Create AI-powered tutorials effortlessly: Learn, teach, and share knowledge with our intuitive platform. (Get started for free)
Mastering Python's Dynamic Variable Declaration A Deep Dive for AI Developers
Mastering Python's Dynamic Variable Declaration A Deep Dive for AI Developers - Understanding Python's Memory Allocation for Variables
Python's management of variable memory relies on a sophisticated system, including garbage collection, to automate the creation and disposal of objects. This contrasts with languages where variables directly store values. In Python, variables act as pointers to objects stored elsewhere in memory. This design optimizes memory by allowing multiple variables to reference a single object if they share the same value. The system's dynamic nature adapts to the particular type and size of the object being created, further refining memory usage. Importantly, Python's garbage collector actively manages cyclical references, preventing memory leaks by automatically cleaning up unreachable objects. This behind-the-scenes memory management is especially relevant for AI developers who frequently work with complex and large models, requiring both efficient code and memory usage. A thorough understanding of how Python allocates and manages memory is crucial for writing optimal code, especially within the demanding world of AI development.
Python's memory management relies on a system called reference counting, where each object keeps track of how many variables point to it. When this count hits zero, the memory is freed up. This mechanism is crucial for avoiding memory leaks, a common issue in other languages. However, Python's dynamic nature allows variables to change types on the fly, a feature that contrasts with statically-typed languages. This flexibility, though convenient, can impact performance due to the added overhead of type checks and garbage collection routines.
Python's memory layout often involves a header for every object, containing information like type and reference count. This structure adds overhead, especially when dealing with smaller objects. Furthermore, Python maintains a private heap, a dedicated memory space for dynamically allocated objects, which helps ensure safety and prevents direct user access. While this approach bolsters security and integrity, it can complicate optimizing memory usage.
Python cleverly balances speed and versatility in memory allocation by choosing between the stack and the heap for variables, based on their lifespan. Stack-allocated variables, designed for shorter-lived values, tend to be faster due to their simpler management. Heap-allocated variables, on the other hand, provide greater flexibility but come at the expense of speed.
Interestingly, Python includes caches for common small integers and strings. This approach can drastically improve performance by avoiding repeated allocations and deallocations for these frequently used values.
Circular references present a challenge for Python's reference counting. The system can struggle to identify objects no longer needed if they are linked in a cycle. While Python incorporates a cycle detector in its garbage collection, this mechanism adds complexity and can pose difficulties when managing memory efficiently.
The Python interpreter, with its extensive features and libraries, requires a substantial amount of memory for its own operation. This can lead to higher resource consumption compared to more streamlined languages, demanding mindful consideration when using Python in resource-constrained environments.
One of the consequences of Python's dynamic typing is that memory reallocation becomes necessary whenever a variable's type changes. This constant reallocation can fragment memory over time, leading to performance degradation as the system searches for available, contiguous chunks of memory.
Mutable and immutable objects have a profound influence on memory management in Python. Modifying mutable objects can often occur in place, avoiding the need for new memory allocation. Conversely, immutable objects, which cannot be changed, require the creation of a new copy whenever modified, potentially increasing memory consumption and slowing down performance if not carefully managed. Understanding these dynamics is essential for optimizing Python code, particularly for AI applications that often grapple with massive datasets and complex computations.
Mastering Python's Dynamic Variable Declaration A Deep Dive for AI Developers - Enhancing AI Model Performance with Dynamic Typing
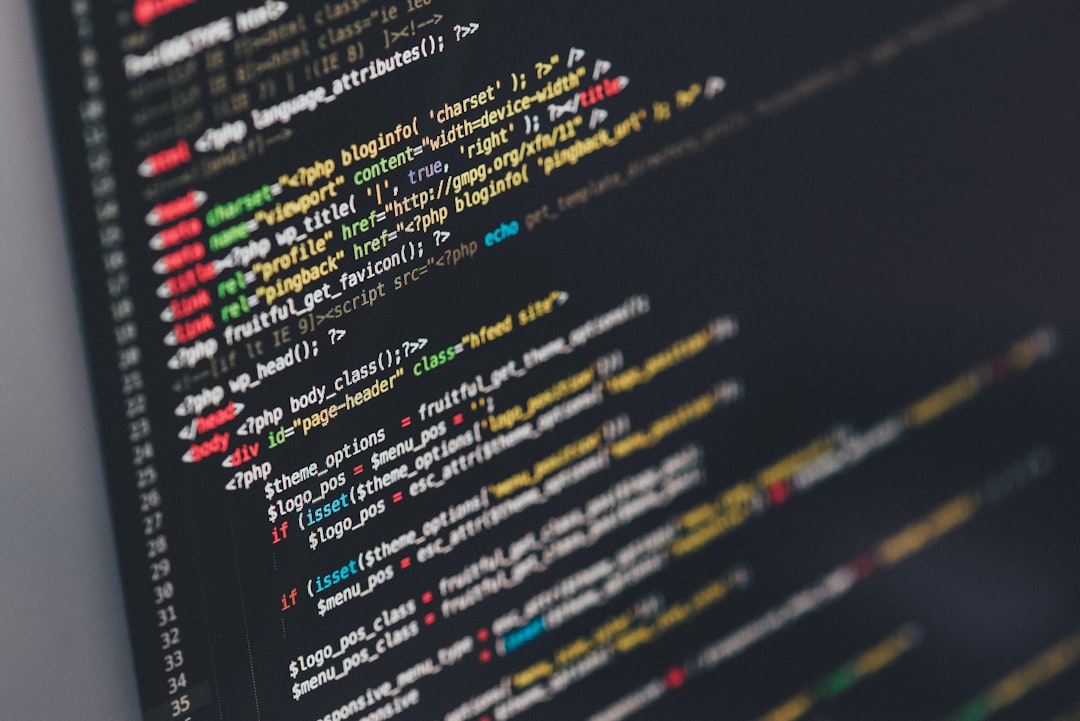
Python's dynamic typing offers a distinct advantage for AI model development by allowing for adjustments to data types and functionalities during program execution. This adaptability empowers researchers to quickly experiment and iterate, which are critical elements of refining AI models to optimally handle varied datasets. Furthermore, the adoption of intricate techniques like self-attention mechanisms becomes more straightforward with Python's dynamic nature, as it simplifies the management of the complex interactions between layers within a model, something that can be restrictive in statically-typed languages. However, this flexibility comes with a price—dynamic typing introduces computational overhead, primarily from the constant need for type checking and more active memory management. So, AI developers must thoughtfully balance the benefits of dynamic typing with its associated costs. The capacity to wield dynamic typing effectively proves to be a significant asset, broadening the potential of AI applications by enabling a more versatile and adaptable approach to machine learning.
Python's dynamic typing, a cornerstone of its flexibility, offers AI developers the freedom to swiftly prototype and adapt models without the rigidity of explicitly declaring variable types. This agile approach can significantly accelerate experimentation and iteration cycles, which are crucial for tackling the rapidly evolving landscape of AI projects. However, this convenience comes with a price. The Python interpreter's need to verify variable types during runtime can introduce performance penalties, especially when dealing with computationally intensive tasks common in machine learning.
One notable consequence of this dynamic nature is the potential for memory fragmentation. As variables change types, memory reallocations become necessary, which can lead to a scattering of memory blocks over time. This fragmentation can gradually diminish performance in long-running AI applications, making proactive memory management strategies a critical concern.
Comparisons with statically typed languages suggest that Python, while incredibly versatile, can sometimes encounter bottlenecks, particularly in scenarios involving numerous tight loops or frequent function calls—a hallmark of many AI training processes. The dynamic nature of Python also enables the creation of collections containing elements of various types, which simplifies data handling for some AI models but also increases the likelihood of encountering type-related errors during debugging, a common frustration in many AI tasks.
Thankfully, Python offers ways to mitigate the downsides of dynamic typing. Type hints, a relatively new feature (PEP 484), allow developers to annotate code with type information. This not only enhances readability but can also enable specific tools like Mypy to conduct static type checks, potentially uncovering and addressing errors before they cause runtime problems and lead to unexpected performance issues.
Unfortunately, the flexibility of dynamic typing can lead to complexities when tackling concurrent programming models. Multi-threaded environments with shared mutable state risk introducing race conditions, emphasizing the importance of careful state and type management to maintain program integrity. Even Python's automatic garbage collection, while a boon for memory management, can add significant overhead in AI workflows, where many temporary objects are created during model training. This overhead can affect model performance, so mindful design is essential.
To stay on top of dynamic typing challenges, developers are advised to employ profiling tools. These tools offer valuable insights into memory usage and identify potential performance bottlenecks, specifically pinpointing the effects of type changes on execution times. For AI tasks that demand real-time data processing, the ability to manipulate data dynamically is a major advantage. However, the associated runtime type checks can hinder the achievement of stringent latency requirements, calling for careful balancing of flexibility and performance optimization within the design of the system.
Mastering Python's Dynamic Variable Declaration A Deep Dive for AI Developers - Practical Applications of Dynamic Variables in Machine Learning
Dynamic variables are a powerful tool in machine learning, offering the ability to adapt to changing data and project needs. Python's dynamic typing allows developers to easily adjust variable types and values on the fly, which is especially valuable when working with complex and fluctuating datasets. By leveraging dynamic variables, developers can optimize model performance and effectively fine-tune hyperparameters, leading to more robust and capable AI models.
The dynamic creation of variables at runtime, made possible by functions like `globals()`, `setattr()`, and `locals()`, further enhances code flexibility and responsiveness. This can be particularly helpful in machine learning projects where conditions change frequently. However, it's crucial to consider the potential impact on performance. Python's flexibility comes with the added burden of type checks and dynamic memory management, which can introduce overhead. Developers need to strike a balance between the advantages of dynamic variables and potential performance trade-offs. Ultimately, the ability to use dynamic variables effectively can greatly expand the possibilities of AI development by supporting adaptable and responsive machine learning applications.
Python's dynamic variable nature provides significant advantages for machine learning, allowing for adaptability and rapid prototyping in AI model development. The ability to easily modify variable types during execution empowers researchers to quickly explore and refine models, particularly in the fast-paced AI research landscape. However, this flexibility comes at a cost. The constant need for type checking during runtime introduces computational overhead, potentially hindering performance optimizations crucial for production environments. We must acknowledge this trade-off when deciding how to utilize dynamic variables.
Creating a dynamic variable is straightforward: assign a name and a value, adhering to Python's naming conventions. Incrementing a dynamic variable's value involves simple operations, like adding to a score variable. This straightforward process helps developers quickly adapt and iterate within their AI workflows.
Furthermore, leveraging functions like `globals()`, `setattr()`, `locals()`, and `exec()` allows developers to generate variables during runtime, enabling greater code adaptability. This feature offers advantages when dealing with dynamic data inputs in machine learning models, particularly for cases where the data structure may evolve throughout a model's operation. This dynamic approach to variable management ties directly to concepts like hyperparameter tuning, where tools like Hydra provide mechanisms to effectively adapt configurations in machine learning applications.
Dynamic variables can also improve the ability to seamlessly integrate various machine learning components and frameworks. However, this dynamic flexibility requires a careful understanding of how it influences memory management. Frequent changes in variable types can lead to memory fragmentation, which can degrade performance in long-running AI applications. This is a notable issue in applications dealing with large models and complex calculations, highlighting the need for thoughtful memory management practices.
The interpreted nature of Python coupled with dynamic typing can introduce performance bottlenecks, particularly in computationally intensive AI tasks that are executed repeatedly. When compared to compiled languages, where type checking occurs during compilation, Python's runtime checks can add overhead. This trade-off is important to consider, as it could make Python an unsuitable choice for performance-critical AI applications.
While the dynamic aspect enables efficient adaptation, it can add complexity to concurrent programming, where shared mutable states can result in race conditions. This necessitates careful attention to type management and thread safety during development, otherwise, unexpected program behavior or errors might arise. Thankfully, techniques like type hints—where developers annotate code with type information—can help to mitigate some of these issues by facilitating static analysis and early error detection.
The power of dynamic variables is particularly relevant when processing data in real time. Dynamically adapting to various data structures and formats provides versatility. However, meeting strict latency requirements can be challenging due to runtime type checks, forcing engineers to carefully balance flexibility and performance. Profiling tools can help to pinpoint performance bottlenecks and guide optimizations, including those arising from the management of dynamic variables.
Finally, understanding how dynamic variables interact with Python's garbage collection is crucial for optimizing AI applications. Dynamically allocated objects, especially during model training, can exert pressure on the garbage collection system, potentially impacting application performance. A well-designed approach to variable management is therefore critical for efficiently handling these situations and ensuring optimal performance.
Python's dynamic variables offer a powerful paradigm for AI development, providing flexibility and rapid iteration. However, a clear awareness of the trade-offs between flexibility and performance is crucial for successfully leveraging dynamic variables in real-world applications. Balancing flexibility and efficient memory management is key to crafting performant and robust AI systems.
Mastering Python's Dynamic Variable Declaration A Deep Dive for AI Developers - Avoiding Common Pitfalls in Dynamic Variable Creation
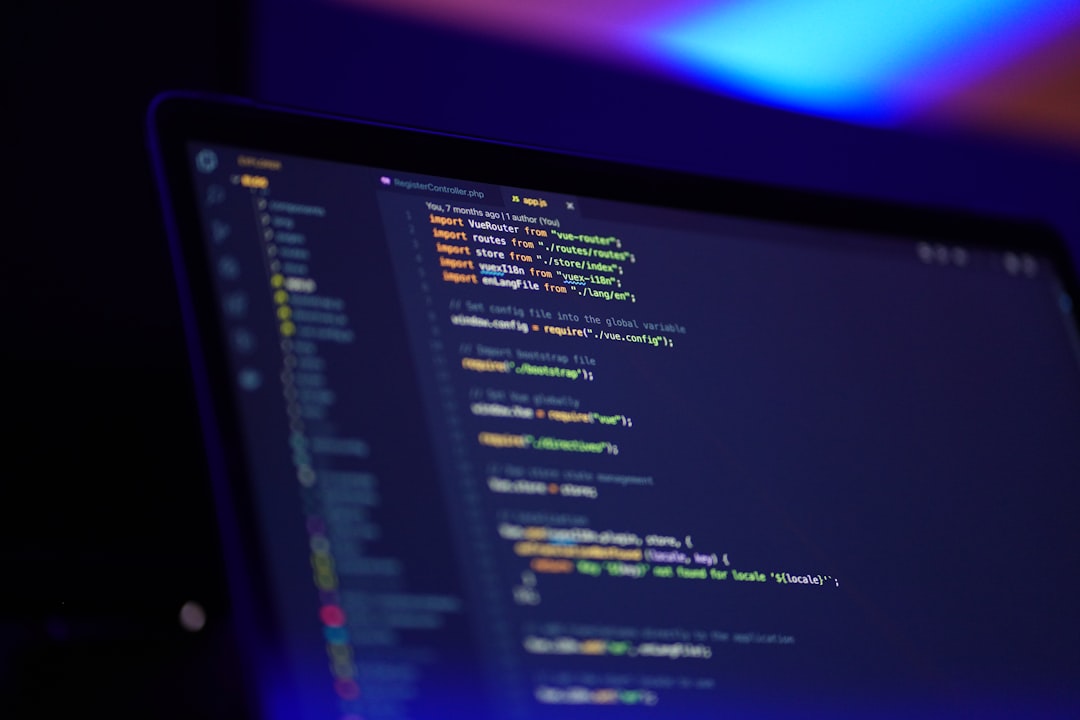
While the dynamic creation of variables in Python offers increased flexibility and adaptability, especially in AI development, it's crucial to be aware of potential pitfalls. Using unclear or ambiguous variable names can significantly impact code readability, making it difficult to understand the purpose of each variable. Furthermore, the various methods for creating dynamic variables, including functions like `globals()`, `setattr()`, and `exec()`, each come with their own set of pros and cons. Choosing the wrong method can lead to difficulties in maintaining and understanding the codebase. It's also essential to prioritize the validation and sanitization of dynamically generated variables, particularly when these variables rely on external data sources or user input, as failing to do so could introduce security risks. While the benefits of dynamic variables are undeniable, overusing them can lead to code that is complex and hard to follow. Strive for a balance that leverages the power of dynamic variables while maintaining code clarity and long-term maintainability.
Python's dynamic nature, while enabling flexibility, presents some unexpected challenges when creating variables on the fly. For instance, the scope of dynamically created variables can be tricky to grasp, especially when using functions like `locals()` or `globals()`. Python's scope resolution order can lead to unanticipated behavior, potentially introducing hard-to-debug issues.
While the ability to create variables dynamically appears convenient, it introduces a performance cost in the form of runtime type checking and memory management. This cost becomes more apparent in tight loops or performance-sensitive parts of your AI code, possibly hindering the overall speed of your application.
The flexibility of dynamic variable creation can inadvertently lead to memory fragmentation. As variables shift types and memory is allocated and deallocated, memory blocks can become scattered, negatively impacting performance, especially in longer-running machine learning projects where memory efficiency is vital.
Functions like `setattr()` and `exec()` provide powerful tools for creating variables, but they introduce a significant level of complexity. This can make code harder to understand and maintain, especially when working on large, evolving AI projects with multiple developers.
The interaction between dynamic variables and Python's garbage collector is more involved than it might seem. Dynamically created objects can cause more frequent garbage collection cycles, impacting performance, particularly during model training, when many short-lived objects are generated.
Creating dynamic variables within a multi-threaded context introduces the challenge of ensuring thread safety. If shared mutable state isn't carefully managed, race conditions can arise, leading to unpredictable outcomes in your AI applications.
Compared to statically-typed languages, Python's dynamic nature necessitates runtime type checks, which can introduce a performance penalty. This slowdown is especially noticeable in AI training loops or functions that are repeatedly called, potentially creating a bottleneck.
Dynamic typing is appealing for quick prototyping and iterations, but this flexibility comes with a performance cost. Developers should be conscious of this added overhead, particularly when dealing with constraints on execution time, like in real-time processing systems.
Type hints, though seemingly counterintuitive to the dynamic nature of Python, can be extremely valuable. They add clarity and maintainability to your code while also assisting static analysis tools, allowing them to identify potential issues before they cause runtime errors.
Dynamically created variables can unintentionally extend the lifespan of objects in memory through persistent references. In lengthy AI processes, this can amplify memory consumption, reinforcing the need for effective memory management practices in your workflows.
Mastering Python's Dynamic Variable Declaration A Deep Dive for AI Developers - Leveraging Python Libraries for Efficient AI Development
Python's strength in AI development stems in part from its vast collection of libraries built specifically for AI and machine learning tasks. Libraries like TensorFlow and PyTorch are indispensable for deep learning, while Scikit-learn proves highly useful for traditional machine learning approaches. These libraries can greatly accelerate the process of building and training models, allowing developers to spend more time focusing on the core logic and innovation behind their projects rather than writing basic building blocks repeatedly. Libraries like NumPy and Pandas are vital for data manipulation and preprocessing, steps critical in AI workflows. These tools can handle extensive datasets and mathematical operations needed to prepare the data for model training, saving time and effort.
However, simply using these libraries without understanding how they function can lead to issues. It's crucial for AI developers to possess a deep understanding of the data they are using and the specific strengths and limitations of the chosen libraries. Not doing so might result in choosing the wrong tools or misapplying them, leading to reduced efficiency, unexpected errors, or suboptimal model performance. The most effective use of Python libraries in AI development involves a blend of understanding and application. Mastering how and when to leverage the powerful tools available is a critical skill for AI developers.
Python's rich ecosystem of libraries and frameworks makes it a strong choice for AI development, particularly for tasks involving machine learning and deep learning. Libraries like TensorFlow and PyTorch are favored for deep learning, while Scikit-learn is commonly used for more traditional machine learning approaches. However, efficiently using these tools depends on a solid understanding of your data. This understanding is essential to effectively leverage Python's strengths in AI.
Python's popularity amongst AI researchers and engineers stems from its straightforward syntax and readability. Some popular Python libraries that are specifically geared towards AI include the well-known TensorFlow, Keras, and Scikit-learn along with numerical libraries like NumPy and Pandas. NumPy, an open-source numerical library, excels at matrix operations and is often considered the go-to library for many machine learning tasks. Pandas, with its data analysis features, provides powerful tools for handling data manipulation, which is crucial for preparing data for AI pipelines. Additionally, SpaCy has proven to be an incredibly powerful library for natural language processing (NLP) tasks.
Becoming skilled in AI development with Python requires not only learning the basics but also gaining practical experience with these libraries and applying best practices. By skillfully using the right libraries, we can streamline AI workflows. This includes making operations more efficient, from the initial stage of data preparation to the development of complex AI algorithms. While Python offers this remarkable set of tools, the effectiveness of these libraries is intertwined with how well Python's memory allocation is understood and managed.
However, Python's dynamic nature, while flexible, also introduces tradeoffs in performance and resource management. It's crucial for AI developers to be aware of these aspects. For example, while Python's dynamic variable allocation can be convenient for tasks involving quick model prototyping or exploring complex interactions, it can also introduce overhead during execution due to type checks. Moreover, the dynamic allocation and deallocation of memory through garbage collection can lead to fragmentation, potentially degrading performance, particularly in lengthy AI operations. We also need to pay close attention to Python's scope resolution rules when dynamically creating variables, especially using functions like `locals()` and `globals()`, since it can result in unexpected behavior if not understood well. In scenarios where concurrency is crucial (such as using multiple threads), careful management of mutable variables is vital, as poorly managed mutable states could easily lead to race conditions.
Despite these potential challenges, integrating type hints can improve clarity and maintainability within the Python codebase while also allowing tools to flag potential runtime errors earlier. The proper use of libraries, alongside a more conscious understanding of Python's internal workings, is critical for efficiently leveraging Python's strengths for AI development. While Python provides a powerful platform for AI development, the journey to mastery requires an understanding of how these libraries interact with the core Python system, especially when considering tasks involving resource constraints or real-time data processing.
Mastering Python's Dynamic Variable Declaration A Deep Dive for AI Developers - Implementing Runtime Variable Generation Techniques
Python's dynamic nature allows for generating variables during runtime, a powerful feature particularly useful within the context of AI development. Techniques such as using `globals()`, `locals()`, or `setattr()` enable the creation of variables with names that are determined during the program's execution, making code more adaptable to dynamic data structures and evolving conditions. This flexibility can be a boon for building systems that react to changing circumstances, enhancing responsiveness.
However, this approach comes with its own set of considerations. For instance, relying on the `exec()` function for runtime variable generation can introduce security risks if not used with extreme caution. Storing dynamically generated variables within dictionaries can simplify data management but might compromise the readability of the code. Also, keep in mind that constantly generating and changing variable types can lead to memory fragmentation, potentially degrading program performance due to increased type checking overhead.
Despite these potential challenges, the ability to implement runtime variable generation offers a potent tool for AI developers who need to work with flexible and ever-changing environments. Understanding the trade-offs between flexibility and performance, as well as the potential security concerns, is vital when integrating these techniques into your projects.
Here are ten interesting points about applying runtime variable generation methods in Python, especially relevant for folks working on AI projects:
1. **The Complexity of Dynamic Creation**: While dynamically creating variables using tools like `globals()`, `locals()`, or `setattr()` appears simple at first, it can complicate code maintenance, particularly when working on large projects with multiple people. The added abstraction can obscure a variable's purpose, potentially making debugging more difficult.
2. **Navigating Scope Resolution**: When generating variables on the fly using functions like `locals()` and `globals()`, the outcome depends on the context of their use. This variability can lead to accidental changes in variables within unintended scopes, creating tough-to-find bugs.
3. **Performance Considerations**: Dynamically generated variables introduce a performance penalty due to the runtime type checking Python does, especially when doing heavy computation like AI model training. This overhead can be noticeable in training loops where the same code runs over and over, potentially slowing down the learning process.
4. **The Risk of Memory Fragmentation**: Frequently changing variable types and the memory allocation/deallocation that comes with dynamic variable generation can lead to memory fragmentation. Over time, this can harm performance, particularly in AI applications that run for extended periods and require efficient memory usage.
5. **Garbage Collection's Added Work**: Dynamically generated objects can cause the garbage collector to run more often, which might introduce latency in AI applications where performance is crucial. Understanding when these cycles occur and how frequently is important for optimizing real-time AI systems.
6. **Unintended Object Lifespans**: Dynamically created variables can unknowingly extend the lifespan of objects in memory by maintaining references to them, affecting memory consumption. In applications that run for a long time, unintended retention of variables can lead to excessive memory use and performance slowdowns.
7. **Concurrency Challenges**: The flexibility of dynamic variables introduces potential problems in concurrent programming, particularly in multi-threaded environments. If shared mutable states aren't handled with care, it can lead to race conditions that cause unpredictable behavior in intricate applications.
8. **The Benefit of Type Hints**: Even though Python is known for its dynamic typing, integrating type hints can improve readability and maintainability significantly. They help with documentation and allow static analysis tools to identify potential type-related problems before runtime, which is critical for complex AI systems.
9. **Debugging Hurdles**: The expressive nature of dynamic variables can complicate debugging. Type-related errors might not be obvious until particular execution paths are followed, making it tough to figure out the exact conditions that led to these errors.
10. **Configurability and Adaptability**: Using tools like Hydra for configuration management can optimize the use of dynamic variables. It helps with better hyperparameter tuning and real-time adaptability in machine learning, though it's important to consider the trade-off between this versatility and performance concerns.
These observations highlight the intricacies and considerations needed for effectively using runtime variable generation methods in AI workflows with Python, showing both the power it offers and the subtleties that need careful attention.
Create AI-powered tutorials effortlessly: Learn, teach, and share knowledge with our intuitive platform. (Get started for free)
More Posts from aitutorialmaker.com: