Create AI-powered tutorials effortlessly: Learn, teach, and share knowledge with our intuitive platform. (Get started for free)
Understanding JavaScript Promise Rejection Events A Deep Dive into Error Handling Patterns
Understanding JavaScript Promise Rejection Events A Deep Dive into Error Handling Patterns - Handling Rejected Promises with the Reject Function and Error Objects
When dealing with asynchronous operations, it's crucial to gracefully manage situations where things don't go as planned. This is where rejected promises and error handling come into play. The `Promise.reject` function offers a clear and concise way to signify a failed operation. You have options – you can either explicitly throw errors, create new rejected promises directly, or directly invoke `Promise.reject` with a specific reason for the failure.
Failing to manage rejected promises can lead to warnings and potential crashes within your JavaScript execution environment. This is why it's important to have a plan. The `catch` mechanism allows you to intercept rejected promises in a chain, providing a fallback to handle unexpected issues. If you're utilizing asynchronous functions, the familiar `try...catch` blocks provide a helpful parallel to synchronous error handling. Developing robust error handling requires a good understanding of how promises are rejected and the tools available to address them. It's a fundamental element of creating stable, reliable applications in the asynchronous world of JavaScript.
Let's delve deeper into how we can manage rejected promises. The `reject` function, available within a Promise's constructor, is our primary tool for signaling that an asynchronous operation has encountered an issue. We can pass various data types as the rejection reason, including dedicated error objects, simple strings, or even other Promises, which offers flexibility in our error handling strategies.
Think of a rejected Promise as a signal that the operation went awry, triggering a shift to the 'rejected' state. This change in state is significant as it reroutes the control flow within the Promise chain. Instead of executing subsequent `.then()` blocks, the execution jumps directly to the nearest `.catch()` block, where we can implement specific actions for handling the error.
Now, why bother with custom error objects? Well, they enable a more detailed representation of the error scenario. JavaScript allows us to extend the fundamental `Error` class, enabling the creation of tailored error types for our application's specific needs. For example, if we have a networking error or a data validation error, distinct custom error types can help differentiate these scenarios. This granular information aids in the creation of better and more effective error handling approaches.
However, this isn't just about error detection; we also need to handle errors in a way that is coherent with JavaScript's asynchronous paradigm. Remember, unlike the immediate exceptions you see in synchronous code, rejected Promises offer a more controlled manner to address failures. This asynchronous nature allows for better error reporting and restoration, especially vital when dealing with complex applications and distributed systems.
We need to acknowledge that handling errors in JavaScript involves more than simply catching them. Unforeseen rejection events can propagate through the code, and if left unattended can result in warnings or unexpected behavior in JavaScript runtime environments. Therefore, it's crucial to meticulously handle rejections to prevent such consequences. We need to be mindful of these issues to ensure our applications remain stable and predictable.
Understanding JavaScript Promise Rejection Events A Deep Dive into Error Handling Patterns - Using Event Listeners to Catch Global Promise Rejection Events
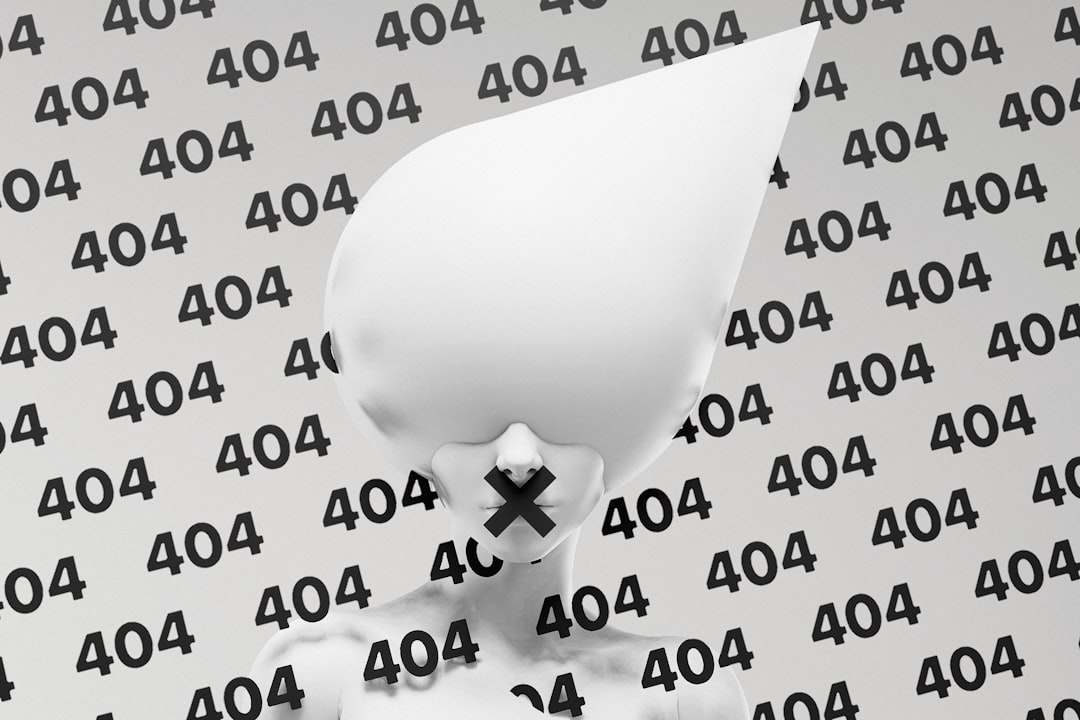
JavaScript's asynchronous nature, while powerful, introduces challenges when dealing with unexpected errors. Unhandled promise rejections, specifically, can lead to unpredictable behavior if not addressed properly. The `unhandledrejection` event plays a crucial role in managing these scenarios. It's triggered whenever a promise is rejected without a corresponding `.catch()` block to handle it. This event essentially bubbles up to the global scope, usually the `window` object in web browsers.
Developers can leverage the `unhandledrejection` event to establish a global error handling mechanism. By attaching an event listener to this event, they gain the ability to define custom logic for processing these uncaught rejections. This approach allows for consistent and centralized handling of errors, improving the stability and reliability of applications. It's important to understand that, by default, many JavaScript environments might log unhandled promise rejections to the console, but this behavior can be controlled by the `unhandledrejection` event handler.
This global event also provides a `PromiseRejectionEvent` object carrying information about the rejected promise and its associated reason. Within the event handler, calling `event.preventDefault()` can inhibit the default behavior of the event, which might involve runtime logging or other system-level reactions. Effectively using this event can create a comprehensive system for managing unhandled rejections. This proactive approach contributes to better error handling practices throughout the JavaScript ecosystem, aiding in creating more stable applications.
JavaScript's asynchronous nature, while powerful, introduces the potential for unhandled promise rejections. These occur when a promise is rejected, but no `.catch()` or `try...catch` block intercepts it. This can lead to unexpected behavior, especially in complex applications.
The `unhandledrejection` event provides a global mechanism to capture these situations. It's emitted to the global scope, typically the `window` object in browsers, signaling that a promise has been rejected without a handler. This event bubbles up the call stack unless intercepted by an event listener.
By attaching a listener to the `unhandledrejection` event, we can implement a custom error handling strategy at a higher level. This allows developers to centralize error management, enabling better logging, reporting, and potential recovery. In many environments like Node.js, unhandled promise rejections trigger default console logging, but these defaults can be overridden with a custom handler. We can specifically configure a function to handle these rejections using the `onunhandledrejection` property of the `WindowEventHandlers` mixin.
However, the `unhandledrejection` event also carries the `PromiseRejectionEvent` object, which contains information about the failed promise, including the rejection reason. This detail can be helpful in debugging and understanding the context of the failure. Furthermore, if needed, `event.preventDefault()` within the event listener allows us to suppress the default actions of the `unhandledrejection` event, such as the logging mechanisms.
It's important to note that the `unhandledrejection` event is triggered only for promises without attached handlers. A promise with a `.catch()` call won't fire this event. Thus, this global event provides a failsafe for scenarios where a promise may have been forgotten or mishandled during development.
Creating global handlers for unhandled rejections allows for better error management across an application. It aids in early detection of potential issues and facilitates the construction of more resilient systems. However, it is crucial to understand the potential trade-offs. Excessive use of global event listeners might have negative performance consequences. Developers must carefully balance the need for error handling with the impact on application responsiveness. Furthermore, the stack traces related to unhandled rejections may deviate from typical synchronous errors. Understanding these potential differences is crucial when debugging.
As we move forward, it's important to be aware of how promises, and specifically the handling of rejections, might change within JavaScript. Keeping up-to-date with changes in language features and community best practices will be important for ensuring the long-term reliability and stability of our code. Testing specifically for promise rejections and how they impact state management is a critical step in creating reliable and predictable systems. The future of promise management is still unfolding, but taking proactive steps to manage unhandled rejections offers developers a valuable toolset to create robust and error-resilient JavaScript applications in this evolving landscape.
Understanding JavaScript Promise Rejection Events A Deep Dive into Error Handling Patterns - Promise Rejection Patterns in Async Functions and Try Catch Blocks
Within the realm of JavaScript's asynchronous operations, effectively managing situations where promises are rejected is critical for application stability. When working with asynchronous functions, the `try...catch` block emerges as a valuable tool, enabling the capture of errors in a way that mirrors synchronous error handling. This approach fosters code clarity and simplifies the maintenance of complex asynchronous logic.
It's essential to recognize that JavaScript incorporates an implicit mechanism for handling exceptions occurring during promise execution. However, ignoring the potential for promise rejections can result in warnings and erratic behavior within your application. Furthermore, establishing clear and well-structured error-handling practices is paramount for enhancing the robustness of your applications, particularly when dealing with nested asynchronous operations. A focused approach to handling promise rejections, incorporating both explicit and implicit mechanisms, will ultimately contribute to the development of more reliable and predictable JavaScript applications.
When a promise is rejected in JavaScript, it doesn't immediately throw an error like synchronous code. Instead, it shifts to a "rejected" state, allowing other asynchronous tasks to continue. This behavior, while beneficial in some ways, can create confusion if not managed carefully, especially when errors cascade through a chain of promises.
Using `try...catch` blocks with async functions offers a familiar error handling structure for asynchronous operations. However, it's worth noting that `try...catch` only catches errors thrown within the `async` function's scope. Rejections from promises outside that function won't be captured.
Unhandled rejections travel up the chain to the nearest `unhandledrejection` event listener. This capability enables developers to centralize error handling across an application, capturing promises that might have slipped through individual `.catch()` blocks. This is a powerful mechanism but can potentially obscure specific, localized errors.
Complex JavaScript programs often involve errors originating from various sources like network hiccups, data parsing issues, or user interactions. In these situations, creating custom error types becomes beneficial. Each custom error type can be paired with unique error handling logic, allowing for a more specific response to particular rejection scenarios.
The global `unhandledrejection` event listener provides a powerful mechanism for catching all unhandled errors, but it's a double-edged sword. While it's useful for detecting unexpected errors, relying too heavily on it might mask underlying problems that should be tackled closer to their origin.
Implementing a global `unhandledrejection` listener adds some overhead to an application's performance. Every time an unhandled rejection occurs, it triggers the listener, potentially creating a performance drain if the event handler isn't designed with performance in mind.
The information associated with a rejected promise can vary. It can be an error object, a string message, or even another promise. This flexibility provides developers with the opportunity to add context around the error, which can greatly assist in debugging and recovery procedures.
Stack traces related to unhandled rejections differ from their synchronous counterparts. This difference can make debugging harder, as the stack trace might not pinpoint the exact location of the promise creation, potentially leading to unnecessary investigation time.
`async/await` provides a clean way to handle rejections, mirroring synchronous exception handling through `try...catch`. While this approach simplifies some things, it's still vital to be vigilant about unhandled rejections outside the `async` function's scope.
The JavaScript landscape is dynamic, and we expect that promise handling will continue to evolve in the future. Keeping informed about new language features and community-driven best practices is essential for engineers to build more robust and resilient applications in this changing world. The future of error handling in JavaScript will likely include refinements to how promises handle rejection, which means keeping up-to-date with best practices will always be important for engineers who want to keep their applications robust and maintainable.
Understanding JavaScript Promise Rejection Events A Deep Dive into Error Handling Patterns - Managing Promise Chain Failures with Multiple Catch Handlers
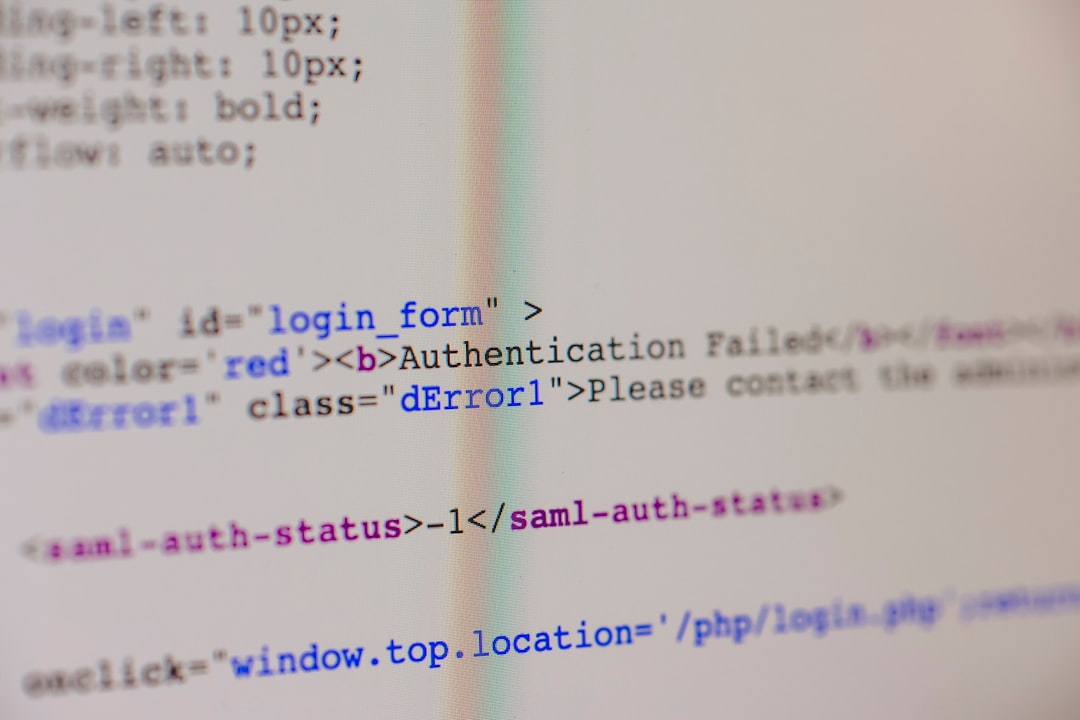
When working with chains of promises in JavaScript, effectively managing potential failures is crucial for creating reliable applications. A powerful technique to address this is using multiple `catch` blocks throughout the promise chain. This approach provides a way to handle errors at specific points in the chain rather than letting them propagate unchecked.
Instead of nesting `catch` blocks deeply, this strategy encourages a cleaner code structure by allowing you to handle different types of errors at different points in the chain. Each `catch` can contain custom logic specific to the stage of the promise chain where it resides. This granular error handling promotes clarity and makes debugging easier.
Utilizing multiple `catch` blocks improves overall code maintainability and contributes to building more reliable applications by preventing unexpected behavior and minimizing the likelihood of unhandled promise rejections. As JavaScript developers hone their skills, mastering error handling patterns like this will undoubtedly help in creating robust and resilient applications. It is a valuable technique that every JavaScript developer should understand to address the inherent challenges associated with asynchronous programming in a way that is both effective and maintainable.
JavaScript's asynchronous nature, while powerful, can introduce subtle issues if we don't manage promise rejections effectively. One concern is that unhandled rejections often lead to silent failures, meaning errors might not be immediately visible, particularly without proper logging or handling. This lack of clear error signals can make it harder to debug issues.
The `unhandledrejection` event offers a safety net, catching any promise rejection that doesn't have a dedicated `.catch()` block. This creates a centralized point for error handling, which can be useful, but it comes at the cost of potentially obscuring the specific origin of an issue.
While a globally centralized approach offers broad visibility, there's a potential performance tradeoff. Each unhandled rejection triggers the listener, which can impact application responsiveness if the event handler is not optimized. We must carefully balance the need for error visibility with the potential for performance degradation.
Using custom error types for specific situations—network issues, validation errors, etc.—provides a lot of clarity. Instead of using a generic error, you can tailor how each error is handled. This leads to more efficient and understandable code.
One challenge with debugging is that the stack trace provided by a promise rejection often doesn't give us clear information on where the original promise was created. This can make it difficult to trace the root of a problem quickly.
`async/await` is helpful for writing cleaner asynchronous code, but it's worth remembering that rejections in `async` functions don't automatically affect the rest of your code unless handled specifically. This can lead to unexpected unhandled rejections if we aren't cautious.
Promise rejection reasons can be diverse—strings, error objects, or even other promises—which offers flexibility but can lead to inconsistent handling. This lack of standardization requires careful planning and organization to prevent confusion.
Errors from rejected promises can move upwards in the call stack until they're caught by a `unhandledrejection` listener. This centralized error handling can become cumbersome as an application grows, as it might generate many error events that are hard to decipher.
The `preventDefault()` method within a `unhandledrejection` event listener provides the capability to override default actions like console logging, giving developers more precise control over handling. This ability to fine-tune the error response is valuable for maintaining a consistent user experience.
Ignoring promise rejections can lead to issues that go beyond performance. Failing to manage these errors can jeopardize overall application stability and hurt user trust. As applications get more complex, handling promise rejections becomes increasingly important, underscoring the necessity of error monitoring tools.
Essentially, handling promise rejections requires careful consideration of the trade-offs between global and local error management, balancing performance impacts, and utilizing custom error types for clarity. Furthermore, understanding the nuances of stack traces in the context of asynchronous operations is crucial for effective debugging. As the JavaScript ecosystem evolves, keeping a watchful eye on changes and incorporating robust error management practices into our applications will help us to ensure the reliability and stability of our software.
Understanding JavaScript Promise Rejection Events A Deep Dive into Error Handling Patterns - Custom Error Classes and Promise Rejection Tracking Strategies
Custom error classes and strategies for tracking promise rejections are valuable tools in the JavaScript developer's arsenal for building more robust applications. By extending the basic `Error` class, we can create custom error types, like `ValidationError` or `NetworkError`, which provide more context when errors occur. This allows for a clearer picture of the problem and ultimately makes debugging easier.
Moreover, JavaScript offers ways to track and handle promise rejections in a more centralized manner. Features like the `unhandledrejection` event allow us to establish a global mechanism for catching errors that don't get caught by individual `catch` blocks within a promise chain. This ensures that no errors slip through the cracks, leading to unexpected application behavior.
However, there's a trade-off. While this global approach is helpful, we need to be aware of how errors propagate asynchronously through JavaScript code. The asynchronous nature of JavaScript can sometimes make it difficult to understand exactly where an error originated. Balancing the benefits of a central error handling strategy with the need to understand the specific circumstances of each rejection event is a challenge developers must address.
Ultimately, developing custom error classes and incorporating strategies for tracking promise rejections are significant steps towards building more reliable JavaScript applications. A deep understanding of these tools and the associated challenges of asynchronous error propagation will help developers write more resilient and predictable code.
Understanding JavaScript Promise Rejection Events A Deep Dive into Error Handling Patterns - Debugging Promise Rejections with Chrome DevTools and Performance Monitoring
This section focuses on using Chrome DevTools and performance monitoring to pinpoint and fix errors related to JavaScript promises that fail. Chrome has made debugging easier by adding features like logging unhandled promise rejections directly in the console and the addition of a Promise Inspector, which gives developers a better view of active promise chains. Using the Chrome DevTools' capabilities, such as placing breakpoints and adding custom event handlers (like `console.error`), lets developers get a more precise understanding of when and why promise rejections occur. While the global `unhandledrejection` event is a powerful tool for handling errors, it can sometimes make it hard to find the original cause of the problem. This highlights the need for a careful balance between global and local error handling strategies when debugging and optimizing for performance. In essence, this section emphasizes the importance of having robust error-handling mechanisms for building stable and high-performance JavaScript applications.
Chrome and other modern browsers have become more helpful in surfacing unhandled promise rejections, moving beyond just warnings to potentially generating full errors that can even crash an application if not handled well. This shift underlines the importance of thoughtful error management in any code using asynchronous operations.
While global events like `unhandledrejection` offer a nice safety net for capturing any errors that slip through local `catch` blocks, over-reliance on them can mask the true origin of errors within larger projects. This can make troubleshooting far more challenging.
The debugging experience for promises can be frustrating because stack traces associated with unhandled rejections may not always point to the exact spot where the initial promise was made. This often requires meticulous logging practices to assist in recreating the flow of events.
Furthermore, global event listeners designed to manage these rejection events can create performance bottlenecks if they aren't optimized. It's important to avoid putting too much complex logic into them, or they can significantly impact an application's overall speed.
Creating custom error classes gives us a more detailed understanding of what went wrong. Instead of generic error messages, they allow us to capture the specific circumstances, such as a validation error or a network failure. This makes debugging more precise and leads to cleaner code.
Rejected promises, and their related errors, can flow up through a chain of asynchronous operations until they're finally caught or handled by a `catch` block. This means that if you're not mindful of where your `catch` calls are positioned, errors can slip through easily.
Having good promise rejection tracking can improve application stability. This could entail building manual logging, or it can be achieved through built-in mechanisms like the `unhandledrejection` event. The goal is to be aware of where in your application issues might arise.
You also have fine-grained control over how errors are logged. The `preventDefault()` method inside a `unhandledrejection` event listener lets you override the browser's default logging behavior and inject your own actions, leading to a cleaner and less noisy debugging experience.
The `PromiseRejectionEvent` contains helpful information about the rejected promise, including details about the cause. This can prove extremely valuable in situations where you need more than just the generic message, offering clues as to what exactly triggered the issue.
The landscape of JavaScript is constantly changing, particularly with how we handle promises and errors. This means that keeping an eye on new features and best practices in error handling is essential for creating robust, stable applications. The way we deal with promise rejections today will likely evolve over time, making continuous learning a critical component of development in this space.
Create AI-powered tutorials effortlessly: Learn, teach, and share knowledge with our intuitive platform. (Get started for free)
More Posts from aitutorialmaker.com: