Using CSS Custom Properties with JavaScript A Deep Dive into Dynamic Style Management
Using CSS Custom Properties with JavaScript A Deep Dive into Dynamic Style Management - Basic Setup For CSS Variables With Root Level Declarations And JavaScript Handlers
Let's explore a foundational setup for using CSS variables effectively with JavaScript. The core idea is to define your custom properties at the root level of your stylesheet, typically using the `:root` pseudo-class, which essentially targets the `` element. This approach makes them globally available throughout your CSS, providing easy access for consistent styling across your site.
JavaScript steps in to make things dynamic. By leveraging the DOM, JavaScript can directly modify the styles associated with the root element, essentially changing the values of these variables. This means that user actions like clicks or other events can trigger style updates, leading to responsive and interactive experiences. This approach simplifies maintaining styles as you can define a variable once and use it in multiple places, adhering to the DRY (Don't Repeat Yourself) principle.
This combination of root-level CSS variable declaration and JavaScript manipulation allows for efficient and sophisticated style management without the need for a page reload each time styles change. It's a powerful technique for creating designs that react to user behavior and enhance the overall user experience.
1. CSS variables, also known as custom properties, are denoted with a double hyphen (`--`) prefix, making them easily identifiable within your stylesheets and providing a clear pathway for JavaScript interaction. This simple syntax makes manipulating them from JavaScript a straightforward process.
2. The `:root` pseudo-class provides a natural location for defining CSS variables that apply globally across your stylesheet. Since it represents the `` element, any variable declared at this level becomes a baseline for styling and eliminates the need for repetitive variable declarations in individual elements.
3. One of the compelling features of CSS variables is that they offer immediate style updates. Unlike traditional CSS or preprocessor variables, changes made through JavaScript are immediately reflected in the browser, giving you live feedback and making dynamic UI updates a smooth experience.
4. The beauty of CSS variables goes beyond basic declarations, they can also inherit values from other variables. This cascading behavior, allowing variables to influence one another, allows developers to craft complex style relationships in a way that still feels organized and maintainable.
5. When incorporating CSS variables with JavaScript, you can utilize familiar methods such as `document.documentElement.style.setProperty()` to change a variable's value. This integration of CSS variables and JavaScript opens the door to creating dynamic user interfaces responsive to user actions or environmental changes.
6. JavaScript's `getComputedStyle()` function offers a way to inspect the computed value of a CSS variable at any given point. This is a powerful debugging and analysis tool that reveals the actual style applied to an element after cascading and inheritance are accounted for.
7. The usefulness of CSS variables extends beyond just color values; they can store values for various CSS properties such as dimensions, timing functions, or almost any other property. This gives designers the tools to manage a wider range of style attributes without bloating the CSS with redundant code.
8. The cascading and inheritance principles that are part of CSS naturally extend to custom properties as well. They can be overridden within specific CSS rules, but also preserve a global default established at a higher level. While this offers great flexibility, it can increase complexity if you are working with very large stylesheets where many rules might impact a single variable.
9. A key difference between CSS variables and traditional preprocessor solutions like Sass or Less is their direct implementation. CSS variables do not need a separate compilation step before they are used in a browser. This streamlined approach makes development workflow smoother, as changes made to a variable are immediately reflected on the webpage.
10. Another use case for CSS variables is in creating responsive designs through media queries. Defining CSS variables within media query blocks allows style rules to be adaptive, effortlessly adjusting to different viewport sizes or other device characteristics, without repeating large quantities of CSS across various media query blocks. This approach highlights the efficiency and adaptability CSS variables provide in modern web development.
Using CSS Custom Properties with JavaScript A Deep Dive into Dynamic Style Management - Manipulating Variables Through getComputedStyle And setProperty Methods
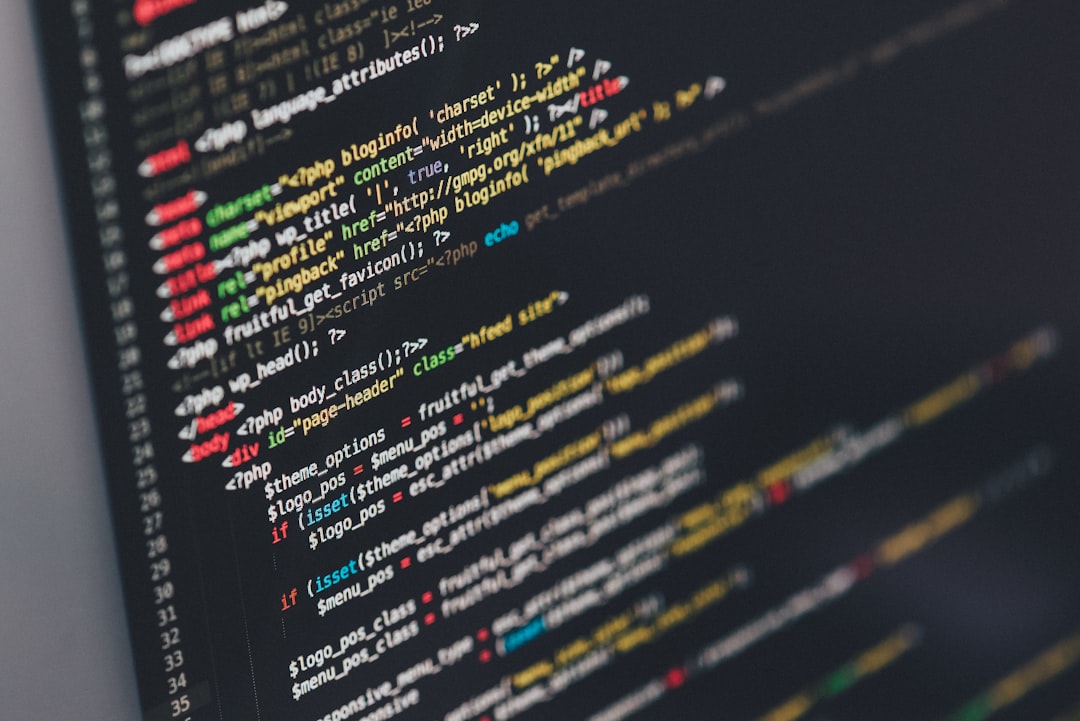
Using JavaScript to directly manipulate CSS custom properties offers a dynamic approach to styling. The `getComputedStyle` method is crucial for gaining insight into the currently applied style values of an element. It provides a snapshot of how CSS variables and other styles interact, including any cascading effects. Armed with this knowledge, you can use the `setProperty` method to directly modify custom property values within your JavaScript. This lets you dynamically change the appearance of elements based on user interactions or other events. This approach is advantageous as it helps prevent the use of inline styles, which often lead to messy and harder-to-maintain code. Instead, you can keep all your style related logic centralized and organized. The result is a cleaner codebase that offers better control over style changes and improves the responsiveness and interactivity of your website. This method promotes a more interactive and engaging user experience.
1. Integrating JavaScript with CSS variables opens up a new realm of interactivity, allowing for dynamic styles previously mostly found within JavaScript frameworks. This gives CSS developers more control over creating dynamic designs without needing to heavily rely on complex JavaScript structures.
2. The `getComputedStyle()` method is not just for getting the current value of a CSS variable. It's also an important debugging tool. It helps us understand how styles cascade and what the final rendered value will be in the browser. This is crucial for keeping track of how changes in one part of your stylesheet might affect other parts.
3. You can use CSS variables along with the `calc()` function to perform calculations directly within your stylesheet. This makes responsive design easier as elements can adapt to changes in your variables, rather than having to use hardcoded values.
4. Unlike JavaScript variables, where you can change the value anytime, CSS variables are more stable. They act as a reference point that doesn't change unless you specifically tell it to. This predictability is good for consistency in larger projects, but it can also limit flexibility in some scenarios.
5. When you use `setProperty()`, there's an option to add `!important` flags. This gives you finer control over style specificity. You can change styles without affecting the core stylesheet, reducing the risk of unintended side-effects.
6. Modifying styles by changing custom properties often performs better than adding or removing entire classes. This is because the browser's work in recalculating styles and rendering the page is generally less. This results in smoother user experiences, particularly in complex UI changes.
7. CSS variables have a limited scope, by default they inherit values. This contained environment makes developing styles easier, but it can make things a bit more complex when trying to track how changes made in one part of a design spread to other parts, especially with nested components.
8. The ability to define a variable once and use it for multiple properties helps eliminate unnecessary duplication in the CSS. This improves maintainability because you have a centralized place to manage changes that can impact many areas at once, but this also creates dependency management problems when the variable changes.
9. CSS variables become particularly useful when managing themes in a website or application. When you want to change a theme, you can change just a single variable. This can save a lot of time compared to manually updating multiple stylesheets or classes.
10. Debugging CSS alongside JavaScript is often easier with CSS variables. You can test adjustments in variables to see how they impact the overall styles without having to rewrite chunks of CSS. This allows for faster and easier iterative design, but sometimes these changes do not update the web page or other parts of your program until a cache is cleared, or you refresh the web page, or you reload your application.
Using CSS Custom Properties with JavaScript A Deep Dive into Dynamic Style Management - Understanding CSS Variable Inheritance And Component Level Scope Management
Understanding how CSS variables inherit values and how to manage their scope within components is crucial when using custom properties. CSS variables, by default, inherit values from their parent elements, creating a flexible and structured styling system. This inheritance can be beneficial, but in larger projects with nested components, it can introduce complexities if not managed carefully. Developers need to carefully consider how variables are defined and how they are overridden throughout the stylesheet to prevent unexpected behavior and maintain a clean codebase. By utilizing custom properties for component-specific styles, you can both enhance the maintainability of your code and enable styles to react to user interaction, leading to a better user experience. Maintaining a clear understanding of how CSS variables inherit and managing their scope within your components is a key step in efficiently leveraging their power while preventing unintended cascading effects that can make stylesheets difficult to understand and maintain. While the DRY (Don't Repeat Yourself) principle is beneficial, it's also important to strike a balance between reusability and understanding where your CSS variables may be affecting other portions of the application.
CSS variables, being part of the CSS cascade, behave similarly to other CSS properties when it comes to inheritance. A child element, for instance, can automatically pick up the value of a variable defined in its parent. This makes it easier to manage variables in complex, nested components. Unlike how JavaScript scopes work, where you might confine variables to specific functions or blocks, CSS variables can be tied to specific selectors. This means you can have different values for the same variable name depending on where it's used, helping to create more modular styles without the chance of accidentally conflicting with global settings.
Currently, most major web browsers support CSS variables well, so you can confidently use them in projects meant to be released to users. Because of the way they're part of the CSS cascade, changes to a variable in a parent element will instantly update any child elements that inherit it. This is quite handy for responsive designs as you can make adjustments to large areas without having to write tons of redundant code.
The way we access CSS variables, through an element's `style` property, makes it possible for them to work dynamically with the DOM beyond just JavaScript tweaks. This even opens the door to animations triggered by changes in variable values. While CSS variables can make things like creating themes and responsive designs much easier, it's essential to be mindful that mismanagement can lead to some tricky situations. For example, if styles are constantly changing, you might end up with unexpected outcomes if you don't keep your CSS organized.
There aren't any specific precedence rules for CSS variables in the same way that we see with regular CSS properties. This means the order of declaration is what matters when determining which value is used. Depending on how your CSS is set up, this can be either helpful or a source of extra complexity. One neat trick with CSS variables is the ability to set "fallback values". This means you can specify a default value in case a variable isn't defined or has an invalid value at a specific point. It's a great way to make styles more resilient.
Using CSS variables can also lead to improvements in how quickly a page renders. They reduce the need to manipulate class names or use inline styles with JavaScript, which in turn reduces the number of "reflows" and "repaints" the browser needs to do. These are computationally expensive tasks that can affect performance. If you use CSS variables with JavaScript, you can create sophisticated interactions. For instance, you can build interactive themes that change based on user actions. This results in styles that adapt to the current state of your application and enhances the overall user experience without introducing a huge amount of extra code.
Using CSS Custom Properties with JavaScript A Deep Dive into Dynamic Style Management - Real Time Theme Switching Through JavaScript Event Listeners
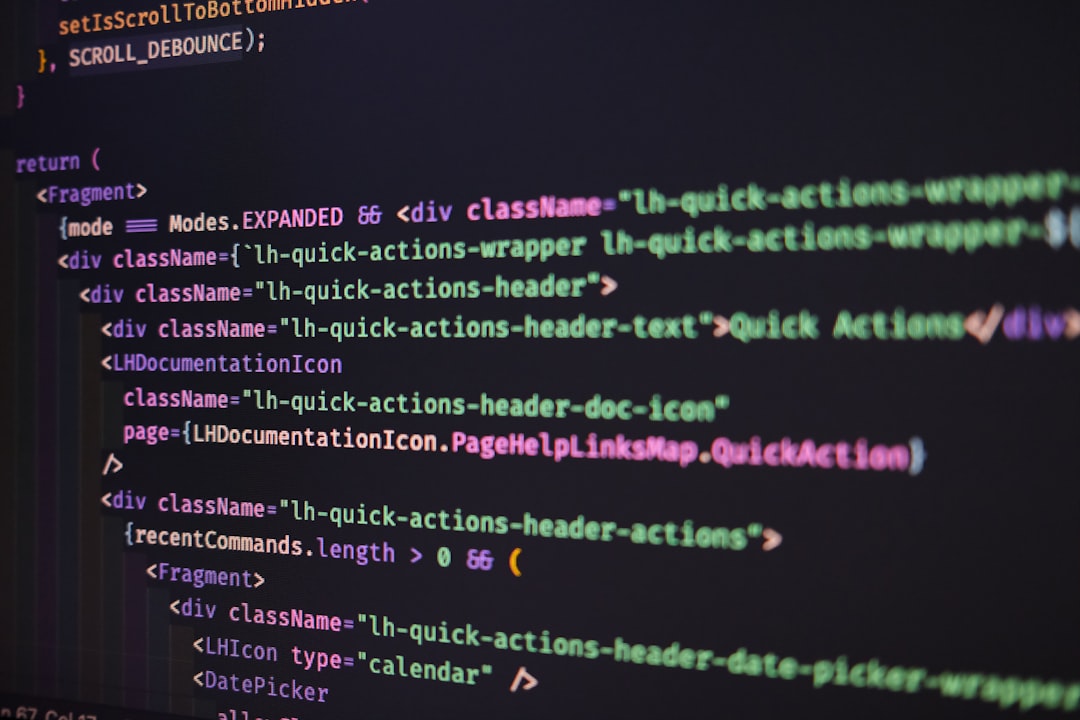
Real-time theme switching using JavaScript event listeners allows for dynamic style changes based on user interactions, providing a more interactive and responsive user experience. The core idea is to utilize CSS custom properties to define themes, making it possible to switch between them without needing separate stylesheets. This avoids duplication and simplifies theme management. By implementing event listeners (like clicks on a theme toggle), your JavaScript code can modify these CSS variables, causing immediate theme shifts. For example, a user could click a button to switch between a light and dark theme.
This approach streamlines theme management and allows for user preferences to be easily stored and applied, offering a more personalized website experience. The ability to update the overall visual appearance of your website simply by adjusting a handful of CSS variables not only simplifies maintenance but also enhances the performance of your site, leading to a snappier feel for your users. Essentially, this method allows you to create more sophisticated and flexible user interfaces with fewer headaches, leading to better user experience and a more efficient development process. However, there's always the risk of making your stylesheet overly complex if not careful. It's easy to accidentally create dependency nightmares if you are not mindful of how your CSS variables relate to each other.
1. Dynamic theme switching through JavaScript event listeners lets us create web experiences that react instantly to user actions, like clicking a button to switch between light and dark modes. This responsiveness adds a level of interactivity that can boost user satisfaction without needing to refresh the entire page, which can interrupt the flow of a user's actions.
2. One of the neat things about using CSS variables with JavaScript is that it can significantly impact how smoothly a webpage responds to style changes. By changing the values of variables instead of constantly adding or removing whole CSS classes, we can minimize the number of times the browser needs to redraw parts of the page. This reduces the strain on the browser, which in turn creates a more fluid and enjoyable user experience.
3. Before CSS variables, getting a similar level of dynamic styling often required complex JavaScript frameworks or other tools that weren't very easy to learn or manage. CSS variables simplify the process, making dynamic styling accessible to a wider range of developers, even if they're not super experienced in writing large amounts of JavaScript.
4. The beauty of JavaScript event listeners is their flexibility. We can hook them up to respond to all sorts of user actions, like mouse movements, button clicks, or even key presses. This allows us to create themes or styles that change based on exactly what the user is doing at any given moment, creating a highly personalized experience.
5. While CSS variables have the handy feature of inheriting values from parent elements, things can get a bit complex when we mix them with JavaScript event listeners. If a listener is changing a lot of different variables, it can be tricky to avoid unintended side-effects or unexpected styling results. Carefully tracking how variable values cascade and are modified is important in these situations.
6. Because CSS variables are dynamic, the changes we make using JavaScript are instantly reflected on the page. This gives users immediate feedback, making it ideal for applications where user preferences need to be visually updated in real-time, like design tools or e-commerce sites where users want to see their product choices reflected right away.
7. CSS variables, in combination with JavaScript, help us build websites that adapt smoothly to different screens and devices. JavaScript can modify variable values within media queries that are triggered when a user switches to a smaller or larger screen, allowing us to make sure that the overall design is consistent across a wide range of devices without needing a ton of repetitive CSS rules.
8. CSS variables let us define default or "fallback" values for our variables. This is really useful for handling situations where JavaScript might fail to set a variable, perhaps due to an error. Having a fallback ensures that at least a basic style will be applied, making our stylesheets more resilient and preventing unexpected design breakages.
9. For complex applications, JavaScript can use real-time data (like the current time or user settings) to adjust CSS variable values on the fly. This enables features like automatically switching to a dark mode or adjusting a website's color scheme based on external data without requiring major overhauls to existing styles.
10. While incredibly useful, it's worth noting that constantly updating many CSS variables through JavaScript could potentially cause performance problems if it's not done carefully. Developers should be mindful of the number of variable updates and aim to optimize the update process to make sure the browsing experience is smooth.
Using CSS Custom Properties with JavaScript A Deep Dive into Dynamic Style Management - Performance Impact Of CSS Variable Updates Versus Traditional Style Changes
When dynamically updating styles, there's a difference in how CSS variables and traditional style changes affect performance. CSS variables offer benefits like making styles more dynamic and easier to maintain. However, especially when you have a parent element with many children, changing a CSS variable on that parent can be slower than changing the same variable on a child element. This suggests that while CSS variables provide a good way to control styles, using them in deeply nested structures can sometimes impact performance negatively. On the other hand, traditional ways of updating styles, like directly changing properties in your CSS, might not introduce the same level of performance overhead. As a result, developers need to make informed decisions about whether the benefits of using CSS variables outweigh any potential performance trade-offs, particularly in designs with a lot of nested components. The decision ultimately depends on the specific needs of the project and the trade-offs between flexibility and speed.
1. When we update CSS variables using JavaScript, browsers seem to be able to optimize how they recalculate styles compared to adding or removing whole CSS classes. This leads to fewer layout changes, which makes for a smoother experience when styles are changing dynamically.
2. CSS variable updates show up immediately in the browser, unlike when we modify styles using class changes where the visual changes might have a slight delay. This instantaneous response is especially important for user experiences where feedback needs to happen quickly.
3. If we need to change a bunch of CSS properties at the same time, updating CSS variables generally performs better than the traditional ways. The browser has less work to do because it's updating fewer things, which reduces how often it has to redraw the screen.
4. While CSS variables are very flexible, they inherit values, so changes made in a parent element can affect its children in ways we might not expect. If you're not careful with how you define and overwrite variables, this can lead to unpredictable styling.
5. We used to have to reload stylesheets to see CSS changes, but with CSS variables, we can make changes "on the fly" with JavaScript. This is much better for users because it creates a more responsive feel, and it's efficient since we don't need a page reload.
6. One neat thing about CSS variables is they make responsive design easier. We can adjust styles based on screen sizes by altering the variables. We don't need to repeat the same CSS rules for different sizes.
7. CSS variables can help us avoid some common problems with CSS specificity when we're using classes to style things. Since we're controlling the styles through variables, there's less risk of styles conflicting with each other.
8. CSS variables are more than just for appearance; they can also affect how quickly animations and transitions happen. This makes it easier to create UI elements that react to data or user interactions in real-time.
9. We can avoid repeating ourselves by updating several CSS properties through a single variable. However, this can create complexities if we aren't careful, especially with large stylesheets. It can be hard to track the relationships between all the variables.
10. CSS variables are built into browsers, so we don't need to pre-process them like we do with some other styling systems. This means faster development, and we reduce the risk of errors, adding to the overall efficiency of using CSS variables.
Using CSS Custom Properties with JavaScript A Deep Dive into Dynamic Style Management - Browser Support And JavaScript Fallback Strategies For CSS Custom Properties
When working with CSS custom properties and JavaScript, it's important to be aware of how different web browsers handle them. While most modern browsers have good support for CSS variables, older browsers, like Internet Explorer, might not support them fully. This means you need to have a backup plan, also known as a fallback strategy, for situations where a browser doesn't understand your CSS variables.
One way to deal with this is to provide default values within your CSS rules themselves. If the browser doesn't understand custom properties, it will simply use the fallback you've set. Additionally, JavaScript can play a part in making sure your variables work properly across different browsers. The `CSS.registerProperty` method gives developers the ability to establish custom properties and assign default values or inheritance behaviors, ensuring consistent behavior for CSS variables.
Another tip to make sure your styles look the same across different browsers is to use vendor prefixes. This basically means adding extra code to your CSS that tells older browsers how to handle your properties. Tools like Autoprefixer can automate this process.
By carefully considering browser compatibility and putting fallback strategies in place, you can create designs that work reliably on a wide range of browsers, keeping your users happy regardless of what browser they choose to use. This ensures a consistent and enjoyable user experience even if a browser doesn't have the latest features. It's about creating flexible and accessible websites, even if the technology isn't perfectly standardized.
1. The push for CSS custom properties, also known as CSS variables, stemmed from a desire to make styling more manageable and dynamic within web development, reducing reliance on JavaScript-heavy solutions. However, this desire to simplify things sometimes introduces complexity in other areas.
2. As of late 2024, most modern browsers have adopted CSS custom properties, boasting a high adoption rate, but some legacy browsers, like older versions of Internet Explorer, still lack full support. This highlights the ongoing challenge of balancing the desire to use new features with the need to ensure that websites remain accessible to everyone. Because of this, developers need to figure out ways to handle older browsers to avoid a bad user experience.
3. A simple approach to deal with older browsers is to include a "fallback" within the CSS itself. This involves using standard CSS rules in addition to custom properties, so if the browser can't handle custom properties, the website won't break. This method allows the website to have a reasonable visual appearance even if a browser doesn't fully support custom properties.
4. When you start nesting CSS custom properties, you can end up with potential performance issues. If you change a property on a parent element, all the related children inherit that change, which can slow down how fast the browser renders a page. It's not usually a massive issue, but it's worth thinking about in large or complex projects where performance is a priority. This brings us back to the idea of always needing to think about performance implications when adding features.
5. When you're using JavaScript to tweak CSS variables, debugging can become a bit tricky due to how browser developer tools often handle caching. The changes you make in JavaScript may not show up instantly in the developer tools which can make troubleshooting hard. This sort of unpredictable behavior can make debugging more difficult than it might be otherwise.
6. CSS custom properties inherit their values, and you can define them locally in certain parts of your website to modify behavior. While this is great for creating modular design, it can become a problem if you're not careful about how you define these custom properties or if you don't document their relationships well. It's another instance where flexibility can introduce complexity.
7. To get CSS custom properties working in older browsers, you may have to utilize "polyfills." These are basically workarounds to make older browsers handle CSS properties they don't normally support. However, introducing a polyfill can slow down a website a bit and may have unexpected consequences that you need to manage. This highlights that sometimes there are trade-offs in implementing new standards across the web.
8. Performance tests have indicated that when you use custom properties instead of messing with CSS class names, browsers need to do less work to update how a page looks. This can lead to a smoother user experience in sites that are highly interactive and have many changes to styles. This type of performance improvement is great, but it also reinforces that when we add new features to our web pages, we have to think about the implications on page performance.
9. If you're concerned about cross-browser compatibility, there are more advanced ways to deal with older browsers than just simple fallbacks. Things like including default values or using JavaScript to check if certain features are available in a browser are ways to achieve more robust cross-browser compatibility. If you're building a site that needs to work on lots of different browsers, taking care of browser compatibility becomes a central part of the development process.
10. CSS custom properties allow you to avoid repeating yourself which can be very useful for organizing your CSS, but excessive use without careful planning or documentation can lead to a tangled mess of dependencies in large projects. This makes it hard to figure out what's going on and can be tough if someone new joins the project. This is a good example of how using features in an organized way can help prevent problems down the line.
More Posts from aitutorialmaker.com: