Demystifying C++ Streams A Deep Dive into Data Flow Abstractions in 2024
Demystifying C++ Streams A Deep Dive into Data Flow Abstractions in 2024 - Understanding the basics of C++ streams in 2024
In 2024, the core principles of C++ streams are still a crucial part of any developer's toolkit, especially those dealing with data movement and handling information in real-time. C++ employs `std::istream` as the standard way to represent input streams. This is how you can make your program interact with data coming from the keyboard or other input sources. The extraction operator, `>>`, plays a crucial role, effortlessly translating raw input from the stream into data types usable within your code.
The significance of streams becomes apparent in scenarios that necessitate instant data processing. Streams provide a clean and efficient way to manage both the input and output facets of a program. As the landscape of data streaming technologies keeps evolving, having a firm grasp on these basic concepts allows developers to better optimize and control the flow of data within their applications. A solid understanding of streams will improve their ability to craft applications capable of robust data analysis and real-time processing.
C++'s stream system is built upon a layered hierarchy of classes, offering both input and output functionalities. This contrasts with the more structured data handling prevalent in other languages. The inclusion of manipulators like `std::setw` and `std::fixed` grants programmers a surprising degree of control over output formatting in a clean way.
C++ streams also employ buffering to optimize input/output performance. Understanding the size and flushing mechanisms of this buffer is crucial, particularly for applications that process large amounts of data. The overloaded operators in C++ streams simplify complex tasks, smoothly integrating object-oriented features into stream handling—a feature that often goes unnoticed.
Exception handling, implemented through the `exceptions()` method, provides a systematic approach for error management and recovery when working with file I/O. This contrasts starkly with many languages where I/O errors often aren't handled well.
The `std::istream` and `std::ostream` base classes open avenues for constructing bespoke stream classes, enabling highly specialized input/output behavior for specific applications. It’s worth noting that this deviates from the rigid I/O models often encountered.
C++ streams are remarkably versatile, handling not only textual data but also binary information seamlessly. This ability to seamlessly shift between modes without disrupting the stream's core functionality is often missed in foundational tutorials.
Stream states, signified by functions like `good()`, `eof()`, `fail()`, and `bad()`, provide valuable insights into the status of a stream. Understanding these states is essential for building robust applications capable of recovering from potential input/output issues.
By integrating character traits, such as locales, streams adapt to cultural norms when it comes to data representation and manipulation. This often overlooked aspect is critical when developing software intended for use in various geographical regions.
C++’s stream library also provides tools for inter-thread communication through streams. The fact that this functionality is part of the core stream design, facilitating data flow management in concurrent programming environments, can be a surprise and opens possibilities for managing interprocess communication.
This unexpected ability to leverage streams for concurrency is a unique aspect of C++'s approach to I/O that adds to its flexibility and power.
Demystifying C++ Streams A Deep Dive into Data Flow Abstractions in 2024 - The evolution of data flow abstractions since C++20
Since the introduction of C++20, the landscape of data flow abstractions has undergone a significant transformation, focusing on making streams more expressive and user-friendly. The core idea of data flow programming—where operations are visualized as interconnected nodes—has been further developed, incorporating features like dynamic interactions between nodes and support for parallel processing. This has led to substantial improvements in performance and managing intricate processes.
Furthermore, newer tools and abstractions have been introduced to better support object-oriented programming paradigms in C++, addressing limitations that were present in previous approaches. Data flow analysis, a technique used to examine how data moves through a program, has become even more central to improving code organization and optimizing how resources are used. This helps in creating applications that are more efficient and easier to maintain.
The ongoing evolution of these abstractions indicates that there's a strong push to modernize how developers interact with data in C++. By refining these tools, the hope is to significantly enhance the efficiency and ease of data processing within the language.
The introduction of ranges in C++20 marks a significant change in how we think about data flow abstractions. Ranges promote a more "lazy" evaluation approach, leading to more concise and readable code, especially when dealing with complex data transformations. This functional-style approach shines when we combine multiple operations on data into pipelines, greatly enhancing both readability and maintainability.
Alongside ranges, C++20 concepts provide a mechanism for making algorithms more type-safe when working with streams. This means we can catch many errors at compile time, a feature that helps avoid tricky runtime errors associated with incorrect stream manipulations. It's also notable that the C++20 coroutine support has extended into the stream domain, enabling asynchronous stream operations. This opens up opportunities for non-blocking I/O, which is particularly beneficial for performance-critical applications that deal with a high volume of data.
One of the interesting benefits of ranges and views is lazy evaluation, which significantly reduces unnecessary data copying. This aspect of C++20 stream improvements is crucial for memory efficiency, particularly in applications dealing with massive datasets where throughput is paramount. It’s not just about how we express data flow, but also how efficiently we manage resources during that flow.
C++20 also made it easier to create custom stream adapters, making it easier to integrate streams with diverse data structures and third-party libraries. This added flexibility suggests an increased focus on making streams a truly versatile component. While we've had threads for a while, the evolution of stream design now offers built-in mechanisms for thread-safe stream sharing. This is essential for modern multi-threaded applications and signals a more sophisticated approach to concurrency within the data flow context.
Stream error handling has also received attention, with C++20 refining how we handle errors via exceptions and standard error codes. This has improved clarity when debugging input/output problems. Moreover, these improvements to stream abstractions have made interoperability with other languages more straightforward. It's no longer just about handling data within a purely C++ context; it's now easier to build bridges with other technologies.
Finally, we're witnessing tighter integration between the evolving C++ stream infrastructure and cutting-edge libraries like those used in machine learning and data analysis. This fusion opens the door to sophisticated data handling previously largely confined to specialized frameworks. The evolution suggests a broader potential for C++ to handle more specialized computational workloads within its own ecosystem.
Demystifying C++ Streams A Deep Dive into Data Flow Abstractions in 2024 - Implementing efficient input/output operations with C++ streams
C++ stream operations are built upon the `iostream` library, providing a foundation for managing input and output across various devices like files and the console. The use of dedicated stream classes within the library allows for a standardized way to interact with these diverse resources, highlighting the object-oriented design principles of C++. A key advantage of C++ streams is automated resource management, handled through destructors, which avoids the manual cleanup procedures prevalent in C-style I/O, thus promoting better memory management. While C++ streams offer a high level of abstraction and ease of use, their performance can vary. Formatting operations, in particular, might not always be as fast as more direct approaches like using `printf`. Despite these nuances, C++ streams ultimately provide a flexible and streamlined method to handle data flow, making them a valuable tool in modern software development.
The C++ `iostream` library offers a powerful and flexible way to handle input and output, making it easier to work with files, devices, and console interactions. It provides a standardized approach through specific classes, like `istream` and `ostream`, that abstract away the underlying complexities of communication channels. These classes act as intermediaries between your code and the target source or destination of your data flow. The `istream` class is particularly useful for input operations, relying on the extraction operator (`>>`) to gracefully convert incoming data into usable types.
One of the advantages of C++ streams is their automated resource management, relying on destructors to cleanly manage resources like file handles and internal buffers. This contrasts with C-style I/O where manual calls to functions like `fclose` are necessary, potentially leading to issues if not handled properly. However, performance can vary between different stream implementations. Some formatting operations with C++ streams can be slower compared to using the more direct `printf` approach, something to be mindful of in performance-critical situations.
A key stream class, `stringstream`, is designed to work with strings. This allows for stream operations within memory, which can be useful for tasks such as parsing complex input data. The `iostream` library consists of several header files, each fulfilling a specific role and contributing to the overall functionality. Overall, C++ streams present a more refined and object-oriented method for I/O compared to the traditional C-style approach.
The GNU C Library (glibc), a fundamental part of many Linux systems, also embodies the stream concept, illustrating how streams are viewed as general communication channels. C++ streams offer mechanisms to efficiently manage buffers for I/O, minimizing the overhead associated with accessing data sources. Operations can be chained together seamlessly, resulting in more compact and readable code. For instance, multiple output operations can be combined into a single line with `std::cout`, which can improve the code's clarity. C++ streams provide both formatted and unformatted output methods. In situations demanding maximum performance, unformatted methods offer a way to significantly enhance speed, particularly for large data transfers.
Further, stream operations are designed to be memory-conscious. Using techniques like dynamic buffer allocation, streams can adapt to incoming data size efficiently. Keeping track of these memory dynamics is vital to building efficient applications. C++ stream implementations are also sensitive to regional preferences and can adjust how they format data, such as numbers, dates, and currencies. This makes it easier to create applications that work seamlessly in different parts of the world. The introduction of concepts in more modern C++ versions also adds a layer of type safety to stream operations. This aspect helps catch many issues during compilation, improving code quality and reducing runtime errors.
C++ allows programmers to extend the base `istream` and `ostream` classes to create specialized stream types. This allows for greater flexibility in adapting to unique I/O needs. While C++ streams aren't intrinsically thread-safe, features available in the language can assist in crafting thread-safe stream sharing mechanisms. This adaptability is particularly valuable in today's multithreaded programming environments. Understanding how to use these features correctly is vital for building concurrent applications. The evolution of C++ streams showcases a constant drive to improve data handling, especially in relation to both performance and maintainability.
Demystifying C++ Streams A Deep Dive into Data Flow Abstractions in 2024 - Stream manipulators and their impact on data processing
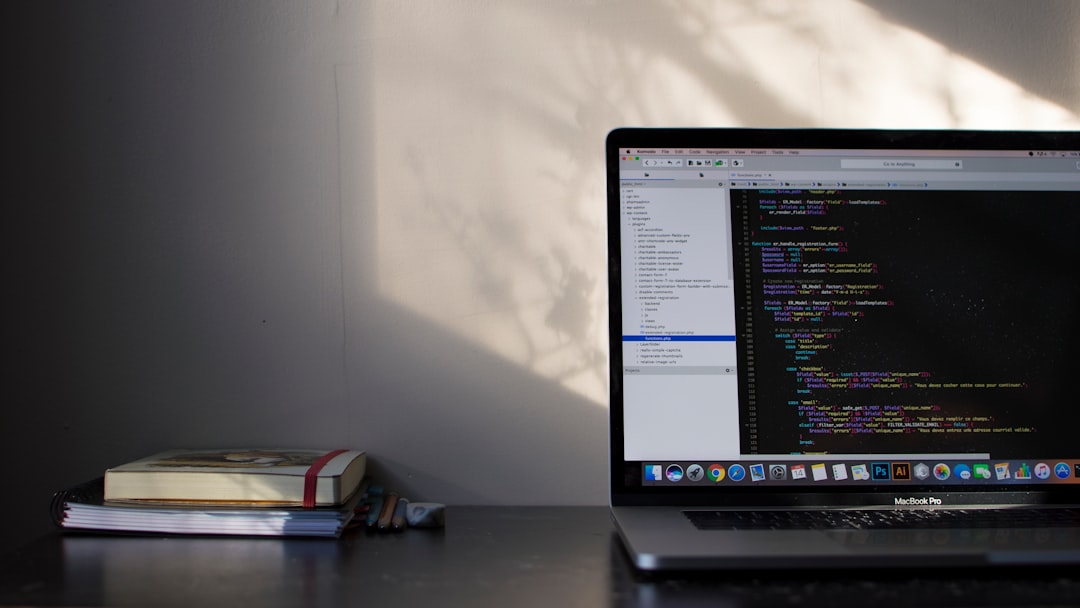
Stream manipulators are a crucial aspect of C++'s stream infrastructure, giving developers finer control over how data is presented and processed. They offer a dynamic way to format output, allowing for the creation of well-structured and human-readable data representations. Manipulators such as `std::setw` provide the ability to adjust the width of output fields, resulting in neatly organized data streams, a feature that becomes increasingly important when dealing with complex datasets. However, this added level of control often comes with a potential performance cost. In certain scenarios, using manipulators may lead to slower output compared to using more basic I/O techniques. As C++ continues its journey of enhancement and refinement, the capacity to shape output through manipulators remains a valuable tool, illustrating the constant need to balance the advantages of convenience and readability with the demands of efficiency in modern software development. Furthermore, with data systems growing increasingly sophisticated, grasping how manipulators affect data processing principles becomes essential for building effective and optimized applications.
Stream manipulators within the C++ stream framework offer a level of control over data representation and processing that's often overlooked. While features like `std::setw` might seem like basic formatting tools, they can subtly influence performance by improving output readability, which in turn can make debugging simpler and reduce cognitive overhead. The ability to precisely control how floating-point numbers are displayed using manipulators like `std::scientific` and `std::fixed` is vital in fields like scientific computing where small discrepancies can lead to significant variations in results.
Interestingly, C++ stream manipulators can also incorporate locale-specific formatting. The `std::use_facet` mechanism, for instance, allows developers to tailor output to different cultural standards, ensuring compliance with regional data formats. This is becoming increasingly important as software applications gain global reach.
Beyond formatting, manipulators also impact memory management in subtle ways. C++ streams leverage buffering to optimize I/O, and the effective management of buffer sizes can drastically influence the speed of data transfer, especially when working with large datasets. It's fascinating how something as seemingly simple as manipulating output can indirectly influence the way a program interacts with memory.
The ability to chain and compose stream manipulators contributes to code readability and maintainability. It encourages a more functional programming style that fits well with modern C++ paradigms. This style of coding makes complex operations easier to understand, both by the programmer and by those who might need to maintain or modify the code in the future.
While manipulators simplify tasks, it's important to be aware that some of them change the internal state of streams. Manipulators like `std::ios::flags()` can influence how subsequent stream operations behave. Grasping these state changes is crucial for effectively debugging and understanding complex data flows.
Furthermore, stream manipulators can act as a means to present errors and other informative feedback without affecting the core data processing logic. This can be helpful in keeping user interfaces clean while still offering valuable insights about runtime conditions. In certain situations, manipulators enable easier interoperability with third-party libraries, especially those that deal with intricate data types or serialization. This capability allows for more efficient data exchange between systems.
One of the more powerful aspects of C++ stream manipulators is the ability for developers to define their own. Custom-made manipulators can be tailored to a specific application's needs, contributing to improved code reuse and maintainability within a project. Moreover, some stream manipulators possess the capability to automatically adapt to different data types, depending on context, particularly when paired with templated functions or generic programming. This adaptable characteristic increases the flexibility of a data processing system's design.
The design of C++ streams, including the concept of manipulators, reflects a continuous evolution towards making data handling both efficient and easier to manage. While these aspects are often overlooked in favor of more traditional or commonly discussed stream features, their subtle impact on code quality, performance, and adaptability make them worth careful consideration in a variety of applications.
Demystifying C++ Streams A Deep Dive into Data Flow Abstractions in 2024 - Integrating C++ streams with modern concurrency models
Integrating C++ streams with modern concurrency models presents an exciting opportunity to optimize data processing in the face of increasingly parallel computing environments. C++20's contributions, like coroutines and enhancements to atomic operations, have breathed new life into the concurrency capabilities of streams, allowing for asynchronous I/O operations without stalling execution. This is particularly beneficial for handling large datasets or complex operations in multithreaded applications. Through concurrency streams, developers can pursue more efficient data processing strategies. Yet, incorporating these concepts also introduces challenges. Ensuring thread safety and efficiently managing inter-thread communication adds a layer of complexity that needs careful consideration. Given the rising prevalence of data-intensive applications, a thorough grasp of how concurrency interacts with streams will become crucial for crafting high-performing and reliable software. The inherent complexity in this integration makes it a fascinating and sometimes tricky aspect of C++'s stream capabilities.
Here's a rephrased version of the text focusing on the integration of C++ streams with modern concurrency models:
Exploring how C++ streams interact with modern concurrency models reveals some interesting aspects and potential challenges. First off, it's important to acknowledge that C++ streams, in their standard form, aren't inherently designed for multi-threaded environments. This means developers have to be mindful of thread safety. While techniques like mutexes or the newer atomic operations offer solutions, it's crucial to plan your system carefully to prevent issues like data races where multiple threads are trying to access the same data at the same time. This can be tricky to debug.
Furthermore, while employing streams to manage concurrent tasks can create a well-structured workflow, there's a performance trade-off. Locking mechanisms introduced to protect shared stream resources can cause bottlenecks, and it's essential to profile your application to identify these slowdown spots.
Interestingly, C++ gives you the option to build your own custom stream buffer strategies. This is powerful because you can potentially tailor your buffer management for improved efficiency in situations involving multiple threads. By carefully designing how buffers are allocated and flushed, it's possible to lessen wait times and potentially push through more data.
C++20 introduced coroutines, which in turn enable asynchronous stream operations, allowing non-blocking I/O. This is a game-changer for many applications. For example, if you're working on a program that interacts with external data sources or handles a lot of file I/O, having this responsive design capability can be very important.
Another interesting facet is the ability to integrate streams with concurrent data structures, like lock-free queues. This is useful because it allows for really efficient data movement between different threads that are working together (think of a scenario where one thread produces data and another consumes it).
Moreover, concurrency allows you to run stream operations in an unordered fashion, which can be useful for increasing the throughput of certain programs. However, this requires great care to make sure that the integrity of your data is maintained. And when it comes to creating your own stream classes, you can build custom implementations optimized for multi-threaded environments. This kind of specialization can really boost performance for specific tasks.
It's also worth noting that when using streams in concurrent environments, you can modify things like the stream's state and buffer size on the fly. This is important because the amount of work your program needs to do can fluctuate. In dynamic environments, this flexibility allows your program to utilize resources more efficiently.
Moving forward, it's encouraging that the stream infrastructure in C++ is aligning with more modern concurrency patterns. It means you can use abstractions like the actor model or data flow programming, which can help make your code cleaner and easier to maintain.
Finally, you need to be conscious of how stream states (things like `good()` or `eof()`) behave when they are accessed by multiple threads. Inconsistencies in state management can create hard-to-predict issues and undermine the reliability of your application.
All in all, it's fascinating how streams can be harnessed for concurrency. However, it's also crucial to understand the various trade-offs and challenges inherent in this integration.
Demystifying C++ Streams A Deep Dive into Data Flow Abstractions in 2024 - Comparing C++ streams to other programming languages' data flow abstractions
When evaluating C++ streams against data flow mechanisms in other programming languages, a notable difference emerges: C++'s approach is intrinsically tied to its object-oriented nature. In contrast to languages with integrated data flow constructs, often designed for straightforwardness and parallel execution, C++'s stream model incorporates a degree of intricate control. It aims to balance low-level performance and high-level abstraction, resulting in a nuanced approach. Languages such as Java or Python frequently rely on dedicated data flow frameworks, whereas C++ allows for a finer-grained manipulation of streams through the `iostream` library and customizable classes like `istream` and `ostream`. This adaptability provides developers with greater control, but can lead to increased complexity when it comes to handling thread safety and resource management, particularly in applications that use multiple threads. Therefore, as the evolution of C++ streams proceeds, comprehending their relationship with contemporary concurrency models becomes crucial for utilizing their capabilities effectively within a variety of programming scenarios.
When comparing C++ streams to data flow abstractions in other programming languages, a few key differences emerge. For instance, C++ allows for a much more flexible approach to data manipulation due to its operator overloading capabilities, unlike Java's more rigid I/O type system. This offers greater control and customizability.
C++20's introduction of coroutines for asynchronous I/O within the stream framework stands out when compared to many other languages. It means that C++ developers can achieve non-blocking I/O more easily than in languages where it requires extra libraries. This provides a more streamlined approach for handling concurrent tasks.
The way C++ streams handle memory with destructors is arguably more sophisticated than the manual management found in languages like C. This automated approach helps significantly reduce the potential for memory leaks, especially related to buffers and files.
Unlike languages with a fixed set of I/O routines, C++ lets you construct your own custom stream buffers. This is valuable for creating fine-grained performance enhancements in high-throughput systems. It's a level of control not as easily achieved elsewhere.
In terms of handling data across different cultural regions, C++ streams excel through the use of locales. This contrasts with Python, where it relies on separate libraries for this. It is quite impressive, however, this aspect is frequently overlooked.
Error handling within the C++ stream infrastructure also takes a more structured approach via the `exceptions()` mechanism. This differs from how it's dealt with in languages like JavaScript, where error handling for streams can be more complicated and less clear-cut.
On the flip side, while C++ streams offer incredible flexibility, it does come with certain trade-offs in performance. Formatting operations can sometimes be slower than more direct approaches like using `printf`, unlike Go, where built-in print functions generally prioritize speed over flexibility. This creates a potential hurdle when performance is a critical factor in an application.
C++ stream states can be modified dynamically. While this is quite powerful, it also increases the potential for unexpected behavior in concurrent environments. This contrasts with languages where stream state management might be less dynamic, perhaps making debugging a bit easier.
While not inherently thread-safe, C++ streams encourage proactive management of shared resources. In contrast, languages that provide built-in thread safety for their I/O mechanisms might not drive developers to think as much about concurrency issues. This forces developers to make more conscious decisions about how threads interact with streams.
Finally, C++ streams mesh beautifully with template metaprogramming. This allows for very general and type-safe stream operations, something less readily achieved in languages like Ruby, where I/O is generally more dynamic and not as subject to compile-time type restrictions. This is perhaps the most distinctive feature of C++ streams.
More Posts from aitutorialmaker.com: