Create AI-powered tutorials effortlessly: Learn, teach, and share knowledge with our intuitive platform. (Get started for free)
Streamlining Remote File Access Implementing Paramiko for SSH Connections in Python
Streamlining Remote File Access Implementing Paramiko for SSH Connections in Python - Setting up Paramiko for SSH connections in Python
Paramiko is a Python library that offers a robust way to connect to remote servers using the Secure Shell (SSH) protocol. Setting up Paramiko involves initializing an SSH client object, which acts as a bridge to the remote server. It's essential to configure host key checking, as Paramiko doesn't inherently trust any servers. You can configure Paramiko to use private keys for authentication, offering a more secure and often more convenient way to connect compared to passwords. Paramiko gracefully handles potential issues, including authentication errors and connection disruptions. This functionality allows you to execute commands remotely, providing an efficient way to automate tasks on those remote systems.
Connecting to remote systems securely using SSH in Python is a common requirement, and Paramiko is a powerful library that simplifies this process. Paramiko, being entirely Python-based, offers a straightforward installation and usage across different platforms without relying on external tools.
This library is flexible, allowing for authentication through both passwords and public keys. It prioritizes security with its implementation of encryption algorithms like AES and Diffie-Hellman key exchange, ensuring robust communication. One of Paramiko's key features is the support for SSH agent forwarding, which allows seamless access to multiple servers without repeated credential input.
Paramiko also offers a straightforward way to manage files on remote systems via its SFTP client. This client provides methods for downloading, uploading, and manipulating remote files. While generally robust, Paramiko might have performance limitations with larger files during SFTP transfers because it doesn't natively utilize parallel transfers.
Furthermore, the library's inherent logging capabilities are very useful for debugging. These detailed logs can quickly reveal and help resolve connection issues. While this library is a strong tool, it's important to acknowledge its lack of native support for asynchronous operations, which could necessitate careful management for applications that require multiple concurrent connections.
Lastly, it is worth noting that some advanced SSH features, like X11 forwarding or port forwarding, might require extra workarounds or external libraries to achieve the desired functionality. In conclusion, Paramiko, despite its limitations, is a useful and popular library for secure SSH connections in Python, and with proper understanding of its limitations, it can be a valuable tool for tasks ranging from simple remote commands to complex automation projects.
Streamlining Remote File Access Implementing Paramiko for SSH Connections in Python - Implementing secure host key verification
When using Paramiko to connect to remote servers via SSH, it's essential to implement secure host key verification. This verification process acts as a safeguard against potential man-in-the-middle attacks. Paramiko, by default, doesn't trust any server, which is why enabling host key checking is crucial for secure connections. This verification process involves comparing the server's public key against a list of known hosts, typically stored on your system. This comparison helps ensure you're connecting to the intended server and not a malicious imposter. While it might seem like an extra step, implementing host key verification significantly strengthens your security posture, ultimately fostering trust in your remote file access operations.
Paramiko's approach to host key verification needs careful consideration, as it's crucial for securing remote connections. By default, Paramiko checks host keys, but its permissive behavior can lead to vulnerabilities. This default acceptance of unknown hosts without prompt needs to be changed to a more secure, "strict" mode where only known hosts are accepted. Failure to implement robust host key verification can leave your application open to various security risks, making it susceptible to impersonation attempts. It's essential to maintain a central list of known host keys, ensuring that each connection is validated against a trusted source. But, even with this, host keys can change, particularly on systems that undergo reinstallations or configuration modifications. This makes a clear policy for updating the known hosts list critical.
It's tempting to rely on automated host key verification, but it can breed complacency. Regular audits of host keys are needed to ensure their integrity and to catch any unauthorized changes. Logging host key events is also important as it provides an audit trail that can help identify potentially malicious activity or unexpected key changes. And, as a precaution, consider fallback strategies for situations where host keys cannot be verified. This might involve alerting users or requiring manual confirmation before allowing connections. It's important to educate users on the significance of unknown host keys and the potential dangers associated with their acceptance. Training users to recognize and verify these keys can help prevent unintentional security breaches. While Paramiko is a powerful tool, you can enhance security by leveraging third-party libraries specializing in host key verification. These libraries typically offer advanced capabilities for managing and validating host keys more effectively.
Streamlining Remote File Access Implementing Paramiko for SSH Connections in Python - Establishing remote connections with authentication
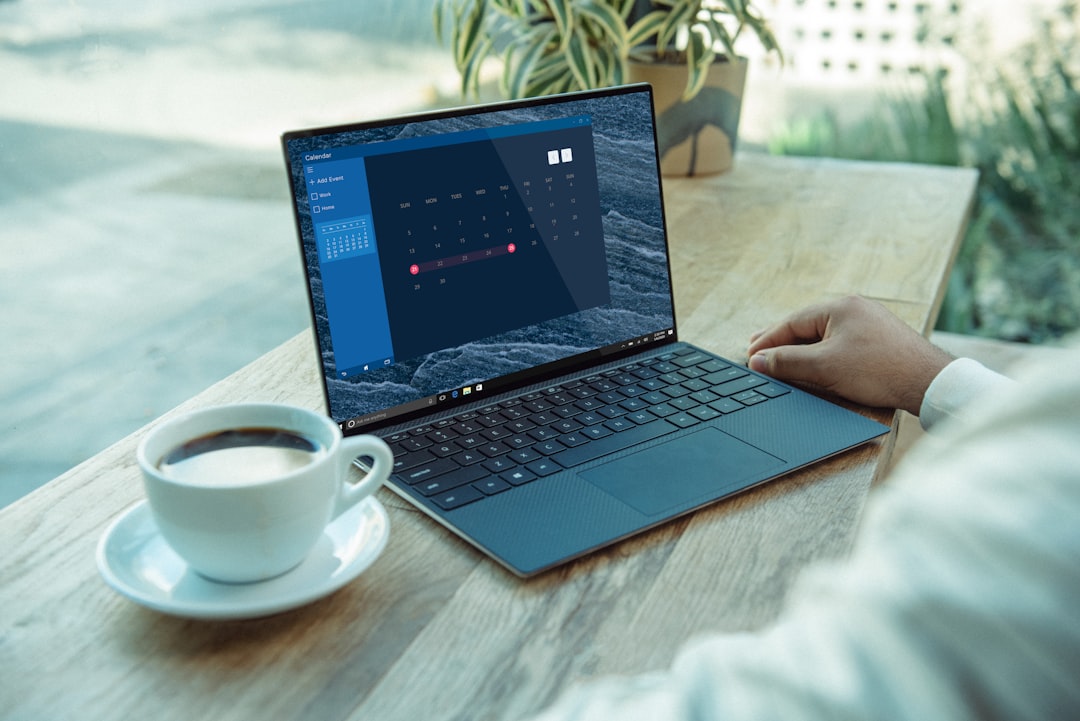
Establishing remote connections with authentication is a crucial step when accessing remote servers securely using SSH. Paramiko, a Python library, excels in facilitating these connections with a strong emphasis on security. Paramiko enables users to authenticate using various methods, notably prioritizing the use of private keys over passwords. This approach enhances security and streamlines the authentication process. Furthermore, properly configuring host key verification is essential. This step helps protect against man-in-the-middle attacks, making it crucial to maintain a trusted list of known hosts. Ultimately, understanding these authentication practices is crucial for safeguarding your remote file access and ensuring secure operations on remote systems.
SSH connections, underpinned by the Paramiko library in Python, are built upon a foundation of robust security measures. While Paramiko streamlines the process of interacting with remote servers, it's critical to understand the underlying security mechanisms to ensure a truly secure environment.
Paramiko's implementation of SSH leverages encryption protocols, most commonly employing AES-256 for data confidentiality. This high-strength encryption protects data during transmission, shielding it from interception or tampering. However, it's worth remembering that even with the strongest encryption, misconfigurations or poorly chosen algorithms can compromise security.
Public key cryptography plays a key role in authentication within SSH. This method allows users to connect without exposing their passwords over the network, significantly reducing vulnerability to password-based attacks. This approach relies on the generation of a public and private key pair, where the public key is distributed to servers while the private key remains secure on the user's system.
But this, too, can be problematic. If the private key is compromised, it completely undermines security. Moreover, SSH agent forwarding, a convenient feature enabling access to multiple servers without repeated authentication, introduces risks if the agent falls into unauthorized hands.
SSH, through Paramiko, offers a variety of authentication methods, including challenge-response mechanisms. These mechanisms add a layer of security by demanding more than a simple password, potentially thwarting brute-force attacks.
Further emphasizing security, SSH relies on host key fingerprinting to verify server identities. This fingerprint, a unique signature, allows users to compare against trusted sources, preventing hijacking attempts.
Despite these safeguards, it's crucial to recognize that SSH security can be compromised by improper configuration, such as using weak algorithms or poorly managed host keys. This is why regular audits and adherence to best practices are essential.
Understanding the SSH connection lifecycle, from key exchange to authentication and command execution, is important for building secure and robust applications. This understanding allows for the development of sophisticated error handling routines, further enhancing security.
Paramiko's implementation of SSH includes logging capabilities. These logs provide valuable data for security analysis, identifying potential breaches or compromised credentials.
Finally, incorporating time-stamped authentication tokens can introduce a dynamic security element, further limiting the potential impact of compromised credentials. The use of these tokens reduces the window of vulnerability, as expired tokens can no longer be used to authenticate.
While SSH connections through Paramiko provide a robust framework for secure communication with remote systems, constant vigilance and adherence to best practices are necessary to mitigate potential risks and maintain a secure environment.
Streamlining Remote File Access Implementing Paramiko for SSH Connections in Python - Utilizing SFTPClient for file operations
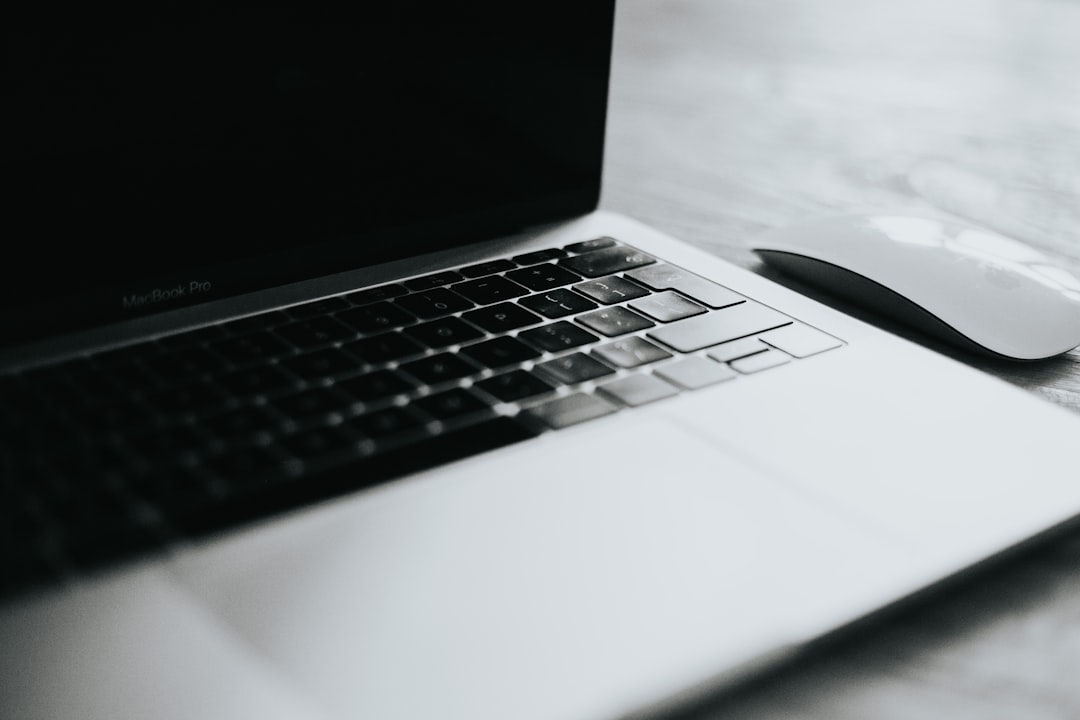
Paramiko's `SFTPClient` class provides a way to securely manage files on remote servers over SFTP. You establish an SSH connection first and then use it to create an `SFTPClient` instance, allowing you to upload, download, and list files. This is very helpful for automating file transfers, improving efficiency, and simplifying file management across multiple servers.
However, it's important to remember that Paramiko doesn't handle parallel file transfers, which can be a limitation when dealing with larger files. You can also make sure you're managing your resources well by using the `SFTPClient` as a context manager, which helps ensure connections are closed properly after each operation.
Paramiko's SFTPClient provides a way to manage files securely over SSH. This contrasts with FTP, which uses separate connections for data and control, leaving it more vulnerable to attacks. SFTP's single connection encrypts both data and control, offering a secure channel.
This secure file transfer protocol offers various features that make it appealing for managing remote files. Its atomic operations guarantee that uploads and downloads either complete fully or fail, preventing data corruption, critical for maintaining data integrity. You can also granularly control file permissions on the remote server through SFTP, setting access rights similar to Unix permissions to ensure security on shared file systems.
Another advantage of SFTP is its ability to resume interrupted transfers. This significantly improves efficiency when dealing with large files or unreliable networks, reducing the need to start from scratch.
SFTP provides more detailed directory listings than traditional FTP, including file size, owner, and modification timestamps. This allows for better file management and facilitates informed decisions about file operations.
While not universally implemented, some SFTP implementations support file compression, reducing transfer times for large files. And the ability to chain multiple file operations into a single transaction, called a single transaction, minimizes round-trip time and enables simultaneous atomic updates for multiple files, improving performance.
While SFTPClient in Paramiko can utilize SSH agent forwarding for key management, providing a convenient way to manage keys without re-entering passwords, there's a performance trade-off to consider. SFTP, with its encryption and integrity checks, can be slower than FTP, especially when dealing with smaller files or constrained networks.
SFTP's protocol differences are notable; it doesn't rely on a separate control channel, simplifying setup and minimizing potential security issues. While SFTP offers a secure way to manage remote files, it's crucial to consider its performance overhead and evaluate trade-offs based on specific requirements. Benchmarking and testing are crucial before committing to SFTP, especially in performance-sensitive environments.
Streamlining Remote File Access Implementing Paramiko for SSH Connections in Python - Managing file transfers and synchronization
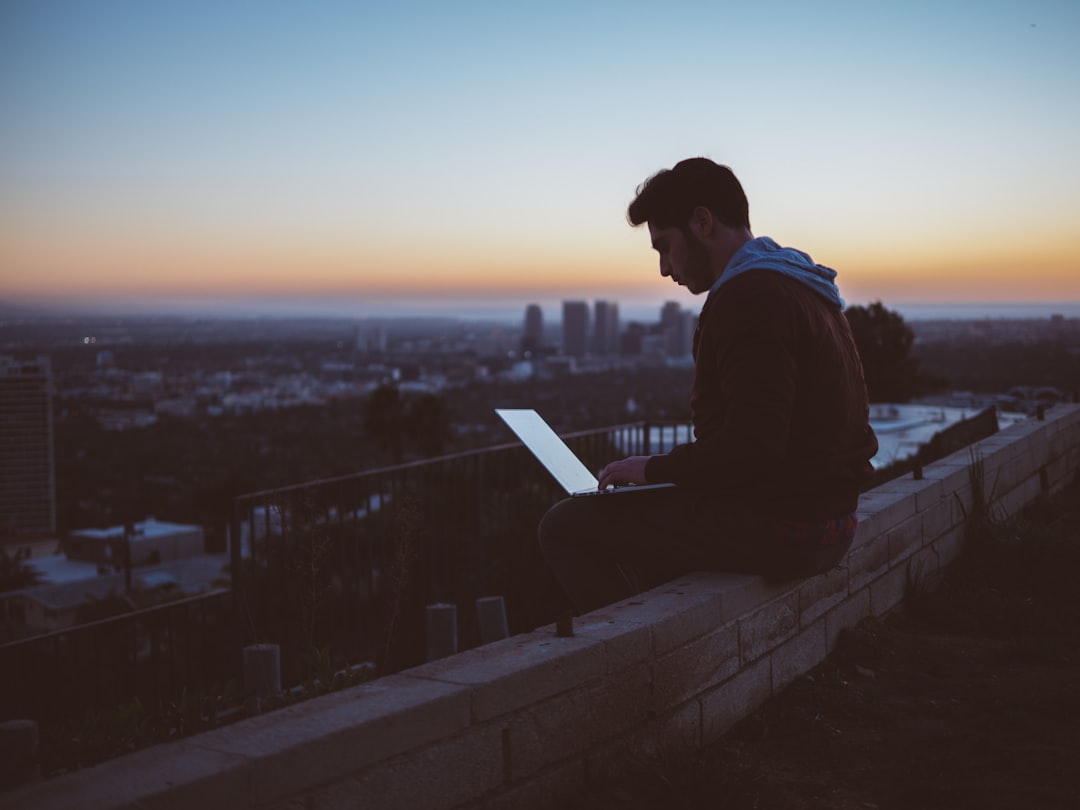
Managing file transfers and synchronization is a key part of working with remote systems. It's all about making sure the right files are in the right place at the right time. Paramiko's `SFTPClient` comes in handy here, making it easy to securely move files around using SFTP. You connect to your SSH server, open an SFTP session, and then you can move files, upload them, download them, and get lists of what's on the server. This is great for automating those routine file transfers and making managing multiple servers much simpler.
However, there's a catch. Paramiko doesn't really handle things like parallel transfers. This can be a problem when you're dealing with huge files. And, sometimes, the speed isn't great for large files. So, you have to be aware of these limitations, and how they might affect your project.
Paramiko's `SFTPClient` is a really powerful tool, and it can do a lot. But to get the most out of it, you need to be aware of how it works, and when its limits might become a problem.
Paramiko's `SFTPClient` class is a handy tool for managing files on remote servers securely. It leverages SFTP over SSH, providing a more robust and secure file transfer method compared to traditional FTP. However, the overhead of encryption can slow things down, especially when transferring large files.
Despite this, SFTP's atomic operations, which ensure that transfers either complete or fail entirely, are vital for preserving data integrity. This trade-off between speed and reliability should be considered when evaluating SFTP's suitability for different tasks.
The performance of SFTP is also dependent on the specific encryption algorithms employed. Using faster algorithms like AES-128 can improve transfer speeds but may compromise security compared to AES-256.
One significant limitation of Paramiko's SFTPClient is its lack of native support for parallel transfers. This can be a major bottleneck when dealing with numerous large files, potentially leading to longer transfer times.
The `SFTPClient` offers a powerful set of features beyond secure transfer, such as resuming interrupted transfers and providing detailed file listings. This is particularly helpful when working with unstable network connections or managing large files in professional settings.
SFTP also provides fine-grained control over file permissions, akin to Unix permissions, ensuring that security measures are properly enforced on shared file systems. This reduces the risk of unauthorized access to sensitive data.
Paramiko's `SFTPClient` also plays a crucial role in resource management. Using it as a context manager ensures that connections are properly closed after each operation, preventing potential resource leaks and crashes in long-running applications.
Overall, while SFTP might not be the fastest file transfer protocol, its emphasis on security and data integrity, coupled with its diverse range of features, make it a valuable tool for managing files across remote systems, especially in environments where security is paramount.
Streamlining Remote File Access Implementing Paramiko for SSH Connections in Python - Executing remote commands via SSHClient
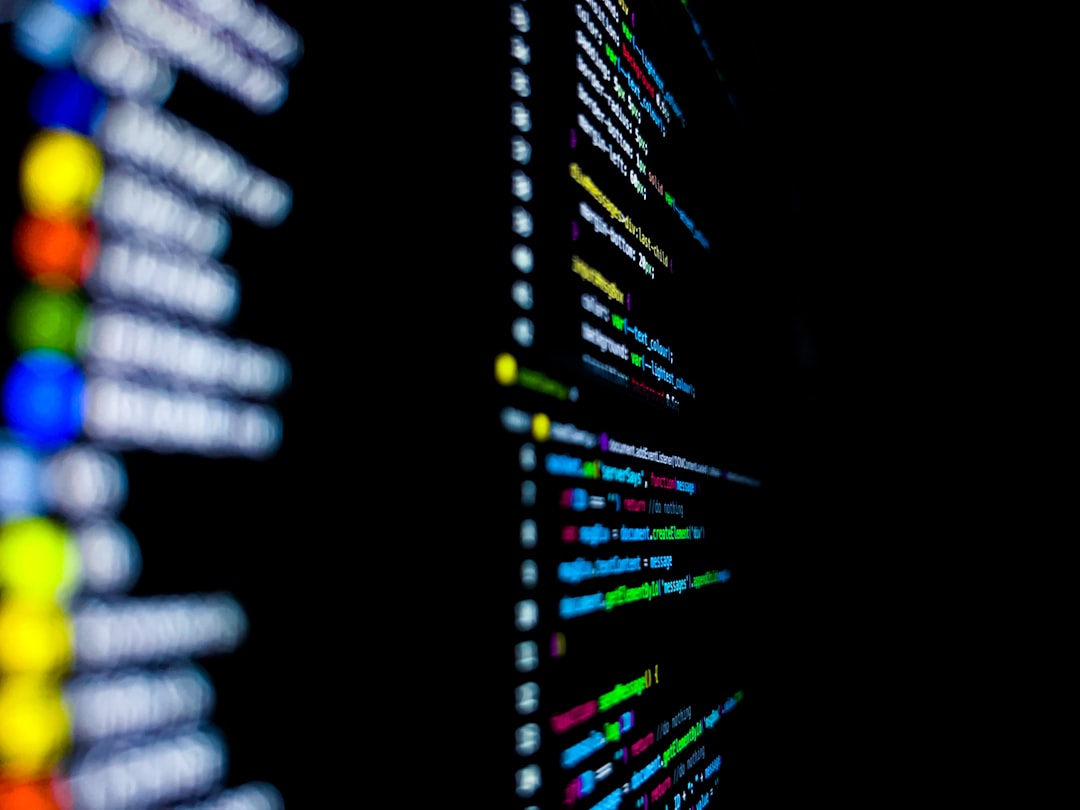
Executing remote commands through Paramiko's `SSHClient` is essential for automating tasks on distant servers. You connect to the server and then use `exec_command()` to run commands as if you were logged in directly. Paramiko is great for this, but you need to make sure security is top-notch by carefully verifying host keys and using strong authentication. This is important for keeping your data safe.
One advantage is that you can upload scripts to execute remotely. This makes managing things easier because you don't need to manually transfer files all the time. But be aware of possible performance issues if you're running lots of commands quickly.
Paramiko's `SSHClient` provides a powerful way to execute commands remotely. It offers a lot of features that make it a great tool for remote management.
First, you can get real-time feedback from commands, which is super helpful for debugging and development. The immediate output helps to streamline the entire process.
Paramiko also manages dedicated channels for each command, ensuring that they don't interfere with each other when running multiple commands at the same time. This approach significantly improves the reliability of simultaneous executions.
Another neat feature is the ability to set timeouts for commands. This prevents long-running commands from holding up your scripts, especially when dealing with unreliable network connections.
Once a command is run, you can check its return code, which lets you build conditional logic into your scripts based on whether the command succeeded or failed. This is crucial for building robust automation workflows.
You can also monitor the progress of commands in real-time as they run, which is fantastic for large jobs where you need ongoing feedback.
Paramiko handles errors effectively, raising specific exceptions for different types of failures. This allows you to create detailed error handling protocols, turning remote command execution from a black box into something you can manage.
It's worth noting that you can execute any command available on the server's shell, not just Python scripts. This makes Paramiko a very versatile tool for diverse engineering environments.
You can also capture both standard output and error streams separately, making debugging easier. Analyzing these outputs helps isolate and resolve issues more effectively.
Paramiko can go beyond password and key-based authentication by supporting SSH tunneling for even more secure communication.
And finally, you can combine Paramiko with other protocols like SCP and SFTP for file transfers during command execution, which provides a complete set of tools for managing your server operations.
Paramiko's SSH command execution capability makes managing remote systems a lot easier, offering flexibility, security, and control for engineers working with remote servers.
Create AI-powered tutorials effortlessly: Learn, teach, and share knowledge with our intuitive platform. (Get started for free)
More Posts from aitutorialmaker.com: