Create AI-powered tutorials effortlessly: Learn, teach, and share knowledge with our intuitive platform. (Get started for free)
Mastering C++ Compilation A Step-by-Step Guide to Using GCC in 2024
Mastering C++ Compilation A Step-by-Step Guide to Using GCC in 2024 - Setting up GCC on your system in 2024
Getting GCC up and running on your system in 2024 is relatively easy, especially on Windows. The most common way to do this is by employing MinGW. Once you've downloaded and installed MinGW, a key step is configuring your system's PATH environment variable to include the MinGW bin directory. This ensures you can readily access GCC through the command prompt. A simple check of the GCC version via the command line confirms a successful setup.
When you're ready to compile a C program using GCC, keep in mind the command structure `gcc sourcefile.c -o programname`. This requires making sure your source file is in the correct directory. Furthermore, if you're working with Visual Studio Code, you'll need to configure it to point to the location of the GCC compiler executable within your system. For developers handling 64-bit projects, using MinGW-w64 might be more suitable due to its improved compatibility. There are occasional installation hurdles which often come down to firewall settings or needing to match the MinGW version with your Windows variant.
To get GCC working on your Windows system in 2024, you'll first need to download the MinGW installer from the official MinGW site. Once installed, make sure the MinGW 'bin' directory is added to your system's PATH environment variable. This will let you use GCC commands in the command prompt.
After the install, check if everything is set up correctly. Open the Command Prompt and type 'gcc version' – you should see the GCC version details printed. To compile a basic C program, you'd use the command like this: "gcc sourcefile.c -o programname", with "sourcefile.c" being your C code and "programname" being the name for the executable you want to create.
If you're using Visual Studio Code, you need to tell it to use the GCC compiler. You can do this by updating the settings to point to the actual GCC executable file.
When you install MinGW, you'll have to select the parts you need and apply those changes. MinGW will then fetch and install the necessary GCC components. Compiling itself happens in stages: the code is preprocessed, then compiled, and finally linked to generate the executable file.
For Windows systems, MinGW-w64 is generally recommended. It provides better support and compatibility especially if you're working with 64-bit applications. It's worth noting that you will likely find yourself using the 'cd' command frequently to switch between directories and locate your source files before compiling.
If you run into problems during the installation, you might want to check your firewall settings and make certain you've downloaded the correct MinGW version that matches your Windows setup. It's surprising that it is still necessary to double check these kinds of details given the level of maturity in software distribution. It's still 2024 and some of these hurdles are yet to be properly addressed. Perhaps it is time to look at containerized approaches more thoroughly.
Mastering C++ Compilation A Step-by-Step Guide to Using GCC in 2024 - Understanding the basic compilation process
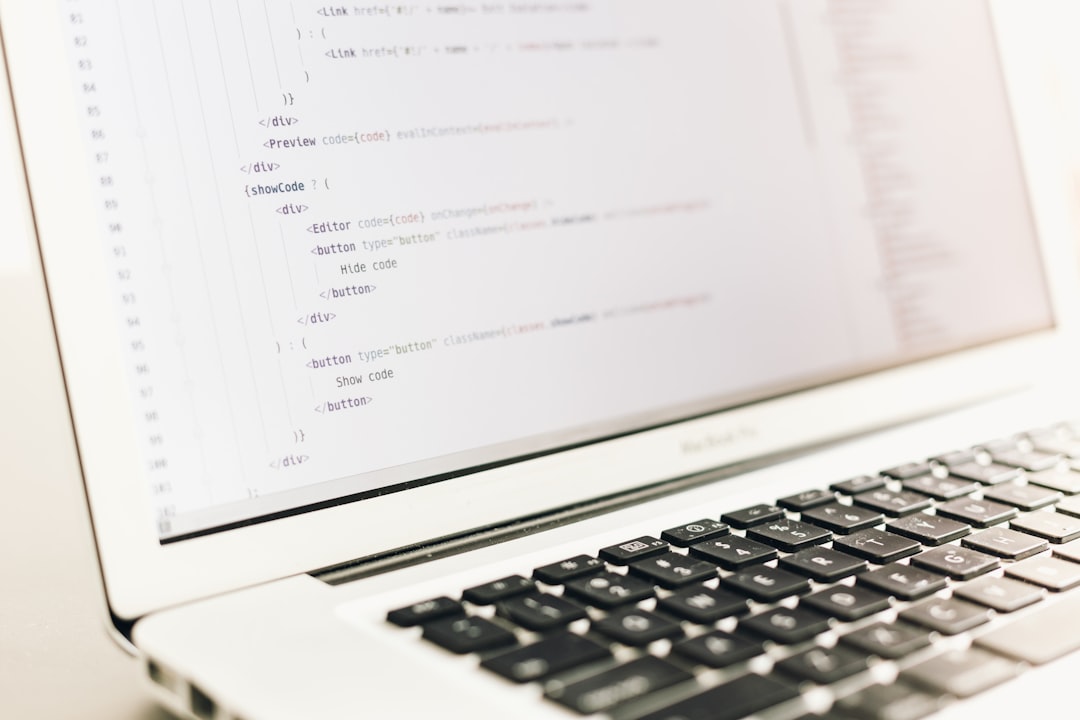
To effectively work with C++ code, understanding how it gets transformed into an executable is crucial. The process, typically managed using the GCC compiler, involves a series of steps that can initially seem complex. First, the preprocessor handles directives like includes and macro definitions, essentially preparing the code. This preprocessed code is then fed into the compiler, which translates it into assembly language, a human-readable representation of machine instructions. Subsequently, the assembler transforms this assembly code into machine code, resulting in an object file. Finally, the linker takes over, combining all related object files and resolving any references to create a single executable program. Understanding how each of these stages contributes to the overall process is essential for debugging issues and ultimately, writing more efficient C++ code. While the process itself might appear somewhat opaque, breaking it down into its component parts reveals the logic and helps programmers gain deeper insight into how their code is executed.
The compilation process, a core aspect of turning our C++ code into executable programs, unfolds in four main stages: preprocessing, compilation, assembly, and linking. Each stage has a unique role to play in the transformation of our code. Preprocessing, often a significant portion of the total compile time, handles things like including header files and expanding macros. Directives like `#include` and `#define` guide the preprocessor, influencing the entire compilation flow.
During the compilation phase, the compiler takes the preprocessed code and transforms it into assembly language tailored for the target processor. Some compilers, like GCC, create an intermediate representation of the code at this point, potentially leading to optimizations or cross-platform compatibility.
The assembly stage converts the assembly language into machine code, leading to an object file (often with a `.o` extension). These object files can be vastly different in size based on code complexity and compiler optimization settings.
The linking stage integrates one or more object files into a single executable. A key part of linking is resolving external references between code sections and external libraries. Developers often encounter "linker errors" during this stage, which stem from missing or incompatible dependencies, and can be frustrating to debug especially for those less familiar with compilation.
GCC, the compiler at the heart of many Linux distributions, offers numerous optimization levels. Using options like `-O3` enables extensive optimizations, which can lead to significantly faster code execution but also might make debugging more complex. Finding the right balance for optimization is often a trade-off between compile time, code clarity, and performance.
Part of the linking process involves constructing a symbol table that catalogs variables and function addresses. While this table aids in optimizing memory usage, it can have a significant impact on build times for large projects with many symbols.
Choosing between static and dynamic linking impacts both executable size and runtime dependencies. Static linking integrates all library code directly into the executable, making it independent of external libraries. Dynamic linking, on the other hand, relies on external libraries at runtime, which could result in version incompatibility issues.
Makefiles become indispensable in projects with multiple source files. They manage dependencies between source code files and ensure only the necessary files are recompiled, streamlining the build process and saving time.
GCC provides features like `-Wall` which generate warnings about potential coding issues. While warnings can help spot potential pitfalls, developers need to balance the benefit of addressing every warning with the associated increase in code complexity. It's important to exercise judgement regarding whether to pursue every warning.
The compilation process, while essential, can be opaque to beginners. Through understanding each of its components and using command-line tools, we can demystify this intricate procedure and gain a deeper appreciation for the transformations that our code undergoes before becoming functional programs.
Mastering C++ Compilation A Step-by-Step Guide to Using GCC in 2024 - Essential GCC command-line options for C++ developers
When working with GCC for C++ compilation, a solid understanding of its command-line options is essential. These options can greatly simplify and enhance the entire development process. Basic options such as `-Wall` for displaying all warning messages, `-O` for defining optimization levels, and `-g` for enabling debugging symbols are fundamental for every developer. Using option files, via the `@` symbol followed by a file name, lets developers manage multiple options in a more organized manner, making the compilation flow smoother. GCC offers tools like `-finstrument-functions` for performance profiling, as well as various 'developer options' designed to help with debugging and analyzing code issues. By becoming adept at leveraging these command-line options, developers can fine-tune the build process, gain more control over the output, and debug more efficiently, significantly improving their overall productivity. While it might seem like a lot to learn initially, the payoff in terms of improved debugging capabilities and faster compile times justifies taking the time to understand these options.
The GNU Compiler Collection (GCC) offers a plethora of command-line options, enabling developers to fine-tune the C++ compilation process. Basic usage follows the format `gcc [options] sourcefile.cpp -o outputfile`, though understanding the many options is crucial for more advanced work.
The `-g` flag, for instance, embeds debugging information in the executable, allowing tools like GDB to directly step through your code rather than just looking at raw machine instructions. It's a godsend when things go wrong.
Speaking of "going wrong", optimization levels (like `-O1`, `-O2`, and `-O3`) can sometimes lead to peculiar program behavior, even as they significantly boost performance. GCC’s aggressive code reordering or inlining might obscure what's really happening during debugging, which is an interesting quirk of this technique.
Another handy option is `-include`, allowing precompiled headers to be included. This approach is beneficial for larger projects, effectively saving compile time by reducing the redundant processing of header files. The trade-off for this is greater complexity in build management.
Choosing between static and dynamic linking via `-static` or `-shared` impacts program size and external library dependencies. Static linking produces self-contained executables, which might be helpful in certain environments. On the other hand, dynamic libraries keep the program compact but introduce runtime library compatibility issues. It's a fascinating trade-off, and selecting the best approach hinges on the intended application of the code.
For managing larger projects, the `-c` flag instructs GCC to compile source files into object files, skipping the linking stage. This allows for building projects in increments and only recompiling changed sections, saving considerable development time. Linking itself is also managed through GCC, using the `-l` to link to specific libraries and `-L` to specify their location. Forgetting to properly point GCC to libraries often results in frustrating linker errors, reminding us how critical the linkage phase is.
The `-v` option generates detailed compiler output, including invoked commands and their intermediate outputs. This is a powerful debugging aid, especially for understanding the more obscure phases of the GCC compilation process. Developers can even tailor GCC to different operating systems and architectures using `--target`– a useful feature when developing for embedded devices or other uncommon hardware.
Another interesting technique in GCC is link-time optimization (`-flto`). This leads to performance gains at the cost of potentially longer compile times. GCC can make optimizations across compilation units with LTO which is not possible otherwise.
Finally, options like `-Wall` and `-Wextra` enable more comprehensive compiler warnings, encouraging cleaner and safer code. Complementing these features is `-std=c++11` (or newer versions), which ensures that code is compliant with the chosen standard and supports the latest features of the language.
It's evident that GCC is a robust and versatile tool, but it's clear that a thorough understanding of the options it offers is needed for true command over your builds and your code's behavior. It's a constant journey of learning, and it's fascinating how much control we have in this age of abstraction.
Mastering C++ Compilation A Step-by-Step Guide to Using GCC in 2024 - Optimizing your code with GCC compiler flags
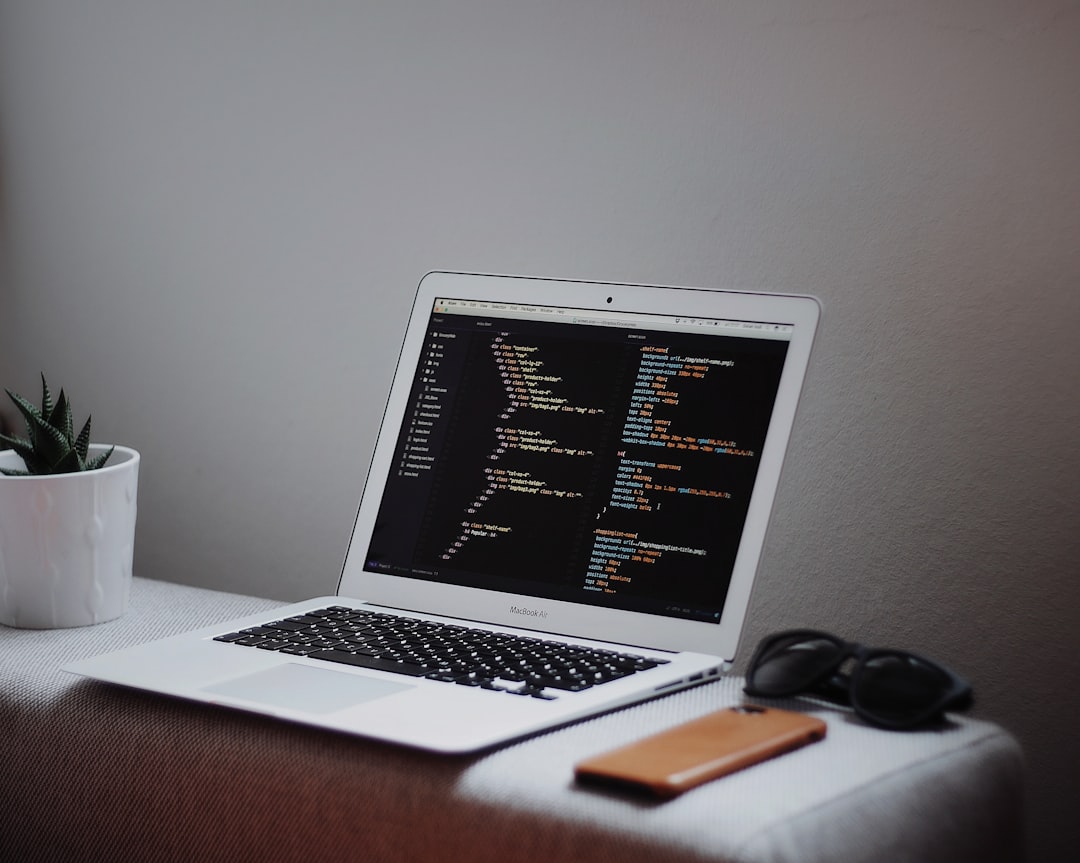
GCC provides a range of optimization flags that you can use to tweak the compilation process and improve the performance of your C++ code. These flags, accessed via the `-O` option followed by a number (like `-O2` or `-O3`), allow you to fine-tune how aggressively the compiler optimizes your code. While these settings can yield impressive speed increases, keep in mind that they often come with trade-offs. Compile times might increase and your executable files could grow in size. The best settings will vary based on your specific project.
Beyond simply choosing an optimization level, you can use other flags to refine the optimization process further. For example, GCC allows for things like dead code elimination which can remove unused sections of your code, leading to smaller and faster binaries. You can also experiment with features like inlining functions, a technique that can improve performance by embedding function calls directly into the calling code. However, inlining everything might lead to larger executables, making careful consideration of this technique essential.
Overall, GCC's optimization capabilities are powerful tools for C++ developers. By carefully exploring and experimenting with the various flags available, developers can create optimized executables that are tailored to specific performance requirements. This ability to adjust compilation at a very low level gives developers tremendous flexibility and can be the difference between a fast running program and a sluggish one.
GCC offers a range of optimization flags that let developers tailor the compilation process to boost performance, but it's a landscape filled with interesting trade-offs. For instance, using `-O3` can lead to massive performance increases, sometimes up to 300%, particularly for computationally intensive tasks. However, it often comes at a cost – larger binaries and potentially less predictable behavior if your code depends on specific execution sequences.
This push for speed has an interesting counterpart in the `-Os` flag, which prioritizes code size reduction. This is ideal when dealing with embedded systems with limited memory, though it might sacrifice some execution speed since certain speed-boosting optimizations are disabled.
One intriguing optimization technique is loop unrolling, where GCC automatically expands loops when optimization flags like `-O2` or `-O3` are in play. This minimizes loop control overhead, significantly improving performance, especially in nested loop scenarios. However, it can lead to noticeably larger binary sizes.
Another intriguing GCC feature is link-time optimization, enabled with `-flto`. This shifts optimization from compile-time to link-time, allowing GCC to consider the entire program for optimization, potentially leading to performance increases of up to 20% across multiple files.
Function inlining, prompted by the `-finline-functions` flag, can reduce function call overhead. But, if overused, this can make your binary swell and increase pressure on the CPU cache, which can actually decrease performance.
It’s fascinating that GCC's optimization process, particularly at higher optimization levels like `-O2` and `-O3`, sometimes leverages speculative execution. Essentially, it tries to predict the execution path and optimizes accordingly. If those predictions are wrong, it can impact performance, creating a delicate balancing act in optimization.
Profile-Guided Optimization (PGO) provides another layer of nuance. With `-fprofile-generate` and `-fprofile-use`, you can collect runtime information to inform optimization choices, which can improve performance by 10-30% or more based on the actual execution flow.
GCC can also automatically vectorize loops, enabling SIMD operations that operate on multiple data points simultaneously when optimization flags are present. While this can greatly increase performance, it requires a CPU with support for SIMD instructions.
Unfortunately, optimizations, particularly at the higher levels, can often strip out crucial debugging information, making it difficult to troubleshoot when errors occur. For that reason, developers might choose to use `-g` with a lower optimization level during development to facilitate easier debugging. This highlights the tension between optimal performance and debuggability.
Finally, GCC can optimize code differently depending on the specific target architecture, as specified with flags like `-march`. It's easy to forget that this behavior exists, leading to portability issues when moving code to different hardware, where the same code may behave very differently.
This overview demonstrates that code optimization with GCC isn't a simple on/off switch. There are many aspects to consider, and developers must carefully assess the trade-offs for a specific codebase. The compiler flags offer a great deal of control, but that control also comes with responsibility to understand the impact of each choice. It's a fascinating domain of exploration for anyone interested in squeezing every ounce of performance from their code.
Mastering C++ Compilation A Step-by-Step Guide to Using GCC in 2024 - Debugging techniques using GCC and associated tools
Debugging C++ code effectively using GCC and its associated tools is essential in 2024. The GCC compiler, when invoked with the `-g` option, includes debugging information within the resulting executable file. This information is key, as it enables the GNU Debugger (GDB) to perform its magic. With GDB, you can strategically place breakpoints at specific locations in your code, inspect variable values at runtime, and step through your code line by line. This level of control is crucial for tracking down and understanding the root cause of errors.
It's also important to leverage GCC's warning system, through flags like `-Wall`, to catch potential coding issues during compilation. Sometimes, compiler optimization techniques, although performance-enhancing, can hinder debugging efforts by rearranging or removing code in ways that are difficult to trace. For this reason, it's wise to use the `-O0` flag during debugging to disable optimizations and ensure the compiled code maintains a closer relationship to the original source.
Understanding the entire compilation process—preprocessing, compilation, assembly, and linking—gives you a stronger debugging foundation. If you grasp how these stages work, you can more efficiently narrow down the source of a problem. By dissecting the various outputs that GCC produces at different stages of compilation, it's possible to home in on where the problem likely lies in your code.
In summary, harnessing GCC's debugging features in conjunction with GDB enhances your ability to identify and address issues within your C++ programs. By leveraging these tools and by comprehending the various steps of the compilation process, developers significantly boost their ability to write robust and reliable code. As a result, it can be expected that development time decreases and confidence increases.
GCC, being the backbone of many compiler toolchains, offers a rich set of debugging tools and features alongside its compilation capabilities. A core element is its interaction with GDB. By using the `-g` compiler flag, you provide debugging information to GDB, making it possible to interact with your running program, set breakpoints, examine variables, and unravel stack traces—a vital aspect of finding the root cause of unexpected program behavior.
While GCC is good at producing optimized code, developers can use the `-Wall` and `-Wextra` options to enable a comprehensive set of warnings. It is surprising how often these warnings are ignored, yet often they hint at subtle logic errors, issues related to uninitialized variables, and other problems that could potentially lead to runtime failures. These warnings are a good example of preventive measures which could prevent a larger problem down the line.
For increased performance, developers can utilize the `-flto` option, enabling link-time optimization (LTO). LTO's potential to improve performance can be significant (up to 20%), however it comes with the added complexity of orchestrating the optimization phases across multiple modules. This is another fascinating feature with many facets to explore.
Higher optimization levels, like `-O3`, can be a mixed bag. While they enhance performance by aggressively optimizing the code, the cost is a potential divergence between the original source and the generated machine code. Reordering instructions or inlining functions might make it hard to debug a specific piece of code based on its original source code representation, requiring careful consideration when tracing program execution.
The balance between speed and efficiency isn't always clear. Optimizations can lead to increased memory use. It is easy to envision how the compiler might change function boundaries in ways that increase the working set size of a program, possibly leading to worse performance even when instructions are processed faster due to optimization flags being used. Developers need to experiment with the codebase and understand the trade-offs associated with choosing different flags.
For projects with a large number of header files, precompiled headers help cut compilation times. The `-include` option tells the compiler to use precompiled headers, but managing these headers across a large project is another layer of complexity to consider.
Profiling a program can give useful insights to understanding how code is behaving, helping to guide optimization efforts. GCC's `-finstrument-functions` flag instrument's the code for this purpose, helping developers pinpoint where their program might be spending the majority of its time.
GCC offers ways to reduce the symbol visibility, using the `-fvisibility` flag. This can improve code loading times in libraries and potentially minimize the impact on system performance, but it also adds complexity to the linking process.
The idea of "hints" which can help improve code generation or performance is an interesting feature. Attributes like `noreturn` and `pure` provide insights on how the function should be handled and this can reduce memory use or improve code efficiency.
GCC supports embedded systems with features like the `-Os` flag, which focuses on minimizing binary size. For environments where code space is a concern, this option is vital.
GCC has many facets and a great deal of flexibility. Understanding the nuances of compiler flags and how they affect program behavior will pay dividends for any developer using this powerful tool. It's a continuous learning journey.
Mastering C++ Compilation A Step-by-Step Guide to Using GCC in 2024 - Integrating GCC with modern C++ development workflows
GCC's integration into contemporary C++ development practices has become increasingly vital for maximizing efficiency and streamlining the compilation process. Modern developers are gravitating towards containerization, employing technologies like Docker to create stable and easily reproducible environments. These containers neatly package GCC alongside its required dependencies, thereby simplifying setup, minimizing version conflicts, and fostering seamless collaboration among team members despite varying system configurations. Further, leveraging sophisticated GCC features like link-time optimization and profiling tools can reveal insights into the performance and efficiency of code, allowing developers to fine-tune applications to meet specific demands. However, developers must remain cognizant of the complexities and trade-offs associated with GCC's optimization and debugging features. It's essential to strike a balance between code optimization and preserving code clarity and maintainability to ensure future development efforts are not hindered by complex and difficult to understand code.
GCC, while often viewed as a solitary compiler, is more accurately understood as a crucial part of a wider toolchain. It effortlessly blends with tools like `make` and `cmake`, and even integrates with modern IDEs. This integration helps streamline the development process through automated building and project management. It's impressive how well GCC has kept pace with the evolution of C++. It offers great support for modern features found in C++11, C++14, C++17, and C++20. This makes it incredibly useful for developers who want to use the newest language constructs without sacrificing performance or compatibility.
GCC also empowers developers to build executables for a wide variety of systems and architectures from a single development environment using cross-compilation. This is particularly helpful when dealing with embedded systems or distributed applications where distinct builds are needed for each platform. However, some debugging challenges are more easily addressed using external tools. While GCC has excellent built-in profiling capabilities, memory leak detection is often aided by using external tools like Valgrind alongside the compiler's output. This combined approach is particularly valuable for gaining deep insights into memory management and performance bottlenecks.
Optimization flags like `-O3` can unlock substantial performance benefits, but there's often a trade-off involved. The more aggressive the optimization, the greater the potential for the program to behave differently from expectations. This stems from GCC’s ability to reorder instructions and inline functions, which can sometimes obscure the logical flow of the code. Consequently, thorough testing under different optimization levels becomes critically important.
The integration of GCC into modern IDEs like CLion and Visual Studio Code is a real boon for developers. These IDEs provide features like auto-completion and refactoring, as well as integrated debugging, streamlining development and eliminating the need for excessive configuration.
GCC provides the `-Werror` flag, which turns compiler warnings into errors, compelling developers to address each warning during the development cycle. This approach undeniably makes for cleaner, safer code. However, it can lead to a stricter development workflow where resolving every warning becomes mandatory, often leading to longer development cycles.
Memory corruption and undefined behavior are common development woes. GCC tackles these challenges through the inclusion of sanitizers like AddressSanitizer and UndefinedBehaviorSanitizer. These runtime tools help to ensure program integrity and can be invaluable for catching potentially damaging issues, leading to more secure software.
GCC's command structure allows developers to build different types of programs by defining configuration options or build types (debug vs release). This is clever because it automatically toggles certain flags, smoothing the workflow and streamlining the build process. This aspect often gets overlooked when discussing the capabilities of the GCC toolchain.
GCC's open-source nature is a powerful asset. It empowers a vibrant community to actively contribute to the development and improvement of the compiler. This fosters a constant cycle of fixes, feature additions, and optimizations. It is worth celebrating this kind of sustained community activity. This ongoing collaborative effort ensures that GCC remains a powerful tool at the cutting edge of compiler technology, benefiting users and fostering innovation. It's quite a testament to the collective power of individuals to work together to improve and enhance our digital world.
Create AI-powered tutorials effortlessly: Learn, teach, and share knowledge with our intuitive platform. (Get started for free)
More Posts from aitutorialmaker.com: