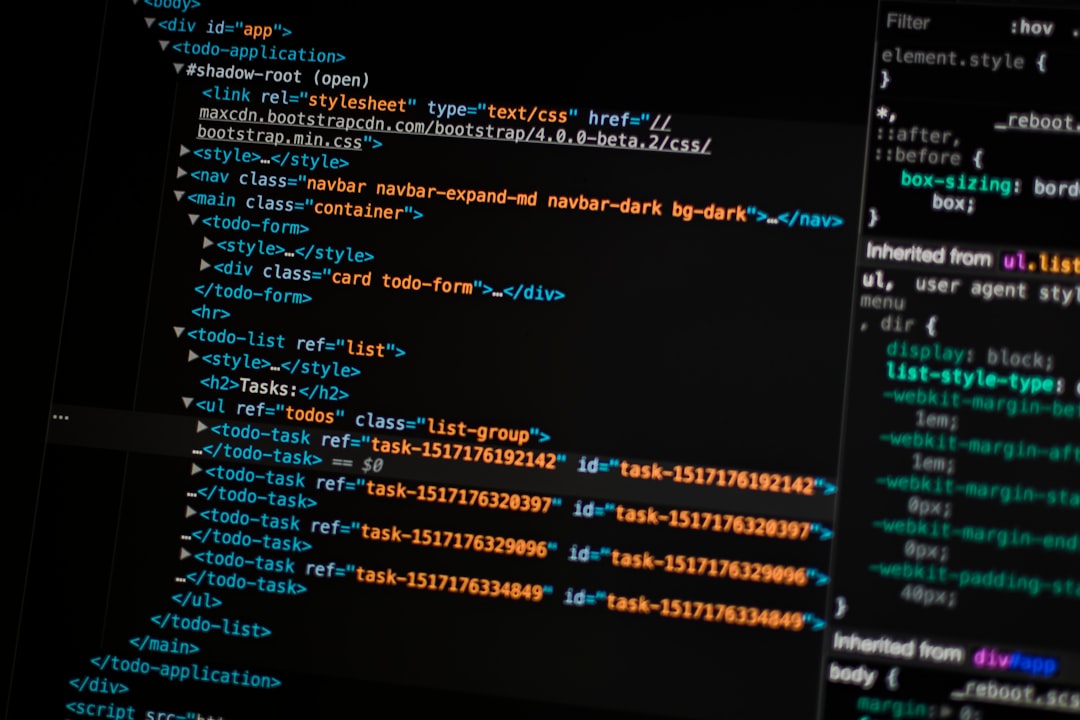
Asynchronous programming is fundamental to building responsive and efficient JavaScript applications. It allows operations, like fetching data from a server, to run concurrently without blocking the main thread of execution. Promises and the `async`/`await` syntax are key tools for tackling this complexity. Promises represent the eventual outcome of an asynchronous operation, potentially succeeding or failing. They introduce a more structured way to manage the state of operations, compared to traditional callbacks. `Async`/`await` then takes this structure and makes the code look almost synchronous, simplifying asynchronous tasks and improving readability.
While the `async`/`await` approach significantly simplifies asynchronous code, it's crucial to recognize the underlying principles. Handling errors effectively, managing the order of operations, and debugging complex asynchronous sequences require a good understanding of how Promises are resolved and rejected. Effectively juggling multiple asynchronous operations, particularly when they have interdependencies, remains a challenge requiring thoughtful planning and structuring. Understanding how these techniques work together is essential to crafting robust JavaScript that interacts with the outside world in a reliable way. Ignoring these concepts in modern web development leads to code that is difficult to read, harder to maintain, and less responsive to user interactions.
JavaScript's asynchronous programming model, particularly with the introduction of Promises and async/await, allows us to handle multiple operations without blocking the main thread. However, it's important to remember that JavaScript still runs on a single thread. While asynchronous code avoids blocking, it doesn't automatically translate to parallel execution. Techniques like Web Workers are needed for true parallelism.
Promises are a fundamental building block for asynchronous operations. They represent the eventual outcome of an asynchronous task, whether successful or unsuccessful. A Promise's three states—pending, fulfilled, or rejected—give us a structured way to handle diverse asynchronous flows, going beyond the limitations of simple callbacks and potentially avoiding the dreaded "callback hell."
Async/await significantly improves asynchronous error handling by integrating smoothly with the familiar `try...catch` blocks. This simplifies debugging and maintenance of asynchronous code, a benefit that's often underestimated by those starting with asynchronous programming.
The ability to chain Promises, where each `.then()` returns a new Promise, makes asynchronous workflows easier to follow. It lets us build intricate asynchronous processes where one operation smoothly transitions to the next based on the outcome of the previous step. This approach helps prevent the complexity that comes with deeply nested callback structures.
When a Promise is resolved, its `.then()` handlers are placed in the microtask queue, which ensures they get executed before the next event loop tick. This prioritization of resolved Promises can have subtle consequences, influencing how tasks are processed within the JavaScript execution environment.
Every async function is a Promise in disguise. This characteristic, while useful, can also be a source of confusion. If a developer isn't aware that async functions automatically return a Promise, it can lead to unforeseen problems.
A key limitation of Promises is the lack of native cancellation. If a Promise-based operation is no longer needed, we can't easily cancel it. Implementing custom cancellation mechanisms using tools like `AbortController` is required to avoid unnecessary resource consumption from lingering asynchronous operations.
While async/await promotes sequential execution, tools like `Promise.all()` enable us to run several asynchronous tasks concurrently. This is extremely useful for performance optimization when we have multiple independent tasks to complete.
Developers transitioning from the comfort of synchronous programming to asynchronous programming with Promises and async/await will likely encounter a higher cognitive load. Understanding the intricacies of how these constructs interact with JavaScript's event loop and execution model is vital to writing correct asynchronous code and to avoid introducing errors due to incorrect reasoning about asynchronous behaviour.
While Promises and async/await are now widely supported across modern browsers, some legacy browsers may lack full compatibility. Using tools like Babel to transpile modern JavaScript syntax into older equivalents can ensure functionality across a broader range of environments, but this adds complexity to the development process.
7 Overlooked JavaScript Concepts Taught in Free Online Courses for 2024 - Prototypal Inheritance and Object-Oriented JavaScript
JavaScript's object-oriented nature is built upon a foundation of prototypal inheritance, a concept that sets it apart from languages employing traditional class-based inheritance. Instead of inheriting through classes, JavaScript objects inherit directly from other objects, creating a chain of prototypes. This allows for a more dynamic and flexible way to structure and reuse code.
Every object has a secret connection to another object, its prototype. When you try to access a property on an object and it's not found there, JavaScript cleverly searches the prototype chain for it. This efficient mechanism ensures that methods and properties are only defined once, saving memory and reducing redundancy. Multiple objects can share the same properties and methods inherited through the prototype chain.
The prototype property associated with constructors acts as a blueprint for instances created from that constructor, holding inherited properties and methods. This model, while powerful, can be initially challenging to grasp for developers accustomed to traditional object-oriented programming. Understanding how prototypal inheritance shapes the behavior of JavaScript objects is crucial for writing maintainable and efficient code in this environment. It reveals a core aspect of how JavaScript works, highlighting its focus on objects rather than classes, and allowing developers to create truly flexible and adaptable code.
Prototypal inheritance in JavaScript presents a distinct approach to object-oriented programming, offering both advantages and challenges compared to classical inheritance found in languages like Java. Each object in JavaScript implicitly connects to another object, its prototype, forming a chain of inheritance. When searching for a property on an object, JavaScript cleverly traverses this prototype chain if the property isn't directly found on the object itself.
This mechanism, while efficient, can also be a source of unexpected behavior. Overriding methods defined on a prototype can lead to subtle issues if developers aren't mindful of the replacement behavior. Similarly, instance-specific properties can overshadow properties from the prototype, which can be confusing when properties are expected to come from the chain instead of being directly attached to the instance.
Since prototype properties are shared across instances, changing a property on a prototype affects all its instances. This shared nature is advantageous from a memory management perspective but carries a risk of unexpected side effects if modifications are not carefully considered. Furthermore, the creation of objects using constructors depends heavily on the correct use of the `new` keyword. If omitted, the resulting object won't inherit from the constructor's prototype, which can be easy to overlook.
JavaScript's flexibility shines through in the ability to modify prototypes at runtime using functions like `Object.setPrototypeOf()`. This dynamic aspect allows for adaptable code but can complicate debugging and impact performance if not handled properly. `Object.create()`, designed for precisely controlling inheritance, provides an alternative to the constructor approach. However, understanding its nuances is crucial to leverage it effectively.
Despite the advantages, this flexible model can also be a source of issues. One risk is prototype pollution, where a dependency or library can accidentally modify core object prototypes, leading to unexpected consequences, including security vulnerabilities. Moreover, navigating intricate prototype chains can become challenging for developers accustomed to a more structured inheritance model.
Prototypal inheritance's flexibility finds a natural synergy with JavaScript's functional paradigm. The two approaches can combine to produce elegant solutions, yet developers must carefully consider the implications of combining these styles to avoid creating overly complex and hard-to-maintain code. Balancing the advantages of prototypal inheritance with a thoughtful approach to functional programming helps ensure that JavaScript's object model remains a powerful and versatile tool for building applications.
7 Overlooked JavaScript Concepts Taught in Free Online Courses for 2024 - Functional Programming Paradigms in JavaScript
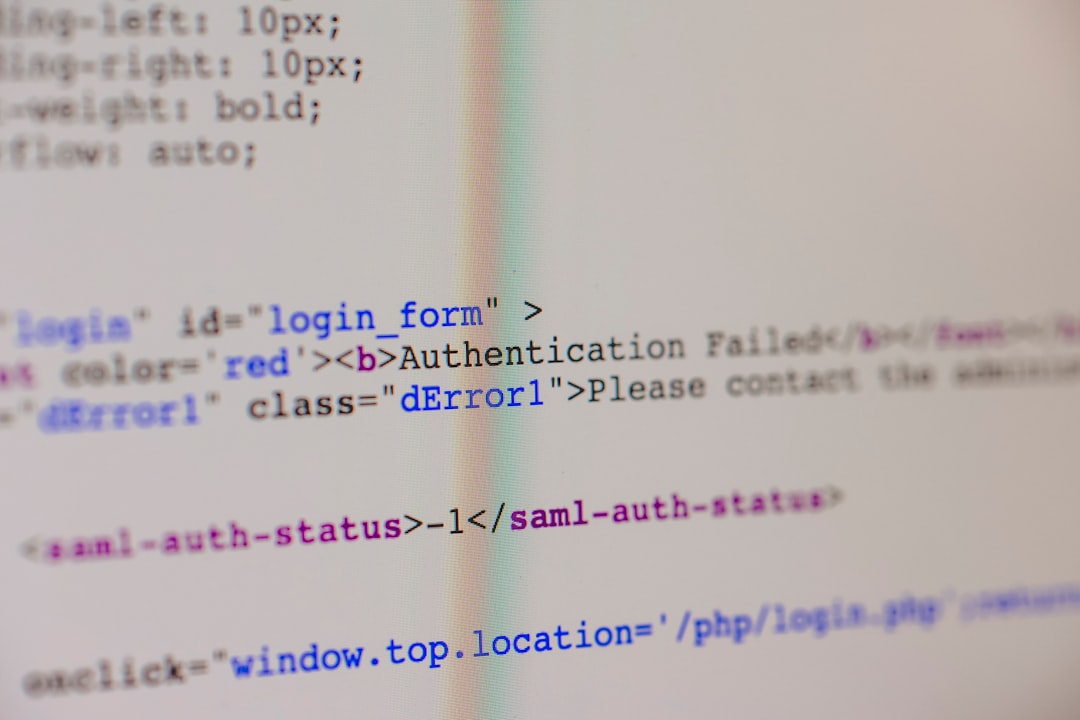
JavaScript, being a multi-paradigm language, embraces functional programming, a style that centers around functions as primary building blocks. This approach minimizes reliance on mutable state and side effects, opting instead for pure functions and immutable data structures. The result is code that's generally easier to reason about and maintain. This functional style has become increasingly popular with the rise of frameworks like React, which often leverage it for features like higher-order functions and streamlined data management. Recognizing this shift, many free online courses now emphasize functional programming in JavaScript, helping developers master the techniques for writing more concise and robust code. While beneficial, it's important to acknowledge that diving into functional programming can present challenges, especially as codebases grow in size and complexity. Developers might face a steeper learning curve as they grapple with new concepts and consider the trade-offs involved in this approach.
Functional programming in JavaScript centers around using expressions and functions without altering the underlying data or state. JavaScript's versatility allows it to incorporate multiple programming styles, including this functional approach. Key elements of functional programming, like pure functions, immutability, and a focus on avoiding side effects, lead to more maintainable and higher-quality code.
Interestingly, modern JavaScript frameworks such as React lean into functional programming concepts, contrasting with the older paradigms used in other frameworks like Svelte. Pure functions, a core idea, don't change anything outside of their own scope, making them easier for developers to analyze. Immutability, a fundamental principle, contributes to easier debugging and maintenance.
Replacing traditional loops with functions can often lead to more readable and elegant JavaScript. There's a noticeable growth in the availability and popularity of online courses covering functional programming in JavaScript, hinting at increasing interest in this style of programming. A solid understanding of functional programming significantly enhances a JavaScript developer's toolkit.
Essentially, functional programming treats computation as evaluating mathematical functions, with an aim of crafting cleaner and more manageable code structures. However, it's important to note that although immutability and pure functions can improve code structure and reduce debugging headaches, they can potentially introduce performance overheads if not managed thoughtfully.
While the adoption of functional programming principles can undoubtedly streamline and enhance code, choosing when to favor functional solutions over more established approaches requires careful evaluation based on the specific use case. As with any paradigm, functional programming in JavaScript brings its own set of considerations and tradeoffs, reminding us that no one approach fits all scenarios. Choosing the appropriate approach to solving a problem is often as much about a developer's experience and comfort with a style than a theoretical advantage. It's a fascinating aspect of JavaScript development to witness these evolving coding styles and their application in today's software.
7 Overlooked JavaScript Concepts Taught in Free Online Courses for 2024 - Event Loop and Concurrency Model Explained
### Surprising Facts about the Event Loop and Concurrency Model in JavaScript
1. **Event Loop's Role in Single-Threaded Concurrency**: While we often hear about JavaScript's concurrent nature, it's fundamentally single-threaded. The event loop acts as the conductor, orchestrating asynchronous operations without blocking the main execution thread. This clever mechanism gives the illusion of concurrent execution, which can be deceiving if not understood properly.
2. **Microtasks vs. Macrotasks**: The subtle difference between microtasks (like Promise callbacks) and macrotasks (like `setTimeout`) can lead to unexpected task execution orders. Microtasks are prioritized, being executed before the next main event loop iteration, which might surprise developers expecting a strict sequential order of operations based on when things are queued.
3. **Callback Hell's Pitfalls**: The event loop's handling of callbacks, while useful, can become a nightmare when deeply nested. This is the infamous "callback hell," highlighting the need for structures like Promises and `async`/`await` to create more understandable and maintainable code that doesn't lose clarity when dealing with multiple asynchronous steps.
4. **Synchronous Tasks Can Block the Event Loop**: If your code involves long-running synchronous operations, the event loop will be blocked, making your user interface seem unresponsive. This is a stark reminder that the advantages of the event loop only apply when dealing with asynchronous operations, and that for computationally-intensive tasks, it's necessary to divide them into smaller pieces or to use mechanisms like Web Workers to utilize more of your computer's capabilities.
5. **Understanding the Event Loop's Phases**: The event loop cycle includes phases like timers, input/output callbacks, and idle periods. Recognizing these different phases is valuable for debugging and optimizing code, allowing developers to pinpoint when particular events are likely to be processed. This can greatly aid in developing more robust code that anticipates when things will execute.
6. **Global Scope's Influence on Asynchronous Operations**: The global execution context, where your script runs, directly interacts with the event loop. This interconnectedness highlights the importance of managing state carefully. Even seemingly benign changes to global variables can unexpectedly affect the asynchronous flow. This might seem like an obscure point, but it can be crucial to understanding some debugging challenges.
7. **Handling Errors in Async Land**: Unhandled exceptions in asynchronous code might not always surface in a straightforward manner. Promises offer `.catch()` blocks for handling errors, which is a reminder that we must create deliberate strategies for managing these situations within event-driven architectures. The silent failure of exceptions can easily lead to hard-to-find bugs.
8. **Performance Depends on Engine Optimization**: The event loop implementation and any optimizations used by different JavaScript engines can affect how smoothly your application performs. This means that profiling and benchmarking become valuable tools for crafting high-performance code, and that you can't always count on performance staying constant across different platforms and browsers.
9. **Animation Optimization with `requestAnimationFrame`**: JavaScript provides `requestAnimationFrame` specifically to improve the rendering of animations. It interacts with the event loop to ensure smooth updates at the best times, minimizing the "jank" that can be seen in poorly optimized animation code.
10. **Concurrency vs. True Parallelism**: It's crucial to remember that the event loop facilitates concurrency, but not parallelism. While JavaScript manages many asynchronous tasks seemingly simultaneously, it's still fundamentally single-threaded. To achieve true parallel execution, developers need to rely on techniques like Web Workers, which can execute code on different threads.
This expanded explanation seeks to provide a more in-depth understanding of the event loop and concurrency model, highlighting some of their more surprising nuances and emphasizing the value of mastering these core JavaScript concepts for writing high-quality applications.
7 Overlooked JavaScript Concepts Taught in Free Online Courses for 2024 - Advanced Array Methods for Efficient Data Manipulation
JavaScript's array methods are foundational for efficiently handling data. However, beyond the basic methods like `push` or `pop`, a set of advanced array methods offer significant advantages. These methods, including `filter`, `map`, `reduce`, and `forEach`, provide powerful ways to manipulate and transform data within arrays. For instance, `filter` lets you easily extract specific elements based on a condition, while `reduce` allows you to aggregate array data into a single value, opening up more complex data processing possibilities. Employing these tools not only makes code cleaner and easier to understand but also helps developers adopt a more functional approach to JavaScript development, a growing trend in modern web development. As JavaScript evolves in 2024, the ability to utilize these array methods will become increasingly valuable for developers seeking to build efficient and robust web applications. While there are potential challenges in initially grasping these new concepts, the long-term benefits in terms of readability, efficiency, and the ability to write more sophisticated code are undeniable.
JavaScript arrays are a cornerstone of data manipulation, and mastering advanced array methods can lead to cleaner, more efficient code. Popular advanced methods include `filter`, `reduce`, `map`, `forEach`, and `concat`, each serving a unique purpose in data manipulation.
The `filter` method excels at creating new arrays containing only elements that satisfy a specific test defined in a callback function. This proves especially useful for isolating subsets of data. On the other hand, `reduce` applies a function to each array element, ultimately producing a single output value, which allows developers to accumulate results from an array in diverse ways. Basic array methods like `push` and `map` efficiently modify and transform data, facilitating a smooth workflow.
Finding an element within an array can be done with methods like `indexOf` and `findIndex`, while `filter` helps to remove specific elements. Additionally, the `lastIndexOf` method is handy for finding the last occurrence of an element in an array. Advanced methods enable developers to tackle complex tasks like iteration and transformation, improving both code efficiency and readability.
Arrays can be created using array literals (square brackets) or the array constructor (`new` keyword). Each method has its own advantages and uses. Interestingly, some developers seem to be unaware of the potential performance implications of using `reduce` for complex tasks when compared to a simple `for` loop in some contexts. The newer `flat` method for dealing with nested arrays is sometimes overlooked, as are `every` and `some` methods, which can provide a more declarative way to evaluate an array without needing to loop over it explicitly. It's worth noting that not all developers use these methods, highlighting a potential knowledge gap.
The increased use of advanced array methods, and the functional programming paradigms they foster, signifies a broader shift within the JavaScript development community. While these methods streamline code and make it easier to read, they also require developers to be mindful of functional programming principles like immutability and purity. Developers who focus on newer, high-level abstractions might easily miss more fundamental optimizations available with classic methods. Understanding these advanced array methods is becoming increasingly important as JavaScript developers face ever-more complex challenges. Mastering these methods paves the way for more sophisticated and efficient web applications in 2024 and beyond.
7 Overlooked JavaScript Concepts Taught in Free Online Courses for 2024 - Modular JavaScript Using ES6 Modules and CommonJS
ES6 modules and CommonJS offer distinct approaches to structuring JavaScript code, each with its own set of characteristics. The way JavaScript locates and uses modules differs between them. ES6 uses a static analysis method during compilation for imports and exports, while CommonJS handles module resolution dynamically at runtime. This can lead to varied performance and behavior, especially in larger codebases.
ES6 modules, by default, load asynchronously, which means importing them doesn't block other code from running. CommonJS, on the other hand, loads synchronously, creating potential performance bottlenecks if you need to import numerous modules.
One benefit of ES6 modules is their compatibility with tree shaking. Tree shaking eliminates unused code during the build process, resulting in smaller file sizes. This feature isn't readily available in CommonJS due to its dynamic nature. This is significant for keeping application bundles as lean as possible.
Another contrast between them is hoisting. In ES6, imports and exports are hoisted to the top, making them accessible regardless of where they're declared. This is different from CommonJS where you need to declare `require()` statements before using the modules you're importing. This creates variations in the way developers write code across the two systems.
Furthermore, how you import files is also different. With ES6, you don't need to include the `.js` file extension when importing. CommonJS requires it to be explicit, causing a minor but impactful change in how developers organize and reference files in projects.
There's also a difference in the top-level `this` keyword. In ES6 modules, it's `undefined`, whereas it refers to the global object in CommonJS. This is something to be aware of when it comes to scope, and it can be particularly important for developers switching from CommonJS to ES6.
In terms of browser support, ES6 modules have better native support compared to CommonJS. Modern browsers generally support ES6 natively, but older or specific environments (like certain Node.js applications) may prefer CommonJS. This variance can impact how developers organize their projects when they need to support multiple runtime environments.
ES6 modules support dynamic imports via the `import()` function, which allows you to load modules on-demand. This level of control is absent in CommonJS, granting ES6 more flexibility for situations where selective loading of components is essential, based on user interactions or conditions.
ES6 also allows the use of namespace imports, where all exports from a module are grouped into a single object. This can simplify the importing process and reduce the risk of name clashes. This feature isn't built into CommonJS.
Combining ES6 modules and CommonJS can be challenging. This is especially true in Node.js environments, where there can be conflicts. Tools like Babel can help bridge this gap, but it also introduces extra complexity when managing syntax and import/export behaviors.
Understanding these subtleties is important for writing modular JavaScript code effectively. This includes knowing when to leverage ES6's strengths and when to apply CommonJS techniques. By mastering the differences, developers can produce more robust and well-structured applications.
Create AI-powered tutorials effortlessly: Learn, teach, and share knowledge with our intuitive platform. (Get started for free)
More Posts from aitutorialmaker.com: