Leveraging Python's Base64 Module with Type Annotations for Robust Data Encoding
Leveraging Python's Base64 Module with Type Annotations for Robust Data Encoding - Understanding Python's Base64 Module and Its Core Functions
Python's Base64 module is a valuable tool for managing data encoding, particularly when dealing with binary data in text-based environments. At its core, it provides the ability to translate binary data into a readily printable ASCII format, and reverse that process. This is achieved through functions like `b64encode` and `b64decode`, allowing you to easily transform between these two data representations. The module follows the specifications laid out in RFC 4648, encompassing a range of encoding algorithms including the widely used Base64, along with Base32 and Base16. This flexibility allows Base64 to be applied to various situations where different encoding schemes might be advantageous.
One key aspect is how easily it integrates with byte-like objects. This feature makes the module particularly well-suited for tasks like transmitting data across channels designed for text rather than raw binary. While this function is useful, it is worth noting that the encoding process can increase the size of the data. Adding type annotations to functions using the Base64 module can offer additional clarity and improve code readability, especially in large projects where multiple developers might work on the codebase. These type hints are useful for documentation and can contribute to a more maintainable codebase.
Python's `base64` module acts as a bridge between binary data and printable ASCII characters, making it suitable for transmitting data through text-oriented channels like email or JSON. However, this convenience comes with a trade-off: the encoded data typically expands by about 33% due to the encoding process, which involves converting three bytes into four ASCII characters. This expansion is something to be mindful of when considering storage and transmission efficiency.
While the `base64` module simplifies encoding and decoding, careless handling of data can lead to corruption or loss. It's important to handle data types correctly, especially considering the encoded output is in bytes, not strings. Incorrectly assuming string format can cause `TypeError` exceptions.
Beyond binary data encoding, `base64` finds applications in data serialization, helping to facilitate data exchange between various programming languages. This ability to serialize data into a common format is particularly useful in diverse development environments. The Base64 algorithm is not a Python-specific invention, but rather a standardized method defined in RFC 4648. This standard encompasses various Base64 variants, like the URL-safe Base64, that address certain application-specific requirements.
The `base64` module also includes variations, like `urlsafe_b64encode()`, which is specifically tailored for situations where the standard Base64 characters '+' and '/' might interfere with URL parsing. It swaps these characters for '-' and '_' respectively. When dealing with very large datasets, encoding them in a streaming fashion using the `base64.encode()` function is recommended. This approach reduces memory consumption and prevents loading the entire dataset into memory at once.
It's vital to understand that Base64 is simply an encoding method, not an encryption method. It's easily reversible, rendering it unsuitable for protecting sensitive data. Instead, developers should prioritize proven cryptographic techniques for security purposes. Despite the wide adoption of Base64, it's wise to be cautious. There might be inconsistencies in how Base64 is implemented across different platforms. Thorough testing is crucial for ensuring the integrity of your data when working with this encoding technique.
Leveraging Python's Base64 Module with Type Annotations for Robust Data Encoding - Implementing Type Annotations for Base64 Encoding and Decoding
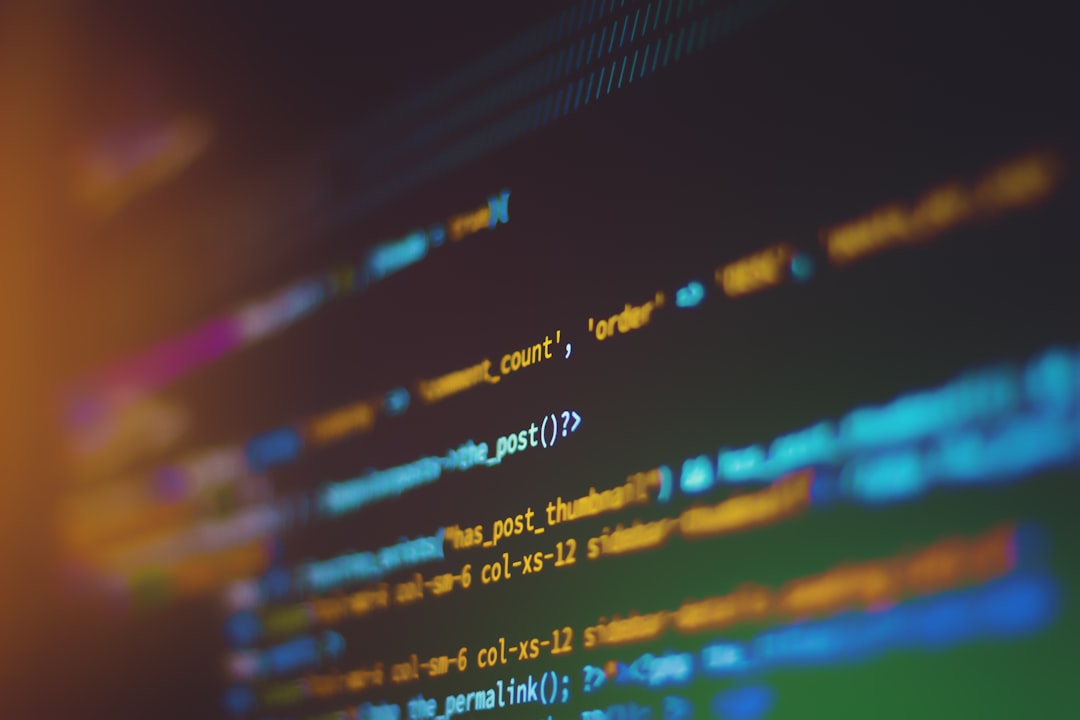
Adding type hints to Base64 encoding and decoding functions significantly improves code quality, especially when multiple people are working on a project. By defining the expected types of inputs and outputs, we can avoid mistakes that often happen when you accidentally treat encoded data as strings instead of bytes. This not only helps with better error handling but also makes the code easier to understand. It's like adding helpful notes for other programmers to easily grasp how the functions work. Since Base64 operations are susceptible to data corruption if not handled carefully, precise type information serves as a safety net. Moreover, type annotations make it easier to update or maintain the code later, which is really helpful as Base64 is used in many different applications. In essence, implementing type annotations contributes to a more reliable and understandable codebase.
Adding type hints to functions within Python's `base64` module can improve the overall robustness and clarity of your code when encoding and decoding data. This practice significantly enhances type safety, minimizing runtime errors stemming from type mismatches. By specifying the expected types for function inputs and outputs, we can create more reliable code, reducing the likelihood of unexpected behavior.
Another advantage is that type annotations allow integrated development environments (IDEs) to provide smarter auto-completion suggestions and more accurate error detection. This improves the development workflow by catching potential issues early on, boosting productivity. When dealing with external systems or libraries that enforce strict type requirements, having type annotations ensures the data encoded via Base64 will comply with those expectations. This helps promote interoperability when interacting with diverse environments.
Furthermore, type hints serve as built-in documentation, enabling easier code review processes. Team members can quickly understand function interfaces and intended use without resorting to digging through external documentation. This becomes especially important in projects with many developers who may have differing levels of familiarity with the codebase.
While these benefits are compelling, we must be cautious about the potential pitfalls. While enhancing clarity and maintainability, type hints don't directly affect runtime performance, a vital consideration when dealing with massive datasets or computationally intense tasks. Moreover, the potential for type mismatch still exists. For example, while a function might be annotated to accept `bytes` data, a `bytearray` object could be passed, potentially leading to confusion or obscure bugs.
Python's type annotations are inherently optional, which means older code using `base64` can continue to work seamlessly. This is a valuable feature for developers maintaining legacy projects that need to gradually adopt type hints over time.
However, type hints shine when integrated with static analysis tools. These tools can detect potential errors before runtime, allowing for faster debugging and higher code stability in complex applications.
Additionally, the use of type hints serves as a supplementary form of documentation. New team members can rapidly grasp how a function operates by examining the annotations without resorting to external documentation. This is a huge boon in collaborative development environments.
One final point to keep in mind is the variety of Base64 encoding variants. If using a function like `urlsafe_b64encode`, accurately annotating function signatures becomes more important to ensure developers recognize the encoded output is specifically optimized for URLs. This distinction is crucial in web development or any context where URL encoding is important. While useful, don't rely on Base64 as a security measure as it can easily be reversed. It's best used for data transformations, not sensitive data protection.
Leveraging Python's Base64 Module with Type Annotations for Robust Data Encoding - Enhancing Data Integrity with Base64 URL-Safe Encoding
When dealing with data in web applications, maintaining data integrity is crucial, especially when transmitting data through URLs. Base64 URL-safe encoding addresses this need by adapting the standard Base64 encoding to be compatible with URL formats. It accomplishes this by substituting the '+' and '/' characters with '-' and '_', respectively. This simple change prevents potential problems that could arise from those characters being misinterpreted within URLs.
This encoding technique is particularly useful when you're embedding data types like images into formats like JSON or XML. Without this modification, the standard Base64 output might cause parsing issues. Base64 URL-safe encoding keeps the encoded data intact and readable, ensuring consistent data integrity when transmitted across systems.
Python's `base64` module readily supports this encoding scheme, offering convenient functions for encoding and decoding. However, it's worth being aware of the potential for padding characters to cause problems. These characters, sometimes added to encoded data, can lead to difficulties on various systems, including web servers, if not handled correctly. By utilizing this URL-safe variant of Base64, developers can improve data integrity and ensure reliable data exchange, specifically when web-based transmission is involved. This ensures data isn't corrupted during its journey between systems.
Base64 URL-safe encoding, as described in RFC 4648, essentially modifies the standard Base64 encoding by replacing the "+" and "/" characters with "-" and "_". This change is vital because it avoids problems that can arise when encoded data is used within URLs, as those characters could be misinterpreted by the URL parsing mechanisms. Keeping data intact within URLs is crucial for ensuring that binary data can be successfully transmitted across networks without corruption.
This modified character set helps to manage data integrity, particularly within URLs that may be subjected to modifications or truncation. The limited set of URL-safe characters helps minimize unexpected encoding errors that could otherwise occur in web contexts like HTML or URLs. By implementing Base64 URL-safe alongside type annotations, we can also improve code efficiency, which is especially useful for high-performance applications.
However, we must be wary of common misconceptions. While the encoding process only involves swapping a few characters, developers sometimes treat the resulting data as simple strings, which can be problematic. This can lead to confusion during decoding, potentially resulting in faulty or incomplete data retrieval.
Base64 URL-safe encoding contributes to enhanced cross-platform compatibility. This helps when we exchange data across different software systems since it promotes a common encoding format that reduces the chance of compatibility issues. We see it used in areas like JSON Web Tokens (JWT) and other authentication protocols, where claims and identifiers must be securely encoded for transmission over the web without interference from URL conventions.
When decoding, we have to be careful. The decoding process also needs to take those specific alterations into account. Failure to reverse the swapped characters can corrupt the decoded output, making it impossible to interpret the data properly.
It's crucial to reiterate that this URL-safe version of Base64 is still not a security measure in itself. While it improves how binary data can be transmitted through URLs, it doesn't provide encryption for sensitive information. We should avoid relying on it for security, as it is still relatively easy to reverse, and this can lead to security vulnerabilities if used improperly.
Since its formalization, the usage of Base64 URL-safe encoding has grown in popularity, especially in web development environments. This reflects a growing need for reliable and compact methods of representing binary data in web applications, highlighting the importance of carefully managing the encoding and decoding processes to ensure data integrity within diverse web systems.
Leveraging Python's Base64 Module with Type Annotations for Robust Data Encoding - Optimizing Performance in Base64 Operations with Type Hints
When it comes to Base64 operations, optimizing performance while maintaining code quality is key. Type hints, through their ability to specify expected data types, play a crucial role in achieving this balance. By clearly defining input and output types for functions, we minimize the risk of runtime errors related to type mismatches, fostering more robust code. Furthermore, type hints contribute to a cleaner, more comprehensible codebase, making it easier for developers to understand how Base64 functions behave, especially in large or complex projects.
The advantages extend beyond error prevention. Type hints empower IDEs to offer more intelligent auto-completion and facilitate earlier error detection during development. This streamlined workflow leads to faster debugging and improved productivity. Moreover, type hints serve as a valuable form of inline documentation, allowing developers to quickly grasp function behavior without constantly referencing external documentation, which is especially helpful in collaborative projects.
Beyond type hints, we can directly impact performance by adopting techniques like utilizing custom Base64 writers designed to process data in larger chunks. This strategy reduces the frequency of smaller encoding/decoding operations, leading to a more efficient use of resources. By adopting this approach and leveraging type hints thoughtfully, we improve not only the clarity of our code but also its performance, creating a more resilient and efficient implementation of Python's Base64 module.
Python's type hints, introduced in PEP 484, don't enforce type checks during runtime, but they are instrumental for developers and tools like mypy to perform type checking and spot potential issues. When it comes to the `base64` module, which handles encoding and decoding binary data into a text-friendly format, type hints can be quite useful. While they primarily benefit code clarity and maintainability, type hints can indirectly impact performance, especially in the context of large datasets.
For instance, Base64 encoding increases data size by about a third, but if we utilize functions like `base64.encode()`, which process data in streams, we can avoid loading huge datasets into memory at once, leading to more efficient processing. Furthermore, when applying type hints to our functions, we are not limited to simple types like `bytes`. We can incorporate `bytearray` or even build our own types through the `typing` module. This flexibility promotes adaptable and maintainable code, especially as projects and teams grow.
The use of type hints can also improve the functionality of your IDE. This means better auto-completion suggestions, which in turn reduces the chances of making silly errors. This can save time for engineers, as they get relevant coding suggestions at their fingertips. We can also leverage type hints to improve our static analysis tools. This can mean static analysis tools get smarter about finding errors, often identifying them before runtime. This proactively reduces the possibility of some runtime errors, contributing to a more stable application.
On the interoperability side, type hints can assure our code will work as intended when it interacts with systems that have strict type requirements. By clearly defining what inputs and outputs we anticipate in our Base64 functions, we lower the chance of type mismatch-related conflicts. Despite these benefits, it's important to recognize that type hints do not inherently improve runtime performance. This means that while we are improving code quality and maintainability, we still need to pay attention to bottlenecks in our performance-critical code.
Type hints can help in understanding how errors might propagate in Base64 operations. For example, incorrectly using string functions with bytes can lead to hard-to-find bugs. The clearer type information you have, the more obvious some of these errors become. Fortunately, type hints can be gradually added to existing Base64-based code. This gradual integration helps when maintaining older code that hasn't benefited from type hints yet.
It is worth noting that while Base64 is a standardized method, different platform implementations can vary, possibly leading to unexpected behaviors. With type hints, we can establish clear expectations for data formats and behaviours across systems, making it easier to maintain consistency. It's also important to reiterate that Base64 is not a replacement for encryption. Sometimes developers may incorrectly assume it is because it makes data less human-readable. Type hints and code reviews can help ensure that developers don't assume the function of Base64 is more than it is. Proper cryptographic methods are always needed when handling sensitive data.
In conclusion, while the Base64 module is handy for many common tasks in Python, adopting type hints helps promote code quality, reliability, and readability. These benefits are particularly significant when working in teams, interacting with external systems, or handling large datasets. However, one needs to remember that type hints are a tool, not a magic bullet, and they must be judiciously applied to gain the intended advantages. This careful application of type hints and other best practices will lead to a more robust and optimized `base64` workflow.
Leveraging Python's Base64 Module with Type Annotations for Robust Data Encoding - Handling Binary Data Transmission Using Base64 in Web Applications
Web applications often need to send binary data (like images or files) across networks, but HTTP, the standard protocol for web communication, primarily works with text. This is where Base64 encoding becomes essential. It transforms binary data into a readily printable ASCII format, making it safe for transport through channels like email, JSON, or even embedded within URLs. This process aligns with the specifications outlined in RFC 4648.
Python's `base64` module comes in handy for encoding and decoding binary data using Base64. You can use functions like `b64encode` and `b64decode` to easily switch between binary and ASCII formats. However, it's worth noting that Base64 encoding does increase the size of the data by about 33%, which can become a concern when dealing with large datasets and network bandwidth.
To handle scenarios where Base64 encoded data is used in URLs, a special variant called URL-safe Base64 exists. This variant replaces certain characters (like "+" and "/") with more URL-friendly alternatives ("-" and "_"). This helps prevent encoding issues related to URL-specific limitations, enhancing data integrity when URLs are used as a transport mechanism.
It's crucial to remember that Base64 is purely an encoding technique, not a security measure. While it makes binary data printable, it's easily reversible, meaning it's not suitable for protecting sensitive information. Securely protecting data requires the use of encryption algorithms specifically designed for that purpose. Developers should carefully consider the tradeoffs and avoid relying on Base64 for confidentiality.
When working with Base64 in web applications, it's crucial to understand the trade-offs involved. Base64 encoding, while useful, inflates data by about a third, potentially increasing storage and transmission costs, particularly for large datasets. This factor requires careful consideration in resource-intensive scenarios.
For web applications, URL-safe Base64 is valuable because it replaces characters like '+' and '/' with '-' and '_'. This subtle change ensures data is transmitted smoothly in URLs, avoiding parsing errors and guaranteeing data integrity.
When faced with vast amounts of data, utilizing `base64.encode()` offers a solution for handling data in chunks, preventing the system from loading the entire dataset into memory at once. This is helpful on systems with limited resources.
However, one needs to be aware that padding characters, sometimes added to encoded data, can lead to data corruption if not handled correctly during encoding or decoding. It's a detail that can easily cause unexpected issues.
Python's Base64 encoding isn't exclusive to Python. It's a widely adopted standard, which makes it a convenient way to exchange data across systems written in various languages. This compatibility across diverse systems highlights Base64's value as a common format for data exchange.
Type annotations, through their ability to provide hints about the nature of data being processed, empower integrated development environments to improve the developer experience. This improvement in IDE functionality manifests in features like auto-completion and error detection, which streamline the development process and reduce the chance of silly mistakes.
Despite its usefulness, Base64 isn't a replacement for encryption. It is a common misconception that Base64 offers protection. It's crucial to remember that Base64 is simply a reversible encoding method. This means that sensitive information still requires the protection of established cryptographic techniques.
There's a potential for inconsistencies across platforms in how Base64 is implemented, which can result in errors if not carefully considered. Testing is essential to ensure data isn't corrupted when using Base64 across diverse systems or frameworks.
Base64 finds significant applications in modern authentication schemes. JSON Web Tokens, a common way to convey identity and security data over web applications, rely on Base64 to ensure the information is properly encoded for transmission within URLs.
Python's type annotations are not limited to simple types. They can handle advanced data formats like `bytearray`, offering a lot of flexibility when writing code that interacts with Base64. This adaptability allows for versatile code that can handle diverse scenarios within Base64 operations.
These aspects of Base64 are worth remembering when developing web applications or applications that need to handle the transfer of binary data. It's a valuable tool, but like any other tool, understanding its limits and how to best use it leads to a better final product.
Leveraging Python's Base64 Module with Type Annotations for Robust Data Encoding - Integrating Base64 Encoding with Python's Type Checking Tools
Integrating Base64 encoding with Python's type checking tools provides a way to improve the reliability and readability of code that handles encoded data. Type annotations allow developers to specify the expected input and output types for Base64 functions, making it easier to catch potential issues like mistakenly treating encoded bytes as strings. This level of detail helps make the codebase more maintainable and easier to understand, especially for teams working on a project together. Additionally, type hints empower IDEs to provide better auto-completion and error detection, resulting in a smoother development process. It's important to keep in mind that while Base64 offers a simple way to represent binary data in a text-based format, it is not a security tool. Base64 encoding is reversible, so for truly sensitive data, encryption is the only reliable protection. Developers must be aware of these limitations when deciding how to apply Base64 encoding within their projects.
Base64 encoding, while helpful for transforming binary data into a printable ASCII format, leads to an approximate 33% increase in data size. This is a key aspect to consider when working with constrained environments like mobile devices or systems with limited bandwidth. The encoding process itself is prone to errors if not handled correctly. For instance, mistakenly treating encoded data as a string instead of bytes can result in `TypeError` exceptions. To mitigate this, you can employ the `base64.encode()` function, which processes large datasets in manageable chunks. This approach helps prevent memory overload, particularly useful for resource-constrained systems.
Base64's URL-safe variant addresses the need to encode data for use in URLs by exchanging the '+' and '/' characters with '-' and '_'. This simple adjustment highlights the importance of character selection to ensure data integrity within web contexts. When considering code robustness, type hints can help establish compatibility with other systems and libraries. This is especially relevant when dealing with codebases where type correctness is strictly enforced. These type hints also become valuable information for static analysis tools to perform type checks and detect potential issues before they result in runtime errors.
The importance of Base64 extends to security frameworks as well. Authentication mechanisms like JSON Web Tokens (JWT) rely on Base64 to transmit critical data securely within URLs. However, it's crucial to be mindful of the padding characters ('=') sometimes added to encoded data, which, if not properly handled, can lead to data corruption. The flexibility of Python's type hints extends beyond basic types. You can utilize the `typing` module to define more intricate types, fostering adaptability in Base64 operations and overall code maintainability.
Base64 is a standardized method defined by RFC 4648, promoting universal compatibility across platforms. But, as with any standard, slight variations in implementation across different systems can lead to unexpected outcomes. It's important to be aware of these discrepancies and test code thoroughly to ensure that data integrity is preserved. Notably, Base64 should not be mistaken for a security method as it can easily be reversed. While it offers a convenient method for encoding binary data for various purposes, it's not a solution for securing sensitive information.
More Posts from aitutorialmaker.com: