Create AI-powered tutorials effortlessly: Learn, teach, and share knowledge with our intuitive platform. (Get started for free)
Understanding Variable Scope and Memory Management in C++ A Deep Dive into Static Variables
Understanding Variable Scope and Memory Management in C++ A Deep Dive into Static Variables - Memory Layout in C++ Static Variables vs Stack and Heap
Within the C++ environment, how variables are stored and managed across different memory segments is fundamental for effective memory management. Static variables are stored in a designated memory region and persist for the entire lifespan of your program. This characteristic makes them valuable when you need to maintain data across function calls. The stack, however, is geared towards temporary data, allocating and releasing memory automatically as functions execute and complete. This approach prioritizes speed. Meanwhile, the heap provides the ability to allocate larger or variable-sized data structures, but demands careful management by the developer to prevent issues like memory leaks. Each memory segment caters to specific needs, ultimately shaping how variables are both defined and accessed within the program's operational flow. Understanding this interplay between the different memory locations is essential to write efficient and stable C++ code.
Let's delve into how static variables interact with the different memory areas in C++. Static variables, unlike their automatic counterparts, enjoy a longer lifespan—they exist for the entire duration of the program's execution. This is because they reside in a dedicated memory region, typically alongside other global or static variables. In contrast, variables defined within a function's scope (local variables) are allocated on the stack. This stack is a region of memory managed using a LIFO (Last-In, First-Out) approach—variables are created as the function starts and are automatically removed as the function exits.
Then we have the heap. The heap is another memory area, but it operates differently. Memory on the heap needs to be explicitly allocated and deallocated by us—we use `new` and `delete` for this. The heap is often used for things like dynamically sized data structures where the exact size isn't known at compile time. One downside is that forgetting to deallocate memory can result in the dreaded memory leak.
The way functions are called impacts the stack. When a function is called, its local variables are stored on the stack. Any pointers associated with heap-allocated memory are also stored there, serving as a guide to the data actually located in the heap.
Stack allocation tends to be faster due to its contiguous nature and how it interacts with the operating system. Getting memory on the stack often simply involves adjusting a pointer, not requesting a new chunk from the operating system, which can be comparatively slower. On the other hand, the heap's flexibility comes at a price. It can accommodate much larger memory allocations than the stack, but is also susceptible to issues like fragmentation due to its more dynamic nature.
The memory layout of a program often includes the code segment (text segment, which usually can't be altered), data segments (for global variables with initial values and those without), the stack, and the heap. The stack is great for short-lived data, while the heap supports more flexible lifetime management, allowing us to keep data around longer than just for a single function call.
There are interesting tradeoffs. While the stack is often the better choice for simpler tasks, the heap can become a source of headaches. Problems related to managing static variables throughout a program's entire life can be particularly tricky to pin down and diagnose. Static variables, due to their longevity, are especially problematic in multithreaded environments, as their access can be prone to race conditions if not properly managed.
Understanding Variable Scope and Memory Management in C++ A Deep Dive into Static Variables - Static Variable Initialization and Default Values
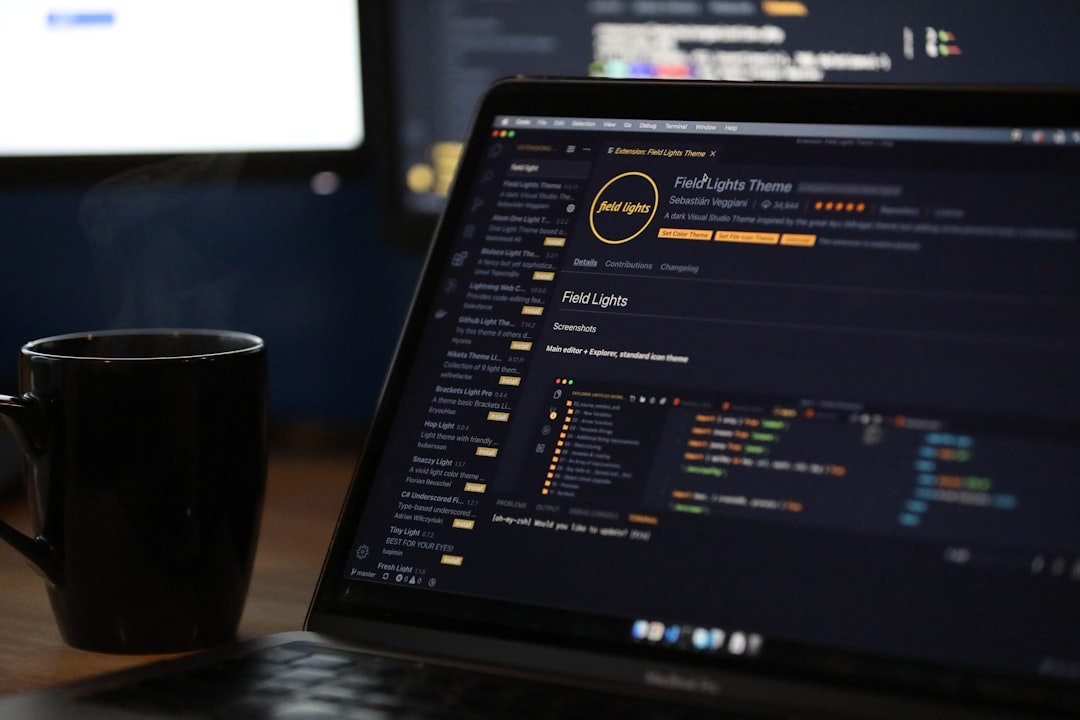
Static variables in C++ are designed to persist throughout a program's execution, making them valuable for storing data that needs to be retained across multiple function calls. When a static variable is not explicitly given an initial value, it automatically receives a default value: integers are set to 0, floating-point numbers to 0.0, and characters to the null character ('\0'). This automatic initialization, although helpful, also highlights the importance of understanding how static variables behave, especially when it comes to data encapsulation within a specific scope while preserving the variable's value. It's important to recognize that mismanaging static variables can lead to unexpected outcomes, including unwanted data persistence and complications like race conditions in multithreaded code. Therefore, understanding how static variables are initialized and their default behavior is crucial for creating C++ applications that are both efficient and reliable.
Static variables in C++ are initialized only once, at the program's start, unlike regular variables that are initialized each time a function is called. This one-time initialization helps maintain consistent data across multiple function calls without the unnecessary overhead of repeated setup. Unlike their automatic counterparts, which disappear when they go out of scope, static variables retain their values even after a function has completed. This behavior makes them quite handy when you need to preserve information between different calls.
When it comes to default values, static variables follow a pattern based on their type. Basic types like integers and floats are automatically set to zero, while user-defined objects use their default constructors. So a statically-declared class will always be constructed, whereas an `int` might be just given the value of 0. Static variables are typically tucked away within the "data segment" of the memory map for a program. This location makes identifying and tracing memory-related issues easier.
However, these variables can present challenges, especially in programs with multiple threads. Having multiple threads attempt to modify or access a shared static variable at the same time can lead to all sorts of problems, including unexpected behavior or corruption of the data itself. Compared to global variables that can be reached from anywhere, a static variable's scope is limited to the file where it's defined. This helps reduce conflicts and promotes a tidier design.
One interesting aspect is that static variables get initialized before `main()` is even executed, so they're ready when the program kicks off. This helps with dependencies on static variables that are crucial for the program's operation. While convenient, static variables can lead to more memory being used as your program runs. The memory stays allocated until the entire program terminates, which, while helpful in some cases, might negatively affect memory efficiency in larger programs.
As the program exits, the termination process for static variables can be complex, particularly if they're managing dynamically allocated memory. It's important to design a proper cleanup mechanism for those variables in order to avoid potential memory leaks. Overall, static variables can sometimes be misused or lead to misunderstanding. It's beneficial for developers, especially newer ones, to fully comprehend how static variables impact program state and memory management to prevent unexpected or tricky bugs.
Understanding Variable Scope and Memory Management in C++ A Deep Dive into Static Variables - Static Variable Lifetime From Program Start to Termination
In C++, static variables enjoy a lifespan that coincides with the program itself, starting when the program begins and ending only when it terminates. This differs from the behavior of automatic (local) variables, which are allocated on the stack and exist only within the scope of the function they are declared in. Static variables, in contrast, are allocated in a specific memory area and remain there throughout the program's entire run. This feature enables them to maintain their values across multiple function calls, which can be advantageous in situations where preserving state is crucial. However, their persistent nature can present challenges, specifically within multithreaded contexts, where concurrent access can create issues like race conditions and cause unpredictable program behavior. Therefore, developers must carefully consider the use of static variables, understanding their implications for memory management and potential complexities, to ensure the creation of robust and efficient C++ applications.
Static variables in C++, from the moment the program starts until it ends, hold a unique place in memory management. Their initialization occurs even before the `main` function begins, setting them up as potential building blocks for the rest of the code. This early preparation can be vital if other parts of the program rely on these initial settings. Unlike local variables that vanish once their enclosing function finishes, static variables persist until the program terminates, safeguarding whatever data they contain. However, this permanence can lead to trouble, especially when dealing with sensitive information that shouldn't outlive the program's execution.
One area where this longevity becomes particularly problematic is in multithreaded programs. Since static variables are shared among all threads, it's crucial to manage access to them carefully. Otherwise, you risk race conditions—situations where multiple threads try to alter the same variable at the same time, resulting in unexpected outcomes or corruption. While the persistence of static variables can boost efficiency by avoiding repetitive initializations, it's a double-edged sword. The cost of this convenience is a potentially larger memory footprint since these variables' allocated space doesn't get released until the program ends. This can impact performance, especially in large applications where unnecessary data retention can weigh heavily.
Thankfully, static variables have a restricted scope that is usually limited to the file where they are declared. This helps maintain cleaner code by reducing potential conflicts with similarly-named variables in other files. It's a nice way to compartmentalize your code and keep things tidy. Additionally, static variables receive default values based on their type; integers are initialized to 0, floats to 0.0, etc. However, this feature can easily obscure potential bugs caused by inadvertently retaining old data in variables that you thought were fresh.
Program termination involves a complex dance for static variables. Their destruction happens in reverse order of their initialization, which can make the cleanup process tricky, especially if they manage dynamic memory (like with `new` and `delete`). Proper cleanup strategies are vital to prevent memory leaks. Static variables typically live in a specific area of the memory map called the data segment. This centralized location makes identifying and tracking down memory allocation-related problems easier. However, if this area is not managed well, it can become a source of errors or difficulty tracking problems. While static variables can improve performance by reusing the same memory and stored values, the programmer should be mindful of potential overuse of these variables to avoid unintended consequences.
Furthermore, developers need to be cautious of unintended consequences. The nature of static variables, which retain data across function calls, can lead to surprising results if not handled properly. A function that relies on a static variable might produce different outputs depending on prior calls, even if the inputs haven't changed. This behavior can create bugs that are harder to track than those that only appear once due to being completely contained within the function. Static variables can be very useful but they can also cause some headaches. It's always important for developers to have a clear understanding of how static variables interact with program flow and memory management in order to create robust and well-behaved applications.
Understanding Variable Scope and Memory Management in C++ A Deep Dive into Static Variables - Local Static Variables Inside Functions and State Preservation
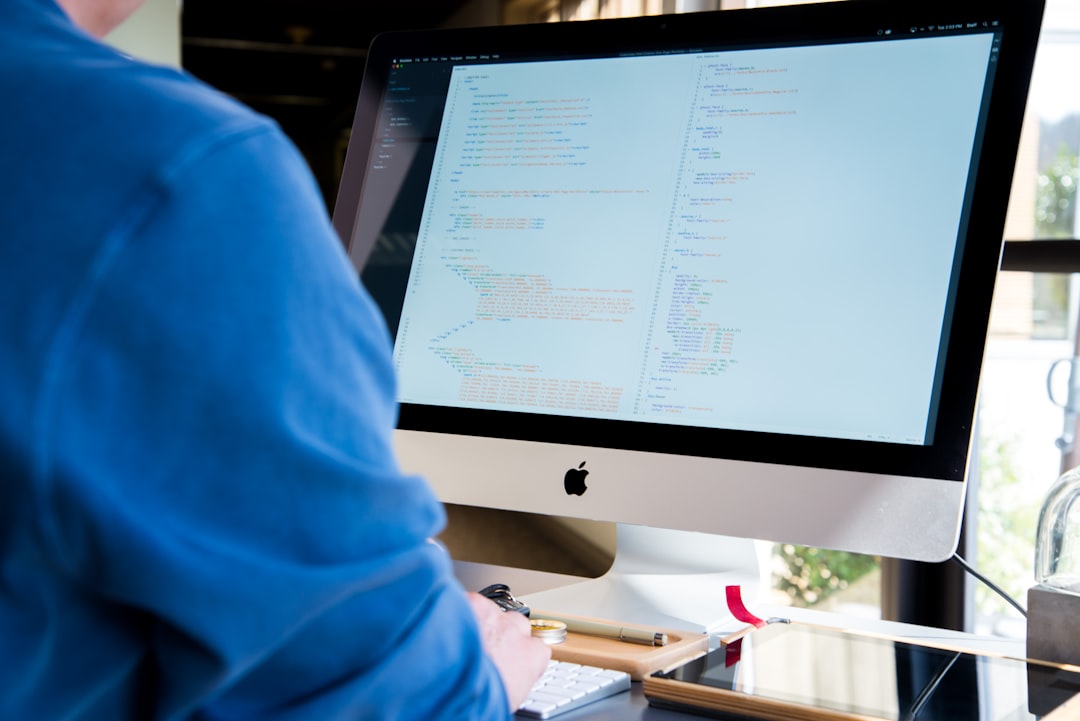
Within the confines of a C++ function, local static variables provide a mechanism for maintaining state across multiple calls. Unlike ordinary local variables that are recreated and reset each time a function is called, local static variables are initialized only once, at the very beginning of the program's execution. This means their values are preserved from one call to the next, potentially simplifying your code by avoiding redundant initializations. However, this persistence can introduce challenges, particularly within multi-threaded programs. When multiple threads attempt to access and modify the same static variable concurrently, race conditions can emerge, leading to unpredictable results. Moreover, improperly managing static variables can result in unexpected behaviors due to the retention of possibly outdated data, creating difficult-to-diagnose bugs. Therefore, a firm grasp of how these variables function is crucial for both maintaining control over the program's state and managing memory effectively within C++ applications.
Static variables within functions in C++ offer a unique approach to state preservation that differs from the typical behavior of automatically allocated variables. They maintain their values throughout the entire program's lifespan, unlike local variables which are reinitialized each time a function is called. This longevity is convenient when needing to track information across function calls, but it also makes memory management more intricate. When a static variable isn't explicitly initialized, it automatically receives a default value. Integers get zero, floating-point numbers get 0.0, and so on. While helpful, this default initialization can sometimes obscure accidental retention of old data, possibly leading to subtle bugs.
The program's start and end are critical times for static variables. Their initialization occurs before the main function even begins, making them available for use from the very outset. This early initialization allows us to rely on them when necessary. In contrast, during program termination, their destruction occurs in reverse order of initialization, potentially increasing the complexity of ensuring a proper clean-up. Failing to release dynamically allocated memory correctly through proper `new` and `delete` handling can lead to memory leaks, which can be surprisingly hard to fix.
One of the major challenges with static variables becomes apparent when dealing with multiple threads of execution. Because static variables reside in a shared space, multiple threads attempting to modify the same variable at the same time can introduce nasty race conditions. Such conditions are notorious for causing erratic program behavior due to the unpredictability of the timing of access to the variable. Furthermore, the enduring nature of static variables impacts the program's overall memory usage. They stay allocated until program termination, which while convenient can potentially increase the memory footprint, especially in larger projects where the extra memory might lead to performance slowdowns.
It's also important to understand the difference in scope between static and global variables. While both retain their values throughout the program's execution, the scope of static variables is usually restricted to the file they're declared in. This reduced scope helps limit the chances of encountering the same variable name in different files and provides better code modularity. However, this limitation can sometimes lead to a misconception that these variables are isolated when, in fact, their states linger throughout the program. This longevity can cause debugging challenges. If a function's output is unexpected, a static variable's prior modifications might be the culprit, making the issue more difficult to pinpoint compared to a purely local variable. Additionally, static variables are useful when interacting with external hardware or systems across multiple function calls. They can maintain state information that's crucial for controlling an external system. Yet, this very functionality can lead to issues if the state becomes stale or incorrect, which can affect subsequent function calls, data integrity, and the program's overall behavior.
It's essential for developers, especially as their skills progress, to understand the subtle differences between static and global variables. While both types are intended to persist for the entire life of the program, global variables are accessible from anywhere, potentially leading to problems across the codebase. Conversely, static variables are only accessible within the file where they're declared, promoting a more structured and compartmentalized approach. Static variables can be powerful tools, but when misused or not thoroughly understood, they can contribute to issues that can be harder to track and understand.
Understanding Variable Scope and Memory Management in C++ A Deep Dive into Static Variables - Global Static Variables and File Scope Boundaries
Global static variables in C++ operate within the boundaries of a single file, meaning they are only visible and accessible within the compilation unit where they're defined. This file-scope restriction helps prevent naming conflicts and promotes cleaner code organization compared to regular global variables which are accessible across the entire program. Essentially, they act as a form of encapsulation, limiting the scope of influence to the file they're defined in, reducing the likelihood of unintended interactions between different parts of your project.
Even though these variables are confined to a specific file, their memory is shared across all instances within that file. This means their values are preserved across function calls and remain in existence for the duration of the program. However, it's crucial to exercise careful consideration when using global static variables, particularly within multithreaded programs. The shared nature of their memory allocation can lead to difficulties when multiple threads attempt to modify the same variable simultaneously, creating potential race conditions that result in unpredictable behavior and challenging debugging experiences. It's a trade-off – a way to contain access to a particular variable but potentially introducing race conditions in multithreaded settings.
Global static variables, though existing throughout the program's run, operate within a narrower scope than traditional global variables. Their reach is confined to the specific source file where they are declared, preventing accidental access or modification from elsewhere. This characteristic can enhance modularity and reduce unintended interactions between distinct parts of the code.
One noteworthy aspect of static variables is their initialization sequence. Unlike other variables, they come into existence and are set to their initial values before the program's `main` function begins. This early initialization can be crucial if other parts of the program rely on pre-set values before any user-level code is run. Moreover, their values persist between function calls, unlike local variables that are recreated each time a function is executed. While this persistence is useful for maintaining states or accumulating data, it introduces potential complexities in multi-threaded scenarios.
Multithreading and static variables can be a tricky combination. If multiple threads attempt to access or update the same static variable simultaneously, it can result in race conditions, leading to unexpected and unpredictable program behavior. This potential for data corruption underlines the importance of careful synchronization mechanisms when using static variables in a concurrent environment.
When a static variable isn't specifically given a starting value by the programmer, it will be given a default one—0 for integers, 0.0 for floating-point types, etc. This default behavior is helpful, but it can sometimes obscure issues where old, unexpected values are unintentionally retained in the variable. It is important to track these variables closely, especially in complex systems or long-running processes.
Unlike local variables on the stack, static variables contribute to a persistent memory footprint, being allocated at the start of the program and only freed upon termination. This can be helpful, but it also carries a potential penalty, particularly for resource-intensive applications or in environments with strict memory constraints. When your program gets bigger, the overhead of maintaining these static variables can impact overall memory use, leading to difficulties with memory management and possibly affecting the performance of the application.
When the program eventually finishes, the order of static variable destruction mirrors the reverse order of their initialization. This can lead to complications, especially if static variables manage dynamically allocated resources (e.g., memory allocated using `new`). If not carefully managed, this can be a source of insidious errors.
The confined scope of static variables, limited to the source file they are defined in, can improve the overall structure of the code. Yet, it can also lead to misunderstandings regarding their true behavior. Developers might think that these variables are completely insulated, but they still retain values from function call to function call within the file they are contained in.
Maintaining state between function calls for external devices or systems can be a prime use case for static variables. However, this functionality also presents a challenge if the state becomes stale or incorrect, possibly affecting subsequent interactions with the external system. If the values are not aligned with the current context of the external system, or if the state is in an invalid condition, it can result in errors or malfunctions.
Static variables can introduce subtle and challenging-to-detect bugs into programs. Since their values can change across multiple calls, pinpointing the root cause of inconsistent function outputs can be tricky. While helpful, it is crucial to realize that, especially in larger programs, a static variable's prior modifications might be the cause of unexpected program behavior.
Static variables are typically stored in a dedicated section of the program's memory layout, known as the data segment. This centralized storage area makes debugging memory-related issues potentially easier, but problems associated with static variable management can still be tricky to diagnose and solve.
In summary, static variables offer a mechanism for maintaining state and memory across function calls, but their extended scope and persistence demand careful consideration to prevent unintended consequences and ensure program reliability. Understanding the subtleties of their behavior is crucial for designing efficient and robust C++ applications.
Understanding Variable Scope and Memory Management in C++ A Deep Dive into Static Variables - Thread Safety Considerations with Static Variables in Modern C++
Static variables in modern C++ offer a convenient way to retain data across function calls, but this convenience comes with a potential cost: thread safety. Because static variables are accessible to all threads within a program, they can become a source of problems if not handled carefully in a multithreaded environment. When multiple threads try to change a static variable at the same time, data corruption and unexpected behavior can result due to a phenomenon known as a race condition. To mitigate these issues, developers need to use explicit synchronization techniques, like mutexes, whenever they modify static variables within threads.
Beyond thread safety, managing static variables effectively involves considering their initialization and destruction order, which can be intricate, especially in complex programs. Additionally, static variables maintain their memory allocation throughout the program's entire runtime, a feature that can be helpful but also presents challenges in memory management. It's crucial to remember that static variables, while offering efficiency in certain situations, can become a source of trouble if not managed with awareness of potential threading issues. It's a fine balance–static variables can make life easier, but they require mindful development to prevent issues with how the program runs.
Static variables in C++ are initialized before the `main` function even begins, making them readily available when the program starts. This early initialization can be quite useful for variables that need to be set up before any other part of the program executes. However, this shared access in multithreaded environments is problematic. They are accessible to all threads, so developers need to be extra cautious to prevent race conditions – situations where multiple threads attempt to modify the same variable simultaneously. This can result in unpredictable results and data corruption.
If you don't initialize a static variable explicitly, C++ will give it a default value. For integers, it's 0; floats get 0.0; and so on. While convenient, this default initialization can hide issues where old values might linger in a variable longer than intended, potentially leading to bugs that are hard to find.
Static variables have a long life - they exist for the entire time a program is running. This can be advantageous for maintaining state across different parts of the program. On the other hand, this persistence can increase memory usage, especially if you're using a lot of static variables. If your program is using a lot of memory, you might find that it takes longer to run.
When a program ends, static variables are destroyed in the reverse order they were created. This process can get complicated, particularly if static variables are managing memory allocated with `new`. Developers need to be careful here because failing to clean up allocated memory correctly can cause memory leaks.
Global static variables are only visible within the file where they are defined, a good way to avoid conflicts with variable names from other files. While this helps with modularity, developers still need to consider that these variables maintain their state throughout the execution of that file. Their visibility is confined to a single file, but their lifespan spans the whole program.
Local static variables inside a function maintain their state between function calls. This is a useful mechanism for preserving information across function executions. However, the behavior of a function that uses a static variable can become unpredictable, as the output might change based on previous calls. These kinds of situations can be tricky to debug.
Static variables differ from global variables in scope and visibility. Global variables can be accessed from anywhere in the program, which can increase the complexity of the code and introduce errors. Static variables, on the other hand, are only visible within the file where they are defined, resulting in less complex, cleaner code. However, their persistent nature, within the confines of the file, can still lead to confusion regarding the overall state of the program.
Static variables can lead to hard-to-track issues. For example, a function relying on a static variable might behave differently depending on previous executions, even if the inputs are the same. It's important to understand that these seemingly innocent variables can, when misused, result in unexpected and complicated issues.
Static variables are stored in a particular region of memory known as the data segment. This location can make debugging memory problems slightly easier. However, issues related to static variables can still be complex to resolve.
Static variables can be useful but, if misused, can lead to problems. Their scope, lifespan, and shared nature within a file or across threads require close attention to avoid surprises and unexpected behavior. As your understanding of C++ deepens, a nuanced understanding of the complexities of static variables becomes essential for creating reliable and efficient code.
Create AI-powered tutorials effortlessly: Learn, teach, and share knowledge with our intuitive platform. (Get started for free)
More Posts from aitutorialmaker.com: