Understanding C System Function 7 Common Pitfalls in Command Execution and Security
Understanding C System Function 7 Common Pitfalls in Command Execution and Security - Buffer Overflow Risks in Command String Construction
When crafting command strings within C programs, a subtle yet potentially devastating risk emerges: buffer overflows related to command string construction. This arises because C, unlike some other languages, doesn't inherently check if data being written into a buffer exceeds its designated size. Functions like `strcpy`, `scanf`, and `sprintf` can easily lead to buffer overflows if developers aren't vigilant about ensuring the data doesn't overshoot the buffer's boundaries.
If a buffer overflow occurs within a global variable (static or global), it can contaminate other global variables leading to surprising and hard-to-debug program failures.
Stack-based buffer overflows, unfortunately, tend to be easier to exploit since memory layout on the stack tends to be somewhat predictable. This is a big reason why they're a primary target for attackers who might attempt to inject malicious code that can be executed when a program runs. Although modern OS's provide defenses, the risk still persists particularly on older or less updated systems.
The good news is we can take preventative measures. Employing string operations that have built-in bounds checks is a vital step. Tools for detecting potential vulnerabilities are also quite helpful, though they can be overlooked. Furthermore, developers need to be conscious of good memory management practices to avoid these problems.
It's crucial that developers not lightly undertake their own re-implementation of string manipulation functions. Without a deep understanding of the security landscape, developers may introduce unforeseen risks.
Fundamentally, having a firm grasp of how memory works in C can equip developers to better manage buffer overflow hazards. Failures in this area can easily lead to crashes and potentially worse. These vulnerabilities also offer an avenue for attackers to gain control of systems which underscores the need to pay attention to coding security.
Historically, buffer overflow attacks have had a lasting influence, driving the field of cybersecurity forward. For example, the famous 'fingerd' vulnerability within UNIX dramatically altered the direction of security precautions, and these efforts continue today. The consequences of insecure code continue to be relevant even today.
Understanding C System Function 7 Common Pitfalls in Command Execution and Security - Uncontrolled Environment Variables Leading to Path Injection
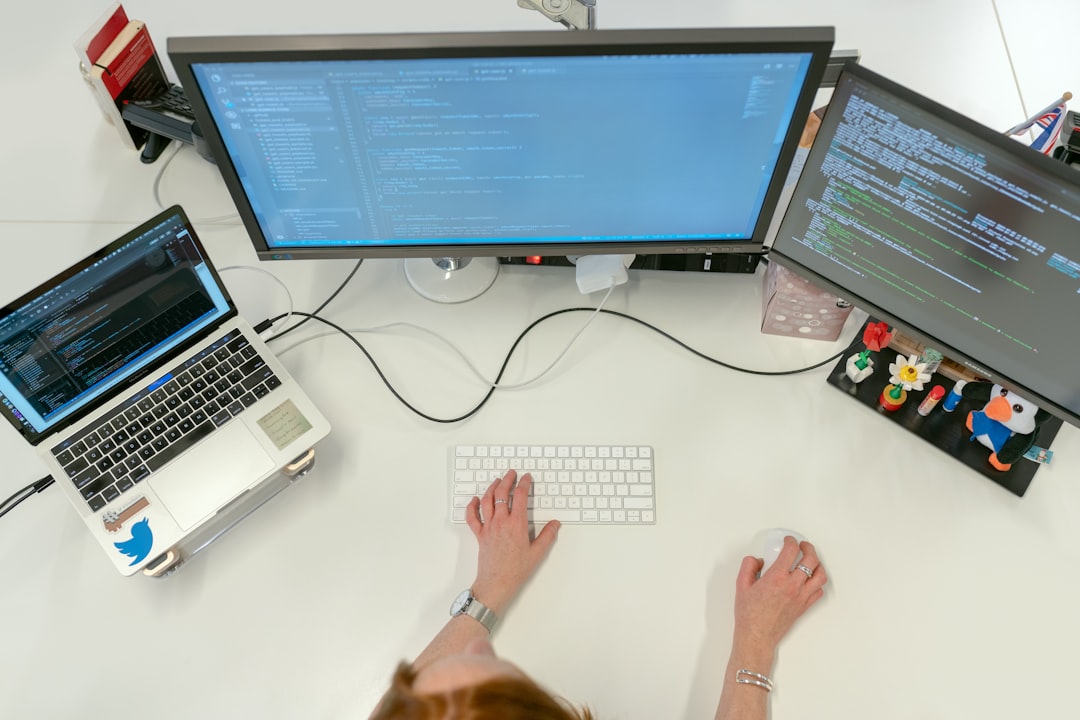
Within the realm of C programs that execute system commands, a critical security concern arises from the mishandling of environment variables. Specifically, if these variables are not properly managed, it can lead to path injection attacks. This occurs when user-provided data is carelessly incorporated into command strings or environment variables without sufficient validation or sanitization.
Attackers can exploit this weakness by manipulating the execution environment of the program. For instance, an attacker might cleverly craft input that redirects a command to an unexpected location or even executes arbitrary code, thereby compromising the application's security.
The PATH environment variable is particularly vulnerable in this context. It determines the locations the system searches for executable files, allowing users to invoke commands without specifying a full path. If an attacker can influence this variable, they might successfully redirect execution to a malicious file.
Developers must take great care to prevent this vulnerability. Prioritizing input validation and sanitization is crucial. This involves meticulously examining all user input intended for environment variables, carefully scrutinizing path separators and potentially malicious sequences. Moving away from the `system` function and implementing functions that offer more granular control over the execution environment is also advisable. By addressing these vulnerabilities and adopting a more cautious approach to environment variables, developers can enhance the security and robustness of their programs.
The `system` function in C lets programs run shell commands by using a command processor, like a shell on Unix-like systems or CMD.EXE on Windows. However, if user-provided data is used directly in command strings without proper checks, it can create command injection vulnerabilities that attackers can exploit.
There are safer alternatives for handling environment variables, like `getenv_s` and `putenv_s` in the Microsoft C library, which offer better security than their standard counterparts. Ideally, `system` should be avoided because of its inherent security risks. Functions like `execle`, `execlpe`, `execve`, or `execvpe` provide more explicit control over the environment passed to the executed command, making them more secure options.
The POSIX `popen` function is another option for running commands, establishing a pipe to read the output. This can be a better choice when you need to capture the command's output as it provides a bit more control and isolation.
When dealing with paths or environment variables, it's extremely important to clean any user input used in path expressions to prevent unauthorized access or manipulation of directories. A common mistake in command execution is embedding user input directly into command strings without proper validation or failing to understand how inherited environment variables can be manipulated by attackers. The C standard library header, `stdlib.h`, defines the `system` function. However, its use carries security risks that need careful attention and implementation of defense measures.
If user input contains path separators or certain escape sequences, developers should carefully examine and reject such data to prevent path injection vulnerabilities. Attackers often focus on the `PATH` environment variable as modifying it can lead to executables being called without specifying the full path. This highlights the crucial need for strict control over this variable to maintain security.
Environment variables are a potent attack surface, because they can potentially influence the behavior of programs in unexpected ways. An attacker might modify them to change the way applications behave to suit their malicious purposes. Furthermore, sandboxing techniques might provide some level of protection from unexpected changes to environment variables, but it does not offer a complete solution. The responsibility lies with developers to account for potential vulnerabilities within those contexts.
Unfortunately, handling environment variables and paths in C safely still requires significant care and attention due to the limitations of the libraries. Developers are tasked with writing appropriate validation checks and input sanitization. It's easy to overlook the security implications of environment variables as it's common to think of them as existing only within the confines of the user environment; however, these are vulnerabilities that attackers can exploit to gain access or elevate their privileges.
It's also crucial to thoroughly test across various environment configurations, as attackers commonly leverage unexpected pathways in order to gain unauthorized access. If left unaddressed, environment variables can enable privilege escalation allowing attackers to gain more control and access than originally intended. This makes them a significant security challenge in C and any language that interacts directly with the system environment.
Understanding C System Function 7 Common Pitfalls in Command Execution and Security - Command Injection Through Unsanitized User Input
When using the `system` function in C to execute shell commands, a critical security risk emerges if user input isn't properly cleaned and validated. Essentially, if an attacker can sneak malicious commands into the input a program expects, they can hijack the program's ability to run commands on the system. This vulnerability, known as command injection, stems from the direct incorporation of unsanitized user input into system commands.
An attacker can exploit this weakness by injecting harmful commands, potentially resulting in unauthorized code execution, data theft, or even a complete system takeover. The consequences of this security flaw can be severe, and it highlights the importance of careful input validation and sanitization when designing and building applications that rely on the `system` function.
While it's essential to ensure that users can provide meaningful input, this must be balanced with the need to prevent any form of attack. Implementing mechanisms to filter and validate inputs—for instance, restricting inputs to specific character sets or regular expressions—can help minimize these dangers. However, it's crucial to be mindful that even these measures may not be fully effective across different operating systems because systems handle commands slightly differently. Developers must fully understand these variations to prevent unexpected consequences from arising. Ultimately, taking these measures when incorporating user input into system commands is key to crafting more secure software.
Command injection vulnerabilities are a common security issue, often appearing high on vulnerability lists. Attackers can exploit these weaknesses by manipulating user input to execute unintended commands on a system. This can lead to a range of problems, from data theft to complete system compromise, emphasizing the need for careful input validation.
User input containing shell metacharacters, like `&`, `|`, and `;`, can significantly change how a command is interpreted. Attackers can use this to string together multiple commands or disrupt the intended sequence of execution. Historically, the Morris Worm demonstrated the serious implications of these flaws, highlighting the importance of secure coding practices.
Many developers mistakenly believe that functions like `system()` automatically clean user input. However, these functions simply pass the input directly to the shell, making them susceptible to injection attacks. While escaping certain characters can reduce the risk, it's not a foolproof solution as attackers may find ways to bypass those escapes in various contexts. Encoding methods can also be tricky as they can be tied to specific contexts and might not always prevent attacks.
The impact of a command injection attack depends on the privileges of the application running the command. If an application is running with elevated privileges, a successful attack can give attackers complete control over the system. Several major security breaches, such as the GitHub incident in 2015, show us the potential severity of these vulnerabilities.
To minimize the risk, using alternatives like `exec()` can be beneficial, as it avoids invoking the shell, making it harder for attackers to manipulate commands. While static code analysis tools can help uncover these vulnerabilities before code is released, unfortunately, many development teams aren't taking full advantage of this kind of tool, potentially leaving exploitable security issues in deployed code.
This understanding of command injection is crucial for building secure systems in C. The ease with which an attacker can use these vulnerabilities, even in well-established platforms like GitHub, highlights the continuing need for awareness and mitigation efforts. Carefully considered approaches to user input and command execution are critical when working with the `system` function and other functions that pass commands to the shell.
Understanding C System Function 7 Common Pitfalls in Command Execution and Security - Shell Command Portability Issues Across Operating Systems
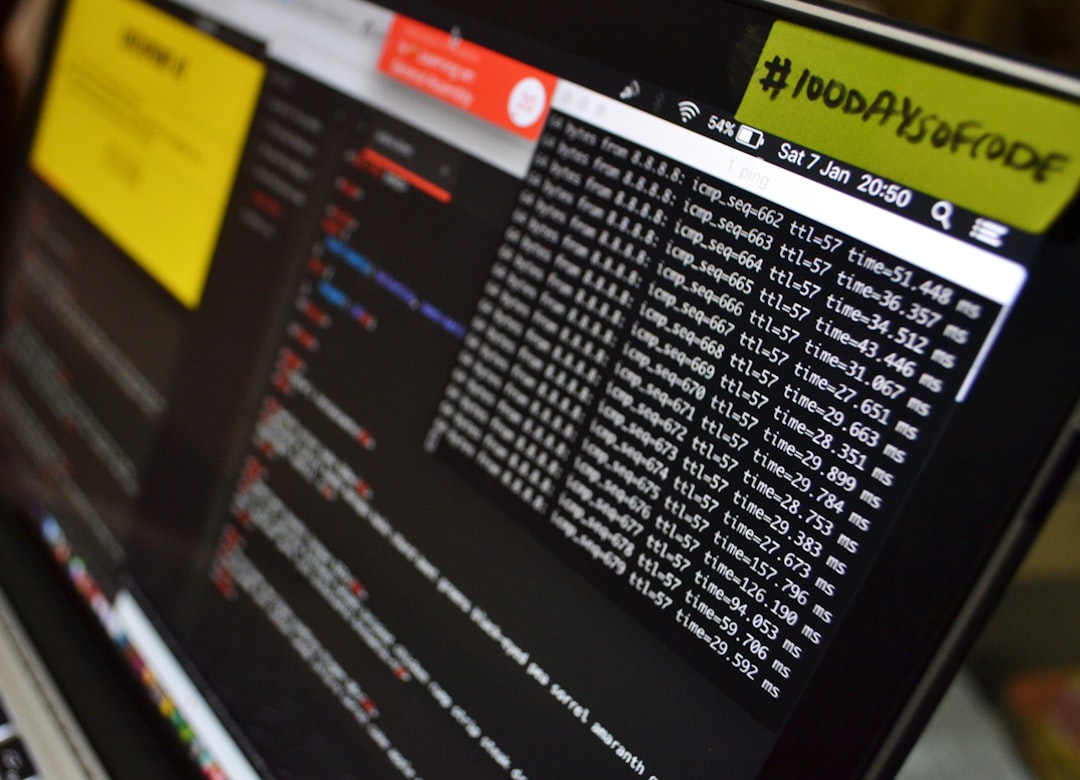
Shell commands can behave differently across operating systems due to variations in the underlying shell environments. These differences can affect how commands are interpreted, how variables are handled, and even how scripts are executed. When creating C programs that need to work on various platforms, developers have to ensure their commands function consistently, which can be tricky as the shells used by different OS's are not identical.
For example, the ways shell variables are expanded and how executable permissions are managed can vary significantly between systems like Linux, macOS, and Solaris. These discrepancies can make it more difficult to develop software that functions reliably on different operating systems. The `system()` function can also introduce security concerns, as commands executed through it can be vulnerable to attack if user inputs aren't thoroughly checked.
Because of the potential for these kinds of issues, developers should test their code on a variety of different platforms as early in the development process as possible. This allows them to discover any potential portability issues before deploying the software, potentially preventing security vulnerabilities or unexpected behavior. It's critical that developers understand how the target platforms will interact with the code and design code to account for different operating system specificities in shell command execution.
Shell commands can be a source of portability headaches when moving C programs between operating systems. One major issue is that shells themselves are different. UNIX-like systems generally use `/bin/sh`, while Windows typically uses `CMD.EXE` or PowerShell. If you don't account for these differences when writing your code, scripts can easily fail when moved between environments.
Another aspect to consider is how each OS handles environment variables. For instance, UNIX-based systems tend to be very case-sensitive when it comes to variable names, whereas Windows is more lenient. This can lead to cryptic errors if you're not careful about using consistent naming conventions in your scripts. Even something as seemingly trivial as path separators can cause issues, as UNIX systems rely on forward slashes (`/`) while Windows employs backslashes (`\`). You have to keep this in mind when working with file paths or trying to find executables across platforms.
Furthermore, the way shells interpret escape characters can also vary. A backslash (`\`) is frequently used for escaping in Unix-like shells, but Windows' Command Prompt uses a caret (`^`). This can result in confusing errors when you try to execute the same script on a different system. Similarly, built-in shell commands might not be available across platforms. For example, a command like `dir` on Windows does much the same as `ls` on a UNIX-based system, but if you try to use the wrong one, you're in for trouble.
It's also crucial to understand how user permissions and the execution environment can affect commands across operating systems. Scripts needing administrator privileges on Windows may not translate as smoothly to UNIX environments where permissions might be handled differently. Even whitespace and quoting are worth considering since different shells might have different behaviors related to these aspects.
Signal handling, a feature that gives programs a way to gracefully handle interrupts like Ctrl+C, is a mature feature in Unix-like environments, but it's less flexible in Windows. This means programs might not behave identically in terms of stability and how they respond to these kinds of events. Also, when dealing with locale-dependent settings like date formatting or regional settings, there can be variation. For example, if you're formatting dates within a script, you'll probably get different outputs based on the regional settings of the system, making it trickier to create universally compatible scripts.
In short, the portability of shell commands from C programs needs thoughtful consideration due to inherent operating system differences. If developers are not attentive to these challenges during development, they can lead to all kinds of headaches when the code needs to run across various platforms. It seems the realization that operating systems are vastly different led to attempts to directly address compatibility issues by making changes to the OS itself, which underscores how challenging the problem has historically been. These are aspects that are important to consider when dealing with system calls, and they highlight a crucial challenge for developers who write programs that interact with the operating system in these ways.
Understanding C System Function 7 Common Pitfalls in Command Execution and Security - Return Value Mishandling in System Function Calls
When using the `system` function in C to execute shell commands, a common oversight is neglecting to properly check the function's return value. This return value, a crucial piece of information, signals whether the command executed successfully or encountered an error. However, C doesn't have built-in exception handling, leaving developers with the responsibility of checking for and handling errors appropriately. If a developer doesn't check the return value, a program might continue operating even after a command has failed, leading to unexpected behavior or security risks.
For example, if a command fails due to incorrect input or insufficient privileges, the `system` function will return a non-zero value, indicating an error. Without a check for this error, the program might not be aware of the failure and could proceed as if the command succeeded. This can potentially result in security vulnerabilities, data corruption, or application crashes.
The core takeaway here is that careful attention to the return values of `system` and other system calls is crucial for maintaining program reliability and security. By consistently checking for errors indicated by these values, developers can implement robust error handling and significantly reduce the chance of vulnerabilities in their applications. It's a simple yet essential step that prevents many potential pitfalls.
The `system` function in C, defined within `stdlib.h`, lets programs execute shell commands. Understanding its return value is crucial, as it provides valuable insights into the outcome of command execution. A successful command execution typically results in a return value of 0, signifying a clean execution. However, if the passed command is `NULL`, the return value's meaning changes—it indicates whether a shell is available (non-zero) or not (zero).
The `system` function's return value actually reflects the exit status of the shell itself. Essentially, the last command executed within the shell determines the return value, unless something like Ctrl+C disrupts it. It's important to note that if the program can't create a child process or can't retrieve its status, a value of 1 is returned, and `errno` will be set with more information about the failure.
Another crucial aspect of `system` is that it pauses program execution until the executed command finishes. This behavior can be important to consider during program design. Unfortunately, C lacks built-in exception handling, making the return value the primary way to detect and address errors during command execution.
Because the return value holds vital information about command execution, proper handling of it is important for error management. However, retrieving the specific exit code of the executed command itself might require using operating system specific tools like `echo` on Unix or `echo ERRORLEVEL` on Windows.
Studying the documentation for system calls is essential for understanding how each call works and how to properly handle errors. This is an important step for developers who intend to utilize `system`. In many ways, `system` is a tool for interacting with the underlying operating system, extending the functionality of C programs to execute commands that might not have an equivalent within the C standard libraries. However, overlooking these details can leave programs vulnerable to exploitation and subtle failures.
The lack of consistent practices across different C projects can make it difficult to analyze how well the return value is handled. It's also worth noting that older code often ignores the return value, which is unfortunate because these situations can be security risks. While new tooling exists to help automatically find issues, this is not universally adopted. There's a tendency to rely on conventions that may not be widely accepted, resulting in confusion and inconsistencies in how errors are managed. All of this suggests that developers who utilize `system` must consider its nuances carefully.
Understanding C System Function 7 Common Pitfalls in Command Execution and Security - Race Conditions During Command Execution
When multiple threads or processes in a C program try to access and modify shared resources at the same time, it can create what's called a race condition during command execution. The problem with these race conditions is that the final result of the operations depends on the precise order in which things happen. This means the outcome becomes unpredictable and unreliable. Because of this, data can be corrupted, leading to inconsistencies in the program's state.
While using techniques like critical sections and locking can help control access to shared resources and minimize these issues, there are still challenges. For example, if critical sections aren't managed carefully, you can end up with a deadlock, a situation where the program gets stuck waiting for a condition that will never be met. This is a serious problem as it can freeze the application.
Race conditions become even more important to consider when a C program executes commands. Because of the way commands are handled, poorly synchronized access to shared data can produce temporary, invalid states within the system. These unexpected states can then cause a wide range of unpredictable problems within a program or even lead to security vulnerabilities.
Developers need to understand how race conditions work and implement strategies to handle concurrent access correctly. This includes thoughtful design and planning that prioritizes handling concurrency issues, as this is a common pitfall that can easily be overlooked when dealing with complex C programs that use system functions. By paying close attention to these potential hazards, developers can build safer and more reliable C programs.
### Race Conditions During Command Execution
When we're working with C and using the `system` function to run commands, a set of issues can arise that are often overlooked. It's not simply a matter of sending a command and forgetting about it. In C, how commands execute isn't always predictable, especially when multiple parts of a program or multiple processes are running at the same time. This can lead to what we call race conditions. Essentially, these race conditions occur when multiple parts of a program try to access and change shared resources like files or environment variables without coordinating with each other.
Think about the scenario where one part of a program checks if a file exists and then goes to read it, but another part of the program removes the file in between. It's a simple example, but it highlights how these race conditions can sneak up on you. This 'time-of-check to time-of-use' or TOCTOU issue is a common culprit in many security exploits, allowing malicious actors to take advantage of the unpredictable timings.
Another scenario that's noteworthy is when parts of a program use and manipulate file descriptors. File descriptors are basically numbers that represent open files. If a process opens a file but doesn't have a way to block other parts of the system from changing the file, it's vulnerable to attacks or corruption. These situations can manifest as security vulnerabilities that might not show up in testing.
Unfortunately, C doesn't provide built-in solutions like other languages to handle all this synchronously. It's left to developers to add the necessary locks and other controls. This can add complexity to the code and introduce issues if it's not managed well. The problem is that the exact order of execution is not always deterministic, which means that race conditions may not happen every time.
Environment variables can also be a breeding ground for race conditions. One part of a program might try to reset an environment variable, but before it's finished, another part of the system is trying to read it. This can lead to unintended consequences. Moreover, different shell implementations, like Bash and CMD, might behave differently when executing commands, leading to subtle differences in how race conditions play out.
Symbolic links can be exploited to cause trouble, enabling attackers to redirect command execution and potentially gain unintended access. These links basically act as pointers to other files, and if not handled carefully, can be a tool for attackers.
Finally, debugging race conditions in C can be quite challenging, as many race condition errors only appear under specific timing circumstances. This can make debugging with traditional techniques much harder. The non-deterministic nature of these issues adds another layer of complexity.
The overall message here is that race conditions can be a hidden problem that surfaces in unexpected ways in C. It's important for developers to consider them during design, implement appropriate locking and other concurrency control methods, and use specialized tooling to test and track these issues, especially within multi-process and multi-threaded environments. The problem becomes more acute when we are looking at environments that are subject to external changes, making it a crucial concern for security and robustness. While these challenges are difficult, understanding their potential presence is the first step towards mitigating them in software.
Understanding C System Function 7 Common Pitfalls in Command Execution and Security - Security Implications of Running External Programs
Utilizing external programs within C code, especially through functions like `system`, carries significant security implications. While convenient for executing commands, `system` introduces vulnerabilities like command injection, allowing attackers to potentially execute arbitrary code if user input isn't carefully managed. This emphasizes the need for thorough input validation before executing any external program. Employing functions like `execve` that bypass the shell can reduce this risk, as they provide more granular control over the execution environment.
Beyond command injection, the environment in which the program runs also presents challenges. Attackers might exploit unvalidated environment variables to influence a program's behavior, potentially leading to unwanted or malicious actions. Controlling how a program interacts with its environment is thus an important aspect of security.
It's essential for developers to prioritize security best practices, such as input validation and sanitization. Minimizing the privileges granted to programs that interact with the system can reduce the impact of attacks. Developers must be vigilant in their approach to these common pitfalls to create secure applications. While it can be difficult to mitigate all potential issues, an understanding of these common vulnerabilities is a crucial step in creating more secure C code.
More Posts from aitutorialmaker.com: