Create AI-powered tutorials effortlessly: Learn, teach, and share knowledge with our intuitive platform. (Get started for free)
Mastering Python's 'def' Keyword 7 Essential Aspects for Function Definition
Mastering Python's 'def' Keyword 7 Essential Aspects for Function Definition - Syntax and Structure of Python Function Definitions
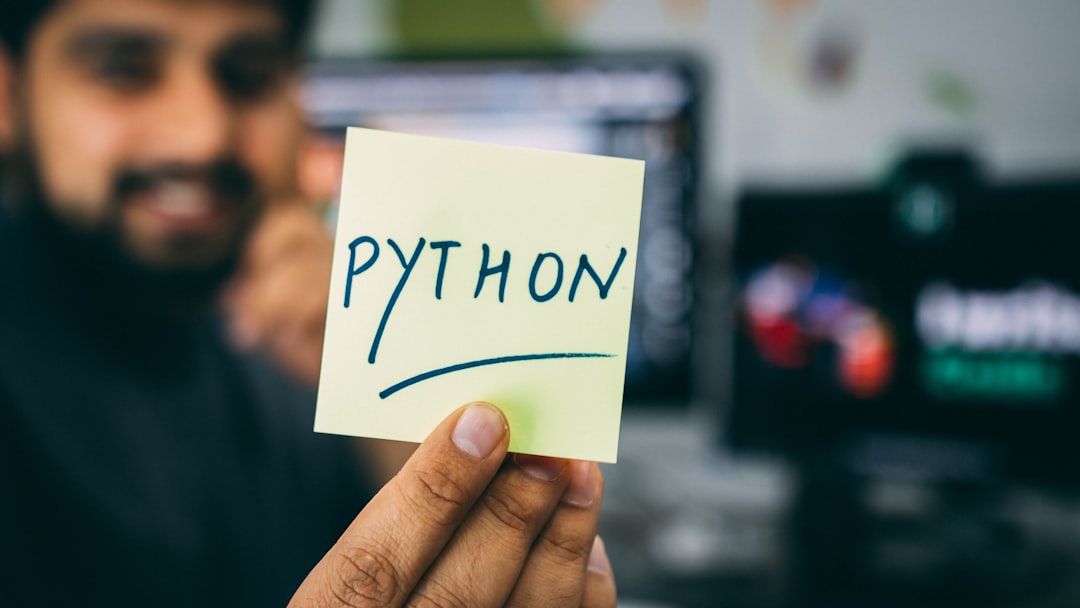
Python's function definitions adhere to a specific syntax. It starts with the `def` keyword, signifying the intention to create a new function. Following the `def` comes the chosen name of the function and parentheses that might contain parameters, which serve as input for the function. A crucial part of the syntax is the colon (:) after the parentheses, marking the beginning of the function's code block. This code block, which constitutes the core of the function, needs to be properly indented.
The ability to receive input via parameters is optional, and similarly, functions don't always need to return a value. The `return` statement facilitates this output, allowing functions to actively influence other parts of the program by returning data. It's also worth noting that Python 3 introduced type hints (via PEP 3107), a feature that lets developers annotate the intended return type of the function, though the lack of a mandatory declaration offers flexibility.
Ultimately, a deep grasp of Python's function definition syntax and structure is paramount. It enables programmers to build Python code that's modular, maintainable, and readily reused throughout the program, leading to cleaner and more efficient programs.
Let's delve into the nuts and bolts of how we define functions in Python. It all starts with the `def` keyword, which essentially signals to the Python interpreter that we're about to craft a new function. Following `def` comes the function's name, a crucial element for identifying and calling it later. Parentheses `()` follow the name, and this is where we define the input parameters, if any.
It's important to remember that every function definition must conclude with a colon `:`, signifying the beginning of the function's code block. And just like any well-structured code, this block needs proper indentation. The body of the function—the heart of what it does—resides within this indented block.
Functions are essentially named chunks of code designed to perform specific actions. And sometimes, they need to hand back some data to the part of the program that called them. The `return` statement is the mechanism for achieving this. The returned value then becomes the output of the function call.
Python has this interesting feature—type hints—which were introduced in Python Enhancement Proposal 3107. These hints provide an optional way to document what types of data the function expects and what type of data it intends to give back. However, it's important to remember that Python does not force type checking during execution, making functions remarkably flexible with respect to input and output types. This can be a double-edged sword, promoting flexibility but also introducing the risk of runtime errors if we don't exercise caution with how we design our functions.
It's pretty clear that defining functions is a fundamental aspect of building Python applications. They act as building blocks that enable code reuse and modular design, which leads to programs that are easier to understand, modify, and debug. Overall, mastering the art of function definition is a key stepping stone on the path to becoming a more proficient Python programmer.
Mastering Python's 'def' Keyword 7 Essential Aspects for Function Definition - Function Parameters and Arguments
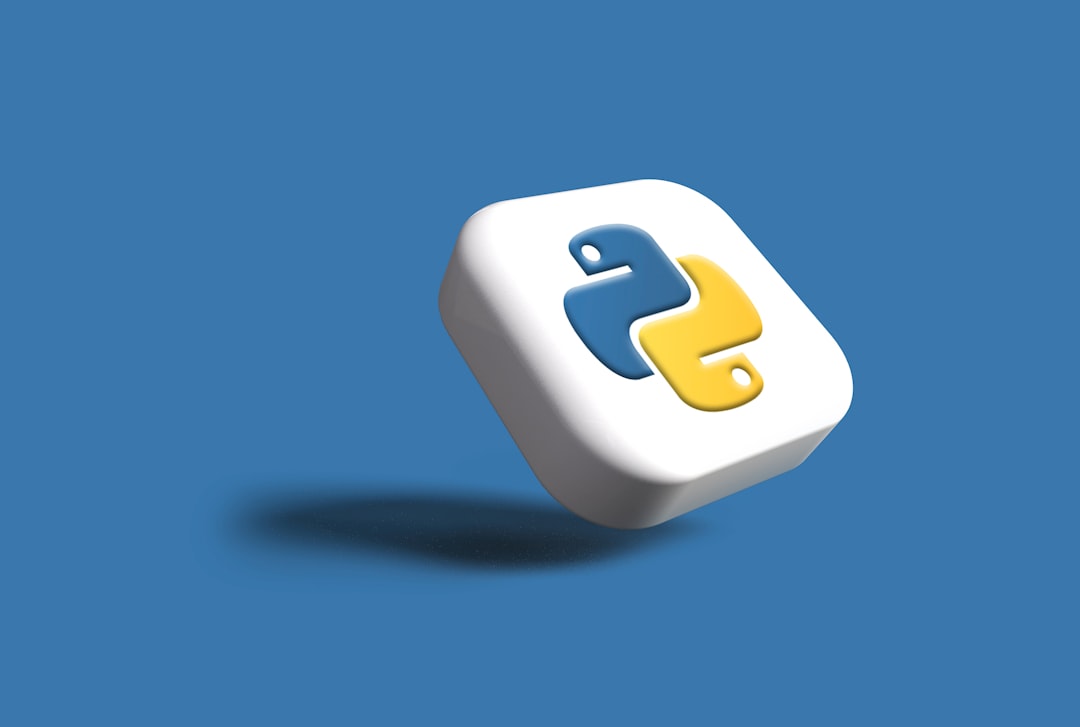
When defining functions in Python using the `def` keyword, we encounter the concepts of parameters and arguments. Parameters are essentially variables declared within the function's definition, serving as placeholders for the data the function expects to receive. Arguments, on the other hand, are the concrete values you provide when you actually call the function. Python offers a diverse range of ways to handle these inputs. You can use positional parameters, which must be passed in a specific order matching the function's definition. Alternatively, you can utilize keyword arguments, which offer more flexibility as you can pass them in any order by explicitly stating the parameter name.
Functions can also have parameters with pre-assigned default values. This means you can call the function without passing an argument for that parameter, and the function will fall back on the default value. Furthermore, Python offers mechanisms to handle an unspecified number of arguments through the `*args` syntax, packing them into a tuple, and the `**kwargs` syntax, packing them into a dictionary for keyword arguments. While this flexibility is powerful, it's essential to remember that positional arguments must be provided before keyword arguments when calling the function.
Using keyword arguments can significantly boost the readability of your code, particularly when dealing with numerous parameters where their purpose isn't immediately obvious based only on their position. This enhanced readability makes your code more accessible and easier to understand, contributing to more maintainable programs. Ultimately, a solid understanding of parameters and arguments is crucial for writing clear, well-structured, and reusable functions in Python, a foundational practice in achieving better Python code.
Function parameters and arguments are core concepts in Python, directly impacting how we interact with functions. Parameters act as placeholders within a function's definition, representing the inputs it expects. Arguments, on the other hand, are the actual values we supply when we call the function, providing the concrete data to be processed.
Python's flexibility shines through in the various parameter types it supports. We can have positional parameters, where the order of arguments in the function call strictly matters. Then there are keyword parameters, which let us specify the argument name along with its value, allowing for calls in any order. This is particularly helpful when functions have a large number of parameters.
Functions can also be designed to utilize default values for parameters. This allows the function to be called without requiring all parameters to be specified, adding convenience and flexibility. It's worth noting that Python mandates that arguments with default values must follow parameters without default values in the definition.
Another aspect of Python's design that adds considerable flexibility is the ability to define functions that accept a varying number of arguments. We achieve this using `*args` for a variable number of positional arguments and `**kwargs` for keyword arguments. These essentially pack the arguments into a tuple and a dictionary respectively.
It's crucial to respect the order of parameter specification when making a function call. Python expects us to provide positional parameters first, followed by keyword parameters. Failure to adhere to this can lead to errors, and consequently, functions that don't behave as intended.
Keyword arguments, in particular, can lead to more readable code. They make it easier to decipher what each argument is meant to represent, especially in complex functions. Further, allowing calls without strict adherence to a defined argument order also enhances the flexibility of Python code.
There is one rather insidious issue related to default values: mutable data structures. When using mutable objects (like lists or dictionaries) as default parameter values, a bit of caution is needed. If we are not careful, the side effects of a particular function call may impact subsequent calls because the default object gets altered over time.
Python's embrace of functions as first-class objects is another noteworthy facet of the language. It's like functions are treated like any other variable, which allows them to be passed as arguments into other functions or returned as output. This approach forms the basis for several sophisticated programming paradigms, like callbacks, and generally adds to the flexibility and power of the language.
It's interesting to note that while Python has introduced type hints, it's still a dynamically typed language. While these hints enhance readability and offer a degree of static verification via tooling, they are not enforced at runtime. This emphasizes the balance in Python's design between strictness and flexibility, which can be beneficial but also can contribute to errors at runtime.
Overall, function parameters and arguments provide the mechanisms by which we control and manipulate functions in Python. While at times the dynamic nature can be a source of errors, it adds to the power and flexibility of Python for a variety of development needs.
Mastering Python's 'def' Keyword 7 Essential Aspects for Function Definition - Return Statements and Function Output
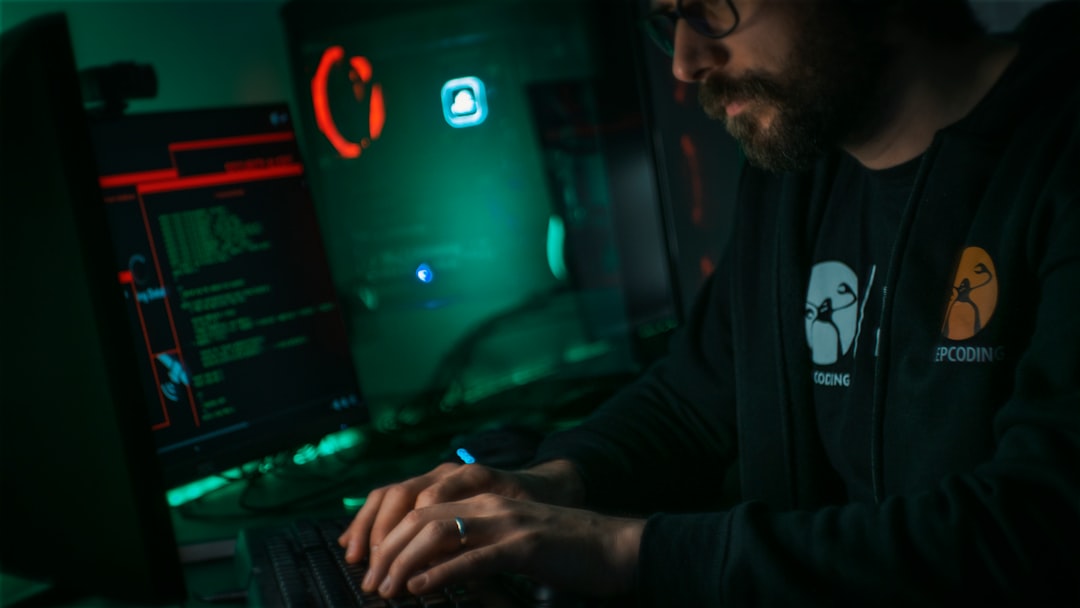
Within the context of Python functions, the `return` statement plays a crucial role in defining the function's output and controlling program flow. This statement allows a function to send back a value—which can be any Python object—to the part of the program that initiated the function call. The returned value essentially becomes the result of the function's execution. Crucially, when a `return` statement is encountered, the function immediately stops executing. If a function doesn't explicitly include a `return` statement, it implicitly returns `None`.
While `print` statements simply display information, `return` statements provide a means for a function to actively contribute to the program's broader execution. The value a function returns can be used as input for other functions, building a foundation for complex program logic and reusable code segments. This emphasizes the importance of mastering `return` statements as a cornerstone of managing output and shaping program flow when working with Python functions. Without an understanding of how `return` works, the ability to create modular and powerful programs is significantly limited.
When we explore how Python functions produce results, the `return` statement stands out as the primary mechanism. It's how a function sends information back to the part of the code that called it. A return statement is straightforward, consisting of the `return` keyword followed by a value (or nothing at all). This value can be any Python object, from simple numbers to complex data structures, like lists or dictionaries.
Interestingly, if a function doesn't have a `return` statement, it automatically returns `None`. This implicit return might catch some off guard, especially if they're used to other languages or forget to check for it explicitly. Also, while a function can contain multiple `return` statements, only the first one encountered will be executed. Once a `return` is executed, the function immediately exits, halting further execution within that function's code block.
The ability to return values unlocks a whole world of possibilities in terms of building larger, more complex programs. Function outputs can be used as inputs to other functions, helping to connect pieces of our code together seamlessly. This capability promotes a modular approach to design, where each function handles a specific part of the problem, promoting reusability and clarity.
Perhaps a counterintuitive point is that `print()` statements and `return` statements are fundamentally different in their behavior. The `print()` statement simply displays output to the console—it doesn't alter the program's flow in the way that a `return` does.
On a more technical note, Python, as of version 3.8, offers control over function parameters in a new way. We can declare positional-only parameters or keyword-only parameters. This can be especially helpful for managing larger, more complex function definitions that can benefit from some enforced rules regarding parameter usage.
Understanding the role of `return` statements is crucial for writing well-structured and maintainable Python code. Functions, after all, are meant to calculate something, and a `return` statement acts as the final declaration of a function's results. When we consider how we can break a complex problem into smaller, manageable chunks with functions, the `return` statement acts as the glue holding them together.
The fact that a function's output can be passed to another part of the program really emphasizes code modularity and reuse. It enables us to create libraries of reusable function components that can be called from other parts of a program or even by other programmers.
But there is an inherent risk in complex programs: it's easy to introduce bugs when combining different functions. Each time a function relies on the output of another function, we must ensure that the output we receive is exactly what is expected. It can be tricky to trace the source of an error if we have multiple functions connected to each other by their outputs.
So, while the `return` statement plays a central role in Python's modular programming style, we must remain vigilant about designing functions that have well-defined inputs and outputs. Clear and concise functions, which communicate their results effectively via the `return` statement, remain the best strategy for avoiding bugs and building complex and flexible Python programs.
Mastering Python's 'def' Keyword 7 Essential Aspects for Function Definition - Scope and Namespace in Function Definitions
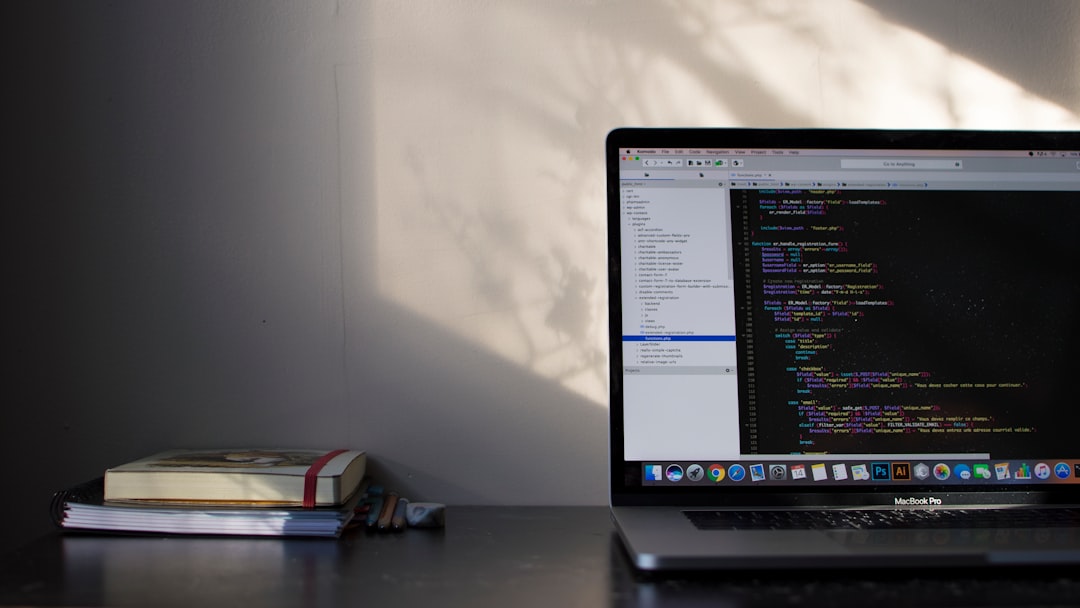
When you're defining functions in Python, it's crucial to understand how scope and namespace affect where variables can be accessed. Each function creates its own local scope, meaning any variable declared inside the function is only visible and usable within that function. Think of it as a little bubble where the function operates. When the function is done, that bubble disappears and the variables are gone.
On a larger scale, you have the global namespace, which essentially holds variables defined at the highest level of your Python file or module. And there's also a built-in namespace that offers access to Python's core functions like `print` or `len`– you don't need to import these.
To make things slightly more complex, there's the `nonlocal` keyword which lets nested functions modify variables from a scope that's higher up but not global. Similarly, `global` lets you explicitly tell Python that you're working with a variable in the module's top-level scope.
Properly managing scopes and namespaces helps prevent confusion and errors. If you don't understand scope, you might accidentally use the same variable name in different functions which can lead to unintended consequences. By being mindful of these concepts and using the related keywords when needed, you can write more robust and organized Python functions. It really helps to ensure you have more understandable and reliable code. Having a clear grasp of this whole area is key to becoming better at defining functions and more proficient in Python overall.
Here's a revised version of the text focusing on scope and namespaces within Python function definitions:
When we delve into the world of Python functions, we encounter the intriguing concepts of scope and namespace, which govern the accessibility and visibility of variables. Scope essentially refers to the region of a program where a variable or function can be accessed. Think of it as a boundary within which a variable is "known." In contrast, a namespace is a container that organizes identifiers—variables, functions, and so on—along with their associated objects. It's like a directory structure that helps Python keep track of all the elements involved in a program.
Python utilizes different scopes to manage the visibility of variables. One important distinction is between local scope, which is confined to a function, and global scope, which pertains to variables defined at the top level of a module (or file). Additionally, there is the built-in scope, which contains Python's predefined functions like `print` and `len`. It's like a hierarchy, with each layer impacting how variables are resolved during execution.
Variables declared within a function have a local scope, which means they are accessible only within the confines of that function. When the function finishes, the local namespace associated with it is effectively discarded, making those local variables disappear.
There's a special keyword, `nonlocal`, that allows us to modify variables in an enclosing (non-global) scope when dealing with nested functions. Using `nonlocal` impacts both the inner and outer functions after execution, changing the values outside the immediate scope of the inner function.
Another interesting wrinkle is the `global` keyword, which is used to signal that a variable inside a function needs to interact with a global variable (defined outside of any function) and, therefore, be modified in the global namespace.
Each time a function is defined, it creates a new namespace, ensuring that each function operates in a distinct, isolated space. This isolation of variables and their associated names prevents conflicts when we have the same variable names in different functions—a mechanism that enhances code organization and avoids unintended side effects.
For clear, maintainable function definitions, we should strive to create functions that perform only one task and ensure that scopes are easy to follow. This leads to code that is much easier to understand and debug.
Python's built-in scope is where functions like `print` and `len` reside. These functions are automatically available everywhere within a program without needing to be imported—they are available globally.
Having a firm grasp of namespaces and scopes is crucial for writing high-quality Python code. Understanding how they function helps us create code that works as intended and avoids unintended modifications of variables, which can cause problems.
Navigating variable names often involves understanding a hierarchy that goes from local to enclosing to global to built-in scopes. The hierarchy is important because it determines the order in which Python looks for the variable that is actually being used. When resolving variable names, Python follows a specific order, first checking the local scope and then progressively moving outward through the scope hierarchy. This ensures that the correct variable is chosen, which is important for creating code that behaves as expected.
Understanding the intricacies of scopes and namespaces is a journey for a Python programmer. Recognizing that Python is a dynamically typed language, which, by its very nature, doesn't provide much explicit guidance about what a particular variable might be, and that namespaces add another level of implicitness to this language, adds complexity to the task of coding. In a world where data types can change over time and where the scopes of variables can change in subtle ways, there are many opportunities for coding errors.
Mastering Python's 'def' Keyword 7 Essential Aspects for Function Definition - Function Documentation with Docstrings
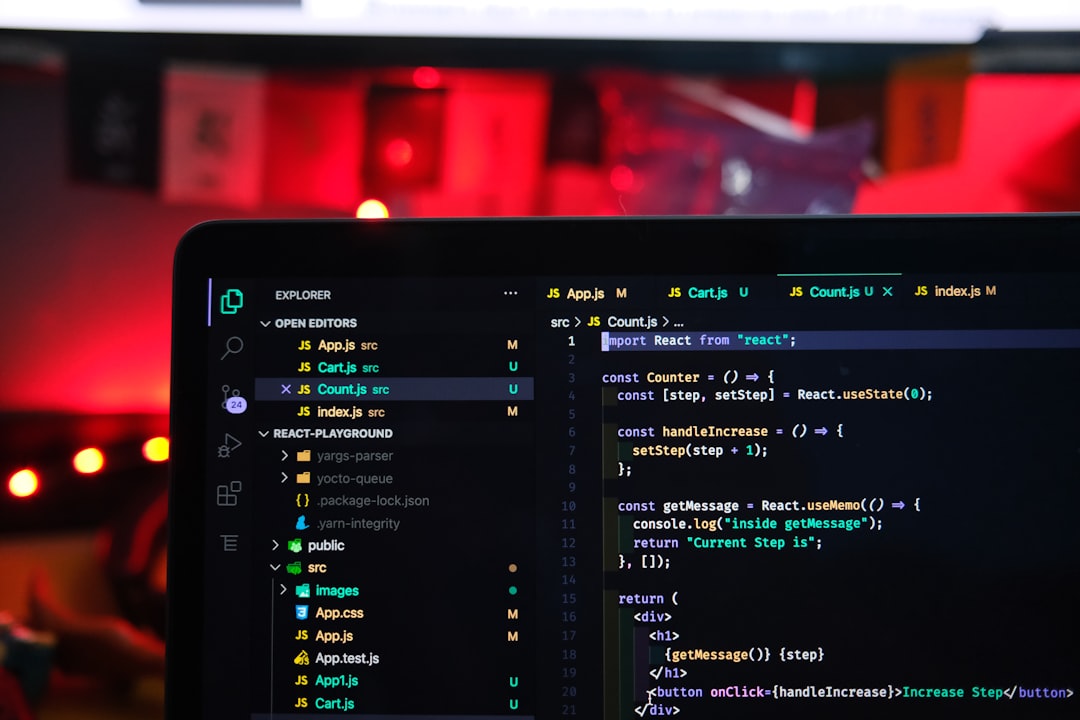
Within the structure of Python functions, docstrings play a crucial role in making your code easier to understand. These are essentially string literals that appear directly after the `def` keyword, serving as a built-in form of documentation. Docstrings explain what the function is intended to do, describe the input parameters, and detail what the function returns. Adhering to the standard format for writing docstrings (outlined in PEP 257) ensures consistency and readability, benefitting both you and any other person reviewing the code. For more detailed information about the parameters and outputs, it's best to leverage the markup language used by Sphinx; this provides clarity and comprehensive documentation for the function. Ultimately, adding thoughtfully written docstrings can dramatically improve the overall maintainability of a codebase, simplifying the process for future developers to understand and maintain the code in the future.
Docstrings in Python are a fascinating way to add documentation directly within the code itself. While seemingly simple, they hold a surprising amount of power and utility, especially for researchers and engineers who need to understand how code works and interacts.
First off, though any string can be used, the standard practice is to use triple quotes for multi-line docstrings. This not only improves readability but also paves the way for tools like Sphinx to automatically generate nicely formatted documentation. It's almost like Python has a built-in way to make comments more structured and informative.
Secondly, we can access docstrings programmatically via the `.__doc__` attribute of a function object. This makes it possible to write code that interacts with documentation, like dynamic help systems or interactive documentation explorers right within the Python code. It's like the docstring isn't just a comment – it's data Python can use.
Then there is the issue of type hinting within docstrings. While type hints via PEP 484 provide one mechanism, docstrings give us another path to communicating parameter and return types. This combination offers considerable flexibility and provides a way for tools to automatically perform static analysis without requiring a change to the code itself.
The built-in `help()` function is a clever design choice. It will print the docstring directly, providing quick and easy access to information about how to use a function. It's a time saver when we have to explore many unfamiliar functions.
Docstrings are at the heart of "self-documenting code." We strive to convey the intent, purpose, and behaviors of a function directly within the code, reducing the need for separate documentation files. Maintaining self-contained modules, along with their documentation, simplifies program modification and understanding.
However, different coding styles exist (NumPy, Google, reStructuredText), with their own ways of formatting docstrings. This leads to some heterogeneity and a need for adopting a consistent style within a project or across a group of engineers. It would be nice if there were one universally agreed upon style, but alas, in a world where choices abound, some of this heterogeneity seems unavoidable.
It's interesting to note that if a class inherits a function, the new class can inherit the docstring of the base class, but also override it. It's like we have both a global (base class) and local (derived class) perspective for the documentation. This inheritance structure adds a bit more complexity to understanding how docstrings propagate, but also offers a degree of freedom in documenting functions that are modified.
It's often the case that IDEs (Integrated Development Environments) have special help panels that display docstrings. This visual interaction between code and documentation is especially useful for beginners or anyone who is learning how a particular function is used.
Tools that generate documentation often directly incorporate docstrings in the output. This is useful because we don't have to write two separate documentation formats: one for the code itself and one for the official project documentation. The link between docstrings and generated documentation promotes consistency.
Finally, while usually minor, docstrings can impact performance due to their storage overhead. Larger applications can consume more memory with the presence of large docstrings. For applications running on constrained hardware, there is a tradeoff between documenting the code thoroughly and the memory footprint that it requires. As always in engineering, it's a balancing act!
In conclusion, docstrings are a clever feature that promote understanding. They enhance code clarity and maintenance, making them invaluable for researchers and engineers. Though some style variations exist, they promote a degree of introspection into code, which is helpful when exploring how things work. While they do have a small memory cost in complex programs, the benefit they provide clearly outweighs the cost in most applications.
Mastering Python's 'def' Keyword 7 Essential Aspects for Function Definition - Default and Optional Arguments in Functions
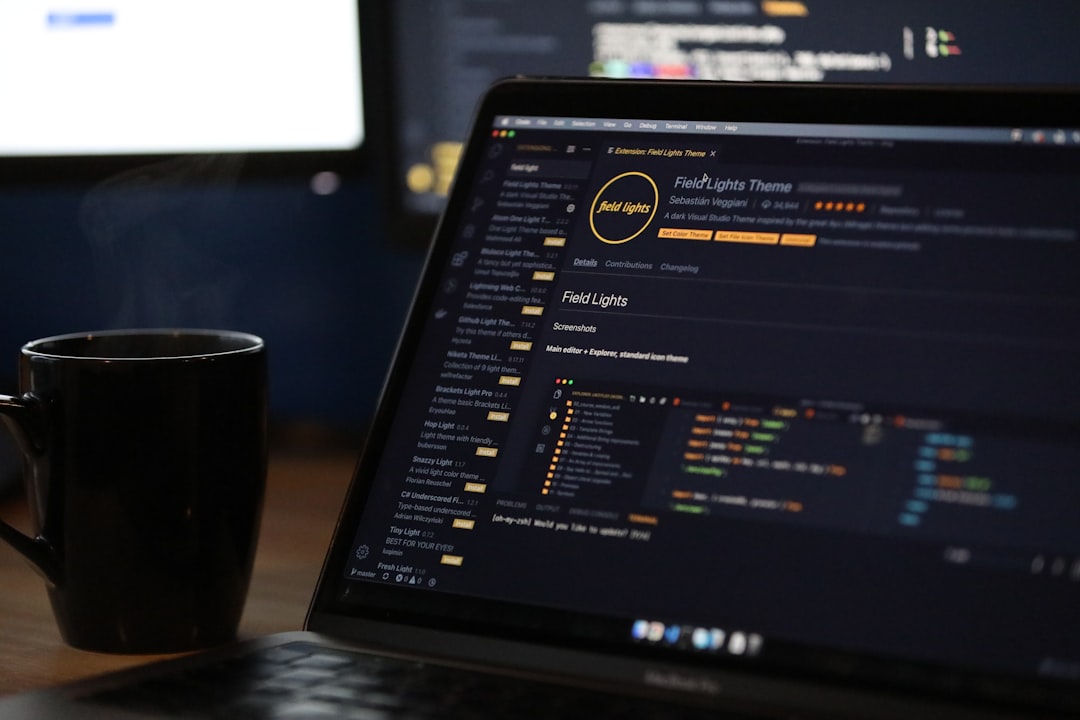
Python functions can be made more adaptable and user-friendly through the use of default and optional arguments. Default arguments are assigned predetermined values within the function's definition. When a function is called without providing a value for a parameter with a default, the function automatically uses the default value assigned during definition. Optional arguments are a way to design functions that can accept a variable number of inputs. The `*args` syntax handles positional arguments, packing them into a tuple, while `**kwargs` manages keyword arguments, bundling them into a dictionary. However, to avoid ambiguity, it's essential to place default arguments after those without default values in the function signature. By understanding and effectively using these features, you create more flexible and readable code. It simplifies the creation of reusable function components and significantly improves the maintenance of your Python code. It contributes to functions that are easier to work with, particularly in larger Python projects, where readability and reusability are highly valued. Essentially, the deliberate use of default and optional arguments streamlines coding and improves the overall experience of working with functions.
Here are 10 interesting points about default and optional arguments in Python functions that could pique the interest of both beginning and experienced programmers:
1. **Defaults and Mutable Objects**: When defining functions with default values, be cautious when using mutable data types like lists or dictionaries. If you modify the default argument within the function, the change will persist across subsequent calls, potentially leading to surprising behavior. It's akin to the default value being shared across all calls, which can be an unexpected gotcha.
2. **Evaluation Time for Defaults**: Default arguments have a curious characteristic—they're evaluated only once, at the time the function is defined, not every time it's called. This can become a bit of a pitfall when the default argument involves mutable objects or calls to other functions that modify state. If we are not careful, we can end up with complex interdependencies between function definitions, which can be hard to follow.
3. **The Power of Keyword Arguments**: Python grants us the flexibility to use keyword arguments in any order when calling functions. This can significantly improve readability, particularly for functions with several parameters, as it makes it very clear which value corresponds to which parameter. This clarity is particularly beneficial when collaborating with other programmers or reviewing code some time later.
4. **`*args` and `**kwargs` Enhancements**: The `*args` and `**kwargs` mechanisms expand the possibilities of using optional arguments. `*args` lets us handle a variable number of positional arguments, packing them into a tuple, and `**kwargs` handles keyword arguments in a dictionary, allowing functions to adapt to a wide range of usage scenarios.
5. **One Definition, Multiple Behaviours**: Default values let us define a function that can behave differently based on the arguments provided. Instead of needing to define multiple functions that perform similar tasks with minor variations, we can leverage defaults to create a single, more flexible function.
6. **Order of Arguments**: Python requires positional parameters to appear before default arguments within the function definition. This seemingly small detail creates a more predictable and manageable behavior. It ensures we call the function with the correct order of arguments and reduces confusion.
7. **Type Hints and Default Values**: Type hints, introduced in PEP 484, add an optional way to document expected types for arguments. While they don't enforce typing checks during execution, they can be especially valuable when used alongside default values, making it clearer what type of value is expected in each situation and helping prevent errors.
8. **Preventing Runtime Errors with Defaults**: Providing default values can help avoid potential runtime errors. For example, if a function expects a list but doesn't receive one, a default empty list can prevent issues. This is a clever way of proactively addressing potential pitfalls in program execution, a valuable feature in Python's design.
9. **Shift Towards Keyword Arguments**: We observe a trend in Python development towards greater usage of keyword arguments. The rationale is to improve readability and reduce the risk of errors associated with positional arguments. This trend highlights a shift in practices in the larger Python community, a sign of a growing preference for more self-explanatory code.
10. **Customizing Default Behaviour**: We can set the default argument value to `None` and then initialize it within the function body based on contextual information. This allows us to define dynamic defaults, making our functions more adaptive. For example, if the function is within a module, perhaps the default value depends on an external configuration file or another environmental setting.
Understanding the finer details of default and optional arguments is key to designing well-structured Python functions. It helps us leverage Python's flexibility in a controlled manner, leading to better and more maintainable code in the longer run.
Mastering Python's 'def' Keyword 7 Essential Aspects for Function Definition - Variable-Length Argument Lists with *args and **kwargs
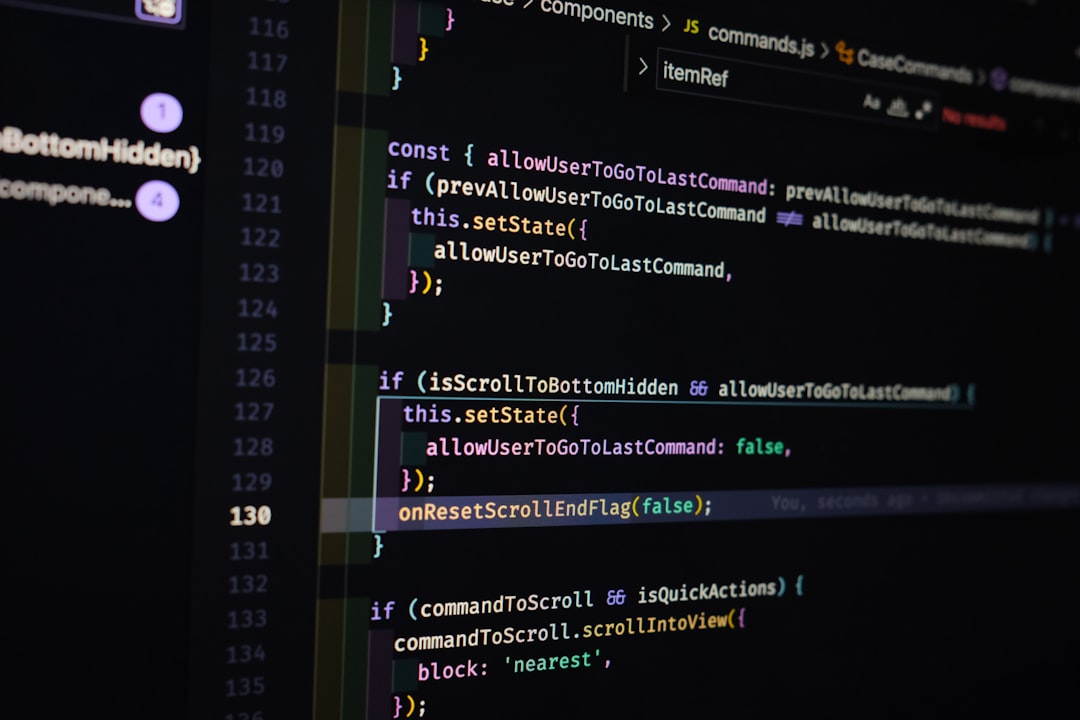
Python offers a neat way to handle functions that need to take a variable number of inputs: using `*args` and `**kwargs`. `*args` lets you pass any number of positional arguments to a function, gathering them into a tuple. On the other hand, `**kwargs` handles keyword arguments, packing them into a dictionary. This means you don't have to specify the exact number of arguments beforehand, which is super helpful when you don't know how many inputs a function will need.
But there are a few caveats to keep in mind. The order in which you define your parameters matters. Positional arguments should come first, followed by `*args`, then regular keyword arguments, and finally `**kwargs`. Not following this order can lead to errors.
Despite the potential for errors, `*args` and `**kwargs` are incredibly valuable because they make functions more flexible. You can easily change how a function works later on without having to significantly alter its structure. This can save you a lot of time and effort, and helps to ensure that your code is adaptable to future needs. In short, `*args` and `**kwargs` provide a neat solution for crafting more dynamic and maintainable functions in Python.
Create AI-powered tutorials effortlessly: Learn, teach, and share knowledge with our intuitive platform. (Get started for free)
More Posts from aitutorialmaker.com: