Create AI-powered tutorials effortlessly: Learn, teach, and share knowledge with our intuitive platform. (Get started for free)
Mastering Python's *args and **kwargs A Deep Dive into Arbitrary Parameters
Mastering Python's *args and **kwargs A Deep Dive into Arbitrary Parameters - Understanding the Basics of *args in Python
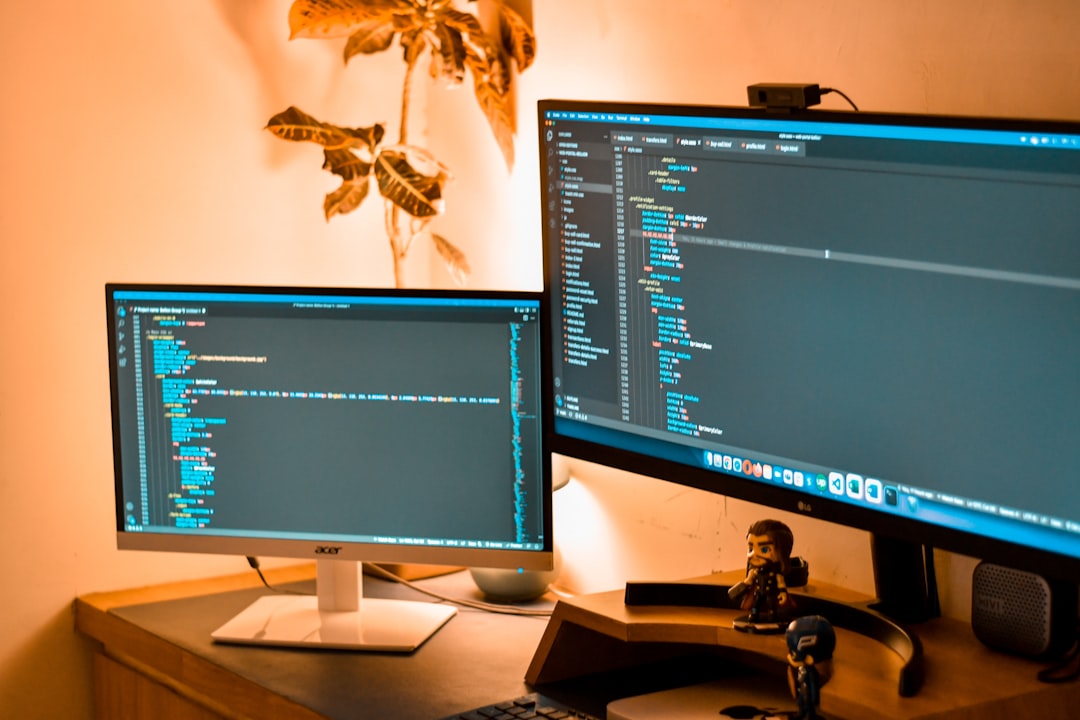
`*args` is a powerful tool in Python that lets you create functions that can take any number of positional arguments. Imagine you're writing a function to calculate the average of a set of numbers. You don't know beforehand how many numbers the user might want to average. This is where `*args` comes in handy. It essentially packs all the positional arguments passed to your function into a tuple. This gives your function a dynamic nature, letting it work with different inputs without needing multiple function definitions for each possible number of arguments. This simplifies your code and makes it more adaptable.
The `*args` syntax in Python allows us to define functions that can accept a variable number of positional arguments. This is incredibly useful when you don't know in advance how many inputs a function might need.
For example, you can use `*args` to create a function that can calculate the average of any number of numbers, without having to define separate parameters for each. This flexibility is a core strength of Python. But, as is often the case, this freedom comes with a responsibility to use it wisely.
Inside the function, the `*args` gathers all these arguments into a tuple. This lets you iterate through them, apply functions like `sum` and `max`, and even manipulate the data in various ways.
You can think of `*args` as a way to create functions that are more adaptable to different situations. It's a very powerful tool, but you need to be careful to use it appropriately and to document your code clearly to avoid confusion. Otherwise, it might make your code less maintainable.
`*args` can also be used in class constructors, allowing you to create objects that can be initialized with varying sets of parameters, which adds to the dynamism of Python.
It's worth emphasizing that the name `args` itself is simply a convention; you can choose any valid name, like `*inputs` or `*elements`, but keeping it as `*args` for clarity is generally good practice.
One thing I find interesting is that `*args` can handle a range of different data types, including integers, strings, and even lists. This makes `*args` even more flexible and useful. However, this freedom also means that you need to be careful about how you use `*args`, as you might need to carefully examine the data types of your inputs.
For instance, I've found myself using `*args` in functions that process different kinds of data—say, a set of numbers and a list of filenames.
Sometimes, `*args` can make your code more efficient, especially if you have a lot of different inputs. It simplifies the code, potentially improving performance as well.
While `*args` is very convenient, it's crucial to document your code well if you use it. Otherwise, it might become challenging for others (and even yourself) to understand what the function expects.
If you use `*args` in conjunction with `**kwargs` (for keyword arguments), you can create highly configurable functions that can handle both positional and keyword arguments, making your code even more adaptable and dynamic.
However, there's a trade-off. Debugging functions that use `*args` can be tricky, especially when you have a large number of arguments. Error messages might not clearly indicate which specific input is causing the problem, making it more challenging to isolate the source of the error.
It seems that the power of `*args` lies in its flexibility, which can make your code more concise and powerful but also creates challenges that need to be addressed carefully.
Mastering Python's *args and **kwargs A Deep Dive into Arbitrary Parameters - Exploring **kwargs and Their Role in Function Flexibility
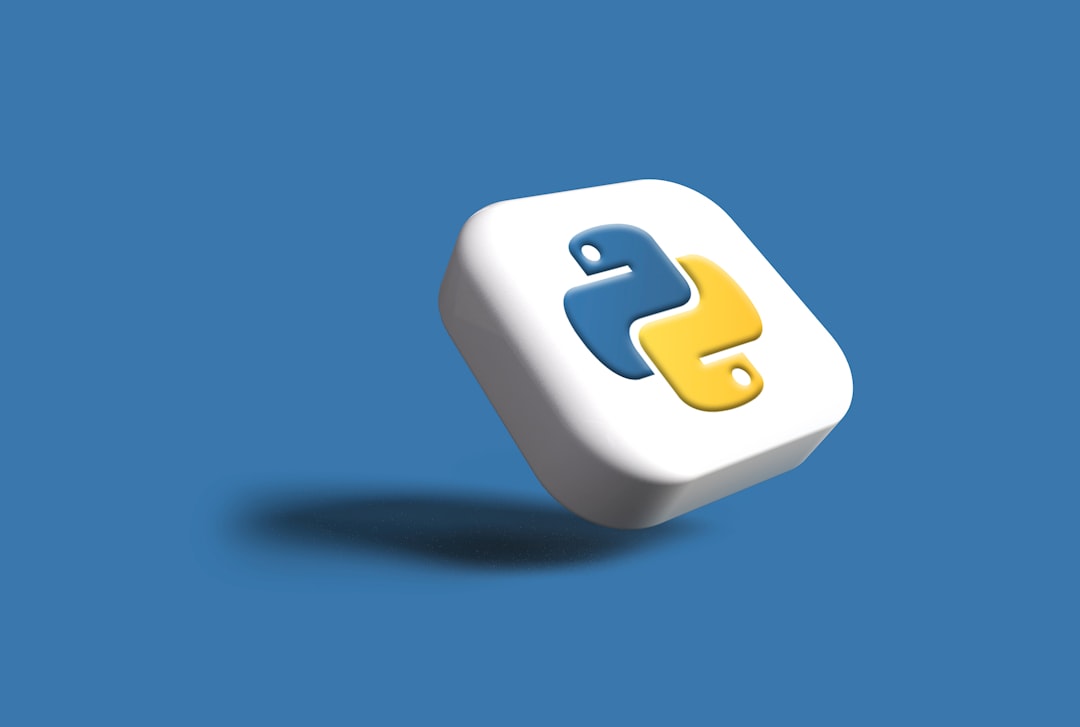
`**kwargs` in Python offers a powerful way to make functions more flexible. It allows a function to accept an unlimited number of keyword arguments, effectively turning them into a dictionary. This provides a dynamic way to handle named parameters, especially helpful when you're unsure about the exact set of inputs a function might need. For example, imagine you're creating a function that sends data to an API. You might need to specify various options like the API key, the endpoint, and potentially other custom parameters. This is where `**kwargs` shines.
Think of it as a way to define functions that are adaptable to different input scenarios. `**kwargs` lets you dynamically adjust how the function receives and processes data. You can then iterate through the `**kwargs` dictionary inside the function, using each key-value pair to customize the behavior of the function.
Using the conventional name `kwargs` helps keep the code clear, but you can actually use any valid name you want. The key is the double asterisk (`**`) before the name.
While `**kwargs` adds flexibility to your code, it comes with the responsibility of documenting what arguments are expected, particularly when building functions meant for others to use. Otherwise, your function might be less clear and even challenging to debug.
`**kwargs` is an intriguing Python feature that empowers you to write functions with an almost limitless degree of flexibility. It essentially lets you pass any number of named arguments to your functions. These arguments are gathered into a dictionary, so you can access them within the function by their corresponding key names.
The key benefit of `**kwargs` is that you don't need to explicitly declare every possible argument your function might receive. This makes your code much more concise and adaptable.
One interesting detail is that Python 3.7 and later versions retain the order of arguments within the `**kwargs` dictionary. This is helpful because you can easily iterate through the arguments in the order they were provided, simplifying debugging and making your code more readable.
Another nice feature is that `**kwargs` allows you to override default values without changing the function's signature. This makes your functions reusable, as you can easily adapt them to specific needs while maintaining the core functionality.
The combination of `*args` and `**kwargs` is particularly powerful. It lets you create functions that accept both positional and keyword arguments. This opens up a whole range of possibilities for building APIs and libraries.
For forward compatibility, `**kwargs` is a godsend. You can add new keyword arguments to your functions without breaking existing code that uses the function. This makes your code more robust and less prone to errors.
However, `**kwargs` comes with its share of potential issues. If you're not careful, functions that accept too many keyword arguments can become confusing, making it hard to understand the function's behavior and to document it properly.
One creative use of `**kwargs` is that you can use it to unpack dictionaries into function calls. This can be handy for simplifying function invocations that require multiple parameters.
As with any powerful tool, `**kwargs` needs to be used with care. Pay attention to potential naming collisions, as using common names for arguments in multiple function calls can lead to unexpected behavior.
In the end, `**kwargs` is a very versatile feature that adds another layer of flexibility to Python. If you use it judiciously, it can make your code cleaner, more adaptable, and more robust. But, it's essential to be aware of the potential pitfalls, especially when dealing with many keyword arguments.
Mastering Python's *args and **kwargs A Deep Dive into Arbitrary Parameters - Combining *args and **kwargs in Function Definitions
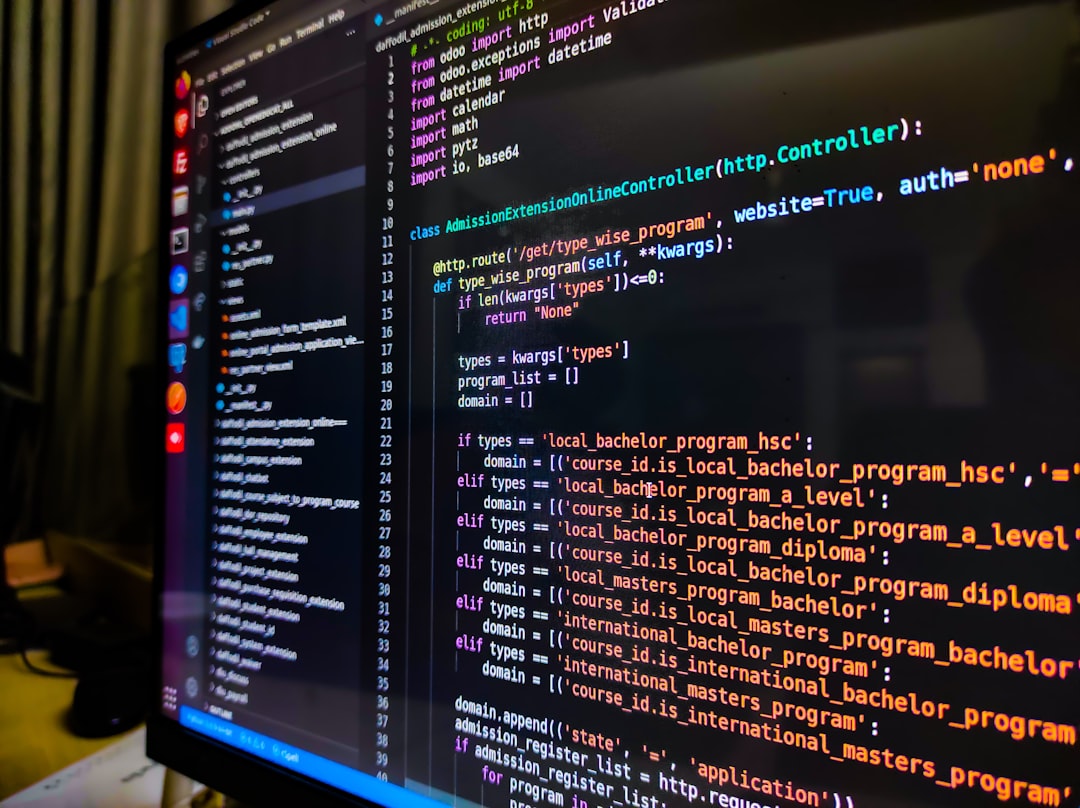
Combining `*args` and `**kwargs` in function definitions offers a powerful way to create flexible and adaptable Python functions. Using `*args` lets you accept an unlimited number of positional arguments, which are conveniently gathered into a tuple. Meanwhile, `**kwargs` provides a mechanism for accepting an arbitrary number of keyword arguments, organizing them into a dictionary. This synergy allows you to design functions that can seamlessly handle a wide range of inputs without needing to define fixed parameters.
The convenience of this approach comes with its own set of considerations. While using `*args` and `**kwargs` can make functions highly flexible, they can also introduce potential issues. As the number of arguments increases, code readability can suffer, making it challenging to understand the function's purpose and the purpose of each argument. Additionally, managing a growing number of arguments increases the potential for bugs and errors, highlighting the importance of clear documentation and careful function design to ensure maintainability. Striking a balance between flexibility and readability is crucial for effectively utilizing these powerful features in your Python code.
The combination of `*args` and `**kwargs` in Python function definitions is a powerful tool for creating functions that can accept a variable number of arguments. It's like having a Swiss Army Knife for your functions, giving you the ability to handle different input scenarios with ease.
Let's break down the key points about using both of these features:
1. **Order Matters**: In a function definition, `*args` must come before `**kwargs`. This is because Python needs to unpack the positional arguments first. This reinforces the order and clarity in function signatures.
2. **Tuples and Dictionaries**: `*args` collects positional arguments into a tuple, while `**kwargs` takes keyword arguments and turns them into a dictionary. Understanding this distinction is crucial for how you manage and manipulate arguments within your functions.
3. **Beyond Functions**: The power of `*args` and `**kwargs` extends to class methods as well. You can use them in class constructors to pass arguments dynamically, making inheritance and object creation even more flexible.
4. **Unpacking with Asterisks**: You can also use `*` and `**` to unpack arguments when calling functions. This lets you pass in collections or dictionaries directly, enhancing reusability and making your code more modular.
5. **Debugging Challenges**: While these features are flexible, they can add complexity to debugging. Error messages might be less specific, making it harder to pin down the root cause of an error.
6. **Argument Mixing**: It's possible to mix standard named arguments with `*args` and `**kwargs` in a single function definition. This offers a lot of flexibility, but you'll need to be careful to document your code clearly so it's easy for others to understand how to use it.
7. **Overloading Simplified**: By using `*args` and `**kwargs`, you can overload functions without explicitly defining every possible argument signature. This makes your code more adaptable and easier to maintain, as you can add new arguments without breaking existing calls.
8. **Order Preservation**: Starting with Python 3.7, `**kwargs` retains the order of keyword arguments as defined. This makes it easier to track and debug the sequence in which parameters were passed.
9. **Dynamic APIs**: Combining `*args` and `**kwargs` is ideal for building APIs that can handle optional and variable parameters. This lets you create adaptable endpoints that can meet the requirements of various clients without constant adjustments.
10. **Clash Prevention**: It's important to be aware that there's a risk of parameter name clashes when using both `*args` and `**kwargs`. Establishing clear naming conventions is essential, particularly in larger projects, to avoid unexpected behaviors from overlapping names.
As with any powerful tool, `*args` and `**kwargs` are best used with care. If you're mindful of potential pitfalls, they can add a level of flexibility and adaptability to your Python functions, allowing you to write cleaner, more adaptable, and more robust code.
Mastering Python's *args and **kwargs A Deep Dive into Arbitrary Parameters - Common Use Cases for Arbitrary Parameters in Python
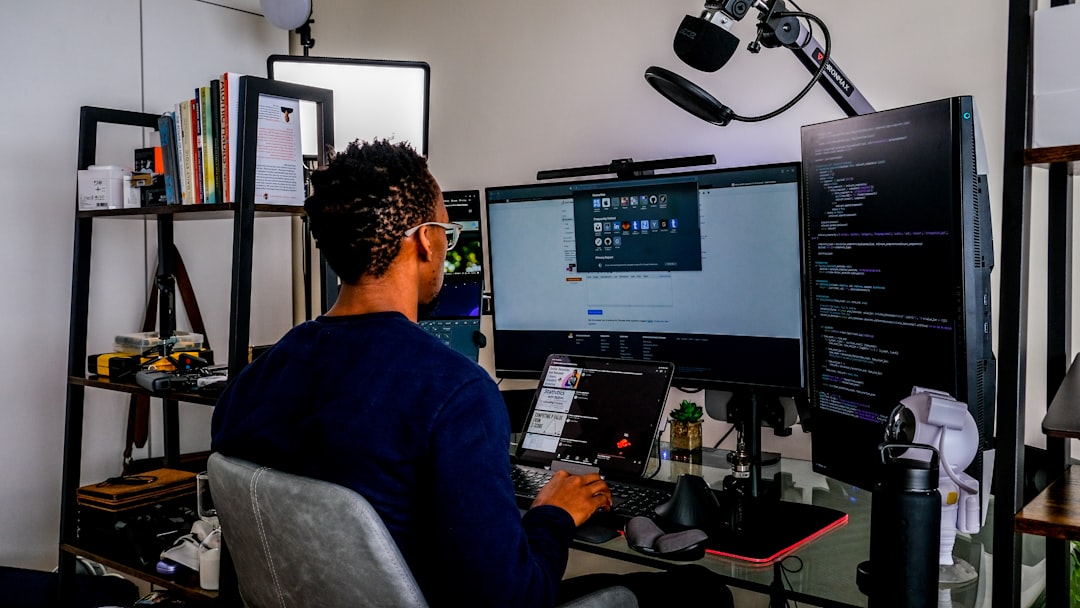
Arbitrary parameters like `*args` and `**kwargs` are powerful tools in Python that enhance the flexibility of function definitions. These allow functions to accept varying numbers of inputs, making them more adaptable to different situations. For example, a mathematical function can use `*args` to calculate the average of any number of provided numbers, or an API function might use `**kwargs` to handle a variety of optional parameters.
While this dynamic nature is valuable, it comes with potential drawbacks. Code readability can be hampered by the unpredictable nature of `*args` and `**kwargs`, making it challenging to understand the function's purpose and the role of each argument. Additionally, debugging can become more complex, as the lack of fixed arguments makes it harder to pinpoint the source of errors.
Therefore, when using these features, developers must strike a careful balance between flexibility and clarity. This involves clear documentation, well-structured code, and thoughtful design practices to ensure that the power of `*args` and `**kwargs` is harnessed without sacrificing maintainability.
The common use cases of `*args` and `**kwargs` in Python have intriguing implications. It's not just about accepting an unlimited number of arguments. For example, `*args` can aggregate results from multiple function calls. Imagine a statistical function that combines data from diverse sources – you can pass them as positional arguments using `*args`.
You might be surprised to learn that decorators rely on `*args` to pass arguments dynamically from the decorator to the wrapped function. This provides decorators with flexibility without hardcoding parameters.
You can use `*args` to initialize objects with a varying number of attributes, creating a flexible design pattern for objects. And when designing APIs, using `*args` and `**kwargs` allows clients to submit optional parameters, making for a much more usable interface.
Inheritance is another area where `*args` and `**kwargs` make their mark. When overriding methods in inherited classes, they simplify method signatures. This allows child classes to accommodate additional parameters while maintaining compatibility with parent class implementations.
And who knew `**kwargs` could enhance Python's functional programming capabilities? It enables quick parameter modifications on-the-fly. You can rapidly alter how functions operate with keyword arguments, enhancing functionality without breaking existing implementations.
You can even use `*args` in lambda functions to create adaptable inline functions. This opens the door for dynamic computations with fewer restrictions on input size.
It gets even more interesting when you think about how `**kwargs` can be used to unpack nested dictionaries into function arguments. This simplifies function calls involving complex data structures.
Logging and debugging also benefit from `**kwargs` . Logging functions can capture varying details about execution by using `**kwargs` to provide additional context dynamically. This improves debugging workflows.
Last but not least, `*args` and `**kwargs` support version compatibility. When modifying functions in libraries, you can add new parameters without disrupting existing functionality by using `*args` and `**kwargs`. This protects user experiences across different versions.
As you see, the possibilities with `*args` and `**kwargs` go beyond simple argument handling. It's important to approach them with a discerning eye to unlock their full potential, but their versatility adds a remarkable dimension to Python's expressiveness.
Mastering Python's *args and **kwargs A Deep Dive into Arbitrary Parameters - Advanced Techniques for Leveraging Arbitrary Parameters in Python
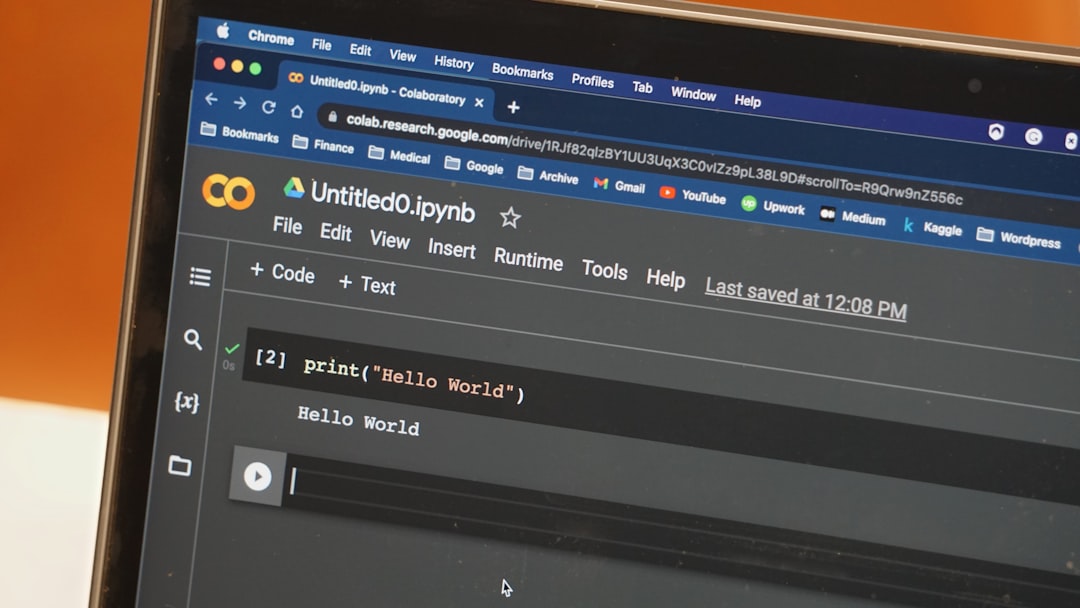
The section on "Advanced Techniques for Leveraging Arbitrary Parameters in Python" explores more advanced ways to utilize `*args` and `**kwargs`. It dives into how these powerful features can be used to create flexible and well-maintained code. These tools are essential for building functions that can adapt to various situations, such as handling dynamic inputs or designing robust APIs. By combining `*args` and `**kwargs` effectively, developers can write functions that handle a variable number of arguments, both positional and keyword-based. However, as with any powerful tool, there are downsides. As the complexity increases, it can make debugging harder and reduce the readability of the code. This highlights the crucial need to carefully consider both flexibility and clarity. While leveraging `*args` and `**kwargs` provides valuable adaptability, maintaining clear and well-documented code remains paramount to avoid potential pitfalls.
`*args` and `**kwargs` are powerful tools in Python for creating functions that can adapt to different input scenarios. However, their flexibility comes with a few considerations.
First, while these features make your code more concise, they can also make it more difficult to read and debug. It's important to document your code well and to use clear variable names.
Second, there can be performance trade-offs when using `*args` and `**kwargs`. These features often involve additional overhead for argument unpacking, which can impact execution speed in performance-critical applications.
Finally, with the introduction of positional-only parameters in Python 3.8, the use of `*args` becomes a bit more complex. You need to pay attention to function signatures to ensure compatibility between positional-only parameters and variable-length parameters.
But, despite these challenges, `*args` and `**kwargs` remain powerful tools that can make your code more flexible and adaptable. They can be used in a variety of scenarios, such as:
* **Dynamic Method Resolution**: Using these features allows functions to dynamically resolve the methods they need to invoke based on the input parameters. This is particularly useful for libraries and frameworks that need to be highly configurable.
* **Data Class Initialization**: `*args` and `**kwargs` can streamline the initialization of data classes.
* **API Versioning Flexibility**: `*args` and `**kwargs` allow new parameters to be added to APIs without breaking existing endpoints.
* **Functional Testing Scenarios**: You can use these features to iterate through a variety of input cases in parameterized tests.
* **Decorator Customization**: Decorators often use these features to accept and pass any number of arguments to the wrapped function, allowing them to remain flexible and reusable.
* **Overriding Built-in Functions**: `*args` and `**kwargs` can make your custom implementations of built-in functions more adaptable.
* **Debugging Strategies**: While these features can obscure the root causes of errors, implementing robust logging mechanisms can help you identify issues effectively.
As a researcher or engineer, I find `*args` and `**kwargs` to be fascinating aspects of Python. They offer a great deal of power and flexibility, but like any tool, it's essential to use them with care and to be aware of their potential pitfalls.
Create AI-powered tutorials effortlessly: Learn, teach, and share knowledge with our intuitive platform. (Get started for free)
More Posts from aitutorialmaker.com: