Troubleshooting Directory Creation Issues in Python 310 A Comprehensive Guide
Troubleshooting Directory Creation Issues in Python 310 A Comprehensive Guide - Understanding os Module for Directory Operations in Python 10
Python's `os` module serves as a bridge to your operating system, providing tools to interact with files and directories. It offers a range of capabilities for tasks like directory navigation, creation, and deletion. Essential functions within `os` include `os.makedirs()` for creating directories and their parent paths if needed, and `os.listdir()` for retrieving a directory's contents.
When working with directories, understanding how to handle errors is vital. The `os` module can raise exceptions if you try to create an existing directory or remove one that's not empty. It's important to include appropriate error handling in your code to prevent unexpected behavior.
A significant advantage of the `os` module is its cross-platform nature, ensuring your Python scripts work consistently on various systems such as Windows, macOS, or Linux. This consistency simplifies the development of applications that need to interact with file systems in a dependable manner. Mastering the `os` module is foundational for effectively managing directories and, consequently, troubleshooting potential issues encountered during script development and deployment.
The `os` module acts as a bridge between Python and the operating system, offering a consistent way to manage directories across various platforms. This consistency is crucial, allowing developers to write code that functions identically on Windows, macOS, or Linux without needing to rewrite specific sections for each system.
One noteworthy aspect of the `os` module is its ability to interact directly with the file system's underlying mechanisms. This direct access enables operations like renaming or moving directories using native system commands, often offering efficiency gains compared to higher-level abstractions.
The `os.makedirs()` function stands out for its capability to generate a directory structure, including any necessary parent directories, in a single command. This can streamline code, especially when creating complex directory trees, by reducing the need for multiple checks and error handling for individual subdirectories.
However, using the `os` module requires caution when dealing with permissions. Unexpected errors can arise if a script attempts to create directories in restricted areas of the file system. Therefore, a thorough understanding of the operating system's permission structure is vital for avoiding such complications.
One of the ways developers can avoid errors is by checking if a directory exists before attempting to interact with it. This can be done using the `os.path.exists()` or `os.path.isdir()` functions. Such checks are essential for maintaining the stability and reliability of a script by handling scenarios where the target directory might not exist.
When creating directories using `os.makedirs()`, it is important to be aware of the potential for error if the target directory already exists. By default, `os.makedirs()` will raise an exception in this scenario. However, the `exist_ok` parameter can be set to `True`, enabling a more graceful handling of this situation.
While `os` offers a broad set of functionalities, it lacks built-in support for asynchronous directory operations. This limitation may be a constraint for projects where scalability is critical and requires non-blocking input/output operations for optimal performance.
Knowing where your Python script is running can be invaluable for debugging file path problems. `os.getcwd()` retrieves the current working directory, allowing developers to quickly assess the context of their script's operations.
The `os.listdir()` function provides a programmatic way to inspect the contents of a directory. This feature is useful for understanding the directory's structure before applying further operations. It can streamline scripts that need to inspect the contents before making changes or deciding on next steps.
While the `os` module primarily focuses on file system interactions, Python 3.10 introduced the `zoneinfo` module. This module enhances time zone handling and can be used in conjunction with the `os` module when dealing with timestamps associated with files and directories. This combination can be beneficial in applications that require precise management of time-related data.
Troubleshooting Directory Creation Issues in Python 310 A Comprehensive Guide - Conditional Directory Creation Using os.makedirs
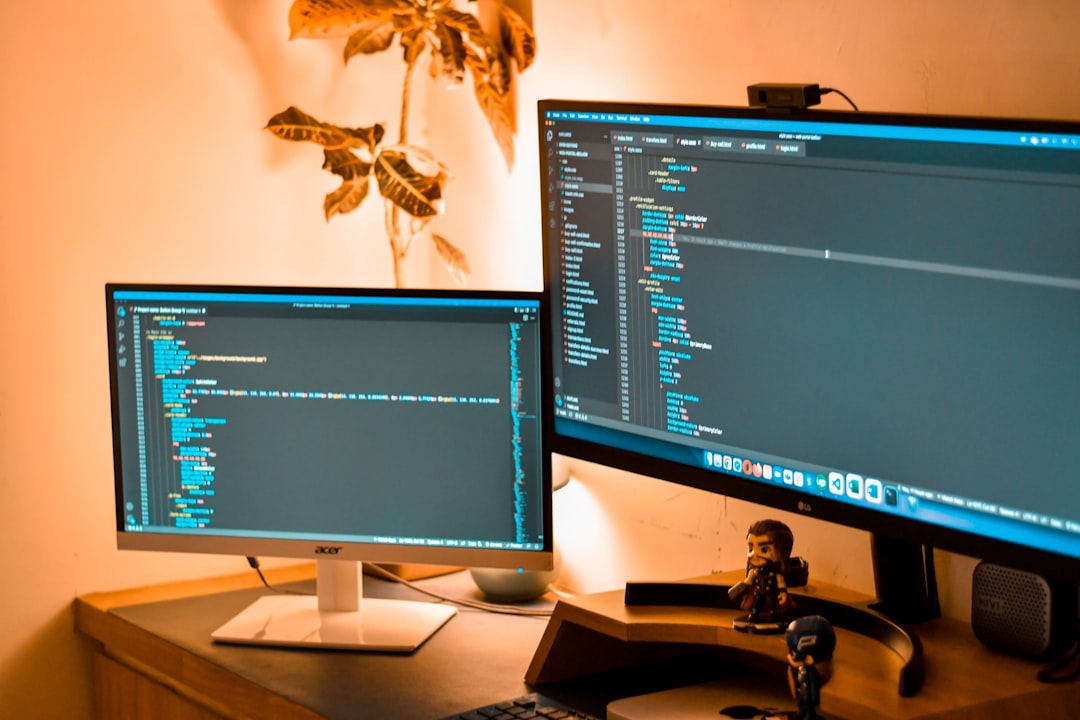
When creating directories in Python, the `os.makedirs` function offers a convenient way to manage the process, especially when dealing with nested directory structures. It can create all the necessary intermediate directories in a single command, which can streamline your code. However, if a directory already exists, it can cause issues unless handled correctly.
The `exist_ok=True` parameter within `os.makedirs` is a powerful tool for preventing errors in such situations. By specifying this option, you can ensure the function creates a directory only if it does not already exist. This is especially useful when your script might encounter situations where multiple directories are being created at the same time, which can lead to exceptions related to permissions or existence.
Keep in mind that when dealing with directory permissions on Unix-like systems, the user's `umask` can impact the permissions applied to newly created directories. If your scripts aren't producing the desired permissions, it's worth considering the `umask` settings to ensure they don't unintentionally restrict access to the directories. If the `umask` isn't set appropriately, you might find yourself needing to manually adjust the directory permissions later using functions like `os.chmod`. By carefully using `os.makedirs` with `exist_ok` and considering `umask` implications, you can avoid common directory creation pitfalls and create more robust and reliable Python scripts.
Python's `os.makedirs()` function offers a streamlined approach to creating directories, particularly when dealing with nested structures. Its recursive nature simplifies directory hierarchy establishment in a single command, reducing the need for multiple, often tedious, calls. While generally efficient, it's important to recognize that it can introduce complexity if not handled correctly.
For example, if a directory already exists, `os.makedirs()` will, by default, throw an error. Thankfully, the `exist_ok` parameter allows for a more graceful approach by preventing this exception when set to `True`. This functionality offers a clean way to manage conditional directory creation without needing to check if the path already exists.
Operating system limitations, such as the user's `umask` value, can interfere with the permissions of newly created directories. Understanding the potential impact of these platform-specific factors is vital for ensuring the function operates as intended. If permission errors occur, the created directories may require manual adjustment using `os.chmod`, which is something to keep in mind when debugging errors.
While `os.makedirs()` often provides a convenient method, it's important to be mindful of its behavior in diverse scenarios. For instance, creating directories that include special characters may lead to errors if the character encoding isn't managed properly across different operating systems. This can easily be missed and require a bit of exploration, especially when working on systems with less common configurations.
The functionality of `os.makedirs()` can be affected by various factors, such as permission issues and path length limitations. Exceeding certain operating system limitations with overly complex path structures can lead to an `OSError`. Recognizing these potential pitfalls allows for more effective debugging and troubleshooting when encountering errors during directory creation.
Furthermore, when considering overall performance, `os.makedirs()` tends to be more efficient than creating directories individually, especially for complex hierarchies. The ability to create a multitude of nested directories in a single function call leads to fewer interactions with the operating system, minimizing overhead for directory creation. And it's not just about speed; when creating directories, it helps ensure that partially-created directories aren't left behind in cases of errors, preserving the integrity of your file system.
However, while offering significant advantages, `os.makedirs()` also reveals some interesting characteristics when it comes to error handling and debugging. Examining its behavior in different situations, like dealing with existing directories or permission errors, provides crucial insights into how Python interacts with the underlying operating system. Understanding these interactions contributes to a more robust debugging and error resolution process when issues surface.
As Python continues to evolve, the functionality of `os.makedirs()` becomes increasingly valuable, especially when combined with modules like `zoneinfo`, introduced in Python 3.10. This combination can help to better track directory creation events via the system's built-in time information, allowing for advanced logging and timestamping of directory creations. This subtle point has potentially far-reaching implications for data integrity and logging operations.
Troubleshooting Directory Creation Issues in Python 310 A Comprehensive Guide - Basic Syntax for Creating New Directories
The `os` module in Python provides tools for working with directories, including creating new ones. The fundamental method for creating a single directory is through `os.mkdir`. This function takes the desired path as an argument, along with an optional 'mode' argument that defines file permissions. Typically, `os.mkdir('path/to/directory', mode=0o777)` is used, where 0o777 allows full access for all users.
For situations involving multiple nested directories, `os.makedirs` proves more effective. This function efficiently creates the entire directory tree, including all intermediate paths, with a single command. It's important to be mindful that Unix-like systems' user permissions, controlled by `umask`, might interfere with the permissions of newly created directories. To prevent potential errors, consider using `exist_ok=True` with `os.makedirs`, which gracefully handles cases where the target directory already exists.
By mastering the `os.mkdir` and `os.makedirs` functions, as well as understanding how user permissions interact, developers can manage and troubleshoot directory creation issues with greater ease. These capabilities, along with appropriate error handling, contribute to more robust and reliable Python scripts.
1. The `os.makedirs()` function is a handy tool for crafting intricate directory structures with multiple levels in a single command. This not only reduces the overall complexity of your code but also helps to prevent errors during the creation process, making your code more robust.
2. When you use `os.makedirs()` by default, it's programmed to throw an exception if the directory you're trying to create already exists. This behavior can be modified with the `exist_ok=True` argument. This way, you can avoid the exception and have a smoother directory creation process, thereby minimizing the need for complex error handling in your scripts.
3. The permissions applied to newly created directories can be influenced by operating system features. Specifically on Unix-like systems, the user's `umask` value directly affects these permissions. This can lead to unexpected access issues if not managed properly.
4. Many operating systems have a limit on the maximum number of characters allowed in a directory path, often around 260 characters. Exceeding this limit can lead to an `OSError` when you try to create a directory. It's crucial to be aware of these constraints and plan your directory structures accordingly to avoid such errors.
5. Special characters in directory names can pose difficulties across different operating systems. The encoding of characters might not be consistently handled, leading to errors. It's worth digging into how Python and your operating system interact when dealing with characters to avoid such scenarios.
6. While Python's `asyncio` module facilitates asynchronous operations for various tasks, this feature hasn't been extended to the `os` module. This can be a limitation for programs that need non-blocking directory operations to optimize performance. Developers need to explore alternatives like different libraries if they require asynchronous directory management.
7. Python 3.10 brought in the `zoneinfo` module that improves how timestamps are managed with directory creation events. This can lead to more sophisticated logging of file system changes. This is quite useful when you want to have a good understanding of changes to your data over time.
8. One benefit of `os.makedirs()` is that it prevents the creation of incomplete or half-finished directory structures. These can often happen when creating directories one at a time, leaving behind leftover directories that might be hard to clean up. This feature helps keep the file system clean in cases of errors.
9. Interestingly, the `os` module has capabilities for handling symbolic links. Functions like `os.symlink()`, used in conjunction with `os.makedirs()`, give you more flexibility when creating directory structures. This can be quite valuable for handling complex file management scenarios.
10. The role of `os.listdir()` extends beyond just listing a directory's contents. It can be used to check the conditions of a directory before creating a new one. This preemptive step adds another layer of robustness to your script's error handling and can avoid unexpected situations.
Troubleshooting Directory Creation Issues in Python 310 A Comprehensive Guide - Checking Directory Existence Before Creation
When creating directories within Python, it's wise to first confirm whether the directory already exists. This preventative measure helps avoid errors and enhances the efficiency of your code. The `os.path.isdir()` function lets you pinpoint if a path is indeed a directory, while `os.path.exists()` can verify if a path exists, regardless of whether it's a file or directory. This verification becomes particularly important when working with functions like `os.makedirs()`, which by default can generate exceptions if the directory already exists. However, you can sidestep this by setting the `exist_ok` parameter to `True`. Incorporating these checks into your scripts reinforces their reliability and prevents complications during directory creation. A thorough grasp of these basic practices is vital for efficiently managing and resolving directory creation issues that commonly appear in Python 3.10 and subsequent versions.
1. Verifying if a directory exists before attempting to create it can significantly reduce the overhead of handling errors, which can be computationally expensive. Minimizing unnecessary exception handling is key for crafting more efficient scripts.
2. The `os.path` module offers two core functions for confirming the existence of a directory: `os.path.exists()` and `os.path.isdir()`. While `os.path.exists()` can identify both files and directories, `os.path.isdir()` specifically verifies if a path points to a directory, making code intentions clearer.
3. The distinction between files and directories might not always be straightforward across different file systems. Certain Unix-like systems, for example, may represent directories as specially flagged files. Understanding these nuances can influence how existence checks are implemented.
4. Using `os.path.exists()` can sometimes result in false negatives, particularly in networked or cloud-based storage environments where latency can influence the accuracy of directory information. This aspect is often overlooked by developers creating scripts for distributed systems.
5. Python's underlying mechanism for verifying directory existence relies on POSIX calls, which can be significantly faster than alternative approaches. This can be especially important when managing large file systems where input/output operations can become a performance bottleneck.
6. Operating system caching can impact the outcome of directory existence checks. If a directory is created and then immediately checked for existence, the results might not reflect the actual state due to cache delays.
7. In applications using multiple threads, relying solely on pre-creation checks can introduce the possibility of race conditions. These scenarios can lead to situations where two or more threads attempt to create a directory concurrently, possibly resulting in unintended errors.
8. Ensuring the consistency of directory existence checks across various operating systems is critical for scripts intended to function across different platforms. The way file systems handle directories can differ between Windows and Unix-like environments, requiring thoughtful code design even when leveraging the `os.path` module.
9. When creating a large number of directories, employing a set to keep track of previously checked directories can drastically reduce redundant checks. This approach can improve performance, compared to a basic list-based search.
10. Integrating directory existence checks into a comprehensive logging strategy can greatly aid in debugging. By logging these checks and their results, developers can gain insights into which paths are being prematurely or incorrectly validated, ultimately leading to improved troubleshooting.
Troubleshooting Directory Creation Issues in Python 310 A Comprehensive Guide - Common Directory Operation Errors and Solutions
Directory operations in Python can be tricky, especially when you're using the `os` module. Common problems often stem from permission issues, where your code lacks the necessary permissions to create directories. You'll often encounter the dreaded "No such file or directory" error, signaling that either the path you're providing is incorrect or the parent directory doesn't exist. To mitigate these issues, make sure that the parent directory is already created before attempting to create the target directory. Using `os.makedirs()` with the `exist_ok` parameter can help avoid errors if the directory you're trying to create already exists. Importantly, error handling is essential when dealing with directories. Employ try-except blocks to manage errors like `FileNotFoundError` or `PermissionError`, improving your scripts' stability. These are fundamental practices for effective directory management and error resolution in Python 3.10.
1. Directory creation problems in Python can arise not just from permission issues, but also from fundamental filesystem restrictions, like maximum path length. Many systems cap path lengths around 260 characters, resulting in an `OSError` if exceeded. Planning your directory naming carefully can avoid these issues.
2. The `exist_ok` parameter within `os.makedirs()` is more than a minor tweak—it's a game-changer for conditional directory creation. By default, it throws an error if a directory already exists, but setting `exist_ok` to `True` prevents these disruptions in automated scripts, improving the overall process flow.
3. On certain operating systems, particularly Unix-based ones, the permissions assigned during directory creation are influenced by the `umask` setting. This can unintentionally limit access if not considered during script development, often an overlooked aspect.
4. When directories include special characters, you have to pay close attention to character encoding. Differences in how operating systems handle character sets can lead to errors, especially when dealing with non-ASCII characters within directory names.
5. From a performance perspective, handling directory creation exceptions can be computationally expensive. When a directory creation fails and throws an exception, the associated processing overhead can impact script speed, making preemptive existence checks beneficial.
6. Operating system caching can create inconsistencies when checking if a directory exists. If a directory was just created, checking immediately might show outdated information due to caching, potentially impacting the decisions made within your script.
7. The `os` module doesn't support asynchronous directory creation, which can be a hurdle in high-performance or real-time apps. Developers needing non-blocking operations need to explore alternative libraries, which adds complexity to managing asynchronous workflows.
8. Race conditions are more likely in multi-threaded environments when checking for directory existence before creating. This can lead to multiple threads trying to make the same directory at the same time, potentially overriding intended error handling.
9. Detailed logging of directory creation attempts significantly aids debugging. By recording both the existence checks and the outcomes of creation attempts, developers get a clearer picture of their script's execution and where errors originate.
10. Python's error handling for directory operations can highlight limitations that may not be obvious without careful testing. For example, `os.makedirs()` might fail quietly under certain circumstances—such as with networked filesystems—making robust testing crucial for reliable applications.
Troubleshooting Directory Creation Issues in Python 310 A Comprehensive Guide - Implementing Exception Handling for Robust Directory Management
When managing directories in Python using the `os` module, effectively handling exceptions becomes crucial for creating robust and reliable applications. This involves strategically using `try`, `except`, `else`, and `finally` blocks to anticipate and manage potential issues like creating a directory that already exists or encountering permission errors. This approach significantly improves script stability by allowing for graceful error management, preventing unexpected crashes, and promoting proactive identification of potential issues. However, it's important to remember that exceptions should be reserved for genuinely unusual situations. Using them for normal program flow can make code more complex and less readable. By thoughtfully implementing exception handling in this way, Python developers create more resilient applications capable of handling unforeseen directory management issues.
1. Exception handling within Python's directory management routines can have a substantial effect on performance. Effectively managing exceptions helps avoid situations that can lead to slowdowns, particularly when working with many directory operations.
2. The `os.makedirs()` function handles exceptions differently compared to other functions. It employs the `exist_ok` parameter for smoother execution, allowing developers to concentrate on the essential parts of their code without unnecessary error management.
3. It's crucial to be aware of operating system limitations such as the maximum file path length and permissions schemes. Ignoring these boundaries can lead to cryptic error messages and unexpected behavior from `os.makedirs()`.
4. Using try-except blocks for error handling can add overhead. If overused, it can hinder directory creation, especially in scripts that perform these operations rapidly.
5. The `umask` setting on Unix-like systems subtly but significantly affects directory permissions. A lack of understanding of this setting can lead to perplexing access issues for both developers and users.
6. While asynchronous programming in Python is gaining traction, the `os` module doesn't offer support for asynchronous directory operations. This can be a constraint for applications requiring non-blocking directory management.
7. Network latency can impact directory existence checks, especially in environments like cloud or distributed storage. The inherent delay can create situations with inaccurate results (false negatives), making error handling more intricate.
8. Multi-threaded applications introduce new complications. If not handled carefully with mechanisms like locks, directory existence checks can result in race conditions where multiple threads try to create the same directory at once, potentially causing unexpected failures.
9. While good logging practices can boost debugging efforts, understanding how the directory management functions work in detail is important. Effective logging should include both attempted actions and the actual results for comprehensive analysis.
10. Pay attention to character encoding differences between various operating systems when creating directories. Characters that are acceptable in one environment can trigger exceptions or lead to odd behavior in another, particularly affecting cross-platform compatibility.
More Posts from aitutorialmaker.com: