Mastering Python's 'import' Statement A Deep Dive into File Importing Techniques
Mastering Python's 'import' Statement A Deep Dive into File Importing Techniques - Understanding the Basics of Python's import Statement
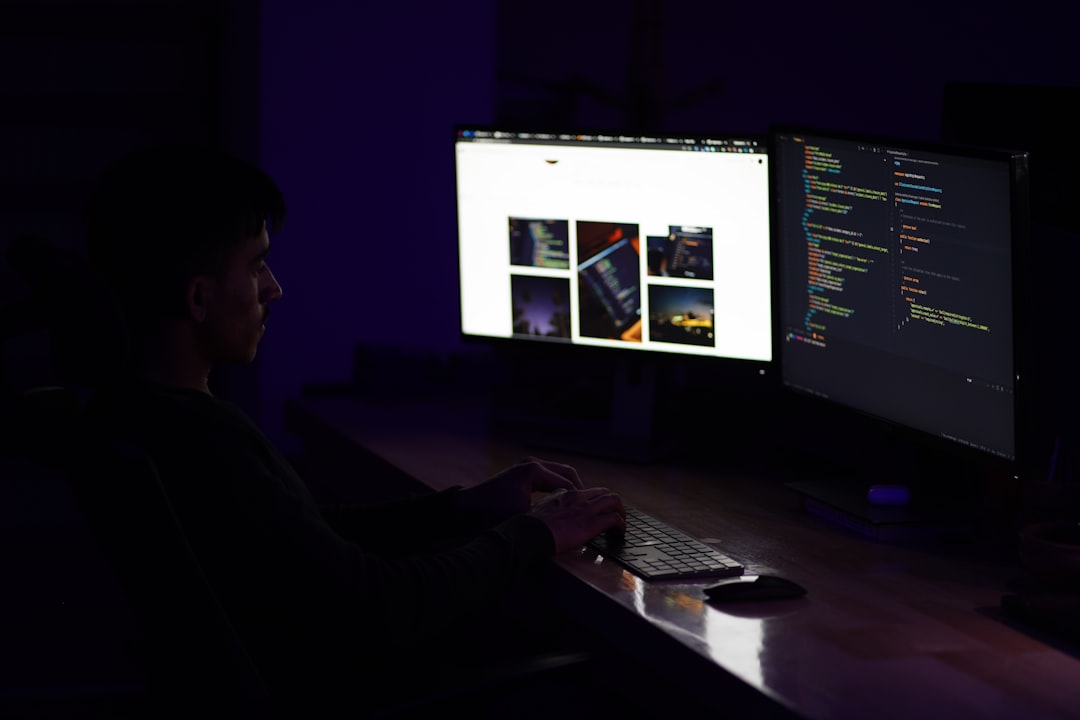
The `import` statement in Python acts as a bridge to access code from other files, allowing you to reuse functions, classes, and variables across your projects. Python's `sys.modules` plays a key role in efficiently managing these imports by keeping track of previously loaded modules, saving time and resources. It's common practice to place all import statements at the start of a file, which makes it clear which external code is being used. Understanding the difference between modules (single files) and packages (directories containing modules) is crucial when working with larger projects. While `import` is the standard method for bringing in code, Python also provides alternative ways to do so using functions like `importlib.import_module()` and `__import__()`. These functions offer greater control over the import process and can be useful in specific scenarios.
The `import` statement in Python seems simple on the surface, but it's actually a fascinating journey into Python's internal workings. It's not just about pulling in code; it's about executing that code, potentially causing side effects if it interacts with global states. The way Python searches for modules can be complex, leading to unexpected behavior if module names clash.
Python offers various import styles, each influencing scope and namespace in unique ways. `import *` is a notorious culprit for namespace pollution, making code harder to understand and maintain. Re-importing a module doesn't necessarily execute it again, leading to potential confusion during debugging. Circular dependencies can emerge when modules rely on each other, requiring careful module organization to avoid runtime errors.
The `__import__()` function offers dynamic import capabilities, particularly useful for plugins and dynamic components. Python caches imported modules, speeding up subsequent imports but making testing tricky. Even across different Python implementations, import behavior can vary due to optimization strategies, which impacts performance.
Modules control what they expose using the `__all__` list, allowing us to fine-tune import behavior for better encapsulation and code visibility. Understanding these nuances of Python's import statement is crucial for writing efficient, maintainable, and well-structured code.
Mastering Python's 'import' Statement A Deep Dive into File Importing Techniques - Exploring Different import Syntax Options
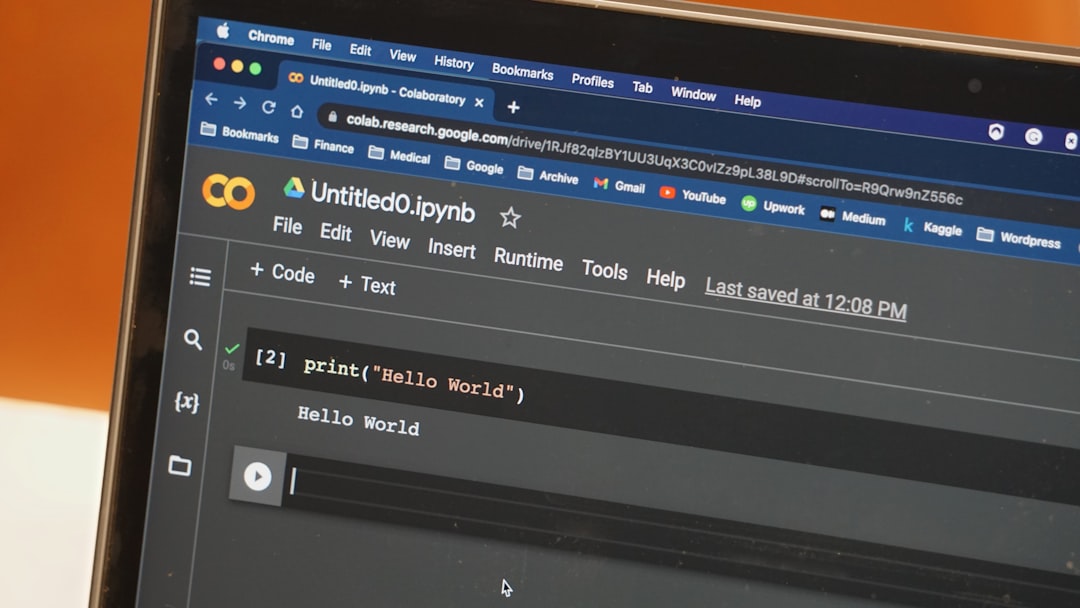
In "Exploring Different Import Syntax Options," we go deeper into the different ways Python lets you use code from other files. You can import whole modules using `import module`, but you can also bring in specific parts using `from module import item`, which keeps your code clean and organized. Using `import module as alias` creates shortcuts for long module names, making your code easier to read. It's best practice to put all your import statements at the top of your code, so everyone knows which external tools you're using and how they're organized. You'll also learn about conditional and relative imports, which are essential for building large projects without getting tangled up in dependencies.
Python's import system, while seemingly straightforward, presents a complex and dynamic world beneath the surface. The ability to dynamically import modules at runtime offers incredible flexibility for larger applications. However, this power comes with a trade-off, as it can complicate code readability and debugging efforts.
While using `import *` might seem like a convenient way to access all entities within a module, it lacks clarity and obscures dependencies. This lack of transparency can lead to messy and difficult-to-maintain code, especially in large projects.
The `import` statement goes beyond simply accessing code; it triggers the execution of the module's top-level code, potentially introducing unexpected side effects. Modules with code that interacts with global variables or performs actions during import can lead to unforeseen consequences.
Circular imports, where two modules rely on each other, can create tricky situations where modules are only partially initialized. Careful planning and structuring of module dependencies are vital to prevent runtime errors.
The `sys.modules` dictionary keeps a cache of imported modules, which can significantly improve performance by avoiding redundant loading. However, this caching mechanism can lead to surprises when modules are modified after the initial load, requiring explicit reloads to reflect those changes.
The `__all__` attribute within a module serves as a gatekeeper, controlling which entities are accessible when using `from module import *`. It allows for better encapsulation and prevents the import of unintended elements, fostering improved modular design.
Import performance can vary greatly depending on the specific Python implementation, like CPython versus PyPy. These variations in optimization strategies highlight the importance of thorough testing in the targeted environment to ensure predictable behavior.
Namespace collisions can occur when multiple modules have entities with the same name. Adopting specific import syntax, like `from module import function`, can resolve these issues and make the code cleaner and easier to maintain.
The `importlib` module provides advanced tools for managing imports programmatically, including control over relative and absolute imports. It can simplify the management of complex import scenarios.
Python uses a systematic search path to find modules, starting with built-in modules and then traversing directories listed in `sys.path`. Understanding this hierarchical search mechanism is key for resolving import errors and managing dependencies effectively.
Mastering Python's 'import' Statement A Deep Dive into File Importing Techniques - Managing Module Namespaces with import as
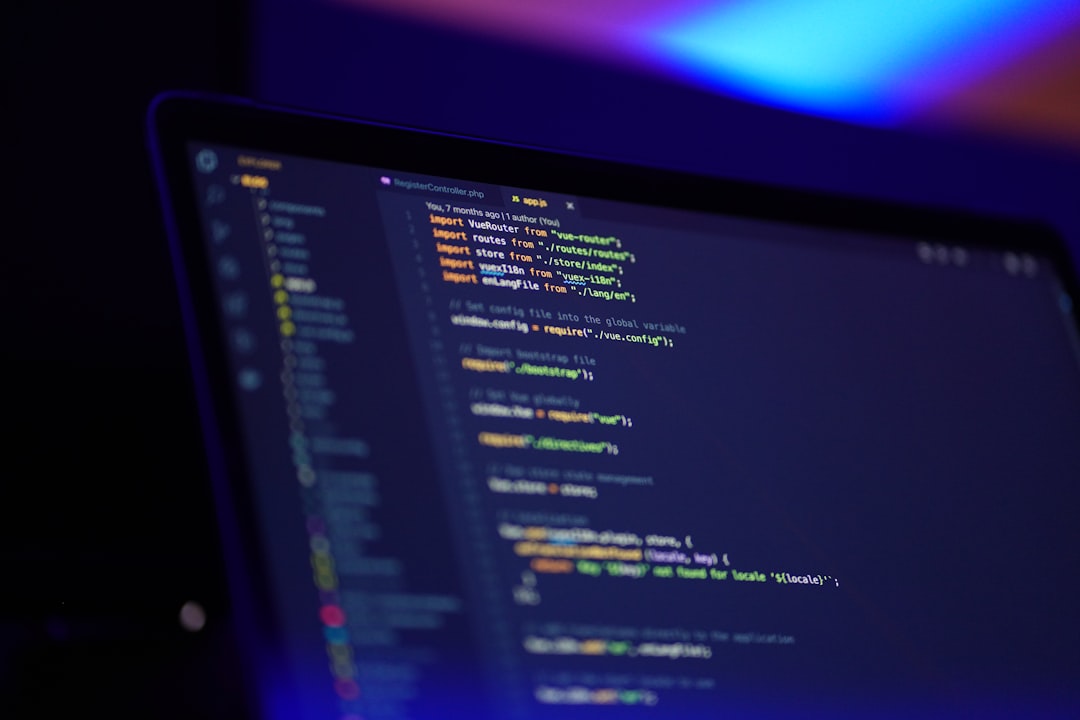
The section on "Managing Module Namespaces with import as" highlights the value of clarity and organization when working with Python's import system. Using the `import module as alias` syntax offers a neat way to rename modules as they're imported. This feature is crucial to avoid naming clashes, especially in large projects with many interacting modules. Renaming imported modules with `as` makes code easier to read, promoting better maintainability and understanding. In essence, managing module namespaces with `import as` helps ensure a clean coding environment by preventing clutter and making your code more intuitive.
Python's `import` statement might seem simple on the surface, but beneath it lies a complex world of nuanced behavior. While it allows us to bring in external code for reusability, it's crucial to understand the implications of various import techniques to write robust and maintainable code.
For instance, using `import *` to import everything from a module can lead to what's called "namespace pollution," creating confusion and potential name clashes. Python keeps track of imported modules in `sys.modules` to optimize subsequent imports, but this can lead to stale code execution if modules are modified after being initially loaded. It's important to realize that the top-level code of a module is executed upon import, which can introduce unexpected side effects if that code interacts with global state. Circular imports, where modules depend on each other, can also be tricky as modules might not be fully initialized.
Python's `__import__()` function lets you dynamically import modules based on strings, adding flexibility but making the code potentially more complex. Import performance might vary across different Python implementations due to optimization strategies, highlighting the need for comprehensive testing in the target environment.
The `__all__` attribute provides a mechanism for modules to explicitly control what they expose during imports. This helps ensure cleaner interfaces and better modular design. We can choose between relative and absolute imports, depending on the desired import path, which can be especially important in larger projects. The order of imports in a file can affect execution, making consistent ordering crucial. Python uses a structured search path to find modules, and understanding its hierarchy is key for efficient error resolution and dependency management.
By carefully considering the ramifications of various import techniques, developers can write code that is not only efficient but also clear, predictable, and easily maintained.
Mastering Python's 'import' Statement A Deep Dive into File Importing Techniques - Leveraging from import for Selective Importing
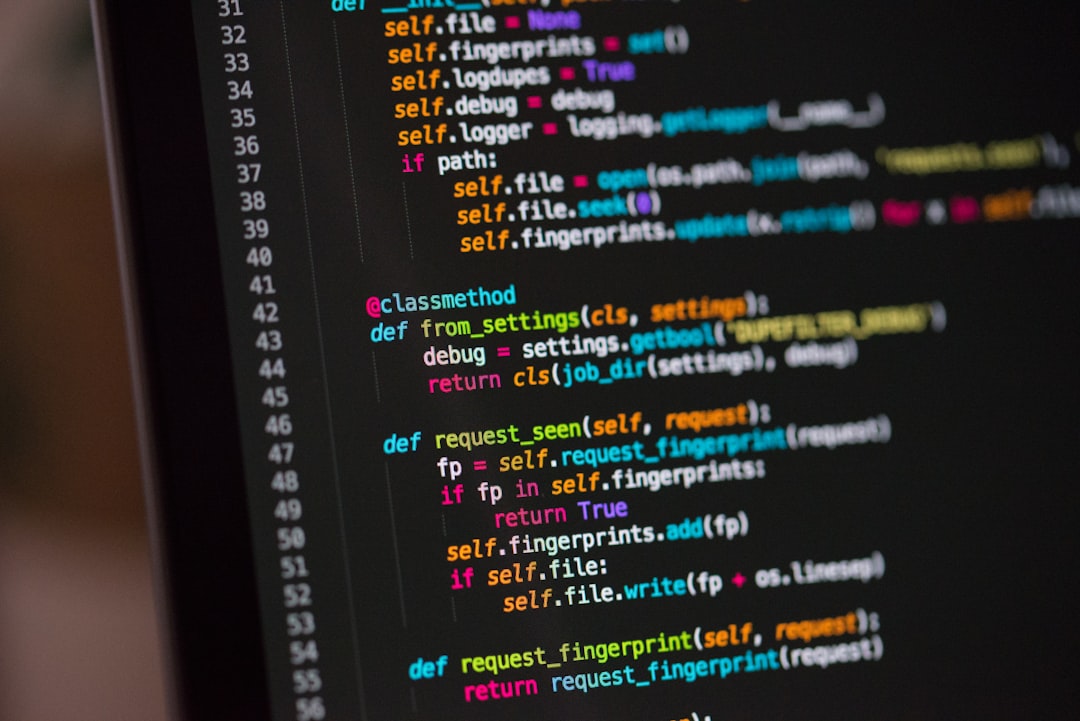
"Leveraging from import for Selective Importing" explores a smarter way to use Python's `import` statement. Instead of bringing in an entire module, you can use `from module import item` to import only the specific functions or classes you need. This keeps your code cleaner, uses less memory, and makes debugging easier. It's especially helpful for large projects where you want to be extra mindful of how much you're importing. It's not just about importing less, it's about importing more strategically.
Selective importing, achieved by using the `from module import specific_function` syntax, is a powerful technique in Python that offers several advantages. First, it allows for greater memory efficiency by only importing the necessary parts of a module, reducing unnecessary load and improving performance. Second, this approach helps maintain a clean namespace, essential for larger projects where name collisions can create confusing and hard-to-find bugs. This enhanced organization contributes to more readable code.
Python also offers the ability to import modules dynamically at runtime using the `importlib` module. This is particularly useful for applications that rely on plugin systems or runtime configuration, as it allows for greater flexibility in the program's structure.
Layered importing, using relative imports like `from . import module`, simplifies dependency management in larger applications. By specifying paths relative to the current module, this method helps streamline maintenance when restructuring project directories.
It's important to remember that each time a module is imported, its top-level code is executed. While this is standard behavior, it can lead to performance bottlenecks if the module performs intensive initialization tasks. This emphasizes the importance of using selective imports when possible to minimize overhead.
Selective importing also offers advantages for unit testing. By importing only the specific functions required for each test, developers can target smaller code sections, leading to faster feedback loops and more focused debugging efforts.
Despite various import methods, Python consistently manages imported modules through the `sys.modules` dictionary. This dictionary records all loaded modules, ensuring that subsequent imports access the cached version, eliminating redundant module execution.
The benefits of selective importing extend beyond individual modules, influencing the overall codebase. By simplifying the modules themselves, this approach contributes to a more optimized and maintainable codebase, enhancing long-term code quality.
This strategy can also help address challenges associated with circular imports. By carefully managing the order and methods of module imports, developers can prevent scenarios where mutually dependent modules are not fully initialized.
Lastly, the `__all__` attribute within a module grants developers granular control over what is exposed during import. This provides a layer of encapsulation that helps prevent unintended interference from external code, further promoting cleaner and more robust module design.
Mastering Python's 'import' Statement A Deep Dive into File Importing Techniques - Implementing Relative Imports in Package Structures
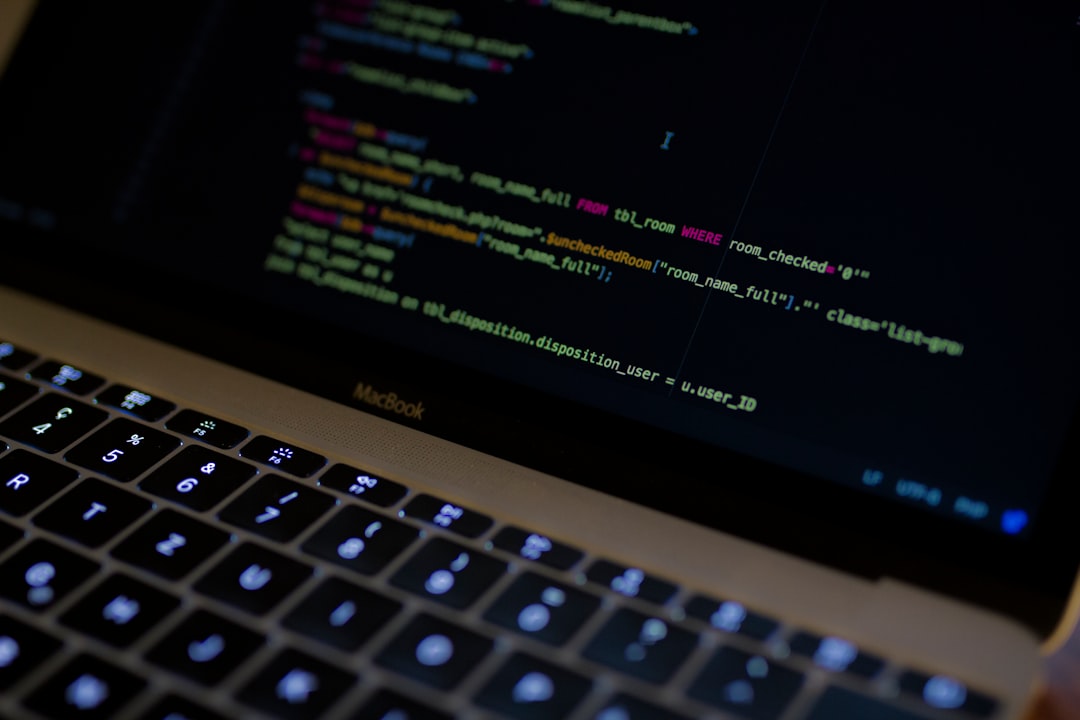
Using relative imports within your Python package structure allows you to establish relationships between modules, creating a clearer and more organized code base. The `.` notation lets you easily specify the location of a module relative to the current file, whether it's in the same directory or a parent directory.
But it's vital to remember that relative imports are only valid within a properly defined package. This means your project folder must include an `__init__.py` file, signifying it as a package. This is a common point of confusion for those transitioning from simpler scripts, as running a script with relative imports often requires you to invoke it as a module using the `python -m app.main` command to ensure the correct context.
Understanding the ins and outs of relative imports is crucial, especially as your project grows larger and its dependencies become more intricate. It's the key to maintaining a clean, efficient, and easily manageable codebase.
Relative imports, using dot notation like `from . import module`, provide a way to import modules within the context of a package, offering several benefits and potential pitfalls. This approach is particularly helpful for navigating larger projects and managing dependencies within a structured codebase.
One advantage of relative imports is the ability to access sibling modules within a package without explicitly specifying the entire package path, leading to cleaner and more maintainable code. However, relative imports introduce the possibility of circular dependencies, where two modules refer to each other, potentially resulting in partially initialized modules if not handled carefully. This risk necessitates meticulous attention to module organization and import order.
Furthermore, relative imports offer improved namespace clarity, particularly within large packages, by reducing the likelihood of name collisions, promoting cleaner and less error-prone code. But, when a module containing relative imports is executed directly, it can lead to unexpected behavior due to the lack of a clearly defined package context, often resulting in inaccurate or misinterpreted module resolution. This issue can lead developers to assume their imports are functioning correctly when they’re actually not, creating potential errors.
Despite these complications, relative imports greatly simplify package structuring, especially for large codebases. They promote a clear hierarchy and organization, streamlining project navigation and dependency management. However, using relative imports can complicate testing, as the execution context might change outside the package, often requiring modifications to import statements or testing frameworks to resolve module paths correctly.
Additionally, Python 3 encourages the use of relative imports within packages, demonstrating their importance in modern Python development. However, it's worth noting that relative imports cannot be used in script files executed as the main program, limiting their usage in some cases. While relative imports enhance organization within a package, they can also limit the reusability of modules, potentially making it difficult to extract them for standalone use in different projects due to their dependency on specific relative paths.
Understanding the benefits and drawbacks of relative imports is crucial when working with packages. Careful planning and testing are essential to effectively leverage this technique and maintain a well-structured and error-free codebase.
Mastering Python's 'import' Statement A Deep Dive into File Importing Techniques - Optimizing Code Organization with Strategic Imports
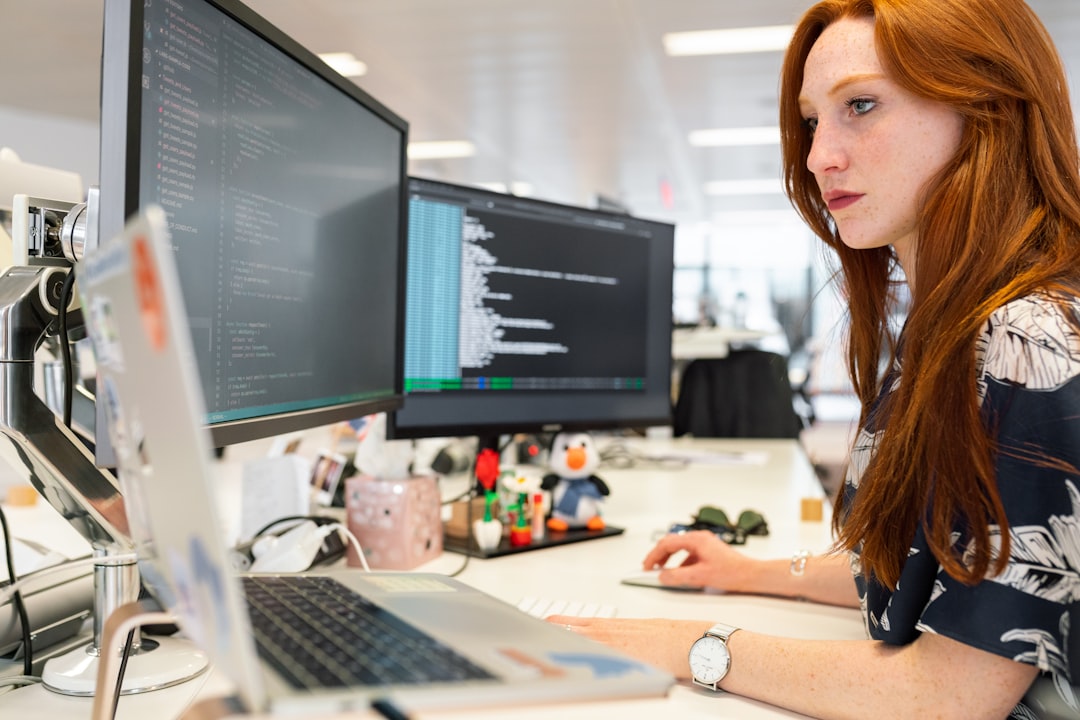
Optimizing code organization through strategic imports is crucial in Python development, enhancing readability, maintainability, and efficiency. It's not just about importing less, it's about importing more strategically. By leveraging different import techniques, you can create a more streamlined and efficient codebase. For example, selectively importing specific components with `from module import item` minimizes namespace clutter and optimizes memory usage, especially in larger projects where dependencies can become complex. The `import as` syntax provides a clear way to avoid naming conflicts and further improves code readability. As your Python projects grow, utilizing these advanced import strategies can significantly contribute to a well-structured, efficient, and easily navigable codebase.
Python's `import` statement, while appearing straightforward, reveals a complex world of optimization and organization when explored deeply. The way you structure your imports can significantly impact performance, particularly when you consider the potential for circular dependencies among your modules. Keeping namespaces clear with aliases like `import module as alias` becomes crucial as your project grows, preventing confusion and making your code more understandable.
One important consideration is that Python executes the top-level code of a module upon import, which can introduce unexpected behavior if that code interacts with global variables or performs I/O operations. The dynamic importing capabilities offered by functions like `importlib.import_module()` allow for flexibility, but can also make static analysis and code readability more challenging.
Python's caching mechanism is a double-edged sword. It's great for performance, but if a module is modified and not reloaded, it can lead to unexpected behavior during testing. While the `__all__` list allows modules to control which components are visible during wildcard imports, it also highlights the potential for subtle issues with module encapsulation.
Understanding the impact of imports on testing is vital. Relative imports can be a challenge when testing since the execution context may change outside of the package. Even with all these complexities, Python's systematic search path, starting with built-in modules and moving through directories in `sys.path`, helps us resolve import errors and manage dependencies effectively. In conclusion, strategic import techniques, alongside careful planning and testing, are critical for writing clean, efficient, and well-structured Python code.
More Posts from aitutorialmaker.com: