How to Create Variadic Functions in C++ for Dynamic Parameter Handling
How to Create Variadic Functions in C++ for Dynamic Parameter Handling - Understanding Parameter Pack Basics in Modern C++
Parameter packs are a core element of modern C++ that allows us to write flexible functions and templates that can handle a varying number of inputs. At their core, they're essentially a collection of parameters – zero or more – which can be either template or function parameters. This flexibility is a direct result of the power offered by variadic templates, functions or classes which can be designed to receive an indefinite number of arguments.
The ellipsis (`...`) is the key symbol in this system, signifying a parameter pack. On the left side, it defines a pack; on the right side, it helps expand the pack into individual elements. Furthermore, C++17 introduces fold expressions, offering a way to collapse a parameter pack by applying a binary operator to its elements. This greatly reduces the clutter often associated with manually iterating through each element of a pack.
The capabilities of parameter packs extend even further when combined with tools like `std::tuple` and `std::index_sequence`. This combination allows for intricate data manipulation and the creation of dynamic functionality that would be challenging to achieve using traditional methods. Through pack indexing, developers can directly access individual elements within the pack, giving them fine-grained control over how the pack is processed. And by carefully applying unpacking techniques, often using recursion, you can manage parameter packs and their components efficiently.
Ultimately, parameter packs serve as a powerful tool for developers, particularly library designers. Variadic functions – which leverage parameter packs – create a mechanism for developing functions that can adapt to various inputs without requiring prior knowledge of the number of arguments that will be supplied. This flexibility is beneficial for crafting powerful, reusable components in C++.
1. Parameter packs, introduced in C++11, provide a mechanism for functions and templates to handle a variable number of arguments, significantly broadening the scope of generic programming. This capability simplifies the creation of functions that can operate on diverse sets of inputs without needing to anticipate the precise number or types beforehand.
2. The core syntax for defining a parameter pack relies on the ellipsis (`...`) operator. This seemingly simple construct empowers engineers to build functions effortlessly adaptable to varying numbers and types of parameters, fostering a level of genericity previously challenging to achieve.
3. The same ellipsis operator used to declare parameter packs also plays a key role during function calls where packs can be expanded. This allows for convenient forwarding of multiple arguments to other functions, thus simplifying and unifying the overall handling of function parameters.
4. The combination of parameter packs and template metaprogramming unlocks a new level of generic functionality. It enables the creation of highly flexible and reusable components capable of adapting their behavior based on the types and number of arguments received. This can lead to remarkably elegant and reusable solutions.
5. For advanced manipulation of pack elements, techniques like integer sequences through `std::index_sequence` offer an avenue for accessing and processing specific pack elements. However, it is worth mentioning that the utility of this approach hinges on situations where fine-grained control and potential optimization over raw pack operations are required.
6. The C++ Standard Library's constructs like `std::tuple` work seamlessly with parameter packs. This integration enables the elegant definition of complex data structures with variable element types and quantities, providing a mechanism for concise and adaptable data representations.
7. Recursive template instantiation is a common pattern when working with parameter packs, offering a way to handle each argument individually. This mechanism effectively demonstrates the power of templates in handling variadic functions. However, recursive structures can be difficult to follow, hence careful thought to code readability is important.
8. While undeniably powerful, the excessive use of parameter packs can lead to a decrease in code readability and maintainability. Striking a balance between leveraging their capabilities and ensuring code clarity is essential to avoid complex and unwieldy function implementations.
9. The flexibility provided by variadic templates brings with it the need to carefully manage type safety. Failing to handle types correctly can lead to ambiguities that manifest as confusing compiler errors, causing development slowdowns and added debugging efforts.
10. The capability of parameter packs allows C++ functions to handle heterogeneous argument types effectively. This attribute makes it significantly easier to design APIs where the number and types of inputs aren't predetermined, paving the way for more adaptable and robust software interfaces.
How to Create Variadic Functions in C++ for Dynamic Parameter Handling - Template Recursion for Handling Dynamic Parameters
Template recursion is a key technique for managing the dynamic nature of parameters within C++'s variadic functions. This technique involves recursively processing parameter packs, which are collections of arguments with varying types and numbers. Through recursion, developers gain the ability to handle a wide range of input scenarios, fostering more flexible function designs and promoting type safety. While this approach offers significant advantages in creating reusable components, developers need to prioritize code readability and avoid overly complex structures. The nature of recursion can sometimes complicate debugging, making robust type management crucial in variadic templates. It's worth noting that while template recursion empowers developers to harness the versatility of dynamic parameters, it is important to strike a balance between its use and overall code simplicity. Template recursion effectively showcases how C++'s template mechanisms can be utilized to solve complex programming problems elegantly.
1. Template recursion, when used with templates, can create solutions that address dynamic parameters at compile time. This means the compiler figures out the types of parameters and their appropriate behavior before the program even runs, potentially leading to more efficient code. However, compiler limitations can exist.
2. The depth of recursion we can achieve with templates is bound by a compiler-specific limit – generally hundreds of recursion levels – as dictated by the C++ standard. So while templates are powerful, this limitation does exist.
3. C++ provides tools like SFINAE (Substitution Failure Is Not An Error) and type traits that can help manage the complexity of recursive template metaprogramming. These allow us to conditionally activate or deactivate function templates based on the arguments received, which can make the logic easier to manage.
4. While recursion can be the backbone of managing variadic templates, sometimes it's advantageous to hide the complexity. Helper structures or type traits can often be used to abstract the recursion process, improving the usability of a function while ensuring the code stays clear and functional.
5. It's intriguing that template recursion, a seemingly complex concept, can achieve outcomes similar to conventional loops. Tasks like adding up a series of values or accumulating data can be accomplished with a cleaner, more type-safe approach using template recursion compared to iterative approaches. It is interesting, isn't it?
6. The efficiency of recursive template expansion versus its iterative counterpart is usually indistinguishable with small datasets. But, if the parameter pack becomes substantial, the compile-time overhead associated with recursive expansion might grow. This can become a factor in the overall efficiency and is good to keep in mind.
7. Advanced user-defined data types fit comfortably within recursive templates. This allows for customized behaviors when handling groupings of these types. For example, we could potentially create unique serialization or data transformation logic specific to these user-defined types.
8. Combining recursive templates with variadic templates and fold expressions can lead to impressive results. It enables us to perform dynamic aggregations of results across multiple types within a single operation, enhancing the versatility of our solutions.
9. Unfortunately, debugging template recursion can be a challenge. The error messages, generated during compile time, can be confusing and obscure. This difficulty is partly because the error messages arise during the compilation process which may not reflect the programmer's intended code. To mitigate some of the challenges of debugging, static assertions can help diagnose errors earlier.
10. A little-known, but interesting, property of recursive template parameter packs is their ability to enforce compile-time checks on the number of arguments. Essentially, it means we can make sure function calls adhere to our specifications without the need for runtime checks. This ability to enforce constraints early, at compile time, helps prevent certain runtime errors.
How to Create Variadic Functions in C++ for Dynamic Parameter Handling - Memory Management with Variable Arguments
When working with variadic functions, managing memory becomes particularly important. These functions, capable of accepting a variable number of arguments, introduce a degree of flexibility that can be very useful, but also require careful handling to prevent problems. If not managed properly, these functions can lead to undefined behavior or memory leaks due to the unknown nature of the inputs they might receive.
To ensure safe memory management, you need to use the tools provided by `stdarg.h` (like `va_start` and `va_end`). These tools help you iterate through the arguments and ensure that resources are released correctly. Furthermore, the inherent complexity of working with different argument types can make memory management more difficult, so developers should focus on creating clear and well-defined code structures. Failing to do so can result in hard-to-debug issues that impact application reliability and correctness. Variadic functions can be powerful, but it's crucial to use them responsibly and maintain control of the memory associated with their inputs. Otherwise, the potential for bugs and unpredictable program behavior increases considerably.
1. When it comes to memory management, how we handle variable arguments in functions can significantly impact performance. If we're not careful, we can easily introduce memory leaks or buffer overflows, which are especially problematic in demanding applications. Keeping a tight rein on memory management is crucial for maintaining stability.
2. Variadic functions, while flexible, can lead to larger binary files. This happens because the compiler needs to create a unique version of the function for every possible combination of argument types. This behavior is a direct result of the template system.
3. The level of optimization that the compiler applies to variadic templates can vary depending on the specific situation. This means performance gains from using variadic functions can be inconsistent, and we should consider this when dealing with systems where resources are limited.
4. Mixing parameter packs with move semantics provides an interesting avenue for memory management, especially when working with large objects. Using rvalue references alongside parameter packs can allow us to efficiently transfer ownership of resources rather than copying them, thus reducing overhead and potentially saving valuable time.
5. C++'s variadic functions can handle both basic data types and complex user-defined types, which opens up a lot of possibilities for memory management. However, we have to carefully control type safety to avoid undefined behavior when managing resources across different types. It's a balancing act.
6. The way we use template metaprogramming and recursion in variadic functions can unexpectedly affect stack memory allocation. If we're not mindful of how deeply we recurse, we can easily run into stack overflow errors, particularly in systems with limited stack space.
7. Variadic templates inevitably lead to a complex interplay of overloads and possible type combinations. It's important to think carefully about how we design our functions to make sure memory allocation and deallocation are consistent with the expected types. Good documentation and established coding conventions are vital for clarity in such complex systems.
8. Variadic templates allow us to write less repetitive code, but this gain comes with a price. The added complexity requires thorough testing to prevent subtle bugs or performance slowdowns related to memory management.
9. Since variadic functions handle different data types, implicit conversions can sometimes lead to surprising outcomes when managing memory. These conversions can lead to mismanaged resources or incorrect allocations. Therefore, thorough type checks and clear documentation are even more important than usual.
10. The introduction of fold expressions in C++17 has considerably streamlined operations involving parameter packs. Now we can perform cumulative operations across many arguments directly. This helps reduce overhead and decreases the likelihood of errors while managing memory during aggregation operations.
How to Create Variadic Functions in C++ for Dynamic Parameter Handling - Using va_list and va_start in Legacy Code
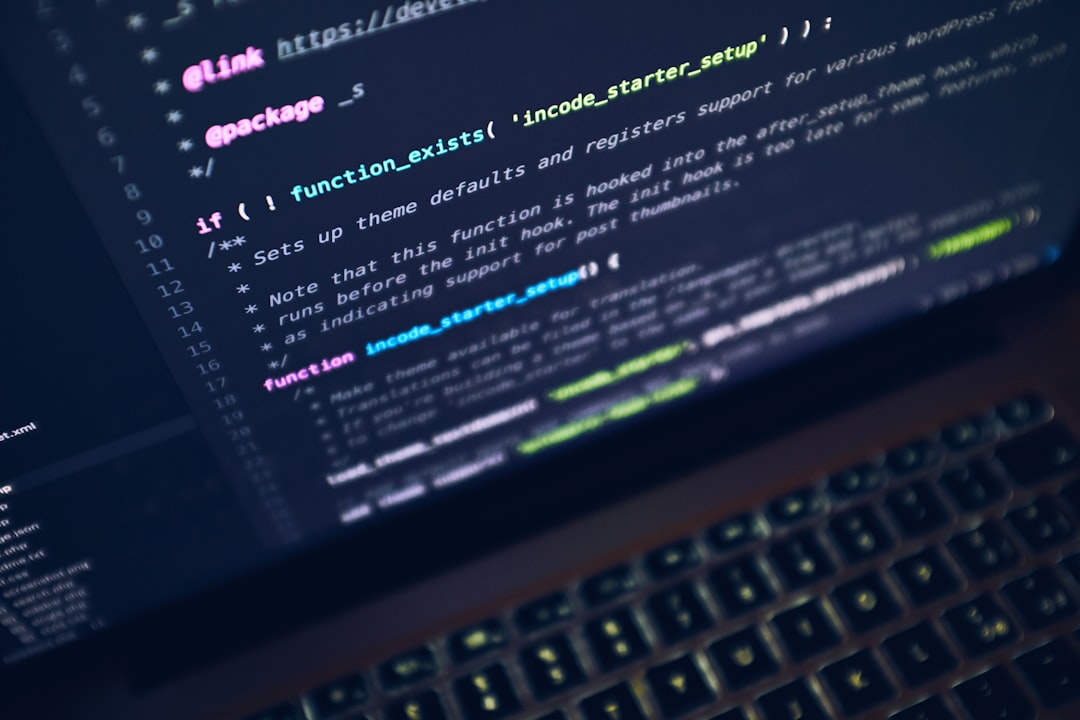
When dealing with older C code, understanding how to use `va_list` and `va_start` becomes essential, especially when functions need to handle a variable number of arguments. These tools, part of the C standard library, are the foundation for creating functions that can accept a flexible range of input parameters. However, implementing them correctly requires diligence in tracking argument types. If you don't manage them carefully, you risk running into runtime errors. It's vital to use `va_end` to release resources related to the `va_list` to prevent memory leaks or related problems. While these functions provide a valuable level of flexibility, they also introduce more complexity into the code. Developers must strive to write code that's both adaptable and reliable. Otherwise, the increased flexibility may simply introduce further opportunities for problems.
### Surprising Facts about Using `va_list` and `va_start` in Legacy Code
1. The existence of `va_list` and its associated macros like `va_start` shows us that handling a variable number of arguments has been a concern in C for a long time. It's a reminder that some aspects of traditional C linger, even in modern codebases. While modern C++ offers powerful alternatives, there are times when it's simply not feasible to switch over in legacy code.
2. Using `va_list` isn't as type-safe as modern C++ variadic templates. We don't get the compiler's help in verifying the types of the arguments until runtime, which can make debugging tricky. This means rigorous testing and detailed comments within code are even more crucial when working with legacy code that relies on `va_list`.
3. There's a cost to this flexibility. Because `va_list` gathers all the extra arguments into a single block, it can potentially lead to bigger memory consumption. For programs that run for a long time and use `va_list` frequently, this could lead to noticeable memory use, and worse, possible memory leaks if not managed precisely.
4. The dynamic nature of arguments handled by `va_list` can impact performance. Functions using `va_list` might not be as fast as functions with fixed argument lists because the runtime has to work a bit harder to deal with the various types of inputs. This performance hit might be substantial in programs that prioritize speed or run on resource-constrained hardware.
5. Compared to modern C++ techniques, `va_list` has its limitations. Features like automatic argument type inference or the ability to easily expand argument packs aren't available. This makes certain tasks more cumbersome in older code and introduces the possibility of more human errors compared to newer techniques.
6. Interestingly, C offers variadic macros alongside `va_list`. Variadic macros are a clever way to build functions that can accept a flexible number of arguments within a macro. While it's a feature that can be helpful for things like logging or debugging, these macros face the same type safety issues we've discussed with `va_list`.
7. The implementation and behavior of `va_list` can differ across compilers and platforms. This can be a headache if we're writing software that needs to work on various systems. It adds another level of complexity and can potentially lead to unexpected issues in cross-platform projects.
8. When bugs appear, tracing errors within `va_list`-based code can be quite tricky. Debugging tools might not always understand and present variable arguments correctly, making it harder to pinpoint where the issues are stemming from during the processing of these arguments.
9. Maintaining the proper order of arguments when using `va_list` is crucial. Unlike some modern techniques where order might be less strict, this traditional approach requires precise adherence to the sequence defined by the function. The lack of flexibility can inadvertently increase the chance of coding errors.
10. When we're working with `va_list`, it often feels like manipulating a stack. Understanding how arguments are "pushed" onto the stack when the function is called, and then how they're "popped" off as we process them is fundamental. Mixing these up can lead to unexpected and frustrating corruption of the stack, which can cause our program to behave in very strange and hard-to-predict ways.
How to Create Variadic Functions in C++ for Dynamic Parameter Handling - Implementing Type Safe Parameter Packs
Following up on the introduction to parameter packs, this section focuses on how we can implement them in a way that ensures type safety. C++'s variadic templates offer a significant improvement over older C-style variadic functions when dealing with a changing number of arguments. Instead of relying on runtime checks like in traditional C, C++ variadic templates enforce type safety at compile time. This ensures the code's integrity by catching errors early on, before the program even executes, leading to more robust code.
We can further enhance our control over the parameters by incorporating elements like `std::tuple` or `std::integral_constant` within our variadic templates. These tools allow for a more refined management of parameter types and sizes, giving us more flexibility to create functions that handle a wide range of inputs dynamically while keeping the code type-safe.
However, it's crucial to be aware that the power of variadic templates comes at a cost—complexity. Carefully managing the level of complexity introduced by type-safe parameter packs is essential to ensure code maintainability and readability. If not handled carefully, type-safe parameter packs can make code difficult to understand and maintain.
1. C++'s type-safe parameter packs offer a compelling advantage: compile-time type checking, which significantly cuts down on the runtime errors we often encounter when dealing with a variable number of arguments. This early detection of type mismatches can make debugging much easier, allowing us to fix problems sooner.
2. Achieving type safety with parameter packs often involves using type traits and SFINAE (Substitution Failure Is Not An Error). These advanced features guide the compiler to selectively enable or disable specific template versions based on the types of arguments used. It's a clever way to keep things flexible while also enforcing type correctness.
3. Pairing type-safe parameter packs with C++20's concepts lets us define constraints right within the template parameters. This approach helps enforce the desired types and relationships at compile-time, leading to more robust code and better adherence to intended API behaviors.
4. While valuable, the implementation of type-safe parameter packs can necessitate deeper knowledge of template metaprogramming. This might make the learning curve steeper for newcomers to the field. Understanding this kind of advanced template work could deter some developers from fully embracing the power of type-safe code.
5. Though beneficial, excessive reliance on complex templates can hurt code readability. Over-engineered solutions, even if type-safe, can make code hard to understand and maintain. It's vital to strike a balance between achieving type safety and writing clear, understandable code.
6. One interesting aspect of type-safe parameter packs is their ability to work with intricate type hierarchies, including user-defined types. This opens up possibilities for specialized type handling, like creating custom serialization methods. Such approaches wouldn't be easy or safe using older methods of handling a variable number of arguments.
7. The efficiency of operations with type-safe parameter packs can be impacted by the extent of template instantiation. While recursive techniques elegantly handle expansion of packs, too much recursion can sometimes exceed compiler limits. When this happens, it can cause bewildering compilation errors, even for experienced developers.
8. When using type-safe parameter packs, we need to pay close attention to the lifetimes of temporary objects. If we aren't careful, we could end up with dangling references or objects in unexpected states, leading to subtle and hard-to-track issues.
9. Type-safe parameter packs let us express our intentions more directly within function signatures. We can define the types explicitly, making the code easier to read and understand, while also ensuring that the underlying logic remains sound. It emphasizes the importance of clarity and robustness.
10. When deciding whether to use type-safe parameter packs, it's worth considering any potential performance trade-offs, particularly with large parameter packs. Although compile-time checks prevent many issues, the initial compilation process can sometimes take longer, especially in environments with limited resources.
How to Create Variadic Functions in C++ for Dynamic Parameter Handling - Error Handling Strategies for Multiple Arguments
Variadic functions in C++ offer a powerful way to work with a dynamic number of arguments, but this flexibility comes with a responsibility to manage potential errors effectively. Because these functions can handle multiple arguments of different types, the possibility of mismatches between the expected and provided arguments increases, leading to potential runtime errors.
A simple way to approach this is using sentinel values, a special marker that indicates the end of the argument list. This allows the function to clearly understand when to stop processing inputs, avoiding accidental overrun or access to unintended data. Another method involves validating the type and count of arguments before calling the function. This approach, while more cautious, does impose some overhead because it requires extra checks within the code.
Modern C++ provides a more type-safe approach using variadic templates. These powerful templates, introduced in newer versions of C++, let the compiler perform checks for argument type and count issues *before* your code runs. This can reduce runtime issues because mismatches are detected early. However, the features that offer type safety in these templates also add a level of complexity to the code. Keeping this code clean and well-documented becomes critical because too much complexity can obscure the purpose of the code. This aspect is important when you're designing a library or when maintainability matters long term.
1. When dealing with multiple arguments in variadic functions, we often find that the performance characteristics can vary. Variadic functions, by their nature, require runtime type checks, potentially leading to slower execution compared to parameter packs. Parameter packs, in contrast, leverage compile-time checks, making them more efficient in some cases, highlighting a potential trade-off we need to consider.
2. The flexibility of parameter packs can create a challenge known as "template bloat." This happens when the compiler generates an abundance of code for various combinations of arguments. This increase in binary size could be detrimental in specific scenarios, especially when running in resource-constrained environments such as embedded systems or applications requiring strict performance guarantees.
3. While the type flexibility of variadic templates is quite valuable, it also brings with it the potential for ambiguities. Type mismatches, if they occur during template expansion, can lead to hard-to-predict runtime behavior and significantly complicate debugging. This unpredictability contrasts with fixed arity functions, where type behavior is more predictable.
4. The ability to use `std::apply` in conjunction with parameter packs has become quite popular for simplifying the handling of argument lists. `std::apply` makes it easier to invoke callable objects on tuple-like structures by unpacking their elements. This function is a helpful tool to streamline the management of multiple arguments, thus reducing complexity in various scenarios.
5. We have a balancing act to perform when using parameter packs. The type safety they offer during compile time comes with a trade-off against runtime flexibility. If an application requires optimal performance, we need to consider the value of type safety relative to the adaptability offered by traditional variadic functions.
6. When combining parameter packs and template specialization, we need to be very careful. Mistakes during specialization can lead to the wrong overload being selected, causing hard-to-track bugs that pop up unexpectedly. This behavior can lead to debugging sessions that are longer and more challenging than we would like.
7. C++17's introduction of fold expressions provided a significant improvement in how we process parameter packs. Fold expressions, however, need to be applied carefully. Incorrect application of fold expressions can inadvertently introduce subtle logic errors that are not as apparent as errors in traditional for loops, emphasizing the need for detailed testing.
8. The powerful capabilities of variadic templates come at the price of increased complexity. Developers accustomed to more traditional C-style coding can experience a bit of a learning curve when transitioning to variadic templates. This transition, if not approached carefully, could lead to a decrease in initial development productivity as developers become more comfortable with the associated features and behaviors.
9. When we engage in recursive template processing, especially with a larger number of parameters, we need to ensure we don't exceed compiler limitations in terms of template instantiation depth. This can lead to exceptionally cryptic and unhelpful compiler error messages, posing a particular challenge for even more experienced developers.
10. While the design of APIs that employ parameter packs allows for fantastic flexibility, it can lead to a function signature that is difficult to understand at a glance. This increased complexity necessitates exceptionally detailed documentation to clearly convey intended usage and behavior. This detailed documentation is crucial for ensuring that developers who consume our APIs do so correctly and with minimal difficulty.
More Posts from aitutorialmaker.com: