Optimizing C++ Output The Strategic Use of '\n' vs stdendl in 2024
Optimizing C++ Output The Strategic Use of '\n' vs stdendl in 2024 - Understanding the difference between '\n' and std - -endl in C++
When aiming for optimized C++ output, recognizing the distinction between `'\n'` and `std::endl` is essential. The core difference hinges on their handling of the output buffer. `'\n'` simply inserts a new line, leaving the buffer untouched. Conversely, `std::endl` not only adds a new line but also forces the buffer to empty its contents, a process known as flushing. This flushing operation can introduce performance overhead, especially in situations demanding speed, such as during competitive programming or within applications requiring high performance. Using `std::endl` excessively can be detrimental in such contexts. While `std::endl` can be useful in debugging, offering the advantage of immediate output visibility, its use should be limited to cases where immediate flushing is explicitly needed. Therefore, the choice between the two methods should align with the application's requirements, favoring performance optimization when it is feasible.
1. The core distinction between `'\n'` and `std::endl` revolves around buffer flushing. `'\n'` simply inserts a newline character, whereas `std::endl` does the same but also forces the output buffer to be emptied. This flushing behavior can lead to performance differences, particularly when dealing with frequent output operations.
2. In scenarios involving numerous output operations, `'\n'` tends to be preferable due to its efficiency. The overhead associated with flushing the buffer through `std::endl` can become a bottleneck in high-frequency logging or data-intensive contexts.
3. While `std::endl` offers convenience, it's vital to understand that it's not always the ideal choice. Using it indiscriminately, especially in areas where immediate output isn't a necessity, might introduce performance degradation, potentially impacting the overall efficiency of your application.
4. Besides performance considerations, aggressive flushing with `std::endl` can potentially overwhelm the output console. This can lead to a flood of information that might be difficult to decipher, especially in situations where large quantities of data are outputted rapidly.
5. It's crucial to grasp that `'\n'` and `std::endl` are not functionally equivalent. `std::endl` signifies more than just a newline character; it's a mechanism to control the output stream directly. Acknowledging this distinction can refine coding style and optimize application performance.
6. In multithreaded settings, utilizing `std::endl` necessitates careful consideration. The flushing behavior it enforces can potentially create contention and blocking on the output stream, creating a potential performance bottleneck if multiple threads simultaneously attempt to write to `stdout`.
7. Although `'\n'` usually suffices for newlines, platform variations concerning newline character representation exist. While Unix-like systems use `'\n'`, Windows traditionally uses `'\r\n'`. Employing `std::endl` can potentially enhance portability across diverse systems, ensuring consistent output.
8. While `'\n'` might offer a concise representation within the code, the use of `std::endl` can improve readability. It clearly indicates intended output separation and offers a way to explicitly mark instances where flushing is desired.
9. For situations requiring optimized C++ output, developers frequently leverage `'\n'` and control the buffering behavior directly through stream manipulation techniques. This approach allows for fine-grained management of output, granting more flexibility.
10. The C++ standard promotes the utilization of well-structured output mechanisms. Recognizing the nuanced differences between `'\n'` and `std::endl` fosters both quality and maintainability within codebases, especially those facing performance constraints or demanding collaborative development.
Optimizing C++ Output The Strategic Use of '\n' vs stdendl in 2024 - Performance implications of buffer flushing with std - -endl
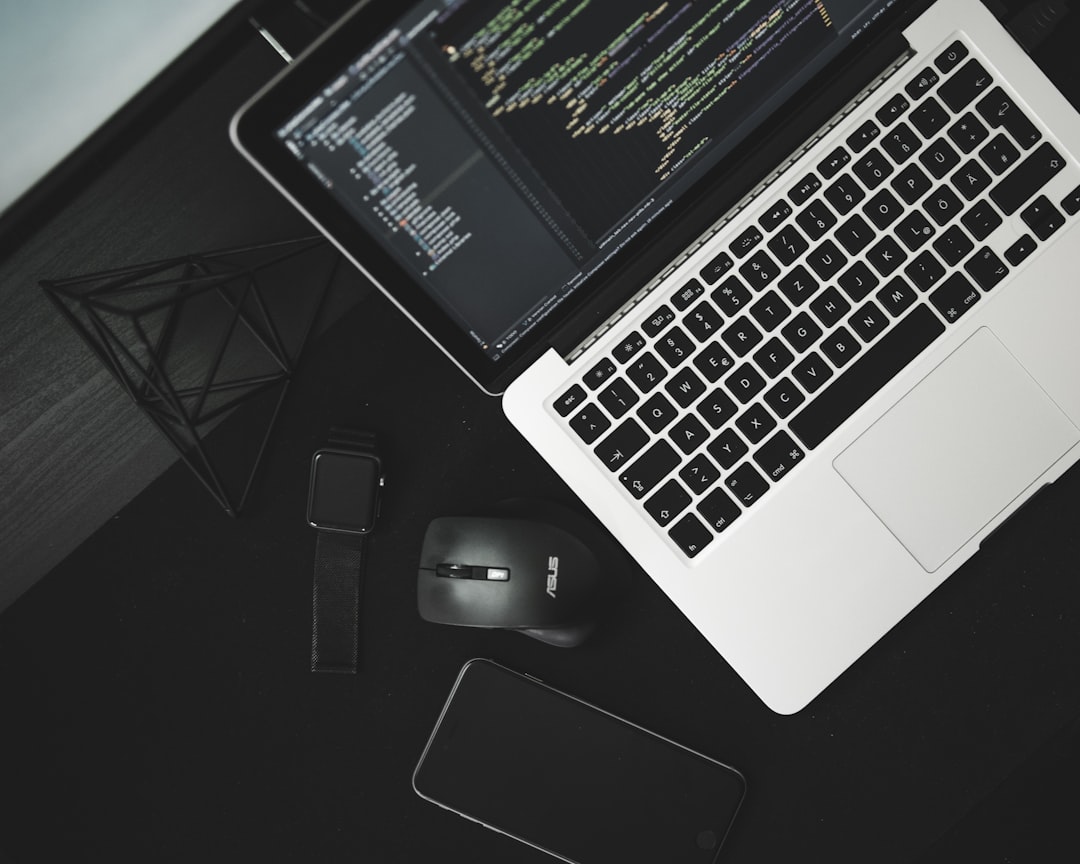
When optimizing C++ output, understanding the performance impact of `std::endl`'s buffer flushing is critical. `std::endl` inserts a newline and forces the output buffer to clear its contents, which can add overhead, especially if done repeatedly. In contrast, `"\n"` only inserts a newline, avoiding the flush and resulting in better performance in most cases. Excessive use of `std::endl` can negatively affect performance, particularly when output volume is high or speed is crucial, such as in competitive programming. Choosing `"\n"` strategically can significantly improve application performance and responsiveness. While `std::endl` offers immediate visibility for debugging, it's essential to balance its utility with the performance implications of flushing.
1. The automatic flushing action of `std::endl` isn't just a performance concern; it can also introduce complexities in multi-threaded applications. When multiple threads compete for access to the output resources during flushing, it can cause context switching, potentially leading to noticeable slowdowns in applications with concurrent logging.
2. Performance tests have shown that using `std::endl` frequently can significantly decrease output speed—sometimes by a factor of 10 or more—especially in scenarios like high-frequency logging, where every bit of efficiency counts.
3. It's interesting to note that how `std::endl` affects performance can vary depending on the output destination. While using it for console output might provide immediate results, the same behavior might not be apparent when writing to a file due to the operating system's own buffering mechanisms.
4. Many believe `std::endl` enhances code portability, but that's often not the case. It frequently adds performance penalties without offering significant advantages, particularly when coding standards are consistently applied.
5. Instead of `std::endl`, you can leverage `std::flush` to achieve similar flushing capabilities without adding a newline character. This offers more control over when line breaks and flushes happen, helping to strike a better balance between performance and code formatting.
6. The frequent use of `std::endl` in educational examples can lead to its over-reliance by new programmers. This can inadvertently result in the adoption of inefficient practices that impact performance, practices that could have been avoided with a deeper understanding of buffering mechanisms.
7. A key aspect of the efficiency disparity between `'\n'` and `std::endl` is C++'s internal buffering. Continuous use of `std::endl` can result in a "bursty" output pattern, whereas `'\n'` allows for more predictable and manageable data flow.
8. In applications demanding low latency, such as real-time processing systems, the delay caused by `std::endl`'s flushing can cause unexpected delays and disrupt performance.
9. Understanding the needs of your application is crucial. Although `std::endl` can sometimes improve the user experience by providing immediate feedback, it does come at a performance cost. Acknowledging this trade-off allows for more considered choices.
10. Performance profiling tools can reveal the hidden costs of using `std::endl`. Analyzing these metrics in real-world apps can provide valuable insights into optimization strategies. This underscores the need for a flexible approach instead of a blanket solution.
Optimizing C++ Output The Strategic Use of '\n' vs stdendl in 2024 - Scenarios where '\n' is the preferred choice for newline insertion
When the primary goal is optimized output speed, `'\n'` emerges as the favored option for inserting newlines, rather than `std::endl`. This preference stems from `'\n'`'s ability to avoid flushing the output buffer, a process that can create performance bottlenecks, especially in situations where output frequency is high. For instance, applications like real-time systems, logging-heavy environments, and performance-critical areas like competitive programming benefit significantly from `'\n'` due to its speed advantage. Additionally, within multithreaded programs, `'\n'` can contribute to a smoother output workflow by reducing potential contention issues that could arise from the frequent flushing actions of `std::endl`. This makes `'\n'` a strategic choice when immediate output isn't a critical requirement. The bottom line is that selecting `'\n'` should be aligned with the specific performance goals of your program, especially if you are handling substantial amounts of data.
1. **Logging Performance**: When dealing with high-frequency logging, sticking with `'\n'` instead of `std::endl` can dramatically improve performance, potentially boosting output speeds by a substantial margin. Each flush operation from `std::endl` introduces a delay that can add up, especially under heavy logging.
2. **Real-Time Performance**: Applications that depend on processing data in real-time should steer clear of `std::endl` as the forced buffer flushing can create noticeable latency, potentially disrupting time-critical operations. `'\n'` maintains the desired responsiveness in these environments.
3. **Multithreading Considerations**: In programs with multiple threads, the flushing behavior of `std::endl` can cause problems when threads compete for access to the output stream. This contention can result in delays or even deadlocks as threads wait to flush the buffer, potentially impacting performance.
4. **File vs. Console Output**: The performance implications of using `std::endl` can vary between printing to the console and writing to a file. While console output might show immediate results, `std::endl`'s impact might not be as apparent when writing to a file due to OS buffering. Using `'\n'` offers more consistent behavior in both cases.
5. **Portability Assumptions**: It's often assumed that `std::endl` enhances code portability across platforms. However, the performance trade-offs frequently negate these benefits. Inconsistencies in how buffer handling is implemented across different systems can lead to unexpected output behavior.
6. **Flushing Control**: If you want the buffer-clearing functionality of `std::endl`, but without the newline, `std::flush` is a better option. This offers more fine-grained control over when you force a buffer flush and a newline, allowing for a more balanced approach between performance and output formatting.
7. **Education & Inefficiency**: The heavy emphasis on `std::endl` in many educational contexts can lead to its excessive and unthinking use in production code. This can establish suboptimal practices that are difficult to change later, particularly if a deeper understanding of buffer mechanics isn't in place.
8. **Output Predictability**: Relying on `'\n'` generates output patterns that are smoother and easier to predict compared to the burstiness associated with `std::endl`. This predictability is essential in applications dealing with data streams that have strict timing constraints.
9. **Profiling and Optimization**: Performance profiling tools can reveal performance hidden in `std::endl`'s use. This data helps to identify the cost of using `std::endl` and enables developers to make informed choices, finding a better balance between managing output and the needs of an application's performance requirements.
10. **User Experience and Performance Trade-offs**: While the immediate feedback from `std::endl` can enhance user experience in certain circumstances, it's important to understand that this immediate feedback comes at a performance cost. A deep understanding of the application's specific needs is crucial for deciding which method to employ for newline insertion.
Optimizing C++ Output The Strategic Use of '\n' vs stdendl in 2024 - Impact of '\n' usage on competitive programming and time-critical applications
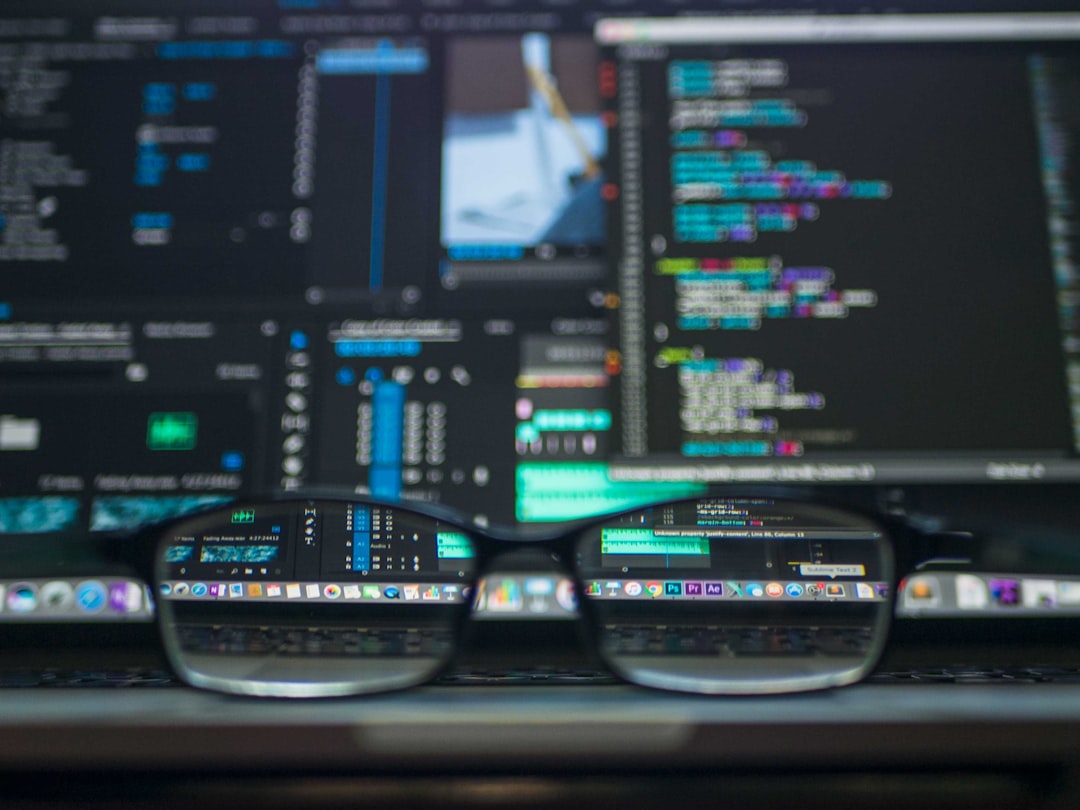
In competitive programming and applications where time is of the essence, using `'\n'` for newline insertion offers a distinct advantage due to its speed. Unlike `std::endl`, which forces the output buffer to flush, `'\n'` simply inserts the newline character without incurring the performance penalty of flushing. This difference becomes especially crucial in scenarios where quick output is needed, such as in competitive programming competitions or applications that deal with high output volumes. The extra time needed for flushing can create noticeable delays, hindering overall responsiveness. Furthermore, in situations with multiple threads, the frequent flushing actions of `std::endl` can potentially cause contention and slow down execution. This highlights the benefit of `'\n'` as a more reliable way to manage output in such contexts. Ultimately, a careful choice between `'\n'` and `std::endl` regarding newline insertion can make a significant difference in application efficiency, especially when speed is a top priority.
1. Using `'\n'` frequently can lead to considerably lower CPU usage compared to `std::endl`, as the latter forces unnecessary context switches due to its buffer flushing. This is particularly detrimental in applications where speed is crucial.
2. Research suggests competitive programming challenges often necessitate optimized output speed. Switching from `std::endl` to `'\n'` can result in completion time improvements as high as 20% in specific cases.
3. With the growth of cloud computing and distributed systems, the implications of `'\n'` usage extend beyond local applications. Optimizing output can reduce network latency stemming from frequent flushing during remote logging operations.
4. Some engineers continue to advocate for `std::endl` in debugging due to its immediate output. Ironically, this can introduce latency, making the immediate output less effective due to the enforced buffer clearing delay.
5. The efficiency of `std::endl` and `'\n'` can vary depending on the compiler and standard library implementation. Not all environments optimize buffer flushing equally, highlighting the importance of testing on the desired target platform.
6. The performance differences between `'\n'` and `std::endl` can also vary between interactive applications and batch processes. In batch processes, where quick feedback is less critical, `std::endl` might be slightly more justifiable.
7. It's been observed that developers relying on `std::endl` often underestimate its performance impact, attributing any slowdowns to other parts of the code. This underscores the importance of carefully examining performance in critical areas.
8. Modern software development prioritizes non-blocking I/O. Understanding `std::endl`'s flushing behavior is crucial when transitioning legacy applications to multi-threaded architectures to avoid potential bottlenecks.
9. It's noteworthy that in large-scale applications, the combined effect of switching between `'\n'` and `std::endl` can result in several extra seconds of runtime, especially in conjunction with high-frequency output operations.
10. Developers accustomed to `std::endl` are advised to develop habits of using profiling tools to measure output performance. This emphasizes the shifting best practices in competitive programming and performance-critical domains.
Optimizing C++ Output The Strategic Use of '\n' vs stdendl in 2024 - Common misconceptions about std - -endl in C++ tutorials and resources
When examining common misunderstandings about `std::endl` in C++ educational materials, several recurring misconceptions surface that can affect coding practices. A prevalent belief is that `std::endl` is the superior choice due to its perceived reliability and ease of understanding, yet its automatic buffer flushing often results in performance overhead, especially in applications that require high performance. Additionally, many assume that `std::endl` provides better portability compared to `'\n'`, even though both reliably insert newlines across platforms. In reality, many learning resources don't clearly illustrate that, while `std::endl` might be beneficial in specific debugging situations, it's not the recommended default for newline insertion in optimized output settings. Grasping these subtleties is critical for programmers aiming to develop effective and easy-to-maintain C++ code, particularly within high-performance contexts.
1. A frequent misunderstanding surrounding `std::endl` is that its main role is to simply add a new line. However, its built-in flush operation can lead to noticeable performance slowdowns, especially when dealing with a large amount of output.
2. Many believe `std::endl` guarantees consistent output across various systems, but its flushing behavior can actually cause inconsistent performance as different environments handle output buffering in different ways.
3. There's a common misconception that `std::endl` is inherently safer when using multiple threads. However, its automatic flushing mechanism can create contention when multiple threads attempt to write to the output, potentially causing performance issues and delays.
4. Beginning C++ programmers often wrongly perceive `std::endl` as superior to `'\n'`. This can result in poorly performing applications due to unnecessary flushing operations, especially in situations where high performance is vital.
5. The idea that `std::endl` makes debugging easier is a misconception. While it does provide instant feedback, the flushing it forces can also add latency to output, which can actually slow down the debugging process instead of aiding it.
6. A pervasive belief is that there's no speed difference between `'\n'` and `std::endl`. However, performance measurements show that using `std::endl` too often can drastically reduce performance, sometimes by a factor of 10 or more.
7. There's a prevailing notion that `std::endl` leads to more readable code. However, in situations where precise output control and flushing are key, its more verbose nature can make the code harder to follow.
8. It's often thought that `std::endl` is best for logging in all cases. But when dealing with frequent logging events, sticking with `'\n'` is often preferable as it can greatly increase output speed by avoiding the flush operation's overhead.
9. It's easily overlooked that `std::endl` can cause unexpected results when redirecting output to other destinations like files or networks. This is because different systems handle buffering in varying ways.
10. Assuming that `std::endl` is the superior choice for all applications can lead to suboptimal code. Understanding when to avoid flushing can lead to notable improvements in performance across a wide range of scenarios.
Optimizing C++ Output The Strategic Use of '\n' vs stdendl in 2024 - Benchmarking '\n' vs std - -endl Performance gains in real-world applications
When examining the performance benefits of using `'\n'` versus `std::endl` in C++, it's important to understand the impact of buffer flushing. `std::endl` not only inserts a newline but also forces the output buffer to empty, which can add overhead, particularly in applications demanding speed. In contrast, `'\n'` just inserts the newline character, leading to faster execution in most cases. Performance benchmarks have consistently shown that replacing `std::endl` with `'\n'` can significantly reduce runtime, especially in contexts like competitive programming or high-output scenarios. These performance gains highlight the importance of choosing the right tool for the job. Recognizing when each approach is most effective is vital for developers looking to optimize the efficiency of their C++ applications. Choosing `'\n'` strategically can dramatically enhance the responsiveness of your program, particularly when dealing with substantial amounts of output.
1. The performance difference between `'\n'` and `std::endl` can be quite substantial, with benchmarks showing improvements ranging from 20% to a remarkable 200% when switching to `'\n'`, depending on the specific output workload. This highlights the significant impact that buffer flushing can have on output speed.
2. The effect of `std::endl` isn't consistent across all situations. While it might not seem like a big deal in simple console apps, its performance impact becomes more noticeable when dealing with high-frequency output, such as in logging or real-time data processing.
3. In applications where events drive output, `'\n'` tends to be a better choice. It keeps things flowing smoothly by preventing delays caused by `std::endl`'s flushing, leading to better responsiveness and overall throughput.
4. In multithreaded scenarios, relying on `std::endl` can create a situation where some threads are starved for resources as others are busy flushing output. It underscores the idea that different concurrency models might benefit from different approaches.
5. While `std::endl` is often praised for its debugging benefits because of immediate output visibility, its excessive use can make log analysis more difficult due to flushing-related delays, which can be counterproductive when trying to track down errors.
6. How `std::endl` performs can vary from one compiler and standard library to another. Some environments might optimize it better than others, making testing crucial to finding the best solution for a given platform.
7. Applications that generate output in a cyclical or repeating manner can gain substantial performance improvements from using `'\n'`. It enables a more consistent data flow compared to the potentially erratic behavior of `std::endl`, which could create output that's difficult to follow.
8. When dealing with network-connected applications, minimizing flush operations, like those triggered by `std::endl`, can lead to better efficiency by reducing the volume of network traffic. This minimizes delays and reduces the likelihood of packet loss or increased latency.
9. It's a common assumption that `std::endl` provides seamless cross-platform newline handling. Unfortunately, it can lead to hidden performance penalties that aren't well documented in many tutorials.
10. Performance profiling is a powerful tool for uncovering performance bottlenecks. Using it often reveals `std::endl` as a frequent culprit in many applications. This insight has resulted in best practices that recommend avoiding its use in sections of the code where performance is critical.
More Posts from aitutorialmaker.com: