Create AI-powered tutorials effortlessly: Learn, teach, and share knowledge with our intuitive platform. (Get started for free)
Mastering Python's refindall() A Practical Guide to Extracting Multiple Pattern Matches
Mastering Python's refindall() A Practical Guide to Extracting Multiple Pattern Matches - Understanding the Basics of re.findall() Function
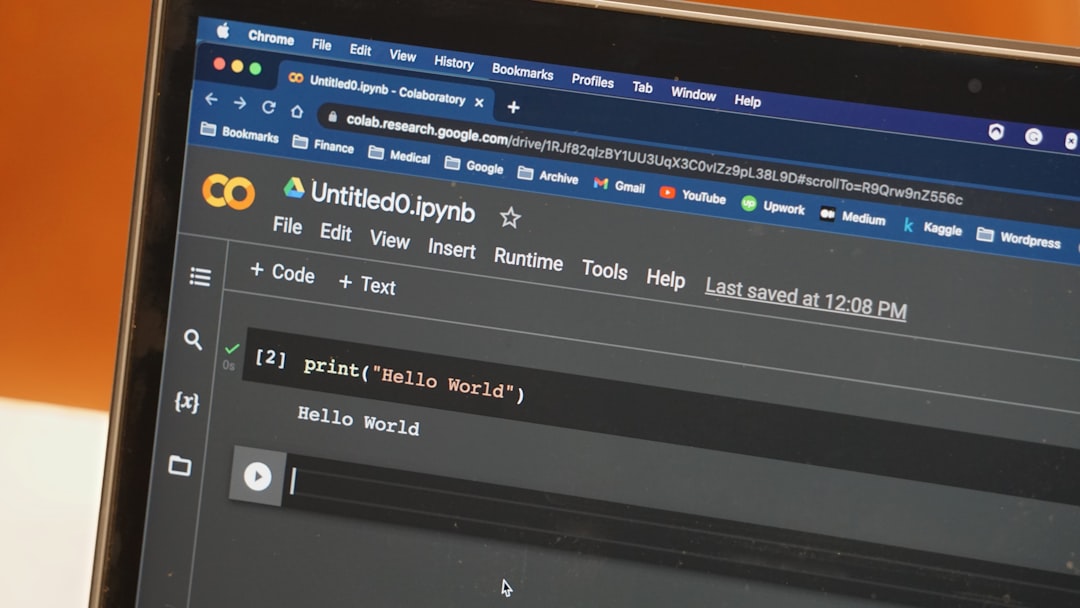
`re.findall()` is a powerful function in Python's `re` module for efficiently finding and extracting multiple instances of a specific pattern from a text. It's a versatile tool for data analysis and text processing, enabling you to readily pull out data like email addresses or phone numbers from large blocks of text. The function scans the text sequentially, looking for all non-overlapping matches to your specified pattern. While this makes it convenient for many tasks, it also means that overlapping matches are ignored. The function returns its findings as a list, providing a straightforward way to access the extracted data. If your pattern includes capturing groups, the results will be formatted as a list of tuples, each tuple representing a match with its captured groups. Understanding how to utilize `re.findall()` effectively can significantly enhance your ability to manipulate and analyze text data in Python.
The `re.findall()` function is a versatile tool in Python's regular expression library. It uses the powerful syntax of regular expressions, originally conceived by Stephen Cole Kleene, to find multiple instances of a pattern within a string. One noteworthy aspect of `re.findall()` is its focus on non-overlapping matches, meaning that you might miss matches if you're not mindful of potential overlaps.
When dealing with capturing groups within your regular expression, `re.findall()` returns a list of tuples. Each tuple represents a successful match and contains the matched groups, contrasting with approaches that only return the entire matched string. Additionally, for performance optimization, `re.findall()` accepts pre-compiled regex patterns. Compiling a pattern once and then reusing it can be beneficial for large-scale applications, saving valuable computation time.
Despite its capabilities, `re.findall()`'s simplicity can occasionally be deceiving. Complex regex patterns can lead to unforeseen outcomes, requiring careful pattern design to ensure intended results. Although primarily used with strings, `re.findall()` can also be applied to byte objects by combining encoding and decoding techniques, making it adaptable for different data processing contexts. Furthermore, in the event of no matches, `re.findall()` returns an empty list rather than `None`, a crucial distinction when considering error handling and program flow.
Understanding the nuances of greedy versus non-greedy matching within regex patterns is critical. Adding a simple `?` modifier to a pattern can significantly impact results. Performance analysis shows that `re.findall()` is efficient with large texts, but extremely intricate regex patterns can lead to an exponential increase in search time, highlighting the need to optimize regex for optimal performance. Debugging complex regular expressions can be a daunting task due to their compactness and versatility. Utilizing online regex tools or specialized debug environments can greatly simplify pattern visualization and testing before deployment.
Mastering Python's refindall() A Practical Guide to Extracting Multiple Pattern Matches - Syntax and Parameters Explained
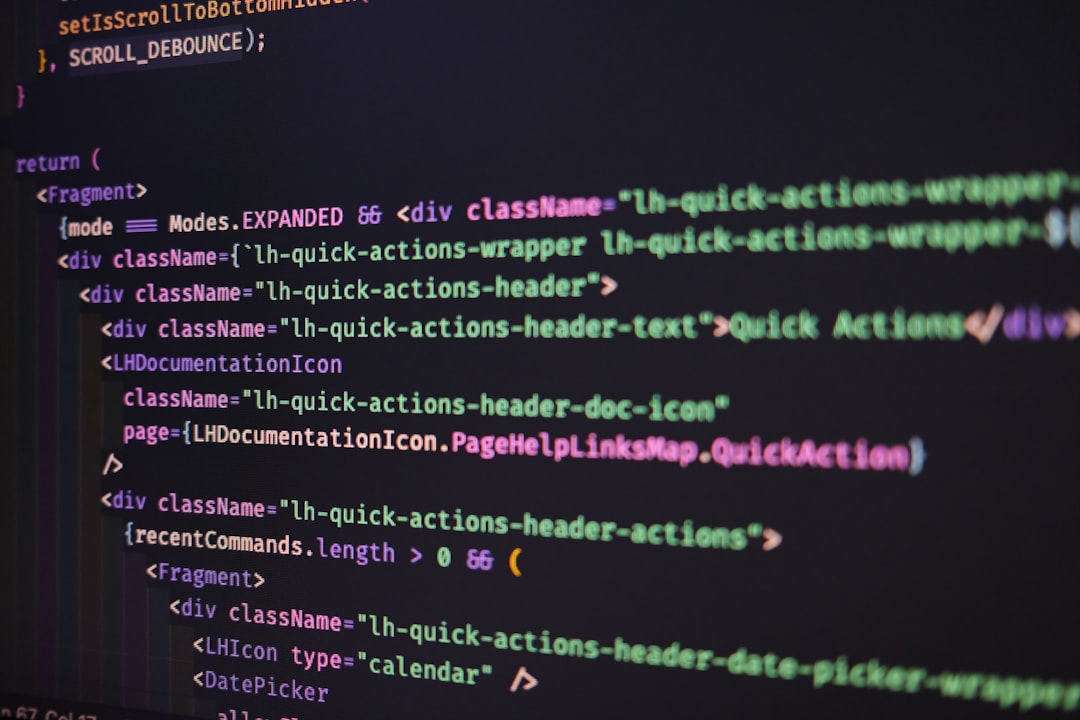
Understanding how to use Python's `re.findall()` function is key to efficiently extracting multiple matching patterns from a text. This function's syntax is fairly straightforward: `re.findall(pattern, string, flags=0)`. The `pattern` is the regular expression you're searching for, the `string` is the text you're scanning, and the optional `flags` modify the search behavior. It's worth noting that `re.findall()` focuses on non-overlapping matches, which means you might miss matches if your pattern could overlap. Furthermore, if your pattern includes capturing groups, the function returns a list of tuples where each tuple represents a match, and its contained groups. By understanding these elements, you can significantly enhance your ability to analyze and manipulate text data in Python.
The `re.findall()` function in Python's `re` module provides a robust mechanism to find multiple instances of a pattern within a string. While it's a powerful tool for data analysis and text processing, it's important to acknowledge its limitations and nuances. The complexity of regular expressions can lead to unexpected results, highlighting the importance of careful pattern design. Engineers need to consider performance implications, balancing regex complexity with efficiency, especially when dealing with large datasets.
One crucial aspect is the distinction between greedy and non-greedy matching. A simple `?` modifier can drastically alter matching behavior. It's important to understand the impact of greediness on the outcomes. Although primarily used for strings, `re.findall()` can also work with byte objects by leveraging encoding and decoding techniques, expanding its utility. Pre-compiled patterns can improve performance for applications involving repetitive pattern searches.
When capturing groups are present, `re.findall()` returns a list of tuples, containing captured group data. This differentiates it from functions that only return the full matched string. The fact that `re.findall()` returns an empty list rather than `None` for no matches simplifies error handling and streamlines program flow. Extracted data often requires additional cleanup or processing, including removal of unwanted characters. Debugging complex regular expressions can be challenging due to their compactness and versatility. Online tools for regex testing are invaluable for visualizing matches and streamlining debugging.
`re.findall()` is often integrated with other libraries, such as Pandas, for data analysis. This synergistic approach enables the manipulation and analysis of textual data alongside structured datasets, enhancing data science capabilities.
Mastering Python's refindall() A Practical Guide to Extracting Multiple Pattern Matches - Handling Single and Multiple Groups in Patterns
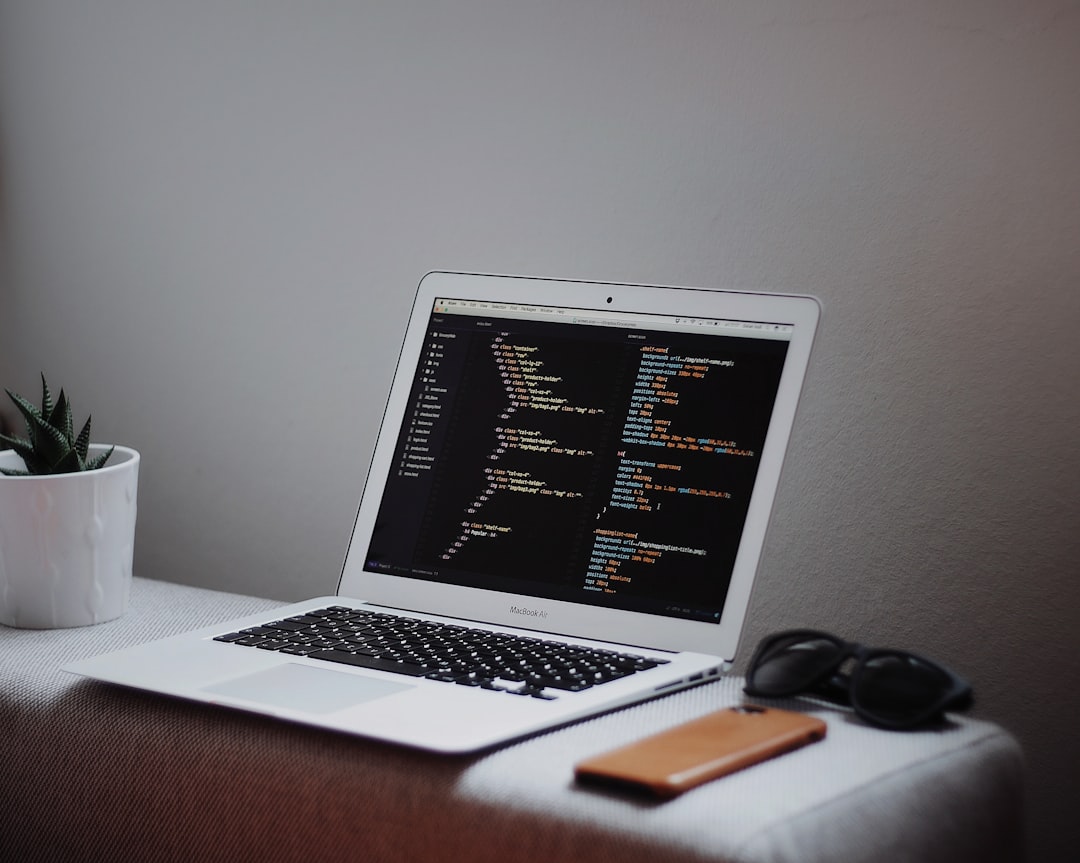
Understanding how to manage single and multiple groups in patterns is critical when using Python's `re.findall()` function. This function goes beyond just finding multiple matches in a string. It provides a powerful way to handle capturing groups, which is extremely useful for extracting structured data. When your regular expression includes one or more groups, `re.findall()` returns a list of tuples. Each tuple represents a single match and contains the matched groups for that occurrence. This distinction between full matches and their components allows for more refined data manipulation. You can pull out exactly what you need while maintaining the context of the match. This feature makes `re.findall()` more versatile and more powerful for working with text data.
While `re.findall()` is a powerful tool for extracting data from text, it's important to understand its quirks. The function's performance, for example, is highly dependent on the complexity of the regex pattern used. Simple patterns usually run efficiently, but intricate ones can slow things down drastically.
Additionally, `re.findall()` only returns non-overlapping matches, which could lead to missed data if patterns could potentially overlap. Carefully designing patterns is essential to ensure all potential matches are captured.
For scenarios where your regex has capturing groups, `re.findall()` returns a list of tuples, each representing a match and its captured groups. This structure makes organizing and extracting specific components from complex strings much easier.
The behavior of `re.findall()` can be customized using flags like `re.IGNORECASE` for case-insensitive searches. Choosing the right flags is critical for ensuring the function behaves as expected.
It's also worth noting that `re.findall()` returns an empty list rather than `None` when no matches are found, which simplifies error handling. This eliminates the need for extra code to check for `None` values, making error-checking more straightforward.
Pre-compiling your regex patterns with `re.compile()` can significantly improve performance if you are using the same pattern repeatedly. This eliminates the overhead of compiling the pattern each time, especially useful when working with large datasets.
While `re.findall()` is primarily used with strings, you can adapt it to work with byte objects by employing encoding and decoding techniques. This flexibility expands its applicability across various data processing scenarios.
The difference between greedy and non-greedy matching can significantly affect your results. Using `?` in your regex patterns can help you gain fine-grained control over the length of matches and potentially improve data extraction accuracy.
Debugging regex patterns can be a nightmare. Utilizing visual tools that illustrate match behavior can greatly simplify this process, enhancing accuracy and easing the debugging experience.
Finally, `re.findall()` often works seamlessly with other libraries, like Pandas, to unlock the power of text data manipulation within structured datasets. This integration provides a smooth pathway to seamlessly combine unstructured and structured data for robust data analysis workflows.
Mastering Python's refindall() A Practical Guide to Extracting Multiple Pattern Matches - Practical Examples Using re.findall()
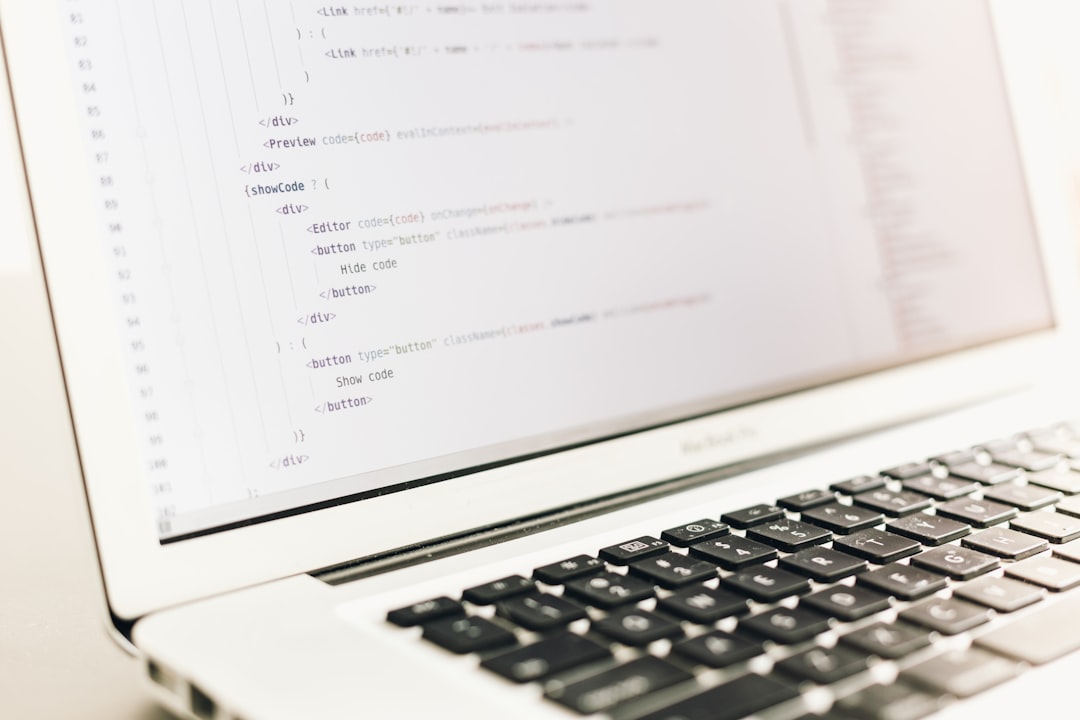
The "Practical Examples Using re.findall()" section aims to demonstrate how the `re.findall()` function can be utilized in real-world scenarios, strengthening your understanding of Python's pattern matching capabilities. For example, it'll showcase how to extract email addresses or specific data points from structured text, highlighting the function's ability to return ordered lists of matches. You'll also see how to handle complex patterns, including capturing groups, illustrating the versatility of `re.findall()` in data extraction tasks.
This section emphasizes the crucial importance of careful regex design to avoid common pitfalls like missing overlapping matches. Overall, this section builds upon previous discussions by providing concrete applications that solidify your knowledge of `re.findall()` in practical coding situations.
`re.findall()` is a powerful Python tool for finding multiple pattern matches within a string. While its simplicity is tempting, there are nuances and potential pitfalls to be aware of. The speed at which `re.findall()` operates can vary dramatically based on the complexity of the regular expression you're using. Simple patterns lead to efficient execution, but intricate patterns can exponentially increase the search time, emphasizing the importance of careful pattern design.
Additionally, `re.findall()` only returns non-overlapping matches, meaning that if two patterns could potentially match the same text, only the first match will be returned. This can lead to missed data if overlaps are a possibility. Understanding the difference between greedy and non-greedy matching is crucial. The addition of a simple `?` can change the length of matched strings, significantly impacting results. Furthermore, debugging complex regular expressions can be a frustrating experience. Using visual regex testers is highly recommended, as they can help visualize how the patterns match against text before implementation, making the debugging process much smoother.
When your pattern includes capturing groups, `re.findall()` returns a list of tuples, with each tuple representing a match and its captured groups. This is a significant advantage compared to functions that only return the entire matched string, allowing for more precise data extraction without the need for additional processing. One of the more convenient features of `re.findall()` is its handling of unsuccessful searches. When no matches are found, it returns an empty list instead of `None`, simplifying error handling in code and eliminating the need to check for `None` values. While `re.findall()` is primarily used with strings, it can be extended to work with byte objects by using encoding and decoding techniques.
Another helpful feature is the ability to pre-compile your regular expression pattern using `re.compile()`, a technique that greatly improves performance for applications that use the same pattern repeatedly. Additionally, `re.findall()` can be customized with flags such as `re.MULTILINE` or `re.IGNORECASE`, allowing you to tailor searches to specific requirements and ensure the extraction of the correct data.
`re.findall()` is also compatible with various other Python libraries like Pandas, enhancing its versatility in data analysis. This integration allows you to seamlessly combine data from both structured and unstructured sources, further amplifying the capabilities of this useful Python tool.
Mastering Python's refindall() A Practical Guide to Extracting Multiple Pattern Matches - Common Pitfalls and How to Avoid Them
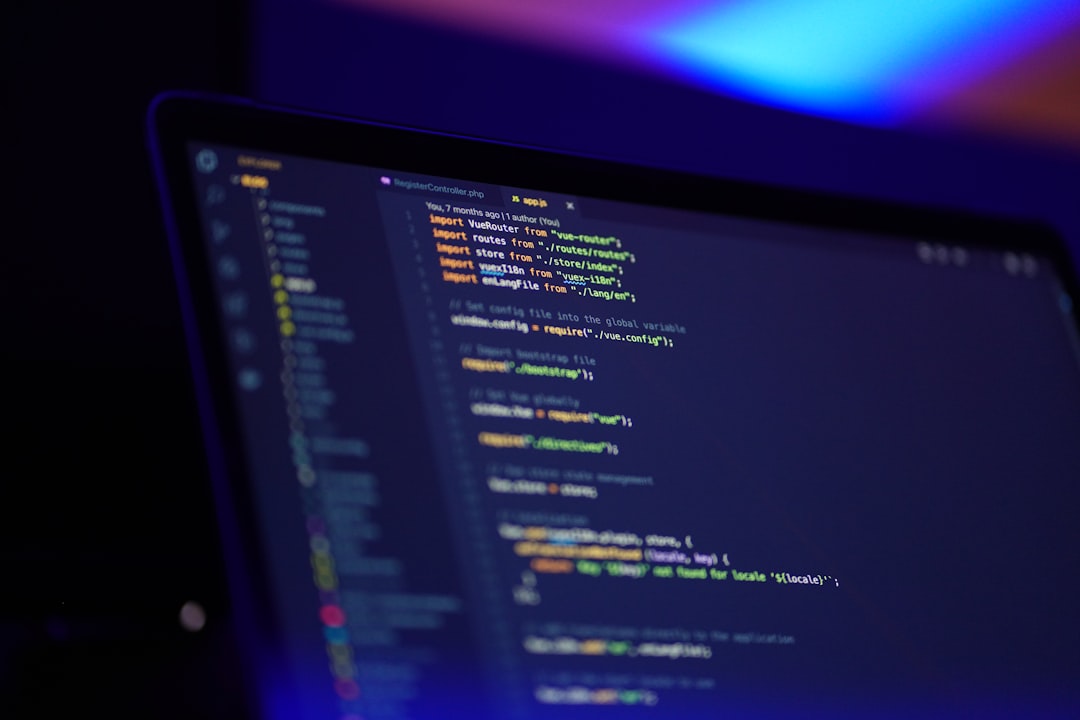
Using `re.findall()` effectively requires awareness of common pitfalls to avoid errors and ensure accurate data extraction. One pitfall is misunderstanding the behavior of non-capturing groups, which can lead to unexpected matches or missed data. Special character escapes can also be problematic, so using raw strings, especially when backslashes are involved, is recommended. Additionally, ensure your regular expressions handle potential overlaps correctly, as `re.findall()` prioritizes non-overlapping matches. Always test your patterns iteratively to gain clarity and confirm that the expected matches are being identified before applying them to large datasets. This iterative approach ensures better performance and reliable data extraction results.
The `re.findall()` function in Python, part of the `re` module, is a powerful tool for extracting multiple matching patterns from a string. While simple in appearance, it has some nuances that can lead to unexpected results if not understood properly. One crucial aspect is the handling of overlapping matches. `re.findall()` only returns non-overlapping matches, which means that if two patterns could potentially match the same text, only the first one will be returned. This can cause data loss if the regex pattern isn't designed with overlaps in mind.
Another important consideration is performance. `re.findall()` is fast with simple regex patterns, but when the patterns become complex or intricate, the search time can increase exponentially, making code less efficient. This highlights the importance of optimization for intricate patterns.
Understanding the return structure is key as well. `re.findall()` returns different types of results based on whether the pattern includes capturing groups. If there are capturing groups, it returns a list of tuples, with each tuple containing the matched groups. This provides more detailed data than a simple list of matched strings, which is what you get without capturing groups.
The behavior of `re.findall()` can be modified using flags like `re.IGNORECASE` or `re.MULTILINE`. However, many users are unaware of how much a simple flag can alter the scope of the search. Careful consideration of flags is essential for ensuring the function operates as intended.
A helpful technique for improving performance in applications that repeatedly use the same regex pattern is pre-compilation with `re.compile()`. This pre-compilation avoids the overhead of compiling the pattern each time, making the code more efficient.
The function also simplifies error handling by returning an empty list instead of `None` when no matches are found. This eliminates the need to check for `None` values, streamlining code logic.
Despite its power, debugging complex regular expressions can be a challenging task. This is where online regex visualizers and testers come in handy, offering a clear way to see how the patterns match text. These tools can make debugging significantly easier, reducing frustration.
Another point to consider is the difference between greedy and non-greedy matching. Adding a `?` to a pattern can change not only the number of matches but also the precision of the extracted data. This subtlety is often overlooked but can have a significant impact on the output.
While `re.findall()` is primarily intended for strings, it can also be used with byte objects through encoding and decoding. This extends its utility to a wider range of data types.
Finally, integrating `re.findall()` with data-focused libraries like Pandas creates powerful possibilities for text data manipulation. This allows for more complex data analysis and processing workflows, further enhancing the overall capabilities of Python for data science tasks.
Mastering Python's refindall() A Practical Guide to Extracting Multiple Pattern Matches - Advanced Techniques for Complex Pattern Matching
Advanced techniques for complex pattern matching in Python delve deeper into the capabilities of regular expressions, allowing you to craft intricate and multifaceted search patterns. Combining multiple patterns, utilizing features like wildcards and character classes, and understanding the nuances of greedy and non-greedy matches enables more comprehensive and efficient data extraction. For example, you might need to search for email addresses in a text, but you want to make sure you only capture addresses that are not enclosed in parentheses or brackets. This could be achieved by combining multiple patterns that define the structure of an email address and then specifying those parts that are invalid. The addition of a simple `?` to a pattern can alter the behavior of greedy matches, which grab the largest possible match, turning them into non-greedy matches that grab the smallest match. It's also important to consider performance. Pre-compiling your regular expressions using `re.compile()` can significantly improve performance, especially when you need to run the same pattern multiple times. This allows you to optimize regex operations for improved efficiency and speed. These advanced techniques enhance text manipulation skills, enabling more precise and powerful search functionalities across various applications.
Python's `re.findall()` offers a powerful tool for extracting multiple instances of a pattern within a string. While its simplicity makes it tempting to dive in, its capabilities go far beyond basic string manipulation. Let's explore 10 aspects that illustrate the surprising power and complexity of using `re.findall()` in advanced pattern matching scenarios.
First, quantifiers like `*`, `+`, and `{n,m}` control how many times a preceding pattern can repeat. Be careful - overly greedy quantifiers can gobble up more text than intended, leading to inaccurate results and unnecessary processing.
Next, capturing groups nested within regex patterns allow for structured data extraction. For instance, `((\d{3})-(\d{2})-(\d{4}))` captures the area code, central office code, and line number of a phone number, providing well-organized data. This goes beyond simple matching and provides a foundation for efficient data analysis.
Backreferences, like `\1` and `\2`, introduce a powerful element of pattern validation. These references can ensure that repeated patterns within a string adhere to your expectations. This can be crucial for data validation tasks, especially in scenarios where a relationship between different string components needs to be enforced.
Importantly, Python's `re` module supports Unicode. This opens up the ability to process text from a wide range of languages and character sets, making it relevant for international applications. However, this also introduces complexity, as the design of your regex patterns must account for encoding variations.
While simple regex patterns are processed swiftly, complex ones can lead to "catastrophic backtracking," a performance nightmare. In essence, the regex engine gets stuck trying out multiple permutations of the input, dramatically increasing processing time. This underscores the importance of optimizing regex for intricate patterns.
Adding flags like `re.MULTILINE` or `re.DOTALL` can alter how `re.findall()` interprets your data. For instance, `re.DOTALL` allows the `.` metacharacter to match newline characters, a crucial feature when working with multiline strings. Understanding how flags impact your search is vital.
Regular expressions can be programmatically constructed using string operations, adding an element of dynamicism to your pattern matching. This means you can build regex patterns based on user input or conditional logic, adding flexibility to your search tasks.
For situations where overlaps are essential, alternative strategies are needed. Techniques such as modifying the pattern to include lookaheads or using a loop to shift search boundaries can capture overlapping matches, although this can complicate your pattern.
Lookaheads and lookbehinds offer a level of contextual awareness to your patterns. These assertions allow you to match patterns based on what precedes or follows them without actually including that context in the result. This provides a more refined extraction capability.
Finally, remember that `re.findall()` returns an empty list instead of `None` when no matches are found, aiding developers in error handling. This simple design choice contributes to cleaner, more intuitive code.
The power of pattern matching with Python's `re.findall()` extends far beyond basic text manipulation. By understanding these advanced aspects and using them judiciously, developers can leverage regex for powerful and accurate data extraction, ultimately enhancing their capabilities in various programming scenarios.
Create AI-powered tutorials effortlessly: Learn, teach, and share knowledge with our intuitive platform. (Get started for free)
More Posts from aitutorialmaker.com: