Understanding Ruby's Block-to-Proc Conversion A Deep Dive into &block Syntax
Understanding Ruby's Block-to-Proc Conversion A Deep Dive into &block Syntax - Block Passing Methods in Ruby code A Foundational Overview
Ruby's method design incorporates the concept of block passing, a feature central to the language's flexibility and dynamism. Methods can accept code blocks as input, enabling the execution of custom code segments using the `yield` keyword. Essentially, `yield` acts as a mechanism to invoke the block that was passed in. This approach, known as behavior injection, lets you tailor method functionality without altering the core method structure. While `yield` provides a simple, implicit way to use blocks, Ruby offers a more explicit way using `Proc` objects. These objects encapsulate blocks of code, giving developers the ability to store, pass, and manage code segments just like any other variable. This strengthens the functional programming paradigm within Ruby, allowing for more reusable and modular code. The benefits of this approach are numerous. Not only can you separate concerns efficiently, you can also adapt your methods to different circumstances without the need for extensive rewriting. However, it's worth mentioning that while block passing expands the options, it also introduces complexities that one needs to be aware of when crafting applications. It's imperative to grasp the subtle differences between passing data and passing behavior in the context of block passing. It's a fundamental shift in how methods can operate, significantly influencing the overall expressiveness and versatility of Ruby code.
1. Passing blocks into methods in Ruby promotes code reuse by avoiding redundant code snippets across different parts of a project. This fundamental aspect significantly improves maintainability, a critical concern in large and complex codebases.
2. Ruby's block-passing mechanism isn't just a convenient syntax; it's a fundamental component of the language's functional programming paradigm. It enables developers to treat code itself as a primary entity, paving the way for advanced techniques like higher-order functions.
3. Ruby's `&block` syntax provides a straightforward way to convert blocks into procs, leading to greater flexibility when dealing with behavioral elements. Comprehending this mechanism helps developers write more intricate and expressive code.
4. An intriguing aspect of Ruby's block passing is its potential impact on closure performance. While blocks are usually more memory-efficient than procs, using them carefully within the appropriate context is crucial to avoid unanticipated side effects.
5. The ability to pass blocks as arguments is a unique feature in Ruby compared to many other languages, making the management of callbacks and asynchronous operations more elegant. This can lead to cleaner and more compact code.
6. Though blocks in Ruby are instances of the `Proc` class, they have a more lightweight nature. While procs and functions share similarities, their subtle differences become significant when understanding the impact on memory consumption and performance in different scenarios.
7. Ruby automatically transforms single-block parameters into the `&block` syntax, resulting in a more intuitive method call process. However, this feature can lead to surprising outcomes if developers are unclear about the method's design and the expected types of input.
8. The scope within which a block operates is crucial because blocks can capture variables from the enclosing context, resulting in closures. This feature allows for effective programming strategies but requires careful consideration to prevent unintended interactions with the surrounding environment.
9. Developers working with Ruby blocks can leverage the `yield` keyword to smoothly shift control between the calling method and the block. This capability offers precise control over when and how code is executed.
10. A deep understanding of block passing and its conversion processes opens doors to optimization, especially in situations where performance is paramount. Developers can reduce overhead and improve code clarity by employing blocks effectively.
Understanding Ruby's Block-to-Proc Conversion A Deep Dive into &block Syntax - Understanding The & Symbol and Block Parameter Syntax
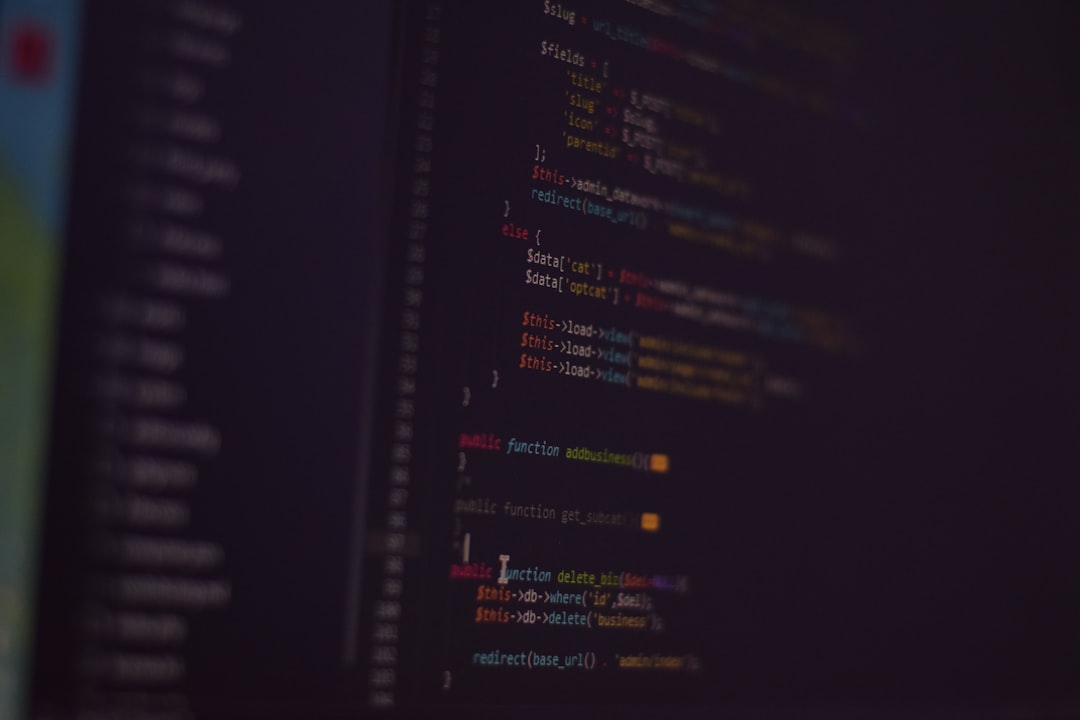
Within Ruby, the ampersand (&) symbol plays a pivotal role in handling blocks of code passed to methods. When placed before a method parameter, the & signifies that the method anticipates receiving a block as an argument. This approach, known as the &block syntax, provides a structured way for methods to interact with code blocks.
Essentially, methods can now accept code snippets, similar to how they would handle data arguments. The `yield` keyword within the method then enables the execution of this passed-in block. Converting blocks into `Proc` objects through the & symbol enhances the versatility of blocks, effectively treating them as first-class citizens within Ruby. This conversion allows for storage and manipulation of these blocks, furthering the possibilities of functional programming within the language. It encourages a style of programming where behaviors themselves can be passed, reused, and manipulated. This, in turn, leads to more modular and flexible code in your projects.
Understanding how to use the & symbol and the related syntax allows developers to write Ruby code that's more expressive and readily adaptable. It provides a crucial pathway towards constructing more sophisticated and organized programs.
The `&` symbol, particularly in the context of `&block`, provides a mechanism for seamlessly converting blocks into Proc objects. This capability empowers Ruby developers to define and apply callable code snippets dynamically across different parts of their programs. While seemingly simple, blocks and procs have subtle distinctions in how they interact with variable scope. This difference can lead to unforeseen behavior, particularly when dealing with variable bindings, highlighting the need for a deeper understanding.
From a memory standpoint, Ruby's blocks are generally more frugal. They don't introduce an extra level of scope like procs, promoting memory efficiency. This can be valuable in situations where performance is critical. Ruby's handling of block parameters makes callbacks, especially essential in asynchronous programming, more manageable. This results in significantly cleaner code compared to the traditional callback functions found in many other languages.
However, the implicit conversion of single arguments to blocks, a feature of Ruby, can also be a source of confusion. A method expecting a block might be called without explicitly providing one. While this provides a degree of flexibility, it's an area that can lead to confusion for new Ruby developers.
Blocks can capture variables from their enclosing environment, a concept called closures. This feature offers incredible power but can introduce challenges. Developers must consider carefully the implications of variable shadowing and potential unintended consequences in the surrounding context.
The `yield` keyword, central to block execution, serves a dual purpose: it transfers control and enables a form of simple context switching. This lets developers create adaptable methods that can behave differently based on the block supplied to them.
While the flexibility of passing blocks encourages rapid development, it demands a strong understanding of Ruby's execution path. It's crucial to avoid potential pitfalls, including unexpected late binding and undue reliance on external variables.
Delving into the details of block-to-proc conversion significantly impacts application optimization. Developers need to carefully consider how various code paths and memory allocations influence runtime performance.
The emphasis on blocks encourages a more functional approach, fostering code that is often more expressive and declarative. This can challenge conventional object-oriented programming styles, pushing Ruby developers to devise innovative problem-solving strategies.
Understanding Ruby's Block-to-Proc Conversion A Deep Dive into &block Syntax - Converting Blocks into Proc Objects Step by Step
Ruby's ability to convert blocks into Proc objects is a key element of its flexibility. This conversion process allows you to treat blocks, which are essentially anonymous functions, like regular objects. The core mechanism for this conversion is the ampersand operator (`&`). By applying `&` to a block, you transform it into a Proc object. This Proc then becomes storable within variables and passable to other methods, just like any other Ruby object. This added flexibility is beneficial when working on larger, more complex projects where the ability to store and reuse specific blocks of code is desirable.
However, this increased power comes with a small cost. Using Procs can often create a slight performance overhead compared to using blocks directly. This difference, though minor, underscores the importance of considering your application's needs. It's important to evaluate if the added benefit of manipulating blocks as objects outweighs any potential performance impact. For code where efficiency is paramount, blocks might be the preferred choice.
A clear understanding of how Ruby converts blocks to Procs, particularly the use of `&` and the `call` method to invoke them, is valuable for developing more sophisticated and adaptable Ruby code. It allows you to think about code segments in new ways, leading to increased code reusability and better overall program organization.
1. Understanding how Ruby transforms blocks into `Proc` objects is fundamental because it enables developers to treat code as data—a key concept in many functional programming approaches. This transition can significantly refine how we design and implement methods, ultimately boosting code quality.
2. The `&block` syntax not only encapsulates behavior but also boosts Ruby's expressiveness, allowing for cleaner and more declarative code. This is particularly helpful when dealing with complex logic, as it encourages more abstraction.
3. Every time a block becomes a `Proc`, a new object is created with its own context and state. This characteristic can inflate memory usage if not handled carefully, particularly within loops. It's crucial to be aware of the potential for increased memory consumption when repeatedly converting blocks to procs.
4. Ruby blocks are closures capable of accessing variables within their scope, but when transformed into `Proc` objects, they capture variable bindings at the moment of conversion. This subtle detail is crucial to understanding and preventing bugs linked to variable shadowing in nested scopes.
5. Block-passing offers flexibility, supporting features like mixins and callbacks, but it can lead to confusion if the programmer doesn't fully grasp late binding, which happens when variables relied on change unexpectedly. This can introduce tricky bugs.
6. `Proc` objects come with a slight performance overhead that blocks don't have, primarily due to their extra scope. When performance matters, using blocks might be more efficient than procs, especially in performance-critical applications.
7. Ruby's `&` syntax allows blocks to be passed as first-class objects, fundamentally changing how methods can interact with code. This functional aspect helps developers create more modular and easily testable code, encouraging better software design practices.
8. The design of block-to-proc conversion supports Ruby's goal of reducing boilerplate code, potentially speeding up development. However, it's important to balance this benefit with the cognitive load of understanding when blocks are preferable to standard method arguments.
9. Ruby's block-passing approach is somewhat different from many other languages because it supports implicit block conversion without needing specific arguments. This quirk can create inconsistencies in method behavior that might seem counterintuitive, particularly for developers accustomed to other languages.
10. Viewing blocks as flexible tools for organizing code pushes Ruby's programming model into areas often dominated by functional languages, hinting at a more elegant coding style while simultaneously challenging the traditional object-oriented paradigms ingrained within Ruby.
Understanding Ruby's Block-to-Proc Conversion A Deep Dive into &block Syntax - Method Arguments with Block to Proc Transformation
Method Arguments with Block to Proc Transformation
Ruby's ability to transform blocks into Proc objects using the ampersand (&) operator significantly impacts how we handle method arguments. By employing the & symbol before a parameter, we signal that a method is designed to accept a block. This transformation effectively converts the block into a Proc, making it a fully-fledged object that can be passed to other methods, stored in variables, and treated like any other Ruby object. This allows methods to seamlessly incorporate customized behaviors without needing changes to their core structure, essentially enabling "behavior injection" within the method's logic.
However, this dynamic behavior comes with potential trade-offs. The overhead of converting blocks to Procs can impact memory and performance, particularly in performance-critical situations. Further, it introduces considerations about variable scope and binding, which might lead to confusion if not managed carefully. While the &block approach promotes code flexibility and modularity, it's essential to understand these implications to write clean, efficient, and reliable Ruby code. It's not just about using the ampersand. It's about realizing that the act of converting a block to a proc transforms its behavior in a way that one needs to understand for it to be helpful.
1. The conversion of blocks to `Proc` objects in Ruby introduces a noteworthy aspect of memory management. While blocks are often more memory-efficient, `Proc` objects, due to their context retention, can potentially increase memory usage if not carefully managed, especially within loops or recurring calls. This is something to be aware of during development.
2. The `&` operator used with method parameters implicitly signals Ruby to convert a block into a `Proc`. While this feature provides convenience, it also creates the potential for unforeseen issues if developers aren't mindful of the context switching and variable bindings that occur. Inattentiveness here can lead to bugs that might be challenging to identify.
3. In contrast to how some languages treat functions as unchanging elements, Ruby's treatment of blocks adds another layer of complexity. When blocks are converted to `Proc` objects, they capture their surrounding environment during the conversion process. This can sometimes lead to confusion about which variables are accessible later on in the code's execution.
4. Upon conversion into a `Proc`, blocks gain the ability to be passed and stored much like any other object in Ruby. This supports more advanced programming styles, such as higher-order functions, making Ruby's functional programming approach a bit different from the more traditional object-oriented paradigms found in other languages.
5. While the use of blocks and procs encourages more modular and reusable code, it also subtly encourages a shift in thinking towards a functional approach. This can prompt Ruby developers to rethink their approach to traditional object-oriented programming in a way that may ultimately result in cleaner and more maintainable code.
6. When discussing the conversion of blocks to `Proc` objects, performance considerations are essential. Although the performance overhead of using `Proc` objects is often minimal, it's possible that it can accumulate in performance-critical parts of a program. As a result, developers need to carefully weigh the benefits of this flexibility against the potential for performance impact in certain situations.
7. The `&` syntax not only facilitates the transformation of blocks into `Proc` objects, but it also acts as a bridge between the procedural and functional aspects of Ruby's programming capabilities. This duality enables methods to become more expressive and adaptable, potentially leading to more sophisticated software architectures.
8. Closures in Ruby, specifically in the context of the block-to-Proc mechanism, give developers finer control over how methods interact with variable scope. While this is powerful, it also presents potential risks. Developers need to be cautious of issues such as variable shadowing and unintended side effects.
9. Ruby's handling of blocks and procs is somewhat unique compared to how other languages like JavaScript or Python approach the concept. This difference can occasionally lead to confusion, particularly for those developers transitioning from other languages.
10. The enhanced expressiveness that results from block-to-Proc conversion helps to write cleaner and more maintainable code, as well as making it possible to implement complex programming structures. This makes Ruby suitable for both rapid prototyping and the development of more complex and robust applications.
Understanding Ruby's Block-to-Proc Conversion A Deep Dive into &block Syntax - Performance Implications of Block Conversions in Ruby
When Ruby converts blocks into Proc objects, it gains flexibility—blocks become storable and reusable like any other object. However, this flexibility comes with a performance trade-off. Converting a block to a Proc can increase memory consumption and potentially introduce a performance overhead, especially when compared to using the `yield` keyword.
In simpler code structures, using `yield` directly is usually faster than the block-to-Proc conversion. But as methods become more nested, the performance difference between these two approaches can change. The exact impact on performance is situation-dependent, influenced by factors like the code's structure and how deeply blocks are passed as arguments.
Furthermore, converting blocks to Procs requires careful consideration of variable scope and binding. This conversion can sometimes lead to unforeseen effects, particularly when dealing with variables that might change unexpectedly. Issues like variable shadowing can become a real concern, potentially introducing tricky bugs that can be hard to track down.
Overall, the decision of whether to convert blocks into Procs should be a careful balancing act. While the conversion's flexibility can lead to more adaptable and reusable code, it's important to understand the potential performance cost and the complexities around variable scope and binding that it introduces. A conscious effort to weigh the benefits against the trade-offs ensures that you write efficient and reliable Ruby applications.
1. Ruby's clever block-to-proc conversion seamlessly integrates with its object model, enabling developers to treat blocks like any other object. This offers more flexible method design compared to languages where functions are often treated separately.
2. While the automatic conversion of blocks to Procs is convenient, it can blur the line between method arguments and blocks. This can be confusing, especially for developers coming from languages with stricter function handling.
3. Each block conversion to a Proc creates a new object, each with its own environment. This highlights the need to carefully consider memory usage, as frequent conversions within loops can increase memory consumption, impacting performance.
4. Capturing variables from the surrounding scope introduces the intricacies of Ruby's closure mechanism. Although handy, it can create unexpected behaviors if the surrounding context changes due to issues like variable shadowing.
5. Ruby's block-centric approach encourages a functional programming style, emphasizing immutability and less reliance on mutable state. This often leads to cleaner, more modular code that is easier to test and maintain.
6. Converting blocks to Procs can bypass some of Ruby's internal optimizations for regular method calls. It's crucial to assess performance implications in situations where speed is a major concern.
7. Transforming blocks into Procs unlocks the ability to chain and pass multiple behaviors, improving code clarity. However, this requires a firm grasp of the underlying mechanics to avoid unintended consequences due to shared state.
8. The `&` operator's role in conversion isn't just about transformation—it also provides a clear indication of the method's intent. This is beneficial for team projects and large codebases where consistent and easy-to-understand code is crucial.
9. Blocks are generally more lightweight and memory-efficient than Procs. However, the convenience of conversion might lead to overuse, resulting in unnecessary memory consumption, which can be a problem in resource-constrained or real-time applications.
10. Understanding block-to-proc conversion is a key to writing efficient and clear Ruby code. Using this mechanism wisely helps developers balance expressiveness and performance, improving both the readability and execution speed of their applications.
Understanding Ruby's Block-to-Proc Conversion A Deep Dive into &block Syntax - Block Scope and Variable Binding in Ruby Procs
Within Ruby's programming model, comprehending how blocks interact with scope and variable bindings is essential, especially when working with procs. Blocks, by design, inherit the scope of their surrounding environment at the moment of definition. This means they can access the variables defined in that environment. However, it's important to note that any modifications to these variables after the block's creation will not be reflected within the block itself. This behavior underscores the concept of closures in Ruby—blocks can encapsulate and retain the state of their environment, a powerful feature that can lead to dynamic code.
However, this power comes with a potential for confusion. When a block is converted to a proc object, it gains more flexibility, becoming a first-class object that can be stored, passed around, and invoked like any other Ruby object. This ability enhances the functional nature of Ruby, but also introduces complexities regarding memory management and how variables are bound. It's crucial to understand how the conversion of a block to a proc impacts the environment it interacts with.
While the use of blocks and procs is a core part of the Ruby experience and significantly expands the range of what a developer can do, mastering the nuances of scope and variable binding is crucial for building robust and predictable programs. A careful understanding of how variable environments influence the behavior of blocks and procs is vital for leveraging the power of this feature within your Ruby applications.
Ruby's blocks possess the interesting characteristic of accessing variables from their surrounding environment, resulting in what we call closures. This ability makes our code more flexible, but it can also introduce complexities with unexpected variable changes and a more intricate scope management.
The `&` symbol in Ruby goes beyond simply marking a block parameter; it's actually responsible for transforming the block into a Proc object. This transformation gives us a powerful tool that allows us to store and reuse blocks, and it opens up advanced programming techniques similar to those you'd find in functional programming paradigms.
Unlike some other languages, Ruby's handling of blocks and Procs relies on implicit conversion without explicit type definitions. While flexible, this feature can be a source of confusion when the expectations around method parameters and block acceptance don't match up.
Every time a block morphs into a Proc, Ruby creates a new object that holds onto the original block's environment. In situations like loops where this conversion occurs frequently, we can end up with more memory consumption than we anticipated. For performance-sensitive apps, it's worth paying attention to this aspect.
While blocks are generally known for being more efficient in terms of memory usage, excessive block conversion can potentially lead to memory bloat, especially in prolonged Ruby processes. Careful attention to resource management becomes crucial.
Ruby's closure mechanism captures the variable bindings present at the moment of the conversion. This can create complications if the variables we're referencing later change in a way we don't anticipate outside the block's scope, potentially leading to debugging nightmares.
Because Ruby pushes us toward writing elegant code, developers who make use of blocks and Procs should be wary of the phenomenon of late binding. Essentially, the context of a variable when the block is finally executed might differ from its state at the time the block was first defined. This difference can lead to code that doesn't behave as intended.
Performance-wise, using `yield` directly is usually more efficient than calling a block as a Proc, particularly when we're dealing with scenarios like recursion or deeply nested methods. The choice between blocks and Procs can significantly affect our application's speed.
Ruby's block-centric approach encourages programming styles that emphasize immutability and reduced side effects. This leads to code that's often clearer and easier to test. This perspective starts to nudge developers towards a more functional style of coding, embracing ideas like higher-order functions.
By grasping the subtleties of how blocks are transformed into Procs, we can build more sophisticated and reusable code. But we also need to remain alert to potential issues related to memory usage and scope management. This careful approach leads to creating more robust and dependable Ruby programs.
More Posts from aitutorialmaker.com: