Efficient String Concatenation Techniques in C Beyond strcat
Efficient String Concatenation Techniques in C Beyond strcat - Manual character-by-character concatenation
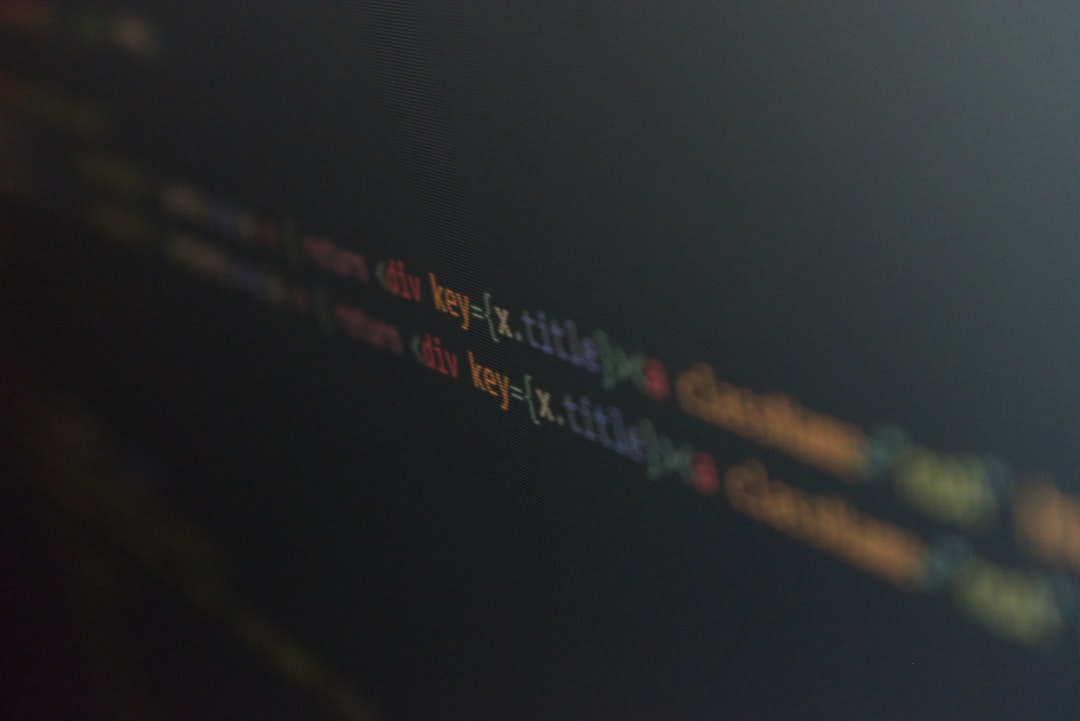
Manual character-by-character concatenation is a straightforward, yet potentially fragile, technique for combining strings. It involves looping through each character of the source string and individually placing it at the end of the destination string. This method provides a granular level of control over the concatenation process, potentially bypassing certain issues associated with higher-level functions like `strcat`. However, it's also susceptible to errors if not carefully implemented. Maintaining correct memory management and ensuring sufficient buffer space becomes the developer's responsibility.
While understanding this low-level approach is beneficial for grasping how string manipulation works, it often lacks the efficiency and robustness of other methods. Developers should assess if the added control truly outweighs the increased risk of mistakes and the possible impact on performance. Ultimately, opting for a balance between control and efficiency when building and manipulating strings in C is a key goal. This approach offers a good understanding of the mechanics of string concatenation, but in practice, its drawbacks often necessitate exploration of alternative techniques.
While conceptually simple, manually concatenating strings character by character can introduce significant performance bottlenecks. Each concatenation often entails iterating through the entire destination string, leading to a time complexity of O(N^2) when concatenating N strings. This can dramatically slow down programs, especially with a growing number of concatenations.
Furthermore, this approach is prone to errors. Manually managing buffer sizes and null terminators requires extreme care, making it easy to introduce buffer overflows or memory leaks that can be very difficult to track down. Unlike library functions like `strcat` or `snprintf`, manual concatenation doesn't leverage internal optimizations, such as memory pooling or pre-allocation, leading to more frequent and potentially inefficient memory allocation calls.
Moreover, the compiler has a harder time optimizing code that uses manual string concatenation. The unpredictable memory access patterns and data dependencies make it difficult to apply many standard optimization techniques.
It's also worth noting that many beginners may initially gravitate towards manual concatenation without recognizing the availability of specialized, optimized library functions. These functions are designed to handle many potential pitfalls, ultimately leading to better performance and reliability.
The size of a string increases exponentially with manual concatenation. If you begin with a string of size N, each new concatenation may require copying all N existing characters, exacerbating the performance issue.
Additionally, repeated allocation and deallocation in long-running applications can lead to memory fragmentation as string sizes grow. This fragmentation can impact overall system performance and make it harder to allocate large chunks of contiguous memory.
Traditionally, manual concatenation might be presented in educational settings to help understand fundamental string manipulation. However, this approach often doesn't reflect best practices in real-world applications.
When working with Unicode characters, the challenges increase. Variable-length encoding schemes require careful handling to avoid data corruption during concatenation.
Interestingly, while typically not the best approach for performance or reliability, manual concatenation provides granular control over the string construction process. This flexibility can be beneficial for specialized tasks involving formatting or character escaping, although these operations can be tedious using standard library functions.
Efficient String Concatenation Techniques in C Beyond strcat - Using memcpy and strlen for faster concatenation
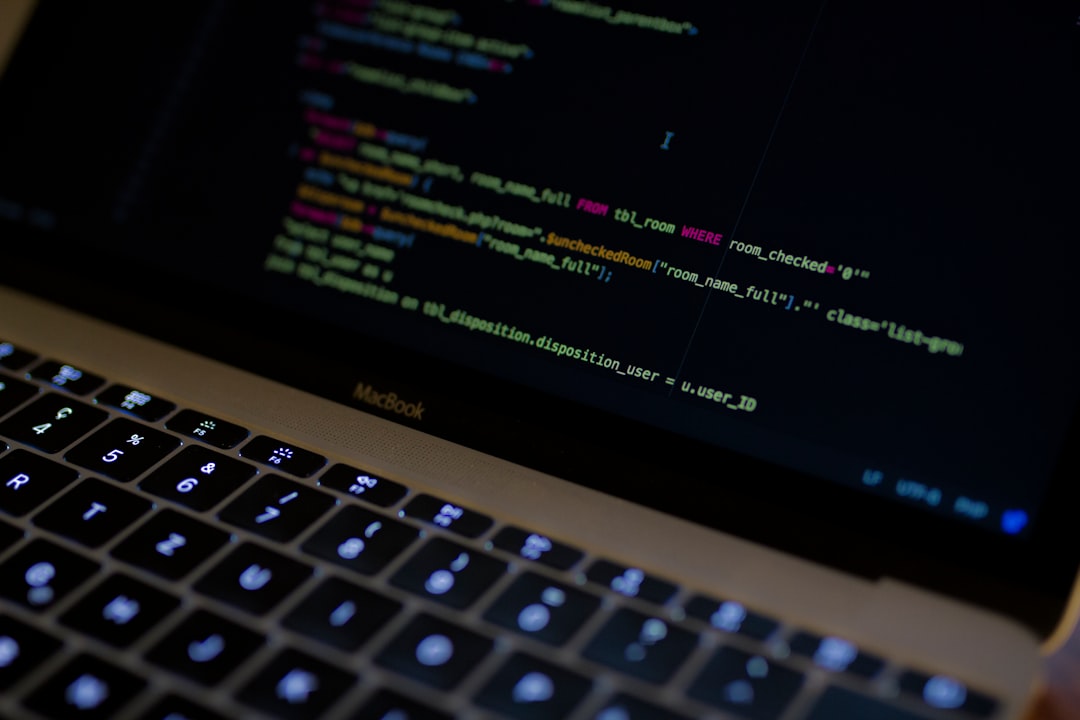
When aiming for faster string concatenation in C, exploring alternatives to `strcat` can yield significant improvements. One approach involves using `memcpy` and `strlen` for more efficient memory copying. Instead of character-by-character copying, `memcpy` can swiftly copy entire memory blocks, especially advantageous when you know the length of the source string being appended. However, relying on `memcpy` requires mindful attention to potential pitfalls. If a string is not null-terminated, `strlen` might provide an inaccurate length, risking buffer overflows. To mitigate this risk and optimize further, consider allocating a single memory block for all concatenated strings. This approach minimizes memory fragmentation, which can enhance performance, particularly in applications where memory resources are constrained. While offering a performance boost in suitable scenarios, this technique needs cautious implementation due to the potential for buffer overflows if not used responsibly.
Here are ten interesting points regarding the use of `memcpy` and `strlen` for potentially faster string concatenation within the C language:
1. **Reduced Overhead**: Employing `memcpy` for string concatenation offers a reduction in function call overhead compared to techniques like `strcat`. This is due to `memcpy`'s ability to copy memory blocks directly, which often bypasses multiple pointer dereferences and null-termination checks.
2. **Improved Time Complexity**: Utilizing `memcpy` can significantly reduce the time complexity of concatenation operations, from the potentially quadratic O(N^2) complexity seen in character-by-character approaches to a more manageable O(N). This attribute makes `memcpy` more suitable for operations on larger datasets.
3. **Enhanced Memory Management**: `memcpy`'s ability to copy data in bulk, as opposed to the incremental character-by-character approach of some other methods, improves overall memory utilization. It minimizes the number of calls to memory allocation functions, potentially resulting in improved performance.
4. **Potentially Better Buffer Management**: When used properly, `memcpy` helps to create contiguous blocks of memory when concatenating strings. This can be advantageous because it reduces the potential for memory fragmentation, especially during repeated dynamic allocation calls.
5. **Reduced Null Terminator Dependencies**: Unlike some string operations, `memcpy` doesn't inherently rely on null-terminated strings during the copying process. This can simplify development when working with various string structures and reduces the possibility of common string handling errors related to forgetting to manage null terminators.
6. **Compiler Optimization Opportunities**: The nature of block memory operations like `memcpy` allows modern compilers to apply more aggressive optimizations. This includes leveraging specific CPU features to improve the speed of the copy operation, potentially achieving faster execution times than with higher-level string functions.
7. **Improved Efficiency with Large Strings**: When dealing with very large strings, `memcpy`'s ability to perform bulk copying operations can help minimize the number of data moves. This is especially beneficial when concatenating strings of known sizes as developers can pre-allocate memory in a more optimal way.
8. **Leveraging Platform Specific Optimizations**: Compilers often offer optimized implementations of `memcpy` tailored for the underlying hardware. These versions can offer significant performance gains, especially when dealing with substantial memory blocks. This makes `memcpy` potentially more advantageous in specific contexts.
9. **Faster Buffer Size Determination**: When string lengths are relatively small, calculating the length with `strlen` takes constant time, or O(1). In combination with `memcpy`, this allows developers to easily determine the necessary size of the destination buffer when preparing for concatenation.
10. **Need for Careful Management**: Despite the potential speed benefits, using `memcpy` requires careful management. Developers must ensure the destination buffer has sufficient space to accommodate the concatenated string to prevent buffer overflows. Handling these allocation details properly becomes an added responsibility that might make the code more complex if not handled carefully.
While `memcpy` offers a pathway to potentially faster string concatenation, understanding its limitations and potential drawbacks is important for responsible use. It provides a useful illustration of how optimizing string manipulations can be achieved through the careful use of lower-level memory operations.
Efficient String Concatenation Techniques in C Beyond strcat - Implementing a custom string_concat function
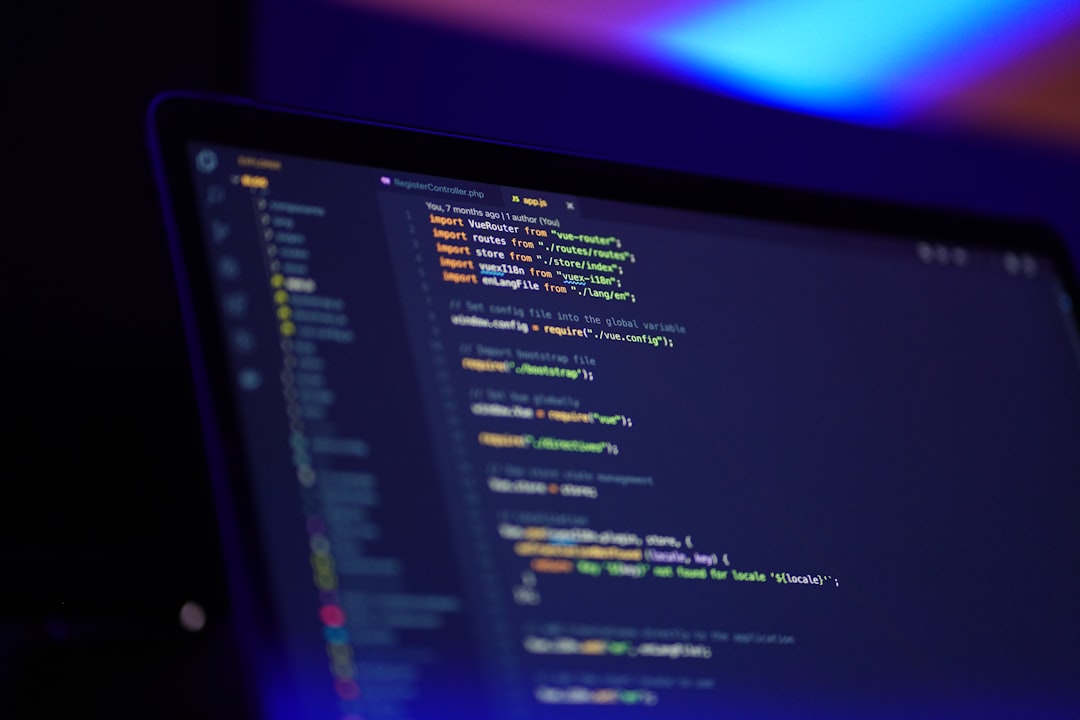
Crafting a custom `string_concat` function offers a path to improve how strings are handled in C, going beyond standard tools like `strcat`. This approach empowers developers to shape the concatenation process to fit their specific needs, potentially optimizing memory usage and improving speed. One tactic to enhance performance is to cache the lengths of the strings involved before starting the concatenation. This can prevent repetitive, time-consuming length calculations. However, a crucial concern is the danger of buffer overflows. With a custom function, managing memory and allocating space for the resulting string becomes the programmer's responsibility, demanding careful attention to avoid errors. While a tailored solution can deliver efficiency gains, programmers should carefully consider if the added complexity justifies the potential performance advantages.
Crafting a custom `string_concat` function in C opens up intriguing possibilities for optimizing string concatenation beyond the capabilities of standard functions like `strcat`. By carefully designing the function's implementation, we can potentially achieve significant performance gains and enhance safety.
One compelling aspect is the ability to leverage hardware memory alignment. By structuring the function to copy data using methods like `memcpy`, we might take advantage of the processor's ability to access aligned data more efficiently, resulting in faster string operations. This is particularly relevant for modern processors that excel at working with aligned blocks of memory.
Moreover, a custom function can potentially sidestep the overhead associated with multiple function calls inherent in functions like `strcat`. Instead of treating each concatenation as a separate call, a custom `string_concat` function can potentially batch multiple concatenations together, streamlining the process and reducing the number of context switches. This can be incredibly beneficial when dealing with scenarios where a series of strings need to be combined.
Furthermore, a custom implementation offers the chance to employ more intelligent memory management strategies. Instead of incrementally growing strings, leading to frequent reallocations, we can design `string_concat` to anticipate the final string size and allocate the memory upfront. This can significantly reduce the number of times memory is reallocated, leading to smoother and potentially faster string concatenation operations.
Of course, crafting a robust custom function also provides an opportunity to enhance safety by incorporating comprehensive buffer overflow checks. Unlike some standard functions, where buffer overflows can occur due to inadequate validation, a custom function can enforce buffer bounds and guard against these potentially disastrous errors. This level of care is important for developing more secure software.
Furthermore, a custom function can explore advanced memory management techniques, such as memory pooling. By allocating strings from a pre-allocated buffer, we might be able to decrease the frequency of calls to `malloc`, minimizing overhead and potentially smoothing out performance variations in resource-constrained environments.
Beyond these aspects, a custom implementation gives us a higher degree of control over the string formatting process during concatenation. We can implement specific formatting styles, inject delimiters, or handle various character encodings more easily than standard functions might allow. This added flexibility can be particularly beneficial when we need highly customized output strings.
However, custom functions can benefit from specialized optimizations when we have a good understanding of the scenarios where they'll be employed. Analyzing expected string sizes and types allows us to fine-tune the implementation, potentially maximizing efficiency for specific use cases.
Similarly, we can potentially implement batch processing for further optimization. By concatenating multiple strings at once, rather than iteratively, the overall execution time can be significantly reduced. This is particularly relevant for applications where many strings need to be joined efficiently.
Another subtle advantage of custom functions lies in the ability to provide helpful hints to the compiler about the characteristics of input strings. These hints can potentially enable further compiler optimizations that are not available for generic string functions like `strcat`. This ability to leverage compiler expertise can boost performance even further.
Finally, it's worth noting that crafting a custom `string_concat` function can enable better adaptation to different string types and character encodings. We can design it to accommodate wide characters, multibyte characters, and various encoding schemes, leading to greater flexibility and adaptability.
While developing and deploying a custom `string_concat` function carries its own development effort, it clearly holds the potential to enhance both performance and safety. It highlights the intricate world of string manipulation and shows that thoughtfully considering the underlying mechanisms can lead to meaningful improvements for certain applications.
Efficient String Concatenation Techniques in C Beyond strcat - Leveraging sprintf for formatted string joining
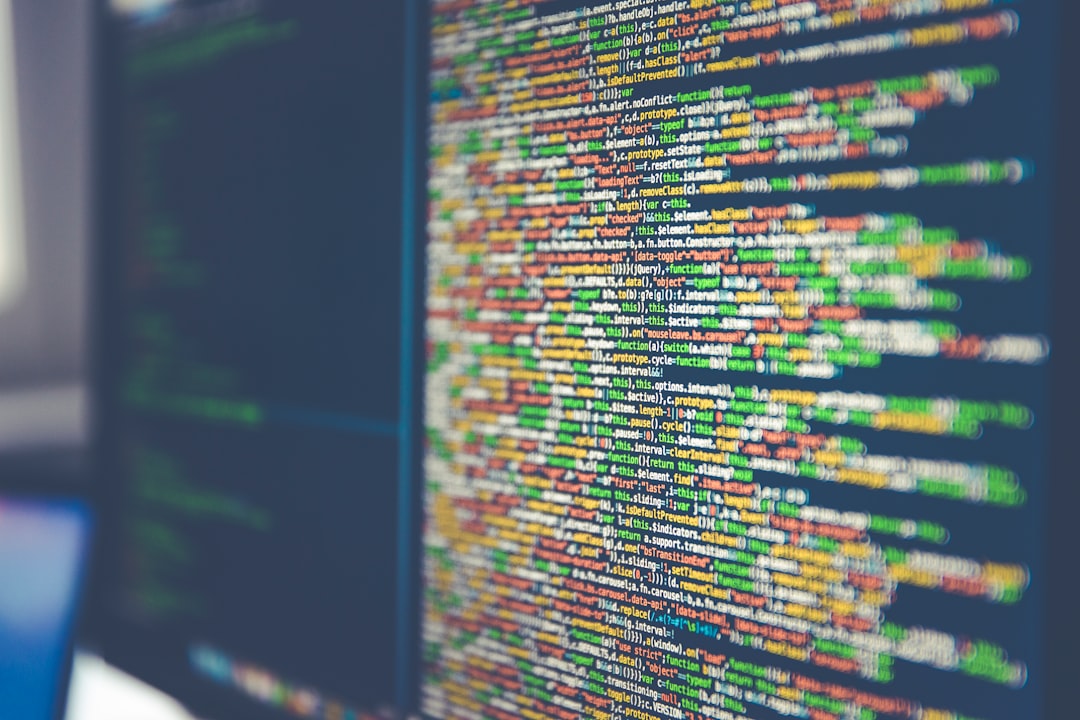
`sprintf` offers a valuable approach to string concatenation in C, surpassing the limitations of simpler methods like `strcat`. By integrating format specifiers, it allows for seamless combination of strings and variables into a formatted output with a single function call. This can avoid the performance drawbacks of repeatedly finding the end of a destination string, a problem that can arise with some concatenation approaches. However, using `sprintf` responsibly requires attention to buffer management to prevent potential overflows. A safer alternative, `snprintf`, offers a solution by letting programmers specify the maximum size of the output buffer, helping to avoid unintended buffer overruns. This approach represents a practical and efficient technique for formatted string concatenation in C, highlighting the need for vigilant buffer handling when working with these powerful string manipulation tools.
The `sprintf` function offers a way to combine string literals and variable values into a formatted string, providing an alternative to `strcat` for string concatenation. However, it introduces a different set of considerations. While `sprintf` can handle diverse data types, its formatting capability comes at a performance cost due to the overhead of parsing format specifiers. This makes it potentially slower than simpler approaches for straightforward string joins.
Unlike `strcat`'s in-place appending, `sprintf` can produce new strings when used with buffer management techniques. Developers need to carefully allocate enough memory to store the formatted output, otherwise, buffer overflows can arise, especially in scenarios involving loops. One benefit of `sprintf` is that it automatically null-terminates the resulting string, eliminating the manual management of string boundaries needed with other techniques.
The true strength of `sprintf` is in its format specifiers. Developers have control over aspects like width, precision, and more, making it a good choice for applications that demand precise output like logs or reports. Furthermore, `snprintf` exists as a safer version, helping prevent buffer overflows by limiting the number of characters written.
`sprintf` comes with a wide array of format options, including left and right alignment, and control over scientific notation. This makes it a valuable tool in fields requiring highly structured string output. Yet, because `sprintf` relies on parsing the format string at runtime, it might not always allow for the same degree of optimization as specialized functions that can be optimized during compilation.
Security is a concern with `sprintf` since buffer overflows are a risk if buffer sizes are not properly handled. Therefore, using techniques like `snprintf` is encouraged for production code. Lastly, `sprintf` integrates well with C libraries that expect formatted strings, resulting in cleaner codebases. However, it's important to use it judiciously and avoid potential issues arising from mismanagement of format strings and buffers.
Efficient String Concatenation Techniques in C Beyond strcat - Buffer management techniques for efficient concatenation
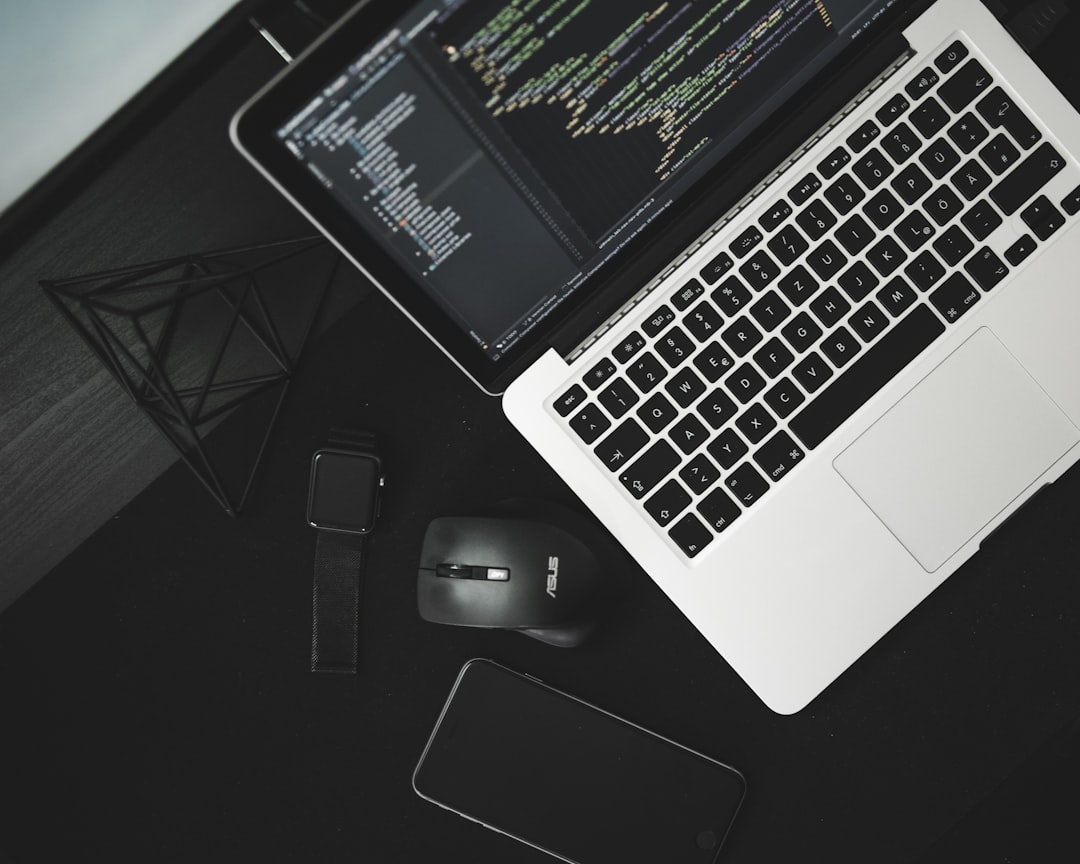
When it comes to efficient string concatenation in C, buffer management techniques become critically important. Unlike simpler methods like `strcat` which can often lead to a lot of memory being allocated in inefficient ways, managing buffers effectively involves carefully planning ahead. This means figuring out the total size of the string you need before you start concatenating and allocating the necessary memory space just once. This approach greatly reduces memory fragmentation and improves performance. Furthermore, methods like using `memcpy` for copying large chunks of data can speed up the concatenation process significantly, making it faster. However, it's crucial to handle memory responsibly with `memcpy` to prevent any buffer overflows. Overall, smart buffer management isn't just about speed, it also helps create C applications that are more stable and reliable. By being strategic about how buffers are used, concatenation operations can be streamlined and overall program performance enhanced.
When it comes to efficient string concatenation, managing buffers effectively is crucial. One clever approach is **memory pooling**, where we allocate a single, large chunk of memory and reuse portions of it for storing multiple strings during concatenation. This method can significantly reduce memory fragmentation and the overhead that comes with constantly allocating and deallocating smaller memory blocks.
Furthermore, buffer management often involves employing sophisticated **copying strategies**. This might include clever tricks like unrolled loops, which basically reduce the number of times the loop checks if it's done, or even specialized CPU instructions (SIMD) that can copy multiple pieces of data at once. These can offer major speed gains over simpler approaches.
Another essential concept is **pre-allocation**. By carefully estimating the total size of all the strings that will be combined, we can allocate enough memory space upfront. This strategy helps to avoid frequent reallocations, which can be a huge drain on performance, especially when dealing with large strings. It also helps keep data in the same area in memory, improving how quickly the CPU can access it.
Interestingly, **adaptive buffering** offers a dynamic approach. This technique can adjust the buffer size on the fly based on the anticipated workload. For instance, if a lot of concatenation operations are anticipated, the buffer can be expanded accordingly. Similarly, if the workload is lighter, the buffer might be smaller, helping optimize resources. This adds a level of intelligence to buffer management that can improve responsiveness under different circumstances.
Buffer management can be pushed even further to enable **concurrent operations**. That is, multiple threads could work on different buffers concurrently. This is incredibly useful when running on multi-core processors, as it allows us to break down the work and reduce potential bottlenecks.
Efficient techniques can also capitalize on **CPU cache utilization**. With larger strings, understanding how data is organized in memory is important, as it directly influences how well the CPU cache can work. Minimizing cache misses can dramatically improve speed, particularly when manipulating larger chunks of text.
Introducing **reference counting** into the mix is another interesting approach. By keeping track of the number of times a specific buffer is being used, we can get more granular control over when the memory is actually freed, enhancing memory management.
Naturally, **robust error handling** is vital. By embedding checks at various stages of the concatenation process, we can mitigate the risk of buffer overruns, leading to a more robust and secure application. This meticulous attention to detail is critical in software reliability.
In some cases, **function inlining** can prove useful. Replacing short buffer-related operations with inline code can bypass the overhead of regular function calls. This also gives the compiler more opportunities to optimize the code for specific conditions.
Finally, we can even leverage **compiler hints** within our buffer management techniques. Giving the compiler information about the sizes and data types involved in our concatenations allows it to produce more optimized machine code. This fine-tuning can unlock further speed increases compared to more generic solutions.
While the various buffer management techniques discussed here can significantly improve the efficiency of string concatenation in C, it's important to understand the context and select the method most appropriate for each particular situation. A researcher's curiosity, however, leads them to explore these techniques further, for the sake of it.
Efficient String Concatenation Techniques in C Beyond strcat - Optimizing concatenation in loops and repetitive operations
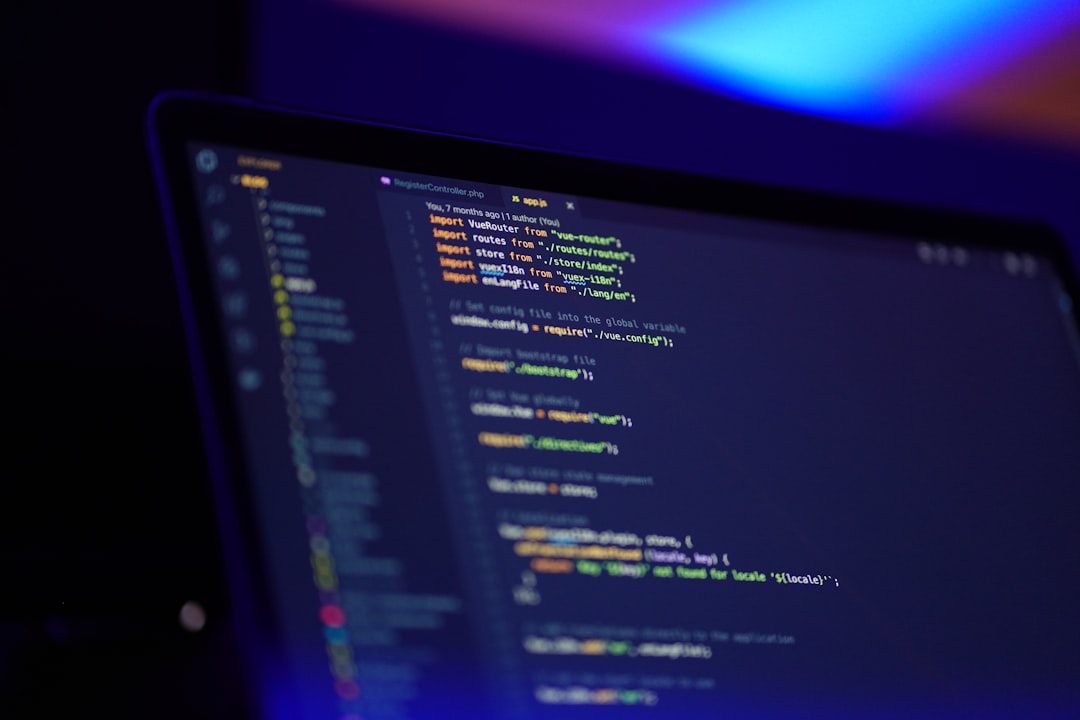
When dealing with string concatenation within loops or repetitive operations in C, achieving optimal performance is key. The standard approach using functions like `strcat` can introduce performance bottlenecks if not handled carefully. These bottlenecks often stem from repeated memory allocation and string traversal, especially when the size of the final string isn't known beforehand.
To mitigate these issues, consider pre-allocating a buffer large enough to hold the expected concatenated string. This pre-allocation minimizes the need for repeated memory reallocations, which can be a major cause of performance degradation, especially in loops. Moreover, leveraging functions like `memcpy` to copy string segments can significantly accelerate concatenation.
While these techniques offer a path to efficiency, it's paramount to maintain careful control over buffer management. Failing to manage buffer sizes appropriately can lead to a variety of problems, including buffer overflows and increased memory fragmentation. These issues can undermine the stability and performance of the application, potentially impacting the user experience and program reliability. Thus, striking a balance between efficient concatenation techniques and careful buffer management is essential for crafting high-performing and robust C code when dealing with string operations in repetitive contexts.
In loops and repetitive operations, inefficient string concatenation can significantly impact performance. One common issue is the repeated allocation of memory for the destination string on each concatenation, leading to extra overhead and slower execution times. Furthermore, as the destination string grows in size with each concatenation, the time needed to copy the existing content increases exponentially, potentially leading to a time complexity of O(N^2) where N is the number of concatenations. This can make the compiler's job of optimization harder since it encounters erratic memory access patterns that are challenging to streamline.
Another aspect to consider is memory fragmentation, which arises from frequent memory allocation and deallocation during the concatenation process. As the program continues, fragmented memory makes it harder to allocate larger contiguous blocks of memory, potentially leading to performance degradation. Similarly, ineffective string concatenation can result in increased cache misses, especially when handling larger strings. When the CPU needs to fetch data from slower main memory instead of the faster cache, performance suffers.
One possible solution is to first calculate the total length of the concatenated string and allocate the necessary memory in one go, thus avoiding repeated allocations within the loop. This type of proactive memory management significantly improves efficiency. It's also worth noting that employing string concatenation within multithreaded environments can complicate memory management. Shared memory locations can introduce race conditions unless they are handled carefully, presenting a challenge for maintaining thread safety.
To optimize this, one could consider using a memory pool. By initially allocating a large block of memory and partitioning it for various string operations, we can reduce the overhead of constant allocation requests. Utilizing Single Instruction, Multiple Data (SIMD) instructions offers another approach. SIMD can enable parallel manipulation of multiple string data units, potentially accelerating the concatenation process considerably.
Finally, implementing more advanced buffer management strategies, such as dynamic resizing or integrating reference counting, can contribute to both safety and efficiency. These features can help to minimize buffer overflows while ensuring optimal resource use. The interplay between buffer management and loop-based concatenation reveals intricate connections that can improve string handling in C. This area, I think, warrants continued investigation to identify potential further refinements for diverse situations and workloads.
More Posts from aitutorialmaker.com: