Unveiling the Mystery 7 Common Causes of 'TypeError int object is not iterable' in Python
Unveiling the Mystery 7 Common Causes of 'TypeError int object is not iterable' in Python - Attempting to Loop Through a Single Integer Value
Python's for loops expect a collection of items, or an iterable, to step through one by one. However, a single integer isn't structured like that. It's just a single value, not a sequence. Attempting to use a plain integer in a loop, expecting it to behave like a list or a range of numbers, will result in Python throwing a "'TypeError: 'int' object is not iterable'" error. This error highlights the fundamental difference between single values and those suitable for iteration. To address this, consider converting your integer into an iterable, typically achieved with the `range()` function. This creates a sequence of numbers up to the integer's value, fulfilling the loop's need for something to iterate through. Recognizing the distinction between an integer and iterables is a vital aspect of avoiding this common mistake in Python.
When you try to loop through a single integer in Python, you'll encounter a "TypeError: 'int' object is not iterable" error. This happens because integers, unlike lists or strings, aren't designed to be iterated over. They represent single values, not a collection of items. It's a core principle of Python's typing system that helps catch errors early.
This distinction between iterable and non-iterable objects is quite significant. Understanding this separation helps us avoid issues later in the program. We must be mindful of the data type we are working with at all times. It’s about more than just avoiding the error; it leads to faster debugging if we have a better understanding of how the system operates.
The 'for' loop and related operations (like list comprehensions) rely on objects that support the `__iter__()` or `__getitem__()` protocols. Integers, being a fundamental data type, simply don't have these features, meaning you cannot directly loop over them. It's not just a matter of the loop failing, it's the whole architecture that prohibits it.
The error not only points out the problem but also reinforces the type-specific nature of Python. Other languages might be more forgiving, but in Python, you're forced to be conscious about how data is used. It promotes cleaner code but may require a shift in thinking compared to languages with more implicit conversions.
For example, if you mistakenly expect an integer to contain multiple items, you'll likely run into logical errors in addition to the `TypeError`. This really emphasizes the importance of understanding the different data structures. In Python, the 'iterability' of an object stems from protocols that guide how they interact with different operations. This knowledge helps us think more strategically when designing data structures and writing code.
This specific `TypeError` provides a helpful learning experience. The message is directly related to the type system. It serves as a valuable educational point in the overall evolution of a programmer.
One workaround if you need to iterate over an integer-like entity is to transform it into a list or a similar collection. It shows how flexible Python can be; you have the ability to alter how data is represented.
The fact that attempting to loop over an integer causes an error like this is a basic example of the more fundamental issues in programming. It highlights the idea of core principles in the object-oriented programming paradigm. It's easy to take these concepts for granted when everything works, but when you hit an error like this, the core concepts really come into focus.
Unveiling the Mystery 7 Common Causes of 'TypeError int object is not iterable' in Python - Misusing the range() Function with Non-Integer Arguments
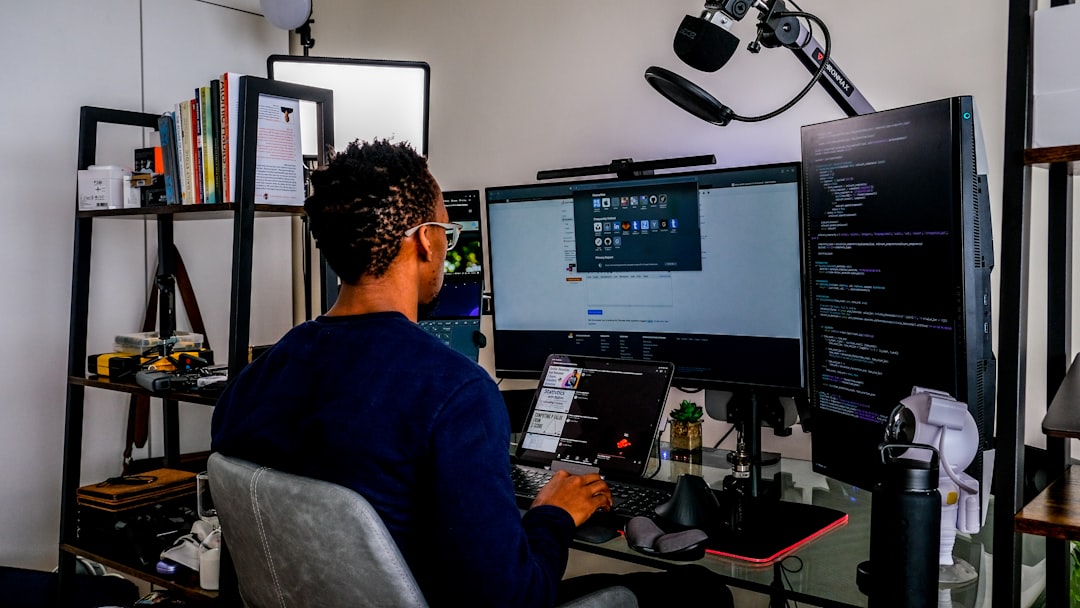
The `range()` function, a cornerstone of Python's looping mechanisms, expects integer arguments to define the sequence it generates. Providing it with anything else, such as a string or a floating-point number, can lead to a "TypeError: 'int' object is not iterable". This happens because `range()`'s core purpose is to produce an ordered sequence of integers, and when given a non-integer, it struggles to fulfill this task. It's akin to trying to build a staircase with mismatched pieces; the structure becomes inconsistent and unusable. To avoid this, you need to ensure that the arguments you pass to `range()` are indeed integers. If you encounter a different data type, consider converting it to an integer before passing it to the function. Simple adjustments like these can prevent this error, promoting smoother and more reliable code execution.
The `range()` function in Python is designed to work with integer arguments, generating a sequence of numbers. However, if you try to provide it with non-integer values like floats or strings, you'll encounter a `TypeError`. This strictness regarding data type is inherent to Python, and it's important to be mindful of this. Even if you cast a float to an integer using `int(3.5)`, it might not behave as expected within `range()`, as it truncates the decimal part instead of rounding, potentially causing unexpected loop iterations.
Python 3's `range()` returns a range object, different from Python 2's list-like behavior. This change can lead to confusion when working with non-integers, especially if you're accustomed to older versions of Python. It's crucial to be aware of the current behavior and avoid trying to force compatibility in situations where it's not defined.
One of the reasons `range()` is designed this way is memory efficiency. It generates values on demand instead of storing them all at once. If you attempt to coerce a non-iterable type into `range()`, you interfere with this efficient operation, possibly creating performance problems in your program.
Further complications arise when `range()` is used within a function. A mismatched argument, say a string, can trigger errors in later parts of the code. It's a reminder of why robust data validation is essential before executing a loop or any iteration. When dealing with more complex structures, mishandling `range()` with non-integer types can make debugging a nightmare. The ripple effect of a single misplaced variable can throw off the entire looping process, highlighting the necessity of tracking data types across your code.
This issue often crops up in real-world scenarios when handling user input or external data. Sometimes, data from unexpected sources sneaks into your functions, causing a crash if you weren't prepared. It serves as a great example of why defensive programming is important. Python’s flexibility allows for creating custom iterable classes. Misuse of `range()` with a non-integer argument acts as a reminder of why certain type requirements exist for both built-in and user-defined iterables.
Additionally, if you're not careful, using `range()` with floating-point numbers can unintentionally create an enormous number of iterations, potentially crippling your program’s performance. It emphasizes the importance of carefully considering how you're using this function. The use of `range()` reveals certain facets of Python's meta-programming aspects. Understanding why type errors occur when it's misused leads to a richer understanding of how decorators and protocols work, giving you a more refined grasp of how Python is designed.
Unveiling the Mystery 7 Common Causes of 'TypeError int object is not iterable' in Python - Forgetting to Convert User Input to a List or Tuple
When you gather user input and don't convert it into a list or tuple before trying to loop through it, Python will often respond with the "TypeError: 'int' object is not iterable" error. This happens because Python's loop mechanisms expect to work with a sequence of items, not a single integer. If user input is treated as a single number instead of a collection, the program can stumble and fail to execute correctly. This type of error emphasizes the importance of preprocessing user data to ensure it is in the format your program expects. It's crucial to validate and transform user input into appropriate data structures, either using specific conversion functions or robust error-handling mechanisms. Understanding how various data types interact with Python's core functionality is vital for writing efficient and error-free code. If you want to iterate, you need to treat the user input as a list or tuple first. Making sure the data has the proper format early on helps avoid unexpected issues and makes your programs more reliable.
Forgetting to transform user input into a list or tuple can lead to sneaky errors, particularly when expecting the input to behave like a collection of items. For example, if a user enters a string of numbers without separating them, and you attempt to use that string directly in a loop, Python will raise a `TypeError`, causing the program to crash.
The importance of ensuring inputs are in an iterable form can't be overstated; it's a direct consequence of Python's strong typing rules. When programmers skip this conversion, they risk encountering not just TypeErrors but also logical errors, stemming from incorrect assumptions about the data structure.
Python promotes explicit type handling, often necessitating active intervention by developers to manage user input. This approach contrasts with some loosely typed languages where automatic conversions might let incorrect code execute without immediately causing errors.
It's easy to mistakenly assume that all strings are iterable, but their behavior significantly differs from that of lists or tuples. When you iterate over a string, each individual character becomes an element, not the intended group of values that a list or tuple would provide.
The dynamic nature of Python allows user input to take diverse forms—integers, strings, and more—but if you don't handle these variations appropriately, it can cause unforeseen issues. Developers need to carefully consider the types of data that will be input into their functions, especially in applications where user interaction plays a key role.
When working with library functions that anticipate iterable inputs, passing an unconverted single integer or a raw string can disrupt the program's flow. Recognizing the need for conversion becomes crucial, especially when combining different data sources or utilizing third-party libraries that have stricter type restrictions.
Error messages generated by forgetting to convert user input often serve as a teaching opportunity, emphasizing the significance of being aware of data structure in Python coding. This allows new programmers to grasp core data type concepts and their consequences earlier in their coding journeys.
One practical solution to minimize these type errors is to develop input validation and conversion functions. Creating a utility that inspects the input type and transforms it into the desired format can streamline the development process and strengthen the overall code's reliability.
A key design consideration is that the failure to convert input can propagate TypeErrors to related parts of your program, making debugging and maintenance more complex. This chain reaction underlines the importance of developers consistently tracking data types throughout their code.
Finally, mastering the proper conversion of user input not only improves functionality but also enhances performance. An optimized type-handling approach can result in more efficient program execution, preventing unnecessary overhead associated with poorly managed input types.
Unveiling the Mystery 7 Common Causes of 'TypeError int object is not iterable' in Python - Accidentally Returning an Integer Instead of an Iterable
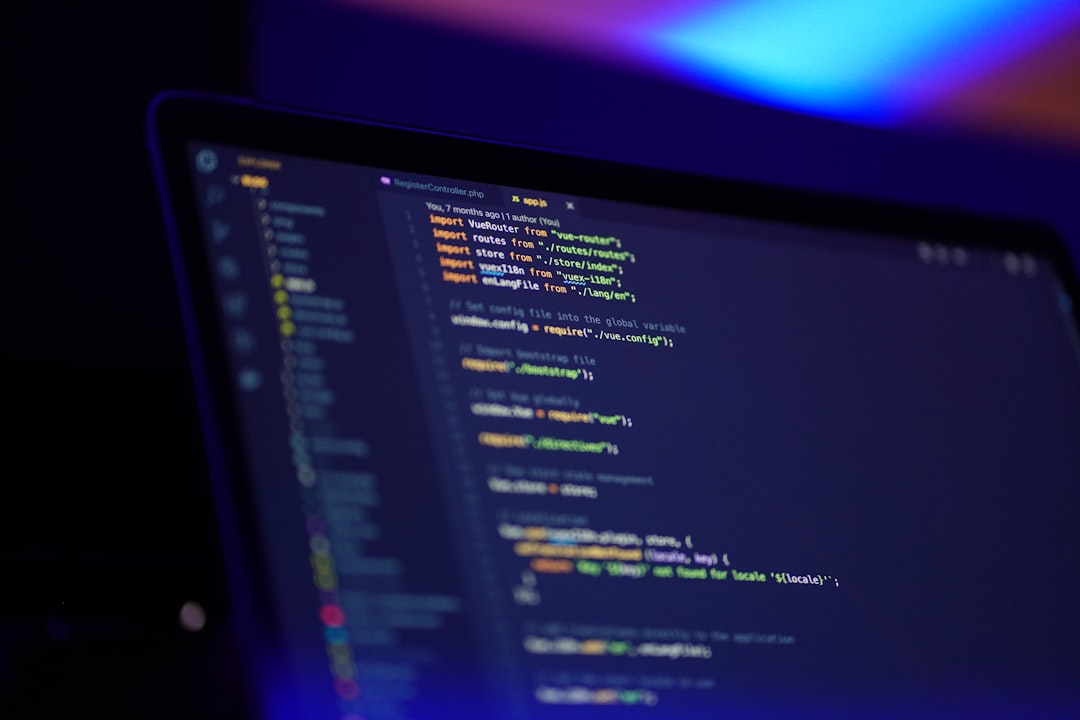
In Python, a frequent coding mishap involves inadvertently returning an integer when a function or operation is expected to yield an iterable. This often results in the infamous "TypeError: 'int' object is not iterable" error. This error crops up when your code tries to loop through or process an integer as if it were a list, tuple, or some other collection of items. The issue commonly occurs when a function like `len()` unintentionally returns a single integer value instead of a sequence or when user inputs are not properly converted to an iterable data structure before being looped through.
To sidestep this error, it's critical to double-check that functions are returning the correct data type. In cases where a function logically should only return a single integer, you might need to manually convert it into a list or a suitable iterable using methods like `range()`. Keeping a keen eye on the types of data your code handles is a crucial skill for debugging and preventing crashes. It's a core element of clean and efficient programming. Understanding how and why certain data types are meant to be iterable can help improve the quality and efficiency of your programs in Python.
1. Integers, unlike lists or dictionaries, are fundamental units of data representing a single value. They inherently lack the structure to hold multiple items, making them unsuitable for iteration. This difference is essential to understanding how Python handles data types.
2. Python's type system enforces strict rules. Any operation designed to handle iterables will trigger a `TypeError` if presented with an integer. This rigidity can surprise developers accustomed to looser type checking in other languages.
3. Variable names can be deceptive. If a variable called `numbers` actually holds an integer, it can easily lead to assumptions in the code, causing errors that are difficult to spot due to the mismatched data type.
4. When an integer is returned instead of an iterable, it may indicate a deeper problem within the logic of your code. Encountering this `TypeError` is a learning opportunity. It pushes developers to consider the overall consequences of how they transform and employ data.
5. Loops designed to work with iterables can mask errors if they incorrectly anticipate multiple values from an integer. When this assumption isn't addressed, it can result in unintended looping or failures, where a precise understanding of the data flow is crucial.
6. Preventing the error often involves a proactive approach. Checking the data type of variables before using them in loops can avoid these situations, improving code quality and promoting smoother program execution.
7. Python emphasizes clarity. Being acutely aware of the repercussions of returning an integer when an iterable is expected promotes more self-explanatory code.
8. Functional programming is increasingly important in Python. Functions should ideally return predictable types. If you return an integer when an iterable is required, it breaks this pattern, and the type error highlights a significant concept in programming fundamentals.
9. Generators are a Pythonic tool to yield data sequentially. It allows for the efficient return of many values. However, misinterpreting a sequence of integers generated this way as a true iterable can lead to incorrect conclusions about how your data is handled.
10. The `TypeError: 'int' object is not iterable` isn't just a roadblock; it's an educational event. It reinforces the significance of grasping data types in programming. These types of errors can stimulate deeper learning of the interplay between data structures and the algorithms that use them, strengthening a programmer's toolkit.
Unveiling the Mystery 7 Common Causes of 'TypeError int object is not iterable' in Python - Confusion Between Index and Iterable in List Comprehensions
Within list comprehensions, a frequent source of the "TypeError: int object is not iterable" error stems from muddling the roles of indices and iterables. Programmers sometimes inadvertently attempt to iterate over an integer, perhaps an index, where Python expects an iterable like a list or a string. This error underscores the need to clearly differentiate between the purposes of index values and the actual items being iterated over within a list comprehension. Understanding this distinction is fundamental to avoiding this error and, more broadly, improving coding practices in Python. Since Python's type system values explicitness, cultivating awareness of the different roles played by indices and iterables can significantly enhance code quality and efficiency, reducing both the incidence of errors and the time spent debugging them.
List comprehensions, while a powerful feature in Python, can sometimes lead to confusion when the distinction between indices and iterables is blurred. The core issue is that a list comprehension needs an iterable object to work with, and mistakenly providing an integer instead triggers a `TypeError`. This error reinforces the idea that Python has a strict type system, preventing you from operating on fundamentally different types of data without explicit conversions.
Python's indexing, starting at 0 for the first element, can further add to this confusion. Sometimes, we might inadvertently treat an integer index like it's a collection of items itself. This tendency can lead to issues, particularly when you combine it with how Python handles data types. In this way, `TypeError`s can be seen as educational moments, highlighting Python's internal type-checking mechanisms.
One of Python's design strengths is that it’s type-safe. When you try to do something that is fundamentally incompatible, like using an integer in a for loop that requires an iterable object, Python stops the program and gives you a useful error message. This strictness can seem frustrating at times, especially if you're coming from other languages with more lax typing rules. However, this rigid type checking is what helps catch many errors at an early stage of development, leading to a smoother debugging experience later on.
Beyond the debugging aspect, performance considerations become relevant as well. Using an integer when you need an iterable can lead to unexpected or extra work being done in the background, making your code less efficient than it could be. It's a small example, but it highlights a larger truth about optimizing your code, so it's valuable to understand the effects that subtle coding choices can have.
Variable names can also play a sneaky role in this issue. If a variable name leads you to expect a list (for example, if the name is something like `my_numbers`), but that variable actually contains a single integer, it can create confusion and lead to harder-to-find bugs in your list comprehensions. It emphasizes the value of using descriptive variable names and reinforces the idea that consistent clarity is beneficial to your overall workflow.
And speaking of confusion, understanding the traceback when you get this error is crucial. The traceback can be complex, and when the root cause is a simple issue of trying to iterate over an integer when an iterable was needed, it can be difficult to understand where the error originated.
The issue extends further when you consider how your programs interact with external data. If you’re misinterpreting data, expecting an iterable but getting an integer, your code may malfunction. It may lead to vulnerability if user input or data is expected to be of a certain type, but in fact isn’t. Understanding how to appropriately manage these external inputs is a vital aspect of programming for practical applications.
The realm of custom-built classes can also fall prey to this error. Creating objects with custom iterator behavior is a great way to manage data in Python. But, if you’re not careful, you can encounter this `TypeError` by forgetting to make the conversion from integers to iterables when dealing with these custom classes.
Testing is a key component in preventing these types of errors. If you write thorough tests, it becomes far easier to see where these issues may creep in. The `TypeError` provides an invaluable clue about unexpected errors that arise from your program’s interaction with data.
Last but not least, the error is a useful learning tool for people who are new to Python. The distinction between iterables and non-iterables can be a bit confusing for those who’ve come from a background of more relaxed type systems. By understanding how these mechanisms work in Python, you’ll find it easier to build programs that are both clear and robust. It’s part of developing a deeper comprehension of how Python’s data management philosophy works, shaping how you approach the structure of your programs in the long run.
Unveiling the Mystery 7 Common Causes of 'TypeError int object is not iterable' in Python - Passing an Integer to a Function Expecting an Iterable
When you encounter the "TypeError: 'int' object is not iterable" error, it often stems from passing an integer to a function or operation that expects a collection of items, also known as an iterable. This happens because integers, by their nature, are singular values representing a single number, not a sequence like a list, tuple, or string. For example, if you attempt to loop through an integer as if it were a list, Python will immediately stop because the integer doesn't have the structure to support iteration. The solution typically involves converting the integer to a suitable iterable using the `range()` function, which generates a sequence of numbers based on the provided integer. This error serves as a clear indication that you're not providing the right kind of input to a function that is expecting an iterable. The need for iterables, and recognizing this distinction from other data types like integers, is a core aspect of Python development and avoiding issues that arise from inconsistent data use. Understanding how data types work is central to building reliable code.
1. **Iteration's Structural Need**: Python's iterative features, like loops, depend on objects with a specific internal makeup—a collection of items. Integers, by their nature, are solitary values, lacking this structure. This distinction is crucial for understanding how iteration works in Python.
2. **Type-Specific Mechanisms**: The `__iter__()` and `__getitem__()` methods are what enable objects to be iterated over. Since integers, in their essence, don't possess these functionalities, using them in situations expecting an iterable results in the error. This underscores how Python enforces type-specific rules for certain operations.
3. **Python's Type-Consciousness**: Python, unlike some languages that allow implicit conversions, has a strong focus on type enforcement. This means you cannot treat integers like iterables without explicit intervention, which, although seemingly strict, helps prevent a lot of problems down the line.
4. **Assumptions and Consequences**: When an integer is passed to something that requires an iterable, the expected behavior of the operation breaks. This can lead to subtle errors that may only surface in specific scenarios, making it important to be cautious about what data you're providing to certain functions or operations.
5. **Misleading Names**: If a variable called `data` actually holds a single integer, it might lead developers to believe it is a list or tuple, potentially leading to issues in how the variable is used. This underlines the importance of using descriptive and meaningful variable names for avoiding confusion.
6. **Performance Impacts**: If you mistakenly use an integer when an iterable is needed, the performance of iterative operations can suffer. This might manifest as slower execution speeds or unpredictable program behavior, reminding us that type accuracy matters in performance-sensitive scenarios.
7. **Errors as Learning Tools**: The appearance of this particular TypeError is a good thing. It helps developers understand how Python's data types work and why it's important to use them correctly. These errors aren't just problems to be fixed, they also provide valuable insights into how Python operates.
8. **Custom Classes**: When crafting custom classes with iterable characteristics, it's essential to ensure that anything you return as part of the iteration is indeed iterable. A stray integer in the mix can disrupt the whole system, highlighting that attention to detail is needed in custom data structures.
9. **Handling Inputs**: Dealing with user input directly without validation is a recipe for these errors. If you simply assume an input is a list when it might be a single integer, the program might fail unexpectedly, underscoring the importance of input validation and pre-processing.
10. **Debugging's Complexity**: This type of error isn't isolated to one line of code. It often suggests that other parts of your program have a mismatch in what they were expecting to get and what they actually received. This hints at the importance of code clarity, making it easier to understand the flow of data throughout your program to help prevent this kind of confusion in the first place.
Unveiling the Mystery 7 Common Causes of 'TypeError int object is not iterable' in Python - Mistakenly Using an Integer Counter as the Iterable Object
Within Python's looping constructs, a frequent error stems from incorrectly using a simple integer where an iterable object is expected, resulting in the "'TypeError: 'int' object is not iterable'" message. This happens because integers are fundamentally single values, unlike lists or strings, which have an inherent structure for sequential processing. Integers don't have the necessary features to be iterated over directly. To rectify this, you must ensure that any integer destined for use within a loop is converted into a structure capable of iteration, like using the `range()` function to create a sequence of numbers. This error highlights the importance of careful data type validation and management. Understanding Python's approach to data types, particularly the distinction between simple values and collections, is vital for writing robust and efficient code. Paying close attention to data types is a crucial step in preventing unexpected behavior and promoting the clarity and stability of your Python programs.
1. **Integers as Single Units**: Unlike containers like lists or tuples designed to hold multiple elements, integers are fundamentally single values. They lack the structure necessary for iteration, such as the ability to provide a sequence of items. This distinction can lead to errors when developers mistakenly treat a simple integer as a more complex data structure.
2. **Error Messages as Guidance**: The error "TypeError: 'int' object is not iterable" serves as a helpful signal from Python's type system. This immediate feedback prompts developers to scrutinize the types of variables and how they interact within their code, cultivating more robust coding practices.
3. **The Iteration Protocols**: The `__iter__()` and `__getitem__()` methods underpin an object's ability to be iterated upon. Integers lack these protocols. Therefore, even if a loop appears syntactically correct, using an integer where an iterable is needed will result in this error. It enforces the importance of respecting type-specific functionalities in Python.
4. **Hidden Logic Issues**: If an integer is erroneously passed where an iterable is expected, it can introduce unexpected and often hidden issues in your program's logic. These can manifest as crashes or convoluted behavior, reinforcing the need for strict type correctness throughout the codebase.
5. **Name Deception Can Be Problematic**: Variables named things like `my_items` might intuitively lead a developer to believe they contain a collection, when in reality they might be storing a single integer. This can create confusion and debugging obstacles, highlighting the importance of clear and self-documenting code to avoid misinterpretations.
6. **Impact on Performance**: When an integer is inappropriately used in place of an iterable, Python might handle things inefficiently or in unexpected ways, potentially hindering the overall performance of your code. This type of error reminds us that respecting types can contribute to optimized code and a more efficient program.
7. **The Importance of Defensive Measures**: This error frequently crops up when user-provided data isn't properly validated. By adding checks to verify data types before performing operations, developers not only prevent TypeErrors but also strengthen their program against unintended input formats.
8. **Errors as Learning Opportunities**: Experiencing this TypeError can be a valuable learning experience, sharpening a developer's understanding of Python's type system. Each instance reveals the nuances of data handling in programming, stimulating a deeper grasp of type interactions within code.
9. **Custom Classes and Iteration**: When crafting custom classes designed to be iterable, there's a risk of mistakenly returning an integer instead of a collection. This underlines the necessity of meticulous design to ensure all returned data types adhere to expected behaviors and maintain the class's functionality.
10. **Enhancing Code Clarity**: Errors like these emphasize the importance of clear code structure and well-defined variable purposes. This improves not only debugging but also enhances long-term code maintainability by promoting code that's more intuitive to understand and modify in the future.
More Posts from aitutorialmaker.com: