Create AI-powered tutorials effortlessly: Learn, teach, and share knowledge with our intuitive platform. (Get started for free)
Optimizing JavaScript JSON String Manipulation A Deep Dive into JSONstringify and JSONparse
Optimizing JavaScript JSON String Manipulation A Deep Dive into JSONstringify and JSONparse - Understanding JSON.stringify and JSON.parse Fundamentals
At the core of JavaScript's JSON handling capabilities lie `JSON.stringify` and `JSON.parse`. `JSON.parse` is your tool for converting JSON text, often received from external sources like APIs, into usable JavaScript objects. This transformation allows you to easily access and work with the data within the structured object format. In contrast, `JSON.stringify` takes JavaScript objects and converts them into JSON strings, suitable for storage, transmission over networks, or other scenarios where a string representation is needed.
Beyond their core functionality, both methods have specific features. For example, `JSON.parse` offers a reviver function, giving you fine-grained control over how parsed values are handled. Similarly, `JSON.stringify` demonstrates unique behavior when confronted with functions, undefined values, and symbols, excluding them from the resulting JSON.
When using these methods, it's important to be aware of potential performance impacts, especially with large datasets. Also, careful attention should be paid to error handling, particularly when parsing JSON strings. Because an improperly formatted string will lead to errors, it's a good idea to use `try...catch` blocks around your `JSON.parse` calls to handle invalid JSON gracefully. Additionally, remember that JSON is case-sensitive, ensuring property names align precisely between the JSON and JavaScript objects to avoid unexpected issues.
JSON.stringify offers a way to customize the serialization process through a replacer function, enabling the selective inclusion of object properties. This can be valuable for streamlining data or enhancing security by removing sensitive information, improving both the size and security of the resulting JSON string. Interestingly, we can also control the format of the output with a "space" parameter, which defines the indentation level, making it easier to read for debugging or logging.
JSON.parse isn't just limited to handling simple JSON objects. It can gracefully process intricate nested structures and arrays, which makes it incredibly versatile for managing real-world data scenarios. However, be mindful of circular references within your JavaScript objects, as they will unfortunately cause JSON.stringify to fail. While there are ways to work around this with structured cloning or manually resolving the loops, it's something that needs careful consideration.
There's a limitation in the JSON format itself that impacts data types—JSON cannot inherently represent certain JavaScript types such as functions, undefined, and symbols. During stringification, these types are either discarded or transformed into null, leading to potential data loss if not handled appropriately. This emphasizes the importance of considering how your JavaScript data interacts with the constraints of the JSON format. Moreover, JSON's handling of numbers isn't entirely straightforward, particularly with leading zeros. This can introduce problems during parsing, leading to errors or unexpected data interpretations, especially in scenarios like IDs or codes.
JSON's handling of BigInt values is noteworthy as well. While stringification can convert BigInt to a string format, JSON does not natively support this data type. As such, transmitting large integer values using JSON can introduce inconsistencies across different systems and needs cautious consideration. Another fascinating behavior is how JSON.stringify interacts with objects possessing a `toJSON` method. When encountered, this method is invoked, potentially altering how the object is serialized, allowing for subtle customization of the output without directly manipulating the object's internal structure.
It's crucial to remember that modifications made to the prototype of native JavaScript objects, such as Array, can impact how JSON.stringify and JSON.parse function. Unexpected serialization outcomes might result if these prototypes are modified, highlighting the importance of preserving the integrity of these fundamental objects. Finally, the performance of these JSON methods is heavily reliant on the intricacies and sheer size of the data. The overhead incurred can vary significantly, which is why benchmarking different scenarios is critical, especially for applications that work with large datasets in real time. This careful analysis can ensure optimized performance within our projects.
Optimizing JavaScript JSON String Manipulation A Deep Dive into JSONstringify and JSONparse - Performance Considerations in JSON String Manipulation
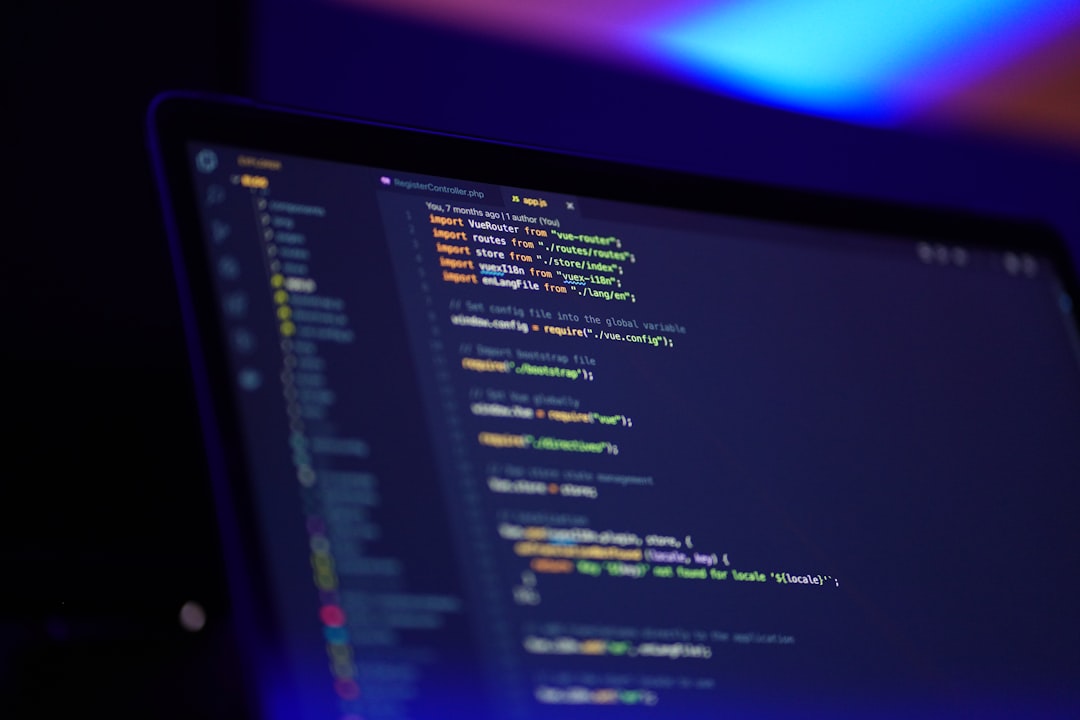
When delving into the performance aspects of manipulating JSON strings, we uncover a complex interplay between efficiency and data size. While `JSON.parse` consistently proves to be a swift method for transforming JSON text into JavaScript objects, the performance of `JSON.stringify` can become a concern, especially when dealing with larger JSON structures. This highlights the need to carefully consider the impact of data size on the choice of manipulation methods.
One of the key reasons for potential performance bottlenecks lies in the nature of JSON itself. Being text-based, it necessitates extensive string operations, which can be less efficient compared to handling binary data. This further underscores the importance of exploring alternative approaches to processing JSON. Leveraging streaming techniques for parsing can provide a substantial improvement, allowing libraries to process data directly from a stream rather than parsing a complete string in memory.
Furthermore, optimizing the surrounding JavaScript code and grasping its execution flow can lead to significant performance gains. Techniques like compression can reduce JSON payload sizes considerably, thereby enhancing transmission speed. However, it's imperative to balance the need for speed with the importance of maintaining data integrity and security to create robust applications that handle JSON effectively. The inherent blocking behavior of JavaScript's parsing mechanism, if not properly managed, can also delay the rendering of HTML, which is something to consider within the bigger picture of how your web app will function.
JSON manipulation in JavaScript, while seemingly straightforward, presents several performance considerations that are important to understand. The efficiency of `JSON.stringify` and `JSON.parse` isn't constant. The size of the data being processed has a substantial impact on performance, with larger datasets exacerbating even minor inefficiencies. For instance, dealing with circular references in objects can cause `JSON.stringify` to stumble since it doesn't inherently handle those cases. Workarounds, like custom serialization strategies, are required.
Moreover, JSON's reliance on UTF-8 encoding for character representation can cause complications when handling data that contains non-standard characters. If encoding isn't carefully managed, it could result in corrupted data or unexpected errors. The depth of object structures within JSON data is another factor. Deeply nested structures require more traversal, causing delays in parsing and stringification.
JSON's reliance on double-precision floating-point numbers can sometimes introduce precision issues, especially when working with large integers. This limitation is rooted in the IEEE 754 standard that JSON adheres to. The replacer function in `JSON.stringify`, though useful for selective property inclusion, can negatively impact readability and maintenance if used excessively.
The `toJSON` method can lead to subtle alterations in the serialization process. While this customization can be helpful, it also introduces unpredictability if not well-documented and understood, particularly within team-based development settings. Similarly, tampering with native JavaScript prototypes like those found in `Array` or `Object` can create unforeseen behaviors in JSON methods. These modifications can lead to unpredictable serialization results, which is why maintaining the integrity of these fundamental objects is crucial.
When parsing large JSON strings, it's important to anticipate memory consumption spikes. This increased memory demand can pose challenges in resource-constrained environments, leading to slower processing or even application crashes. While JSON offers advantages in data exchange, its role in network communications isn't immune to network latency. Simply put, network latency can affect JSON's performance in real-time applications, even for relatively small JSON payloads. Optimization measures, such as compressing JSON text before transmission, can help mitigate this issue.
It's important to strike a balance between the benefits and potential drawbacks of various JSON manipulation techniques. Careful consideration of these factors during the development process ensures a more robust and efficient implementation, improving the overall performance of web applications that rely heavily on JSON data.
Optimizing JavaScript JSON String Manipulation A Deep Dive into JSONstringify and JSONparse - Deep Cloning Objects Using JSON Methods
Deep cloning objects in JavaScript means creating a completely independent copy of an object, including all its nested objects and properties. A common way to achieve deep cloning is through the synergy of `JSON.stringify` and `JSON.parse`. Essentially, you convert the object into a JSON string and then reconstruct it back into an object. This seemingly simple technique, however, has limitations. It cannot handle functions, undefined values, or cyclical object references within the original object. In these scenarios, the cloning process may unintentionally discard or modify these elements, leading to discrepancies between the original and the cloned object.
While the JSON methods are quick and easy for simple objects, more complex or specific cases may benefit from alternative methods. Features like `structuredClone` (if available) or specialized deep cloning functions from libraries like Lodash offer more comprehensive cloning capabilities that address some of the shortcomings of using the JSON approach. These advanced tools tend to be better suited for scenarios where a full and faithful copy is required. When choosing between these different cloning strategies, it's crucial to assess factors like the complexity of your object, whether functions or specific values need to be cloned, and the performance demands of the operation within your project. Ultimately, selecting the right method depends on balancing convenience and the need for thorough cloning across various data types.
Deep cloning objects using JSON methods, while seemingly simple, has several quirks that need careful attention. One major hurdle is the handling of circular references. If an object has properties that point back to itself or other parts of the object structure in a loop, `JSON.stringify` will crash with a `TypeError`. This means we need to come up with specific strategies to deal with objects that have this complex type of interlinking.
Another factor to consider is performance. As the objects we try to clone become bigger, `JSON.stringify` can start slowing down drastically. This performance degradation can be dramatic, especially with deeply nested or very large structures, because of the sheer amount of text processing that needs to happen. So, it's always good practice to understand how big your data is before deciding if JSON methods are suitable for deep cloning.
Another issue we see with using these methods for deep cloning is the loss of certain data types. When the object is converted to a string using `JSON.stringify`, things like functions, `undefined`, and `Infinity` often get discarded. This can lead to unexpected behavior in the cloned object if we were planning on the original object's functions or properties to still be there after the cloning process.
Even numbers can cause problems, especially large ones. Because JSON's numerical representation follows the IEEE 754 standard, large numbers can sometimes lose precision. If we're dealing with large integer IDs, for example, there's a risk of getting an inaccurate clone.
Additionally, `JSON.stringify` handles special characters in a specific way. Characters like newline characters need to be properly escaped, otherwise, our JSON string might get corrupted and parsing will fail. This is especially important to keep in mind when working with user-generated content or data that might contain less standard character sets.
If the objects we're working with have a `toJSON` method, then things get a bit more complex. These methods can alter how the objects are turned into JSON strings, which can make it less predictable how the output will look. This behavior needs careful documentation and management if we're collaborating on a project.
Another aspect to keep in mind is memory usage. If the object is big, the process of converting it to a string and then back to an object with `JSON.parse` can use up a lot of memory, especially in systems with limited resources. This potential memory pressure is important to keep in mind to prevent application crashes or slowdowns.
The depth of the object's structure can also affect performance. The more deeply nested an object is, the longer `JSON.stringify` and `JSON.parse` will take to process it. If we're building a system where quick responses are critical, like in real-time applications, we might need to be mindful of how nested our data structures are to prevent delays.
JSON's use of UTF-8 for encoding can sometimes be a source of problems with characters that aren't in the standard ASCII set. If we don't manage character encoding properly, it can lead to corrupted data or errors during parsing, so being careful and proactive in how we handle these issues is essential.
Lastly, changes to the prototypes of built-in JavaScript objects like `Object` and `Array` can affect how `JSON.stringify` and `JSON.parse` behave. These changes can introduce unpredictable and unintended results. Therefore, it is a good practice to avoid changing these prototypes unless absolutely needed to avoid impacting how these methods operate.
In essence, using JSON methods for deep cloning can be efficient in many cases, but it's vital to be aware of these potential drawbacks, especially when working with large, complex, or unusual data structures. Careful consideration and testing are crucial to ensure the stability and correctness of our JavaScript code.
Optimizing JavaScript JSON String Manipulation A Deep Dive into JSONstringify and JSONparse - Limitations and Edge Cases of JSON-based Operations
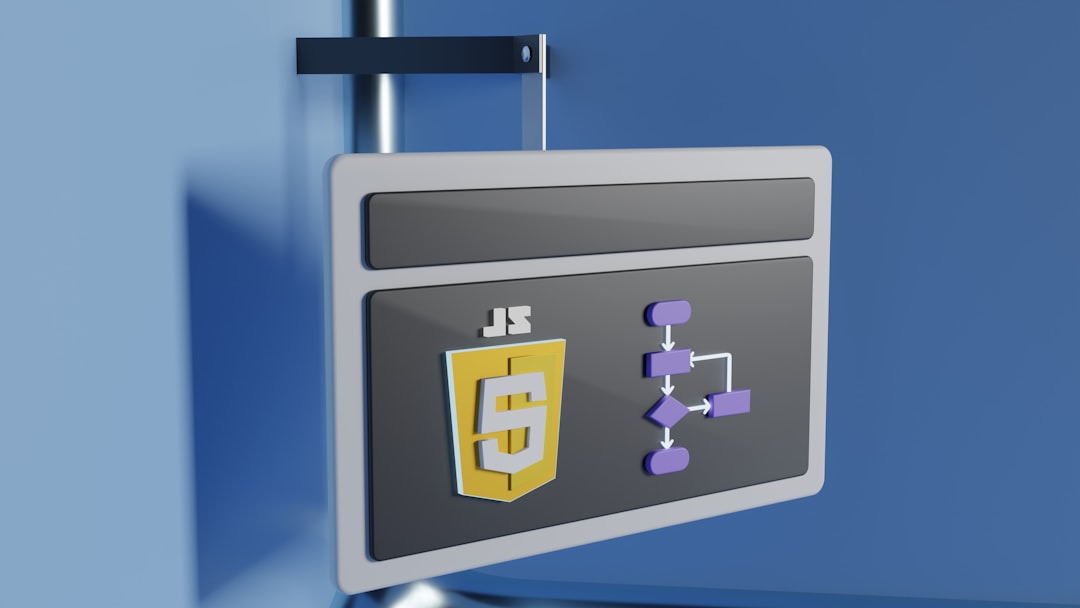
When working with JSON in JavaScript, it's important to be aware of its limitations and how they might affect your code. One key limitation is that `JSON.stringify` can't handle certain JavaScript data types like functions or undefined values. These elements are simply skipped during the conversion to a JSON string. Additionally, if you try to stringify an object that contains circular references—where parts of the object point back to itself or other parts in a loop—you'll get a TypeError. This means you'll need to find ways to address these situations if your data structures have such complex interconnections.
Another factor to consider is that JSON's reliance on string manipulation for operations like parsing and concatenation can lead to performance issues, especially when dealing with large amounts of data or complicated structures. The text-based nature of JSON might make it less efficient compared to other ways of handling data. This suggests that exploring alternative approaches might be beneficial, particularly if you're working with huge datasets or need to process data quickly.
Moreover, modifying how standard JavaScript objects like `Array` or `Object` behave can unexpectedly influence how `JSON.stringify` and `JSON.parse` work. These changes to the underlying prototypes can lead to unpredictable outcomes, making it crucial to avoid making modifications to these core elements of JavaScript unless absolutely necessary. Keeping the integrity of the built-in prototypes ensures that these foundational elements behave as expected. These are just a few of the points to keep in mind as you develop with JSON-based applications.
### Limitations and Edge Cases of JSON-based Operations
1. **Circular Structures Cause Trouble**: If you try to convert an object with circular references (where parts of the object point back to themselves or other parts in a loop) to a JSON string using `JSON.stringify`, it'll throw a `TypeError`. This limitation means we need to find alternative ways to serialize data that contains this kind of interconnectedness if we want to use JSON.
2. **Big Numbers, Little Precision**: JSON uses the IEEE 754 standard to represent numbers. This can cause a loss of precision when dealing with extremely large integers. So, if you're storing things like unique IDs that are very large numbers, you need to be aware that you might lose some accuracy when using `JSON.stringify` and `JSON.parse`.
3. **Not All JavaScript Data Types Are Welcome**: JSON can't handle certain JavaScript data types natively, including functions, `undefined` values, and symbols. When these types are encountered during stringification, they're either ignored or converted to `null`, which could mean losing important data if you're not careful.
4. **Deeply Nested Objects Can Slow Things Down**: The more deeply nested your objects are, the slower `JSON.stringify` and `JSON.parse` will become. A lot of traversing through levels of objects is required which can lead to performance bottlenecks and potential memory issues.
5. **Characters Outside of ASCII Can Be Tricky**: JSON relies on UTF-8 encoding, which can create complications if you have characters in your data that aren't standard ASCII. If encoding isn't carefully managed, you could end up with corrupted data or unexpected errors during parsing. This is especially something to pay attention to when dealing with user-generated data, since it can include all sorts of characters.
6. **Don't Mess with the Prototypes**: Modifying the default behavior of built-in JavaScript objects like `Array` or `Object` (their prototypes) can cause `JSON.stringify` and `JSON.parse` to behave unexpectedly. These changes can disrupt how data is converted, highlighting the importance of not altering these core parts of JavaScript unless strictly needed.
7. **`toJSON` Methods Can Change Things**: Objects that have a `toJSON` method can lead to unexpected results when converting them to JSON. These methods give you the power to control how an object is stringified but can also lead to confusion if not documented properly, particularly in projects where multiple people are working on the code.
8. **Memory Can Be a Constraint**: Deep cloning with JSON can lead to memory usage spikes, especially if the object you're cloning is very large. This can cause issues, especially on systems with limited resources, potentially leading to slowdowns or even crashes.
9. **Handle Parsing Errors Gracefully**: If `JSON.parse` encounters an improperly formatted string, it can throw an error. You should use `try...catch` blocks to gracefully handle invalid JSON and prevent your program from crashing unexpectedly.
10. **Differences Between Systems Can Be Problematic**: JSON doesn't inherently support the `BigInt` data type, which is used to represent large integers. If you're transferring data containing `BigInt` values between different systems, you might encounter problems with loss of information or inconsistent behavior, as some systems might not support `BigInt` in the same way.
Optimizing JavaScript JSON String Manipulation A Deep Dive into JSONstringify and JSONparse - Optimizing JSON Processing for Large Datasets
When dealing with substantial JSON datasets in JavaScript, optimization becomes crucial for maintaining efficient application performance. Since JSON is inherently text-based, processing large amounts can lead to performance issues, especially compared to binary data. To counter this, strategies such as breaking down the JSON into smaller chunks or "processing in pieces" can be vital for improved responsiveness and manageable resource utilization. Stream-based parsing, where possible, provides another efficiency boost by allowing libraries to read data directly from a stream, avoiding the need to load the entire file into memory. Utilizing compression techniques like Brotli or Gzip during data transfer can drastically reduce file size, enhancing overall network performance.
Additionally, meticulous care must be taken in managing JavaScript objects, as a single inefficient operation can significantly hinder your application's responsiveness, particularly when manipulating large JSON datasets. Addressing issues like circular references within objects, which can negatively impact performance, is an important optimization area. Understanding and mastering JavaScript's JSON serialization abilities, particularly `JSON.stringify` and `JSON.parse`, becomes paramount for optimizing data transfer and storage within your applications. By carefully considering these techniques and best practices, you can build more robust and efficient applications that effectively handle large JSON datasets.
When working with sizable JSON datasets, we encounter various performance considerations that can significantly impact our applications. One prominent issue is the potential for memory usage to surge during `JSON.parse`. This can be particularly problematic on systems with limited resources, potentially causing sluggish performance or, in the worst cases, application failures. To mitigate this, it's wise to consider techniques for managing memory usage, especially if dealing with very large JSON files.
Another point to bear in mind is how JSON handles characters outside of the basic ASCII set. Since JSON adheres to UTF-8 encoding, any non-standard characters might lead to parsing errors or corrupted data. This is especially crucial to be aware of when interacting with user-input, which is often unpredictable in nature. To avoid problems here, careful encoding and decoding of JSON strings is recommended.
Moreover, the presence of a `toJSON` method on an object can introduce an element of unpredictability in how `JSON.stringify` converts the object to a string. This can change the serialization process, potentially resulting in unexpected output formats. It's generally wise to understand and thoroughly document these methods if you're working in a team or with codebases that change frequently.
When dealing with complex object structures, it's also essential to recognize the difficulties that arise when circular references are present. If an object has properties that point back to itself or other parts of the structure, `JSON.stringify` will abruptly halt with an error. This limitation demands careful design to avoid situations where such relationships are inherent in the data being worked with.
Working with very large integer values can present some precision challenges. JSON relies on the IEEE 754 standard for its numerical representation, which can introduce rounding or truncation errors when dealing with exceedingly large numbers. This has the potential to introduce inaccuracies or inconsistencies if, for instance, we are using large integers for unique identifiers, and this needs to be acknowledged when designing applications that need extremely high precision or work with sensitive financial data.
Modifications to JavaScript's fundamental object prototypes—such as `Array` and `Object`—can lead to unexpected behavior in JSON handling. These alterations can impact how objects are serialized or parsed, leading to results that may be difficult to predict or debug. Consequently, it's generally best to avoid tampering with these core aspects of JavaScript unless absolutely necessary.
The depth of nesting within our JSON objects can heavily influence the speed of both `JSON.stringify` and `JSON.parse`. The deeper the nesting, the more traversal that's needed to move through the data. This can quickly lead to performance bottlenecks and even memory consumption issues. When building applications that demand speed, optimizing for the structure of your JSON data can improve the user experience.
During serialization, `JSON.stringify` can't directly handle certain data types intrinsic to JavaScript, such as functions and `undefined`. This results in the loss of those pieces of data. It's important to acknowledge this limitation and make sure that any data you need to keep is preserved before JSON stringification.
The parsing process can be interrupted by improperly formatted JSON input, causing parsing errors. This often throws an error and stops the application. When dealing with external data or user-generated content, it's crucial to anticipate the possibility of invalid JSON strings. To ensure your application doesn't crash unexpectedly, it's recommended to implement `try...catch` blocks to gracefully handle these errors.
Lastly, inconsistencies can arise when JSON is used for communication between different systems or environments. This is particularly relevant for the `BigInt` data type, which is not universally supported within JSON. If you are working with data containing `BigInt` values, you need to be conscious of potential compatibility issues.
In conclusion, while JSON is a widely adopted and convenient data format, understanding its inherent limitations and optimizing how it's handled is vital for building robust, high-performance JavaScript applications. By keeping these points in mind, we can design applications that work more effectively with large JSON datasets, minimize issues, and ultimately, improve the user experience.
Optimizing JavaScript JSON String Manipulation A Deep Dive into JSONstringify and JSONparse - Alternatives to JSON.stringify and JSON.parse for Object Manipulation
### Alternatives to JSON.stringify and JSON.parse for Object Manipulation
`JSON.stringify` and `JSON.parse` are the standard tools for working with JSON in JavaScript, but they have limits, especially when dealing with complex data. For example, they can't handle circular references, which can cause problems when an object's properties refer back to itself or other parts of the structure. They also don't work well with `undefined` values and some JavaScript data types, sometimes causing data to be lost during the process of converting to a JSON string.
To improve upon these limitations, we have alternative methods. Libraries dedicated to object serialization can provide more advanced functionality, like properly managing the process of converting functions to strings or enabling deep object cloning. Techniques like structured cloning or the use of specialized serialization libraries can often be faster and more adaptable, especially when you're working with large amounts of data or objects that are intricately linked. As developers continue to refine their work, exploring these alternatives can create more effective JSON handling practices, ultimately enhancing the overall efficiency of applications.
While `JSON.stringify` and `JSON.parse` are the foundational tools for handling JSON in JavaScript, they do have limitations. For instance, they struggle with complex scenarios like handling circular references or preserving data types like `Date` or `Map`. This naturally leads us to explore alternatives that might be better suited for certain situations.
One fascinating alternative is the `structuredClone` API, which offers a modern approach to cloning complex objects. It can even deal with those tricky circular references, unlike the JSON methods. Furthermore, when working with numerical data, typed arrays can potentially deliver a significant performance boost. These arrays can store binary data more efficiently, allowing operations to act directly on memory instead of on the string representation.
The world of serialization goes beyond JSON, with MessagePack presenting a compelling alternative. This binary format packs data in a more compact way, which is incredibly useful for large datasets or resource-constrained environments. We see libraries like `BSON` (Binary JSON) and `Protobuf` (Protocol Buffers) emerging as another set of tools designed for serialization with performance and data type preservation in mind. These libraries are especially relevant if we need to work with data types that JSON doesn't natively support.
If we're aiming for a simpler deep copy, we can leverage `Object.assign`. This technique creates a shallow copy and is typically faster and more straightforward than relying on the `JSON.stringify` and `JSON.parse` combination. In fact, we can explore writing our own serialization functions to handle complex data types in a more nuanced way, giving us much finer-grained control.
Validation before parsing can be a valuable step toward robustness. Libraries like `Joi` or `Yup` can prevent the unexpected errors that arise from invalid JSON, enhancing the stability of our applications. Moreover, data structures from the Immutable.js library offer ways to work with data while maintaining immutability. This is a different approach that could open up a variety of potential performance optimizations. And, of course, optimizing network communications is always a priority. Utilizing compression with Gzip or Brotli can substantially reduce the size of our JSON payloads, which leads to quicker load times for users.
Finally, ES6+ features like arrow functions and the spread syntax help with code readability and make it easier to integrate these alternative techniques into projects. While JSON methods provide the bedrock for working with JSON, keeping an open mind to these alternatives can be beneficial for certain tasks and contexts within our coding endeavours.
Exploring these alternatives provides a broader understanding of the JavaScript ecosystem and equips us with a diverse toolkit for tackling a wider range of challenges when working with JSON data.
Create AI-powered tutorials effortlessly: Learn, teach, and share knowledge with our intuitive platform. (Get started for free)
More Posts from aitutorialmaker.com: