Create AI-powered tutorials effortlessly: Learn, teach, and share knowledge with our intuitive platform. (Get started for free)
Implementing Jetpack Compose in Android Studio A Step-by-Step Guide for Beginners
Implementing Jetpack Compose in Android Studio A Step-by-Step Guide for Beginners - Setting up Android Studio for Jetpack Compose
To get started with Jetpack Compose in Android Studio, make sure you're using the latest version. When starting a fresh project, opt for the "Empty Activity" template and tick the box labeled "Use Jetpack Compose" during the project setup. Jetpack Compose is a new way to build Android UIs using Kotlin code instead of the usual XML files. This shift to declarative programming allows for a more streamlined approach to designing interfaces, making the process more efficient and often less complex.
Enabling specific features within Android Studio will unlock newer Compose features, ensuring access to updated tools. Numerous resources exist to learn more, from readily accessible tutorials and step-by-step guides to official examples. These resources are useful for grasping core concepts of Jetpack Compose and learning how to construct different UI elements like Text, TextField, and layout containers such as Column and Row.
To get started with Jetpack Compose within Android Studio, you'll need to ensure you have the most recent version of the IDE installed. When initiating a new project, select the "Empty Activity" template, making sure to check the "Use Jetpack Compose" option during the project's initial configuration.
Jetpack Compose employs a modern approach for building user interfaces. It leverages Kotlin functions called "composables" to build UIs in a declarative fashion. It's a welcome shift away from XML-based layouts, which can sometimes be cumbersome. This change fundamentally alters the development process, allowing developers to express the desired UI structure within Kotlin code.
One intriguing aspect is the built-in preview function inside Android Studio. It offers a real-time view of your UI as you write code. This feature significantly accelerates the development process, particularly when contrasted with the old trial-and-error nature of XML layouts. It's no secret that the setup for Compose involves some configuration changes within the Android Studio environment. You'll often find yourself toggling preview versions and related options to get things working.
While the fundamentals can be readily grasped, mastery of Jetpack Compose calls for dedicated study. Fortunately, ample resources are available online, including the official documentation and numerous tutorials. These learning tools are invaluable for navigating the transition to Compose. These guides can help demystify UI components such as Text, TextField, Column, and Row.
The official Android developers offer a wealth of Jetpack Compose samples on GitHub. The repository showcases the implementation of the recommended Android architecture principles along with the Jetpack libraries within realistic applications. It's a great source of inspiration to see these patterns used in practice and a useful resource to examine best practices. Exploring those examples may help you avoid common pitfalls when starting out.
Implementing Jetpack Compose in Android Studio A Step-by-Step Guide for Beginners - Creating a new Compose project
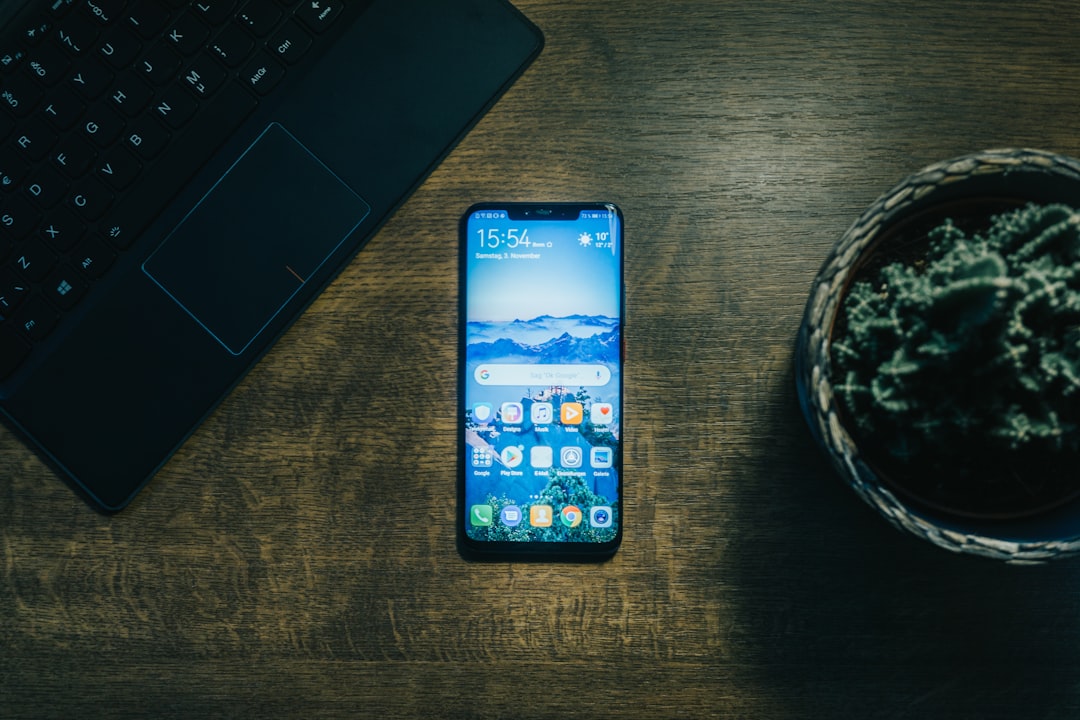
To begin building a new Jetpack Compose application, start with the latest version of Android Studio installed. Creating a new project is as simple as selecting "New Project" from the menu and choosing the "Empty Compose Activity" template. This template specifically targets Jetpack Compose projects and ensures a streamlined setup. When configuring your project, give it a name and define the minimum SDK version. You'll find that Android Studio automatically creates a few basic Compose UI components, which serves as a springboard for further development. It's beneficial to get acquainted with the project structure and Gradle files, as they are vital in correctly configuring the environment for Jetpack Compose and leveraging its features. These initial steps establish a foundation for understanding how Compose uses Kotlin to create UIs declaratively. Understanding the initial project structure and configurations provides a solid footing for the new way Compose designs user interfaces compared to more traditional approaches.
To embark on a new Jetpack Compose project within Android Studio, begin by ensuring you have the most recent version of the IDE installed, as it includes intelligent features that can be invaluable as you develop your application. From the Android Studio menu, initiate a new project and, from the template selection, choose "Empty Compose Activity". This serves as the starting point for your Compose journey.
As part of the project setup, furnish your project with a descriptive name, such as "ComposeTutorial," and specify the minimum SDK version for your application. Opting for a version of 1.0 or later will ensure compatibility and help prevent issues later.
Once the project is created, Android Studio will generate a foundation of Compose UI components. This scaffolding serves as a springboard for your app's features as you meticulously craft it, step-by-step, according to your needs. It's a good idea to familiarise yourself with the project's structure and the Gradle configuration files. Making sure these elements are properly configured to support Jetpack Compose version 1.2 or later is crucial, as it can affect functionality.
One of Compose's more alluring features is the ability to preview and animate the UI in real-time within the Android Studio editor. This feature enables you to quickly observe your creations and helps in identifying UI issues promptly. If you are exploring Compose, you may find the available Compose sample apps to be a valuable learning resource. Alternately, if you're incorporating Compose into an existing project, you can configure Compose through the project's settings.
When crafting your UI, Compose relies on the concept of composable functions. These Kotlin functions are the building blocks of a Compose application and offer a path to creating reusable UI elements that streamline the development process. To streamline your workflow and identify issues, Android Studio comes equipped with a layout inspector and a number of other useful tools. These features can help visualize your UI and debug issues.
While the initial steps might seem easy, becoming proficient in Jetpack Compose calls for a dedicated effort. Luckily, there's an abundance of tutorials and community resources online. Engaging with these materials deepens your understanding of Compose and hones your development abilities. The combination of the practical guidance and the extensive documentation offers a good path for improvement.
Implementing Jetpack Compose in Android Studio A Step-by-Step Guide for Beginners - Understanding Composable functions
Composable functions are the core components in Jetpack Compose, essentially Kotlin functions marked with the `@Composable` annotation. They serve as the building blocks for creating UI elements, allowing for a more streamlined and declarative approach to user interface design, unlike traditional XML layouts. Composable functions empower developers to construct reusable UI parts, promoting efficient and cleaner code. Moreover, they incorporate a reactive model, simplifying the task of keeping the UI updated with changing data. While the initial understanding of composables might be easy to grasp, becoming adept at them necessitates a deeper exploration and regular practice within the world of Jetpack Compose. Understanding how these functions work is foundational to building rich, dynamic UIs with Compose.
Jetpack Compose's core building blocks are composable functions, which are Kotlin functions marked with the `@Composable` annotation. These functions enable the creation of UI elements in a declarative manner, a departure from the traditional XML-based approach. This declarative style makes the UI code often easier to read and maintain compared to traditional views.
Composable functions can be designed to be stateless, relying only on their input parameters to define the UI. This stateless nature fosters reusability and predictability. Interestingly, they are not tied to the Android lifecycle in the same way as traditional views. This means they can be invoked at any point within the application, which simplifies how you interact with them from different parts of your codebase.
Jetpack Compose leverages a reactive programming model, with the runtime intelligently deciding when UI updates are needed. This strategy is called recomposition, and it ensures only the necessary parts of the UI are redrawn, enhancing performance. Recomposition efficiency becomes clearer when you consider the more traditional approach of redrawing the entire screen whenever a minor change is made.
Composable functions benefit from a feature called Composition Local Providers. These providers let you implicitly share information across the UI tree without needing to pass parameters explicitly to each composable. This mechanism reduces parameter clutter and helps simplify UI building for certain use cases.
Modifiers are a foundational part of how composable functions are customized. They act like a decorator or a set of instructions that change the appearance or behavior of a composable without altering its underlying logic. This approach encourages the separation of concerns, making UI customization simpler and less error-prone.
Compose comes with a powerful design-time preview function, allowing you to see UI changes as you edit code. This real-time preview functionality shortens the development feedback loop, as you can observe the results of your changes instantly instead of compiling and running the app. This difference, when contrasted with the older methods, can be a significant improvement in developer productivity.
Compose emphasizes declarative programming, where you describe *what* the UI should be rather than defining the steps for how it is built. This declarative style encourages a cleaner separation of code concerns.
Compose works seamlessly with Kotlin coroutines, a language feature that enables asynchronous programming. This enables you to smoothly integrate network requests, database interactions, and other operations that may take time without hindering the UI's responsiveness. This integration adds flexibility to the UI building process and can lead to a more responsive user experience.
Jetpack Compose has built-in support for accessibility features, ensuring that UIs can be utilized by a broader range of users. This support is usually not an afterthought, but it is frequently a core part of building the user interface. Accessibility often becomes a standard feature rather than an extra element that needs to be added later in the development cycle.
Compose is built to support gradual adoption in projects with existing XML layouts. This means you can incorporate Compose composables into existing XML-based UIs and vice-versa. This feature makes the shift to Compose a more manageable process, especially for projects with legacy code or a mix of technologies.
This exploration of composable functions highlights how Jetpack Compose streamlines UI development for Android applications. While the fundamentals of Compose are fairly easy to learn, mastering its capabilities and best practices necessitates focused study and consistent practice.
Implementing Jetpack Compose in Android Studio A Step-by-Step Guide for Beginners - Building your first UI elements
The journey of building your first UI elements with Jetpack Compose is a great introduction to a more modern way of developing Android apps. Unlike traditional XML layouts, Compose utilizes Kotlin functions, specifically composable functions, to build user interfaces. These functions, marked with `@Composable`, provide a more straightforward and efficient approach to UI design. As you venture into designing with Compose, you'll encounter components like `Text` for displaying text, `TextField` for user input, and layout containers like `Row` and `Column` for structuring your screen. You'll discover that composable functions naturally lend themselves to reusable and modular designs. This declarative approach simplifies building UIs and promotes the development of dynamic and responsive interfaces. Mastering concepts like managing state and understanding how to use modifiers will allow you to build interfaces that adhere to Material Design's principles. While some might find the initial learning curve a bit steep, embracing the concept of composable functions will lead to increased clarity and efficiency in crafting your UIs.
Jetpack Compose introduces a novel approach to constructing Android UIs, emphasizing a declarative style rather than the conventional imperative approach of XML layouts. This shift often results in more readable and maintainable code. Android Studio's real-time preview feature integrated within Jetpack Compose proves quite handy as you build UIs. This instantaneous feedback loop during development is a considerable improvement over the conventional recompilation cycles often associated with XML layouts, bolstering productivity.
Jetpack Compose embraces a reactive programming model, where the UI is automatically updated only when necessary. This mechanism, called recomposition, can dramatically enhance performance by avoiding unnecessary screen redraws, which is a notable upgrade compared to traditional approaches that often redraw the entire UI for even minor changes.
Composable functions within Jetpack Compose, often written in a stateless manner, rely primarily on their input parameters to define the UI. This approach fosters reusability and aids in predicting behavior. Interestingly, these functions aren't tightly coupled to Android's lifecycle, unlike traditional views. Consequently, their invocation can be more flexible, which leads to simpler code interactions across different parts of your app.
Compose offers a unique feature called Composition Local Providers. These providers simplify sharing data across the UI hierarchy, eliminating the need to pass it down explicitly through multiple composable layers. This can significantly clean up the UI code and promote structural clarity. Additionally, Jetpack Compose enables a gradual integration into existing applications that still rely on XML layouts. Developers are free to introduce Compose composables into existing codebases without a complete rewrite, making the transition to the new framework less abrupt.
Modifying UI elements within Compose involves a concept called modifiers. These modifiers function as decorators, allowing modifications to UI aspects without altering the underlying composable logic. This modular design fosters cleaner code and simplifies UI customization. Jetpack Compose also integrates accessibility features directly into UI components, making inclusive design a priority from the outset. This contrasts with conventional approaches where accessibility features are sometimes treated as afterthoughts.
The seamless integration of Kotlin coroutines within Compose enables smooth asynchronous programming, avoiding blockage of the main UI thread during resource-intensive operations such as network requests. This feature can dramatically improve the responsiveness of your applications, contributing to a smoother user experience. Jetpack Compose, with its emphasis on composable functions, has the potential to streamline UI logic. The relative independence from Android lifecycles allows for more freedom in triggering UI components, which can result in a cleaner and more modular code structure.
While the fundamentals of Jetpack Compose are relatively straightforward, becoming adept at leveraging its full capabilities and best practices necessitates sustained learning and practice.
Implementing Jetpack Compose in Android Studio A Step-by-Step Guide for Beginners - Managing state in Compose
Jetpack Compose's ability to manage state is crucial for building interactive and responsive Android apps. The first step is to identify which pieces of information need to be tracked and how they'll be used by different parts of the UI. A key idea in Compose is called "state hoisting," where you move the responsibility for managing a state variable up to a common parent composable for all the elements that need to use it. This helps keep things organized.
Compose's design makes it so that your UI will automatically update when the underlying data changes. This makes it easier to deal with changes in your app and avoids many of the complexities found in traditional Android UI development. Understanding how to split larger states into smaller, manageable chunks and leveraging things like ViewModels makes building more complicated UIs less error-prone. Overall, efficiently handling state becomes a core skill when learning Compose and helps build modern, snappy UIs, whether you're working on a small app or something larger and more intricate.
Jetpack Compose provides a fresh perspective on state management in Android applications. It centers around the relationship between the data, or state, and the UI elements (composables) that display that data. A key aspect is understanding the various state-related components like `State`, `MutableState`, and functions like `remember`. This understanding helps pave the way for the reactive programming approach that Compose uses. The way Compose handles updates is unlike the traditional model, as it automatically triggers UI updates whenever the underlying state changes, making the UI responsive to the data changes.
One of the notable differences from the older Android development paradigm is the way Compose separates state management from the UI. Traditionally, managing UI state and UI logic were intertwined. However, in Compose, it is preferable to keep these concerns apart. This separation allows for more organized and modular architectures, like MVVM, where the state of the UI is managed independently of the UI code itself. This decoupling results in more manageable and testable code.
Composable UI elements benefit from having a single, clear source for their associated data, encouraging developers to store this state in a central place—often in a ViewModel. This approach promotes consistency in the way data is presented across the UI and also helps eliminate common problems like displaying stale or incorrect data, leading to an app with more predictable behavior.
Compose provides a specialized list called `SnapshotStateList` that's designed to react to changes in data. This list is quite powerful as it efficiently handles UI updates by informing Compose whenever an element within the list has been modified. This dynamic behavior means you do not need to explicitly tell Compose about changes.
The way Compose updates the UI when state changes is quite thoughtful, with the framework efficiently determining which UI elements need updating. This smart update strategy, known as recomposition, results in a major performance enhancement compared to other approaches where the entire UI is often redrawn with even small changes.
It's not just about performance, either; the integration of state management with `MaterialTheme` allows for a flexible way to create adaptive themes that react to changes in the application's state. This results in consistent visual cues that are directly related to what is happening in the app, improving the overall user experience.
A common design pattern with Compose is the concept of state hoisting. It means the management of state moves up to parent composables, leading to a more adaptable and flexible architecture. This higher-level control of the flow of data in the UI allows parents to manage data shared between several composables.
One neat thing about Compose is its compatibility with existing Android Architecture Components like `LiveData` and `Flow`. This feature gives you the flexibility to continue to utilize reactive data sources in your UI as you build with Compose. This helps with a smoother transition to Compose for developers already working with traditional frameworks.
Interestingly, Compose greatly simplifies UI testing by making state manipulation within tests easier. Developers can quickly set up different UI states, allowing them to test components under various conditions. This ability can be particularly useful for components and interactions that were historically difficult to test in legacy approaches, enabling more robust testing within your applications.
It's notable that state management in Compose is tightly connected with theming. Developers can create themes that automatically adjust based on state changes. This capability facilitates smooth UI transitions and provides a responsive visual feedback mechanism for user actions or system events, enhancing the overall user experience.
The way Compose handles state helps streamline UI development and creates a more consistent and predictable app. While the fundamental concepts are fairly easy to learn, reaching mastery often involves considerable practice. However, once the key concepts are understood, it becomes easier to build more dynamic and responsive user interfaces.
Implementing Jetpack Compose in Android Studio A Step-by-Step Guide for Beginners - Integrating Compose with existing Android apps
Integrating Jetpack Compose into your existing Android apps is designed to be a smooth process, minimizing the need for a complete overhaul. You can introduce Compose gradually by updating your project's Gradle files to include the necessary Compose libraries. This approach lets you transition at your own pace, keeping your existing View-based elements running while you introduce Compose functionality.
A commonly recommended approach is to migrate incrementally. This allows both Compose and your traditional views to coexist within your application during the transition period, minimizing any abrupt changes to your user experience. AndroidViewBinding can serve as a useful bridge, enabling seamless integration between XML layouts and Compose components.
Rather than trying to migrate the entire app at once, it is often beneficial to start small. Begin by replacing small sections, such as individual features or screens, with Jetpack Compose implementations. This allows you and your team to gain experience with Compose gradually and gain confidence with the new development paradigm before undertaking more extensive changes. This measured approach can help manage the transition to a new UI framework.
Jetpack Compose offers a flexible integration path with existing Android applications, allowing you to gradually introduce composable functions into your existing XML-based layouts or vice versa. This incremental approach is valuable for projects with a large legacy codebase, allowing for a smoother and less disruptive migration process. One of the more compelling aspects of Compose is its live preview feature within Android Studio. This real-time preview offers a significantly improved feedback loop compared to the traditional recompilation cycle required with XML layouts, leading to more efficient development iterations.
The way Compose handles state, through concepts like "state hoisting," diverges from older UI practices where state management and UI logic were intertwined. This shift results in cleaner and more testable code structures, which is an intriguing aspect to investigate. Jetpack Compose's rendering process, known as "recomposition," cleverly focuses on updating only the portions of the UI affected by data changes, contributing to noticeably improved performance compared to the older methods that often entailed full UI redraws with each minor change.
Composable functions interact with lists through the `SnapshotStateList`, which automatically updates the UI in response to list modifications. This approach simplifies the handling of dynamic lists, common in many app designs. Compose incorporates accessibility considerations from the start, integrating them into its core building blocks, which provides a compelling foundation for inclusive app design. Compose's composition model allows developers to pinpoint performance bottlenecks in the UI by pinpointing specific expensive parts of the UI. This ability is particularly useful for optimizing high-use components and screens, ultimately improving the app's overall performance.
Jetpack Compose's core Kotlin integration allows the use of features like coroutines to manage asynchronous operations. This integration helps keep the UI responsive, a key aspect when dealing with network requests and other potentially time-consuming processes. Themes can dynamically adapt to changes in the app's state, which elevates user experience by providing responsive visual cues to the user. Testing UI components in Compose is often easier than with older approaches, as you can directly manipulate the state within tests to create various UI scenarios, allowing for more extensive testing that ensures the correctness of various UI components under different conditions.
While the fundamental aspects of Compose are not overly complex, achieving mastery involves dedicated time spent practicing and exploring. But once grasped, you'll find yourself designing UIs in a far more streamlined and efficient manner. It's a testament to how even seemingly simple improvements in workflow can dramatically reshape the way we develop applications, and the more you investigate, the more you realize how powerful Compose can be.
Create AI-powered tutorials effortlessly: Learn, teach, and share knowledge with our intuitive platform. (Get started for free)
More Posts from aitutorialmaker.com: