Create AI-powered tutorials effortlessly: Learn, teach, and share knowledge with our intuitive platform. (Get started for free)
Understanding Java Virtual Machine (JVM) A Deep Dive into Memory Management and Garbage Collection
Understanding Java Virtual Machine (JVM) A Deep Dive into Memory Management and Garbage Collection - Memory Areas Inside JVM Stack Heap and Method Areas
The Java Virtual Machine (JVM) meticulously divides memory into several distinct zones to streamline performance and manage resources efficiently. The JVM stack, a crucial part of this architecture, functions as a temporary storage space for local variables and method invocations, operating on a last-in, first-out (LIFO) principle. The JVM heap, a more enduring memory area, is where Java objects and arrays reside. This region also encompasses garbage collection, a mechanism for automatically releasing memory occupied by objects that are no longer needed. The method area, another vital region, stores essential class-level information such as class structures, method data, and constant values. This allows the JVM to readily access the required data during program execution. Beyond these core areas, the JVM also utilizes specialized regions like the native method stack, used for native method interactions, and the Code Cache, which stores compiled code for faster program execution. A strong grasp of how these distinct memory areas function is indispensable for Java developers. Efficiently utilizing these areas leads to the creation of robust and high-performing Java applications, mitigating potential performance bottlenecks and ensuring stability.
The Java Virtual Machine (JVM) carves up memory into a few key zones: the stack, the heap, and the method area, each with its own specific duty during program execution. The stack is the designated area for temporary storage, holding local variables and information related to active method calls. Conversely, the heap is the main storage area for dynamic memory allocation of objects. This means it's where Java objects and arrays live during runtime.
The stack, in its organization, follows a strict "Last In, First Out" (LIFO) pattern. This approach keeps method execution neat and efficient. When a method finishes, its corresponding stack frame is removed, and the memory it occupied is available for reuse.
Interestingly, the heap is shared across all threads in the Java program. Each thread, however, gets its own individual stack. This shared nature of the heap can lead to potential problems if multiple threads attempt to access and modify the same object simultaneously without the proper synchronization. This can result in race conditions, where the final state of the shared object is unpredictable.
The method area, sometimes referred to as Metaspace in newer Java versions, serves as a repository for class-level information. This includes things like the structure of a class, the data associated with its methods, and constant values. By holding class-related data separately, it creates a clean separation between the definition of a class and the instances of that class created during the program.
When the JVM's garbage collector starts cleaning up, its primary focus is on the heap. A variety of clever algorithms are employed, one of the more common being generational garbage collection, to find and dispose of objects that are no longer accessible by any part of the program. This process ensures that memory is not wasted by objects that are no longer needed.
One aspect of the stack worth considering is that it usually has a predetermined size, set when the thread is first created. This can occasionally result in a dreaded `StackOverflowError` if a thread attempts to allocate more stack frames than the allotted space—a common pitfall when using recursion excessively.
Metaspace, the modern incarnation of the method area in newer Java releases, cleverly uses native memory rather than the traditional Java virtual memory. This allows the method area to expand dynamically when necessary, offering increased flexibility. Unlike its predecessors, its size isn't constrained by the Java heap, making it a much more scalable approach.
References in Java are important for memory management, especially with the difference between strong references and weak references. Strong references keep objects in memory for as long as there's a live reference to them. In contrast, weak references allow objects to be potentially reclaimed by garbage collection when memory is tight. This gives programmers a more nuanced level of control over how and when objects are removed from memory.
Although the heap is called a "heap," it doesn't necessarily utilize the typical data structures associated with heap algorithms. The JVM employs a combination of clever techniques, like mark-and-sweep and compaction algorithms, to manage memory effectively. These strategies are what help keep memory allocation and deallocation efficient.
One thing to keep in mind is that the dreaded `OutOfMemoryError` can strike in both the heap and the method area. When this happens, it indicates that either the JVM can no longer allocate memory for new objects in the heap, or it's run out of memory to hold class metadata. These conditions can impact application stability and functionality, so understanding their origins is vital.
Understanding Java Virtual Machine (JVM) A Deep Dive into Memory Management and Garbage Collection - How JVM Handles Object Creation and Memory Allocation
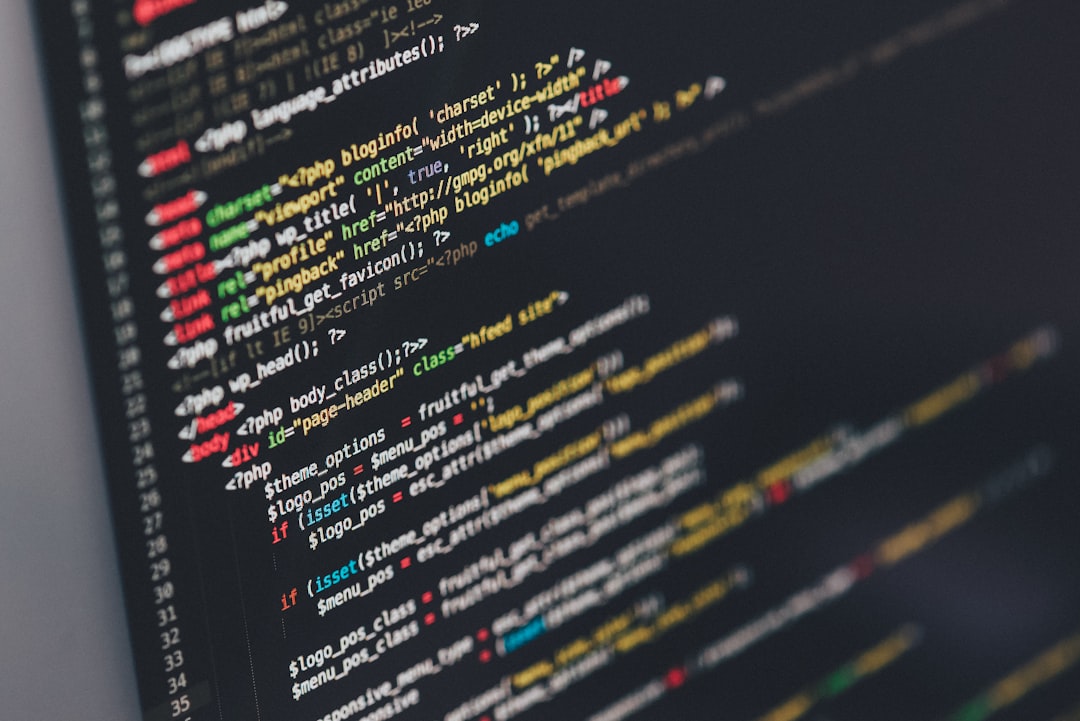
When delving into how the Java Virtual Machine (JVM) handles the creation of objects and memory allocation, the heap emerges as a central player. It's the dedicated area where Java objects and arrays are dynamically allocated during runtime. The JVM implements intelligent strategies, such as generational garbage collection, to track and release the memory associated with objects that are no longer needed. This automated approach not only prevents memory leaks but also contributes to a smoother and more efficient application experience. However, developers need to be mindful of the potential impact of how objects are handled. For example, modifying immutable objects like strings repeatedly can lead to an excessive buildup of new objects, potentially putting pressure on the memory resources available. Furthermore, the JVM's capacity to adapt memory allocation to the specific needs of the program at any given time is a key feature; however, misconfigured settings can trigger errors such as `OutOfMemoryError`, which can destabilize application execution. Achieving a strong understanding of these intricate processes allows Java developers to optimize application performance effectively.
The JVM, in its role as the maestro of Java application memory, faces the constant task of managing object creation and memory allocation. Each new object comes with a built-in overhead, including metadata for garbage collection and synchronization. While this seems like a small detail, it can add up, especially when dealing with a multitude of smaller objects.
JVM object creation, however, isn't inherently safe in multi-threaded scenarios. Developers need to implement mechanisms like synchronized blocks or leverage concurrency utilities to prevent race conditions. This layer of precaution, while necessary for thread safety, inevitably increases complexity and potentially impacts performance if not carefully managed.
Interestingly, the JVM can allocate memory outside the typical heap, using direct memory access features within the `java.nio` package. This lets developers utilize off-heap memory, leading to performance enhancements in certain I/O-bound operations. However, this feature also shifts responsibility for memory management back to the developer.
An intriguing technique called escape analysis allows the JVM to analyze an object's scope of use. If it determines an object is only utilized within a single thread (not escaping), it might choose to allocate it on the stack instead of the heap. This clever maneuver can offer significant performance boosts, reducing the load on the garbage collector.
The heap can suffer from fragmentation if objects are rapidly created and then discarded. To mitigate this, the JVM utilizes strategies such as creating "surrogate" objects that act as placeholders, reducing the impact of constant object creation.
During object allocation, the JVM meticulously aligns objects in memory, sometimes requiring padding bytes. While necessary for optimized access on modern hardware, this can lead to unexpected memory overhead if not considered during development.
JVM memory management isn't set in stone; developers can adjust various garbage collection parameters. This tuning ability provides remarkable flexibility but necessitates careful performance profiling and tuning. Improperly adjusted settings can easily impact overall application performance.
The heap itself is thoughtfully segmented into two main areas, known as the young and old generations. Newly created objects reside in the young generation. As they persist through garbage collection cycles, they eventually migrate to the old generation. This generational approach impacts the specific garbage collection algorithms used for each segment.
Tools like JVM profilers provide invaluable insights into how objects are being created and consumed. Through visualizations of heap behavior over time, they reveal potential areas for improvement, including the detection of memory leaks or inefficient object creation which could lead to performance degradation.
JVM implementations incorporate optimizations specifically tailored for small objects (generally under 64 bytes). Techniques like pooled memory allocation or biased locking help ensure that these commonly used objects are allocated and managed swiftly and efficiently.
This intricate dance of object creation, memory allocation, and garbage collection, while generally transparent to developers, remains a critical aspect of JVM performance. Understanding the mechanisms behind it can empower developers to optimize applications for various platforms. It's a never-ending process of balancing tradeoffs and learning about the hidden mechanics of the virtual machine.
Understanding Java Virtual Machine (JVM) A Deep Dive into Memory Management and Garbage Collection - Garbage Collection Algorithms Young Generation and Old Generation
The Java Virtual Machine (JVM) utilizes a generational approach to garbage collection, separating memory into two main areas: the Young Generation and the Old Generation. This approach centers on the idea that objects have varying lifespans. Newly created objects are typically placed in the Young Generation. Because many objects are short-lived, the JVM can perform frequent, lightweight garbage collection cycles in this area, clearing out these objects quickly. Objects that survive several of these collection cycles are then moved, or "promoted," to the Old Generation. The Old Generation is where longer-lived objects reside. Here, a more thorough, but potentially slower, garbage collection process is used, often employing a mark-and-sweep algorithm. This two-tiered system helps the JVM optimize garbage collection performance. By focusing on the Young Generation for frequent, quick cleanup, it avoids spending time on expensive collection operations in the Old Generation. The result is more efficient memory management in Java programs. Comprehending these generational GC strategies is vital for developers as it enables them to avoid common issues like memory leaks and fragmentation, ultimately leading to more efficient and stable applications.
The Java Virtual Machine (JVM) employs a clever strategy called generational garbage collection to manage memory more efficiently. This approach is based on the observation that most objects don't live very long. The JVM divides the heap into two main sections: the young generation and the old generation. Newly created objects are typically placed in the young generation, a space optimized for frequent, swift garbage collection cycles. This is because most objects are quickly discarded. The idea is that frequent, smaller garbage collections in the young generation are less disruptive than infrequent, large collections across the entire heap.
Within the young generation, you often find a couple of "survivor spaces". These act as temporary holding areas for objects that outlive the initial garbage collection pass. This shuffling of objects between spaces helps in optimizing memory use. Objects that survive multiple rounds in the young generation are eventually promoted to the old generation. This transition, however, can have a big impact on performance as the garbage collection strategies applied to the old generation tend to be more resource-intensive, potentially leading to longer pauses.
The JVM uses various algorithms for garbage collection, each tailored to the specific generation. The young generation employs young or minor garbage collection algorithms (like copying collectors) while the old generation utilizes full or major garbage collection algorithms (often mark-sweep or mark-compact). This dual strategy adds another layer of complexity when optimizing performance, as you need to consider how each algorithm behaves and the tradeoffs involved in choosing one over another.
Larger objects, usually above a certain threshold, are often allocated directly into the old generation. This bypasses the benefits of the young generation, and if not managed carefully, can lead to longer pauses and potential performance degradation during garbage collection in the old generation.
One noteworthy algorithm for the old generation is called Concurrent Mark-Sweep (CMS). CMS attempts to keep application pauses during garbage collection to a minimum by performing some cleanup work concurrently with the running application. Unfortunately, it can introduce memory fragmentation, making it more prone to running out of contiguous memory and leading to an `OutOfMemoryError` under certain conditions.
The duration of the pauses caused by garbage collection can have a significant impact on the application's responsiveness. This becomes particularly important in applications that demand low latency, pushing developers to carefully tune the garbage collection process and choose the optimal algorithm for the specific needs of the application.
Java objects have the ability to define a `finalize()` method, which is called by the garbage collector before an object is reclaimed. While this may seem useful, relying on finalization for cleanup can lead to unpredictable behavior. The timing of `finalize()` calls isn't guaranteed, leading to potential delays and complications in releasing resources.
Thankfully, there are tools available to help with debugging and optimization. The JVM provides different profiling capabilities that allow developers to track memory usage, object lifespans, and garbage collection behavior. The insights gained from these tools can be used to pinpoint areas for improvement, potentially preventing memory leaks and enhancing performance.
Interestingly, the logical heap size defined when starting the JVM isn't always the same as the physical memory it uses. The JVM can dynamically expand its memory usage up to the system limits. Developers should be aware of this behavior to effectively manage resources.
This discussion highlights that the JVM's garbage collection process is a complex mechanism, but understanding its nuances and features enables Java developers to make more informed choices regarding memory management. Understanding these components allows for optimal performance in various Java applications, minimizing issues related to performance and stability.
Understanding Java Virtual Machine (JVM) A Deep Dive into Memory Management and Garbage Collection - Memory Leaks Common Causes and Prevention Strategies
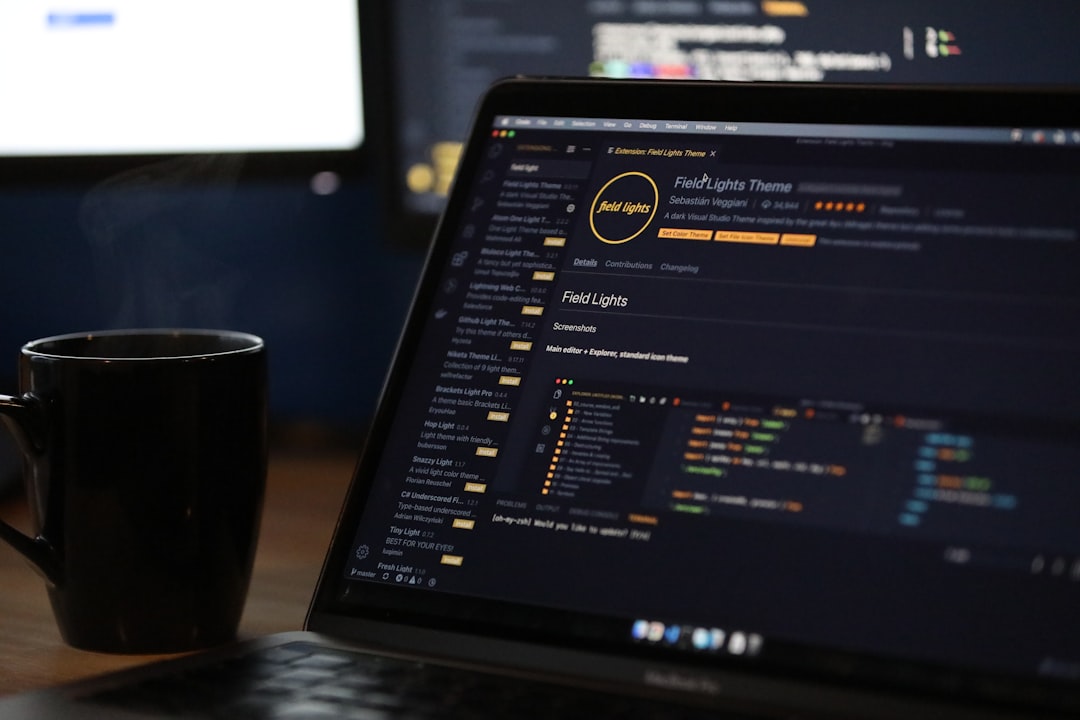
Memory leaks in Java applications occur when objects that are no longer required persist in memory due to lingering references, preventing the JVM's garbage collector from reclaiming the memory. This can lead to performance degradation and even application crashes. Several common scenarios contribute to memory leaks, including unintended retention of object references within collections, static fields, or long-running threads. Even with the JVM's automatic garbage collection, Java applications remain susceptible to memory leaks if not handled carefully.
To prevent these issues, developers should implement strategies to minimize the chances of leaks. Thorough code review and optimization can reveal areas where references are being held unnecessarily. Utilizing weak references, which allow objects to be garbage collected when memory is low, can help manage object lifetimes more precisely. Furthermore, ensuring that event listeners and other callbacks are properly deregistered is vital, as these can often lead to lingering references.
Regularly profiling applications can highlight areas where memory is being used excessively or retained for longer than intended. By identifying these "memory hotspots", developers can address the root cause and refine their code to prevent future issues. It's crucial to stay vigilant in code reviews and to utilize tools to monitor memory usage, which aids in identifying potential memory leaks proactively. This process ensures that Java applications remain stable and performant. Essentially, understanding how memory is managed in Java, in conjunction with consistent vigilance and preventative measures, is fundamental to building high-quality Java applications that can perform without issues.
Java's automatic memory management, while a boon, doesn't eliminate the possibility of memory leaks. These leaks happen when objects are no longer needed but the JVM can't reclaim them because of lingering references. One common culprit is unintentionally holding onto object references, like storing them in static fields or long-lived collections. These unintended references effectively keep objects alive, even when the program no longer needs them, leading to a slow but steady increase in memory consumption.
Event listeners can also be a source of leaks. If we don't unregister listeners when an object's no longer needed, the listeners keep a reference to that object. This means the JVM won't remove the object from memory, leading to a growing memory footprint. Similar problems can arise with `ThreadLocal` variables. If they're not cleaned up properly, they might keep referencing objects as long as the thread they belong to is active. This is particularly problematic in thread pools where threads are constantly recycled.
Dynamically growing collections, like `ArrayList` or `HashMap`, can become a source of memory leaks if not carefully managed. If you keep adding objects without discarding unused ones, the collections continue to hold onto references, essentially hoarding memory.
To address these issues, developers can turn to `WeakReference` to give the garbage collector a hint about reclaiming memory. `WeakReference` only keeps a weak link to an object, which means if there are no other strong references to it, the garbage collector can freely remove it from memory. This is particularly useful for things like caches where we want to store temporary data without blocking memory.
While designed to assist in cleanup, finalizers might actually make the situation worse in terms of memory. Their unpredictable nature can delay memory release. It's best to avoid relying on them heavily for crucial resource management.
Thankfully, developers have tools at their disposal for identifying these sneaky leaks. JVM profilers and specialized tools can help investigate heap dumps and pinpoint exactly where objects are being unnecessarily retained. This detective work aids in identifying the root causes and applying fixes.
However, even with generational garbage collection, memory leaks can persist and become more complex. When short-lived objects somehow migrate to the older generation, they end up staying around longer than intended. This can eventually lead to the older generation being filled, resulting in errors and unexpected application behavior.
Beyond the regular JVM heap, applications utilizing native libraries (through JNI) can suffer from native memory leaks. This is more complex because the JVM's garbage collector doesn't manage this memory.
Weakly reachable objects, those connected to weak references, are supposed to be garbage collected. But a tricky scenario arises with circular references. If a chain of objects links back to itself with some strong references in the loop, the garbage collector might miss the chance to reclaim these objects. This can lead to leaks that are harder to spot because they seem like they should be garbage collected.
Understanding the intricacies of memory leaks and the strategies to mitigate them is crucial. Developing a keen eye for how objects are referenced and understanding the quirks of memory management are key to crafting efficient and stable Java applications. Continuous vigilance and careful testing are essential steps in the pursuit of robust applications.
Understanding Java Virtual Machine (JVM) A Deep Dive into Memory Management and Garbage Collection - JVM Performance Optimization Through Memory Management
JVM performance is significantly impacted by how memory is managed. Optimizing this aspect centers on understanding and fine-tuning the heap, the key area for storing Java objects and arrays. Efficient garbage collection is paramount, and the JVM's generational approach, which distinguishes between short-lived objects (young generation) and long-lived ones (old generation), provides a powerful framework for memory reclamation. Understanding how these generations interact with different garbage collection algorithms is vital for tuning performance.
Furthermore, issues like memory leaks, where unused objects persist due to lingering references, pose a major threat to application stability and responsiveness. Developers can proactively address these concerns by employing practices like utilizing weak references, which allow the garbage collector to reclaim objects more readily, and using tools to profile memory usage. By carefully managing object lifetimes and monitoring memory allocation patterns, developers can significantly reduce the likelihood of memory leaks. A comprehensive understanding of JVM memory management, encompassing both heap tuning and the prevention of memory leaks, is crucial for creating robust, high-performance Java applications that can effectively manage their resources.
The Java Virtual Machine (JVM) is fascinating in how it manages memory and, consequently, impacts application performance. It divides the heap into different generations, like the Young and Old Generations, each with its own specific garbage collection approach. This targeted strategy aims to optimize memory management based on the typical lifespans of objects.
Interestingly, the JVM has a way of analyzing object usage, called escape analysis. If it sees that an object is only used within a single method, it may bypass the heap and allocate it directly on the stack. This can be a sneaky performance boost as it reduces the load on the garbage collector.
Within the Young Generation, there's a system of survivor spaces, temporary holding areas for objects that survive initial garbage collection cycles. This clever dance between spaces helps manage memory more efficiently and lessen fragmentation.
Moreover, developers have the ability to utilize memory outside the traditional heap via `java.nio`, also known as direct memory access. While it offers potential performance advantages for I/O-intensive operations, it places the burden of memory management back on the developer.
Concurrent Mark-Sweep (CMS), one of the garbage collection algorithms, attempts to minimize application pauses during memory cleanup. However, it can result in memory fragmentation, which might lead to performance challenges later on.
The traditional method area has been replaced by Metaspace in modern JVMs. Metaspace uses native memory and can grow as needed, which is a much more flexible approach.
Also, the JVM has specialized techniques for optimizing how smaller objects (< 64 bytes) are managed. Techniques like biased locking and memory pooling can contribute to more efficient heap usage.
Weak references are useful when you want to hold onto objects only as long as they are necessary. These allow objects to be potentially reclaimed by the garbage collector under memory pressure, giving developers a bit more control over the memory footprint.
JVM garbage collection parameters are adjustable, which offers developers a powerful way to customize it. But with great power comes great responsibility. Improperly tweaked settings can have negative consequences on performance, so it is a practice that should be undertaken with care and with the use of profiling tools.
Finally, the `finalize()` method may seem appealing for cleaning up objects. However, it can cause unpredictable delays in the release of resources, potentially leading to unexpected memory retention.
In essence, appreciating these less obvious aspects of the JVM's memory management can greatly influence how Java developers structure their applications. It's about making educated decisions about how objects are created, how long they live, and how memory is used, all leading to a path towards improved performance and greater application reliability.
Understanding Java Virtual Machine (JVM) A Deep Dive into Memory Management and Garbage Collection - JVM Threading Model and Memory Access Patterns
The JVM's threading model and how threads access memory are essential for understanding how Java applications behave, especially when multiple threads are involved. Every Java thread gets its own private stack, creating a separate area for executing code and storing local data. However, all threads share the heap, the area where objects and arrays reside. This shared heap introduces challenges like race conditions, where multiple threads trying to change the same object at the same time can result in unpredictable outcomes. Understanding these memory access patterns is crucial for Java developers when it comes to optimizing multi-threaded code and managing memory efficiently. Developers can significantly improve application performance and reliability by knowing how these memory access patterns work and applying them correctly. Essentially, a strong understanding of how threads and memory interact is fundamental for building robust Java programs in complex, multi-threaded environments.
Here's a rewrite of the provided text about JVM threading model and memory access patterns, in a similar style and length, focusing on the perspective of a curious researcher/engineer, and avoiding repetition of prior content:
The Java Virtual Machine (JVM), a runtime environment that executes Java bytecode, presents an intriguing array of features related to thread management and memory access patterns. Exploring these features reveals both opportunities and challenges for developers aiming to build high-performance, robust applications.
Let's consider a few points that stand out in this fascinating interplay. For starters, each Java thread maintains its own private stack, a dedicated memory region for local variables and method calls. This arrangement simplifies context switching—the process of switching between threads—because the JVM only needs to manipulate stack pointers, significantly reducing the overhead of moving or copying large data structures between threads. It's a remarkably efficient system for handling concurrent tasks.
However, efficient as this is, it's vital to recognize how the Java Memory Model (JMM) plays a role in ensuring that changes made by one thread are visible to other threads. Without mechanisms like synchronization, this memory visibility isn't guaranteed. This leads to the possibility of data races, where threads attempt to access and modify shared memory without coordination, potentially leading to unpredictable and incorrect application states. It's a bit of a double-edged sword, as it adds a layer of complexity for developers to manage, but provides the necessary platform to allow efficient multitasking.
Further exploration leads to the intriguing aspect of escape analysis. The JVM is clever and constantly looking for ways to optimize program behavior. With escape analysis, the JVM examines the usage of an object to determine if it's confined to a single thread or if its visibility extends to multiple threads. If it's used within just one thread, the object's scope is limited, and the JVM can potentially allocate it on the thread's stack. This move can result in performance gains, because it reduces the reliance on the heap and garbage collection.
But this thread-specific performance advantage comes at a cost in situations where the JVM requires garbage collection to reclaim memory. During garbage collection, program execution can pause, which can be a significant challenge in latency-sensitive applications like real-time systems. Developers carefully choose their garbage collection algorithm to minimize pauses and reduce the potential for negative user experience.
The `ThreadLocal` class also offers interesting functionality. It lets each thread maintain its own instance of a variable. This seems quite handy, but if not carefully managed, it can lead to excessive memory use, particularly in cases where a thread remains active for a prolonged period. A bit like having a storage shed for each worker. Each shed is handy, but if each has stuff in it that is never used, it simply increases the area to maintain.
Synchronization blocks, while necessary for enforcing order and safety in multi-threaded environments, often come at the expense of performance. When contention for locks becomes high, threads can experience significant delays waiting to acquire a lock. In essence, if you have many workers, each needing access to the same tools in a limited area, things can get backed up quickly.
However, on the performance front, atomic operations using classes like `AtomicInteger` provide a unique benefit. These classes employ special CPU instructions that eliminate the need for locking during reads and writes to certain variables. This capability delivers improved performance when working with variables across multiple threads, especially in scenarios where a few threads are rapidly updating or reading shared data.
Sometimes, threads may operate on different pieces of data that happen to reside in the same cache line. In this circumstance, an unfortunate phenomenon called "false sharing" can crop up. The threads could potentially interfere with each other's cache entries, leading to unnecessary cache misses and decreased performance.
The JVM leverages memory barriers to maintain consistency among threads. Memory barriers introduce constraints that control the order of instructions and ensure that changes to variables are seen correctly by all threads involved. Without careful management, a multi-threaded system could lead to subtle bugs that may appear to be unrelated to memory access, but in actuality, they are caused by a specific memory access occurring before or after another one.
Finally, in the realm of memory allocation, although the heap is shared across all threads, the JVM has its own set of techniques to improve how memory is managed within it. For instance, memory might be separated for small versus larger objects to prevent fragmentation and to improve allocation performance for smaller objects.
Collectively, these observations and complexities underscore the multifaceted nature of threading and memory management within the JVM. Developers who are able to recognize these complexities and to appropriately consider them during the development lifecycle will likely be able to craft applications that perform to the level that they intend.
Create AI-powered tutorials effortlessly: Learn, teach, and share knowledge with our intuitive platform. (Get started for free)
More Posts from aitutorialmaker.com: