Understanding Variable Type Hinting in Python A Guide to Static Type Declarations
Understanding Variable Type Hinting in Python A Guide to Static Type Declarations - Basic Syntax of Type Hints in Python From Version 5 to Today
Python's journey with type hints started with version 3.5, introduced through PEP 484. This initial step focused on providing hints for function parameters and return values, essentially signaling the intended data types. Python 3.6 took it a step further by integrating variable annotations, allowing developers to directly declare the expected type of a variable within the code itself. Importantly, it's crucial to remember that these type hints are purely for the benefit of developers and tools; they don't alter Python's runtime behavior. This means Python still dynamically determines the type of objects at execution time.
The primary purpose of type hints is to enhance code comprehension and maintenance. By clearly indicating expected data types, they act as a roadmap for other developers and even automated tools, such as linters and static analyzers. This clarity can significantly improve the debugging process and reduce the likelihood of errors related to unexpected data types.
While the initial focus was on basic types like integers and strings, type hinting quickly evolved to encompass more complex scenarios. Collections like lists and dictionaries are also supported, along with ways to express optional types or combinations of types (unions). Although initially part of Python 3, the concept gained traction, leading to partial support in Python 2.7 via specific implementations. However, its primary domain remains Python 3, especially with the continued refinement of the features.
It's worth noting that despite the advantages, overusing type hints can lead to code that's overly verbose and difficult to read. The best practice is to employ type hints strategically in larger projects where clarity and maintainability are paramount. However, ensuring consistency is important. Any modifications to variable types should be mirrored in the corresponding type hints to avoid discrepancies and confusion down the line. PEP 526 and PEP 604 are testament to the continuous refinement and expansion of Python's type hinting system, building upon the foundations of the original PEP 484. These advancements offer enhanced flexibility and capabilities, expanding the potential benefits type hints can provide.
The journey of type hints in Python began with version 3.5, introduced through PEP 484. Initially, it provided a means to describe expected types for function parameters and return values, but it didn't enforce them at runtime. It was essentially a way to improve the documentation aspect of Python code.
Python 3.6 extended the capabilities of type hints with the inclusion of variable annotations, allowing developers to define the intended type for any variable within the code. It made it clearer what kind of data should be stored in a variable, contributing to enhanced code comprehension.
Type hints don't affect how Python executes the code. Their purpose lies in improving clarity, organization, and comprehension of the codebase. This is beneficial for anyone working on the project and can significantly improve the debugging process by giving a better picture of what is expected to happen within a code section.
The flexibility of type hints extends to intricate data structures like lists and dictionaries. They support conditional type checks and specifying multiple possible types for a single variable.
While predominantly focused on Python 3, there were some experimental implementations of type hints in Python 2.7. However, these were more of a precursor to the fully-fledged type hinting system in Python 3.
For larger projects, type hints are a recommended practice. Their usefulness in dealing with complexity and boosting the overall quality of code makes them beneficial in managing larger codebases.
It's crucial, however, to be mindful of the potential drawbacks of using type hints excessively. Too many hints can make the code harder to read. Striking a balance is vital for getting the advantages of better readability without clutter.
Type hints are a form of documentation and should be kept aligned with the actual code. When the expected type changes, the type hint needs to be updated as well. Failure to do so can result in code that might not behave as intended and be prone to subtle bugs.
The straightforward hyphen-based notation, like `num1: int` and `num2: int`, allows for inline declaration of parameter types, making it easy to understand at the function definition itself.
Later developments, notably through PEP 526 and PEP 604, advanced type hints even further, building on the original foundation laid by PEP 484. These enhancements aimed at expanding the expressiveness and flexibility of type hints.
Understanding Variable Type Hinting in Python A Guide to Static Type Declarations - Variable Annotations for Built In Types With Examples
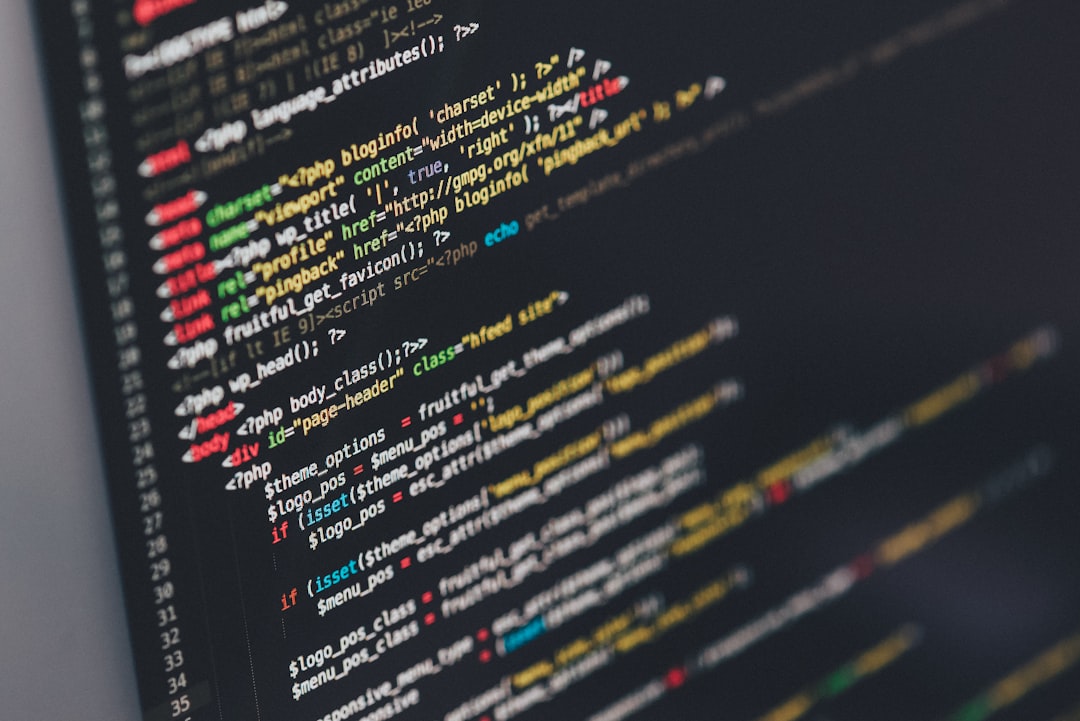
Python's built-in types, like integers (`int`), strings (`str`), lists (`list`), and dictionaries (`dict`), can be explicitly declared using variable annotations. These annotations, introduced in Python 3.6, are essentially a way to document the expected type of a variable within the code itself. For instance, you can express that a variable named `num` is expected to hold an integer using `num: int`.
The key takeaway here is that type hints are primarily for readability and support for automated code analysis. They do not change how Python works at runtime; the language remains dynamically typed. Tools like `mypy` can use type hints to catch type mismatches, helping to prevent errors.
Furthermore, Python's `typing` module offers features like `Annotated`, which enables developers to attach custom metadata to type hints. This is useful for extending type hinting beyond the basic types and offers potential for more sophisticated analysis or code management.
While extremely beneficial for maintaining large or complex projects, there's a risk of overusing type hints. Excessive use can lead to a reduction in readability, which could outweigh the benefits they offer. Finding a healthy balance is key to successfully integrating them into your coding workflow.
Python's type hinting system, introduced with variable annotations in version 3.6, offers a fascinating approach to improving code clarity without fundamentally changing how Python executes code. These annotations are essentially hints for developers and tools, not runtime enforcers. This characteristic preserves Python's dynamic nature while providing a degree of static analysis capabilities.
For instance, we can express the possibility of a variable being either a specific type or `None` using the `Optional` type. This allows for more intricate code designs where null values need to be considered. The ability to define type aliases through annotations simplifies the handling of complex types and contributes to cleaner code. Modern IDEs readily utilize these annotations, offering suggestions and error detection as you code, which accelerates development cycles and reduces debugging time.
One remarkable facet is that type hints maintain backward compatibility with previous Python versions. It allows for the gradual integration of type hints into existing projects without needing a full rewrite. The flexibility of the system also extends to generic types like `List[T]`, making it explicit what kind of data a collection should hold. This is particularly beneficial in scenarios where type safety for function arguments is critical.
Furthermore, type hints can communicate the mutability of variables, differentiating between mutable types like `List[int]` and immutable ones such as `Tuple[int]`. This can help avoid confusion on how a variable might be modified in the program. Static analysis tools like `mypy` effectively leverage type hints to identify type conflicts during the development process, which can significantly reduce time spent debugging.
The combination of variable annotations with the rise of dataclasses has simplified data model creation. Type hints neatly integrate with default values in these structures, making data management much more streamlined and concise. And finally, variable annotations used within function signatures offer a clearer indication of what the function is expected to return. This is especially vital in larger projects, where understanding the outputs of different functions is important for code maintainability.
As of today (26 Nov 2024), variable annotations remain a valuable asset for Python developers seeking to enhance the clarity and maintainability of their code. While Python continues to operate as a dynamically typed language, type hints serve as a bridge to incorporate aspects of static typing, contributing to a more robust and understandable development process. The ongoing evolution of Python's type hinting system promises continued improvements and potentially a more refined way to use these annotations in the future.
Understanding Variable Type Hinting in Python A Guide to Static Type Declarations - Creating Type Hints for Custom Classes and Data Structures
When you're working with custom classes or more involved data structures in Python, type hints can be incredibly helpful. They allow you to specify, within your code, what types of data you expect variables and parameters to hold. This becomes especially relevant when dealing with classes you define yourself or more intricate data like lists of dictionaries, or dictionaries of lists. Adding type hints to these elements can significantly boost code readability and make it much easier to maintain, especially as projects grow larger and more complex.
These hints act as a sort of internal documentation. They offer a clear picture of how different pieces of your code are intended to work together, making it easier for others to understand your code and potentially catch errors more quickly. Moreover, static analysis tools can use the hints to identify potential inconsistencies before you even run your program. This can save you considerable time and frustration during debugging.
While helpful, it's easy to go overboard with type hints. If you try to add hints to everything, your code might become overly verbose and harder to read, which defeats the purpose. Finding that right balance between detailed hints for crucial parts and a little brevity elsewhere is important for achieving the benefits without hindering understanding.
Python's type hinting capabilities extend beyond built-in types to encompass custom classes and data structures, creating a more robust and expressive system for defining code behavior. This ability to annotate custom structures is particularly beneficial when working with complex or intricate data representations, allowing you to effectively communicate the expected types and interactions within these custom creations.
For instance, you can use type hints within a class to specify that a method expects an object of a particular class or a specific type of collection. This helps in clarifying the intent behind your code and facilitates better collaboration within teams. It's interesting to note that, even when a class refers to itself in a type hint (like in a recursive data structure), the system generally handles it without issue, although it's worth paying attention to any implementation-specific quirks.
The use of generics with type hints in custom classes opens a whole new world of flexibility. With `TypeVar`, it's possible to define a custom class that can work with various data types without needing to rewrite the core logic. This feature helps in creating reusable and flexible building blocks. Type hints can even help pinpoint exactly what a particular container inside a class should contain. For example, a custom class representing a stack can use type hints to ensure that it only contains objects of a certain type.
Going a step further, we can use Python's Protocol classes to define behavior expectations for classes that implement it. This concept of "duck typing" with type hints ensures a certain level of conformity, which can help in enforcing API contracts and keeping code within an anticipated design space.
While Python's dynamic nature remains, it is possible to incorporate runtime type checks for custom classes if desired. By employing decorators or specific libraries, you can trigger actions if data doesn't conform to the type hints. This added layer of enforcement can offer greater confidence when handling sensitive data, though it does slightly move away from Python's core principle of duck typing.
Using type hints within class methods similarly helps developers and IDEs understand the intended interaction within that class. This becomes critical as custom class hierarchies get more complex. Modern IDEs leverage these type annotations to enhance developer productivity, offering better code completion, catching potential errors in real-time, and providing more context for what's happening in the code.
Furthermore, the adoption of type hints helps reduce the amount of time spent on debugging related to incorrect data types. The annotations act as a guide, indicating where and how type discrepancies might arise in the complex world of custom classes and data structures.
It's noteworthy that type hints also prove helpful when generating code documentation. Automated tools like Sphinx can use the hints as a guide, resulting in a more complete and accurate description of how your custom classes and methods are meant to be used.
As we've observed, integrating type hints into custom classes improves code readability, aids in static analysis, encourages cleaner API designs, and facilitates automated documentation. All these features collectively make custom classes more maintainable and less prone to subtle type-related bugs. However, it's still important to remember that overuse of type hints can impact code readability, so striving for a balance is always crucial. As the Python ecosystem continues to mature, type hints for custom classes and data structures are likely to become increasingly sophisticated and refined, offering a more intuitive and robust way to design software with Python.
Understanding Variable Type Hinting in Python A Guide to Static Type Declarations - Static Type Checking With Mypy A Practical Guide
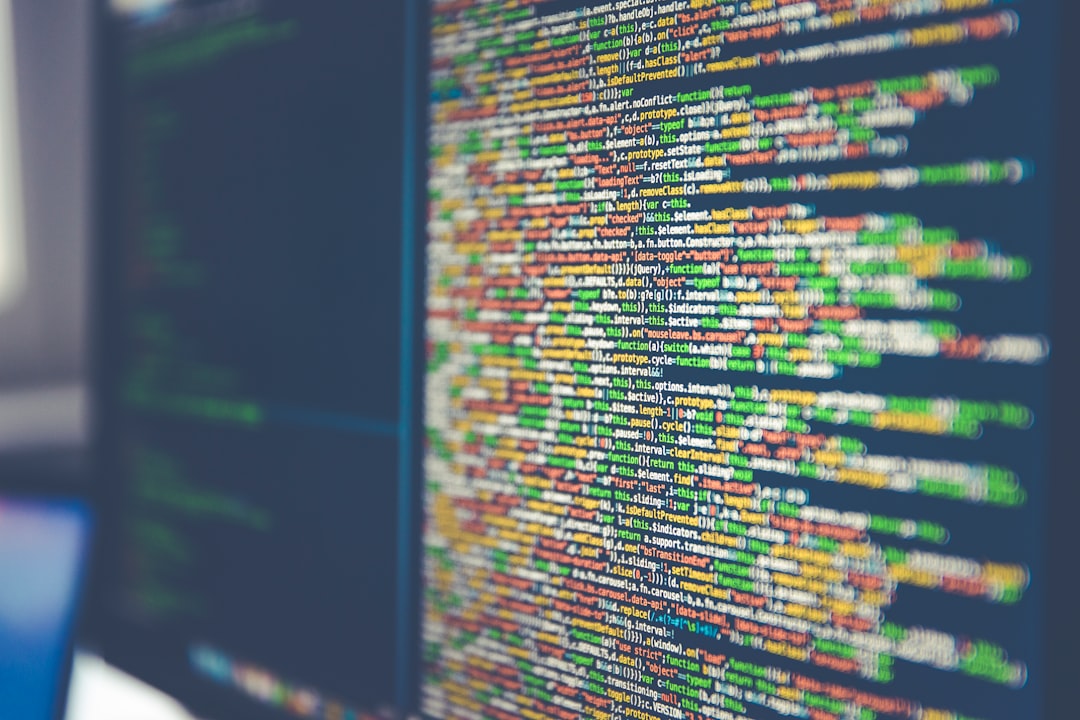
Mypy offers a practical way to enhance Python code through static type checking. It allows you to incorporate type hints into your code, enabling early detection of type-related errors during the development process, rather than encountering them at runtime. This capability builds upon Python's gradually improving type hinting system, which has expanded since the introduction of function annotations and the "typing" module. While Mypy helps address the inherent limitations of Python's dynamic typing, introducing static typing principles, it's important to acknowledge that correctly utilizing type hints is crucial. Misuse can lead to greater confusion rather than clarity. Mypy becomes especially valuable when working on more extensive projects, providing a mechanism for refining the robustness of the codebase. It can be a valuable addition to any Python developer's toolkit, especially for those aiming to improve the overall quality of their code.
Mypy stands out as a robust static type checker, going beyond basic checks to enforce type safety within Python projects, potentially minimizing runtime errors that could otherwise cause significant headaches. Its strength lies in its ability to thoroughly examine large and intricate codebases, revealing type inconsistencies that might not surface until runtime. This is especially useful in complex projects with numerous interdependencies.
One of Mypy's notable strengths is its adaptability to custom types. Developers have the freedom to define and reuse their types, constructing a flexible and modular system for maintaining code consistency. This flexibility proves especially beneficial when dealing with hierarchical systems, where strictly imposed types could lead to needless redundancy.
Mypy promotes a gradual transition to type safety through its support for gradual typing. It allows developers to incrementally integrate type hints into existing code, fostering a smoother adoption process for teams. This gradual approach avoids a complete rewrite of the codebase, making it a less daunting undertaking for projects that need to introduce type checking into their workflows.
While it provides a great level of assurance, it is important to keep in mind that Mypy's static analysis at compile time doesn't negate the need for runtime checks. Because Python is inherently dynamically typed, there are scenarios where errors can surface only during execution, highlighting a complementary relationship between static and dynamic type checking approaches.
Beyond explicit type declarations, Mypy utilizes clever inference mechanisms to determine the types of variables and function outputs. This enhances the development experience by minimizing the need for redundant annotations. The tool can infer intent even when not explicitly declared, which streamlines development tasks.
Mypy plays well with today's popular IDEs, enabling real-time feedback on type-related errors as code is being written. This immediate feedback accelerates development cycles by prompting adjustments on the fly, reducing the time devoted to debugging later on in the development process.
The versatility of Mypy extends to supporting function overloading. This means developers can create multiple function signatures for the same function, adapting to different input types. This adds flexibility to API design while maintaining type safety and making method signatures more streamlined for varied input situations.
Parameterized generics are another facet of Mypy's capabilities. This allows developers to use type parameters within data structures and algorithms, resulting in type-safe collections without excessive boilerplate code. This is particularly helpful in code sections where data structures are reused and require type flexibility.
Type aliases and forward references are readily accommodated by Mypy. This feature makes for cleaner and more manageable code, which is crucial in scenarios involving circular dependencies or highly structured data. It's particularly helpful when documenting and clarifying complex systems.
Mypy's configuration options are quite extensive, allowing developers to opt for more strict type checking. Enforcing more stringent rules can contribute to a more polished and trustworthy codebase, but it necessitates careful code review prior to activating stricter settings.
In conclusion, Mypy is a valuable tool for Python developers looking to enhance code quality and maintainability. Its capacity to integrate seamlessly into existing workflows, promote type safety, and enhance collaboration makes it a compelling option for Python projects, especially as complexity increases. However, as with any tool, awareness of its strengths and limitations are essential to best leverage its benefits. As the Python ecosystem progresses, Mypy is likely to mature further and become even more integrated into how Python code is developed.
Understanding Variable Type Hinting in Python A Guide to Static Type Declarations - Type Hints in Function Arguments and Return Values
Type hints, when applied to function arguments and return values, represent a powerful step towards enhancing Python code's clarity and reliability. By annotating function parameters with expected types (e.g., `arg: int`) and specifying the return type (e.g., `-> float`), developers provide valuable information about the function's intended behavior. This straightforward approach makes code easier to understand and reduces the chance of runtime errors that arise from unexpected data types. The benefits extend beyond simple types like integers and floats, as the `typing` module offers a rich vocabulary for complex structures, including support for collections and functions passed as arguments.
A particularly noteworthy improvement arrived with Python 3.10, which introduced a more intuitive way to declare multiple possible return types using union types. This simplification makes defining function outputs cleaner compared to earlier approaches using `typing.Union`. It's crucial to remember that these type hints are optional, and Python retains its dynamic nature at runtime. The hints themselves do not force specific behavior. Yet, their effective use can improve communication among developers and serve as a form of living documentation for functions. By understanding how the arguments and return values should be typed, developers gain insight into a function's purpose, making the codebase more maintainable and easier for others to understand.
Python's type hinting system, while not altering Python's core dynamic typing, offers a way to enhance code clarity by specifying the intended types of function inputs and outputs. This system allows for expressing intricate data structures within function arguments, like a list of dictionaries, promoting better code organization. Notably, type hints can even reference the class itself, making it possible to represent recursive data structures with greater clarity.
However, finding the right balance is crucial, as overusing type hints can obfuscate code. This delicate balance between improved readability and conciseness should always be considered when integrating type hinting into your projects. Interestingly, while Python doesn't strictly enforce type hints at runtime, you can leverage external libraries or custom mechanisms to achieve runtime type checks if needed, providing more stringent type control for specific cases.
Modern IDEs utilize type hints quite effectively. They offer improved suggestions for code completion and help flag potential type-related errors as you write your code. This real-time feedback streamlines the coding process and reduces debugging time. Notably, type hinting has been designed to be backward-compatible, facilitating a gradual adoption process. You can introduce type hints into existing projects incrementally without the need for a complete rewrite, simplifying the migration process.
The `TypeVar` feature, coupled with the concept of generics, introduces powerful flexibility to your code. It allows the construction of reusable data structures that can seamlessly work with different types while retaining type safety. This flexible approach enhances modularity and code reuse.
Python's type hints also offer compatibility with the concept of Protocol classes. These protocols can define a set of behaviors without requiring inheritance, staying true to Python's dynamic nature while encouraging a degree of contract enforcement. The ability to define type aliases within the code allows for simplifying intricate type declarations, making them easier to read and improving the maintainability of larger projects.
Tools like Mypy are able to enhance the development experience through type inference. In many instances, Mypy can deduce the types without needing explicit type declarations at every step. This feature benefits developers who prefer type clarity but don't necessarily want the added verbosity of type hints everywhere.
This aspect of Python's type system represents an ongoing evolution of the language, encouraging greater code quality, readability, and maintainability. While the dynamic nature of Python is preserved, type hints provide a stepping stone towards a more structured development process by leveraging static typing concepts to improve the overall quality of your codebase.
Understanding Variable Type Hinting in Python A Guide to Static Type Declarations - Optional Type Hints and Dynamic Type Behavior
Python's dynamic nature, where variable types are determined during runtime, remains a core aspect of the language. However, the introduction of type hints, including the concept of optional types, adds a layer of clarity and potential for error prevention. Optional type hints, like using `Optional[int]`, convey that a variable might hold an integer or it might be `None`. This helps developers and static analysis tools better understand the expected behavior of the code. Python 3.10 simplified this further with the `|` (union) operator, enabling more concise type hints, like `str | None`.
It's crucial to remember that these type hints are suggestions, not constraints enforced by Python during execution. The core dynamic typing behavior remains unchanged. However, this information about potential types greatly aids human readers and tools in understanding the code's intended usage. Tools like `mypy` can then employ these hints to spot potential type errors during development, contributing to a more robust and less error-prone codebase. It's important to find a balance, though, as overuse of type hints can reduce readability and increase the cognitive load when reading the code. Using type hints thoughtfully for key parts of a codebase is typically more beneficial than trying to hint absolutely everything. The overall goal is to communicate intent and improve the developer experience while still respecting the core philosophy of Python.
Python's type hinting system, introduced with version 3.5, has been a fascinating development in the language's evolution. It allows developers to provide clues about expected variable types without altering Python's core dynamic typing nature. While optional, these hints significantly improve code clarity, making it easier to understand what data a variable or function is supposed to handle. This is especially helpful when dealing with complex data structures or scenarios where `None` might be a valid value.
It's interesting to note that adding type hints doesn't slow down your code. They are simply instructions for developers and tools, and Python doesn't use them during execution. This means you can add them to existing projects without impacting runtime performance. Modern IDEs have embraced these hints, which has led to improvements in the development workflow. Features like intelligent code completion and real-time error checking make coding in Python more efficient.
This system provides some benefits of static type checking without converting Python into a statically typed language. The flexibility extends to situations where classes might refer to themselves in their definitions, such as in recursive data structures like trees or linked lists. It allows developers to express that a list is intended to hold items of a certain type, using constructs like `List[T]`, making the codebase more understandable.
Another useful feature is protocols, which provide a way to describe behavior without forcing inheritance. This keeps Python's core principle of duck typing intact, but still offers a way to communicate expectations. And when you have complex types, creating type aliases helps make them simpler to understand within your code, simplifying your type hinting strategy.
Even though type hinting is all about hinting, there is still room for runtime type checking in Python. You could use decorators or libraries to put in checks if you need to ensure that data meets certain type requirements, especially in situations where it's crucial to prevent unexpected behavior. It's important to note that while hints can help catch a great deal of issues during development and code review, they don't replace proper runtime validation in all cases.
The gradual adoption of type hinting offers a sensible way to improve existing projects without causing major disruptions. It's a testament to Python's philosophy of evolution rather than revolution, allowing developers to embrace the benefits of enhanced code clarity without needing a wholesale rewrite of their existing systems. As of today, this feature continues to evolve and has become integrated into how many developers approach the creation and maintenance of larger Python projects.
More Posts from aitutorialmaker.com: