Mastering the Arctangent Function in C A Practical Guide to atan() and atan2()
Mastering the Arctangent Function in C A Practical Guide to atan() and atan2() - Understanding the Basics of Arctangent in C Programming
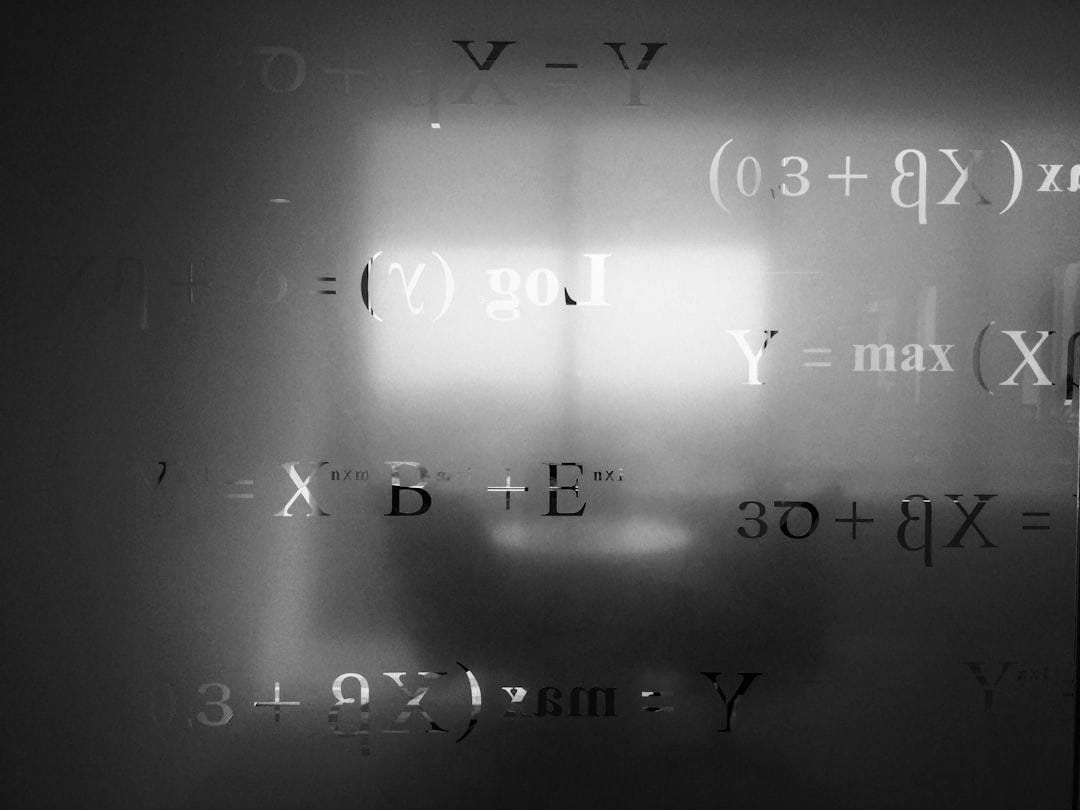
In C programming, the arctangent function is implemented by two functions: `atan` and `atan2`. `atan` computes the arctangent of a single input value, returning an angle within the range of \(-\frac{\pi}{2}\) to \(\frac{\pi}{2}\) radians. However, `atan` struggles with determining the correct quadrant of the angle based on the input value alone.
To address this limitation, `atan2` takes two arguments, `y` and `x`, representing the vertical and horizontal coordinates respectively, effectively calculating the arctangent of their quotient. This allows for a more accurate determination of the angle, considering both coordinates and returning a value within \(-\pi\) to \(\pi\) radians. By utilizing both `atan` and `atan2`, you gain a more comprehensive understanding of the arctangent function in C, enabling you to perform accurate trigonometric calculations in various applications.
The `atan()` function in C, a member of the `math.h` library, returns the angle in radians whose tangent is a given input value. This function, while straightforward in concept, presents some intriguing complexities. First, it operates on a single real number, making it less intuitive than functions like `atan2()`, which require two inputs. Secondly, its output is confined to the range -π/2 to π/2, which can lead to ambiguity when trying to determine the correct quadrant of the angle.
The function `atan2(y, x)` overcomes these issues by taking two arguments, representing the vertical and horizontal coordinates of a point in the Cartesian plane. This allows for accurate angle calculations by factoring in the signs of both inputs, effectively avoiding the quadrant ambiguity associated with `atan()`.
The behavior of `atan()` near infinity is particularly noteworthy. As x approaches positive infinity, `atan(x)` converges to π/2, while approaching negative infinity, it converges to -π/2. This asymptotic behavior is crucial to understanding how the function responds to extreme inputs.
While `atan()` and `atan2()` can be invaluable in various fields like physics and engineering, their use requires a clear grasp of their nuances. The fact that their output is in radians necessitates careful consideration when working with systems that typically use degrees. Moreover, the inherent limitations of floating-point precision can introduce errors, especially when dealing with extreme value scenarios, necessitating a cautious approach to minimize inaccuracies. Ultimately, these functions are fundamental building blocks for a plethora of mathematical algorithms and applications, highlighting their importance in various engineering and scientific endeavors.
Mastering the Arctangent Function in C A Practical Guide to atan() and atan2() - Implementing atan() Function Practical Applications
The `atan()` function in C is a valuable tool for situations where you need to calculate the angle whose tangent is a given value. You might find it helpful for navigation, determining slopes in engineering, or other applications involving angles. However, `atan()` has a limitation - it can't determine the correct quadrant of the angle based on the input alone.
That's where `atan2()` comes in. It takes two inputs - the vertical and horizontal coordinates - and calculates the angle based on the entire Cartesian coordinate system, overcoming the quadrant ambiguity of `atan()`. This makes it more versatile for situations where precise angle calculations are crucial, especially in robotics and computer graphics where movement and transformations often rely on accurate angles.
Ultimately, understanding both `atan()` and `atan2()` and knowing when to use each one is essential for anyone working with angles in C programming.
The `atan()` and `atan2()` functions are more than just mathematical curiosities - they play vital roles in various fields. Let's explore some practical applications:
1. **Polar Coordinate Conversion**: In robotics and computer graphics, these functions are instrumental in transforming Cartesian coordinates (x, y) into polar coordinates (r, θ). This conversion simplifies representing points in circular or spherical spaces, allowing for more intuitive control over movement and orientation.
2. **Angle Normalization**: Working with periodic functions often involves normalizing results to a specific range. `atan2()` proves helpful in this scenario, ensuring smooth transitions in visual representations like animation and simulation. It helps avoid discontinuities that can occur when directly manipulating angles.
3. **Complex Number Operations**: While commonly associated with real numbers, these functions hold importance in complex analysis. They are fundamental for understanding the phase of a complex number, which is pivotal in fields such as electrical engineering and signal processing.
4. **Control Systems**: In control systems engineering, the behavior of `atan()` helps interpret responses in feedback loops. It can model phase shifts and gain changes, which are critical for designing effective controllers in automation.
5. **Visualizing Terrain**: Geographic Information Systems (GIS) often use `atan2()` to calculate slopes and gradients from elevation data. This helps in terrain analysis, enabling engineers to design paths and structures that conform to the landscape's natural features.
6. **Game Mechanics**: In game development, `atan2()` frequently determines projectile angles based on their start and end positions. This ensures characters and objects hit targets accurately, enhancing the realism and engagement of gameplay.
7. **Derivative Calculations**: The derivative of the `atan()` function, \(\frac{1}{1+x^2}\), is crucial in calculus. It highlights the function's monotonically increasing nature and informs various optimization problems involving maximum or minimum value determination.
8. **Gradient Descent Techniques**: In machine learning, understanding the arctangent function contributes to gradient descent algorithms. These algorithms rely on calculating slopes or gradients toward a minimum cost function. Precision in angle calculations directly impacts convergence speed and accuracy.
9. **Digital Signal Processing**: Many signal processing applications use `atan()` for phase unwrapping processes. This technique corrects phase discontinuities in frequency-domain representations, crucial for creating clearer and more accurate signal visualizations.
10. **Historical Mathematical Context**: The arctangent function traces its origins back to ancient mathematics. While computational methods have evolved, the fundamental properties of `atan()` and `atan2()` remain deeply rooted in centuries-old mathematical concepts, highlighting how foundational math continues to influence modern engineering practices.
Mastering the Arctangent Function in C A Practical Guide to atan() and atan2() - Exploring atan2() Function Key Differences and Use Cases
The `atan2()` function in C is defined as `double atan2(double y, double x)`. It's essential for calculating angles within a two-dimensional space, taking into account both the horizontal (`x`) and vertical (`y`) coordinates. This makes it superior to `atan()`, which only takes a single input and therefore struggles to determine the correct quadrant of the angle. `atan2()` resolves this issue by using the signs of both `x` and `y` to accurately place the angle within one of the four quadrants. The function returns an angle in radians, ranging from \(-\pi\) to \(\pi\), providing a complete picture of the angle's orientation. It's particularly valuable in areas like computer graphics, robotics, and the analysis of complex numbers, where accurate angle calculations are crucial. Additionally, it's designed to handle special cases like invalid inputs, where it returns `NaN`. This makes it a reliable and versatile tool for precise trigonometric calculations in a variety of applications.
The `atan2()` function in C is a valuable tool, but understanding its nuances and how it compares to `atan()` is critical for accurate calculations. While `atan()` is limited in its ability to determine the quadrant of an angle based on a single input, `atan2()` overcomes this by incorporating two arguments, representing coordinates in a Cartesian plane.
This nuanced approach allows `atan2()` to determine the angle's quadrant precisely, producing outputs ranging from \(-\pi\) to \(\pi\). This makes it ideal for scenarios requiring accurate directional control, often seen in fields like robotics and game development, where precise movement and orientation are paramount.
`atan2()` also offers a performance advantage in certain applications. Its two-input system, while seemingly more complex, can be advantageous in real-time environments where efficiency is paramount, especially in graphics and game development, where quick and accurate calculations can greatly impact rendering speed.
Beyond its core mathematical applications, `atan2()` has a broader influence in various fields. In artificial intelligence, it plays a significant role in orientation and pathfinding algorithms, showcasing its versatility beyond standard trigonometry.
`atan2()`'s ability to handle zero values in its input also demonstrates its adaptability. When both `y` and `x` are zero, the function returns zero, highlighting a functionality not found in `atan()`. Furthermore, its utilization in complex analysis provides insight into the phase of complex numbers and their relationship to the complex plane, which is vital in understanding stability and oscillations in various systems.
`atan2()` also plays a crucial role in navigation systems. In GPS technology, it helps translate coordinate data into accurate directional angles, ensuring that users receive reliable route information even when factoring in Earth's curvature.
In machine learning, understanding the arctangent function is crucial for gradient ascent calculations. Its derivatives and how they relate to constraints are particularly relevant in optimizing models. Moreover, `atan2()`'s ability to generate smoother transitions in angles is vital in creating realistic animations and simulations. Its continuous range helps to mitigate abrupt changes in motion, which enhances the visual fidelity of virtual environments.
Furthermore, `atan2()` is a key component of physics engines used in computer simulations. It aids in accurately calculating object trajectories, ensuring that motion and collisions are represented realistically in simulations.
The arctangent function has a rich history and deep significance, dating back to ancient mathematics. Its ongoing evolution underscores its foundational nature and impact on modern advancements in engineering and technology. This testament to the longevity of basic mathematical concepts continues to shape the landscape of engineering and technology in our world.
Mastering the Arctangent Function in C A Practical Guide to atan() and atan2() - Handling Edge Cases and Error Scenarios in Arctangent Calculations
Handling edge cases and error scenarios in arctangent calculations is critical for ensuring the accuracy and reliability of mathematical computations in C programming. While `atan()` and `atan2()` are powerful tools, they can exhibit unpredictable behavior when encountering extreme inputs, like zero or infinity. These extreme cases can lead to significant variations in output, potentially introducing errors into your calculations. For example, when both inputs to `atan2()` are zero, the function returns zero, avoiding an error or undefined state. This behavior highlights the importance of understanding how these functions handle edge cases, especially in applications where precise angle determination is essential, such as robotics or game development. Carefully accounting for edge cases in arctangent calculations enhances the numerical stability of your code and ensures that your applications function seamlessly across a range of scenarios.
The `atan()` and `atan2()` functions in C, while valuable for calculating angles, come with their own set of intricacies and edge cases. These functions rely on floating-point representations, which can introduce precision errors, especially for large numbers. This means that calculations involving extreme values might yield unexpected results unless handled carefully.
One interesting aspect is the behavior of `atan2()` when both inputs are zero. It uniquely returns zero radians, whereas `atan(0)` simply returns zero, lacking the quadrant information. This underscores the value of `atan2()` in handling ambiguous inputs.
Another interesting point is `atan2()`'s ability to determine the quadrant of the angle based on the signs of both `x` and `y`. For example, `atan2(-1, -1)` produces \(-\frac{3\pi}{4}\) radians, whereas `atan(-1)` doesn't provide quadrant information. This showcases the importance of `atan2()` when working with scenarios involving multiple quadrants.
`atan2()` also behaves predictably when dealing with values close to zero. For instance, `atan2(y, 0)` (where `y` is non-zero) results in \(\frac{\pi}{2}\) or \(-\frac{\pi}{2}\), depending on the sign of `y`. This behavior is crucial to remember when optimizing calculations near the origin.
These functions gracefully handle invalid inputs. `atan2()` returns `NaN` (Not a Number) when either input is `NaN` and handles infinite values reliably. For example, `atan2(INF, x)` always produces \(\frac{\pi}{2}\). This resilience to invalid inputs makes `atan2()` a safer option compared to `atan()`.
Performance-wise, `atan2()` can save computation time in certain applications, such as rendering engines and control systems where quick and frequent angle calculations are essential.
The continuous output of `atan2()` across its domain is crucial in animations and real-time graphics, as discontinuities in angle calculations can lead to glitches or erratic behaviors in visual outputs.
Interestingly, `atan2()`'s utility extends beyond real numbers, connecting to the polar representation of complex numbers. The angle it computes represents the argument of a complex number, which is critical in signal processing and circuitry.
In GPS and navigation algorithms, the ability of `atan2()` to adjust for the signs of `x` and `y` coordinates prevents miscalculations in directional angles, particularly in complex terrains.
Lastly, `atan()`'s asymptotic descent towards \(\pm \frac{\pi}{2}\) is a fascinating characteristic of certain functions in calculus. This understanding is valuable for engineers modeling real-world phenomena, especially in physics and resource constraints where quick proximity evaluations are necessary.
Understanding these nuances and edge cases is crucial when working with these functions, ensuring accurate and efficient calculations in various engineering and scientific applications.
Mastering the Arctangent Function in C A Practical Guide to atan() and atan2() - Optimizing Performance Tips for Efficient atan() and atan2() Usage
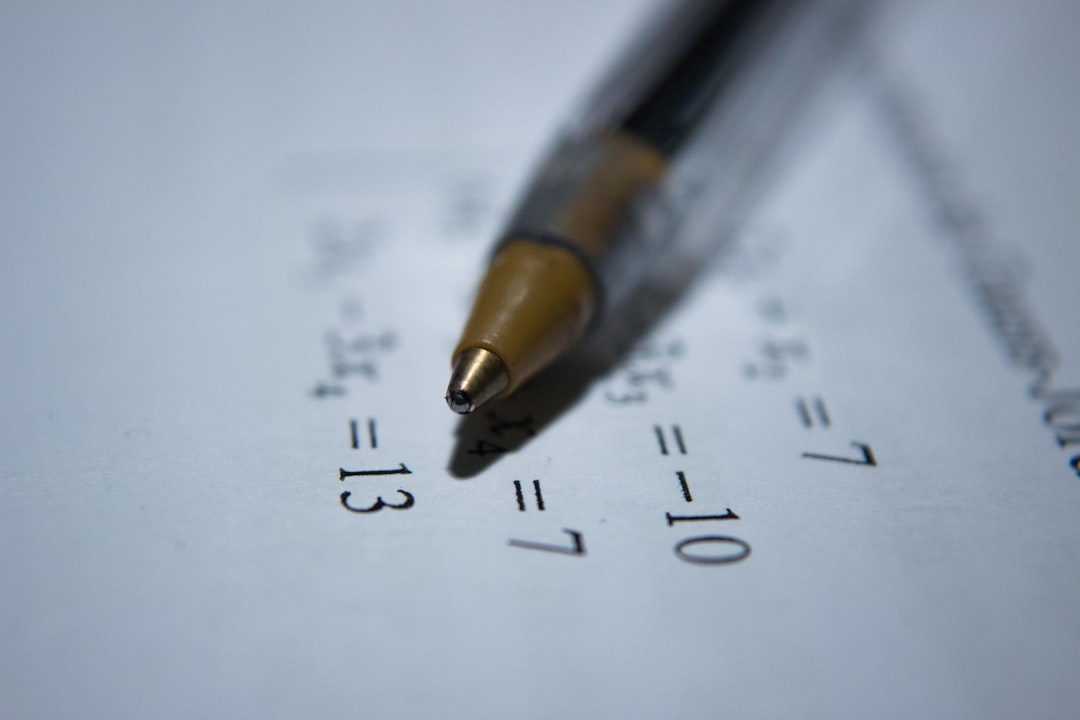
When optimizing for performance, remember that the speed of `atan()` and `atan2()` functions can vary significantly based on the specific hardware architecture. For example, on modern x86 systems, the `sqrt()` function might run faster than `atan2()` because of specialized hardware optimizations for square roots. While `atan2()` excels at determining accurate angles in all four quadrants, which is crucial in fields like gaming and robotics, it's important to consider using approximations when high-performance calculations are paramount. Approximations allow you to balance speed and accuracy, making your code run faster without sacrificing too much precision. Furthermore, always pay close attention to handling special cases such as zero inputs to `atan2()`, which returns zero. Carefully addressing these edge cases helps prevent unexpected errors and improves the overall reliability of your applications.
The `atan()` and `atan2()` functions are powerful tools for calculating angles in C, but they come with their own set of nuances and potential pitfalls. It's essential to understand these limitations to ensure accurate and reliable results in your calculations.
Firstly, `atan()` can produce significant precision errors when dealing with floating-point numbers, especially for values close to zero or very large numbers. This is due to the inherent limitations of floating-point arithmetic and underscores the importance of considering precision in engineering applications.
Secondly, `atan2()` stands out for its ability to handle cases where both input values are zero. It returns zero, providing a defined output in an otherwise ambiguous scenario. This contrasts with `atan()`, which cannot handle such cases gracefully.
The key advantage of `atan2()` is its ability to determine the quadrant of an angle based on the signs of both its input values. For instance, `atan2(1, -1)` yields \(\frac{3\pi}{4}\), demonstrating its ability to provide a more complete picture of an angle's location.
Another intriguing characteristic of `atan()` is its asymptotic behavior. As its input approaches positive infinity, it converges to \(\frac{\pi}{2}\) and to \(-\frac{\pi}{2}\) for negative infinity. This knowledge is valuable for designing systems, particularly feedback control systems, where understanding these limits can be crucial for stability.
It's helpful to remember that both `atan2()` and `atan()` are implemented in hardware-level floating-point libraries. This means their computation speed can vary depending on the chip architecture, highlighting the importance of performance considerations in time-sensitive applications like simulations.
The derivative of the `atan()` function is also essential for understanding optimization techniques, especially in machine learning. Its monotonic nature allows for guaranteed convergence in gradient ascent algorithms, which is fundamental for training models effectively.
In real-world applications, `atan2()` conditionally reflects whether its `y` input is positive or negative, which is crucial when working with polar coordinates or physical systems. This is one of the key differences between the functions, as `atan()` does not provide this additional context.
Game developers frequently utilize `atan2()` to calculate projectile angles and character orientation. Its ability to deliver precise directional information contributes significantly to the realism of interactions within virtual environments.
The continuous nature of `atan2()` is essential for real-time computer graphics as it helps mitigate issues like "jumping" or "snapping" angles due to discontinuities, which can negatively impact the user experience, especially in dynamic simulations.
Finally, `atan2()` is heavily utilized in complex analysis, where it helps determine the angle of complex numbers in the polar coordinate system. This application is crucial in fields like electrical engineering and signal processing, where phase information can significantly impact system behavior.
The `atan()` and `atan2()` functions are deeply intertwined with various engineering and scientific fields, underscoring the crucial role that a fundamental understanding of these functions plays in achieving accurate and reliable results. By carefully considering their nuances and potential pitfalls, developers can ensure that their applications function seamlessly and provide accurate insights.
Mastering the Arctangent Function in C A Practical Guide to atan() and atan2() - Real-world Examples Arctangent in Graphics and Game Development
### Real-world Examples of Arctangent in Graphics and Game Development
The arctangent function, specifically `atan2`, plays a critical role in graphics and game development, providing a way to accurately calculate angles based on two coordinates. This is particularly useful for tasks that involve spatial orientation, like character movement or aiming a weapon.
`atan2` is essential for creating realistic interactions in virtual environments. It's far more effective than basic angle functions for handling directional ambiguity. In essence, `atan2` can figure out the correct angle, even if your character or projectile is moving in a direction where you might expect unexpected behavior. This precision is essential for everything from a player smoothly navigating a 3D world to a projectile hitting its target.
Beyond its ability to calculate angles, `atan2` also handles edge cases quite well. Edge cases are situations where you might expect strange outcomes, such as when inputs are zero or approach infinity. `atan2`'s ability to work smoothly in these scenarios is vital for preventing errors and ensuring that simulations or animations run smoothly. Essentially, it allows developers to focus on creating realistic experiences rather than worrying about unexpected bugs or glitches caused by unexpected inputs. In short, `atan2` is a powerful tool that helps developers craft more accurate and believable game worlds and applications.
The arctangent function, particularly `atan2`, is more than just a mathematical tool. It's a hidden hero in graphics and game development, quietly enhancing realism and interactivity in surprising ways.
Let's take a peek behind the curtain:
1. **3D Perspective**: Think first-person shooters. You see the world through the character's eyes. How does the game know which direction to show you? `atan2` calculates the camera angles based on your movement and provides that seamless, immersive perspective.
2. **Projectile Physics**: Imagine a ball flying across a game world. `atan2` is the mastermind behind its trajectory, calculating the angle between launch point and target. This ensures that the ball lands where it should, adding realism and precision to the game physics.
3. **AI on the Move**: When an AI character navigates a world, `atan2` guides its pathfinding. It calculates the most efficient direction to reach its goal, giving AI characters a sense of purposeful movement and a more believable presence in the game.
4. **Smooth Animations**: Think about how characters move. `atan2` calculates their rotations, ensuring that their joints move in a smooth, continuous manner. This avoids the jarring "snapping" that can break immersion, making the characters feel lifelike.
5. **Collision Response**: If two objects collide in a game, `atan2` determines the angle of the impact. This information is crucial for the realistic response, such as how a ball bounces or how a character slides off a surface.
6. **Mobile UI Responsiveness**: When you tilt your phone, some games adjust their menus to match. `atan2` is behind this, interpreting the device orientation and repositioning elements accordingly, for a more user-friendly experience.
7. **Lighting the Way**: `atan2` even affects the way light interacts with objects. By calculating the angle of light incidence on surfaces, `atan2` enhances shading and textures, creating more realistic lighting effects.
8. **Climbing and Navigating Terrain**: In games with diverse environments, `atan2` helps characters climb hills and navigate tricky landscapes by computing slopes and gradients, creating more believable navigation.
9. **Contextual Actions**: When you're playing a game and your character interacts with the environment, `atan2` helps determine the direction of the action. If you're near an enemy, your character might automatically take a defensive stance, adding to the depth of the gameplay.
10. **RTS Warfare**: In Real-time Strategy games, `atan2` calculates the angle of attack for units like artillery and ranged weapons. This ensures that weapons fire at the correct targets, affecting the outcome of battles in real-time.
These examples highlight the power and versatility of the arctangent function in game development, often working in the background to create a more engaging and immersive experience. It seems that even in the digital realm, the basics of math continue to play a key role in shaping how we experience our virtual worlds.
More Posts from aitutorialmaker.com: