Create AI-powered tutorials effortlessly: Learn, teach, and share knowledge with our intuitive platform. (Get started for free)
Efficient String Sanitization in Rails Mastering the 'strip' Method
Efficient String Sanitization in Rails Mastering the 'strip' Method - Understanding the basics of string sanitization in Rails
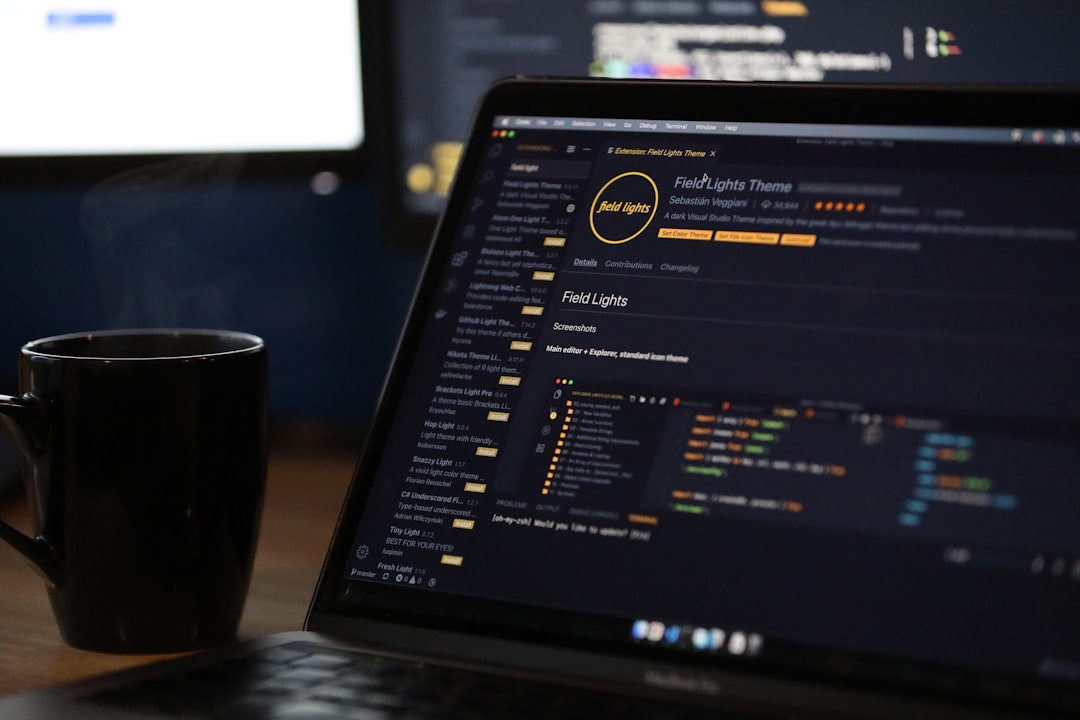
In the realm of Rails development, ensuring the safety of your application is paramount, especially when it comes to user input. This is where string sanitization comes into play, acting as a crucial defense mechanism against malicious content. Rails provides a suite of tools designed to clean user input, including the versatile `sanitize` helper for HTML, the whitespace-trimming `strip` method, and more. While these tools are powerful, it's essential to remember that they are not a substitute for proper input validation. Applying sanitization before thorough validation can create vulnerabilities, potentially jeopardizing your application's security. As Rails evolves and introduces features like the HTML5 parser in version 7.1, keeping abreast of best practices for input handling becomes more vital than ever.
Rails provides a number of built-in methods for sanitizing user input, designed to prevent security vulnerabilities like SQL injection and cross-site scripting (XSS). These methods automatically sanitize parameters when passed as hashes in query methods.
While `strip` is often used for removing leading and trailing whitespace, Rails' sanitization capabilities go beyond simply cleaning up strings. The `sanitize` helper, for example, cleans HTML input by stripping non-whitelisted tags and attributes, as well as blocking unsafe protocols like JavaScript.
However, it's crucial to remember that these sanitization methods are not schema-aware, meaning they don't perform type casting or consider different data types when sanitizing input. Additionally, they don't take into account potential encoding issues, which can complicate the process, especially when dealing with strings containing special characters.
For this reason, it's important to be cautious when using these sanitization methods. Never apply them directly to user input without proper validation. Failing to do so can open your application to security risks.
The `ActionView::Helpers::SanitizeHelper` class provides additional functionality for sanitizing HTML input, ultimately contributing to a safer user experience. It's important to be mindful of the potential impact of sanitization on performance, particularly in systems handling large volumes of data, and to ensure that you're not introducing additional vulnerabilities by relying on these methods too heavily.
Efficient String Sanitization in Rails Mastering the 'strip' Method - Implementing the 'strip' method for input cleaning
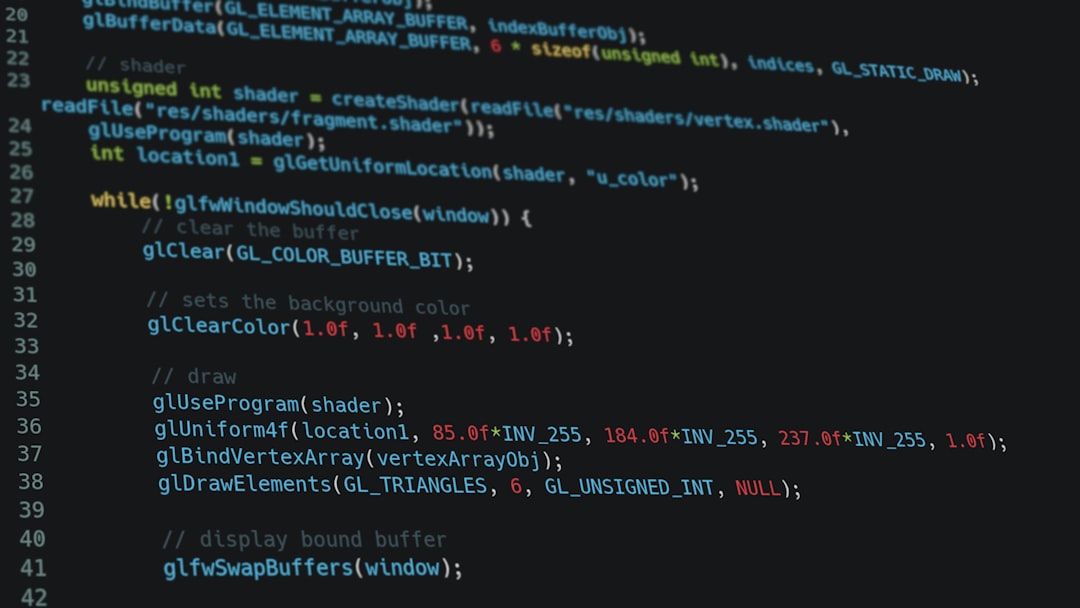
The `strip` method in Rails is a basic but essential tool for cleaning user input. It's designed to remove leading and trailing whitespace, ensuring that input is correctly formatted. However, it's crucial to recognize that `strip` alone is not enough to protect your application. While it helps with basic formatting, it doesn't address more sophisticated threats like SQL injection or cross-site scripting (XSS). A comprehensive security strategy requires more robust validation and sanitization methods beyond just using `strip`. It's a helpful tool, but don't rely on it as your primary defense.
The `strip` method in Ruby isn't just for removing whitespace. You can use it to remove other specified characters from the start and end of a string, adding a layer of flexibility to input cleaning. While `strip` works under UTF-8 encoding, which is great for many web applications, you need to be careful when dealing with different encodings to avoid unexpected errors. Be mindful of performance too, since stripping large strings can be computationally heavy.
Don't get complacent! While `strip` is simple and useful, don't solely rely on it for sanitization. More thorough validation processes are crucial for mitigating risks like SQL injection and XSS. It's important to remember that `strip` doesn't modify the original string. It returns a new one, which is a great reminder of the immutability concept in Ruby, preventing unintended consequences when manipulating strings.
When using `strip`, consider its potential impact on other methods that rely on trailing whitespace, like database operations. It's important to understand the broader context of data handling when applying this method. While `strip` handles leading and trailing characters, it's crucial to remember that it won't touch whitespace within the string.
You'll commonly find `strip` employed in form processing for user input sanitization. It's very useful for catching those accidental spaces, but it should be a part of a broader sanitization strategy that includes validation. Be extra cautious when using `strip` with strings from different languages because its behavior can be inconsistent with character sets beyond basic ASCII. Lastly, always remember the potential for subtle debugging nightmares that can arise when overlooking the influence of `strip` on string comparisons. Leading and trailing whitespace can change logic operation outcomes.
Efficient String Sanitization in Rails Mastering the 'strip' Method - Exploring additional sanitization techniques in Rails 1
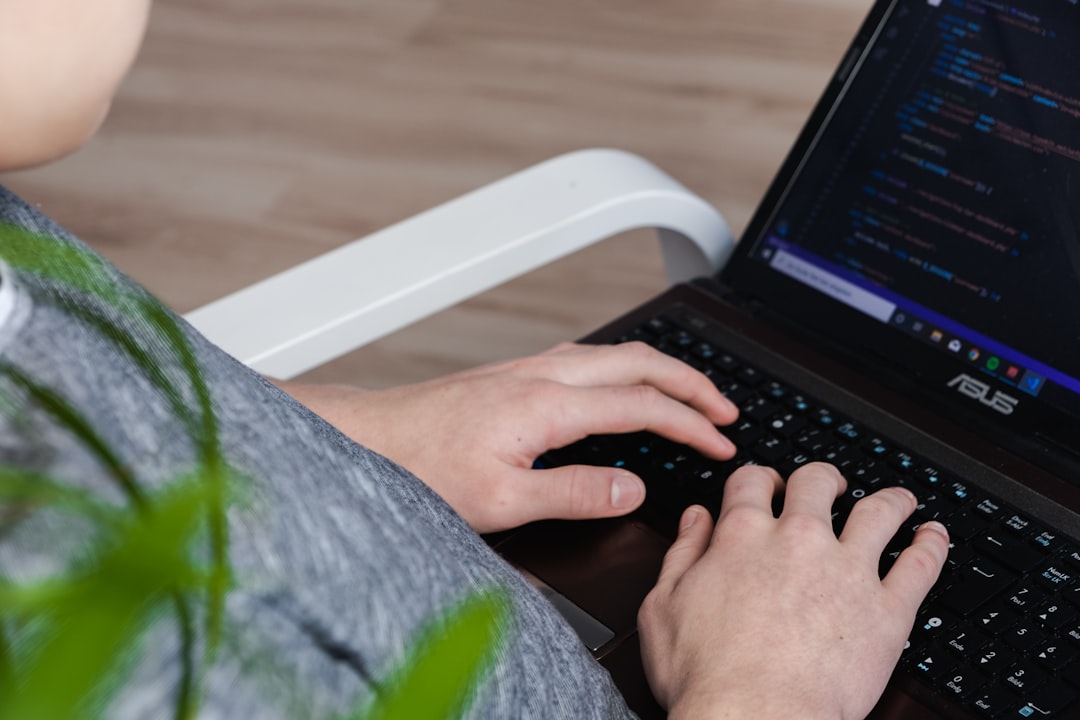
In Rails, the world of string sanitization goes beyond the basic `strip` method. We have tools like the `sanitize` method, which can filter HTML input and allow only specific tags, as well as blacklisting techniques that prevent harmful characters from making it into our code. Methods like `sanitize_sql` offer the possibility of safely integrating user input into SQL queries, but it's important to be aware that they aren't schema-aware. Since Rails 7.1 introduced a more sophisticated HTML5 parser, understanding and correctly using these sanitization methods is crucial to maintaining a secure application. While a multifaceted approach to sanitization is great, relying solely on one method can leave your application open to vulnerabilities. In short, we have the tools, but we need to be smart about how we use them to make sure our Rails applications are safe.
Rails 1, while a pioneering framework, took a more hands-off approach to sanitization, relying on developers to manually validate user input. This reliance can leave applications vulnerable to attacks that modern frameworks like Rails 7.1 mitigate with more robust measures. While the `strip` method, designed for removing leading and trailing whitespace, is a helpful tool, its effectiveness is limited when dealing with varied character encodings. Unicode characters, in particular, can cause inconsistencies. Additionally, `strip`'s performance impact can be overlooked, especially when dealing with large strings or when used repeatedly in loops. The introduction of the `sanitize` helper in later Rails versions signaled a shift towards a more comprehensive approach to sanitization, but it also highlighted the need for careful validation. `strip` has applications beyond basic input cleaning, such as preprocessing CSV files, but its use should be part of a broader strategy that considers the context of the data. While simple, `strip` and other sanitization methods rely on Ruby’s immutability principle, ensuring that data integrity is preserved. Rails 1 also focused primarily on sanitizing text inputs, neglecting the complexities of file uploads and binary data, which can present security vulnerabilities if not handled appropriately. A key takeaway from Rails 1's sanitization approach is the crucial need for developer awareness. Without a comprehensive understanding of the limitations and capabilities of methods like `strip`, developers risk introducing critical security flaws into their applications.
Efficient String Sanitization in Rails Mastering the 'strip' Method - Leveraging ActionViewHelpersSanitizeHelper for HTML content
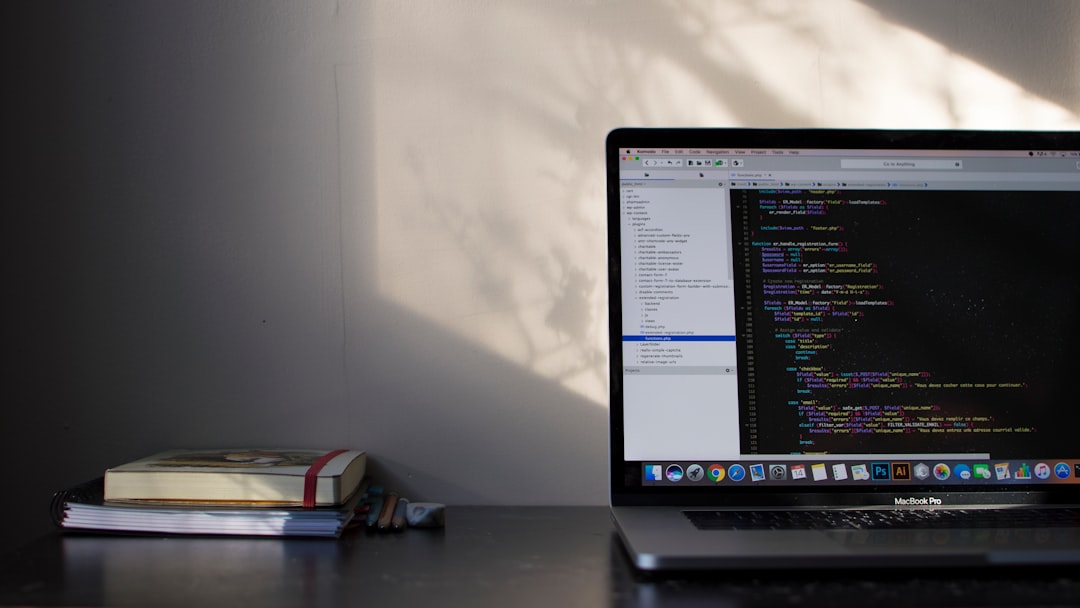
Rails applications often deal with user-generated HTML content, which can pose security risks if not properly sanitized. The `ActionView::Helpers::SanitizeHelper` module provides the tools for safely handling this type of data. It's a vital component for protecting your application against potential vulnerabilities like cross-site scripting (XSS). Rails 7.1 introduced the HTML5 parser as the default sanitizer, but developers have the flexibility to use the `HTML::WhiteListSanitizer` for more control over sanitization rules. For simple HTML tag removal, the `strip_tags` method is a valuable tool, removing unwanted tags without sacrificing security. However, it's important to remember that sanitizing user-provided HTML doesn't guarantee well-formed markup. The sanitization process itself won't always catch all potential vulnerabilities, so developers must carefully consider the limitations of these methods and ensure they are implemented correctly to prevent security risks.
The `ActionView::Helpers::SanitizeHelper` class provides a set of methods for cleaning up HTML content, ensuring that only allowed tags and attributes are present, and that malicious scripts are not injected. While this is a welcome tool for developers, it is not a magical solution to security vulnerabilities. It's a potent weapon but a limited one.
This tool can be tailored to meet specific application needs by allowing the developer to define their own whitelist of tags and attributes, further enhancing the sanitization process and tightening the grip on those pesky XSS vulnerabilities. However, don't get complacent. This tool, while powerful, comes with its own set of considerations.
Performance is a constant concern, especially when dealing with high volumes of data, and overusing `SanitizeHelper` can lead to slowdowns. It's crucial to use it judiciously, striking a delicate balance between security and performance. With the arrival of the HTML5 parser in Rails 7.1, `sanitize` has become even more potent, handling a broader spectrum of HTML input. This upgrade, however, necessitates a careful understanding of its capabilities, as the evolution of web standards often requires the developer to adapt.
Different character encodings, as always, present unique challenges. These encoding differences can affect sanitization, leading to unpredictable behavior when dealing with strings containing multibyte characters or special characters. This is a reminder of the crucial need to carefully manage data types and encodings.
It's critical to remember that even with powerful sanitization tools like `SanitizeHelper`, relying solely on them is a dangerous game. Implementing robust input validation practices alongside sanitization methods creates a truly impenetrable barrier against vulnerabilities. While sanitizing user input often results in the generation of a new string to maintain immutability, a key principle in Ruby, developers need to understand how these methods manipulate strings, both in terms of memory and state, to avoid potential issues.
As always, relying on frameworks alone can be risky. Developers must be actively engaged in the process, implementing their own validation measures alongside the frameworks. Neglecting this responsibility can lead to unsanitized data sneaking into an application, opening it up to vulnerability.
The shift from Rails 1, with its hands-off approach to sanitization, highlights the progress in modern frameworks. Rails 7.1's arsenal of tools has been significantly strengthened, but the responsibility still falls on developers to understand and utilize them effectively.
It's important to consider performance overhead when applying `sanitize` repeatedly in loops or on large datasets. Developers must be mindful of scenarios where sanitization can be minimized without compromising security, optimizing for efficiency. And finally, modern applications are complex, processing not only text but also diverse input types such as file uploads and AJAX requests. Sanitizing this complex data is critical for maintaining security, as file content can carry different security risks compared to traditional HTML input.
Efficient String Sanitization in Rails Mastering the 'strip' Method - Utilizing Rails HTML Sanitizers gem for enhanced security
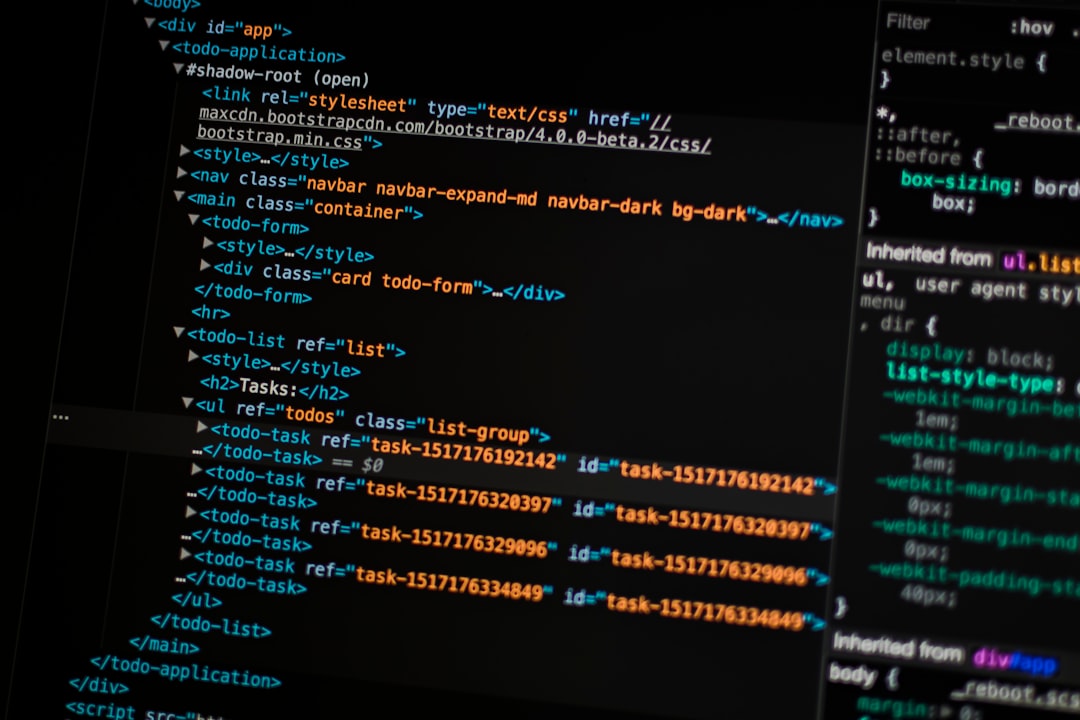
The Rails HTML Sanitizers gem is a valuable tool for enhancing application security in the Rails framework. It's been a part of Rails since version 4.2 and provides developers with a powerful set of methods, like `sanitize`, to clean HTML content. This gem is especially important because it helps prevent XSS attacks, a common web vulnerability. While the integration of the HTML5 parser in Rails 7.1 offers improved HTML handling, remember that sanitization shouldn't be your only defense.
You need to validate user input thoroughly before applying any sanitization methods to truly protect your application. There is a delicate balance to maintain between security and performance when using sanitizers. Overuse can slow down your app, but failing to use them effectively leaves your application vulnerable.
The Rails HTML Sanitizers gem provides a robust way to sanitize HTML content in Rails applications, offering more than just preventing XSS attacks. It can be valuable for cleaning input from APIs and other integrations, making it relevant for both front-end and back-end development. Unlike some tools that rely on blacklisting, this gem primarily uses whitelisting, ensuring that only explicitly allowed tags and attributes are permitted. However, using the gem with large datasets or heavily nested HTML might introduce performance challenges, especially if employed frequently in rendering loops or with extensive user input. Moreover, its sensitivity to character encodings, particularly with multibyte characters, requires vigilance from developers.
The `ActionView::Helpers::SanitizeHelper` offers a level of flexibility by allowing developers to implement context-specific sanitization, enabling unique rules based on the nature of content in different parts of the application. With the introduction of Rails 7.1, the gem now uses an HTML5 parser by default, which brings improved compliance with modern web standards and handling for newer tags and attributes, indicating a proactive approach to keeping up with changing web security needs.
It's important to note that the gem, in line with Ruby's immutability principle, returns new sanitized strings instead of altering the original input, safeguarding data integrity and preventing potential issues from in-place mutations. Some developers even implement real-time sanitization feedback to enhance the user experience, providing visual cues as users type.
To further enhance sanitization, the HTML Sanitizers gem can be used alongside other Gems like `gem 'loofah'`, which can augment its capabilities with advanced parsing and cleaning methodologies. Since security threats constantly evolve, developers must continually update their knowledge and practices to ensure their applications remain secure.
Efficient String Sanitization in Rails Mastering the 'strip' Method - Combining 'strip' with other methods for comprehensive data cleansing
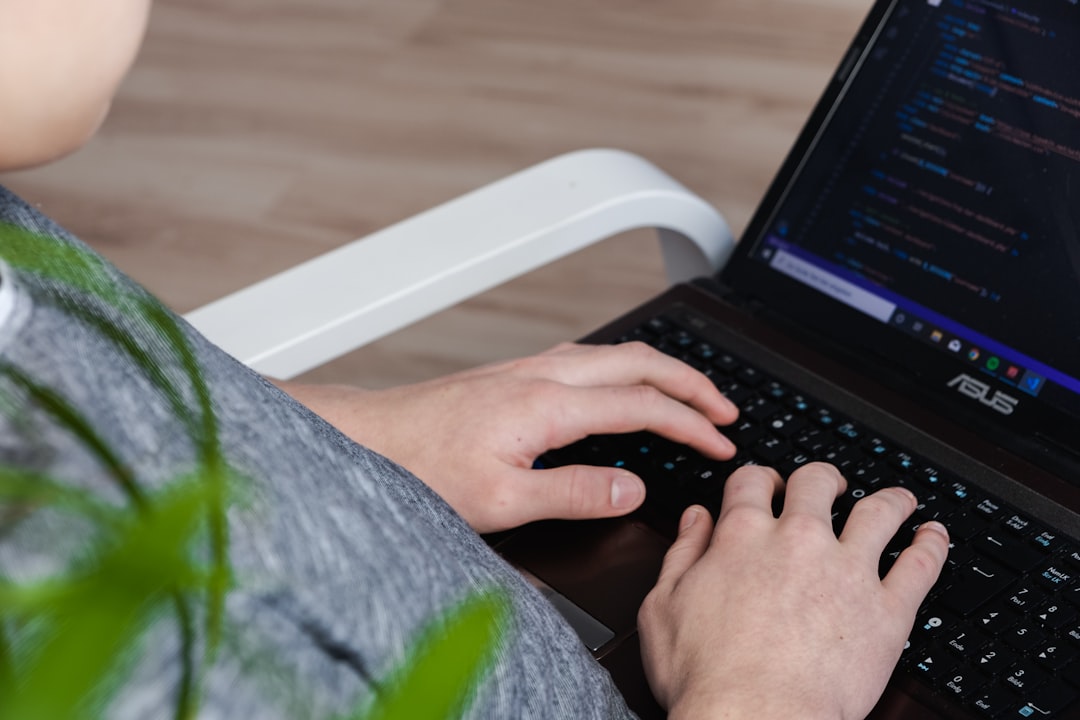
Combining the `strip` method with other techniques for cleaning strings is crucial for creating secure and reliable Rails applications. While `strip` effectively removes unwanted whitespace, it's only a starting point. You need to address other potential vulnerabilities, like SQL injection and cross-site scripting (XSS), by using a more comprehensive strategy. This involves methods like pattern matching and character filtering, which provide stronger protection. A multi-faceted approach not only guards against malicious content but also preserves the usefulness of the data, allowing applications to function correctly. It's crucial to remember the limitations of every method to create resilient and secure applications that can protect sensitive information.
The `strip` method in Rails is a valuable starting point for data sanitization, but it's crucial to think beyond just removing whitespace. Combining `strip` with other techniques creates a comprehensive defense against malicious inputs. For example, using `strip` along with input validation methods ensures proper data types are sanitized, mitigating potential coercion vulnerabilities. Regular expressions can be used alongside `strip` for removing more complex characters or patterns, enabling more advanced cleaning for user-generated content.
While `strip` is simple, its performance shouldn't be overlooked. In large string scenarios or when applied frequently, its computational overhead can accumulate, highlighting the importance of profiling and optimization. Encoding differences can cause unexpected behavior, especially when working with strings containing multibyte characters or special characters, demanding careful attention to data type and encoding management.
The immutable nature of Ruby influences how `strip` works, returning a new string instead of changing the original. This maintains data integrity but requires managing the output effectively to avoid confusion when the original input is still referenced. Pairing `strip` with Rails model validations creates a more robust defense, ensuring both front-end and back-end validations are in place. Tailoring sanitization rules based on user input context, like using `strip` for forms but stricter checks for APIs, demonstrates the importance of adaptability.
Using middleware that combines `strip` with other sanitization methods can further minimize risks, consistently applying sanitization across all incoming requests. Tracking a sanitized input audit trail can be invaluable for understanding how user data evolves over time, helping to pinpoint potential security vulnerabilities and troubleshoot issues related to `strip` and other methods.
Create AI-powered tutorials effortlessly: Learn, teach, and share knowledge with our intuitive platform. (Get started for free)
More Posts from aitutorialmaker.com: