Unveiling the Anatomy of C++'s Classic Hello World Program in 2024
Unveiling the Anatomy of C++'s Classic Hello World Program in 2024 - The Evolution of C++ Syntax in 2024
The C++ syntax we see in 2024 is notably different from its earlier iterations. It leans towards more concise and expressive code while prioritizing safety. We've seen a continuous stream of language additions that help streamline the coding process, making code shorter without compromising readability. Type inference and automatic deduction have become increasingly prominent, reducing boilerplate code. C++20's introduction of concepts and ranges has opened the door to further refinements, bringing C++ syntax more in line with modern coding styles. This evolution is visible even in the classic "Hello World" program, which now acts as a window into not only the language's fundamental syntax but also its capacity to tackle complex challenges with grace and speed. These changes are shaping how we interact with C++, creating a more intuitive and powerful language for developers. While the core principles of C++ remain, this evolution is making the language more adaptable and efficient.
The C++ syntax landscape in 2024 reflects a continued evolution, incorporating features that influence how even the most basic programs, like "Hello World", are written. The integration of functional programming concepts encourages a shift away from traditional object-oriented approaches, leading programmers to think more in terms of functions and algorithms.
The C++20 features, now fully integrated into the 2024 standard, have strengthened type checking and template metaprogramming without sacrificing clarity. This advancement is particularly helpful for debugging larger projects. The `auto` keyword has gained more power through auto-deduction guides, reducing repetitive code and improving readability, even in simple examples.
The handling of asynchronous operations has been fundamentally altered with enhancements to coroutine support, simplifying the management of I/O operations. The new syntax also offers improved capabilities for lambdas, allowing more flexible capture and less restrictive lambda expressions, ultimately influencing the way encapsulated behaviors are designed.
The introduction of ranges as a built-in feature facilitates more expressive data manipulation, letting programmers communicate their intentions more succinctly. It's now easier to avoid verbose iterations in introductory programs. C++'s syntax now encourages better memory management through a greater emphasis on smart pointers, guiding developers towards safer practices and mitigating the risk of memory leaks that plagued previous versions.
The spaceship operator, a three-way comparison operator, has been fully adopted, streamlining the creation of custom comparison logic. This simplifies algorithms like sorting by reducing redundant code. The evolution of modular programming in C++ has modified how header and source files are organized, potentially impacting compilation times. This directly impacts how simple examples like "Hello World" can be structured in a more modular way.
Finally, a push for better code readability in C++2024 is evident in the emphasis on naming conventions and formatting standards. This trend promotes cleaner and more consistent coding styles, even in the simplest programs, facilitating collaboration among developers. It's interesting to observe how these evolving syntax elements shape the foundational "Hello World" program and ultimately, the larger C++ ecosystem.
Unveiling the Anatomy of C++'s Classic Hello World Program in 2024 - Breaking Down the #include Directive
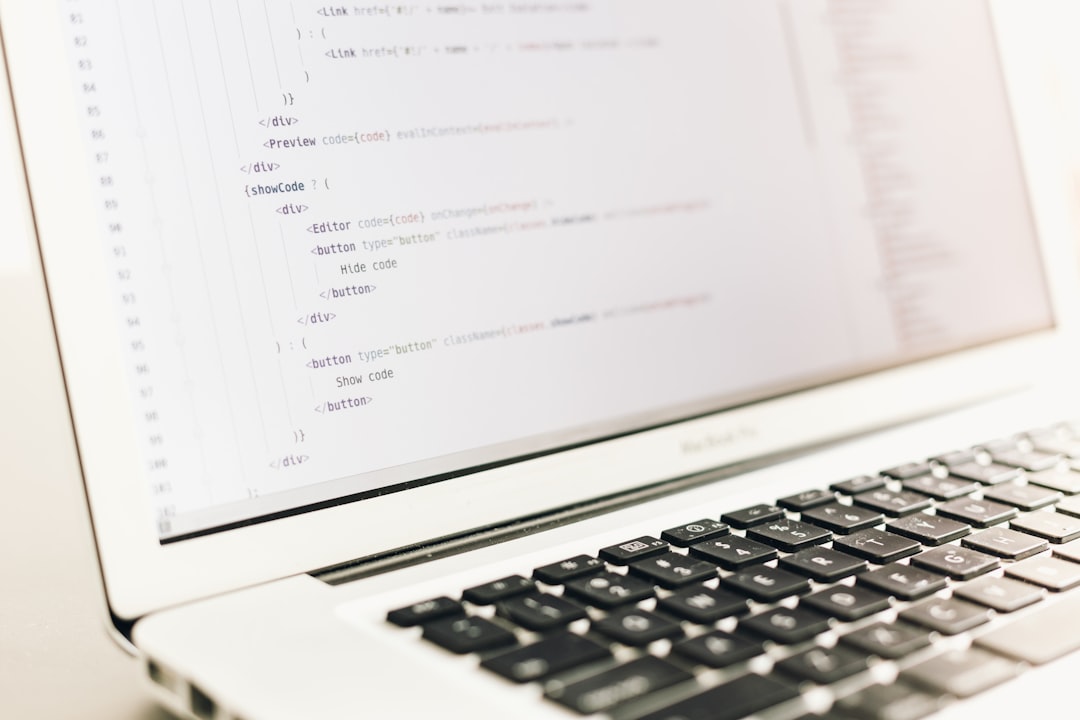
In the core of any C++ program, even the simplest "Hello World," lies the `#include` directive. It acts as a bridge, bringing in external code, typically from header files, before the compilation process begins. This allows us to tap into the power of pre-written libraries and functions, including essential ones like ``, which is indispensable for input and output operations. Without it, tasks like displaying output to the console in our "Hello World" wouldn't be possible.
While the `#include` directive simplifies our lives by providing access to readily available tools, it also creates dependencies. If a program relies heavily on specific external files, it can become harder to move or modify without considering the implications. This is especially relevant in today's landscape where C++ continues to evolve.
Learning how to use the `#include` directive proficiently is a cornerstone of C++ development. It's fundamental for crafting programs that are not only functional but also well-organized and easy to manage. The increasing complexity of modern C++ projects highlights the need for this basic knowledge to build efficient and maintainable code. As the language continues to embrace new paradigms and features, effectively utilizing this essential directive becomes more important than ever.
The `#include` directive acts as a preprocessor command, meaning it's processed before the compilation stage. It shapes how our source code is organized and compiled in C++. Unlike many other languages where libraries are linked during runtime, C++'s `#include` mechanism directly incorporates header files' contents into our source code. While this can lead to faster compilation times, it can also contribute to larger executable sizes if not handled carefully.
This inclusion process can trigger a cascade of other includes, potentially resulting in a situation called "include hell." In this scenario, extensive dependency chains cause a significant increase in compilation times. To mitigate this, techniques like header guards or `#pragma once` become crucial. These prevent the same header file from being included multiple times, which avoids redefinition errors and keeps compile times reasonable.
The `#include` directive can also bring in standard libraries. The way we specify the path – using angle brackets (`<>`) or quotes (`""`) – determines whether the compiler searches in system directories or user-defined locations. This highlights C++'s flexibility, allowing us to tap into a rich ecosystem of readily available libraries and fostering modular code development.
However, relying on relative paths in `#include` directives can introduce portability problems, particularly if the file organization within a project changes. It's become more common in modern C++ projects to use absolute paths or structured directory arrangements to enhance the stability of the code.
The `#include` directive contributes to C++'s "One Definition Rule" (ODR), ensuring that functions, variables, and types have only one definition throughout the program to prevent linker issues. While the ODR's implications aren't always rigidly enforced in practice, it remains a foundational principle of C++'s design.
As C++ evolves, the interplay between `#include` and modular programming approaches in C++20 becomes increasingly interesting. It allows for more distinct separation of interfaces and implementations, ultimately improving code maintenance and potentially reducing compilation times.
The simplicity of `#include` can be deceiving. It plays a vital role in C++ development, serving as the link between the core language and its extensive libraries. This emphasizes the importance of carefully managing dependencies in order to craft efficient and effective programs. It's an interesting mechanism that highlights some of the choices made in the design of C++.
Unveiling the Anatomy of C++'s Classic Hello World Program in 2024 - The Main Function Structure and Its Purpose
The `main` function in C++ acts as the entry point for any program, conventionally declared as `int main()`. This function signifies the initial point of execution, establishing the flow of the program. Its return type, `int`, allows it to signal whether the program has run successfully or encountered errors. Typically, a return value of zero indicates a successful completion. Inside the `main` function, developers can utilize components of the C++ standard library, such as `std::cout` for outputting messages to the console. This enables programmers to easily interact with the user, even in basic programs. Structuring code logically within the `main` function is crucial for readability and maintainability, highlighting the importance of good programming practices. As C++ continues to evolve, the `main` function retains its fundamental role while integrating new language features and coding approaches.
The `main()` function in C++ serves as the starting point for program execution, acting as the entry door for the operating system to begin running the code. Importantly, it's designed to return an integer, which is passed back to the OS as an exit status code. This convention, where a return value of 0 usually signifies success and non-zero values suggest errors, is a remnant from C and offers a standardized way to understand program outcomes.
While C++ offers flexibility in defining `main()`, including the option to accept parameters, the most basic and often preferred form – `int main()` – keeps things simple and readable, particularly for introductory examples like the "Hello World" program. It's interesting to note that compilers usually demand exactly one `main()` function to orchestrate the execution flow, meaning multiple definitions will result in a compilation failure. This highlights its central role in defining the program's overall structure.
Some C++ implementations allow omitting the return statement from `main()` and implicitly supply a default return type. However, this is not considered good practice because it introduces uncertainty about how a program terminates. It’s always safer and clearer to provide explicit return statements in `main()` for better program control and understanding.
The C++ standard provides leeway in the number of arguments that `main()` can accept, although the most common forms are either no parameters or a single parameter (an array), yet this flexibility might cause confusion or lead to unexpected behavior. This is a side effect of inherited structure from C programming, for compatibility. This simple function, in essence, reflects the design ethos of both languages: providing straightforward and efficient coding approaches.
Interestingly, `main()` can lay the groundwork for sophisticated features, such as parsing command-line arguments. Users can then pass specific values when they launch the program, leading to dynamic behavior controlled by user input. While this is useful in larger applications, it emphasizes that this function is not just the starting point for execution, but a communication link between the program and the user.
The C++ standard puts certain limitations on the use of global variables before the execution of `main()`, demonstrating a degree of control over initialization order to avoid undefined behavior during startup. This implies a thoughtful orchestration of initialization sequences, emphasizing a cautious approach towards system stability.
Finally, even before `main()` starts, certain events can be triggered through static initializers placed in the global scope. This enables programmers to establish the program's initial environment or perform specific checks before the core logic takes over. It showcases the level of control that C++ offers in managing program startup. However, there's a potential for some compiler-specific variations in how this setup happens due to platform differences or optimization settings. This variation could impact the program's behavior or performance across different platforms.
This discussion underscores that while `main()` may seem straightforward in the "Hello World" program, it plays a much more crucial role in the broader context of C++ applications. Its structure, while simple, shapes the entire program's flow, interaction with the system, and how initialization processes are handled. It's a testament to how carefully structured even the foundational blocks of a program can be.
Unveiling the Anatomy of C++'s Classic Hello World Program in 2024 - Understanding cout and the iostream Library
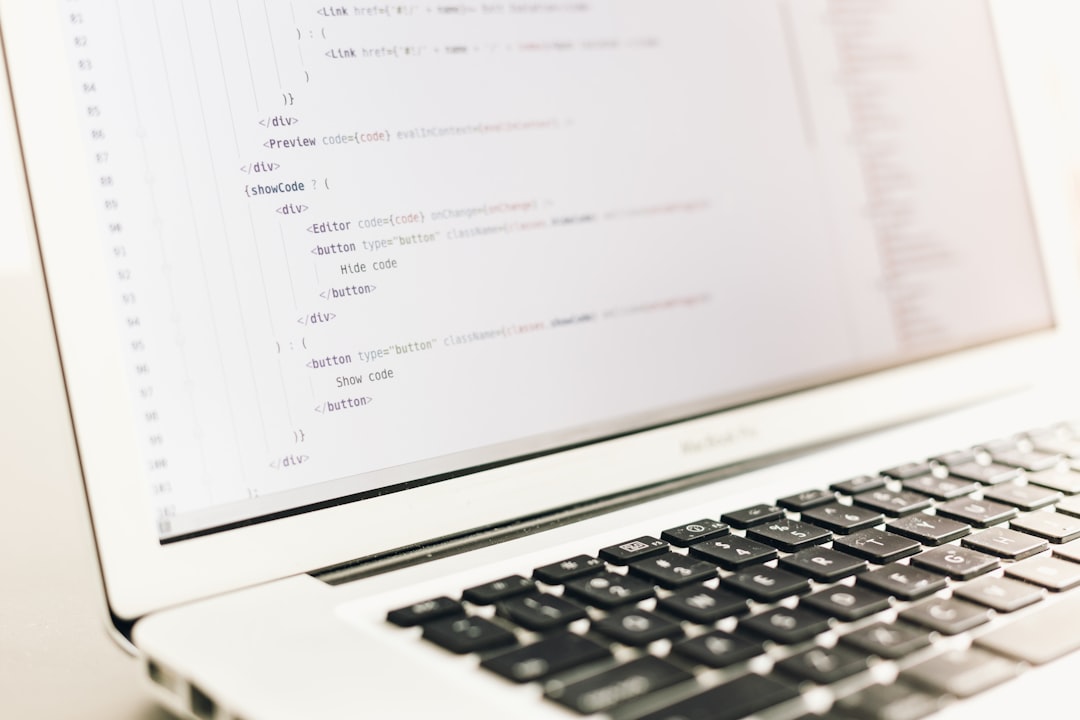
The `iostream` library, accessed through `#include `, is fundamental to C++'s input and output capabilities. Central to this library is `cout`, an object representing the standard output stream, where programs send information to be displayed on the console. Programmers commonly utilize manipulators like `endl` alongside `cout` to control the formatting of output, like inserting new lines and ensuring data is promptly written to the console. Beyond basic output, the `iostream` library provides type safety when interacting with different data types, reflecting C++'s emphasis on flexibility and control. Further, it offers additional output streams such as `cerr` and `clog`, enabling more refined management of error messages and log output, making the language more suitable for today's complex applications. These elements of the `iostream` library are a vital part of how C++ programs interact with users and the wider system.
The `#include ` directive is essential for using the `cout` object and its associated functions within a C++ program. `cout`, essentially an object belonging to the `ostream` class, represents the standard output stream (stdout). Interestingly, it gets initialized before any `ios_base` object is constructed, hinting at its foundational role in C++'s output mechanisms.
The `endl` manipulator is frequently paired with `cout` to inject a newline character and flush the output buffer. This ensures that all output is promptly written to the console, which can be important in various situations where immediate visual feedback is crucial. The `iostream` library, as its name implies, handles both input and output operations in C++. `cin` manages standard input (stdin), while `cout` is responsible for standard output. Both of these are part of the `std` namespace, necessitating the inclusion of the `iostream` library.
Besides `cout`, the `iostream` library also provides other output stream objects like `cerr` and `clog`. `cerr` is unbuffered and commonly used for displaying error messages, whereas `clog` is buffered and intended for logging purposes. This variety offers programmers more flexibility in managing output based on context.
The library's object-oriented design empowers developers to perform input and output operations on various data types, including files and streams. This flexibility extends beyond the simple `cout` and `cin` interaction. A typical C++ program that utilizes `iostream` will typically include input statements that prompt the user for information (through `cin`) and output statements that display results (via `cout`).
It's crucial to note that `iostream` isn't an integral part of the C++ language itself but rather a component of the C++ standard library. This characteristic sets C++ apart from C, which doesn't have an equivalent built-in mechanism for input/output. This reliance on the standard library provides an additional layer of flexibility and extensibility to the language. Special formatting characters like `\n` can be integrated with `cout` for controlling output, such as advancing to a new line. This demonstrates the level of control that C++ gives us over how information is presented to the user.
Unveiling the Anatomy of C++'s Classic Hello World Program in 2024 - Exploring Modern C++ String Handling
Within the realm of modern C++, the way we handle strings has undergone a substantial transformation. The older C-style strings, essentially character arrays that needed a null terminator to mark the end, have been largely replaced by the more robust `std::string` class. This shift offers several advantages, including easier manipulation and a degree of safety against potential memory issues like buffer overflows that were quite common in older C++ code. Modern C++ provides a collection of methods specifically designed to work with `std::string` objects, facilitating tasks like concatenation and string modification in a much more intuitive and safer manner. Notably, C++20 introduced features such as ranges and concepts that refine string processing, ultimately contributing to improved performance and better readability. It's a compelling illustration of how the evolution of C++ emphasizes greater ease of use while simultaneously providing more expressive capabilities to developers. These enhancements in string handling are a significant step toward making C++ more intuitive and developer-friendly, particularly for those coming to the language from other contexts.
Exploring the realm of string handling in modern C++ reveals a fascinating evolution from the more rudimentary C-style approaches. We find ourselves with tools that are both powerful and surprisingly nuanced.
Let's consider the use of string literals and rvalue references. C++11 introduced the ability to treat string literals as rvalue references. This offers a chance to use move semantics in a more efficient way when you pass them to functions. This is especially helpful for applications where performance is crucial because it minimizes needless copying.
Another interesting facet is `std::string_view`, introduced in C++17. This feature lets you have non-owning views of string data. In essence, you can access and work with string data without making a copy. This can significantly improve performance and memory usage in situations where you're working with substrings.
Unicode support has also become more prominent in C++20. Enhanced libraries now make it easier to handle UTF-8 encoded strings. This is a significant change, particularly in the context of globalization and software development. It's now easier to cater to a broader audience without making code overly complex.
Unlike raw char arrays, `std::string` conveniently manages memory automatically. This removes the potential for common issues such as buffer overflows or dangling pointers. This built-in safety net is paramount for today's applications where security and stability are of utmost concern.
However, there are subtle nuances to be aware of. For instance, string concatenation using the '+' operator can have performance overheads due to the generation of temporary strings. Modern C++ recommends using `std::string::append` for a more efficient approach. This is a good example of how understanding the performance implications of seemingly simple operations is crucial.
C++ also provides an interesting avenue for customized memory management through the use of custom allocators for `std::string`. This flexibility allows for more advanced memory management strategies, especially in situations where specialized handling can improve performance. This ability to create custom solutions to memory management is particularly useful when working with applications in very performance-constrained software environments.
While other languages have embraced the ease of string interpolation, C++ hasn't taken quite the same route. But, there's a convenient alternative, `std::format`, part of the C++20 standard. It provides a way to construct complex string outputs in a more expressive and powerful way.
Furthermore, C++ encourages immutable operations when working with strings. Operations often involve generating new strings instead of directly modifying the existing one. We need to understand how this immutability can impact performance when aiming for highly efficient applications.
The introduction of ranges in C++20 has had a beneficial impact on string manipulations. They allow us to perform more concise and expressive operations. This approach not only makes code easier to understand, but it can also lead to better performance due to lazy evaluation when working with string sequences.
Despite the advances in C++ string handling, developers need to be mindful of internationalization challenges. These include potential locale-specific behavior and string normalization, which can affect how strings are displayed and processed across diverse user communities. This emphasizes the importance of thorough testing across different platforms to mitigate any unexpected surprises.
Ultimately, C++ string handling has undergone significant refinement, offering developers more powerful tools while maintaining a focus on performance and safety. This is especially interesting when considering how much more sophisticated software development has become.
Unveiling the Anatomy of C++'s Classic Hello World Program in 2024 - Compilation Process and Memory Management in 2024 C++
In 2024, the C++ compilation process and memory management have undergone refinements, influencing how developers work with the language. The compilation process remains a multi-step journey, involving stages such as preprocessing and optimization. Modern C++20 features like modular programming potentially enhance compilation speed and efficiency. Memory management continues to evolve with a strong focus on smart pointers and automated memory handling, reducing the likelihood of memory leaks that plagued earlier C++ code. This shift makes C++ more robust and reliable for today's applications. Furthermore, advanced tools like custom allocators let developers tailor memory management strategies to specific application needs, enhancing both performance and security in resource management. As C++ adapts to modern software development styles, these developments in compilation and memory management highlight a commitment to enhancing both efficiency and the overall experience for developers.
The compilation process and memory management within the C++ landscape of 2024 have undergone significant refinements. One of the more notable changes is the increased emphasis on breaking down programs into separate compilation units. This modular approach not only makes code easier to maintain but can also significantly reduce compilation times, especially when only a portion of a project needs to be rebuilt. This contrasts with earlier practices where recompiling even minor code changes required reprocessing the entire project.
Another notable development is the widespread adoption of Link Time Optimization (LTO). LTO enables compilers to optimize across different parts of the program, leading to more efficient executable code compared to earlier versions of C++, where optimization was typically confined to individual files. This advancement creates a more holistic approach to optimizing code.
Memory management practices have also been improved with the rise of memory pool allocators. These allocators can decrease memory fragmentation, leading to more efficient use of system resources. C++ 2024's support for custom allocators provides even greater flexibility, allowing developers to fine-tune memory management to optimize resource usage in performance-critical applications. This fine-grained control represents a move towards more sophisticated resource management.
While traditionally requiring manual intervention, C++ 2024 now offers features that have made memory management more “semi-automatic”. The use of smart pointers such as `std::unique_ptr` has gained traction, significantly reducing the risk of memory leaks that were common in older C++ code while still retaining developer control over object lifetimes. This balance is noteworthy, providing a safe path forward without compromising expressiveness.
The way asynchronous operations manage memory has also been altered through the implementation of coroutines. These features allow for memory efficient concurrent applications by temporarily yielding execution without the need for substantial stack management. While this has been a significant change, it might also confuse those who are more accustomed to traditional stack-based programming.
Furthermore, C++'s move semantics, introduced in C++11, has been refined in C++ 2024. This feature minimizes the need for unnecessary copying of data structures, resulting in more efficient resource management. This, combined with improvements in smart pointers, particularly `std::weak_ptr`, has increased the control that programmers have over managing resources and eliminating issues like circular references, leading to improved code quality.
Modern compilation processes often incorporate static analysis tools as part of the build process. These tools can detect potential memory management errors and enforce coding standards at compile time. This early-stage identification of issues leads to more reliable code before reaching runtime, which is significant for larger teams working on complex projects.
The design of C++ encourages the use of zero-cost abstractions. This core design principle means that high-level programming constructs do not come at the expense of runtime efficiency. This is relevant to both memory management and other syntactic aspects, resulting in optimized code that performs just as efficiently as lower-level implementations.
Finally, advancements in `constexpr` and template programming in C++ 2024 have made it possible to resolve certain memory management tasks entirely at compile time. This ability to shift resource allocation to the compilation stage, rather than runtime, can lead to improved runtime performance and efficiency. While this aspect is quite powerful, it can introduce added complexity when it comes to troubleshooting compile-time errors.
These evolutions demonstrate how C++'s compilation process and memory management have progressed over time. It's an interesting path where the language is becoming more user-friendly while still providing enough tools to fine-tune performance for a wide range of tasks.
More Posts from aitutorialmaker.com: