Create AI-powered tutorials effortlessly: Learn, teach, and share knowledge with our intuitive platform. (Get started for free)
Efficient JSON Parsing with jQuery A Deep Dive into the getJSON Method
Efficient JSON Parsing with jQuery A Deep Dive into the getJSON Method - Understanding the Basics of jQuery's getJSON Method
jQuery's `getJSON` method offers a streamlined approach to retrieving JSON data using asynchronous HTTP GET requests. The basic structure involves providing a URL (required) and optionally adding extra data or a function to handle successful responses. Developers find it user-friendly due to its simple syntax and ability to incorporate optional parameters. Further enhancing its flexibility, the method returns the XMLHttpRequest object, granting you management over the entire request process.
Since jQuery 1.5, the `getJSON` method has allowed for chaining with functions like `.done()`, `.fail()`, and `.always()`, which is useful when you need more complex error handling and post-retrieval actions. While the default behavior is to return a string, it's beneficial to ensure that the received JSON data is parsed into a JavaScript object to readily utilize its structure. Essentially, `getJSON` simplifies the AJAX process by facilitating the retrieval of server-side data, leading to dynamic website content updates based on the data received. This makes it a valuable asset for crafting more responsive web applications that readily update content based on information from a server.
1. jQuery's `getJSON` method is essentially a streamlined version of the more general `$.ajax` method, specifically tailored for fetching JSON data through HTTP GET requests. It simplifies the process of making these requests, potentially reducing coding errors and speeding up development.
2. One of its key advantages is the automatic parsing of the received JSON response into a JavaScript object. This bypasses the need for manual parsing with `JSON.parse()`, contributing to cleaner and more concise code.
3. If the targeted JSON data is not accessible or isn't in the correct JSON format, the `getJSON` method triggers a `fail` callback. This facilitates robust error handling within your application, preventing unexpected crashes or undesirable behaviour.
4. Interestingly, `getJSON` leverages jQuery's built-in caching system. This means that, by default, it stores responses for a specific URL, potentially boosting performance by avoiding unnecessary server requests for repeated calls to the same endpoint.
5. The method allows you to include optional query string parameters within the URL itself. This feature is useful for passing dynamic variables that can affect the server's response based on user actions or other client-side events.
6. It's important to be aware of security protocols like Cross-Origin Resource Sharing (CORS) and the Same-Origin Policy when working with `getJSON`. Ignoring these could lead to blocked requests if you attempt to access data from a different domain and the server doesn't have CORS enabled.
7. To ensure clarity and consistency, `getJSON` automatically sets the request header to expect JSON data. Manually adjusting this can lead to mismatches in expectations and potential logic errors between the client and server.
8. One of the strengths of `getJSON` is its ability to be chained with other jQuery methods. This enables a more flowing coding structure and facilitates efficient handling of further actions, like rendering data on the page after a successful response.
9. While it offers numerous benefits, it's crucial to acknowledge that `getJSON` can be synchronous in some scenarios, potentially leading to performance bottlenecks if not managed correctly. This becomes particularly important when handling frequent data requests where blocking operations can impede user experience.
10. As JavaScript frameworks like React and Vue gain traction, understanding how `getJSON` can be incorporated into modern asynchronous programming practices is vital. This knowledge enables a smoother transition for developers transitioning from traditional jQuery approaches to contemporary frameworks, ensuring their skills remain relevant in a constantly evolving landscape.
Efficient JSON Parsing with jQuery A Deep Dive into the getJSON Method - Syntax and Parameters of getJSON
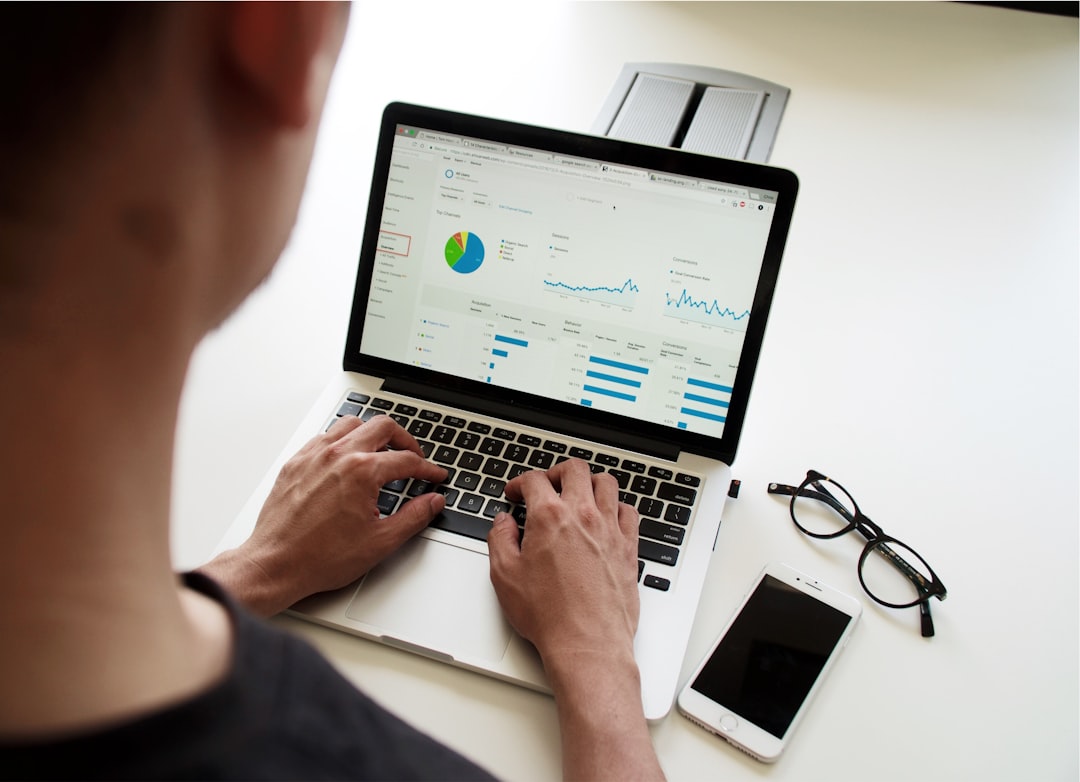
jQuery's `getJSON` method provides a concise way to fetch JSON data from a server using an asynchronous HTTP GET request. Its basic structure involves specifying the URL of the resource (which is required) and optionally adding extra data or a callback function to handle successful responses.
The syntax is fairly straightforward: `$.getJSON(url, data, success)`. The `url` parameter, essential for any request, indicates the server-side location of the desired JSON data. The `data` parameter, while optional, allows you to pass additional information along with the request, often in the form of query string parameters. Lastly, the `success` parameter defines a callback function that jQuery executes if the request is successful. This function receives the fetched JSON data as its argument, making it easy to process and integrate into your application.
The method streamlines the process of retrieving JSON data, making it a popular choice for developers wanting efficient client-server interaction. It's crucial to be aware, however, that the server's response must be valid JSON. If not, it can lead to errors. Furthermore, security implications, particularly CORS, should be considered when retrieving data from different domains. While `getJSON` simplifies the request process, developers must remain mindful of these potential pitfalls to ensure a smooth implementation.
Here are ten noteworthy and sometimes surprising aspects of jQuery's `getJSON` method that might pique the interest of a curious engineer:
1. While `getJSON` conveniently handles JSON parsing, it's important to note that if the server returns invalid JSON, the method fails quite abruptly, often before you can effectively debug it. This emphasizes the need for strong server-side validation to avoid unexpected client-side failures.
2. When working across different domains, `getJSON` abides by the Same-Origin Policy unless the server enables Cross-Origin Resource Sharing (CORS). This security feature can be a source of confusion when interacting with public APIs, as it requires coordination between the client-side and server-side configurations.
3. Beyond just retrieving data, `getJSON` allows you to pass data to the server through query parameters in the URL. This becomes very useful when you want to dynamically retrieve data based on user interactions within your application.
4. The asynchronous nature of `getJSON` can introduce complexities. If developers aren't careful with the management of their callback functions, especially when chaining multiple requests together, they might run into tricky race conditions.
5. Developers sometimes overlook that the basic error callback provided by `getJSON` doesn't distinguish between HTTP errors (e.g., 404 errors) and network problems. This limitation can make debugging more complex and necessitates more sophisticated error handling practices beyond what is readily offered.
6. By default, `getJSON` relies on the HTTP GET method. This can be an issue for large datasets where POST requests might be more efficient. Understanding the strengths and weaknesses of various HTTP methods is key for optimal performance, particularly when handling large databases.
7. While not explicitly part of its core function, `getJSON` can surprisingly be used in scenarios where JSONP (JSON with Padding) is needed. If formatted appropriately, it can facilitate cross-domain communication, even though this isn't its primary intended purpose.
8. The jQuery caching mechanism built into `getJSON` can reduce server load and enhance performance for repetitive requests. But, it can also present a challenge if the cache isn't managed carefully. This can lead to stale data being displayed on the client side, underscoring the importance of proper cache management.
9. One limitation of `getJSON` is its lack of built-in timeout mechanisms. This means that if a request gets stuck, it can potentially hang indefinitely unless you add extra code to manage timeouts, demanding proactive consideration during implementation.
10. In the realm of web development, there's a growing trend toward using native JavaScript features, like `fetch()`, for tasks that `getJSON` previously handled. As web development frameworks like React and Vue gain prominence, this trend may indicate a potential change in the relevance of `getJSON` compared to these more modern, performant alternatives.
Efficient JSON Parsing with jQuery A Deep Dive into the getJSON Method - Handling JSON Responses with getJSON
Successfully integrating JSON data into your web application hinges on effectively handling responses from the server, and jQuery's `getJSON` method streamlines this process. Its primary function is retrieving JSON data asynchronously using HTTP GET requests, and it conveniently parses the response into a JavaScript object, simplifying its use in your code. However, it's vital to recognize that improper JSON formatting from the server can result in sudden failures within `getJSON`, often without providing readily understandable error messages. This highlights the importance of having strong server-side validation to prevent such disruptions. Furthermore, effectively handling errors within the `getJSON` method can be tricky since it doesn't readily differentiate between network hiccups and genuine HTTP error codes. Developers should plan for such situations to create more resilient web applications. By being aware of these aspects and implementing sound error handling practices, you'll create more reliable and dynamic web experiences.
Here are ten intriguing aspects of using jQuery's `getJSON` method to handle JSON responses that might spark the curiosity of a researcher or engineer:
1. While `getJSON` typically expects JSON, it can handle other content types if the server properly sets the `Content-Type` header. This adaptability can prove useful during testing or when dealing with servers that aren't strictly JSON-focused, making debugging across different environments a bit easier.
2. Despite its ability to fetch data from various sources, `getJSON` still adheres to the browser's Same-Origin Policy unless the server explicitly enables CORS. This can lead to unexpected hurdles when working with public APIs that haven't been configured to support cross-origin requests, hindering interaction with third-party services.
3. While not designed for JSONP specifically, `getJSON` can be adapted to leverage it for cross-domain requests by using a `